Why the dtype changed differently after convert two lists with same type to numpy array?
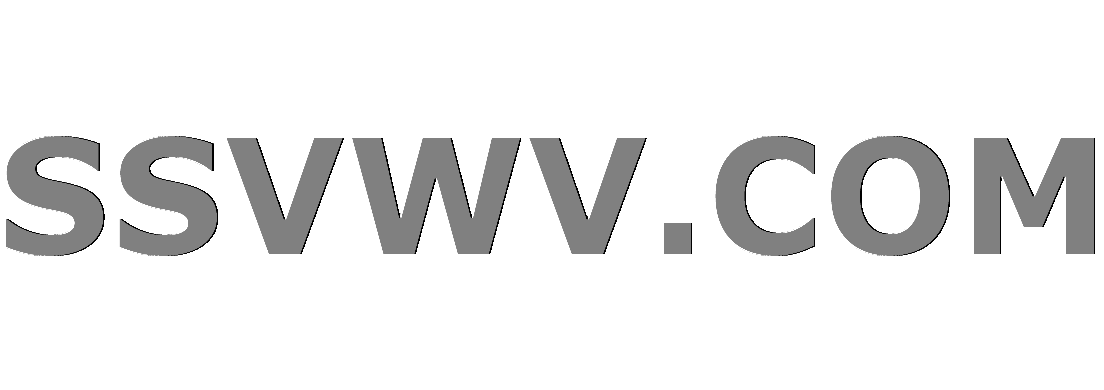
Multi tool use
up vote
0
down vote
favorite
When I convert two lists of numpy arrays to numpy arrays of numpy arrays, something confused happened.
The first list X_s changed to a numpy array with shape of (1980, 384, 448, 1), which is good for training, but the second list X_l chaned to a numpy arrays with shape of (2013,).
I check their dtype, and the first become float64 while the second become object of numpy array.
Why this happened?
print(len(X_s)) # 1980
print(len(X_l)) # 2013
print(X_s[0].dtype) # float64
print(X_l[0].dtype) # float64
print(X_s[0].shape) # (384, 448, 1)
print(X_l[0].shape) # (384, 448, 1)
for i in range(len(X_l)):
X_l[i] = np.array(X_l[i], dtype = np.float64)
for i in range(len(X_s)):
X_s[i] = np.array(X_s[i], dtype = np.float64)
X_s = np.array(X_s)
X_l = np.array(X_l)
print(type(X_s[0])) # <class 'numpy.ndarray'>
print(type(X_l[0])) # <class 'numpy.ndarray'>
print(X_s.dtype) # flaot64
print(X_l.dtype) # object
print(X_s.shape) # (1980, 384, 448, 1)
print(X_l.shape) # (2013,)
After added two for loops to make sure the elements are in uniform type, nothing changed.
python arrays list numpy types
add a comment |
up vote
0
down vote
favorite
When I convert two lists of numpy arrays to numpy arrays of numpy arrays, something confused happened.
The first list X_s changed to a numpy array with shape of (1980, 384, 448, 1), which is good for training, but the second list X_l chaned to a numpy arrays with shape of (2013,).
I check their dtype, and the first become float64 while the second become object of numpy array.
Why this happened?
print(len(X_s)) # 1980
print(len(X_l)) # 2013
print(X_s[0].dtype) # float64
print(X_l[0].dtype) # float64
print(X_s[0].shape) # (384, 448, 1)
print(X_l[0].shape) # (384, 448, 1)
for i in range(len(X_l)):
X_l[i] = np.array(X_l[i], dtype = np.float64)
for i in range(len(X_s)):
X_s[i] = np.array(X_s[i], dtype = np.float64)
X_s = np.array(X_s)
X_l = np.array(X_l)
print(type(X_s[0])) # <class 'numpy.ndarray'>
print(type(X_l[0])) # <class 'numpy.ndarray'>
print(X_s.dtype) # flaot64
print(X_l.dtype) # object
print(X_s.shape) # (1980, 384, 448, 1)
print(X_l.shape) # (2013,)
After added two for loops to make sure the elements are in uniform type, nothing changed.
python arrays list numpy types
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
When I convert two lists of numpy arrays to numpy arrays of numpy arrays, something confused happened.
The first list X_s changed to a numpy array with shape of (1980, 384, 448, 1), which is good for training, but the second list X_l chaned to a numpy arrays with shape of (2013,).
I check their dtype, and the first become float64 while the second become object of numpy array.
Why this happened?
print(len(X_s)) # 1980
print(len(X_l)) # 2013
print(X_s[0].dtype) # float64
print(X_l[0].dtype) # float64
print(X_s[0].shape) # (384, 448, 1)
print(X_l[0].shape) # (384, 448, 1)
for i in range(len(X_l)):
X_l[i] = np.array(X_l[i], dtype = np.float64)
for i in range(len(X_s)):
X_s[i] = np.array(X_s[i], dtype = np.float64)
X_s = np.array(X_s)
X_l = np.array(X_l)
print(type(X_s[0])) # <class 'numpy.ndarray'>
print(type(X_l[0])) # <class 'numpy.ndarray'>
print(X_s.dtype) # flaot64
print(X_l.dtype) # object
print(X_s.shape) # (1980, 384, 448, 1)
print(X_l.shape) # (2013,)
After added two for loops to make sure the elements are in uniform type, nothing changed.
python arrays list numpy types
When I convert two lists of numpy arrays to numpy arrays of numpy arrays, something confused happened.
The first list X_s changed to a numpy array with shape of (1980, 384, 448, 1), which is good for training, but the second list X_l chaned to a numpy arrays with shape of (2013,).
I check their dtype, and the first become float64 while the second become object of numpy array.
Why this happened?
print(len(X_s)) # 1980
print(len(X_l)) # 2013
print(X_s[0].dtype) # float64
print(X_l[0].dtype) # float64
print(X_s[0].shape) # (384, 448, 1)
print(X_l[0].shape) # (384, 448, 1)
for i in range(len(X_l)):
X_l[i] = np.array(X_l[i], dtype = np.float64)
for i in range(len(X_s)):
X_s[i] = np.array(X_s[i], dtype = np.float64)
X_s = np.array(X_s)
X_l = np.array(X_l)
print(type(X_s[0])) # <class 'numpy.ndarray'>
print(type(X_l[0])) # <class 'numpy.ndarray'>
print(X_s.dtype) # flaot64
print(X_l.dtype) # object
print(X_s.shape) # (1980, 384, 448, 1)
print(X_l.shape) # (2013,)
After added two for loops to make sure the elements are in uniform type, nothing changed.
python arrays list numpy types
python arrays list numpy types
edited 18 hours ago
asked 18 hours ago
Salmon
226
226
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
It looks very likely that the elements of the original X_l
list are not of uniform type. (You only show us the type of the first element but not the rest.)
When NumPy tries to convert that list to an array, it notices that and coerces everything to object
.
Demo:
In [10]: X_s = [np.array([1]), np.array([2])]
In [11]: X_l = [np.array([1]), 2]
In [12]: np.array(X_s)
Out[12]:
array([[1],
[2]])
In [13]: np.array(X_l)
Out[13]: array([array([1]), 2], dtype=object)
(This example is made up but consistent with your observations.)
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
It looks very likely that the elements of the original X_l
list are not of uniform type. (You only show us the type of the first element but not the rest.)
When NumPy tries to convert that list to an array, it notices that and coerces everything to object
.
Demo:
In [10]: X_s = [np.array([1]), np.array([2])]
In [11]: X_l = [np.array([1]), 2]
In [12]: np.array(X_s)
Out[12]:
array([[1],
[2]])
In [13]: np.array(X_l)
Out[13]: array([array([1]), 2], dtype=object)
(This example is made up but consistent with your observations.)
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
add a comment |
up vote
1
down vote
It looks very likely that the elements of the original X_l
list are not of uniform type. (You only show us the type of the first element but not the rest.)
When NumPy tries to convert that list to an array, it notices that and coerces everything to object
.
Demo:
In [10]: X_s = [np.array([1]), np.array([2])]
In [11]: X_l = [np.array([1]), 2]
In [12]: np.array(X_s)
Out[12]:
array([[1],
[2]])
In [13]: np.array(X_l)
Out[13]: array([array([1]), 2], dtype=object)
(This example is made up but consistent with your observations.)
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
add a comment |
up vote
1
down vote
up vote
1
down vote
It looks very likely that the elements of the original X_l
list are not of uniform type. (You only show us the type of the first element but not the rest.)
When NumPy tries to convert that list to an array, it notices that and coerces everything to object
.
Demo:
In [10]: X_s = [np.array([1]), np.array([2])]
In [11]: X_l = [np.array([1]), 2]
In [12]: np.array(X_s)
Out[12]:
array([[1],
[2]])
In [13]: np.array(X_l)
Out[13]: array([array([1]), 2], dtype=object)
(This example is made up but consistent with your observations.)
It looks very likely that the elements of the original X_l
list are not of uniform type. (You only show us the type of the first element but not the rest.)
When NumPy tries to convert that list to an array, it notices that and coerces everything to object
.
Demo:
In [10]: X_s = [np.array([1]), np.array([2])]
In [11]: X_l = [np.array([1]), 2]
In [12]: np.array(X_s)
Out[12]:
array([[1],
[2]])
In [13]: np.array(X_l)
Out[13]: array([array([1]), 2], dtype=object)
(This example is made up but consistent with your observations.)
answered 18 hours ago
NPE
342k57729864
342k57729864
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
add a comment |
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
I used a loop before the convert to make sure that the elements of the origin X_l list are in uniform type just now. But It didn't work.
– Salmon
18 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
After check the shape of every element in X_l, I found some element's shape is not (384, 448, 1).Thank you for your guidance.
– Salmon
17 hours ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237676%2fwhy-the-dtype-changed-differently-after-convert-two-lists-with-same-type-to-nump%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
e35kdesMob,QfDPqvji