MySQL Before Delete trigger to avoid deleting multiple rows
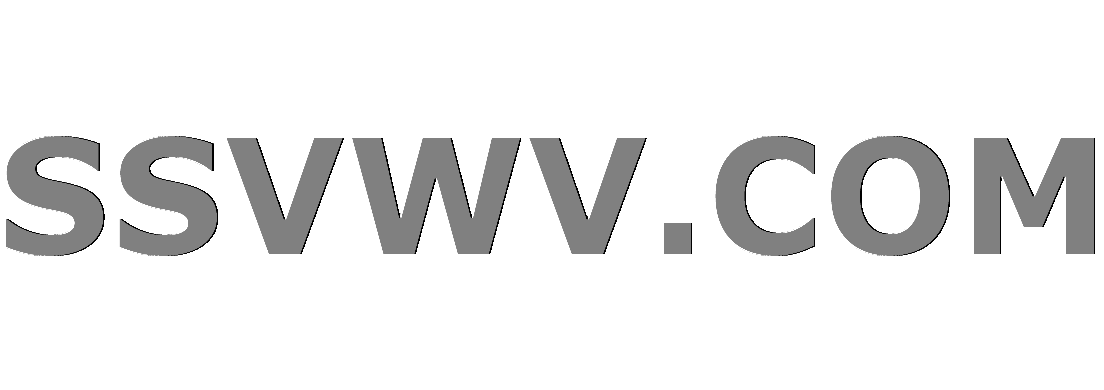
Multi tool use
up vote
1
down vote
favorite
I am trying to avoid deletion of more than 1 row at a time in MySQL by using a BEFORE DELETE trigger.
The sample table and trigger are as below.
Table test:
DROP TABLE IF EXISTS `test`;
CREATE TABLE `test` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`a` int(11) NOT NULL,
`b` int(11) NOT NULL,
PRIMARY KEY (`id`));
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (1, 1, 2);
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (2, 3, 4);
Trigger:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion;
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON test
FOR EACH STATEMENT
BEGIN
IF(ROW_COUNT()>=2) THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
This is still allowing multiple rows deletion. Even if I change the IF to >= 1, still allows the operation.
I my idea is to avoid operations such as:
DELETE FROM `test` WHERE `id`< 5;
Can you help me? I know that the current version of MySQL doesn't allow FOR EACH STATEMENT triggers.
Thank you!
mysql triggers sql-delete delete-row
add a comment |
up vote
1
down vote
favorite
I am trying to avoid deletion of more than 1 row at a time in MySQL by using a BEFORE DELETE trigger.
The sample table and trigger are as below.
Table test:
DROP TABLE IF EXISTS `test`;
CREATE TABLE `test` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`a` int(11) NOT NULL,
`b` int(11) NOT NULL,
PRIMARY KEY (`id`));
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (1, 1, 2);
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (2, 3, 4);
Trigger:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion;
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON test
FOR EACH STATEMENT
BEGIN
IF(ROW_COUNT()>=2) THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
This is still allowing multiple rows deletion. Even if I change the IF to >= 1, still allows the operation.
I my idea is to avoid operations such as:
DELETE FROM `test` WHERE `id`< 5;
Can you help me? I know that the current version of MySQL doesn't allow FOR EACH STATEMENT triggers.
Thank you!
mysql triggers sql-delete delete-row
I think thatROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.
– Madhur Bhaiya
Nov 2 at 14:40
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to avoid deletion of more than 1 row at a time in MySQL by using a BEFORE DELETE trigger.
The sample table and trigger are as below.
Table test:
DROP TABLE IF EXISTS `test`;
CREATE TABLE `test` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`a` int(11) NOT NULL,
`b` int(11) NOT NULL,
PRIMARY KEY (`id`));
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (1, 1, 2);
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (2, 3, 4);
Trigger:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion;
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON test
FOR EACH STATEMENT
BEGIN
IF(ROW_COUNT()>=2) THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
This is still allowing multiple rows deletion. Even if I change the IF to >= 1, still allows the operation.
I my idea is to avoid operations such as:
DELETE FROM `test` WHERE `id`< 5;
Can you help me? I know that the current version of MySQL doesn't allow FOR EACH STATEMENT triggers.
Thank you!
mysql triggers sql-delete delete-row
I am trying to avoid deletion of more than 1 row at a time in MySQL by using a BEFORE DELETE trigger.
The sample table and trigger are as below.
Table test:
DROP TABLE IF EXISTS `test`;
CREATE TABLE `test` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`a` int(11) NOT NULL,
`b` int(11) NOT NULL,
PRIMARY KEY (`id`));
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (1, 1, 2);
INSERT INTO `test` (`id`, `a`, `b`)
VALUES (2, 3, 4);
Trigger:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion;
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON test
FOR EACH STATEMENT
BEGIN
IF(ROW_COUNT()>=2) THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
This is still allowing multiple rows deletion. Even if I change the IF to >= 1, still allows the operation.
I my idea is to avoid operations such as:
DELETE FROM `test` WHERE `id`< 5;
Can you help me? I know that the current version of MySQL doesn't allow FOR EACH STATEMENT triggers.
Thank you!
mysql triggers sql-delete delete-row
mysql triggers sql-delete delete-row
asked Nov 2 at 14:28
Gustavo Lessa
153
153
I think thatROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.
– Madhur Bhaiya
Nov 2 at 14:40
add a comment |
I think thatROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.
– Madhur Bhaiya
Nov 2 at 14:40
I think that
ROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.– Madhur Bhaiya
Nov 2 at 14:40
I think that
ROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.– Madhur Bhaiya
Nov 2 at 14:40
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Firstly, getting some syntax error(s) out of our way, from your original attempt:
- Instead of
FOR EACH STATEMENT
, it should beFOR EACH ROW
. - Since you have already defined the Delimiter to
//
; you need to use//
(instead of;
) in theDROP TRIGGER IF EXISTS ..
statement.
Row_Count()
will have 0 value in aBefore Delete Trigger
, as no rows have been updated yet. So this approach will not work.
Now, the trick here is to use Session-level Accessible (and Persistent) user-defined variables. We can define a variable, let's say @rows_being_deleted
, and later check whether it is already defined or not.
For Each Row
runs the same set of statements for every row being deleted. So, we will just check whether the session variable already exists or not. If it does not, we can define it. So basically, for the first row (being deleted), it will get defined, which will persist as long as the session is there.
Now if there are more rows to be deleted, Trigger would be running the same set of statements for the remaining rows. In the second row, the previously defined variable would be found now, and we can simply throw an exception now.
Note that there is a chance that within the same session, multiple delete statements may get triggered. So before throwing exception, we need to set the @rows_being_deleted
value back to null
.
Following will work:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion //
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON `test`
FOR EACH ROW
BEGIN
-- check if the variable is already defined or not
IF( @rows_being_deleted IS NULL ) THEN
SET @rows_being_deleted = 1; -- set its value
ELSE -- it already exists and we are in next "row"
-- just for testing to check the row count
-- SET @rows_being_deleted = @rows_being_deleted + 1;
-- We have to reset it to null, as within same session
-- another delete statement may be triggered.
SET @rows_being_deleted = NULL;
-- throw exception
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
DB Fiddle Demo 1: Trying to delete more than row.
DELETE FROM `test` WHERE `id`< 5;
Result:
Query Error: Error: ER_SIGNAL_EXCEPTION: Cannot delete more than one
order per time!
DB Fiddle Demo 2: Trying to delete only one row
Query #1
DELETE FROM `test` WHERE `id` = 1;
Deletion successfully happened. We can check the remaining rows using
Select
.
Query #2
SELECT * FROM `test`;
| id | a | b |
| --- | --- | --- |
| 2 | 3 | 4 |
1
Thanks! Thestatement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.
– Gustavo Lessa
Nov 2 at 23:33
1
@GustavoLessa beforeset signal state
we can set it to null back again. Please check the edited answer.
– Madhur Bhaiya
2 days ago
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Firstly, getting some syntax error(s) out of our way, from your original attempt:
- Instead of
FOR EACH STATEMENT
, it should beFOR EACH ROW
. - Since you have already defined the Delimiter to
//
; you need to use//
(instead of;
) in theDROP TRIGGER IF EXISTS ..
statement.
Row_Count()
will have 0 value in aBefore Delete Trigger
, as no rows have been updated yet. So this approach will not work.
Now, the trick here is to use Session-level Accessible (and Persistent) user-defined variables. We can define a variable, let's say @rows_being_deleted
, and later check whether it is already defined or not.
For Each Row
runs the same set of statements for every row being deleted. So, we will just check whether the session variable already exists or not. If it does not, we can define it. So basically, for the first row (being deleted), it will get defined, which will persist as long as the session is there.
Now if there are more rows to be deleted, Trigger would be running the same set of statements for the remaining rows. In the second row, the previously defined variable would be found now, and we can simply throw an exception now.
Note that there is a chance that within the same session, multiple delete statements may get triggered. So before throwing exception, we need to set the @rows_being_deleted
value back to null
.
Following will work:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion //
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON `test`
FOR EACH ROW
BEGIN
-- check if the variable is already defined or not
IF( @rows_being_deleted IS NULL ) THEN
SET @rows_being_deleted = 1; -- set its value
ELSE -- it already exists and we are in next "row"
-- just for testing to check the row count
-- SET @rows_being_deleted = @rows_being_deleted + 1;
-- We have to reset it to null, as within same session
-- another delete statement may be triggered.
SET @rows_being_deleted = NULL;
-- throw exception
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
DB Fiddle Demo 1: Trying to delete more than row.
DELETE FROM `test` WHERE `id`< 5;
Result:
Query Error: Error: ER_SIGNAL_EXCEPTION: Cannot delete more than one
order per time!
DB Fiddle Demo 2: Trying to delete only one row
Query #1
DELETE FROM `test` WHERE `id` = 1;
Deletion successfully happened. We can check the remaining rows using
Select
.
Query #2
SELECT * FROM `test`;
| id | a | b |
| --- | --- | --- |
| 2 | 3 | 4 |
1
Thanks! Thestatement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.
– Gustavo Lessa
Nov 2 at 23:33
1
@GustavoLessa beforeset signal state
we can set it to null back again. Please check the edited answer.
– Madhur Bhaiya
2 days ago
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
add a comment |
up vote
1
down vote
accepted
Firstly, getting some syntax error(s) out of our way, from your original attempt:
- Instead of
FOR EACH STATEMENT
, it should beFOR EACH ROW
. - Since you have already defined the Delimiter to
//
; you need to use//
(instead of;
) in theDROP TRIGGER IF EXISTS ..
statement.
Row_Count()
will have 0 value in aBefore Delete Trigger
, as no rows have been updated yet. So this approach will not work.
Now, the trick here is to use Session-level Accessible (and Persistent) user-defined variables. We can define a variable, let's say @rows_being_deleted
, and later check whether it is already defined or not.
For Each Row
runs the same set of statements for every row being deleted. So, we will just check whether the session variable already exists or not. If it does not, we can define it. So basically, for the first row (being deleted), it will get defined, which will persist as long as the session is there.
Now if there are more rows to be deleted, Trigger would be running the same set of statements for the remaining rows. In the second row, the previously defined variable would be found now, and we can simply throw an exception now.
Note that there is a chance that within the same session, multiple delete statements may get triggered. So before throwing exception, we need to set the @rows_being_deleted
value back to null
.
Following will work:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion //
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON `test`
FOR EACH ROW
BEGIN
-- check if the variable is already defined or not
IF( @rows_being_deleted IS NULL ) THEN
SET @rows_being_deleted = 1; -- set its value
ELSE -- it already exists and we are in next "row"
-- just for testing to check the row count
-- SET @rows_being_deleted = @rows_being_deleted + 1;
-- We have to reset it to null, as within same session
-- another delete statement may be triggered.
SET @rows_being_deleted = NULL;
-- throw exception
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
DB Fiddle Demo 1: Trying to delete more than row.
DELETE FROM `test` WHERE `id`< 5;
Result:
Query Error: Error: ER_SIGNAL_EXCEPTION: Cannot delete more than one
order per time!
DB Fiddle Demo 2: Trying to delete only one row
Query #1
DELETE FROM `test` WHERE `id` = 1;
Deletion successfully happened. We can check the remaining rows using
Select
.
Query #2
SELECT * FROM `test`;
| id | a | b |
| --- | --- | --- |
| 2 | 3 | 4 |
1
Thanks! Thestatement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.
– Gustavo Lessa
Nov 2 at 23:33
1
@GustavoLessa beforeset signal state
we can set it to null back again. Please check the edited answer.
– Madhur Bhaiya
2 days ago
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Firstly, getting some syntax error(s) out of our way, from your original attempt:
- Instead of
FOR EACH STATEMENT
, it should beFOR EACH ROW
. - Since you have already defined the Delimiter to
//
; you need to use//
(instead of;
) in theDROP TRIGGER IF EXISTS ..
statement.
Row_Count()
will have 0 value in aBefore Delete Trigger
, as no rows have been updated yet. So this approach will not work.
Now, the trick here is to use Session-level Accessible (and Persistent) user-defined variables. We can define a variable, let's say @rows_being_deleted
, and later check whether it is already defined or not.
For Each Row
runs the same set of statements for every row being deleted. So, we will just check whether the session variable already exists or not. If it does not, we can define it. So basically, for the first row (being deleted), it will get defined, which will persist as long as the session is there.
Now if there are more rows to be deleted, Trigger would be running the same set of statements for the remaining rows. In the second row, the previously defined variable would be found now, and we can simply throw an exception now.
Note that there is a chance that within the same session, multiple delete statements may get triggered. So before throwing exception, we need to set the @rows_being_deleted
value back to null
.
Following will work:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion //
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON `test`
FOR EACH ROW
BEGIN
-- check if the variable is already defined or not
IF( @rows_being_deleted IS NULL ) THEN
SET @rows_being_deleted = 1; -- set its value
ELSE -- it already exists and we are in next "row"
-- just for testing to check the row count
-- SET @rows_being_deleted = @rows_being_deleted + 1;
-- We have to reset it to null, as within same session
-- another delete statement may be triggered.
SET @rows_being_deleted = NULL;
-- throw exception
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
DB Fiddle Demo 1: Trying to delete more than row.
DELETE FROM `test` WHERE `id`< 5;
Result:
Query Error: Error: ER_SIGNAL_EXCEPTION: Cannot delete more than one
order per time!
DB Fiddle Demo 2: Trying to delete only one row
Query #1
DELETE FROM `test` WHERE `id` = 1;
Deletion successfully happened. We can check the remaining rows using
Select
.
Query #2
SELECT * FROM `test`;
| id | a | b |
| --- | --- | --- |
| 2 | 3 | 4 |
Firstly, getting some syntax error(s) out of our way, from your original attempt:
- Instead of
FOR EACH STATEMENT
, it should beFOR EACH ROW
. - Since you have already defined the Delimiter to
//
; you need to use//
(instead of;
) in theDROP TRIGGER IF EXISTS ..
statement.
Row_Count()
will have 0 value in aBefore Delete Trigger
, as no rows have been updated yet. So this approach will not work.
Now, the trick here is to use Session-level Accessible (and Persistent) user-defined variables. We can define a variable, let's say @rows_being_deleted
, and later check whether it is already defined or not.
For Each Row
runs the same set of statements for every row being deleted. So, we will just check whether the session variable already exists or not. If it does not, we can define it. So basically, for the first row (being deleted), it will get defined, which will persist as long as the session is there.
Now if there are more rows to be deleted, Trigger would be running the same set of statements for the remaining rows. In the second row, the previously defined variable would be found now, and we can simply throw an exception now.
Note that there is a chance that within the same session, multiple delete statements may get triggered. So before throwing exception, we need to set the @rows_being_deleted
value back to null
.
Following will work:
DELIMITER //
DROP TRIGGER IF EXISTS prevent_multiple_deletion //
CREATE TRIGGER prevent_multiple_deletion
BEFORE DELETE ON `test`
FOR EACH ROW
BEGIN
-- check if the variable is already defined or not
IF( @rows_being_deleted IS NULL ) THEN
SET @rows_being_deleted = 1; -- set its value
ELSE -- it already exists and we are in next "row"
-- just for testing to check the row count
-- SET @rows_being_deleted = @rows_being_deleted + 1;
-- We have to reset it to null, as within same session
-- another delete statement may be triggered.
SET @rows_being_deleted = NULL;
-- throw exception
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Cannot delete more than one order per time!';
END IF;
END //
DELIMITER ;
DB Fiddle Demo 1: Trying to delete more than row.
DELETE FROM `test` WHERE `id`< 5;
Result:
Query Error: Error: ER_SIGNAL_EXCEPTION: Cannot delete more than one
order per time!
DB Fiddle Demo 2: Trying to delete only one row
Query #1
DELETE FROM `test` WHERE `id` = 1;
Deletion successfully happened. We can check the remaining rows using
Select
.
Query #2
SELECT * FROM `test`;
| id | a | b |
| --- | --- | --- |
| 2 | 3 | 4 |
edited 2 days ago
answered Nov 2 at 14:56


Madhur Bhaiya
13.8k52035
13.8k52035
1
Thanks! Thestatement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.
– Gustavo Lessa
Nov 2 at 23:33
1
@GustavoLessa beforeset signal state
we can set it to null back again. Please check the edited answer.
– Madhur Bhaiya
2 days ago
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
add a comment |
1
Thanks! Thestatement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.
– Gustavo Lessa
Nov 2 at 23:33
1
@GustavoLessa beforeset signal state
we can set it to null back again. Please check the edited answer.
– Madhur Bhaiya
2 days ago
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
1
1
Thanks! The
statement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.– Gustavo Lessa
Nov 2 at 23:33
Thanks! The
statement
was a typo, but the delimiter part was driving me insane. Until I inserted it before the Delimiter.– Gustavo Lessa
Nov 2 at 23:33
1
1
@GustavoLessa before
set signal state
we can set it to null back again. Please check the edited answer.– Madhur Bhaiya
2 days ago
@GustavoLessa before
set signal state
we can set it to null back again. Please check the edited answer.– Madhur Bhaiya
2 days ago
1
1
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
Thanks Madhur. I replied to your solution here and started editing the answer to add exactly the same line you added now. You were faster than me =)
– Gustavo Lessa
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53120440%2fmysql-before-delete-trigger-to-avoid-deleting-multiple-rows%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
vQIFj5sKur8kTsHc,eCuqfaHrtmupThzAp f9,nx4V gQ phWa 79605VG2 I,Sg6,w1LU7I1GP0ORsX
I think that
ROW_COUNT()
will have 0 value (rows affected) only, in a Before Delete Trigger. Since, no rows have been affected until now.– Madhur Bhaiya
Nov 2 at 14:40