null parameters miscounts id on nodes
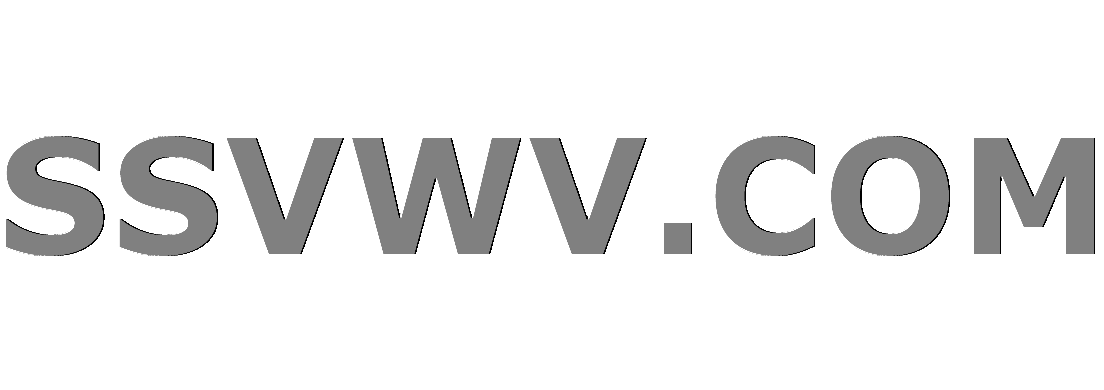
Multi tool use
up vote
0
down vote
favorite
So for my cs class we need to make a family tree with nodes with two nodes as roots. Every-time a node is created it has a unique id and the counter will keep increasing for every new node. I have a question regarding instantiating a class and setting items to null and counting every time a new node is created.
public class Node<T> implements Comparable<Node<T>> {
// instance variables
static int instanceCounter = 0;
int id = 0;
private T element;
private Node<T> fparent;
private Node<T> sparent;
private List<Node<T>> children;
public Node() {
instanceCounter++;
id = instanceCounter;
this(null, null, null, null, null); // -> this does not work
}
public Node(T element) {
this.element = element;
instanceCounter++;
id = instanceCounter;
}
public Node(T element, Node<T> fparent, Node<T> sparent, List<Node<T>> children) {
instanceCounter++;
id = instanceCounter;
this.element = element;
this.setFparent(fparent);
this.setSparent(sparent);
this.setChildren(children);
}
However this works:
public Node() {
this(null, null, null, null, null);
instanceCounter++;
id = instanceCounter;
}
What I want is :
Node 1 : Id = 1
Node 2 : Id = 2
Node 3 : Id = 3
but this what I get when the node instantiated with nothing in the parameters:
Node 1 : Id = 2
Node 2 : Id = 3
Node 3 : Id = 4
java
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
So for my cs class we need to make a family tree with nodes with two nodes as roots. Every-time a node is created it has a unique id and the counter will keep increasing for every new node. I have a question regarding instantiating a class and setting items to null and counting every time a new node is created.
public class Node<T> implements Comparable<Node<T>> {
// instance variables
static int instanceCounter = 0;
int id = 0;
private T element;
private Node<T> fparent;
private Node<T> sparent;
private List<Node<T>> children;
public Node() {
instanceCounter++;
id = instanceCounter;
this(null, null, null, null, null); // -> this does not work
}
public Node(T element) {
this.element = element;
instanceCounter++;
id = instanceCounter;
}
public Node(T element, Node<T> fparent, Node<T> sparent, List<Node<T>> children) {
instanceCounter++;
id = instanceCounter;
this.element = element;
this.setFparent(fparent);
this.setSparent(sparent);
this.setChildren(children);
}
However this works:
public Node() {
this(null, null, null, null, null);
instanceCounter++;
id = instanceCounter;
}
What I want is :
Node 1 : Id = 1
Node 2 : Id = 2
Node 3 : Id = 3
but this what I get when the node instantiated with nothing in the parameters:
Node 1 : Id = 2
Node 2 : Id = 3
Node 3 : Id = 4
java
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So for my cs class we need to make a family tree with nodes with two nodes as roots. Every-time a node is created it has a unique id and the counter will keep increasing for every new node. I have a question regarding instantiating a class and setting items to null and counting every time a new node is created.
public class Node<T> implements Comparable<Node<T>> {
// instance variables
static int instanceCounter = 0;
int id = 0;
private T element;
private Node<T> fparent;
private Node<T> sparent;
private List<Node<T>> children;
public Node() {
instanceCounter++;
id = instanceCounter;
this(null, null, null, null, null); // -> this does not work
}
public Node(T element) {
this.element = element;
instanceCounter++;
id = instanceCounter;
}
public Node(T element, Node<T> fparent, Node<T> sparent, List<Node<T>> children) {
instanceCounter++;
id = instanceCounter;
this.element = element;
this.setFparent(fparent);
this.setSparent(sparent);
this.setChildren(children);
}
However this works:
public Node() {
this(null, null, null, null, null);
instanceCounter++;
id = instanceCounter;
}
What I want is :
Node 1 : Id = 1
Node 2 : Id = 2
Node 3 : Id = 3
but this what I get when the node instantiated with nothing in the parameters:
Node 1 : Id = 2
Node 2 : Id = 3
Node 3 : Id = 4
java
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
So for my cs class we need to make a family tree with nodes with two nodes as roots. Every-time a node is created it has a unique id and the counter will keep increasing for every new node. I have a question regarding instantiating a class and setting items to null and counting every time a new node is created.
public class Node<T> implements Comparable<Node<T>> {
// instance variables
static int instanceCounter = 0;
int id = 0;
private T element;
private Node<T> fparent;
private Node<T> sparent;
private List<Node<T>> children;
public Node() {
instanceCounter++;
id = instanceCounter;
this(null, null, null, null, null); // -> this does not work
}
public Node(T element) {
this.element = element;
instanceCounter++;
id = instanceCounter;
}
public Node(T element, Node<T> fparent, Node<T> sparent, List<Node<T>> children) {
instanceCounter++;
id = instanceCounter;
this.element = element;
this.setFparent(fparent);
this.setSparent(sparent);
this.setChildren(children);
}
However this works:
public Node() {
this(null, null, null, null, null);
instanceCounter++;
id = instanceCounter;
}
What I want is :
Node 1 : Id = 1
Node 2 : Id = 2
Node 3 : Id = 3
but this what I get when the node instantiated with nothing in the parameters:
Node 1 : Id = 2
Node 2 : Id = 3
Node 3 : Id = 4
java
java
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 17 hours ago


peter-cs
12
12
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
peter-cs is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
instanceCounter is incremented twice when you call the default constructor. You should change your default constructor code to.
public Node() {
instanceCounter++;
id = instanceCounter;
//No call required here as all elements are already null
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
instanceCounter is incremented twice when you call the default constructor. You should change your default constructor code to.
public Node() {
instanceCounter++;
id = instanceCounter;
//No call required here as all elements are already null
}
add a comment |
up vote
0
down vote
instanceCounter is incremented twice when you call the default constructor. You should change your default constructor code to.
public Node() {
instanceCounter++;
id = instanceCounter;
//No call required here as all elements are already null
}
add a comment |
up vote
0
down vote
up vote
0
down vote
instanceCounter is incremented twice when you call the default constructor. You should change your default constructor code to.
public Node() {
instanceCounter++;
id = instanceCounter;
//No call required here as all elements are already null
}
instanceCounter is incremented twice when you call the default constructor. You should change your default constructor code to.
public Node() {
instanceCounter++;
id = instanceCounter;
//No call required here as all elements are already null
}
answered 17 hours ago
Abhisar
965
965
add a comment |
add a comment |
peter-cs is a new contributor. Be nice, and check out our Code of Conduct.
peter-cs is a new contributor. Be nice, and check out our Code of Conduct.
peter-cs is a new contributor. Be nice, and check out our Code of Conduct.
peter-cs is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237719%2fnull-parameters-miscounts-id-on-nodes%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
i4C3mCoWScdt4Yo