Spring Boot not able to detect the values that I have key in
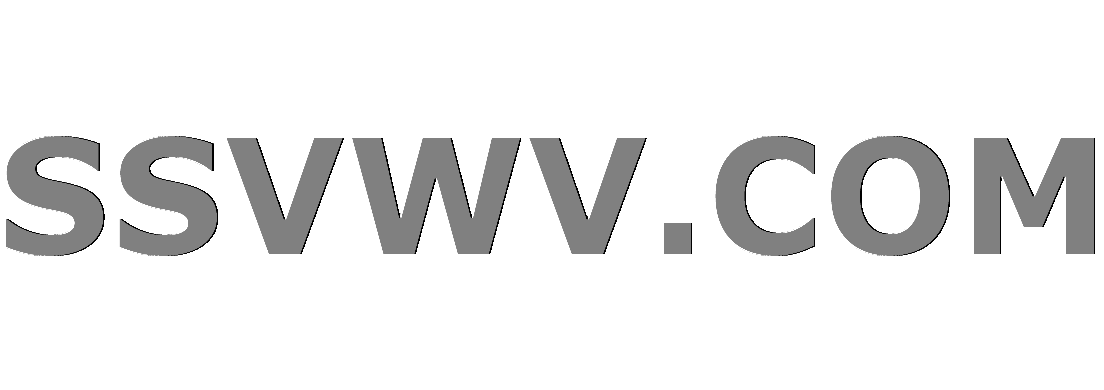
Multi tool use
up vote
0
down vote
favorite
I have problem regarding inserting data from angular to spring boot by rest API using mongodb atlas as the database. I have create another variable called numbers to test it out. But it turns out that the values was able to capture on angular but not in spring boot. Below are my codes:
Angular code:
<div class="todo-content">
<h1 class="page-title">My Todos</h1>
<div class="todo-create">
<form #todoForm="ngForm" (ngSubmit) = "createTodo(todoForm)" novalidate>
<input type="text" id="title" class="form-control" placeholder="Type a todo and press enter..."
required
name="title" [(ngModel)]="newTodo.title"
#title="ngModel" >
<input type="text" id="numbers" class="form-control" placeholder="Type a todo and press enter..."
required
name="numbers" [(ngModel)]="newTodo.numbers"
#numbers="ngModel" >
<div *ngIf="title.errors && title.dirty"
class="alert alert-danger">
<div [hidden]="!title.errors.required">
Title is required.
</div>
</div>
<td><button class="btn btn-danger" (ngSubmit) = "createTodo(todoForm)"> submit User</button></td>
</form>
</div>
<ul class="todo-list">
<li *ngFor="let todo of todos" [class.completed]= "todo.completed === true" >
<div class="todo-row" *ngIf="!editing || editingTodo.id != todo.id">
<a class="todo-completed" (click)="toggleCompleted(todo)">
<i class="material-icons toggle-completed-checkbox"></i>
</a>
<span class="todo-title">
{{todo.title}}
</span>
<span class="todo-actions">
<a (click)="editTodo(todo)">
<i class="material-icons edit">edit</i>
</a>
<a (click)="deleteTodo(todo.id)">
<i class="material-icons delete">clear</i>
</a>
</span>
</div>
<div class="todo-edit" *ngIf="editing && editingTodo.id === todo.id">
<input class="form-control" type="text"
[(ngModel)]="editingTodo.title" required>
<span class="edit-actions">
<a (click)="updateTodo(editingTodo)">
<i class="material-icons">done</i>
</a>
<a (click)="clearEditing()">
<i class="material-icons">clear</i>
</a>
</span>
</div>
</li>
</ul>
<div class="no-todos" *ngIf="todos && todos.length == 0">
<p>No Todos Found!</p>
</div>
todo-list.component.ts
import { Component, Input, OnInit } from '@angular/core';
import { TodoService } from './todo.service';
import { Todo } from './todo';
import {NgForm} from '@angular/forms';
@Component({
selector: 'todo-list',
templateUrl: './todo-list.component.html'
})
export class TodoListComponent implements OnInit {
todos: Todo;
newTodo: Todo = new Todo();
editing: boolean = false;
editingTodo: Todo = new Todo();
constructor(
private todoService: TodoService,
) {}
ngOnInit(): void {
this.getTodos();
}
getTodos(): void {
this.todoService.getTodos()
.then(todos => this.todos = todos );
}
createTodo(todoForm: NgForm): void {
console.log(this.newTodo.numbers);
console.log(todoForm);
this.todoService.createTodo(this.newTodo)
.then(createTodo => {
todoForm.reset();
this.newTodo = new Todo();
this.todos.unshift(createTodo)
});
}
deleteTodo(id: string): void {
this.todoService.deleteTodo(id)
.then(() => {
this.todos = this.todos.filter(todo => todo.id != id);
});
}
updateTodo(todoData: Todo): void {
console.log(todoData);
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
this.clearEditing();
});
}
toggleCompleted(todoData: Todo): void {
todoData.completed = !todoData.completed;
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
});
}
editTodo(todoData: Todo): void {
this.editing = true;
Object.assign(this.editingTodo, todoData);
}
clearEditing(): void {
this.editingTodo = new Todo();
this.editing = false;
}
}
todo.service.ts
import { Injectable } from '@angular/core';
import { Todo } from './todo';
import { Headers, Http } from '@angular/http';
import 'rxjs/add/operator/toPromise';
@Injectable()
export class TodoService {
private baseUrl = 'http://localhost:8080';
constructor(private http: Http) { }
getTodos(): Promise<Todo> {
return this.http.get(this.baseUrl + '/api/todos/')
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
createTodo(todoData: Todo): Promise<Todo> {
console.log("inside service");
console.log(todoData);
console.log(todoData.numbers);
return this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
.toPromise().then(response => response.json() as Todo)
.catch(this.handleError);
}
updateTodo(todoData: Todo): Promise<Todo> {
return this.http.put(this.baseUrl + '/api/todos/' + todoData.id, todoData)
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
deleteTodo(id: string): Promise<any> {
return this.http.delete(this.baseUrl + '/api/todos/' + id)
.toPromise()
.catch(this.handleError);
}
private handleError(error: any): Promise<any> {
console.error('Some error occured', error);
return Promise.reject(error.message || error);
}
}
todo.ts
export class Todo {
id: string;
title: string;
numbers: string;
completed: boolean;
createdAt: Date;
}
Spring Boot:
TodoController
package com.example.todoapp.controllers;
import javax.validation.Valid;
import com.example.todoapp.models.Todo;
import com.example.todoapp.repositories.TodoRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api")
@CrossOrigin("*")
public class TodoController {
@Autowired
TodoRepository todoRepository;
@GetMapping("/todos")
public List<Todo> getAllTodos() {
Sort sortByCreatedAtDesc = new Sort(Sort.Direction.DESC, "createdAt");
return todoRepository.findAll(sortByCreatedAtDesc);
}
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo, String numbers) {
System.out.println(todo.getNumber());
System.out.println(todo.getTitle());
System.out.println(numbers+ "numbers");
todo.setCompleted(false);
return todoRepository.save(todo);
}
@PostMapping("/contact")
public Todo createContact(@Valid @RequestBody Todo todo) {
todo.setCompleted(false);
return todoRepository.save(todo);
}
@GetMapping(value="/todos/{id}")
public ResponseEntity<Todo> getTodoById(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> ResponseEntity.ok().body(todo))
.orElse(ResponseEntity.notFound().build());
}
@PutMapping(value="/todos/{id}")
public ResponseEntity<Todo> updateTodo(@PathVariable("id") String id,
@Valid @RequestBody Todo todo) {
return todoRepository.findById(id)
.map(todoData -> {
todoData.setTitle(todo.getTitle());
todoData.setCompleted(todo.getCompleted());
Todo updatedTodo = todoRepository.save(todoData);
return ResponseEntity.ok().body(updatedTodo);
}).orElse(ResponseEntity.notFound().build());
}
@DeleteMapping(value="/todos/{id}")
public ResponseEntity<?> deleteTodo(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> {
todoRepository.deleteById(id);
return ResponseEntity.ok().build();
}).orElse(ResponseEntity.notFound().build());
}
}
TodoRepository
package com.example.todoapp.repositories;
import com.example.todoapp.models.Todo;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface TodoRepository extends MongoRepository<Todo, String> {
}
Todo
package com.example.todoapp.models;
import java.util.Date;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection="todos")
@JsonIgnoreProperties(value = {"createdAt"}, allowGetters = true)
public class Todo {
@Id
private String id;
@NotBlank
@Size(max=100)
@Indexed(unique=true)
private String title;
@Size(max=100)
@Indexed(unique=false)
private String numbers;
private Boolean completed = false;
private Date createdAt = new Date();
public Todo() {
super();
}
public Todo(String title, String numbers) {
this.title = title;
this.numbers= numbers;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getCompleted() {
return completed;
}
public void setCompleted(Boolean completed) {
this.completed = completed;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public String getNumber() {
return numbers;
}
public void setNumber(String number) {
this.numbers = number;
}
@Override
public String toString() {
return String.format(
"Todo[id=%s, title='%s',numbers='%s', completed='%s']",
id, title,numbers, completed);
}
}
Error from angular:
fff
todo-list.component.ts:32 NgForm {_submitted: true, ngSubmit: EventEmitter, form: FormGroup}
todo.service.ts:20 inside service
todo.service.ts:21 Todo {a: true, title: "My Web Application went blank on private mode", numbers: "fff"}
todo.service.ts:22 fff
Error From spring boot:
. ____ _ __ _ _
/\ / ___'_ __ _ _(_)_ __ __ _
( ( )___ | '_ | '_| | '_ / _` |
\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |___, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-11-10 16:13:21.940 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Starting TodoappApplication on NCS-180417AV05 with PID 9436 (E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2targetclasses started by royyzh in E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2)
2018-11-10 16:13:21.944 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : No active profile set, falling back to default profiles: default
2018-11-10 16:13:21.992 INFO 9436 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:23.367 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-11-10 16:13:23.393 INFO 9436 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2018-11-10 16:13:23.393 INFO 9436 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet Engine: Apache Tomcat/8.5.28
2018-11-10 16:13:23.399 INFO 9436 --- [ost-startStop-1] o.a.catalina.core.AprLifecycleListener : The APR based Apache Tomcat Native library which allows optimal performance in production environments was not found on the java.library.path: [C:Program FilesJavajre1.8.0_181bin;C:windowsSunJavabin;C:windowssystem32;C:windows;C:/Program Files/Java/jre1.8.0_181/bin/server;C:/Program Files/Java/jre1.8.0_181/bin;C:/Program Files/Java/jre1.8.0_181/lib/amd64;C:ProgramDataOracleJavajavapath;C:Program Files (x86)InteliCLS Client;C:Program FilesInteliCLS Client;C:windowssystem32;C:windows;C:windowsSystem32Wbem;C:windowsSystem32WindowsPowerShellv1.0;C:Program FilesIntelWiFibin;C:Program FilesCommon FilesIntelWirelessCommon;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsDAL;C:Program FilesIntelIntel(R) Management Engine ComponentsDAL;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsIPT;C:Program FilesIntelIntel(R) Management Engine ComponentsIPT;C:Program FilesTortoiseSVNbin;C:Program FilesPuTTY;C:Program FilesMicrosoft VS Codebin;C:Program FilesGitcmd;C:apache-maven-3.5.4bin;C:curl;C:Program Filesnodejs;D:eclipes java eemongodb-win32-x86_64-2008plus-ssl-4.0.3bin;C:UsersroyyzhAppDataRoamingnpm;C:UsersroyyzhAppDataLocalProgramsEmEditor;D:sts-bundlests-3.9.5.RELEASE;;.]
2018-11-10 16:13:23.692 INFO 9436 --- [ost-startStop-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2018-11-10 16:13:23.693 INFO 9436 --- [ost-startStop-1] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 1705 ms
2018-11-10 16:13:23.792 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.ServletRegistrationBean : Servlet dispatcherServlet mapped to [/]
2018-11-10 16:13:23.797 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'characterEncodingFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'hiddenHttpMethodFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'httpPutFormContentFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'requestContextFilter' to: [/*]
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Cluster created with settings {hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], mode=MULTIPLE, requiredClusterType=REPLICA_SET, serverSelectionTimeout='30000 ms', maxWaitQueueSize=500, requiredReplicaSetName='RoyYipProjectCluster-shard-0'}
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.746 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.747 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:25.123 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by com.mongodb.Mongo$4@2e52fb3e from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.138 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:1, serverValue:4829}] to royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.149 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}
2018-11-10 16:13:25.158 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by WritableServerSelector from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.201 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:3, serverValue:4482}] to royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.204 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:2, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.209 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=7415729, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621301859146}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_PRIMARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=5801588, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=7fffffff0000000000000005, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621302845567}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max election id to 7fffffff0000000000000005 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max set version to 1 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Discovered replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.296 INFO 9436 --- [ main] org.mongodb.driver.connection : Opened connection [connectionId{localValue:4, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.698 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerAdapter : Looking for @ControllerAdvice: org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:25.763 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/contact],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createContact(com.example.todoapp.models.Todo)
2018-11-10 16:13:25.765 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[GET]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getTodoById(java.lang.String)
2018-11-10 16:13:25.766 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createTodo(com.example.todoapp.models.Todo,java.lang.String)
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[GET]}" onto public java.util.List<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getAllTodos()
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[PUT]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.updateTodo(java.lang.String,com.example.todoapp.models.Todo)
2018-11-10 16:13:25.768 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[DELETE]}" onto public org.springframework.http.ResponseEntity<?> com.example.todoapp.controllers.TodoController.deleteTodo(java.lang.String)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error]}" onto public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error],produces=[text/html]}" onto public org.springframework.web.servlet.ModelAndView org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.errorHtml(javax.servlet.http.HttpServletRequest,javax.servlet.http.HttpServletResponse)
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.840 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.995 INFO 9436 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-11-10 16:13:26.060 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-11-10 16:13:26.064 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Started TodoappApplication in 4.443 seconds (JVM running for 6.802)
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-11-10 16:13:33.000 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 18 ms
null
My Web Application went blank on private mode
nullnumbers
null
My Web Application went blank on private mode
nullnumbers
What should I do to make it work? Which part of my code needs to be changed and why?
java spring angular mongodb spring-boot
add a comment |
up vote
0
down vote
favorite
I have problem regarding inserting data from angular to spring boot by rest API using mongodb atlas as the database. I have create another variable called numbers to test it out. But it turns out that the values was able to capture on angular but not in spring boot. Below are my codes:
Angular code:
<div class="todo-content">
<h1 class="page-title">My Todos</h1>
<div class="todo-create">
<form #todoForm="ngForm" (ngSubmit) = "createTodo(todoForm)" novalidate>
<input type="text" id="title" class="form-control" placeholder="Type a todo and press enter..."
required
name="title" [(ngModel)]="newTodo.title"
#title="ngModel" >
<input type="text" id="numbers" class="form-control" placeholder="Type a todo and press enter..."
required
name="numbers" [(ngModel)]="newTodo.numbers"
#numbers="ngModel" >
<div *ngIf="title.errors && title.dirty"
class="alert alert-danger">
<div [hidden]="!title.errors.required">
Title is required.
</div>
</div>
<td><button class="btn btn-danger" (ngSubmit) = "createTodo(todoForm)"> submit User</button></td>
</form>
</div>
<ul class="todo-list">
<li *ngFor="let todo of todos" [class.completed]= "todo.completed === true" >
<div class="todo-row" *ngIf="!editing || editingTodo.id != todo.id">
<a class="todo-completed" (click)="toggleCompleted(todo)">
<i class="material-icons toggle-completed-checkbox"></i>
</a>
<span class="todo-title">
{{todo.title}}
</span>
<span class="todo-actions">
<a (click)="editTodo(todo)">
<i class="material-icons edit">edit</i>
</a>
<a (click)="deleteTodo(todo.id)">
<i class="material-icons delete">clear</i>
</a>
</span>
</div>
<div class="todo-edit" *ngIf="editing && editingTodo.id === todo.id">
<input class="form-control" type="text"
[(ngModel)]="editingTodo.title" required>
<span class="edit-actions">
<a (click)="updateTodo(editingTodo)">
<i class="material-icons">done</i>
</a>
<a (click)="clearEditing()">
<i class="material-icons">clear</i>
</a>
</span>
</div>
</li>
</ul>
<div class="no-todos" *ngIf="todos && todos.length == 0">
<p>No Todos Found!</p>
</div>
todo-list.component.ts
import { Component, Input, OnInit } from '@angular/core';
import { TodoService } from './todo.service';
import { Todo } from './todo';
import {NgForm} from '@angular/forms';
@Component({
selector: 'todo-list',
templateUrl: './todo-list.component.html'
})
export class TodoListComponent implements OnInit {
todos: Todo;
newTodo: Todo = new Todo();
editing: boolean = false;
editingTodo: Todo = new Todo();
constructor(
private todoService: TodoService,
) {}
ngOnInit(): void {
this.getTodos();
}
getTodos(): void {
this.todoService.getTodos()
.then(todos => this.todos = todos );
}
createTodo(todoForm: NgForm): void {
console.log(this.newTodo.numbers);
console.log(todoForm);
this.todoService.createTodo(this.newTodo)
.then(createTodo => {
todoForm.reset();
this.newTodo = new Todo();
this.todos.unshift(createTodo)
});
}
deleteTodo(id: string): void {
this.todoService.deleteTodo(id)
.then(() => {
this.todos = this.todos.filter(todo => todo.id != id);
});
}
updateTodo(todoData: Todo): void {
console.log(todoData);
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
this.clearEditing();
});
}
toggleCompleted(todoData: Todo): void {
todoData.completed = !todoData.completed;
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
});
}
editTodo(todoData: Todo): void {
this.editing = true;
Object.assign(this.editingTodo, todoData);
}
clearEditing(): void {
this.editingTodo = new Todo();
this.editing = false;
}
}
todo.service.ts
import { Injectable } from '@angular/core';
import { Todo } from './todo';
import { Headers, Http } from '@angular/http';
import 'rxjs/add/operator/toPromise';
@Injectable()
export class TodoService {
private baseUrl = 'http://localhost:8080';
constructor(private http: Http) { }
getTodos(): Promise<Todo> {
return this.http.get(this.baseUrl + '/api/todos/')
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
createTodo(todoData: Todo): Promise<Todo> {
console.log("inside service");
console.log(todoData);
console.log(todoData.numbers);
return this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
.toPromise().then(response => response.json() as Todo)
.catch(this.handleError);
}
updateTodo(todoData: Todo): Promise<Todo> {
return this.http.put(this.baseUrl + '/api/todos/' + todoData.id, todoData)
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
deleteTodo(id: string): Promise<any> {
return this.http.delete(this.baseUrl + '/api/todos/' + id)
.toPromise()
.catch(this.handleError);
}
private handleError(error: any): Promise<any> {
console.error('Some error occured', error);
return Promise.reject(error.message || error);
}
}
todo.ts
export class Todo {
id: string;
title: string;
numbers: string;
completed: boolean;
createdAt: Date;
}
Spring Boot:
TodoController
package com.example.todoapp.controllers;
import javax.validation.Valid;
import com.example.todoapp.models.Todo;
import com.example.todoapp.repositories.TodoRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api")
@CrossOrigin("*")
public class TodoController {
@Autowired
TodoRepository todoRepository;
@GetMapping("/todos")
public List<Todo> getAllTodos() {
Sort sortByCreatedAtDesc = new Sort(Sort.Direction.DESC, "createdAt");
return todoRepository.findAll(sortByCreatedAtDesc);
}
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo, String numbers) {
System.out.println(todo.getNumber());
System.out.println(todo.getTitle());
System.out.println(numbers+ "numbers");
todo.setCompleted(false);
return todoRepository.save(todo);
}
@PostMapping("/contact")
public Todo createContact(@Valid @RequestBody Todo todo) {
todo.setCompleted(false);
return todoRepository.save(todo);
}
@GetMapping(value="/todos/{id}")
public ResponseEntity<Todo> getTodoById(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> ResponseEntity.ok().body(todo))
.orElse(ResponseEntity.notFound().build());
}
@PutMapping(value="/todos/{id}")
public ResponseEntity<Todo> updateTodo(@PathVariable("id") String id,
@Valid @RequestBody Todo todo) {
return todoRepository.findById(id)
.map(todoData -> {
todoData.setTitle(todo.getTitle());
todoData.setCompleted(todo.getCompleted());
Todo updatedTodo = todoRepository.save(todoData);
return ResponseEntity.ok().body(updatedTodo);
}).orElse(ResponseEntity.notFound().build());
}
@DeleteMapping(value="/todos/{id}")
public ResponseEntity<?> deleteTodo(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> {
todoRepository.deleteById(id);
return ResponseEntity.ok().build();
}).orElse(ResponseEntity.notFound().build());
}
}
TodoRepository
package com.example.todoapp.repositories;
import com.example.todoapp.models.Todo;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface TodoRepository extends MongoRepository<Todo, String> {
}
Todo
package com.example.todoapp.models;
import java.util.Date;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection="todos")
@JsonIgnoreProperties(value = {"createdAt"}, allowGetters = true)
public class Todo {
@Id
private String id;
@NotBlank
@Size(max=100)
@Indexed(unique=true)
private String title;
@Size(max=100)
@Indexed(unique=false)
private String numbers;
private Boolean completed = false;
private Date createdAt = new Date();
public Todo() {
super();
}
public Todo(String title, String numbers) {
this.title = title;
this.numbers= numbers;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getCompleted() {
return completed;
}
public void setCompleted(Boolean completed) {
this.completed = completed;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public String getNumber() {
return numbers;
}
public void setNumber(String number) {
this.numbers = number;
}
@Override
public String toString() {
return String.format(
"Todo[id=%s, title='%s',numbers='%s', completed='%s']",
id, title,numbers, completed);
}
}
Error from angular:
fff
todo-list.component.ts:32 NgForm {_submitted: true, ngSubmit: EventEmitter, form: FormGroup}
todo.service.ts:20 inside service
todo.service.ts:21 Todo {a: true, title: "My Web Application went blank on private mode", numbers: "fff"}
todo.service.ts:22 fff
Error From spring boot:
. ____ _ __ _ _
/\ / ___'_ __ _ _(_)_ __ __ _
( ( )___ | '_ | '_| | '_ / _` |
\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |___, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-11-10 16:13:21.940 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Starting TodoappApplication on NCS-180417AV05 with PID 9436 (E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2targetclasses started by royyzh in E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2)
2018-11-10 16:13:21.944 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : No active profile set, falling back to default profiles: default
2018-11-10 16:13:21.992 INFO 9436 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:23.367 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-11-10 16:13:23.393 INFO 9436 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2018-11-10 16:13:23.393 INFO 9436 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet Engine: Apache Tomcat/8.5.28
2018-11-10 16:13:23.399 INFO 9436 --- [ost-startStop-1] o.a.catalina.core.AprLifecycleListener : The APR based Apache Tomcat Native library which allows optimal performance in production environments was not found on the java.library.path: [C:Program FilesJavajre1.8.0_181bin;C:windowsSunJavabin;C:windowssystem32;C:windows;C:/Program Files/Java/jre1.8.0_181/bin/server;C:/Program Files/Java/jre1.8.0_181/bin;C:/Program Files/Java/jre1.8.0_181/lib/amd64;C:ProgramDataOracleJavajavapath;C:Program Files (x86)InteliCLS Client;C:Program FilesInteliCLS Client;C:windowssystem32;C:windows;C:windowsSystem32Wbem;C:windowsSystem32WindowsPowerShellv1.0;C:Program FilesIntelWiFibin;C:Program FilesCommon FilesIntelWirelessCommon;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsDAL;C:Program FilesIntelIntel(R) Management Engine ComponentsDAL;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsIPT;C:Program FilesIntelIntel(R) Management Engine ComponentsIPT;C:Program FilesTortoiseSVNbin;C:Program FilesPuTTY;C:Program FilesMicrosoft VS Codebin;C:Program FilesGitcmd;C:apache-maven-3.5.4bin;C:curl;C:Program Filesnodejs;D:eclipes java eemongodb-win32-x86_64-2008plus-ssl-4.0.3bin;C:UsersroyyzhAppDataRoamingnpm;C:UsersroyyzhAppDataLocalProgramsEmEditor;D:sts-bundlests-3.9.5.RELEASE;;.]
2018-11-10 16:13:23.692 INFO 9436 --- [ost-startStop-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2018-11-10 16:13:23.693 INFO 9436 --- [ost-startStop-1] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 1705 ms
2018-11-10 16:13:23.792 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.ServletRegistrationBean : Servlet dispatcherServlet mapped to [/]
2018-11-10 16:13:23.797 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'characterEncodingFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'hiddenHttpMethodFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'httpPutFormContentFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'requestContextFilter' to: [/*]
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Cluster created with settings {hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], mode=MULTIPLE, requiredClusterType=REPLICA_SET, serverSelectionTimeout='30000 ms', maxWaitQueueSize=500, requiredReplicaSetName='RoyYipProjectCluster-shard-0'}
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.746 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.747 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:25.123 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by com.mongodb.Mongo$4@2e52fb3e from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.138 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:1, serverValue:4829}] to royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.149 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}
2018-11-10 16:13:25.158 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by WritableServerSelector from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.201 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:3, serverValue:4482}] to royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.204 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:2, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.209 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=7415729, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621301859146}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_PRIMARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=5801588, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=7fffffff0000000000000005, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621302845567}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max election id to 7fffffff0000000000000005 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max set version to 1 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Discovered replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.296 INFO 9436 --- [ main] org.mongodb.driver.connection : Opened connection [connectionId{localValue:4, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.698 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerAdapter : Looking for @ControllerAdvice: org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:25.763 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/contact],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createContact(com.example.todoapp.models.Todo)
2018-11-10 16:13:25.765 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[GET]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getTodoById(java.lang.String)
2018-11-10 16:13:25.766 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createTodo(com.example.todoapp.models.Todo,java.lang.String)
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[GET]}" onto public java.util.List<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getAllTodos()
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[PUT]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.updateTodo(java.lang.String,com.example.todoapp.models.Todo)
2018-11-10 16:13:25.768 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[DELETE]}" onto public org.springframework.http.ResponseEntity<?> com.example.todoapp.controllers.TodoController.deleteTodo(java.lang.String)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error]}" onto public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error],produces=[text/html]}" onto public org.springframework.web.servlet.ModelAndView org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.errorHtml(javax.servlet.http.HttpServletRequest,javax.servlet.http.HttpServletResponse)
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.840 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.995 INFO 9436 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-11-10 16:13:26.060 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-11-10 16:13:26.064 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Started TodoappApplication in 4.443 seconds (JVM running for 6.802)
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-11-10 16:13:33.000 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 18 ms
null
My Web Application went blank on private mode
nullnumbers
null
My Web Application went blank on private mode
nullnumbers
What should I do to make it work? Which part of my code needs to be changed and why?
java spring angular mongodb spring-boot
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have problem regarding inserting data from angular to spring boot by rest API using mongodb atlas as the database. I have create another variable called numbers to test it out. But it turns out that the values was able to capture on angular but not in spring boot. Below are my codes:
Angular code:
<div class="todo-content">
<h1 class="page-title">My Todos</h1>
<div class="todo-create">
<form #todoForm="ngForm" (ngSubmit) = "createTodo(todoForm)" novalidate>
<input type="text" id="title" class="form-control" placeholder="Type a todo and press enter..."
required
name="title" [(ngModel)]="newTodo.title"
#title="ngModel" >
<input type="text" id="numbers" class="form-control" placeholder="Type a todo and press enter..."
required
name="numbers" [(ngModel)]="newTodo.numbers"
#numbers="ngModel" >
<div *ngIf="title.errors && title.dirty"
class="alert alert-danger">
<div [hidden]="!title.errors.required">
Title is required.
</div>
</div>
<td><button class="btn btn-danger" (ngSubmit) = "createTodo(todoForm)"> submit User</button></td>
</form>
</div>
<ul class="todo-list">
<li *ngFor="let todo of todos" [class.completed]= "todo.completed === true" >
<div class="todo-row" *ngIf="!editing || editingTodo.id != todo.id">
<a class="todo-completed" (click)="toggleCompleted(todo)">
<i class="material-icons toggle-completed-checkbox"></i>
</a>
<span class="todo-title">
{{todo.title}}
</span>
<span class="todo-actions">
<a (click)="editTodo(todo)">
<i class="material-icons edit">edit</i>
</a>
<a (click)="deleteTodo(todo.id)">
<i class="material-icons delete">clear</i>
</a>
</span>
</div>
<div class="todo-edit" *ngIf="editing && editingTodo.id === todo.id">
<input class="form-control" type="text"
[(ngModel)]="editingTodo.title" required>
<span class="edit-actions">
<a (click)="updateTodo(editingTodo)">
<i class="material-icons">done</i>
</a>
<a (click)="clearEditing()">
<i class="material-icons">clear</i>
</a>
</span>
</div>
</li>
</ul>
<div class="no-todos" *ngIf="todos && todos.length == 0">
<p>No Todos Found!</p>
</div>
todo-list.component.ts
import { Component, Input, OnInit } from '@angular/core';
import { TodoService } from './todo.service';
import { Todo } from './todo';
import {NgForm} from '@angular/forms';
@Component({
selector: 'todo-list',
templateUrl: './todo-list.component.html'
})
export class TodoListComponent implements OnInit {
todos: Todo;
newTodo: Todo = new Todo();
editing: boolean = false;
editingTodo: Todo = new Todo();
constructor(
private todoService: TodoService,
) {}
ngOnInit(): void {
this.getTodos();
}
getTodos(): void {
this.todoService.getTodos()
.then(todos => this.todos = todos );
}
createTodo(todoForm: NgForm): void {
console.log(this.newTodo.numbers);
console.log(todoForm);
this.todoService.createTodo(this.newTodo)
.then(createTodo => {
todoForm.reset();
this.newTodo = new Todo();
this.todos.unshift(createTodo)
});
}
deleteTodo(id: string): void {
this.todoService.deleteTodo(id)
.then(() => {
this.todos = this.todos.filter(todo => todo.id != id);
});
}
updateTodo(todoData: Todo): void {
console.log(todoData);
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
this.clearEditing();
});
}
toggleCompleted(todoData: Todo): void {
todoData.completed = !todoData.completed;
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
});
}
editTodo(todoData: Todo): void {
this.editing = true;
Object.assign(this.editingTodo, todoData);
}
clearEditing(): void {
this.editingTodo = new Todo();
this.editing = false;
}
}
todo.service.ts
import { Injectable } from '@angular/core';
import { Todo } from './todo';
import { Headers, Http } from '@angular/http';
import 'rxjs/add/operator/toPromise';
@Injectable()
export class TodoService {
private baseUrl = 'http://localhost:8080';
constructor(private http: Http) { }
getTodos(): Promise<Todo> {
return this.http.get(this.baseUrl + '/api/todos/')
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
createTodo(todoData: Todo): Promise<Todo> {
console.log("inside service");
console.log(todoData);
console.log(todoData.numbers);
return this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
.toPromise().then(response => response.json() as Todo)
.catch(this.handleError);
}
updateTodo(todoData: Todo): Promise<Todo> {
return this.http.put(this.baseUrl + '/api/todos/' + todoData.id, todoData)
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
deleteTodo(id: string): Promise<any> {
return this.http.delete(this.baseUrl + '/api/todos/' + id)
.toPromise()
.catch(this.handleError);
}
private handleError(error: any): Promise<any> {
console.error('Some error occured', error);
return Promise.reject(error.message || error);
}
}
todo.ts
export class Todo {
id: string;
title: string;
numbers: string;
completed: boolean;
createdAt: Date;
}
Spring Boot:
TodoController
package com.example.todoapp.controllers;
import javax.validation.Valid;
import com.example.todoapp.models.Todo;
import com.example.todoapp.repositories.TodoRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api")
@CrossOrigin("*")
public class TodoController {
@Autowired
TodoRepository todoRepository;
@GetMapping("/todos")
public List<Todo> getAllTodos() {
Sort sortByCreatedAtDesc = new Sort(Sort.Direction.DESC, "createdAt");
return todoRepository.findAll(sortByCreatedAtDesc);
}
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo, String numbers) {
System.out.println(todo.getNumber());
System.out.println(todo.getTitle());
System.out.println(numbers+ "numbers");
todo.setCompleted(false);
return todoRepository.save(todo);
}
@PostMapping("/contact")
public Todo createContact(@Valid @RequestBody Todo todo) {
todo.setCompleted(false);
return todoRepository.save(todo);
}
@GetMapping(value="/todos/{id}")
public ResponseEntity<Todo> getTodoById(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> ResponseEntity.ok().body(todo))
.orElse(ResponseEntity.notFound().build());
}
@PutMapping(value="/todos/{id}")
public ResponseEntity<Todo> updateTodo(@PathVariable("id") String id,
@Valid @RequestBody Todo todo) {
return todoRepository.findById(id)
.map(todoData -> {
todoData.setTitle(todo.getTitle());
todoData.setCompleted(todo.getCompleted());
Todo updatedTodo = todoRepository.save(todoData);
return ResponseEntity.ok().body(updatedTodo);
}).orElse(ResponseEntity.notFound().build());
}
@DeleteMapping(value="/todos/{id}")
public ResponseEntity<?> deleteTodo(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> {
todoRepository.deleteById(id);
return ResponseEntity.ok().build();
}).orElse(ResponseEntity.notFound().build());
}
}
TodoRepository
package com.example.todoapp.repositories;
import com.example.todoapp.models.Todo;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface TodoRepository extends MongoRepository<Todo, String> {
}
Todo
package com.example.todoapp.models;
import java.util.Date;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection="todos")
@JsonIgnoreProperties(value = {"createdAt"}, allowGetters = true)
public class Todo {
@Id
private String id;
@NotBlank
@Size(max=100)
@Indexed(unique=true)
private String title;
@Size(max=100)
@Indexed(unique=false)
private String numbers;
private Boolean completed = false;
private Date createdAt = new Date();
public Todo() {
super();
}
public Todo(String title, String numbers) {
this.title = title;
this.numbers= numbers;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getCompleted() {
return completed;
}
public void setCompleted(Boolean completed) {
this.completed = completed;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public String getNumber() {
return numbers;
}
public void setNumber(String number) {
this.numbers = number;
}
@Override
public String toString() {
return String.format(
"Todo[id=%s, title='%s',numbers='%s', completed='%s']",
id, title,numbers, completed);
}
}
Error from angular:
fff
todo-list.component.ts:32 NgForm {_submitted: true, ngSubmit: EventEmitter, form: FormGroup}
todo.service.ts:20 inside service
todo.service.ts:21 Todo {a: true, title: "My Web Application went blank on private mode", numbers: "fff"}
todo.service.ts:22 fff
Error From spring boot:
. ____ _ __ _ _
/\ / ___'_ __ _ _(_)_ __ __ _
( ( )___ | '_ | '_| | '_ / _` |
\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |___, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-11-10 16:13:21.940 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Starting TodoappApplication on NCS-180417AV05 with PID 9436 (E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2targetclasses started by royyzh in E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2)
2018-11-10 16:13:21.944 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : No active profile set, falling back to default profiles: default
2018-11-10 16:13:21.992 INFO 9436 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:23.367 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-11-10 16:13:23.393 INFO 9436 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2018-11-10 16:13:23.393 INFO 9436 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet Engine: Apache Tomcat/8.5.28
2018-11-10 16:13:23.399 INFO 9436 --- [ost-startStop-1] o.a.catalina.core.AprLifecycleListener : The APR based Apache Tomcat Native library which allows optimal performance in production environments was not found on the java.library.path: [C:Program FilesJavajre1.8.0_181bin;C:windowsSunJavabin;C:windowssystem32;C:windows;C:/Program Files/Java/jre1.8.0_181/bin/server;C:/Program Files/Java/jre1.8.0_181/bin;C:/Program Files/Java/jre1.8.0_181/lib/amd64;C:ProgramDataOracleJavajavapath;C:Program Files (x86)InteliCLS Client;C:Program FilesInteliCLS Client;C:windowssystem32;C:windows;C:windowsSystem32Wbem;C:windowsSystem32WindowsPowerShellv1.0;C:Program FilesIntelWiFibin;C:Program FilesCommon FilesIntelWirelessCommon;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsDAL;C:Program FilesIntelIntel(R) Management Engine ComponentsDAL;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsIPT;C:Program FilesIntelIntel(R) Management Engine ComponentsIPT;C:Program FilesTortoiseSVNbin;C:Program FilesPuTTY;C:Program FilesMicrosoft VS Codebin;C:Program FilesGitcmd;C:apache-maven-3.5.4bin;C:curl;C:Program Filesnodejs;D:eclipes java eemongodb-win32-x86_64-2008plus-ssl-4.0.3bin;C:UsersroyyzhAppDataRoamingnpm;C:UsersroyyzhAppDataLocalProgramsEmEditor;D:sts-bundlests-3.9.5.RELEASE;;.]
2018-11-10 16:13:23.692 INFO 9436 --- [ost-startStop-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2018-11-10 16:13:23.693 INFO 9436 --- [ost-startStop-1] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 1705 ms
2018-11-10 16:13:23.792 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.ServletRegistrationBean : Servlet dispatcherServlet mapped to [/]
2018-11-10 16:13:23.797 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'characterEncodingFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'hiddenHttpMethodFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'httpPutFormContentFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'requestContextFilter' to: [/*]
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Cluster created with settings {hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], mode=MULTIPLE, requiredClusterType=REPLICA_SET, serverSelectionTimeout='30000 ms', maxWaitQueueSize=500, requiredReplicaSetName='RoyYipProjectCluster-shard-0'}
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.746 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.747 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:25.123 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by com.mongodb.Mongo$4@2e52fb3e from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.138 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:1, serverValue:4829}] to royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.149 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}
2018-11-10 16:13:25.158 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by WritableServerSelector from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.201 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:3, serverValue:4482}] to royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.204 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:2, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.209 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=7415729, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621301859146}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_PRIMARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=5801588, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=7fffffff0000000000000005, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621302845567}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max election id to 7fffffff0000000000000005 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max set version to 1 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Discovered replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.296 INFO 9436 --- [ main] org.mongodb.driver.connection : Opened connection [connectionId{localValue:4, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.698 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerAdapter : Looking for @ControllerAdvice: org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:25.763 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/contact],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createContact(com.example.todoapp.models.Todo)
2018-11-10 16:13:25.765 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[GET]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getTodoById(java.lang.String)
2018-11-10 16:13:25.766 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createTodo(com.example.todoapp.models.Todo,java.lang.String)
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[GET]}" onto public java.util.List<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getAllTodos()
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[PUT]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.updateTodo(java.lang.String,com.example.todoapp.models.Todo)
2018-11-10 16:13:25.768 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[DELETE]}" onto public org.springframework.http.ResponseEntity<?> com.example.todoapp.controllers.TodoController.deleteTodo(java.lang.String)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error]}" onto public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error],produces=[text/html]}" onto public org.springframework.web.servlet.ModelAndView org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.errorHtml(javax.servlet.http.HttpServletRequest,javax.servlet.http.HttpServletResponse)
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.840 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.995 INFO 9436 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-11-10 16:13:26.060 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-11-10 16:13:26.064 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Started TodoappApplication in 4.443 seconds (JVM running for 6.802)
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-11-10 16:13:33.000 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 18 ms
null
My Web Application went blank on private mode
nullnumbers
null
My Web Application went blank on private mode
nullnumbers
What should I do to make it work? Which part of my code needs to be changed and why?
java spring angular mongodb spring-boot
I have problem regarding inserting data from angular to spring boot by rest API using mongodb atlas as the database. I have create another variable called numbers to test it out. But it turns out that the values was able to capture on angular but not in spring boot. Below are my codes:
Angular code:
<div class="todo-content">
<h1 class="page-title">My Todos</h1>
<div class="todo-create">
<form #todoForm="ngForm" (ngSubmit) = "createTodo(todoForm)" novalidate>
<input type="text" id="title" class="form-control" placeholder="Type a todo and press enter..."
required
name="title" [(ngModel)]="newTodo.title"
#title="ngModel" >
<input type="text" id="numbers" class="form-control" placeholder="Type a todo and press enter..."
required
name="numbers" [(ngModel)]="newTodo.numbers"
#numbers="ngModel" >
<div *ngIf="title.errors && title.dirty"
class="alert alert-danger">
<div [hidden]="!title.errors.required">
Title is required.
</div>
</div>
<td><button class="btn btn-danger" (ngSubmit) = "createTodo(todoForm)"> submit User</button></td>
</form>
</div>
<ul class="todo-list">
<li *ngFor="let todo of todos" [class.completed]= "todo.completed === true" >
<div class="todo-row" *ngIf="!editing || editingTodo.id != todo.id">
<a class="todo-completed" (click)="toggleCompleted(todo)">
<i class="material-icons toggle-completed-checkbox"></i>
</a>
<span class="todo-title">
{{todo.title}}
</span>
<span class="todo-actions">
<a (click)="editTodo(todo)">
<i class="material-icons edit">edit</i>
</a>
<a (click)="deleteTodo(todo.id)">
<i class="material-icons delete">clear</i>
</a>
</span>
</div>
<div class="todo-edit" *ngIf="editing && editingTodo.id === todo.id">
<input class="form-control" type="text"
[(ngModel)]="editingTodo.title" required>
<span class="edit-actions">
<a (click)="updateTodo(editingTodo)">
<i class="material-icons">done</i>
</a>
<a (click)="clearEditing()">
<i class="material-icons">clear</i>
</a>
</span>
</div>
</li>
</ul>
<div class="no-todos" *ngIf="todos && todos.length == 0">
<p>No Todos Found!</p>
</div>
todo-list.component.ts
import { Component, Input, OnInit } from '@angular/core';
import { TodoService } from './todo.service';
import { Todo } from './todo';
import {NgForm} from '@angular/forms';
@Component({
selector: 'todo-list',
templateUrl: './todo-list.component.html'
})
export class TodoListComponent implements OnInit {
todos: Todo;
newTodo: Todo = new Todo();
editing: boolean = false;
editingTodo: Todo = new Todo();
constructor(
private todoService: TodoService,
) {}
ngOnInit(): void {
this.getTodos();
}
getTodos(): void {
this.todoService.getTodos()
.then(todos => this.todos = todos );
}
createTodo(todoForm: NgForm): void {
console.log(this.newTodo.numbers);
console.log(todoForm);
this.todoService.createTodo(this.newTodo)
.then(createTodo => {
todoForm.reset();
this.newTodo = new Todo();
this.todos.unshift(createTodo)
});
}
deleteTodo(id: string): void {
this.todoService.deleteTodo(id)
.then(() => {
this.todos = this.todos.filter(todo => todo.id != id);
});
}
updateTodo(todoData: Todo): void {
console.log(todoData);
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
this.clearEditing();
});
}
toggleCompleted(todoData: Todo): void {
todoData.completed = !todoData.completed;
this.todoService.updateTodo(todoData)
.then(updatedTodo => {
let existingTodo = this.todos.find(todo => todo.id === updatedTodo.id);
Object.assign(existingTodo, updatedTodo);
});
}
editTodo(todoData: Todo): void {
this.editing = true;
Object.assign(this.editingTodo, todoData);
}
clearEditing(): void {
this.editingTodo = new Todo();
this.editing = false;
}
}
todo.service.ts
import { Injectable } from '@angular/core';
import { Todo } from './todo';
import { Headers, Http } from '@angular/http';
import 'rxjs/add/operator/toPromise';
@Injectable()
export class TodoService {
private baseUrl = 'http://localhost:8080';
constructor(private http: Http) { }
getTodos(): Promise<Todo> {
return this.http.get(this.baseUrl + '/api/todos/')
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
createTodo(todoData: Todo): Promise<Todo> {
console.log("inside service");
console.log(todoData);
console.log(todoData.numbers);
return this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
.toPromise().then(response => response.json() as Todo)
.catch(this.handleError);
}
updateTodo(todoData: Todo): Promise<Todo> {
return this.http.put(this.baseUrl + '/api/todos/' + todoData.id, todoData)
.toPromise()
.then(response => response.json() as Todo)
.catch(this.handleError);
}
deleteTodo(id: string): Promise<any> {
return this.http.delete(this.baseUrl + '/api/todos/' + id)
.toPromise()
.catch(this.handleError);
}
private handleError(error: any): Promise<any> {
console.error('Some error occured', error);
return Promise.reject(error.message || error);
}
}
todo.ts
export class Todo {
id: string;
title: string;
numbers: string;
completed: boolean;
createdAt: Date;
}
Spring Boot:
TodoController
package com.example.todoapp.controllers;
import javax.validation.Valid;
import com.example.todoapp.models.Todo;
import com.example.todoapp.repositories.TodoRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api")
@CrossOrigin("*")
public class TodoController {
@Autowired
TodoRepository todoRepository;
@GetMapping("/todos")
public List<Todo> getAllTodos() {
Sort sortByCreatedAtDesc = new Sort(Sort.Direction.DESC, "createdAt");
return todoRepository.findAll(sortByCreatedAtDesc);
}
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo, String numbers) {
System.out.println(todo.getNumber());
System.out.println(todo.getTitle());
System.out.println(numbers+ "numbers");
todo.setCompleted(false);
return todoRepository.save(todo);
}
@PostMapping("/contact")
public Todo createContact(@Valid @RequestBody Todo todo) {
todo.setCompleted(false);
return todoRepository.save(todo);
}
@GetMapping(value="/todos/{id}")
public ResponseEntity<Todo> getTodoById(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> ResponseEntity.ok().body(todo))
.orElse(ResponseEntity.notFound().build());
}
@PutMapping(value="/todos/{id}")
public ResponseEntity<Todo> updateTodo(@PathVariable("id") String id,
@Valid @RequestBody Todo todo) {
return todoRepository.findById(id)
.map(todoData -> {
todoData.setTitle(todo.getTitle());
todoData.setCompleted(todo.getCompleted());
Todo updatedTodo = todoRepository.save(todoData);
return ResponseEntity.ok().body(updatedTodo);
}).orElse(ResponseEntity.notFound().build());
}
@DeleteMapping(value="/todos/{id}")
public ResponseEntity<?> deleteTodo(@PathVariable("id") String id) {
return todoRepository.findById(id)
.map(todo -> {
todoRepository.deleteById(id);
return ResponseEntity.ok().build();
}).orElse(ResponseEntity.notFound().build());
}
}
TodoRepository
package com.example.todoapp.repositories;
import com.example.todoapp.models.Todo;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface TodoRepository extends MongoRepository<Todo, String> {
}
Todo
package com.example.todoapp.models;
import java.util.Date;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection="todos")
@JsonIgnoreProperties(value = {"createdAt"}, allowGetters = true)
public class Todo {
@Id
private String id;
@NotBlank
@Size(max=100)
@Indexed(unique=true)
private String title;
@Size(max=100)
@Indexed(unique=false)
private String numbers;
private Boolean completed = false;
private Date createdAt = new Date();
public Todo() {
super();
}
public Todo(String title, String numbers) {
this.title = title;
this.numbers= numbers;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getCompleted() {
return completed;
}
public void setCompleted(Boolean completed) {
this.completed = completed;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public String getNumber() {
return numbers;
}
public void setNumber(String number) {
this.numbers = number;
}
@Override
public String toString() {
return String.format(
"Todo[id=%s, title='%s',numbers='%s', completed='%s']",
id, title,numbers, completed);
}
}
Error from angular:
fff
todo-list.component.ts:32 NgForm {_submitted: true, ngSubmit: EventEmitter, form: FormGroup}
todo.service.ts:20 inside service
todo.service.ts:21 Todo {a: true, title: "My Web Application went blank on private mode", numbers: "fff"}
todo.service.ts:22 fff
Error From spring boot:
. ____ _ __ _ _
/\ / ___'_ __ _ _(_)_ __ __ _
( ( )___ | '_ | '_| | '_ / _` |
\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |___, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-11-10 16:13:21.940 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Starting TodoappApplication on NCS-180417AV05 with PID 9436 (E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2targetclasses started by royyzh in E:Projectportfolio_websitetemplatesspring-boot-mongodb-angular-todo-app-masterspring-boot-backendv2)
2018-11-10 16:13:21.944 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : No active profile set, falling back to default profiles: default
2018-11-10 16:13:21.992 INFO 9436 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:23.367 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-11-10 16:13:23.393 INFO 9436 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2018-11-10 16:13:23.393 INFO 9436 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet Engine: Apache Tomcat/8.5.28
2018-11-10 16:13:23.399 INFO 9436 --- [ost-startStop-1] o.a.catalina.core.AprLifecycleListener : The APR based Apache Tomcat Native library which allows optimal performance in production environments was not found on the java.library.path: [C:Program FilesJavajre1.8.0_181bin;C:windowsSunJavabin;C:windowssystem32;C:windows;C:/Program Files/Java/jre1.8.0_181/bin/server;C:/Program Files/Java/jre1.8.0_181/bin;C:/Program Files/Java/jre1.8.0_181/lib/amd64;C:ProgramDataOracleJavajavapath;C:Program Files (x86)InteliCLS Client;C:Program FilesInteliCLS Client;C:windowssystem32;C:windows;C:windowsSystem32Wbem;C:windowsSystem32WindowsPowerShellv1.0;C:Program FilesIntelWiFibin;C:Program FilesCommon FilesIntelWirelessCommon;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsDAL;C:Program FilesIntelIntel(R) Management Engine ComponentsDAL;C:Program Files (x86)IntelIntel(R) Management Engine ComponentsIPT;C:Program FilesIntelIntel(R) Management Engine ComponentsIPT;C:Program FilesTortoiseSVNbin;C:Program FilesPuTTY;C:Program FilesMicrosoft VS Codebin;C:Program FilesGitcmd;C:apache-maven-3.5.4bin;C:curl;C:Program Filesnodejs;D:eclipes java eemongodb-win32-x86_64-2008plus-ssl-4.0.3bin;C:UsersroyyzhAppDataRoamingnpm;C:UsersroyyzhAppDataLocalProgramsEmEditor;D:sts-bundlests-3.9.5.RELEASE;;.]
2018-11-10 16:13:23.692 INFO 9436 --- [ost-startStop-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2018-11-10 16:13:23.693 INFO 9436 --- [ost-startStop-1] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 1705 ms
2018-11-10 16:13:23.792 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.ServletRegistrationBean : Servlet dispatcherServlet mapped to [/]
2018-11-10 16:13:23.797 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'characterEncodingFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'hiddenHttpMethodFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'httpPutFormContentFilter' to: [/*]
2018-11-10 16:13:23.798 INFO 9436 --- [ost-startStop-1] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'requestContextFilter' to: [/*]
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Cluster created with settings {hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], mode=MULTIPLE, requiredClusterType=REPLICA_SET, serverSelectionTimeout='30000 ms', maxWaitQueueSize=500, requiredReplicaSetName='RoyYipProjectCluster-shard-0'}
2018-11-10 16:13:24.698 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.746 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:24.747 INFO 9436 --- [ main] org.mongodb.driver.cluster : Adding discovered server royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017 to client view of cluster
2018-11-10 16:13:25.123 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by com.mongodb.Mongo$4@2e52fb3e from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.138 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:1, serverValue:4829}] to royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.149 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}
2018-11-10 16:13:25.158 INFO 9436 --- [ main] org.mongodb.driver.cluster : No server chosen by WritableServerSelector from cluster description ClusterDescription{type=REPLICA_SET, connectionMode=MULTIPLE, serverDescriptions=[ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=UNKNOWN, state=CONNECTING}, ServerDescription{address=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=9102165, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621241304334}]}. Waiting for 30000 ms before timing out
2018-11-10 16:13:25.201 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:3, serverValue:4482}] to royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.204 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.connection : Opened connection [connectionId{localValue:2, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.209 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_SECONDARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=7415729, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=null, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621301859146}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Monitor thread successfully connected to server with description ServerDescription{address=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, type=REPLICA_SET_PRIMARY, state=CONNECTED, ok=true, version=ServerVersion{versionList=[4, 0, 4]}, minWireVersion=0, maxWireVersion=7, maxDocumentSize=16777216, logicalSessionTimeoutMinutes=30, roundTripTimeNanos=5801588, setName='RoyYipProjectCluster-shard-0', canonicalAddress=royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, hosts=[royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-01-uwgni.gcp.mongodb.net:27017, royyipprojectcluster-shard-00-02-uwgni.gcp.mongodb.net:27017], passives=, arbiters=, primary='royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017', tagSet=TagSet{}, electionId=7fffffff0000000000000005, setVersion=1, lastWriteDate=Sat Nov 10 16:13:26 SGT 2018, lastUpdateTimeNanos=33621302845567}
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max election id to 7fffffff0000000000000005 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Setting max set version to 1 from replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.210 INFO 9436 --- [ngodb.net:27017] org.mongodb.driver.cluster : Discovered replica set primary royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.296 INFO 9436 --- [ main] org.mongodb.driver.connection : Opened connection [connectionId{localValue:4, serverValue:4726}] to royyipprojectcluster-shard-00-00-uwgni.gcp.mongodb.net:27017
2018-11-10 16:13:25.698 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerAdapter : Looking for @ControllerAdvice: org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@327b636c: startup date [Sat Nov 10 16:13:21 SGT 2018]; root of context hierarchy
2018-11-10 16:13:25.763 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/contact],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createContact(com.example.todoapp.models.Todo)
2018-11-10 16:13:25.765 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[GET]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getTodoById(java.lang.String)
2018-11-10 16:13:25.766 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[POST]}" onto public com.example.todoapp.models.Todo com.example.todoapp.controllers.TodoController.createTodo(com.example.todoapp.models.Todo,java.lang.String)
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos],methods=[GET]}" onto public java.util.List<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.getAllTodos()
2018-11-10 16:13:25.767 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[PUT]}" onto public org.springframework.http.ResponseEntity<com.example.todoapp.models.Todo> com.example.todoapp.controllers.TodoController.updateTodo(java.lang.String,com.example.todoapp.models.Todo)
2018-11-10 16:13:25.768 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/api/todos/{id}],methods=[DELETE]}" onto public org.springframework.http.ResponseEntity<?> com.example.todoapp.controllers.TodoController.deleteTodo(java.lang.String)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error]}" onto public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-11-10 16:13:25.771 INFO 9436 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error],produces=[text/html]}" onto public org.springframework.web.servlet.ModelAndView org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.errorHtml(javax.servlet.http.HttpServletRequest,javax.servlet.http.HttpServletResponse)
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.793 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.840 INFO 9436 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-11-10 16:13:25.995 INFO 9436 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-11-10 16:13:26.060 INFO 9436 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-11-10 16:13:26.064 INFO 9436 --- [ main] com.example.todoapp.TodoappApplication : Started TodoappApplication in 4.443 seconds (JVM running for 6.802)
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-11-10 16:13:32.981 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-11-10 16:13:33.000 INFO 9436 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 18 ms
null
My Web Application went blank on private mode
nullnumbers
null
My Web Application went blank on private mode
nullnumbers
What should I do to make it work? Which part of my code needs to be changed and why?
java spring angular mongodb spring-boot
java spring angular mongodb spring-boot
edited 14 hours ago


James Z
11.1k71735
11.1k71735
asked 17 hours ago


Roy Yup
11
11
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
There are a few mistakes. Here are the fixes you need to make.
this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
What is the "a"
doing in the POST
call. You can remove that in createTodo
method declared in todo.service.ts
In Todo.java
the variable is declared as numbers
and the setter
is declared as
public void setNumber(String number) {
Change this to
public void setNumbers(String numbers) {
this.numbers = numbers;
}
Now you can update createTodo
method in TodoController.java
to the following
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo) {
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
There are a few mistakes. Here are the fixes you need to make.
this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
What is the "a"
doing in the POST
call. You can remove that in createTodo
method declared in todo.service.ts
In Todo.java
the variable is declared as numbers
and the setter
is declared as
public void setNumber(String number) {
Change this to
public void setNumbers(String numbers) {
this.numbers = numbers;
}
Now you can update createTodo
method in TodoController.java
to the following
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo) {
add a comment |
up vote
1
down vote
There are a few mistakes. Here are the fixes you need to make.
this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
What is the "a"
doing in the POST
call. You can remove that in createTodo
method declared in todo.service.ts
In Todo.java
the variable is declared as numbers
and the setter
is declared as
public void setNumber(String number) {
Change this to
public void setNumbers(String numbers) {
this.numbers = numbers;
}
Now you can update createTodo
method in TodoController.java
to the following
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo) {
add a comment |
up vote
1
down vote
up vote
1
down vote
There are a few mistakes. Here are the fixes you need to make.
this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
What is the "a"
doing in the POST
call. You can remove that in createTodo
method declared in todo.service.ts
In Todo.java
the variable is declared as numbers
and the setter
is declared as
public void setNumber(String number) {
Change this to
public void setNumbers(String numbers) {
this.numbers = numbers;
}
Now you can update createTodo
method in TodoController.java
to the following
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo) {
There are a few mistakes. Here are the fixes you need to make.
this.http.post(this.baseUrl + '/api/todos/', todoData, "a")
What is the "a"
doing in the POST
call. You can remove that in createTodo
method declared in todo.service.ts
In Todo.java
the variable is declared as numbers
and the setter
is declared as
public void setNumber(String number) {
Change this to
public void setNumbers(String numbers) {
this.numbers = numbers;
}
Now you can update createTodo
method in TodoController.java
to the following
@PostMapping("/todos")
public Todo createTodo(@Valid @RequestBody Todo todo) {
answered 15 hours ago
GSSwain
1,3412611
1,3412611
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237650%2fspring-boot-not-able-to-detect-the-values-that-i-have-key-in%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
XbUL lG91T dMbpN1N,91X R 6,TiF,4jOQSBD vvOPf17mY7EowsscWW9L2pjmklFnff1,SZ9UsZPmZmtTE7