React BarChart using D3 scrollable X-Axis
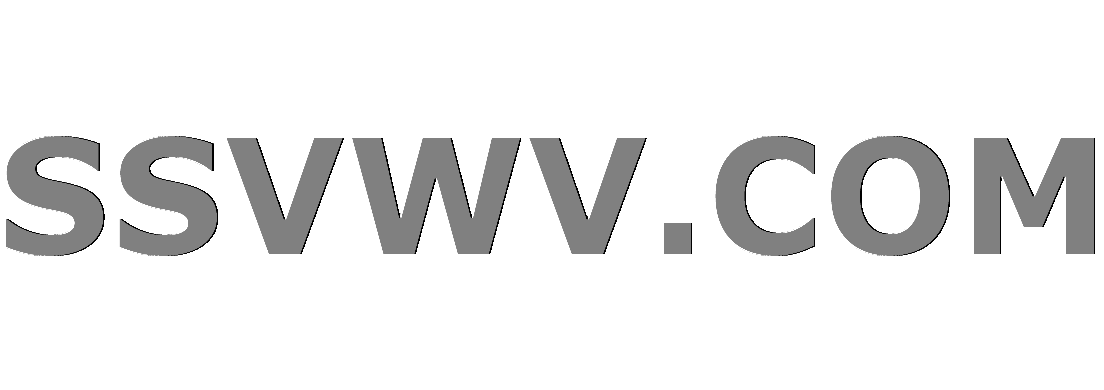
Multi tool use
up vote
5
down vote
favorite
I am currently trying my best to implement a bar chart that allows the user to scroll on the x-axis while the Y-Axis remains in place. I am using React as my framework.
export default [
{ activity: 'Main Idea and Detail', value: 90, color: '#2A78E4' },
{ activity: 'Character and Plot', value: 80, color: '#2A78E4' },
{ activity: 'Elements of Poetry', value: 70, color: '#2A78E4' },
{ activity: 'Standard 8.10', value: 60, color: '#2A78E4' },
{ activity: '8.1.3', value: 50, color: '#2A78E4' },
{ activity: 'Skill 6', value: 40, color: '#2A78E4' },
{ activity: 'Skill 7', value: 30, color: '#2A78E4' },
{ activity: 'Skill 8', value: 21, color: '#2A78E4' },
{ activity: 'Skill 9', value: 10, color: '#2A78E4' },
{ activity: 'Skill 10', value: 5, color: '#2A78E4' },
{ activity: '8.1.34', value: 50, color: '#2A78E4' },
{ activity: 'Skill 60', value: 40, color: '#2A78E4' },
{ activity: 'Skill 70', value: 30, color: '#2A78E4' },
{ activity: 'Skill 80', value: 21, color: '#2A78E4' },
{ activity: 'Skill 90', value: 10, color: '#2A78E4' },
{ activity: 'Skill 100', value: 5, color: '#2A78E4' },
{ activity: 'Skill 900', value: 100, color: '#2A78E4' },
{ activity: 'Skill 1000', value: 50, color: '#2A78E4' },
{ activity: 'Skill -1', value: 5, color: '#2A78E4' },
{ activity: '8.1.35', value: 50, color: '#2A78E4' },
{ activity: 'Skill 160', value: 40, color: '#2A78E4' },
{ activity: 'Skill 10', value: 30, color: '#2A78E4' },
{ activity: 'Skill 20', value: 21, color: '#2A78E4' },
{ activity: 'Skill 80', value: 10, color: '#2A78E4' },
{ activity: 'Skill 650', value: 5, color: '#2A78E4' },
{ activity: 'Skill 300', value: 100, color: '#2A78E4' },
{ activity: 'Skill 3000', value: 50, color: '#2A78E4' }
];
My code I am using to generate my scales are:
generateScales = () => {
const { height, margin, width } = this.state;
const xScales = d3
.scaleBand()
.domain(this.props.data.map(d => d.activity))
.range([margin.left, width - margin.right])
.padding(0.5);
const yScales = d3
.scaleLinear()
.domain([0, 100])
.range([height - margin.bottom, 0]);
this.setState({
xScales,
yScales
});
};
To generate my scales I use the renderAxis function:
renderAxis = () => {
const xAxisBottom = d3.axisBottom().scale(this.state.xScales);
const axisLeft = d3.axisLeft().scale(this.state.yScales);
d3.select(this.refs.xAxis).call(xAxisBottom);
d3.select(this.refs.yAxis).call(axisLeft);
};
I was trying to use this example as a clue, but I am unable to get this line to properly work "d3.behavior.drag().on("drag", display)" http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6.
I receive an error that this is not part of the d3 module, but the example is clearly using it. I guess I do not know where to start or how to tackle this problem. I tried to solve it also by using CSS, but to no avail
I was able to create a sandbox and if anybody could give me a clue on how to achieve a scrollable X-axis while having the Y-axis stay in place it will be really appreciated using React. Is the up above example the right place to be looking?
I have tried to solve my issue by using the zoom feature it seems like it's the best solution. I am still running into problems when using d3.event.transform.rescaleX it seems that the rescaleX method does not appear on the d3.event.transform object. I am really frustrated as all the examples appear to be using this function. Any help will be greatly appreciated.
https://codesandbox.io/s/k0pj5m8q93
reactjs d3.js bar-chart
This question has an open bounty worth +100
reputation from Louis345 ending in 4 days.
This question has not received enough attention.
A working example of how to make the Y-axis static while the user scrolls on the X-axis. I have provided a code sandbox and would be grateful if someone can take my existing work and make the x-axis scrollable horizontally.
add a comment |
up vote
5
down vote
favorite
I am currently trying my best to implement a bar chart that allows the user to scroll on the x-axis while the Y-Axis remains in place. I am using React as my framework.
export default [
{ activity: 'Main Idea and Detail', value: 90, color: '#2A78E4' },
{ activity: 'Character and Plot', value: 80, color: '#2A78E4' },
{ activity: 'Elements of Poetry', value: 70, color: '#2A78E4' },
{ activity: 'Standard 8.10', value: 60, color: '#2A78E4' },
{ activity: '8.1.3', value: 50, color: '#2A78E4' },
{ activity: 'Skill 6', value: 40, color: '#2A78E4' },
{ activity: 'Skill 7', value: 30, color: '#2A78E4' },
{ activity: 'Skill 8', value: 21, color: '#2A78E4' },
{ activity: 'Skill 9', value: 10, color: '#2A78E4' },
{ activity: 'Skill 10', value: 5, color: '#2A78E4' },
{ activity: '8.1.34', value: 50, color: '#2A78E4' },
{ activity: 'Skill 60', value: 40, color: '#2A78E4' },
{ activity: 'Skill 70', value: 30, color: '#2A78E4' },
{ activity: 'Skill 80', value: 21, color: '#2A78E4' },
{ activity: 'Skill 90', value: 10, color: '#2A78E4' },
{ activity: 'Skill 100', value: 5, color: '#2A78E4' },
{ activity: 'Skill 900', value: 100, color: '#2A78E4' },
{ activity: 'Skill 1000', value: 50, color: '#2A78E4' },
{ activity: 'Skill -1', value: 5, color: '#2A78E4' },
{ activity: '8.1.35', value: 50, color: '#2A78E4' },
{ activity: 'Skill 160', value: 40, color: '#2A78E4' },
{ activity: 'Skill 10', value: 30, color: '#2A78E4' },
{ activity: 'Skill 20', value: 21, color: '#2A78E4' },
{ activity: 'Skill 80', value: 10, color: '#2A78E4' },
{ activity: 'Skill 650', value: 5, color: '#2A78E4' },
{ activity: 'Skill 300', value: 100, color: '#2A78E4' },
{ activity: 'Skill 3000', value: 50, color: '#2A78E4' }
];
My code I am using to generate my scales are:
generateScales = () => {
const { height, margin, width } = this.state;
const xScales = d3
.scaleBand()
.domain(this.props.data.map(d => d.activity))
.range([margin.left, width - margin.right])
.padding(0.5);
const yScales = d3
.scaleLinear()
.domain([0, 100])
.range([height - margin.bottom, 0]);
this.setState({
xScales,
yScales
});
};
To generate my scales I use the renderAxis function:
renderAxis = () => {
const xAxisBottom = d3.axisBottom().scale(this.state.xScales);
const axisLeft = d3.axisLeft().scale(this.state.yScales);
d3.select(this.refs.xAxis).call(xAxisBottom);
d3.select(this.refs.yAxis).call(axisLeft);
};
I was trying to use this example as a clue, but I am unable to get this line to properly work "d3.behavior.drag().on("drag", display)" http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6.
I receive an error that this is not part of the d3 module, but the example is clearly using it. I guess I do not know where to start or how to tackle this problem. I tried to solve it also by using CSS, but to no avail
I was able to create a sandbox and if anybody could give me a clue on how to achieve a scrollable X-axis while having the Y-axis stay in place it will be really appreciated using React. Is the up above example the right place to be looking?
I have tried to solve my issue by using the zoom feature it seems like it's the best solution. I am still running into problems when using d3.event.transform.rescaleX it seems that the rescaleX method does not appear on the d3.event.transform object. I am really frustrated as all the examples appear to be using this function. Any help will be greatly appreciated.
https://codesandbox.io/s/k0pj5m8q93
reactjs d3.js bar-chart
This question has an open bounty worth +100
reputation from Louis345 ending in 4 days.
This question has not received enough attention.
A working example of how to make the Y-axis static while the user scrolls on the X-axis. I have provided a code sandbox and would be grateful if someone can take my existing work and make the x-axis scrollable horizontally.
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I am currently trying my best to implement a bar chart that allows the user to scroll on the x-axis while the Y-Axis remains in place. I am using React as my framework.
export default [
{ activity: 'Main Idea and Detail', value: 90, color: '#2A78E4' },
{ activity: 'Character and Plot', value: 80, color: '#2A78E4' },
{ activity: 'Elements of Poetry', value: 70, color: '#2A78E4' },
{ activity: 'Standard 8.10', value: 60, color: '#2A78E4' },
{ activity: '8.1.3', value: 50, color: '#2A78E4' },
{ activity: 'Skill 6', value: 40, color: '#2A78E4' },
{ activity: 'Skill 7', value: 30, color: '#2A78E4' },
{ activity: 'Skill 8', value: 21, color: '#2A78E4' },
{ activity: 'Skill 9', value: 10, color: '#2A78E4' },
{ activity: 'Skill 10', value: 5, color: '#2A78E4' },
{ activity: '8.1.34', value: 50, color: '#2A78E4' },
{ activity: 'Skill 60', value: 40, color: '#2A78E4' },
{ activity: 'Skill 70', value: 30, color: '#2A78E4' },
{ activity: 'Skill 80', value: 21, color: '#2A78E4' },
{ activity: 'Skill 90', value: 10, color: '#2A78E4' },
{ activity: 'Skill 100', value: 5, color: '#2A78E4' },
{ activity: 'Skill 900', value: 100, color: '#2A78E4' },
{ activity: 'Skill 1000', value: 50, color: '#2A78E4' },
{ activity: 'Skill -1', value: 5, color: '#2A78E4' },
{ activity: '8.1.35', value: 50, color: '#2A78E4' },
{ activity: 'Skill 160', value: 40, color: '#2A78E4' },
{ activity: 'Skill 10', value: 30, color: '#2A78E4' },
{ activity: 'Skill 20', value: 21, color: '#2A78E4' },
{ activity: 'Skill 80', value: 10, color: '#2A78E4' },
{ activity: 'Skill 650', value: 5, color: '#2A78E4' },
{ activity: 'Skill 300', value: 100, color: '#2A78E4' },
{ activity: 'Skill 3000', value: 50, color: '#2A78E4' }
];
My code I am using to generate my scales are:
generateScales = () => {
const { height, margin, width } = this.state;
const xScales = d3
.scaleBand()
.domain(this.props.data.map(d => d.activity))
.range([margin.left, width - margin.right])
.padding(0.5);
const yScales = d3
.scaleLinear()
.domain([0, 100])
.range([height - margin.bottom, 0]);
this.setState({
xScales,
yScales
});
};
To generate my scales I use the renderAxis function:
renderAxis = () => {
const xAxisBottom = d3.axisBottom().scale(this.state.xScales);
const axisLeft = d3.axisLeft().scale(this.state.yScales);
d3.select(this.refs.xAxis).call(xAxisBottom);
d3.select(this.refs.yAxis).call(axisLeft);
};
I was trying to use this example as a clue, but I am unable to get this line to properly work "d3.behavior.drag().on("drag", display)" http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6.
I receive an error that this is not part of the d3 module, but the example is clearly using it. I guess I do not know where to start or how to tackle this problem. I tried to solve it also by using CSS, but to no avail
I was able to create a sandbox and if anybody could give me a clue on how to achieve a scrollable X-axis while having the Y-axis stay in place it will be really appreciated using React. Is the up above example the right place to be looking?
I have tried to solve my issue by using the zoom feature it seems like it's the best solution. I am still running into problems when using d3.event.transform.rescaleX it seems that the rescaleX method does not appear on the d3.event.transform object. I am really frustrated as all the examples appear to be using this function. Any help will be greatly appreciated.
https://codesandbox.io/s/k0pj5m8q93
reactjs d3.js bar-chart
I am currently trying my best to implement a bar chart that allows the user to scroll on the x-axis while the Y-Axis remains in place. I am using React as my framework.
export default [
{ activity: 'Main Idea and Detail', value: 90, color: '#2A78E4' },
{ activity: 'Character and Plot', value: 80, color: '#2A78E4' },
{ activity: 'Elements of Poetry', value: 70, color: '#2A78E4' },
{ activity: 'Standard 8.10', value: 60, color: '#2A78E4' },
{ activity: '8.1.3', value: 50, color: '#2A78E4' },
{ activity: 'Skill 6', value: 40, color: '#2A78E4' },
{ activity: 'Skill 7', value: 30, color: '#2A78E4' },
{ activity: 'Skill 8', value: 21, color: '#2A78E4' },
{ activity: 'Skill 9', value: 10, color: '#2A78E4' },
{ activity: 'Skill 10', value: 5, color: '#2A78E4' },
{ activity: '8.1.34', value: 50, color: '#2A78E4' },
{ activity: 'Skill 60', value: 40, color: '#2A78E4' },
{ activity: 'Skill 70', value: 30, color: '#2A78E4' },
{ activity: 'Skill 80', value: 21, color: '#2A78E4' },
{ activity: 'Skill 90', value: 10, color: '#2A78E4' },
{ activity: 'Skill 100', value: 5, color: '#2A78E4' },
{ activity: 'Skill 900', value: 100, color: '#2A78E4' },
{ activity: 'Skill 1000', value: 50, color: '#2A78E4' },
{ activity: 'Skill -1', value: 5, color: '#2A78E4' },
{ activity: '8.1.35', value: 50, color: '#2A78E4' },
{ activity: 'Skill 160', value: 40, color: '#2A78E4' },
{ activity: 'Skill 10', value: 30, color: '#2A78E4' },
{ activity: 'Skill 20', value: 21, color: '#2A78E4' },
{ activity: 'Skill 80', value: 10, color: '#2A78E4' },
{ activity: 'Skill 650', value: 5, color: '#2A78E4' },
{ activity: 'Skill 300', value: 100, color: '#2A78E4' },
{ activity: 'Skill 3000', value: 50, color: '#2A78E4' }
];
My code I am using to generate my scales are:
generateScales = () => {
const { height, margin, width } = this.state;
const xScales = d3
.scaleBand()
.domain(this.props.data.map(d => d.activity))
.range([margin.left, width - margin.right])
.padding(0.5);
const yScales = d3
.scaleLinear()
.domain([0, 100])
.range([height - margin.bottom, 0]);
this.setState({
xScales,
yScales
});
};
To generate my scales I use the renderAxis function:
renderAxis = () => {
const xAxisBottom = d3.axisBottom().scale(this.state.xScales);
const axisLeft = d3.axisLeft().scale(this.state.yScales);
d3.select(this.refs.xAxis).call(xAxisBottom);
d3.select(this.refs.yAxis).call(axisLeft);
};
I was trying to use this example as a clue, but I am unable to get this line to properly work "d3.behavior.drag().on("drag", display)" http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6.
I receive an error that this is not part of the d3 module, but the example is clearly using it. I guess I do not know where to start or how to tackle this problem. I tried to solve it also by using CSS, but to no avail
I was able to create a sandbox and if anybody could give me a clue on how to achieve a scrollable X-axis while having the Y-axis stay in place it will be really appreciated using React. Is the up above example the right place to be looking?
I have tried to solve my issue by using the zoom feature it seems like it's the best solution. I am still running into problems when using d3.event.transform.rescaleX it seems that the rescaleX method does not appear on the d3.event.transform object. I am really frustrated as all the examples appear to be using this function. Any help will be greatly appreciated.
https://codesandbox.io/s/k0pj5m8q93
reactjs d3.js bar-chart
reactjs d3.js bar-chart
edited Nov 6 at 15:16
asked Nov 5 at 20:27
Louis345
198
198
This question has an open bounty worth +100
reputation from Louis345 ending in 4 days.
This question has not received enough attention.
A working example of how to make the Y-axis static while the user scrolls on the X-axis. I have provided a code sandbox and would be grateful if someone can take my existing work and make the x-axis scrollable horizontally.
This question has an open bounty worth +100
reputation from Louis345 ending in 4 days.
This question has not received enough attention.
A working example of how to make the Y-axis static while the user scrolls on the X-axis. I have provided a code sandbox and would be grateful if someone can take my existing work and make the x-axis scrollable horizontally.
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14
add a comment |
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Code Sandbox with solution:
https://codesandbox.io/s/4q5zxmy3zw
React Scroll Bar Chart based on:
http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6
D3 is used for calculation, React for rendering. I hope this will help.
add a comment |
up vote
0
down vote
In version 5, d3.behavior.drag() has been abandoned and you can get the similar properties in d3.drag().The detail could be found here:https://d3js.org.cn/api/d3-drag/#installing.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Code Sandbox with solution:
https://codesandbox.io/s/4q5zxmy3zw
React Scroll Bar Chart based on:
http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6
D3 is used for calculation, React for rendering. I hope this will help.
add a comment |
up vote
0
down vote
Code Sandbox with solution:
https://codesandbox.io/s/4q5zxmy3zw
React Scroll Bar Chart based on:
http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6
D3 is used for calculation, React for rendering. I hope this will help.
add a comment |
up vote
0
down vote
up vote
0
down vote
Code Sandbox with solution:
https://codesandbox.io/s/4q5zxmy3zw
React Scroll Bar Chart based on:
http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6
D3 is used for calculation, React for rendering. I hope this will help.
Code Sandbox with solution:
https://codesandbox.io/s/4q5zxmy3zw
React Scroll Bar Chart based on:
http://bl.ocks.org/cdagli/ce3045197f4b89367c80743c04bbb4b6
D3 is used for calculation, React for rendering. I hope this will help.
answered 19 hours ago
Boris Traljić
375
375
add a comment |
add a comment |
up vote
0
down vote
In version 5, d3.behavior.drag() has been abandoned and you can get the similar properties in d3.drag().The detail could be found here:https://d3js.org.cn/api/d3-drag/#installing.
add a comment |
up vote
0
down vote
In version 5, d3.behavior.drag() has been abandoned and you can get the similar properties in d3.drag().The detail could be found here:https://d3js.org.cn/api/d3-drag/#installing.
add a comment |
up vote
0
down vote
up vote
0
down vote
In version 5, d3.behavior.drag() has been abandoned and you can get the similar properties in d3.drag().The detail could be found here:https://d3js.org.cn/api/d3-drag/#installing.
In version 5, d3.behavior.drag() has been abandoned and you can get the similar properties in d3.drag().The detail could be found here:https://d3js.org.cn/api/d3-drag/#installing.
answered 13 hours ago
Root
80717
80717
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53161715%2freact-barchart-using-d3-scrollable-x-axis%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
HRKlOjR7fFUek4ZojNa,urQULKUI3KEG3YbS,9LL6XE0,p00UTLt q4D
I think this is a version mismatch issue. that example you show is using d3 version 3 and you're using version 5. There was a massive refactor between v3 and v4 and I think you're trying to use modules that have been split out
– wpercy
Nov 5 at 21:47
Thanks. Since I am using version 5 I cannot get access to d3.event.transform.rescaleX and i searched the internet to no avail. I have updated my code sandbox.
– Louis345
Nov 6 at 15:14