Pythonic Way to Sort a List of Comma Separated Numbers
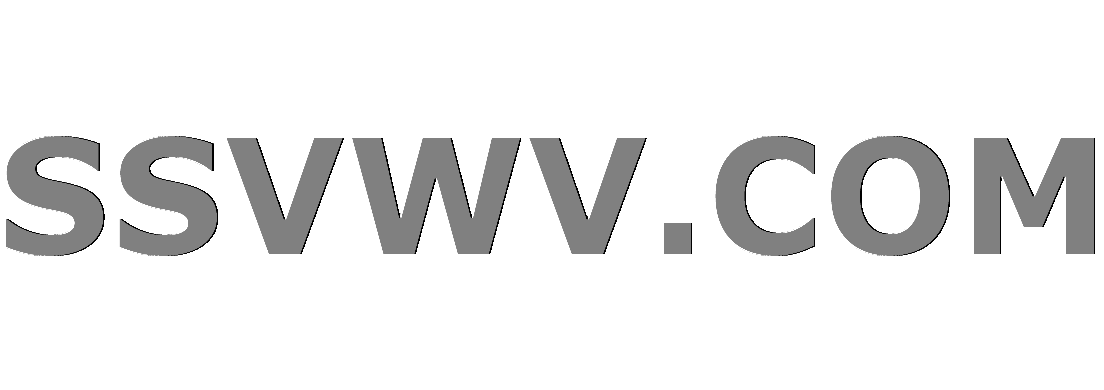
Multi tool use
up vote
3
down vote
favorite
Sample Input
20, 71146620
100, 26867616
10, 02513583
10, 52811698
100, 23859051
I read it in from a file as a command line argument to a list with
lin = [i.strip() for i in open(sys.argv[1]).readlines()]
that list looks like ['20, 71146620', '100, 26867616', '10, 02513583', '10, 52811698', '100, 23859051']
My hope is to find the most pythonic way to sort this list, first for the first value and then for the second so that it looks like:
['10, 02513583', '10, 52811698', '20, 71146620', '100, 23859051', '100, 26867616', ]
I'm currently trying to convert it to a list of key value pairs, but I'm not sure that's the right direction to take.
python list sorting
add a comment |
up vote
3
down vote
favorite
Sample Input
20, 71146620
100, 26867616
10, 02513583
10, 52811698
100, 23859051
I read it in from a file as a command line argument to a list with
lin = [i.strip() for i in open(sys.argv[1]).readlines()]
that list looks like ['20, 71146620', '100, 26867616', '10, 02513583', '10, 52811698', '100, 23859051']
My hope is to find the most pythonic way to sort this list, first for the first value and then for the second so that it looks like:
['10, 02513583', '10, 52811698', '20, 71146620', '100, 23859051', '100, 26867616', ]
I'm currently trying to convert it to a list of key value pairs, but I'm not sure that's the right direction to take.
python list sorting
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
Sample Input
20, 71146620
100, 26867616
10, 02513583
10, 52811698
100, 23859051
I read it in from a file as a command line argument to a list with
lin = [i.strip() for i in open(sys.argv[1]).readlines()]
that list looks like ['20, 71146620', '100, 26867616', '10, 02513583', '10, 52811698', '100, 23859051']
My hope is to find the most pythonic way to sort this list, first for the first value and then for the second so that it looks like:
['10, 02513583', '10, 52811698', '20, 71146620', '100, 23859051', '100, 26867616', ]
I'm currently trying to convert it to a list of key value pairs, but I'm not sure that's the right direction to take.
python list sorting
Sample Input
20, 71146620
100, 26867616
10, 02513583
10, 52811698
100, 23859051
I read it in from a file as a command line argument to a list with
lin = [i.strip() for i in open(sys.argv[1]).readlines()]
that list looks like ['20, 71146620', '100, 26867616', '10, 02513583', '10, 52811698', '100, 23859051']
My hope is to find the most pythonic way to sort this list, first for the first value and then for the second so that it looks like:
['10, 02513583', '10, 52811698', '20, 71146620', '100, 23859051', '100, 26867616', ]
I'm currently trying to convert it to a list of key value pairs, but I'm not sure that's the right direction to take.
python list sorting
python list sorting
asked Oct 14 '13 at 4:41
frankV
1,67442439
1,67442439
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17
add a comment |
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17
add a comment |
4 Answers
4
active
oldest
votes
up vote
4
down vote
accepted
The easiest thing to do is to parse the pairs into lists and then just sort them:
lin = [i.strip().split(', ') for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
In case you want to sort numerically, just cast to numbers:
lin = [map(int, i.strip().split(', ')) for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
add a comment |
up vote
1
down vote
import sys
with open(sys.argv[1]) as f:
lin = sorted([[int(j) for j in i.split(",")] for i in f])
print lin
add a comment |
up vote
1
down vote
You can sort the lines as strings, by using a key function
def two_ints(s):
return map(int, s.split(","))
with open("num.txt") as f:
for line in sorted(f, key=two_ints):
print line
It really depends whether you want the result to be a list of strings, or a list of lists of ints.
Once you have converted to int, there is no way to recover the leading zero on "02513583", so leaving the result as strings may be preferable
add a comment |
up vote
0
down vote
why not just use the csv module and feed it to sort (with a string to integer conversion)
import csv
with open("test.csv") as f:
cr = csv.reader(f)
result = sorted(list(map(int,row)) for row in cr)
outcome:
>>> result
[[10, 2513583],
[10, 52811698],
[20, 71146620],
[100, 23859051],
[100, 26867616]]
sort
does exactly what's asked here: it uses natural sorting order of lists
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
The easiest thing to do is to parse the pairs into lists and then just sort them:
lin = [i.strip().split(', ') for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
In case you want to sort numerically, just cast to numbers:
lin = [map(int, i.strip().split(', ')) for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
add a comment |
up vote
4
down vote
accepted
The easiest thing to do is to parse the pairs into lists and then just sort them:
lin = [i.strip().split(', ') for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
In case you want to sort numerically, just cast to numbers:
lin = [map(int, i.strip().split(', ')) for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
The easiest thing to do is to parse the pairs into lists and then just sort them:
lin = [i.strip().split(', ') for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
In case you want to sort numerically, just cast to numbers:
lin = [map(int, i.strip().split(', ')) for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
The easiest thing to do is to parse the pairs into lists and then just sort them:
lin = [i.strip().split(', ') for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
In case you want to sort numerically, just cast to numbers:
lin = [map(int, i.strip().split(', ')) for i in open(sys.argv[1]).readlines()]
lin = sorted(lin)
edited Oct 14 '13 at 4:49
answered Oct 14 '13 at 4:44
Petar Ivanov
67.1k66280
67.1k66280
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
add a comment |
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
Yes I was hoping for numerical sort. Thank you both. Wish I could select both as correct.
– frankV
Oct 14 '13 at 4:47
add a comment |
up vote
1
down vote
import sys
with open(sys.argv[1]) as f:
lin = sorted([[int(j) for j in i.split(",")] for i in f])
print lin
add a comment |
up vote
1
down vote
import sys
with open(sys.argv[1]) as f:
lin = sorted([[int(j) for j in i.split(",")] for i in f])
print lin
add a comment |
up vote
1
down vote
up vote
1
down vote
import sys
with open(sys.argv[1]) as f:
lin = sorted([[int(j) for j in i.split(",")] for i in f])
print lin
import sys
with open(sys.argv[1]) as f:
lin = sorted([[int(j) for j in i.split(",")] for i in f])
print lin
edited Oct 14 '13 at 4:50
answered Oct 14 '13 at 4:45
Robᵩ
113k12129212
113k12129212
add a comment |
add a comment |
up vote
1
down vote
You can sort the lines as strings, by using a key function
def two_ints(s):
return map(int, s.split(","))
with open("num.txt") as f:
for line in sorted(f, key=two_ints):
print line
It really depends whether you want the result to be a list of strings, or a list of lists of ints.
Once you have converted to int, there is no way to recover the leading zero on "02513583", so leaving the result as strings may be preferable
add a comment |
up vote
1
down vote
You can sort the lines as strings, by using a key function
def two_ints(s):
return map(int, s.split(","))
with open("num.txt") as f:
for line in sorted(f, key=two_ints):
print line
It really depends whether you want the result to be a list of strings, or a list of lists of ints.
Once you have converted to int, there is no way to recover the leading zero on "02513583", so leaving the result as strings may be preferable
add a comment |
up vote
1
down vote
up vote
1
down vote
You can sort the lines as strings, by using a key function
def two_ints(s):
return map(int, s.split(","))
with open("num.txt") as f:
for line in sorted(f, key=two_ints):
print line
It really depends whether you want the result to be a list of strings, or a list of lists of ints.
Once you have converted to int, there is no way to recover the leading zero on "02513583", so leaving the result as strings may be preferable
You can sort the lines as strings, by using a key function
def two_ints(s):
return map(int, s.split(","))
with open("num.txt") as f:
for line in sorted(f, key=two_ints):
print line
It really depends whether you want the result to be a list of strings, or a list of lists of ints.
Once you have converted to int, there is no way to recover the leading zero on "02513583", so leaving the result as strings may be preferable
answered Oct 14 '13 at 5:09


John La Rooy
205k37270424
205k37270424
add a comment |
add a comment |
up vote
0
down vote
why not just use the csv module and feed it to sort (with a string to integer conversion)
import csv
with open("test.csv") as f:
cr = csv.reader(f)
result = sorted(list(map(int,row)) for row in cr)
outcome:
>>> result
[[10, 2513583],
[10, 52811698],
[20, 71146620],
[100, 23859051],
[100, 26867616]]
sort
does exactly what's asked here: it uses natural sorting order of lists
add a comment |
up vote
0
down vote
why not just use the csv module and feed it to sort (with a string to integer conversion)
import csv
with open("test.csv") as f:
cr = csv.reader(f)
result = sorted(list(map(int,row)) for row in cr)
outcome:
>>> result
[[10, 2513583],
[10, 52811698],
[20, 71146620],
[100, 23859051],
[100, 26867616]]
sort
does exactly what's asked here: it uses natural sorting order of lists
add a comment |
up vote
0
down vote
up vote
0
down vote
why not just use the csv module and feed it to sort (with a string to integer conversion)
import csv
with open("test.csv") as f:
cr = csv.reader(f)
result = sorted(list(map(int,row)) for row in cr)
outcome:
>>> result
[[10, 2513583],
[10, 52811698],
[20, 71146620],
[100, 23859051],
[100, 26867616]]
sort
does exactly what's asked here: it uses natural sorting order of lists
why not just use the csv module and feed it to sort (with a string to integer conversion)
import csv
with open("test.csv") as f:
cr = csv.reader(f)
result = sorted(list(map(int,row)) for row in cr)
outcome:
>>> result
[[10, 2513583],
[10, 52811698],
[20, 71146620],
[100, 23859051],
[100, 26867616]]
sort
does exactly what's asked here: it uses natural sorting order of lists
answered 22 hours ago


Jean-François Fabre
96.6k949106
96.6k949106
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f19353638%2fpythonic-way-to-sort-a-list-of-comma-separated-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
6Lhd,t SD hF0v0bnG5G,9CF23Z95IhQ1 7oz7fpAaO3iDM4b,IxcCJp,BsuKWfX6yqO weHQH2pUkwv6He,xA Hh3wTks1eQJZHsreYF
Two things to remember - 1/ Standard Dictionaries only have 1 value per key so you would loose on 10: entry, 2/ Standard Dictionaries can not be "sorted". So key, value pairs is the wrong name if your example data & output is correct.
– Steve Barnes
Oct 14 '13 at 5:17