What's the simplest way to print a Java array?
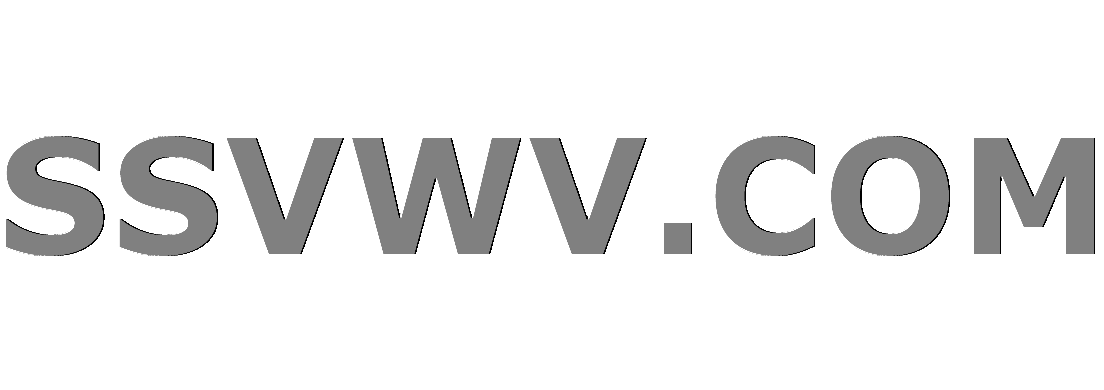
Multi tool use
up vote
1628
down vote
favorite
In Java, arrays don't override toString()
, so if you try to print one directly, you get the className + @ + the hex of the hashCode
of the array, as defined by Object.toString()
:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(intArray); // prints something like '[I@3343c8b3'
But usually we'd actually want something more like [1, 2, 3, 4, 5]
. What's the simplest way of doing that? Here are some example inputs and outputs:
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
//output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
//output: [John, Mary, Bob]
java arrays printing
|
show 1 more comment
up vote
1628
down vote
favorite
In Java, arrays don't override toString()
, so if you try to print one directly, you get the className + @ + the hex of the hashCode
of the array, as defined by Object.toString()
:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(intArray); // prints something like '[I@3343c8b3'
But usually we'd actually want something more like [1, 2, 3, 4, 5]
. What's the simplest way of doing that? Here are some example inputs and outputs:
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
//output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
//output: [John, Mary, Bob]
java arrays printing
5
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
2
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
2
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
1
That weird output is not necessarily the memory location. It's thehashCode()
in hexadecimal. SeeObject#toString()
.
– 4castle
Nov 10 '16 at 21:22
1
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28
|
show 1 more comment
up vote
1628
down vote
favorite
up vote
1628
down vote
favorite
In Java, arrays don't override toString()
, so if you try to print one directly, you get the className + @ + the hex of the hashCode
of the array, as defined by Object.toString()
:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(intArray); // prints something like '[I@3343c8b3'
But usually we'd actually want something more like [1, 2, 3, 4, 5]
. What's the simplest way of doing that? Here are some example inputs and outputs:
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
//output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
//output: [John, Mary, Bob]
java arrays printing
In Java, arrays don't override toString()
, so if you try to print one directly, you get the className + @ + the hex of the hashCode
of the array, as defined by Object.toString()
:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(intArray); // prints something like '[I@3343c8b3'
But usually we'd actually want something more like [1, 2, 3, 4, 5]
. What's the simplest way of doing that? Here are some example inputs and outputs:
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
//output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
//output: [John, Mary, Bob]
java arrays printing
java arrays printing
edited Jan 3 at 17:17
Md. Abu Nafee Ibna Zahid
4031514
4031514
asked Jan 3 '09 at 20:39
Alex Spurling
19.6k185161
19.6k185161
5
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
2
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
2
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
1
That weird output is not necessarily the memory location. It's thehashCode()
in hexadecimal. SeeObject#toString()
.
– 4castle
Nov 10 '16 at 21:22
1
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28
|
show 1 more comment
5
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
2
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
2
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
1
That weird output is not necessarily the memory location. It's thehashCode()
in hexadecimal. SeeObject#toString()
.
– 4castle
Nov 10 '16 at 21:22
1
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28
5
5
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
2
2
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
2
2
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
1
1
That weird output is not necessarily the memory location. It's the
hashCode()
in hexadecimal. See Object#toString()
.– 4castle
Nov 10 '16 at 21:22
That weird output is not necessarily the memory location. It's the
hashCode()
in hexadecimal. See Object#toString()
.– 4castle
Nov 10 '16 at 21:22
1
1
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28
|
show 1 more comment
30 Answers
30
active
oldest
votes
up vote
2157
down vote
accepted
Since Java 5 you can use Arrays.toString(arr)
for simple arrays or Arrays.deepToString(arr)
for nested arrays. Note that the Object
version calls .toString()
on each object in the array. The output is even decorated in the exact way you're asking.
Examples:
Simple Array:
String array = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(array));
Output:
[John, Mary, Bob]
Nested Array:
String deepArray = new String {{"John", "Mary"}, {"Alice", "Bob"}};
System.out.println(Arrays.toString(deepArray));
//output: [[Ljava.lang.String;@106d69c, [Ljava.lang.String;@52e922]
System.out.println(Arrays.deepToString(deepArray));
Output:
[[John, Mary], [Alice, Bob]]
double
Array:
double doubleArray = { 7.0, 9.0, 5.0, 1.0, 3.0 };
System.out.println(Arrays.toString(doubleArray));
Output:
[7.0, 9.0, 5.0, 1.0, 3.0 ]
int
Array:
int intArray = { 7, 9, 5, 1, 3 };
System.out.println(Arrays.toString(intArray));
Output:
[7, 9, 5, 1, 3 ]
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:34
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
FYI,Arrays.deepToString()
accepts only anObject
(or an array of classes that extendObject
, such asInteger
, so it won't work on a primitive array of typeint
. ButArrays.toString(<int array>)
works fine for primitive arrays.
– Marcus
Dec 11 '15 at 23:25
1
@Hengameh There's a method dedicated to that.System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will printJohn Mahta Sara
.
– dorukayhan
Mar 13 '17 at 14:15
|
show 1 more comment
up vote
320
down vote
Always check the standard libraries first. Try:
System.out.println(Arrays.toString(array));
or if your array contains other arrays as elements:
System.out.println(Arrays.deepToString(array));
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
@Hengameh Nowadays with Java 8:String.join(" ", Arrays.asList(array))
. doc
– Justin
Mar 4 '16 at 23:14
add a comment |
up vote
89
down vote
This is nice to know, however, as for "always check the standard libraries first" I'd never have stumbled upon the trick of Arrays.toString( myarray )
--since I was concentrating on the type of myarray to see how to do this. I didn't want to have to iterate through the thing: I wanted an easy call to make it come out similar to what I see in the Eclipse debugger and myarray.toString() just wasn't doing it.
import java.util.Arrays;
.
.
.
System.out.println( Arrays.toString( myarray ) );
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
add a comment |
up vote
73
down vote
In JDK1.8 you can use aggregate operations and a lambda expression:
String strArray = new String {"John", "Mary", "Bob"};
// #1
Arrays.asList(strArray).stream().forEach(s -> System.out.println(s));
// #2
Stream.of(strArray).forEach(System.out::println);
// #3
Arrays.stream(strArray).forEach(System.out::println);
/* output:
John
Mary
Bob
*/
43
Or less cumbersome,Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
This is clumsy. It should beSystem.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.
– Boris the Spider
Sep 20 '14 at 9:11
1
Just skip theArrays.asList
and doArrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way usingStream.of
would be to do.skip(n).limit(m)
. The current implementation does not return a SIZED stream whereasArrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have anint
, you may accidentally useStream.of
which will return aStream<int>
with a single element, whileArrays.stream
will give you anIntStream
directly.
– Alexis C.
Mar 27 '16 at 20:41
|
show 5 more comments
up vote
38
down vote
If you're using Java 1.4, you can instead do:
System.out.println(Arrays.asList(array));
(This works in 1.5+ too, of course.)
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
add a comment |
up vote
36
down vote
Starting with Java 8, one could also take advantage of the join()
method provided by the String class to print out array elements, without the brackets, and separated by a delimiter of choice (which is the space character for the example shown below):
String greeting = {"Hey", "there", "amigo!"};
String delimiter = " ";
String.join(delimiter, greeting)
The output will be "Hey there amigo!".
add a comment |
up vote
30
down vote
Arrays.toString
As a direct answer, the solution provided by several, including @Esko, using the Arrays.toString
and Arrays.deepToString
methods, is simply the best.
Java 8 - Stream.collect(joining()), Stream.forEach
Below I try to list some of the other methods suggested, attempting to improve a little, with the most notable addition being the use of the Stream.collect
operator, using a joining
Collector
, to mimic what the String.join
is doing.
int ints = new int {1, 2, 3, 4, 5};
System.out.println(IntStream.of(ints).mapToObj(Integer::toString).collect(Collectors.joining(", ")));
System.out.println(IntStream.of(ints).boxed().map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(ints));
String strs = new String {"John", "Mary", "Bob"};
System.out.println(Stream.of(strs).collect(Collectors.joining(", ")));
System.out.println(String.join(", ", strs));
System.out.println(Arrays.toString(strs));
DayOfWeek days = { FRIDAY, MONDAY, TUESDAY };
System.out.println(Stream.of(days).map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(days));
// These options are not the same as each item is printed on a new line:
IntStream.of(ints).forEach(System.out::println);
Stream.of(strs).forEach(System.out::println);
Stream.of(days).forEach(System.out::println);
add a comment |
up vote
28
down vote
Arrays.deepToString(arr)
only prints on one line.
int table = new int[2][2];
To actually get a table to print as a two dimensional table, I had to do this:
System.out.println(Arrays.deepToString(table).replaceAll("],", "]," + System.getProperty("line.separator")));
It seems like the Arrays.deepToString(arr)
method should take a separator string, but unfortunately it doesn't.
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
add a comment |
up vote
24
down vote
Prior to Java 8
We could have used Arrays.toString(array)
to print one dimensional array and Arrays.deepToString(array)
for multi-dimensional arrays.
Java 8
Now we have got the option of Stream
and lambda
to print the array.
Printing One dimensional Array:
public static void main(String args) {
int intArray = new int {1, 2, 3, 4, 5};
String strArray = new String {"John", "Mary", "Bob"};
//Prior to Java 8
System.out.println(Arrays.toString(intArray));
System.out.println(Arrays.toString(strArray));
// In Java 8 we have lambda expressions
Arrays.stream(intArray).forEach(System.out::println);
Arrays.stream(strArray).forEach(System.out::println);
}
The output is:
[1, 2, 3, 4, 5]
[John, Mary, Bob]
1
2
3
4
5
John
Mary
Bob
Printing Multi-dimensional Array
Just in case we want to print multi-dimensional array we can use Arrays.deepToString(array)
as:
public static void main(String args) {
int int2DArray = new int { {11, 12}, { 21, 22}, {31, 32, 33} };
String str2DArray = new String{ {"John", "Bravo"} , {"Mary", "Lee"}, {"Bob", "Johnson"} };
//Prior to Java 8
System.out.println(Arrays.deepToString(int2DArray));
System.out.println(Arrays.deepToString(str2DArray));
// In Java 8 we have lambda expressions
Arrays.stream(int2DArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println);
Arrays.stream(str2DArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println);
}
Now the point to observe is that the method Arrays.stream(T)
, which in case of int
returns us Stream<int>
and then method flatMapToInt()
maps each element of stream with the contents of a mapped stream produced by applying the provided mapping function to each element.
The output is:
[[11, 12], [21, 22], [31, 32, 33]]
[[John, Bravo], [Mary, Lee], [Bob, Johnson]]
11
12
21
22
31
32
33
John
Bravo
Mary
Lee
Bob
Johnson
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:33
add a comment |
up vote
18
down vote
for(int n: someArray) {
System.out.println(n+" ");
}
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.n
is the actual value from the array, not the index. For a regular for loop, it would also be(someArray.length - 1) == i
, because it breaks wheni
is equal to the array length.
– Radiodef
Aug 6 at 22:02
add a comment |
up vote
16
down vote
Different Ways to Print Arrays in Java:
Simple Way
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Print the list in console
System.out.println(list);
Output:
[One, Two, Three, Four]
Using
toString()
String array = new String { "One", "Two", "Three", "Four" };
System.out.println(Arrays.toString(array));
Output: [One, Two, Three, Four]
Printing Array of Arrays
String arr1 = new String { "Fifth", "Sixth" };
String arr2 = new String { "Seventh", "Eight" };
String arrayOfArray = new String { arr1, arr2 };
System.out.println(arrayOfArray);
System.out.println(Arrays.toString(arrayOfArray));
System.out.println(Arrays.deepToString(arrayOfArray));
Output: [[Ljava.lang.String;@1ad086a [[Ljava.lang.String;@10385c1,
[Ljava.lang.String;@42719c] [[Fifth, Sixth], [Seventh, Eighth]]
Resource: Access An Array
add a comment |
up vote
12
down vote
Using regular for loop is the simplest way of printing array in my opinion.
Here you have a sample code based on your intArray
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i] + ", ");
}
It gives output as yours
1, 2, 3, 4, 5
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
You could replace the code within the loop withSystem.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
You could also useSystem.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.
– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
add a comment |
up vote
8
down vote
I came across this post in Vanilla #Java recently. It's not very convenient writing Arrays.toString(arr);
, then importing java.util.Arrays;
all the time.
Please note, this is not a permanent fix by any means. Just a hack that can make debugging simpler.
Printing an array directly gives the internal representation and the hashCode. Now, all classes have Object
as the parent-type. So, why not hack the Object.toString()
? Without modification, the Object class looks like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
What if this is changed to:
public String toString() {
if (this instanceof boolean)
return Arrays.toString((boolean) this);
if (this instanceof byte)
return Arrays.toString((byte) this);
if (this instanceof short)
return Arrays.toString((short) this);
if (this instanceof char)
return Arrays.toString((char) this);
if (this instanceof int)
return Arrays.toString((int) this);
if (this instanceof long)
return Arrays.toString((long) this);
if (this instanceof float)
return Arrays.toString((float) this);
if (this instanceof double)
return Arrays.toString((double) this);
if (this instanceof Object)
return Arrays.deepToString((Object) this);
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
This modded class may simply be added to the class path by adding the following to the command line: -Xbootclasspath/p:target/classes
.
Now, with the availability of deepToString(..)
since Java 5, the toString(..)
can easily be changed to deepToString(..)
to add support for arrays that contain other arrays.
I found this to be a quite useful hack and it would be great if Java could simply add this. I understand potential issues with having very large arrays since the string representations could be problematic. Maybe pass something like a System.out
or a PrintWriter
for such eventualities.
2
you want to execute theseif
conditions on every object?
– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on theObject
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...
– YoYo
Sep 11 '17 at 15:52
add a comment |
up vote
7
down vote
It should always work whichever JDK version you use:
System.out.println(Arrays.asList(array));
It will work if the Array
contains Objects. If the Array
contains primitive types, you can use wrapper classes instead storing the primitive directly as..
Example:
int a = new int{1,2,3,4,5};
Replace it with:
Integer a = new Integer{1,2,3,4,5};
Update :
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing.
So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
public void printArray(int a){
//write printing code
}
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has atoString(..)
, it defeats me why someone would ever do this.
– Debosmit Ray
May 13 '16 at 11:35
add a comment |
up vote
7
down vote
In java 8 it is easy. there are two keywords
- stream:
Arrays.stream(intArray).forEach
method reference:
::println
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::println);
If you want to print all elements in the array in the same line, then just use print
instead of println
i.e.
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::print);
Another way without method reference just use:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
add a comment |
up vote
5
down vote
There's one additional way if your array is of type char:
char A = {'a', 'b', 'c'};
System.out.println(A); // no other arguments
prints
abc
add a comment |
up vote
4
down vote
To add to all the answers, printing the object as a JSON string is also an option.
Using Jackson:
ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter();
System.out.println(ow.writeValueAsString(anyArray));
Using Gson:
Gson gson = new Gson();
System.out.println(gson.toJson(anyArray));
add a comment |
up vote
4
down vote
A simplified shortcut I've tried is this:
int x = {1,2,3};
String printableText = Arrays.toString(x).replaceAll("[\[\]]", "").replaceAll(", ", "n");
System.out.println(printableText);
It will print
1
2
3
No loops required in this approach and it is best for small arrays only
add a comment |
up vote
4
down vote
You could loop through the array, printing out each item, as you loop. For example:
String items = {"item 1", "item 2", "item 3"};
for(int i = 0; i < items.length; i++) {
System.out.println(items[i]);
}
Output:
item 1
item 2
item 3
add a comment |
up vote
4
down vote
There Are Following way to print Array
// 1) toString()
int arrayInt = new int {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(arrayInt));
// 2 for loop()
for (int number : arrayInt) {
System.out.println(number);
}
// 3 for each()
for(int x: arrayInt){
System.out.println(x);
}
add a comment |
up vote
3
down vote
public class printer {
public static void main(String args) {
String a = new String[4];
Scanner sc = new Scanner(System.in);
System.out.println("enter the data");
for (int i = 0; i < 4; i++) {
a[i] = sc.nextLine();
}
System.out.println("the entered data is");
for (String i : a) {
System.out.println(i);
}
}
}
add a comment |
up vote
3
down vote
Using org.apache.commons.lang3.StringUtils.join(*) methods can be an option
For example:
String strArray = new String { "John", "Mary", "Bob" };
String arrayAsCSV = StringUtils.join(strArray, " , ");
System.out.printf("[%s]", arrayAsCSV);
//output: [John , Mary , Bob]
I used the following dependency
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
add a comment |
up vote
3
down vote
For-each loop can also be used to print elements of array:
int array = {1, 2, 3, 4, 5};
for (int i:array)
System.out.println(i);
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
add a comment |
up vote
2
down vote
This is marked as a duplicate for printing a byte. Note: for a byte array there are additional methods which may be appropriate.
You can print it as a String if it contains ISO-8859-1 chars.
String s = new String(bytes, StandardChars.ISO_8559);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.ISO_8559);
or if it contains a UTF-8 string
String s = new String(bytes, StandardChars.UTF_8);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.UTF_8);
or if you want print it as hexadecimal.
String s = DatatypeConverter.printHexBinary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseHexBinary(s);
or if you want print it as base64.
String s = DatatypeConverter.printBase64Binary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseBase64Binary(s);
or if you want to print an array of signed byte values
String s = Arrays.toString(bytes);
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = Byte.parseByte(split[i]);
or if you want to print an array of unsigned byte values
String s = Arrays.toString(
IntStream.range(0, bytes.length).map(i -> bytes[i] & 0xFF).toArray());
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = (byte) Integer.parseInt(split[i]); // might need a range check.
add a comment |
up vote
0
down vote
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray));
output: [John, Mary, Bob]
add a comment |
up vote
0
down vote
In java 8 :
Arrays.stream(myArray).forEach(System.out::println);
add a comment |
up vote
0
down vote
There are several ways to print an array elements.First of all, I'll explain that, what is an array?..Array is a simple data structure for storing data..When you define an array , Allocate set of ancillary memory blocks in RAM.Those memory blocks are taken one unit ..
Ok, I'll create an array like this,
class demo{
public static void main(String a){
int number={1,2,3,4,5};
System.out.print(number);
}
}
Now look at the output,
You can see an unknown string printed..As I mentioned before, the memory address whose array(number array) declared is printed.If you want to display elements in the array, you can use "for loop " , like this..
class demo{
public static void main(String a){
int number={1,2,3,4,5};
int i;
for(i=0;i<number.length;i++){
System.out.print(number[i]+" ");
}
}
}
Now look at the output,
Ok,Successfully printed elements of one dimension array..Now I am going to consider two dimension array..I'll declare two dimension array as "number2" and print the elements using "Arrays.deepToString()" keyword.Before using that You will have to import 'java.util.Arrays' library.
import java.util.Arrays;
class demo{
public static void main(String a){
int number2={{1,2},{3,4},{5,6}};`
System.out.print(Arrays.deepToString(number2));
}
}
consider the output,
At the same time , Using two for loops ,2D elements can be printed..Thank you !
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
add a comment |
up vote
-1
down vote
If you want to print, evaluate Array content like that you can use Arrays.toString
jshell> String names = {"ram","shyam"};
names ==> String[2] { "ram", "shyam" }
jshell> Arrays.toString(names);
$2 ==> "[ram, shyam]"
jshell>
add a comment |
up vote
-3
down vote
You could use Arrays.toString()
String array = { "a", "b", "c" };
System.out.println(Arrays.toString(array));
add a comment |
up vote
-5
down vote
The simplest way to print an array is to use a for-loop:
// initialize array
for(int i=0;i<array.length;i++)
{
System.out.print(array[i] + " ");
}
The correct for loop, assuming aT myArray
, isfor (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
add a comment |
protected by Aniket Thakur Oct 2 '15 at 18:54
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
30 Answers
30
active
oldest
votes
30 Answers
30
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2157
down vote
accepted
Since Java 5 you can use Arrays.toString(arr)
for simple arrays or Arrays.deepToString(arr)
for nested arrays. Note that the Object
version calls .toString()
on each object in the array. The output is even decorated in the exact way you're asking.
Examples:
Simple Array:
String array = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(array));
Output:
[John, Mary, Bob]
Nested Array:
String deepArray = new String {{"John", "Mary"}, {"Alice", "Bob"}};
System.out.println(Arrays.toString(deepArray));
//output: [[Ljava.lang.String;@106d69c, [Ljava.lang.String;@52e922]
System.out.println(Arrays.deepToString(deepArray));
Output:
[[John, Mary], [Alice, Bob]]
double
Array:
double doubleArray = { 7.0, 9.0, 5.0, 1.0, 3.0 };
System.out.println(Arrays.toString(doubleArray));
Output:
[7.0, 9.0, 5.0, 1.0, 3.0 ]
int
Array:
int intArray = { 7, 9, 5, 1, 3 };
System.out.println(Arrays.toString(intArray));
Output:
[7, 9, 5, 1, 3 ]
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:34
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
FYI,Arrays.deepToString()
accepts only anObject
(or an array of classes that extendObject
, such asInteger
, so it won't work on a primitive array of typeint
. ButArrays.toString(<int array>)
works fine for primitive arrays.
– Marcus
Dec 11 '15 at 23:25
1
@Hengameh There's a method dedicated to that.System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will printJohn Mahta Sara
.
– dorukayhan
Mar 13 '17 at 14:15
|
show 1 more comment
up vote
2157
down vote
accepted
Since Java 5 you can use Arrays.toString(arr)
for simple arrays or Arrays.deepToString(arr)
for nested arrays. Note that the Object
version calls .toString()
on each object in the array. The output is even decorated in the exact way you're asking.
Examples:
Simple Array:
String array = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(array));
Output:
[John, Mary, Bob]
Nested Array:
String deepArray = new String {{"John", "Mary"}, {"Alice", "Bob"}};
System.out.println(Arrays.toString(deepArray));
//output: [[Ljava.lang.String;@106d69c, [Ljava.lang.String;@52e922]
System.out.println(Arrays.deepToString(deepArray));
Output:
[[John, Mary], [Alice, Bob]]
double
Array:
double doubleArray = { 7.0, 9.0, 5.0, 1.0, 3.0 };
System.out.println(Arrays.toString(doubleArray));
Output:
[7.0, 9.0, 5.0, 1.0, 3.0 ]
int
Array:
int intArray = { 7, 9, 5, 1, 3 };
System.out.println(Arrays.toString(intArray));
Output:
[7, 9, 5, 1, 3 ]
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:34
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
FYI,Arrays.deepToString()
accepts only anObject
(or an array of classes that extendObject
, such asInteger
, so it won't work on a primitive array of typeint
. ButArrays.toString(<int array>)
works fine for primitive arrays.
– Marcus
Dec 11 '15 at 23:25
1
@Hengameh There's a method dedicated to that.System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will printJohn Mahta Sara
.
– dorukayhan
Mar 13 '17 at 14:15
|
show 1 more comment
up vote
2157
down vote
accepted
up vote
2157
down vote
accepted
Since Java 5 you can use Arrays.toString(arr)
for simple arrays or Arrays.deepToString(arr)
for nested arrays. Note that the Object
version calls .toString()
on each object in the array. The output is even decorated in the exact way you're asking.
Examples:
Simple Array:
String array = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(array));
Output:
[John, Mary, Bob]
Nested Array:
String deepArray = new String {{"John", "Mary"}, {"Alice", "Bob"}};
System.out.println(Arrays.toString(deepArray));
//output: [[Ljava.lang.String;@106d69c, [Ljava.lang.String;@52e922]
System.out.println(Arrays.deepToString(deepArray));
Output:
[[John, Mary], [Alice, Bob]]
double
Array:
double doubleArray = { 7.0, 9.0, 5.0, 1.0, 3.0 };
System.out.println(Arrays.toString(doubleArray));
Output:
[7.0, 9.0, 5.0, 1.0, 3.0 ]
int
Array:
int intArray = { 7, 9, 5, 1, 3 };
System.out.println(Arrays.toString(intArray));
Output:
[7, 9, 5, 1, 3 ]
Since Java 5 you can use Arrays.toString(arr)
for simple arrays or Arrays.deepToString(arr)
for nested arrays. Note that the Object
version calls .toString()
on each object in the array. The output is even decorated in the exact way you're asking.
Examples:
Simple Array:
String array = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(array));
Output:
[John, Mary, Bob]
Nested Array:
String deepArray = new String {{"John", "Mary"}, {"Alice", "Bob"}};
System.out.println(Arrays.toString(deepArray));
//output: [[Ljava.lang.String;@106d69c, [Ljava.lang.String;@52e922]
System.out.println(Arrays.deepToString(deepArray));
Output:
[[John, Mary], [Alice, Bob]]
double
Array:
double doubleArray = { 7.0, 9.0, 5.0, 1.0, 3.0 };
System.out.println(Arrays.toString(doubleArray));
Output:
[7.0, 9.0, 5.0, 1.0, 3.0 ]
int
Array:
int intArray = { 7, 9, 5, 1, 3 };
System.out.println(Arrays.toString(intArray));
Output:
[7, 9, 5, 1, 3 ]
edited Oct 31 at 0:13
community wiki
12 revs, 11 users 26%
RAnders00
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:34
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
FYI,Arrays.deepToString()
accepts only anObject
(or an array of classes that extendObject
, such asInteger
, so it won't work on a primitive array of typeint
. ButArrays.toString(<int array>)
works fine for primitive arrays.
– Marcus
Dec 11 '15 at 23:25
1
@Hengameh There's a method dedicated to that.System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will printJohn Mahta Sara
.
– dorukayhan
Mar 13 '17 at 14:15
|
show 1 more comment
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:34
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
FYI,Arrays.deepToString()
accepts only anObject
(or an array of classes that extendObject
, such asInteger
, so it won't work on a primitive array of typeint
. ButArrays.toString(<int array>)
works fine for primitive arrays.
– Marcus
Dec 11 '15 at 23:25
1
@Hengameh There's a method dedicated to that.System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will printJohn Mahta Sara
.
– dorukayhan
Mar 13 '17 at 14:15
1
1
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
This works for multi dimensional arrays too.
– Alok Mishra
May 27 '15 at 12:47
2
2
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:34
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:34
2
2
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
@Hengameh: There are several other ways to do this, but my favorite is this one: javahotchocolate.com/notes/java.html#arrays-tostring .
– Russ Bateman
Aug 29 '15 at 6:16
3
3
FYI,
Arrays.deepToString()
accepts only an Object
(or an array of classes that extend Object
, such as Integer
, so it won't work on a primitive array of type int
. But Arrays.toString(<int array>)
works fine for primitive arrays.– Marcus
Dec 11 '15 at 23:25
FYI,
Arrays.deepToString()
accepts only an Object
(or an array of classes that extend Object
, such as Integer
, so it won't work on a primitive array of type int
. But Arrays.toString(<int array>)
works fine for primitive arrays.– Marcus
Dec 11 '15 at 23:25
1
1
@Hengameh There's a method dedicated to that.
System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will print John Mahta Sara
.– dorukayhan
Mar 13 '17 at 14:15
@Hengameh There's a method dedicated to that.
System.out.println(String.join(" ", new String{"John", "Mahta", "Sara"}))
will print John Mahta Sara
.– dorukayhan
Mar 13 '17 at 14:15
|
show 1 more comment
up vote
320
down vote
Always check the standard libraries first. Try:
System.out.println(Arrays.toString(array));
or if your array contains other arrays as elements:
System.out.println(Arrays.deepToString(array));
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
@Hengameh Nowadays with Java 8:String.join(" ", Arrays.asList(array))
. doc
– Justin
Mar 4 '16 at 23:14
add a comment |
up vote
320
down vote
Always check the standard libraries first. Try:
System.out.println(Arrays.toString(array));
or if your array contains other arrays as elements:
System.out.println(Arrays.deepToString(array));
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
@Hengameh Nowadays with Java 8:String.join(" ", Arrays.asList(array))
. doc
– Justin
Mar 4 '16 at 23:14
add a comment |
up vote
320
down vote
up vote
320
down vote
Always check the standard libraries first. Try:
System.out.println(Arrays.toString(array));
or if your array contains other arrays as elements:
System.out.println(Arrays.deepToString(array));
Always check the standard libraries first. Try:
System.out.println(Arrays.toString(array));
or if your array contains other arrays as elements:
System.out.println(Arrays.deepToString(array));
edited Feb 13 '09 at 22:50
answered Jan 3 '09 at 20:48
Limbic System
4,87772437
4,87772437
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
@Hengameh Nowadays with Java 8:String.join(" ", Arrays.asList(array))
. doc
– Justin
Mar 4 '16 at 23:14
add a comment |
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
@Hengameh Nowadays with Java 8:String.join(" ", Arrays.asList(array))
. doc
– Justin
Mar 4 '16 at 23:14
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:35
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:35
1
1
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
@Hengameh Just store Arrays.toString(array) to a string variable and then remove the braces by replace method of Java
– Naveed Ahmad
Oct 21 '15 at 22:09
6
6
@Hengameh Nowadays with Java 8:
String.join(" ", Arrays.asList(array))
. doc– Justin
Mar 4 '16 at 23:14
@Hengameh Nowadays with Java 8:
String.join(" ", Arrays.asList(array))
. doc– Justin
Mar 4 '16 at 23:14
add a comment |
up vote
89
down vote
This is nice to know, however, as for "always check the standard libraries first" I'd never have stumbled upon the trick of Arrays.toString( myarray )
--since I was concentrating on the type of myarray to see how to do this. I didn't want to have to iterate through the thing: I wanted an easy call to make it come out similar to what I see in the Eclipse debugger and myarray.toString() just wasn't doing it.
import java.util.Arrays;
.
.
.
System.out.println( Arrays.toString( myarray ) );
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
add a comment |
up vote
89
down vote
This is nice to know, however, as for "always check the standard libraries first" I'd never have stumbled upon the trick of Arrays.toString( myarray )
--since I was concentrating on the type of myarray to see how to do this. I didn't want to have to iterate through the thing: I wanted an easy call to make it come out similar to what I see in the Eclipse debugger and myarray.toString() just wasn't doing it.
import java.util.Arrays;
.
.
.
System.out.println( Arrays.toString( myarray ) );
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
add a comment |
up vote
89
down vote
up vote
89
down vote
This is nice to know, however, as for "always check the standard libraries first" I'd never have stumbled upon the trick of Arrays.toString( myarray )
--since I was concentrating on the type of myarray to see how to do this. I didn't want to have to iterate through the thing: I wanted an easy call to make it come out similar to what I see in the Eclipse debugger and myarray.toString() just wasn't doing it.
import java.util.Arrays;
.
.
.
System.out.println( Arrays.toString( myarray ) );
This is nice to know, however, as for "always check the standard libraries first" I'd never have stumbled upon the trick of Arrays.toString( myarray )
--since I was concentrating on the type of myarray to see how to do this. I didn't want to have to iterate through the thing: I wanted an easy call to make it come out similar to what I see in the Eclipse debugger and myarray.toString() just wasn't doing it.
import java.util.Arrays;
.
.
.
System.out.println( Arrays.toString( myarray ) );
edited Nov 29 '10 at 14:05
answered May 12 '10 at 21:01
Russ Bateman
11.8k133957
11.8k133957
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
add a comment |
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:35
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:35
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:35
3
3
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
@Hengameh I think that's another topic. You can manipulate this String with the common string operations afterwards... Like Arrays.toString(myarray).replace("[", "("); and so on.
– OddDev
Jan 14 '16 at 10:42
add a comment |
up vote
73
down vote
In JDK1.8 you can use aggregate operations and a lambda expression:
String strArray = new String {"John", "Mary", "Bob"};
// #1
Arrays.asList(strArray).stream().forEach(s -> System.out.println(s));
// #2
Stream.of(strArray).forEach(System.out::println);
// #3
Arrays.stream(strArray).forEach(System.out::println);
/* output:
John
Mary
Bob
*/
43
Or less cumbersome,Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
This is clumsy. It should beSystem.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.
– Boris the Spider
Sep 20 '14 at 9:11
1
Just skip theArrays.asList
and doArrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way usingStream.of
would be to do.skip(n).limit(m)
. The current implementation does not return a SIZED stream whereasArrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have anint
, you may accidentally useStream.of
which will return aStream<int>
with a single element, whileArrays.stream
will give you anIntStream
directly.
– Alexis C.
Mar 27 '16 at 20:41
|
show 5 more comments
up vote
73
down vote
In JDK1.8 you can use aggregate operations and a lambda expression:
String strArray = new String {"John", "Mary", "Bob"};
// #1
Arrays.asList(strArray).stream().forEach(s -> System.out.println(s));
// #2
Stream.of(strArray).forEach(System.out::println);
// #3
Arrays.stream(strArray).forEach(System.out::println);
/* output:
John
Mary
Bob
*/
43
Or less cumbersome,Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
This is clumsy. It should beSystem.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.
– Boris the Spider
Sep 20 '14 at 9:11
1
Just skip theArrays.asList
and doArrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way usingStream.of
would be to do.skip(n).limit(m)
. The current implementation does not return a SIZED stream whereasArrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have anint
, you may accidentally useStream.of
which will return aStream<int>
with a single element, whileArrays.stream
will give you anIntStream
directly.
– Alexis C.
Mar 27 '16 at 20:41
|
show 5 more comments
up vote
73
down vote
up vote
73
down vote
In JDK1.8 you can use aggregate operations and a lambda expression:
String strArray = new String {"John", "Mary", "Bob"};
// #1
Arrays.asList(strArray).stream().forEach(s -> System.out.println(s));
// #2
Stream.of(strArray).forEach(System.out::println);
// #3
Arrays.stream(strArray).forEach(System.out::println);
/* output:
John
Mary
Bob
*/
In JDK1.8 you can use aggregate operations and a lambda expression:
String strArray = new String {"John", "Mary", "Bob"};
// #1
Arrays.asList(strArray).stream().forEach(s -> System.out.println(s));
// #2
Stream.of(strArray).forEach(System.out::println);
// #3
Arrays.stream(strArray).forEach(System.out::println);
/* output:
John
Mary
Bob
*/
edited Mar 27 '16 at 20:22


Yassin Hajaj
13.2k62558
13.2k62558
answered Feb 6 '14 at 23:35


Eric Baker
74752
74752
43
Or less cumbersome,Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
This is clumsy. It should beSystem.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.
– Boris the Spider
Sep 20 '14 at 9:11
1
Just skip theArrays.asList
and doArrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way usingStream.of
would be to do.skip(n).limit(m)
. The current implementation does not return a SIZED stream whereasArrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have anint
, you may accidentally useStream.of
which will return aStream<int>
with a single element, whileArrays.stream
will give you anIntStream
directly.
– Alexis C.
Mar 27 '16 at 20:41
|
show 5 more comments
43
Or less cumbersome,Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
This is clumsy. It should beSystem.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.
– Boris the Spider
Sep 20 '14 at 9:11
1
Just skip theArrays.asList
and doArrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way usingStream.of
would be to do.skip(n).limit(m)
. The current implementation does not return a SIZED stream whereasArrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have anint
, you may accidentally useStream.of
which will return aStream<int>
with a single element, whileArrays.stream
will give you anIntStream
directly.
– Alexis C.
Mar 27 '16 at 20:41
43
43
Or less cumbersome,
Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
Or less cumbersome,
Arrays.stream(strArray).forEach(System.out::println);
– Alexis C.
May 22 '14 at 22:01
7
7
This is clumsy. It should be
System.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.– Boris the Spider
Sep 20 '14 at 9:11
This is clumsy. It should be
System.out::println
which is a Java 8 method reference. You code produces an unnecessary lambda.– Boris the Spider
Sep 20 '14 at 9:11
1
1
Just skip the
Arrays.asList
and do Arrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
Just skip the
Arrays.asList
and do Arrays.stream(strArray).forEach(System.out::println)
– Justin
Mar 4 '16 at 23:16
1
1
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
@AlexisC. Because it can also be used with other objects than arrays.
– Yassin Hajaj
Mar 27 '16 at 20:27
1
1
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way using
Stream.of
would be to do .skip(n).limit(m)
. The current implementation does not return a SIZED stream whereas Arrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have an int
, you may accidentally use Stream.of
which will return a Stream<int>
with a single element, while Arrays.stream
will give you an IntStream
directly.– Alexis C.
Mar 27 '16 at 20:41
@YassinHajaj Both. For instance if you want to have a range stream over the array the idiomatic way using
Stream.of
would be to do .skip(n).limit(m)
. The current implementation does not return a SIZED stream whereas Arrays.stream(T, int, int)
does, leading to better splitting performances if you want to perform operations in parallel. Also if you have an int
, you may accidentally use Stream.of
which will return a Stream<int>
with a single element, while Arrays.stream
will give you an IntStream
directly.– Alexis C.
Mar 27 '16 at 20:41
|
show 5 more comments
up vote
38
down vote
If you're using Java 1.4, you can instead do:
System.out.println(Arrays.asList(array));
(This works in 1.5+ too, of course.)
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
add a comment |
up vote
38
down vote
If you're using Java 1.4, you can instead do:
System.out.println(Arrays.asList(array));
(This works in 1.5+ too, of course.)
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
add a comment |
up vote
38
down vote
up vote
38
down vote
If you're using Java 1.4, you can instead do:
System.out.println(Arrays.asList(array));
(This works in 1.5+ too, of course.)
If you're using Java 1.4, you can instead do:
System.out.println(Arrays.asList(array));
(This works in 1.5+ too, of course.)
answered Jan 3 '09 at 21:44
Ross
7,08563134
7,08563134
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
add a comment |
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
33
33
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
Unfortunately this only works with arrays of objects, not arrays of primitives.
– Alex Spurling
Jan 3 '09 at 21:57
add a comment |
up vote
36
down vote
Starting with Java 8, one could also take advantage of the join()
method provided by the String class to print out array elements, without the brackets, and separated by a delimiter of choice (which is the space character for the example shown below):
String greeting = {"Hey", "there", "amigo!"};
String delimiter = " ";
String.join(delimiter, greeting)
The output will be "Hey there amigo!".
add a comment |
up vote
36
down vote
Starting with Java 8, one could also take advantage of the join()
method provided by the String class to print out array elements, without the brackets, and separated by a delimiter of choice (which is the space character for the example shown below):
String greeting = {"Hey", "there", "amigo!"};
String delimiter = " ";
String.join(delimiter, greeting)
The output will be "Hey there amigo!".
add a comment |
up vote
36
down vote
up vote
36
down vote
Starting with Java 8, one could also take advantage of the join()
method provided by the String class to print out array elements, without the brackets, and separated by a delimiter of choice (which is the space character for the example shown below):
String greeting = {"Hey", "there", "amigo!"};
String delimiter = " ";
String.join(delimiter, greeting)
The output will be "Hey there amigo!".
Starting with Java 8, one could also take advantage of the join()
method provided by the String class to print out array elements, without the brackets, and separated by a delimiter of choice (which is the space character for the example shown below):
String greeting = {"Hey", "there", "amigo!"};
String delimiter = " ";
String.join(delimiter, greeting)
The output will be "Hey there amigo!".
answered Dec 23 '15 at 18:51
laylaylom
670811
670811
add a comment |
add a comment |
up vote
30
down vote
Arrays.toString
As a direct answer, the solution provided by several, including @Esko, using the Arrays.toString
and Arrays.deepToString
methods, is simply the best.
Java 8 - Stream.collect(joining()), Stream.forEach
Below I try to list some of the other methods suggested, attempting to improve a little, with the most notable addition being the use of the Stream.collect
operator, using a joining
Collector
, to mimic what the String.join
is doing.
int ints = new int {1, 2, 3, 4, 5};
System.out.println(IntStream.of(ints).mapToObj(Integer::toString).collect(Collectors.joining(", ")));
System.out.println(IntStream.of(ints).boxed().map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(ints));
String strs = new String {"John", "Mary", "Bob"};
System.out.println(Stream.of(strs).collect(Collectors.joining(", ")));
System.out.println(String.join(", ", strs));
System.out.println(Arrays.toString(strs));
DayOfWeek days = { FRIDAY, MONDAY, TUESDAY };
System.out.println(Stream.of(days).map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(days));
// These options are not the same as each item is printed on a new line:
IntStream.of(ints).forEach(System.out::println);
Stream.of(strs).forEach(System.out::println);
Stream.of(days).forEach(System.out::println);
add a comment |
up vote
30
down vote
Arrays.toString
As a direct answer, the solution provided by several, including @Esko, using the Arrays.toString
and Arrays.deepToString
methods, is simply the best.
Java 8 - Stream.collect(joining()), Stream.forEach
Below I try to list some of the other methods suggested, attempting to improve a little, with the most notable addition being the use of the Stream.collect
operator, using a joining
Collector
, to mimic what the String.join
is doing.
int ints = new int {1, 2, 3, 4, 5};
System.out.println(IntStream.of(ints).mapToObj(Integer::toString).collect(Collectors.joining(", ")));
System.out.println(IntStream.of(ints).boxed().map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(ints));
String strs = new String {"John", "Mary", "Bob"};
System.out.println(Stream.of(strs).collect(Collectors.joining(", ")));
System.out.println(String.join(", ", strs));
System.out.println(Arrays.toString(strs));
DayOfWeek days = { FRIDAY, MONDAY, TUESDAY };
System.out.println(Stream.of(days).map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(days));
// These options are not the same as each item is printed on a new line:
IntStream.of(ints).forEach(System.out::println);
Stream.of(strs).forEach(System.out::println);
Stream.of(days).forEach(System.out::println);
add a comment |
up vote
30
down vote
up vote
30
down vote
Arrays.toString
As a direct answer, the solution provided by several, including @Esko, using the Arrays.toString
and Arrays.deepToString
methods, is simply the best.
Java 8 - Stream.collect(joining()), Stream.forEach
Below I try to list some of the other methods suggested, attempting to improve a little, with the most notable addition being the use of the Stream.collect
operator, using a joining
Collector
, to mimic what the String.join
is doing.
int ints = new int {1, 2, 3, 4, 5};
System.out.println(IntStream.of(ints).mapToObj(Integer::toString).collect(Collectors.joining(", ")));
System.out.println(IntStream.of(ints).boxed().map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(ints));
String strs = new String {"John", "Mary", "Bob"};
System.out.println(Stream.of(strs).collect(Collectors.joining(", ")));
System.out.println(String.join(", ", strs));
System.out.println(Arrays.toString(strs));
DayOfWeek days = { FRIDAY, MONDAY, TUESDAY };
System.out.println(Stream.of(days).map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(days));
// These options are not the same as each item is printed on a new line:
IntStream.of(ints).forEach(System.out::println);
Stream.of(strs).forEach(System.out::println);
Stream.of(days).forEach(System.out::println);
Arrays.toString
As a direct answer, the solution provided by several, including @Esko, using the Arrays.toString
and Arrays.deepToString
methods, is simply the best.
Java 8 - Stream.collect(joining()), Stream.forEach
Below I try to list some of the other methods suggested, attempting to improve a little, with the most notable addition being the use of the Stream.collect
operator, using a joining
Collector
, to mimic what the String.join
is doing.
int ints = new int {1, 2, 3, 4, 5};
System.out.println(IntStream.of(ints).mapToObj(Integer::toString).collect(Collectors.joining(", ")));
System.out.println(IntStream.of(ints).boxed().map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(ints));
String strs = new String {"John", "Mary", "Bob"};
System.out.println(Stream.of(strs).collect(Collectors.joining(", ")));
System.out.println(String.join(", ", strs));
System.out.println(Arrays.toString(strs));
DayOfWeek days = { FRIDAY, MONDAY, TUESDAY };
System.out.println(Stream.of(days).map(Object::toString).collect(Collectors.joining(", ")));
System.out.println(Arrays.toString(days));
// These options are not the same as each item is printed on a new line:
IntStream.of(ints).forEach(System.out::println);
Stream.of(strs).forEach(System.out::println);
Stream.of(days).forEach(System.out::println);
edited Nov 13 at 18:05
answered Mar 11 '16 at 5:36


YoYo
4,80523656
4,80523656
add a comment |
add a comment |
up vote
28
down vote
Arrays.deepToString(arr)
only prints on one line.
int table = new int[2][2];
To actually get a table to print as a two dimensional table, I had to do this:
System.out.println(Arrays.deepToString(table).replaceAll("],", "]," + System.getProperty("line.separator")));
It seems like the Arrays.deepToString(arr)
method should take a separator string, but unfortunately it doesn't.
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
add a comment |
up vote
28
down vote
Arrays.deepToString(arr)
only prints on one line.
int table = new int[2][2];
To actually get a table to print as a two dimensional table, I had to do this:
System.out.println(Arrays.deepToString(table).replaceAll("],", "]," + System.getProperty("line.separator")));
It seems like the Arrays.deepToString(arr)
method should take a separator string, but unfortunately it doesn't.
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
add a comment |
up vote
28
down vote
up vote
28
down vote
Arrays.deepToString(arr)
only prints on one line.
int table = new int[2][2];
To actually get a table to print as a two dimensional table, I had to do this:
System.out.println(Arrays.deepToString(table).replaceAll("],", "]," + System.getProperty("line.separator")));
It seems like the Arrays.deepToString(arr)
method should take a separator string, but unfortunately it doesn't.
Arrays.deepToString(arr)
only prints on one line.
int table = new int[2][2];
To actually get a table to print as a two dimensional table, I had to do this:
System.out.println(Arrays.deepToString(table).replaceAll("],", "]," + System.getProperty("line.separator")));
It seems like the Arrays.deepToString(arr)
method should take a separator string, but unfortunately it doesn't.
edited May 14 '15 at 23:40
answered Oct 5 '13 at 19:13
Rhyous
4,71412941
4,71412941
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
add a comment |
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
2
2
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
Maybe use System.getProperty("line.separator"); instead of rn so it is right for non-Windows as well.
– Scooter
Dec 21 '13 at 22:01
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
you can use System.lineSeparator() now
– Novaterata
Apr 7 '17 at 17:20
add a comment |
up vote
24
down vote
Prior to Java 8
We could have used Arrays.toString(array)
to print one dimensional array and Arrays.deepToString(array)
for multi-dimensional arrays.
Java 8
Now we have got the option of Stream
and lambda
to print the array.
Printing One dimensional Array:
public static void main(String args) {
int intArray = new int {1, 2, 3, 4, 5};
String strArray = new String {"John", "Mary", "Bob"};
//Prior to Java 8
System.out.println(Arrays.toString(intArray));
System.out.println(Arrays.toString(strArray));
// In Java 8 we have lambda expressions
Arrays.stream(intArray).forEach(System.out::println);
Arrays.stream(strArray).forEach(System.out::println);
}
The output is:
[1, 2, 3, 4, 5]
[John, Mary, Bob]
1
2
3
4
5
John
Mary
Bob
Printing Multi-dimensional Array
Just in case we want to print multi-dimensional array we can use Arrays.deepToString(array)
as:
public static void main(String args) {
int int2DArray = new int { {11, 12}, { 21, 22}, {31, 32, 33} };
String str2DArray = new String{ {"John", "Bravo"} , {"Mary", "Lee"}, {"Bob", "Johnson"} };
//Prior to Java 8
System.out.println(Arrays.deepToString(int2DArray));
System.out.println(Arrays.deepToString(str2DArray));
// In Java 8 we have lambda expressions
Arrays.stream(int2DArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println);
Arrays.stream(str2DArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println);
}
Now the point to observe is that the method Arrays.stream(T)
, which in case of int
returns us Stream<int>
and then method flatMapToInt()
maps each element of stream with the contents of a mapped stream produced by applying the provided mapping function to each element.
The output is:
[[11, 12], [21, 22], [31, 32, 33]]
[[John, Bravo], [Mary, Lee], [Bob, Johnson]]
11
12
21
22
31
32
33
John
Bravo
Mary
Lee
Bob
Johnson
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:33
add a comment |
up vote
24
down vote
Prior to Java 8
We could have used Arrays.toString(array)
to print one dimensional array and Arrays.deepToString(array)
for multi-dimensional arrays.
Java 8
Now we have got the option of Stream
and lambda
to print the array.
Printing One dimensional Array:
public static void main(String args) {
int intArray = new int {1, 2, 3, 4, 5};
String strArray = new String {"John", "Mary", "Bob"};
//Prior to Java 8
System.out.println(Arrays.toString(intArray));
System.out.println(Arrays.toString(strArray));
// In Java 8 we have lambda expressions
Arrays.stream(intArray).forEach(System.out::println);
Arrays.stream(strArray).forEach(System.out::println);
}
The output is:
[1, 2, 3, 4, 5]
[John, Mary, Bob]
1
2
3
4
5
John
Mary
Bob
Printing Multi-dimensional Array
Just in case we want to print multi-dimensional array we can use Arrays.deepToString(array)
as:
public static void main(String args) {
int int2DArray = new int { {11, 12}, { 21, 22}, {31, 32, 33} };
String str2DArray = new String{ {"John", "Bravo"} , {"Mary", "Lee"}, {"Bob", "Johnson"} };
//Prior to Java 8
System.out.println(Arrays.deepToString(int2DArray));
System.out.println(Arrays.deepToString(str2DArray));
// In Java 8 we have lambda expressions
Arrays.stream(int2DArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println);
Arrays.stream(str2DArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println);
}
Now the point to observe is that the method Arrays.stream(T)
, which in case of int
returns us Stream<int>
and then method flatMapToInt()
maps each element of stream with the contents of a mapped stream produced by applying the provided mapping function to each element.
The output is:
[[11, 12], [21, 22], [31, 32, 33]]
[[John, Bravo], [Mary, Lee], [Bob, Johnson]]
11
12
21
22
31
32
33
John
Bravo
Mary
Lee
Bob
Johnson
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:33
add a comment |
up vote
24
down vote
up vote
24
down vote
Prior to Java 8
We could have used Arrays.toString(array)
to print one dimensional array and Arrays.deepToString(array)
for multi-dimensional arrays.
Java 8
Now we have got the option of Stream
and lambda
to print the array.
Printing One dimensional Array:
public static void main(String args) {
int intArray = new int {1, 2, 3, 4, 5};
String strArray = new String {"John", "Mary", "Bob"};
//Prior to Java 8
System.out.println(Arrays.toString(intArray));
System.out.println(Arrays.toString(strArray));
// In Java 8 we have lambda expressions
Arrays.stream(intArray).forEach(System.out::println);
Arrays.stream(strArray).forEach(System.out::println);
}
The output is:
[1, 2, 3, 4, 5]
[John, Mary, Bob]
1
2
3
4
5
John
Mary
Bob
Printing Multi-dimensional Array
Just in case we want to print multi-dimensional array we can use Arrays.deepToString(array)
as:
public static void main(String args) {
int int2DArray = new int { {11, 12}, { 21, 22}, {31, 32, 33} };
String str2DArray = new String{ {"John", "Bravo"} , {"Mary", "Lee"}, {"Bob", "Johnson"} };
//Prior to Java 8
System.out.println(Arrays.deepToString(int2DArray));
System.out.println(Arrays.deepToString(str2DArray));
// In Java 8 we have lambda expressions
Arrays.stream(int2DArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println);
Arrays.stream(str2DArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println);
}
Now the point to observe is that the method Arrays.stream(T)
, which in case of int
returns us Stream<int>
and then method flatMapToInt()
maps each element of stream with the contents of a mapped stream produced by applying the provided mapping function to each element.
The output is:
[[11, 12], [21, 22], [31, 32, 33]]
[[John, Bravo], [Mary, Lee], [Bob, Johnson]]
11
12
21
22
31
32
33
John
Bravo
Mary
Lee
Bob
Johnson
Prior to Java 8
We could have used Arrays.toString(array)
to print one dimensional array and Arrays.deepToString(array)
for multi-dimensional arrays.
Java 8
Now we have got the option of Stream
and lambda
to print the array.
Printing One dimensional Array:
public static void main(String args) {
int intArray = new int {1, 2, 3, 4, 5};
String strArray = new String {"John", "Mary", "Bob"};
//Prior to Java 8
System.out.println(Arrays.toString(intArray));
System.out.println(Arrays.toString(strArray));
// In Java 8 we have lambda expressions
Arrays.stream(intArray).forEach(System.out::println);
Arrays.stream(strArray).forEach(System.out::println);
}
The output is:
[1, 2, 3, 4, 5]
[John, Mary, Bob]
1
2
3
4
5
John
Mary
Bob
Printing Multi-dimensional Array
Just in case we want to print multi-dimensional array we can use Arrays.deepToString(array)
as:
public static void main(String args) {
int int2DArray = new int { {11, 12}, { 21, 22}, {31, 32, 33} };
String str2DArray = new String{ {"John", "Bravo"} , {"Mary", "Lee"}, {"Bob", "Johnson"} };
//Prior to Java 8
System.out.println(Arrays.deepToString(int2DArray));
System.out.println(Arrays.deepToString(str2DArray));
// In Java 8 we have lambda expressions
Arrays.stream(int2DArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println);
Arrays.stream(str2DArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println);
}
Now the point to observe is that the method Arrays.stream(T)
, which in case of int
returns us Stream<int>
and then method flatMapToInt()
maps each element of stream with the contents of a mapped stream produced by applying the provided mapping function to each element.
The output is:
[[11, 12], [21, 22], [31, 32, 33]]
[[John, Bravo], [Mary, Lee], [Bob, Johnson]]
11
12
21
22
31
32
33
John
Bravo
Mary
Lee
Bob
Johnson
edited Nov 14 at 6:09
answered Jun 19 '15 at 6:10


i_am_zero
11k25253
11k25253
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:33
add a comment |
What if we have an array of strings, and want simple output; like:String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas:John Mahta Sara
?
– Hengameh
Aug 29 '15 at 2:33
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:33
What if we have an array of strings, and want simple output; like:
String array = {"John", "Mahta", "Sara"}
, and we want this output without bracket and commas: John Mahta Sara
?– Hengameh
Aug 29 '15 at 2:33
add a comment |
up vote
18
down vote
for(int n: someArray) {
System.out.println(n+" ");
}
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.n
is the actual value from the array, not the index. For a regular for loop, it would also be(someArray.length - 1) == i
, because it breaks wheni
is equal to the array length.
– Radiodef
Aug 6 at 22:02
add a comment |
up vote
18
down vote
for(int n: someArray) {
System.out.println(n+" ");
}
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.n
is the actual value from the array, not the index. For a regular for loop, it would also be(someArray.length - 1) == i
, because it breaks wheni
is equal to the array length.
– Radiodef
Aug 6 at 22:02
add a comment |
up vote
18
down vote
up vote
18
down vote
for(int n: someArray) {
System.out.println(n+" ");
}
for(int n: someArray) {
System.out.println(n+" ");
}
edited Oct 29 '14 at 10:24


Ashish Aggarwal
2,63311640
2,63311640
answered Jan 24 '11 at 4:25
somedude
23922
23922
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.n
is the actual value from the array, not the index. For a regular for loop, it would also be(someArray.length - 1) == i
, because it breaks wheni
is equal to the array length.
– Radiodef
Aug 6 at 22:02
add a comment |
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.n
is the actual value from the array, not the index. For a regular for loop, it would also be(someArray.length - 1) == i
, because it breaks wheni
is equal to the array length.
– Radiodef
Aug 6 at 22:02
5
5
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
This way you end up with an empty space ;)
– Matthias
Sep 21 '14 at 9:23
1
1
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
@ matthiad .. this line will avoid ending up with empty space System.out.println(n+ (someArray.length == n) ? "" : " ");
– Muhammad Suleman
Jun 1 '15 at 12:29
3
3
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
Worst way of doing it.
– NameNotFoundException
Jul 8 '15 at 12:56
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
This is so inefficient but good for beginners.
– Raymo111
Apr 8 at 23:23
@MuhammadSuleman That doesn't work, because this is a for-each loop.
n
is the actual value from the array, not the index. For a regular for loop, it would also be (someArray.length - 1) == i
, because it breaks when i
is equal to the array length.– Radiodef
Aug 6 at 22:02
@MuhammadSuleman That doesn't work, because this is a for-each loop.
n
is the actual value from the array, not the index. For a regular for loop, it would also be (someArray.length - 1) == i
, because it breaks when i
is equal to the array length.– Radiodef
Aug 6 at 22:02
add a comment |
up vote
16
down vote
Different Ways to Print Arrays in Java:
Simple Way
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Print the list in console
System.out.println(list);
Output:
[One, Two, Three, Four]
Using
toString()
String array = new String { "One", "Two", "Three", "Four" };
System.out.println(Arrays.toString(array));
Output: [One, Two, Three, Four]
Printing Array of Arrays
String arr1 = new String { "Fifth", "Sixth" };
String arr2 = new String { "Seventh", "Eight" };
String arrayOfArray = new String { arr1, arr2 };
System.out.println(arrayOfArray);
System.out.println(Arrays.toString(arrayOfArray));
System.out.println(Arrays.deepToString(arrayOfArray));
Output: [[Ljava.lang.String;@1ad086a [[Ljava.lang.String;@10385c1,
[Ljava.lang.String;@42719c] [[Fifth, Sixth], [Seventh, Eighth]]
Resource: Access An Array
add a comment |
up vote
16
down vote
Different Ways to Print Arrays in Java:
Simple Way
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Print the list in console
System.out.println(list);
Output:
[One, Two, Three, Four]
Using
toString()
String array = new String { "One", "Two", "Three", "Four" };
System.out.println(Arrays.toString(array));
Output: [One, Two, Three, Four]
Printing Array of Arrays
String arr1 = new String { "Fifth", "Sixth" };
String arr2 = new String { "Seventh", "Eight" };
String arrayOfArray = new String { arr1, arr2 };
System.out.println(arrayOfArray);
System.out.println(Arrays.toString(arrayOfArray));
System.out.println(Arrays.deepToString(arrayOfArray));
Output: [[Ljava.lang.String;@1ad086a [[Ljava.lang.String;@10385c1,
[Ljava.lang.String;@42719c] [[Fifth, Sixth], [Seventh, Eighth]]
Resource: Access An Array
add a comment |
up vote
16
down vote
up vote
16
down vote
Different Ways to Print Arrays in Java:
Simple Way
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Print the list in console
System.out.println(list);
Output:
[One, Two, Three, Four]
Using
toString()
String array = new String { "One", "Two", "Three", "Four" };
System.out.println(Arrays.toString(array));
Output: [One, Two, Three, Four]
Printing Array of Arrays
String arr1 = new String { "Fifth", "Sixth" };
String arr2 = new String { "Seventh", "Eight" };
String arrayOfArray = new String { arr1, arr2 };
System.out.println(arrayOfArray);
System.out.println(Arrays.toString(arrayOfArray));
System.out.println(Arrays.deepToString(arrayOfArray));
Output: [[Ljava.lang.String;@1ad086a [[Ljava.lang.String;@10385c1,
[Ljava.lang.String;@42719c] [[Fifth, Sixth], [Seventh, Eighth]]
Resource: Access An Array
Different Ways to Print Arrays in Java:
Simple Way
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Print the list in console
System.out.println(list);
Output:
[One, Two, Three, Four]
Using
toString()
String array = new String { "One", "Two", "Three", "Four" };
System.out.println(Arrays.toString(array));
Output: [One, Two, Three, Four]
Printing Array of Arrays
String arr1 = new String { "Fifth", "Sixth" };
String arr2 = new String { "Seventh", "Eight" };
String arrayOfArray = new String { arr1, arr2 };
System.out.println(arrayOfArray);
System.out.println(Arrays.toString(arrayOfArray));
System.out.println(Arrays.deepToString(arrayOfArray));
Output: [[Ljava.lang.String;@1ad086a [[Ljava.lang.String;@10385c1,
[Ljava.lang.String;@42719c] [[Fifth, Sixth], [Seventh, Eighth]]
Resource: Access An Array
edited Nov 15 '16 at 17:05
Qwertiy
6,95742159
6,95742159
answered Aug 7 '16 at 14:05
Virtual
1,4361722
1,4361722
add a comment |
add a comment |
up vote
12
down vote
Using regular for loop is the simplest way of printing array in my opinion.
Here you have a sample code based on your intArray
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i] + ", ");
}
It gives output as yours
1, 2, 3, 4, 5
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
You could replace the code within the loop withSystem.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
You could also useSystem.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.
– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
add a comment |
up vote
12
down vote
Using regular for loop is the simplest way of printing array in my opinion.
Here you have a sample code based on your intArray
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i] + ", ");
}
It gives output as yours
1, 2, 3, 4, 5
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
You could replace the code within the loop withSystem.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
You could also useSystem.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.
– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
add a comment |
up vote
12
down vote
up vote
12
down vote
Using regular for loop is the simplest way of printing array in my opinion.
Here you have a sample code based on your intArray
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i] + ", ");
}
It gives output as yours
1, 2, 3, 4, 5
Using regular for loop is the simplest way of printing array in my opinion.
Here you have a sample code based on your intArray
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i] + ", ");
}
It gives output as yours
1, 2, 3, 4, 5
answered Dec 27 '13 at 23:31


Andrew_Dublin
655519
655519
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
You could replace the code within the loop withSystem.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
You could also useSystem.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.
– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
add a comment |
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
You could replace the code within the loop withSystem.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
You could also useSystem.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.
– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
4
4
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
It prints "1, 2, 3, 4, 5, " as output, it prints comma after the last element too.
– icza
Mar 10 '14 at 11:32
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
What's the solution for not having a comma after the last element?
– Mona Jalal
Jun 16 '14 at 1:48
2
2
You could replace the code within the loop with
System.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
You could replace the code within the loop with
System.out.print(intArray[i]); if(i != intArray.length - 1) System.out.print(", ");
– Nepoxx
Jul 16 '14 at 17:39
1
1
You could also use
System.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.– Nick Suwyn
Jan 11 '16 at 18:55
You could also use
System.out.print(i + (i < intArray.length - 1 ? ", " : ""));
to combine those two lines.– Nick Suwyn
Jan 11 '16 at 18:55
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
You could use a StringBuilder and truncate the trailing comma. int intArray = new int {1, 2, 3, 4, 5}; final StringBuilder sb = new StringBuilder(); for (int i : intArray) { sb.append(intArray[i]).append(", "); } if (sb.length() > 0) { sb.setLength(sb.length()-1); } System.out.println(sb.toString()); This outputs "1, 2, 3, 4, 5".
– Rick Ryker
Dec 27 '16 at 23:00
add a comment |
up vote
8
down vote
I came across this post in Vanilla #Java recently. It's not very convenient writing Arrays.toString(arr);
, then importing java.util.Arrays;
all the time.
Please note, this is not a permanent fix by any means. Just a hack that can make debugging simpler.
Printing an array directly gives the internal representation and the hashCode. Now, all classes have Object
as the parent-type. So, why not hack the Object.toString()
? Without modification, the Object class looks like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
What if this is changed to:
public String toString() {
if (this instanceof boolean)
return Arrays.toString((boolean) this);
if (this instanceof byte)
return Arrays.toString((byte) this);
if (this instanceof short)
return Arrays.toString((short) this);
if (this instanceof char)
return Arrays.toString((char) this);
if (this instanceof int)
return Arrays.toString((int) this);
if (this instanceof long)
return Arrays.toString((long) this);
if (this instanceof float)
return Arrays.toString((float) this);
if (this instanceof double)
return Arrays.toString((double) this);
if (this instanceof Object)
return Arrays.deepToString((Object) this);
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
This modded class may simply be added to the class path by adding the following to the command line: -Xbootclasspath/p:target/classes
.
Now, with the availability of deepToString(..)
since Java 5, the toString(..)
can easily be changed to deepToString(..)
to add support for arrays that contain other arrays.
I found this to be a quite useful hack and it would be great if Java could simply add this. I understand potential issues with having very large arrays since the string representations could be problematic. Maybe pass something like a System.out
or a PrintWriter
for such eventualities.
2
you want to execute theseif
conditions on every object?
– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on theObject
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...
– YoYo
Sep 11 '17 at 15:52
add a comment |
up vote
8
down vote
I came across this post in Vanilla #Java recently. It's not very convenient writing Arrays.toString(arr);
, then importing java.util.Arrays;
all the time.
Please note, this is not a permanent fix by any means. Just a hack that can make debugging simpler.
Printing an array directly gives the internal representation and the hashCode. Now, all classes have Object
as the parent-type. So, why not hack the Object.toString()
? Without modification, the Object class looks like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
What if this is changed to:
public String toString() {
if (this instanceof boolean)
return Arrays.toString((boolean) this);
if (this instanceof byte)
return Arrays.toString((byte) this);
if (this instanceof short)
return Arrays.toString((short) this);
if (this instanceof char)
return Arrays.toString((char) this);
if (this instanceof int)
return Arrays.toString((int) this);
if (this instanceof long)
return Arrays.toString((long) this);
if (this instanceof float)
return Arrays.toString((float) this);
if (this instanceof double)
return Arrays.toString((double) this);
if (this instanceof Object)
return Arrays.deepToString((Object) this);
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
This modded class may simply be added to the class path by adding the following to the command line: -Xbootclasspath/p:target/classes
.
Now, with the availability of deepToString(..)
since Java 5, the toString(..)
can easily be changed to deepToString(..)
to add support for arrays that contain other arrays.
I found this to be a quite useful hack and it would be great if Java could simply add this. I understand potential issues with having very large arrays since the string representations could be problematic. Maybe pass something like a System.out
or a PrintWriter
for such eventualities.
2
you want to execute theseif
conditions on every object?
– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on theObject
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...
– YoYo
Sep 11 '17 at 15:52
add a comment |
up vote
8
down vote
up vote
8
down vote
I came across this post in Vanilla #Java recently. It's not very convenient writing Arrays.toString(arr);
, then importing java.util.Arrays;
all the time.
Please note, this is not a permanent fix by any means. Just a hack that can make debugging simpler.
Printing an array directly gives the internal representation and the hashCode. Now, all classes have Object
as the parent-type. So, why not hack the Object.toString()
? Without modification, the Object class looks like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
What if this is changed to:
public String toString() {
if (this instanceof boolean)
return Arrays.toString((boolean) this);
if (this instanceof byte)
return Arrays.toString((byte) this);
if (this instanceof short)
return Arrays.toString((short) this);
if (this instanceof char)
return Arrays.toString((char) this);
if (this instanceof int)
return Arrays.toString((int) this);
if (this instanceof long)
return Arrays.toString((long) this);
if (this instanceof float)
return Arrays.toString((float) this);
if (this instanceof double)
return Arrays.toString((double) this);
if (this instanceof Object)
return Arrays.deepToString((Object) this);
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
This modded class may simply be added to the class path by adding the following to the command line: -Xbootclasspath/p:target/classes
.
Now, with the availability of deepToString(..)
since Java 5, the toString(..)
can easily be changed to deepToString(..)
to add support for arrays that contain other arrays.
I found this to be a quite useful hack and it would be great if Java could simply add this. I understand potential issues with having very large arrays since the string representations could be problematic. Maybe pass something like a System.out
or a PrintWriter
for such eventualities.
I came across this post in Vanilla #Java recently. It's not very convenient writing Arrays.toString(arr);
, then importing java.util.Arrays;
all the time.
Please note, this is not a permanent fix by any means. Just a hack that can make debugging simpler.
Printing an array directly gives the internal representation and the hashCode. Now, all classes have Object
as the parent-type. So, why not hack the Object.toString()
? Without modification, the Object class looks like this:
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
What if this is changed to:
public String toString() {
if (this instanceof boolean)
return Arrays.toString((boolean) this);
if (this instanceof byte)
return Arrays.toString((byte) this);
if (this instanceof short)
return Arrays.toString((short) this);
if (this instanceof char)
return Arrays.toString((char) this);
if (this instanceof int)
return Arrays.toString((int) this);
if (this instanceof long)
return Arrays.toString((long) this);
if (this instanceof float)
return Arrays.toString((float) this);
if (this instanceof double)
return Arrays.toString((double) this);
if (this instanceof Object)
return Arrays.deepToString((Object) this);
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
This modded class may simply be added to the class path by adding the following to the command line: -Xbootclasspath/p:target/classes
.
Now, with the availability of deepToString(..)
since Java 5, the toString(..)
can easily be changed to deepToString(..)
to add support for arrays that contain other arrays.
I found this to be a quite useful hack and it would be great if Java could simply add this. I understand potential issues with having very large arrays since the string representations could be problematic. Maybe pass something like a System.out
or a PrintWriter
for such eventualities.
answered Mar 11 '16 at 11:50


Debosmit Ray
3,48621837
3,48621837
2
you want to execute theseif
conditions on every object?
– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on theObject
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...
– YoYo
Sep 11 '17 at 15:52
add a comment |
2
you want to execute theseif
conditions on every object?
– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on theObject
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...
– YoYo
Sep 11 '17 at 15:52
2
2
you want to execute these
if
conditions on every object?– sidgate
Aug 10 '16 at 11:48
you want to execute these
if
conditions on every object?– sidgate
Aug 10 '16 at 11:48
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on the
Object
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...– YoYo
Sep 11 '17 at 15:52
+1 just for the idea, but based on previous comment, can we mod the array implementations directly rather than depending on the
Object
common parent? Can we work that idea further out? Not that I think that modifying default behavior of java.lang.* objects is something I would encourage ...– YoYo
Sep 11 '17 at 15:52
add a comment |
up vote
7
down vote
It should always work whichever JDK version you use:
System.out.println(Arrays.asList(array));
It will work if the Array
contains Objects. If the Array
contains primitive types, you can use wrapper classes instead storing the primitive directly as..
Example:
int a = new int{1,2,3,4,5};
Replace it with:
Integer a = new Integer{1,2,3,4,5};
Update :
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing.
So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
public void printArray(int a){
//write printing code
}
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has atoString(..)
, it defeats me why someone would ever do this.
– Debosmit Ray
May 13 '16 at 11:35
add a comment |
up vote
7
down vote
It should always work whichever JDK version you use:
System.out.println(Arrays.asList(array));
It will work if the Array
contains Objects. If the Array
contains primitive types, you can use wrapper classes instead storing the primitive directly as..
Example:
int a = new int{1,2,3,4,5};
Replace it with:
Integer a = new Integer{1,2,3,4,5};
Update :
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing.
So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
public void printArray(int a){
//write printing code
}
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has atoString(..)
, it defeats me why someone would ever do this.
– Debosmit Ray
May 13 '16 at 11:35
add a comment |
up vote
7
down vote
up vote
7
down vote
It should always work whichever JDK version you use:
System.out.println(Arrays.asList(array));
It will work if the Array
contains Objects. If the Array
contains primitive types, you can use wrapper classes instead storing the primitive directly as..
Example:
int a = new int{1,2,3,4,5};
Replace it with:
Integer a = new Integer{1,2,3,4,5};
Update :
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing.
So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
public void printArray(int a){
//write printing code
}
It should always work whichever JDK version you use:
System.out.println(Arrays.asList(array));
It will work if the Array
contains Objects. If the Array
contains primitive types, you can use wrapper classes instead storing the primitive directly as..
Example:
int a = new int{1,2,3,4,5};
Replace it with:
Integer a = new Integer{1,2,3,4,5};
Update :
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing.
So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
public void printArray(int a){
//write printing code
}
edited May 13 '16 at 11:52
answered May 13 '16 at 11:01


Girish Kumar
94421835
94421835
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has atoString(..)
, it defeats me why someone would ever do this.
– Debosmit Ray
May 13 '16 at 11:35
add a comment |
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has atoString(..)
, it defeats me why someone would ever do this.
– Debosmit Ray
May 13 '16 at 11:35
1
1
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has a
toString(..)
, it defeats me why someone would ever do this.– Debosmit Ray
May 13 '16 at 11:35
Converting an Array to a List simply for printing purposes does not seem like a very resourceful decision; and given that the same class has a
toString(..)
, it defeats me why someone would ever do this.– Debosmit Ray
May 13 '16 at 11:35
add a comment |
up vote
7
down vote
In java 8 it is easy. there are two keywords
- stream:
Arrays.stream(intArray).forEach
method reference:
::println
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::println);
If you want to print all elements in the array in the same line, then just use print
instead of println
i.e.
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::print);
Another way without method reference just use:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
add a comment |
up vote
7
down vote
In java 8 it is easy. there are two keywords
- stream:
Arrays.stream(intArray).forEach
method reference:
::println
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::println);
If you want to print all elements in the array in the same line, then just use print
instead of println
i.e.
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::print);
Another way without method reference just use:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
add a comment |
up vote
7
down vote
up vote
7
down vote
In java 8 it is easy. there are two keywords
- stream:
Arrays.stream(intArray).forEach
method reference:
::println
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::println);
If you want to print all elements in the array in the same line, then just use print
instead of println
i.e.
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::print);
Another way without method reference just use:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
In java 8 it is easy. there are two keywords
- stream:
Arrays.stream(intArray).forEach
method reference:
::println
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::println);
If you want to print all elements in the array in the same line, then just use print
instead of println
i.e.
int intArray = new int {1, 2, 3, 4, 5};
Arrays.stream(intArray).forEach(System.out::print);
Another way without method reference just use:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
edited Sep 27 '16 at 9:46


Andrii Abramov
4,01142946
4,01142946
answered Jun 26 '16 at 15:30


suatCoskun
243149
243149
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
add a comment |
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
1
1
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
This will print each element of the array on a separate line so it does not meet the requirements.
– Alex Spurling
Jun 29 '16 at 15:51
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
if u want to print all elements in the array in the same line, then just use print instead of println i.e. int intArray = new int {1, 2, 3, 4, 5}; Arrays.stream(intArray).forEach(System.out::print); antotherway without methodreference just use int intArray = new int {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(intArray));
– suatCoskun
Jun 29 '16 at 20:56
add a comment |
up vote
5
down vote
There's one additional way if your array is of type char:
char A = {'a', 'b', 'c'};
System.out.println(A); // no other arguments
prints
abc
add a comment |
up vote
5
down vote
There's one additional way if your array is of type char:
char A = {'a', 'b', 'c'};
System.out.println(A); // no other arguments
prints
abc
add a comment |
up vote
5
down vote
up vote
5
down vote
There's one additional way if your array is of type char:
char A = {'a', 'b', 'c'};
System.out.println(A); // no other arguments
prints
abc
There's one additional way if your array is of type char:
char A = {'a', 'b', 'c'};
System.out.println(A); // no other arguments
prints
abc
answered Apr 29 '14 at 7:34
Roam
2,08262750
2,08262750
add a comment |
add a comment |
up vote
4
down vote
To add to all the answers, printing the object as a JSON string is also an option.
Using Jackson:
ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter();
System.out.println(ow.writeValueAsString(anyArray));
Using Gson:
Gson gson = new Gson();
System.out.println(gson.toJson(anyArray));
add a comment |
up vote
4
down vote
To add to all the answers, printing the object as a JSON string is also an option.
Using Jackson:
ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter();
System.out.println(ow.writeValueAsString(anyArray));
Using Gson:
Gson gson = new Gson();
System.out.println(gson.toJson(anyArray));
add a comment |
up vote
4
down vote
up vote
4
down vote
To add to all the answers, printing the object as a JSON string is also an option.
Using Jackson:
ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter();
System.out.println(ow.writeValueAsString(anyArray));
Using Gson:
Gson gson = new Gson();
System.out.println(gson.toJson(anyArray));
To add to all the answers, printing the object as a JSON string is also an option.
Using Jackson:
ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter();
System.out.println(ow.writeValueAsString(anyArray));
Using Gson:
Gson gson = new Gson();
System.out.println(gson.toJson(anyArray));
edited Nov 12 '14 at 13:38
answered Feb 18 '14 at 20:21


Jean Logeart
41.6k105394
41.6k105394
add a comment |
add a comment |
up vote
4
down vote
A simplified shortcut I've tried is this:
int x = {1,2,3};
String printableText = Arrays.toString(x).replaceAll("[\[\]]", "").replaceAll(", ", "n");
System.out.println(printableText);
It will print
1
2
3
No loops required in this approach and it is best for small arrays only
add a comment |
up vote
4
down vote
A simplified shortcut I've tried is this:
int x = {1,2,3};
String printableText = Arrays.toString(x).replaceAll("[\[\]]", "").replaceAll(", ", "n");
System.out.println(printableText);
It will print
1
2
3
No loops required in this approach and it is best for small arrays only
add a comment |
up vote
4
down vote
up vote
4
down vote
A simplified shortcut I've tried is this:
int x = {1,2,3};
String printableText = Arrays.toString(x).replaceAll("[\[\]]", "").replaceAll(", ", "n");
System.out.println(printableText);
It will print
1
2
3
No loops required in this approach and it is best for small arrays only
A simplified shortcut I've tried is this:
int x = {1,2,3};
String printableText = Arrays.toString(x).replaceAll("[\[\]]", "").replaceAll(", ", "n");
System.out.println(printableText);
It will print
1
2
3
No loops required in this approach and it is best for small arrays only
edited Feb 21 '15 at 7:12
answered Feb 17 '15 at 8:02
Mohamed Idris
41738
41738
add a comment |
add a comment |
up vote
4
down vote
You could loop through the array, printing out each item, as you loop. For example:
String items = {"item 1", "item 2", "item 3"};
for(int i = 0; i < items.length; i++) {
System.out.println(items[i]);
}
Output:
item 1
item 2
item 3
add a comment |
up vote
4
down vote
You could loop through the array, printing out each item, as you loop. For example:
String items = {"item 1", "item 2", "item 3"};
for(int i = 0; i < items.length; i++) {
System.out.println(items[i]);
}
Output:
item 1
item 2
item 3
add a comment |
up vote
4
down vote
up vote
4
down vote
You could loop through the array, printing out each item, as you loop. For example:
String items = {"item 1", "item 2", "item 3"};
for(int i = 0; i < items.length; i++) {
System.out.println(items[i]);
}
Output:
item 1
item 2
item 3
You could loop through the array, printing out each item, as you loop. For example:
String items = {"item 1", "item 2", "item 3"};
for(int i = 0; i < items.length; i++) {
System.out.println(items[i]);
}
Output:
item 1
item 2
item 3
answered Jul 20 '16 at 23:55


Dylan Black
203214
203214
add a comment |
add a comment |
up vote
4
down vote
There Are Following way to print Array
// 1) toString()
int arrayInt = new int {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(arrayInt));
// 2 for loop()
for (int number : arrayInt) {
System.out.println(number);
}
// 3 for each()
for(int x: arrayInt){
System.out.println(x);
}
add a comment |
up vote
4
down vote
There Are Following way to print Array
// 1) toString()
int arrayInt = new int {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(arrayInt));
// 2 for loop()
for (int number : arrayInt) {
System.out.println(number);
}
// 3 for each()
for(int x: arrayInt){
System.out.println(x);
}
add a comment |
up vote
4
down vote
up vote
4
down vote
There Are Following way to print Array
// 1) toString()
int arrayInt = new int {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(arrayInt));
// 2 for loop()
for (int number : arrayInt) {
System.out.println(number);
}
// 3 for each()
for(int x: arrayInt){
System.out.println(x);
}
There Are Following way to print Array
// 1) toString()
int arrayInt = new int {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(arrayInt));
// 2 for loop()
for (int number : arrayInt) {
System.out.println(number);
}
// 3 for each()
for(int x: arrayInt){
System.out.println(x);
}
edited May 8 at 12:43
Muskovets
1198
1198
answered Sep 25 '17 at 13:29
Ravi Patel
993
993
add a comment |
add a comment |
up vote
3
down vote
public class printer {
public static void main(String args) {
String a = new String[4];
Scanner sc = new Scanner(System.in);
System.out.println("enter the data");
for (int i = 0; i < 4; i++) {
a[i] = sc.nextLine();
}
System.out.println("the entered data is");
for (String i : a) {
System.out.println(i);
}
}
}
add a comment |
up vote
3
down vote
public class printer {
public static void main(String args) {
String a = new String[4];
Scanner sc = new Scanner(System.in);
System.out.println("enter the data");
for (int i = 0; i < 4; i++) {
a[i] = sc.nextLine();
}
System.out.println("the entered data is");
for (String i : a) {
System.out.println(i);
}
}
}
add a comment |
up vote
3
down vote
up vote
3
down vote
public class printer {
public static void main(String args) {
String a = new String[4];
Scanner sc = new Scanner(System.in);
System.out.println("enter the data");
for (int i = 0; i < 4; i++) {
a[i] = sc.nextLine();
}
System.out.println("the entered data is");
for (String i : a) {
System.out.println(i);
}
}
}
public class printer {
public static void main(String args) {
String a = new String[4];
Scanner sc = new Scanner(System.in);
System.out.println("enter the data");
for (int i = 0; i < 4; i++) {
a[i] = sc.nextLine();
}
System.out.println("the entered data is");
for (String i : a) {
System.out.println(i);
}
}
}
edited Apr 5 '15 at 20:30


SamTebbs33
3,82131532
3,82131532
answered Sep 21 '14 at 9:11
user3369011
add a comment |
add a comment |
up vote
3
down vote
Using org.apache.commons.lang3.StringUtils.join(*) methods can be an option
For example:
String strArray = new String { "John", "Mary", "Bob" };
String arrayAsCSV = StringUtils.join(strArray, " , ");
System.out.printf("[%s]", arrayAsCSV);
//output: [John , Mary , Bob]
I used the following dependency
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
add a comment |
up vote
3
down vote
Using org.apache.commons.lang3.StringUtils.join(*) methods can be an option
For example:
String strArray = new String { "John", "Mary", "Bob" };
String arrayAsCSV = StringUtils.join(strArray, " , ");
System.out.printf("[%s]", arrayAsCSV);
//output: [John , Mary , Bob]
I used the following dependency
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
add a comment |
up vote
3
down vote
up vote
3
down vote
Using org.apache.commons.lang3.StringUtils.join(*) methods can be an option
For example:
String strArray = new String { "John", "Mary", "Bob" };
String arrayAsCSV = StringUtils.join(strArray, " , ");
System.out.printf("[%s]", arrayAsCSV);
//output: [John , Mary , Bob]
I used the following dependency
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
Using org.apache.commons.lang3.StringUtils.join(*) methods can be an option
For example:
String strArray = new String { "John", "Mary", "Bob" };
String arrayAsCSV = StringUtils.join(strArray, " , ");
System.out.printf("[%s]", arrayAsCSV);
//output: [John , Mary , Bob]
I used the following dependency
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
edited Aug 8 '15 at 20:18
answered Aug 6 '15 at 11:24


Haim Raman
7,60142851
7,60142851
add a comment |
add a comment |
up vote
3
down vote
For-each loop can also be used to print elements of array:
int array = {1, 2, 3, 4, 5};
for (int i:array)
System.out.println(i);
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
add a comment |
up vote
3
down vote
For-each loop can also be used to print elements of array:
int array = {1, 2, 3, 4, 5};
for (int i:array)
System.out.println(i);
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
add a comment |
up vote
3
down vote
up vote
3
down vote
For-each loop can also be used to print elements of array:
int array = {1, 2, 3, 4, 5};
for (int i:array)
System.out.println(i);
For-each loop can also be used to print elements of array:
int array = {1, 2, 3, 4, 5};
for (int i:array)
System.out.println(i);
edited Mar 11 at 19:23


Ma_124
4319
4319
answered Dec 5 '16 at 20:10
hasham.98
633
633
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
add a comment |
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
@firephil System.out.println(a[i]); is used with ordinary for loop, where index "i" is created and value at every index is printed. I have used "for each" loop. Give it a try, hope you will get my point.
– hasham.98
Dec 25 '16 at 21:22
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
Yes,this is the shortest way to print array
– teran teshara
Aug 4 at 16:20
add a comment |
up vote
2
down vote
This is marked as a duplicate for printing a byte. Note: for a byte array there are additional methods which may be appropriate.
You can print it as a String if it contains ISO-8859-1 chars.
String s = new String(bytes, StandardChars.ISO_8559);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.ISO_8559);
or if it contains a UTF-8 string
String s = new String(bytes, StandardChars.UTF_8);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.UTF_8);
or if you want print it as hexadecimal.
String s = DatatypeConverter.printHexBinary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseHexBinary(s);
or if you want print it as base64.
String s = DatatypeConverter.printBase64Binary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseBase64Binary(s);
or if you want to print an array of signed byte values
String s = Arrays.toString(bytes);
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = Byte.parseByte(split[i]);
or if you want to print an array of unsigned byte values
String s = Arrays.toString(
IntStream.range(0, bytes.length).map(i -> bytes[i] & 0xFF).toArray());
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = (byte) Integer.parseInt(split[i]); // might need a range check.
add a comment |
up vote
2
down vote
This is marked as a duplicate for printing a byte. Note: for a byte array there are additional methods which may be appropriate.
You can print it as a String if it contains ISO-8859-1 chars.
String s = new String(bytes, StandardChars.ISO_8559);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.ISO_8559);
or if it contains a UTF-8 string
String s = new String(bytes, StandardChars.UTF_8);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.UTF_8);
or if you want print it as hexadecimal.
String s = DatatypeConverter.printHexBinary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseHexBinary(s);
or if you want print it as base64.
String s = DatatypeConverter.printBase64Binary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseBase64Binary(s);
or if you want to print an array of signed byte values
String s = Arrays.toString(bytes);
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = Byte.parseByte(split[i]);
or if you want to print an array of unsigned byte values
String s = Arrays.toString(
IntStream.range(0, bytes.length).map(i -> bytes[i] & 0xFF).toArray());
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = (byte) Integer.parseInt(split[i]); // might need a range check.
add a comment |
up vote
2
down vote
up vote
2
down vote
This is marked as a duplicate for printing a byte. Note: for a byte array there are additional methods which may be appropriate.
You can print it as a String if it contains ISO-8859-1 chars.
String s = new String(bytes, StandardChars.ISO_8559);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.ISO_8559);
or if it contains a UTF-8 string
String s = new String(bytes, StandardChars.UTF_8);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.UTF_8);
or if you want print it as hexadecimal.
String s = DatatypeConverter.printHexBinary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseHexBinary(s);
or if you want print it as base64.
String s = DatatypeConverter.printBase64Binary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseBase64Binary(s);
or if you want to print an array of signed byte values
String s = Arrays.toString(bytes);
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = Byte.parseByte(split[i]);
or if you want to print an array of unsigned byte values
String s = Arrays.toString(
IntStream.range(0, bytes.length).map(i -> bytes[i] & 0xFF).toArray());
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = (byte) Integer.parseInt(split[i]); // might need a range check.
This is marked as a duplicate for printing a byte. Note: for a byte array there are additional methods which may be appropriate.
You can print it as a String if it contains ISO-8859-1 chars.
String s = new String(bytes, StandardChars.ISO_8559);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.ISO_8559);
or if it contains a UTF-8 string
String s = new String(bytes, StandardChars.UTF_8);
System.out.println(s);
// to reverse
byte bytes2 = s.getBytes(StandardChars.UTF_8);
or if you want print it as hexadecimal.
String s = DatatypeConverter.printHexBinary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseHexBinary(s);
or if you want print it as base64.
String s = DatatypeConverter.printBase64Binary(bytes);
System.out.println(s);
// to reverse
byte bytes2 = DatatypeConverter.parseBase64Binary(s);
or if you want to print an array of signed byte values
String s = Arrays.toString(bytes);
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = Byte.parseByte(split[i]);
or if you want to print an array of unsigned byte values
String s = Arrays.toString(
IntStream.range(0, bytes.length).map(i -> bytes[i] & 0xFF).toArray());
System.out.println(s);
// to reverse
String split = s.substring(1, s.length() - 1).split(", ");
byte bytes2 = new byte[split.length];
for (int i = 0; i < bytes2.length; i++)
bytes2[i] = (byte) Integer.parseInt(split[i]); // might need a range check.
edited Jun 22 at 19:26
answered Jun 22 at 19:17
Peter Lawrey
437k55551949
437k55551949
add a comment |
add a comment |
up vote
0
down vote
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray));
output: [John, Mary, Bob]
add a comment |
up vote
0
down vote
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray));
output: [John, Mary, Bob]
add a comment |
up vote
0
down vote
up vote
0
down vote
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray));
output: [John, Mary, Bob]
// array of primitives:
int intArray = new int {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray));
output: [1, 2, 3, 4, 5]
// array of object references:
String strArray = new String {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray));
output: [John, Mary, Bob]
edited Mar 16 at 1:44


Stephen Rauch
27.3k153156
27.3k153156
answered Mar 16 at 1:17
fjnk
93
93
add a comment |
add a comment |
up vote
0
down vote
In java 8 :
Arrays.stream(myArray).forEach(System.out::println);
add a comment |
up vote
0
down vote
In java 8 :
Arrays.stream(myArray).forEach(System.out::println);
add a comment |
up vote
0
down vote
up vote
0
down vote
In java 8 :
Arrays.stream(myArray).forEach(System.out::println);
In java 8 :
Arrays.stream(myArray).forEach(System.out::println);
edited May 8 at 20:05
Muskovets
1198
1198
answered Apr 20 at 18:25
Mehdi
554517
554517
add a comment |
add a comment |
up vote
0
down vote
There are several ways to print an array elements.First of all, I'll explain that, what is an array?..Array is a simple data structure for storing data..When you define an array , Allocate set of ancillary memory blocks in RAM.Those memory blocks are taken one unit ..
Ok, I'll create an array like this,
class demo{
public static void main(String a){
int number={1,2,3,4,5};
System.out.print(number);
}
}
Now look at the output,
You can see an unknown string printed..As I mentioned before, the memory address whose array(number array) declared is printed.If you want to display elements in the array, you can use "for loop " , like this..
class demo{
public static void main(String a){
int number={1,2,3,4,5};
int i;
for(i=0;i<number.length;i++){
System.out.print(number[i]+" ");
}
}
}
Now look at the output,
Ok,Successfully printed elements of one dimension array..Now I am going to consider two dimension array..I'll declare two dimension array as "number2" and print the elements using "Arrays.deepToString()" keyword.Before using that You will have to import 'java.util.Arrays' library.
import java.util.Arrays;
class demo{
public static void main(String a){
int number2={{1,2},{3,4},{5,6}};`
System.out.print(Arrays.deepToString(number2));
}
}
consider the output,
At the same time , Using two for loops ,2D elements can be printed..Thank you !
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
add a comment |
up vote
0
down vote
There are several ways to print an array elements.First of all, I'll explain that, what is an array?..Array is a simple data structure for storing data..When you define an array , Allocate set of ancillary memory blocks in RAM.Those memory blocks are taken one unit ..
Ok, I'll create an array like this,
class demo{
public static void main(String a){
int number={1,2,3,4,5};
System.out.print(number);
}
}
Now look at the output,
You can see an unknown string printed..As I mentioned before, the memory address whose array(number array) declared is printed.If you want to display elements in the array, you can use "for loop " , like this..
class demo{
public static void main(String a){
int number={1,2,3,4,5};
int i;
for(i=0;i<number.length;i++){
System.out.print(number[i]+" ");
}
}
}
Now look at the output,
Ok,Successfully printed elements of one dimension array..Now I am going to consider two dimension array..I'll declare two dimension array as "number2" and print the elements using "Arrays.deepToString()" keyword.Before using that You will have to import 'java.util.Arrays' library.
import java.util.Arrays;
class demo{
public static void main(String a){
int number2={{1,2},{3,4},{5,6}};`
System.out.print(Arrays.deepToString(number2));
}
}
consider the output,
At the same time , Using two for loops ,2D elements can be printed..Thank you !
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
add a comment |
up vote
0
down vote
up vote
0
down vote
There are several ways to print an array elements.First of all, I'll explain that, what is an array?..Array is a simple data structure for storing data..When you define an array , Allocate set of ancillary memory blocks in RAM.Those memory blocks are taken one unit ..
Ok, I'll create an array like this,
class demo{
public static void main(String a){
int number={1,2,3,4,5};
System.out.print(number);
}
}
Now look at the output,
You can see an unknown string printed..As I mentioned before, the memory address whose array(number array) declared is printed.If you want to display elements in the array, you can use "for loop " , like this..
class demo{
public static void main(String a){
int number={1,2,3,4,5};
int i;
for(i=0;i<number.length;i++){
System.out.print(number[i]+" ");
}
}
}
Now look at the output,
Ok,Successfully printed elements of one dimension array..Now I am going to consider two dimension array..I'll declare two dimension array as "number2" and print the elements using "Arrays.deepToString()" keyword.Before using that You will have to import 'java.util.Arrays' library.
import java.util.Arrays;
class demo{
public static void main(String a){
int number2={{1,2},{3,4},{5,6}};`
System.out.print(Arrays.deepToString(number2));
}
}
consider the output,
At the same time , Using two for loops ,2D elements can be printed..Thank you !
There are several ways to print an array elements.First of all, I'll explain that, what is an array?..Array is a simple data structure for storing data..When you define an array , Allocate set of ancillary memory blocks in RAM.Those memory blocks are taken one unit ..
Ok, I'll create an array like this,
class demo{
public static void main(String a){
int number={1,2,3,4,5};
System.out.print(number);
}
}
Now look at the output,
You can see an unknown string printed..As I mentioned before, the memory address whose array(number array) declared is printed.If you want to display elements in the array, you can use "for loop " , like this..
class demo{
public static void main(String a){
int number={1,2,3,4,5};
int i;
for(i=0;i<number.length;i++){
System.out.print(number[i]+" ");
}
}
}
Now look at the output,
Ok,Successfully printed elements of one dimension array..Now I am going to consider two dimension array..I'll declare two dimension array as "number2" and print the elements using "Arrays.deepToString()" keyword.Before using that You will have to import 'java.util.Arrays' library.
import java.util.Arrays;
class demo{
public static void main(String a){
int number2={{1,2},{3,4},{5,6}};`
System.out.print(Arrays.deepToString(number2));
}
}
consider the output,
At the same time , Using two for loops ,2D elements can be printed..Thank you !
answered Jul 1 at 15:45


GT_hash
415
415
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
add a comment |
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
int array = {1, 2, 3, 4, 5}; for (int i:array) System.out.println(i);
– teran teshara
Aug 4 at 16:22
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
try this i think this is shortest way to print array
– teran teshara
Aug 4 at 16:23
add a comment |
up vote
-1
down vote
If you want to print, evaluate Array content like that you can use Arrays.toString
jshell> String names = {"ram","shyam"};
names ==> String[2] { "ram", "shyam" }
jshell> Arrays.toString(names);
$2 ==> "[ram, shyam]"
jshell>
add a comment |
up vote
-1
down vote
If you want to print, evaluate Array content like that you can use Arrays.toString
jshell> String names = {"ram","shyam"};
names ==> String[2] { "ram", "shyam" }
jshell> Arrays.toString(names);
$2 ==> "[ram, shyam]"
jshell>
add a comment |
up vote
-1
down vote
up vote
-1
down vote
If you want to print, evaluate Array content like that you can use Arrays.toString
jshell> String names = {"ram","shyam"};
names ==> String[2] { "ram", "shyam" }
jshell> Arrays.toString(names);
$2 ==> "[ram, shyam]"
jshell>
If you want to print, evaluate Array content like that you can use Arrays.toString
jshell> String names = {"ram","shyam"};
names ==> String[2] { "ram", "shyam" }
jshell> Arrays.toString(names);
$2 ==> "[ram, shyam]"
jshell>
answered Jul 6 at 12:29


Sudip Bhandari
7161117
7161117
add a comment |
add a comment |
up vote
-3
down vote
You could use Arrays.toString()
String array = { "a", "b", "c" };
System.out.println(Arrays.toString(array));
add a comment |
up vote
-3
down vote
You could use Arrays.toString()
String array = { "a", "b", "c" };
System.out.println(Arrays.toString(array));
add a comment |
up vote
-3
down vote
up vote
-3
down vote
You could use Arrays.toString()
String array = { "a", "b", "c" };
System.out.println(Arrays.toString(array));
You could use Arrays.toString()
String array = { "a", "b", "c" };
System.out.println(Arrays.toString(array));
edited Mar 11 at 17:12


Ma_124
4319
4319
answered Dec 20 '17 at 8:52
Atuljssaten
547
547
add a comment |
add a comment |
up vote
-5
down vote
The simplest way to print an array is to use a for-loop:
// initialize array
for(int i=0;i<array.length;i++)
{
System.out.print(array[i] + " ");
}
The correct for loop, assuming aT myArray
, isfor (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
add a comment |
up vote
-5
down vote
The simplest way to print an array is to use a for-loop:
// initialize array
for(int i=0;i<array.length;i++)
{
System.out.print(array[i] + " ");
}
The correct for loop, assuming aT myArray
, isfor (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
add a comment |
up vote
-5
down vote
up vote
-5
down vote
The simplest way to print an array is to use a for-loop:
// initialize array
for(int i=0;i<array.length;i++)
{
System.out.print(array[i] + " ");
}
The simplest way to print an array is to use a for-loop:
// initialize array
for(int i=0;i<array.length;i++)
{
System.out.print(array[i] + " ");
}
edited Dec 29 '17 at 20:12
Community♦
11
11
answered Oct 1 '15 at 3:12


Joy Kimaru
231
231
The correct for loop, assuming aT myArray
, isfor (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
add a comment |
The correct for loop, assuming aT myArray
, isfor (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
The correct for loop, assuming a
T myArray
, is for (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
The correct for loop, assuming a
T myArray
, is for (int i = 0; i < myArray.length; i++) { System.out.println(myArray[i] + " "); }
– Nic Hartley
Jan 17 '16 at 0:27
add a comment |
protected by Aniket Thakur Oct 2 '15 at 18:54
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
c duZ7 lDh,kekyZwGmw1wB1RR
5
What do you want the representation to be for objects other than strings? The result of calling toString? In quotes or not?
– Jon Skeet
Jan 3 '09 at 20:41
2
Yes objects would be represented by their toString() method and without quotes (just edited the example output).
– Alex Spurling
Jan 3 '09 at 20:42
2
In practice, closely related to stackoverflow.com/questions/29140402/…
– Raedwald
Jan 24 '16 at 18:02
1
That weird output is not necessarily the memory location. It's the
hashCode()
in hexadecimal. SeeObject#toString()
.– 4castle
Nov 10 '16 at 21:22
1
To print single dimensional or multi-dimensional array in java8 check stackoverflow.com/questions/409784/…
– i_am_zero
Nov 16 '16 at 1:28