Creating a Large List of (Large) Vectors with Rcpp
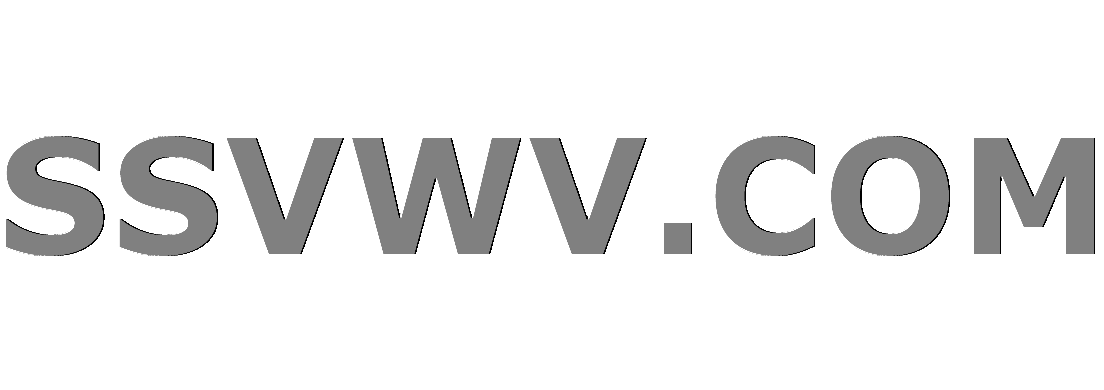
Multi tool use
up vote
1
down vote
favorite
The input of my function consists of two matrices mat1
and mat2
and the number of permutations B
. Both mat1
and mat2
have m
columns, but different number of rows.
The function first permutes the rows of both matrices (while maintaining column information). It then performs some operation that compares columns of permuted versions of mat1
and mat2
.
The following is an example of my function permute_data
. The comparison function CompareMatCols()
outputs a vector of length m
.
Question
What is the best way to initialize my output list object? I've seen several posts indicating the limitations of push_back
. Both B
and m
will be on the order of ~10000, so an efficient way would be ideal.
#include <Rcpp.h>
#include <math.h>
//#include <random> //for std::shuffle
using namespace std;
using namespace Rcpp;
// [[Rcpp::export]]
NumericVector ColMax(NumericMatrix X) {
NumericVector out = no_init(X.cols());
for(int j = 0; j < X.cols(); ++j) {
double omax = X(0,j);
for(int i = 0; i < X.rows(); ++i){
omax = std::max(X(i,j),omax);
}
out[j] = omax;
}
return out;
}
// [[Rcpp::export]]
NumericVector vecmin(NumericVector vec1, NumericVector vec2) {
int n = vec1.size();
if(n != vec2.size()) return 0;
else {
NumericVector out = no_init(n);
for(int i = 0; i < n; i++) {
out[i] = std::min(vec1[i], vec2[i]);
}
return out;
}
}
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // How to initialize this???, Will be large ~10000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
/*std::random_device rng;
std::mt19937 urng(rng()); //uniform rng
std::shuffle(permindx.begin(),permindx.end(),urng);*/
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
/***R
set.seed(1)
X = matrix(rnorm(3*5),ncol=5)
Y = matrix(rnorm(5*5),ncol=5)
B = 5
res = permute_data(X,Y,B)
*/
Edit: Added lines to address @duckmayr's point.
Edit 2: Per Dirk's suggestion, I included a minimally complete verifiable example.
list rcpp
|
show 9 more comments
up vote
1
down vote
favorite
The input of my function consists of two matrices mat1
and mat2
and the number of permutations B
. Both mat1
and mat2
have m
columns, but different number of rows.
The function first permutes the rows of both matrices (while maintaining column information). It then performs some operation that compares columns of permuted versions of mat1
and mat2
.
The following is an example of my function permute_data
. The comparison function CompareMatCols()
outputs a vector of length m
.
Question
What is the best way to initialize my output list object? I've seen several posts indicating the limitations of push_back
. Both B
and m
will be on the order of ~10000, so an efficient way would be ideal.
#include <Rcpp.h>
#include <math.h>
//#include <random> //for std::shuffle
using namespace std;
using namespace Rcpp;
// [[Rcpp::export]]
NumericVector ColMax(NumericMatrix X) {
NumericVector out = no_init(X.cols());
for(int j = 0; j < X.cols(); ++j) {
double omax = X(0,j);
for(int i = 0; i < X.rows(); ++i){
omax = std::max(X(i,j),omax);
}
out[j] = omax;
}
return out;
}
// [[Rcpp::export]]
NumericVector vecmin(NumericVector vec1, NumericVector vec2) {
int n = vec1.size();
if(n != vec2.size()) return 0;
else {
NumericVector out = no_init(n);
for(int i = 0; i < n; i++) {
out[i] = std::min(vec1[i], vec2[i]);
}
return out;
}
}
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // How to initialize this???, Will be large ~10000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
/*std::random_device rng;
std::mt19937 urng(rng()); //uniform rng
std::shuffle(permindx.begin(),permindx.end(),urng);*/
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
/***R
set.seed(1)
X = matrix(rnorm(3*5),ncol=5)
Y = matrix(rnorm(5*5),ncol=5)
B = 5
res = permute_data(X,Y,B)
*/
Edit: Added lines to address @duckmayr's point.
Edit 2: Per Dirk's suggestion, I included a minimally complete verifiable example.
list rcpp
I am not sure if the caveats aboutpush_back
also apply toList
, but you could usestd::vector
instead. BTW, I would not usestd::random_shuffle
, since it has been deprecated/removed from modern C++ versions.
– Ralf Stubner
Nov 11 at 6:51
Unrelated note: Have you used the function as it is yet? I have to imagine the lineM1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements ofpermindx
could include one or more elements greater thanN1
(and/or similarly for the last N2 elements and assigning toM2
)
– duckmayr
Nov 11 at 12:06
@RalfStubner, what alternative would you suggest tostd::random_shuffle
?
– stats134711
Nov 11 at 14:31
1
I would usestd::shuffle
orRcppArmadillo::sample
.
– Ralf Stubner
Nov 11 at 21:28
1
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37
|
show 9 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
The input of my function consists of two matrices mat1
and mat2
and the number of permutations B
. Both mat1
and mat2
have m
columns, but different number of rows.
The function first permutes the rows of both matrices (while maintaining column information). It then performs some operation that compares columns of permuted versions of mat1
and mat2
.
The following is an example of my function permute_data
. The comparison function CompareMatCols()
outputs a vector of length m
.
Question
What is the best way to initialize my output list object? I've seen several posts indicating the limitations of push_back
. Both B
and m
will be on the order of ~10000, so an efficient way would be ideal.
#include <Rcpp.h>
#include <math.h>
//#include <random> //for std::shuffle
using namespace std;
using namespace Rcpp;
// [[Rcpp::export]]
NumericVector ColMax(NumericMatrix X) {
NumericVector out = no_init(X.cols());
for(int j = 0; j < X.cols(); ++j) {
double omax = X(0,j);
for(int i = 0; i < X.rows(); ++i){
omax = std::max(X(i,j),omax);
}
out[j] = omax;
}
return out;
}
// [[Rcpp::export]]
NumericVector vecmin(NumericVector vec1, NumericVector vec2) {
int n = vec1.size();
if(n != vec2.size()) return 0;
else {
NumericVector out = no_init(n);
for(int i = 0; i < n; i++) {
out[i] = std::min(vec1[i], vec2[i]);
}
return out;
}
}
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // How to initialize this???, Will be large ~10000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
/*std::random_device rng;
std::mt19937 urng(rng()); //uniform rng
std::shuffle(permindx.begin(),permindx.end(),urng);*/
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
/***R
set.seed(1)
X = matrix(rnorm(3*5),ncol=5)
Y = matrix(rnorm(5*5),ncol=5)
B = 5
res = permute_data(X,Y,B)
*/
Edit: Added lines to address @duckmayr's point.
Edit 2: Per Dirk's suggestion, I included a minimally complete verifiable example.
list rcpp
The input of my function consists of two matrices mat1
and mat2
and the number of permutations B
. Both mat1
and mat2
have m
columns, but different number of rows.
The function first permutes the rows of both matrices (while maintaining column information). It then performs some operation that compares columns of permuted versions of mat1
and mat2
.
The following is an example of my function permute_data
. The comparison function CompareMatCols()
outputs a vector of length m
.
Question
What is the best way to initialize my output list object? I've seen several posts indicating the limitations of push_back
. Both B
and m
will be on the order of ~10000, so an efficient way would be ideal.
#include <Rcpp.h>
#include <math.h>
//#include <random> //for std::shuffle
using namespace std;
using namespace Rcpp;
// [[Rcpp::export]]
NumericVector ColMax(NumericMatrix X) {
NumericVector out = no_init(X.cols());
for(int j = 0; j < X.cols(); ++j) {
double omax = X(0,j);
for(int i = 0; i < X.rows(); ++i){
omax = std::max(X(i,j),omax);
}
out[j] = omax;
}
return out;
}
// [[Rcpp::export]]
NumericVector vecmin(NumericVector vec1, NumericVector vec2) {
int n = vec1.size();
if(n != vec2.size()) return 0;
else {
NumericVector out = no_init(n);
for(int i = 0; i < n; i++) {
out[i] = std::min(vec1[i], vec2[i]);
}
return out;
}
}
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // How to initialize this???, Will be large ~10000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
/*std::random_device rng;
std::mt19937 urng(rng()); //uniform rng
std::shuffle(permindx.begin(),permindx.end(),urng);*/
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
/***R
set.seed(1)
X = matrix(rnorm(3*5),ncol=5)
Y = matrix(rnorm(5*5),ncol=5)
B = 5
res = permute_data(X,Y,B)
*/
Edit: Added lines to address @duckmayr's point.
Edit 2: Per Dirk's suggestion, I included a minimally complete verifiable example.
list rcpp
list rcpp
edited Nov 11 at 21:32
asked Nov 11 at 4:06
stats134711
243211
243211
I am not sure if the caveats aboutpush_back
also apply toList
, but you could usestd::vector
instead. BTW, I would not usestd::random_shuffle
, since it has been deprecated/removed from modern C++ versions.
– Ralf Stubner
Nov 11 at 6:51
Unrelated note: Have you used the function as it is yet? I have to imagine the lineM1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements ofpermindx
could include one or more elements greater thanN1
(and/or similarly for the last N2 elements and assigning toM2
)
– duckmayr
Nov 11 at 12:06
@RalfStubner, what alternative would you suggest tostd::random_shuffle
?
– stats134711
Nov 11 at 14:31
1
I would usestd::shuffle
orRcppArmadillo::sample
.
– Ralf Stubner
Nov 11 at 21:28
1
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37
|
show 9 more comments
I am not sure if the caveats aboutpush_back
also apply toList
, but you could usestd::vector
instead. BTW, I would not usestd::random_shuffle
, since it has been deprecated/removed from modern C++ versions.
– Ralf Stubner
Nov 11 at 6:51
Unrelated note: Have you used the function as it is yet? I have to imagine the lineM1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements ofpermindx
could include one or more elements greater thanN1
(and/or similarly for the last N2 elements and assigning toM2
)
– duckmayr
Nov 11 at 12:06
@RalfStubner, what alternative would you suggest tostd::random_shuffle
?
– stats134711
Nov 11 at 14:31
1
I would usestd::shuffle
orRcppArmadillo::sample
.
– Ralf Stubner
Nov 11 at 21:28
1
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37
I am not sure if the caveats about
push_back
also apply to List
, but you could use std::vector
instead. BTW, I would not use std::random_shuffle
, since it has been deprecated/removed from modern C++ versions.– Ralf Stubner
Nov 11 at 6:51
I am not sure if the caveats about
push_back
also apply to List
, but you could use std::vector
instead. BTW, I would not use std::random_shuffle
, since it has been deprecated/removed from modern C++ versions.– Ralf Stubner
Nov 11 at 6:51
Unrelated note: Have you used the function as it is yet? I have to imagine the line
M1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements of permindx
could include one or more elements greater than N1
(and/or similarly for the last N2 elements and assigning to M2
)– duckmayr
Nov 11 at 12:06
Unrelated note: Have you used the function as it is yet? I have to imagine the line
M1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements of permindx
could include one or more elements greater than N1
(and/or similarly for the last N2 elements and assigning to M2
)– duckmayr
Nov 11 at 12:06
@RalfStubner, what alternative would you suggest to
std::random_shuffle
?– stats134711
Nov 11 at 14:31
@RalfStubner, what alternative would you suggest to
std::random_shuffle
?– stats134711
Nov 11 at 14:31
1
1
I would use
std::shuffle
or RcppArmadillo::sample
.– Ralf Stubner
Nov 11 at 21:28
I would use
std::shuffle
or RcppArmadillo::sample
.– Ralf Stubner
Nov 11 at 21:28
1
1
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37
|
show 9 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
In the end, the solution I went with is the one seen above:
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // Will be large ~5000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
In the end, the solution I went with is the one seen above:
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // Will be large ~5000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
add a comment |
up vote
0
down vote
accepted
In the end, the solution I went with is the one seen above:
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // Will be large ~5000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
In the end, the solution I went with is the one seen above:
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // Will be large ~5000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
In the end, the solution I went with is the one seen above:
// [[Rcpp::export]]
List permute_data(NumericMatrix mat1,NumericMatrix mat2,int B) {
List out(B); // Will be large ~5000 elements
int N1 = mat1.rows();
int N2 = mat2.rows();
int m = mat1.cols(); //Will be large ~10000 elements
// Row labels to be permuted
IntegerVector permindx = seq(0,N1+N2-1);
NumericMatrix M1 = no_init_matrix(N1,m);
NumericMatrix M2 = no_init_matrix(N2,m);
for(int b = 0; b<B; ++b){
// Permute the N1+N2 rows
permindx = sample(permindx,N1+N2); //Use Rcpp's function to work with R's RNG
for(int j=0; j<m; ++j){
// Pick out first N1 elements of permindx
for(int i=0; i<N1; ++i){
if(permindx[i]>=N1){ //Check that shuffled index is in bounds
M1(i,j) = mat2(permindx[i],j);
} else{
M1(i,j) = mat1(permindx[i],j);
}
}
// Pick out last N2 elements of permindx
for(int k=0; k<N2; ++k){
if(permindx[k+N1]<N1){ //Check that shuffled index is in bounds
M2(k,j) = mat1(permindx[k+N1],j);
} else{
M2(k,j) = mat2(permindx[k+N1],j);
}
}
}
out[b] = vecmin(ColMax(M1),ColMax(M2)); //a vector of length m
}
return(out);
}
answered Nov 14 at 16:32
stats134711
243211
243211
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245752%2fcreating-a-large-list-of-large-vectors-with-rcpp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fajEEdzdvBjlSG0A 8WUpTO7sWcNJX aRn2 YU33HKG0rGfp RAGVQ1SJ,OA,flCK,V 02VODk1
I am not sure if the caveats about
push_back
also apply toList
, but you could usestd::vector
instead. BTW, I would not usestd::random_shuffle
, since it has been deprecated/removed from modern C++ versions.– Ralf Stubner
Nov 11 at 6:51
Unrelated note: Have you used the function as it is yet? I have to imagine the line
M1(i,j) = mat1(permindx[i],j);
will sometimes be problematic since the first N1 elements ofpermindx
could include one or more elements greater thanN1
(and/or similarly for the last N2 elements and assigning toM2
)– duckmayr
Nov 11 at 12:06
@RalfStubner, what alternative would you suggest to
std::random_shuffle
?– stats134711
Nov 11 at 14:31
1
I would use
std::shuffle
orRcppArmadillo::sample
.– Ralf Stubner
Nov 11 at 21:28
1
How about adding a self-answer?
– Ralf Stubner
Nov 13 at 12:37