Importing Runtime-Configurable JSON in Typescript and Angular 7
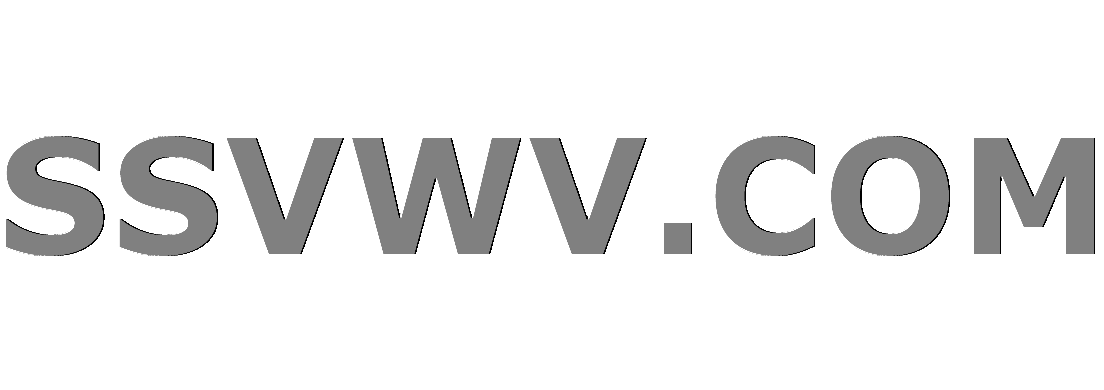
Multi tool use
up vote
1
down vote
favorite
I have a runtime translation system in place in my application, which before switching to Angular 7 was using http requests to load a JSON file filled with translations, like the below:
loadLanguage(lang: string) {
this.http.get('assets/lang/' + lang + '.json)
.toPromise()
.then((translations) => this.translations = translations; )
}
As part of an upgrade to Angular 7 I decided to try out importing the json directly rather than using http to get it. This is the new way I've implemented it:
async loadLanguage(lang: string) {
this.translations = await import('assets/lang/' + lang + '.json);
}
This works the way I would expect in terms of changing the language but the problem I am having is changing the JSON files after compilation has no effect on the output of the import.
From the bundle produced by the compilation it looks like all of the imported files are being compiled into chunks which are then loaded when I ask for that particular json file. Am I just getting the wrong idea with these imports and how they work when used with webpack or is there something obvious that I have missed when refactoring my solution?
Thanks
angular typescript angular7
add a comment |
up vote
1
down vote
favorite
I have a runtime translation system in place in my application, which before switching to Angular 7 was using http requests to load a JSON file filled with translations, like the below:
loadLanguage(lang: string) {
this.http.get('assets/lang/' + lang + '.json)
.toPromise()
.then((translations) => this.translations = translations; )
}
As part of an upgrade to Angular 7 I decided to try out importing the json directly rather than using http to get it. This is the new way I've implemented it:
async loadLanguage(lang: string) {
this.translations = await import('assets/lang/' + lang + '.json);
}
This works the way I would expect in terms of changing the language but the problem I am having is changing the JSON files after compilation has no effect on the output of the import.
From the bundle produced by the compilation it looks like all of the imported files are being compiled into chunks which are then loaded when I ask for that particular json file. Am I just getting the wrong idea with these imports and how they work when used with webpack or is there something obvious that I have missed when refactoring my solution?
Thanks
angular typescript angular7
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a runtime translation system in place in my application, which before switching to Angular 7 was using http requests to load a JSON file filled with translations, like the below:
loadLanguage(lang: string) {
this.http.get('assets/lang/' + lang + '.json)
.toPromise()
.then((translations) => this.translations = translations; )
}
As part of an upgrade to Angular 7 I decided to try out importing the json directly rather than using http to get it. This is the new way I've implemented it:
async loadLanguage(lang: string) {
this.translations = await import('assets/lang/' + lang + '.json);
}
This works the way I would expect in terms of changing the language but the problem I am having is changing the JSON files after compilation has no effect on the output of the import.
From the bundle produced by the compilation it looks like all of the imported files are being compiled into chunks which are then loaded when I ask for that particular json file. Am I just getting the wrong idea with these imports and how they work when used with webpack or is there something obvious that I have missed when refactoring my solution?
Thanks
angular typescript angular7
I have a runtime translation system in place in my application, which before switching to Angular 7 was using http requests to load a JSON file filled with translations, like the below:
loadLanguage(lang: string) {
this.http.get('assets/lang/' + lang + '.json)
.toPromise()
.then((translations) => this.translations = translations; )
}
As part of an upgrade to Angular 7 I decided to try out importing the json directly rather than using http to get it. This is the new way I've implemented it:
async loadLanguage(lang: string) {
this.translations = await import('assets/lang/' + lang + '.json);
}
This works the way I would expect in terms of changing the language but the problem I am having is changing the JSON files after compilation has no effect on the output of the import.
From the bundle produced by the compilation it looks like all of the imported files are being compiled into chunks which are then loaded when I ask for that particular json file. Am I just getting the wrong idea with these imports and how they work when used with webpack or is there something obvious that I have missed when refactoring my solution?
Thanks
angular typescript angular7
angular typescript angular7
edited Nov 11 at 3:58


Goncalo Peres
9731311
9731311
asked Oct 30 at 13:17
peppermcknight
915822
915822
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
I don't think you should be doing this. As import() gets converted to required() post compilation. plus this is not a Angular feature it's a TypeScript feature.
here a very nice and detailed article on Type https://blog.mariusschulz.com/2018/01/14/typescript-2-4-dynamic-import-expressions
As a recommendation you should be using http calls to download dynamic runtime configuration.
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
I don't think you should be doing this. As import() gets converted to required() post compilation. plus this is not a Angular feature it's a TypeScript feature.
here a very nice and detailed article on Type https://blog.mariusschulz.com/2018/01/14/typescript-2-4-dynamic-import-expressions
As a recommendation you should be using http calls to download dynamic runtime configuration.
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
add a comment |
up vote
2
down vote
accepted
I don't think you should be doing this. As import() gets converted to required() post compilation. plus this is not a Angular feature it's a TypeScript feature.
here a very nice and detailed article on Type https://blog.mariusschulz.com/2018/01/14/typescript-2-4-dynamic-import-expressions
As a recommendation you should be using http calls to download dynamic runtime configuration.
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
I don't think you should be doing this. As import() gets converted to required() post compilation. plus this is not a Angular feature it's a TypeScript feature.
here a very nice and detailed article on Type https://blog.mariusschulz.com/2018/01/14/typescript-2-4-dynamic-import-expressions
As a recommendation you should be using http calls to download dynamic runtime configuration.
I don't think you should be doing this. As import() gets converted to required() post compilation. plus this is not a Angular feature it's a TypeScript feature.
here a very nice and detailed article on Type https://blog.mariusschulz.com/2018/01/14/typescript-2-4-dynamic-import-expressions
As a recommendation you should be using http calls to download dynamic runtime configuration.
answered Oct 30 at 13:28
ravi kant
462
462
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
add a comment |
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
Yeah, on typing this question out I had thought that I might had been mistaken trying to use TS dynamic imports for my config. Thanks for your quick reply.
– peppermcknight
Oct 30 at 13:36
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53065172%2fimporting-runtime-configurable-json-in-typescript-and-angular-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AgmOQ9tZ zdNp,mc,oKwcyv D3Nce2b2Db oCItfWvjvQ9 xGMl71cb9BdS,jymdZID1rhMH,deeCRh7g0uf8y0SR4Ep iS