Unit test stopped working when switching from Karma to Jest
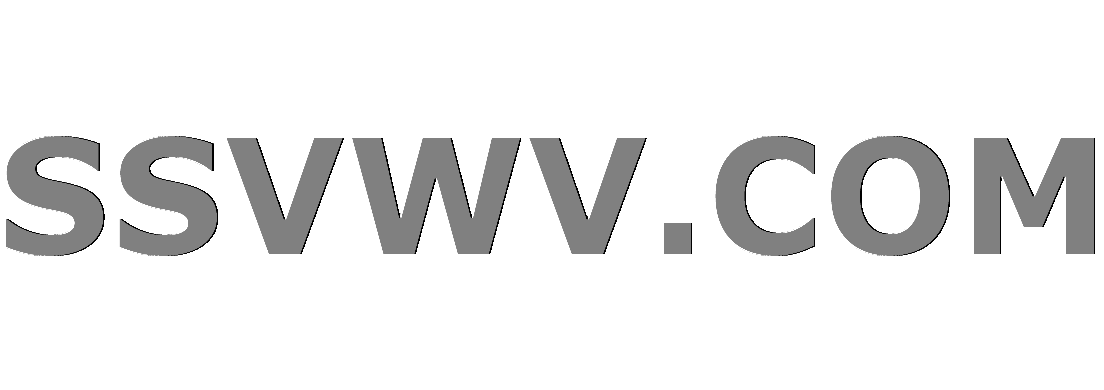
Multi tool use
up vote
0
down vote
favorite
I am working on an Ionic/Angular project and tried switching from Karma to Jest for my testing.
The following test was running fine before the switch:
import {TestBed, inject, async} from '@angular/core/testing';
import { TreasureTableService } from './treasure-table.service';
import {PapaParseModule} from 'ngx-papaparse';
import {HttpClientModule} from '@angular/common/http';
describe('TreasureTableService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientModule, PapaParseModule]
});
});
beforeEach(inject([TreasureTableService], s => {
treasureTableService = s;
}));
it('should get treasure type list', async() => {
let result: string = await treasureTableService.getTreasureTypeList();
expect(result).toContain("A");
expect(result.length).toBe(33);
});
});
After I switched to Jest I got the following error:
console.error node_modules/jsdom/lib/jsdom/virtual-console.js:29
Error: Error: connect ECONNREFUSED 127.0.0.1:80
at Object.dispatchError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxhr-utils.js:65:19)
at Request.client.on.err (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxmlhttprequest.js:676:20)
at emitOne (events.js:121:20)
at Request.emit (events.js:211:7)
at Request.onRequestError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesrequestrequest.js:881:8)
at emitOne (events.js:116:13)
at ClientRequest.emit (events.js:211:7)
at Socket.socketErrorListener (_http_client.js:387:9)
Anyone got an idea what the problem could be?
EDIT:
After I stopped using the HttpClientModule
and switched to the HttpClientTestingModule
the connection Error went away but now I have problem with the async call:
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
95 | function createdPatchedSpec(OriginalSpec, registry) {
96 | function PatchedSpec(attrs) {
> 97 | OriginalSpec.apply(this, arguments);
angular karma-jasmine jestjs
|
show 3 more comments
up vote
0
down vote
favorite
I am working on an Ionic/Angular project and tried switching from Karma to Jest for my testing.
The following test was running fine before the switch:
import {TestBed, inject, async} from '@angular/core/testing';
import { TreasureTableService } from './treasure-table.service';
import {PapaParseModule} from 'ngx-papaparse';
import {HttpClientModule} from '@angular/common/http';
describe('TreasureTableService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientModule, PapaParseModule]
});
});
beforeEach(inject([TreasureTableService], s => {
treasureTableService = s;
}));
it('should get treasure type list', async() => {
let result: string = await treasureTableService.getTreasureTypeList();
expect(result).toContain("A");
expect(result.length).toBe(33);
});
});
After I switched to Jest I got the following error:
console.error node_modules/jsdom/lib/jsdom/virtual-console.js:29
Error: Error: connect ECONNREFUSED 127.0.0.1:80
at Object.dispatchError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxhr-utils.js:65:19)
at Request.client.on.err (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxmlhttprequest.js:676:20)
at emitOne (events.js:121:20)
at Request.emit (events.js:211:7)
at Request.onRequestError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesrequestrequest.js:881:8)
at emitOne (events.js:116:13)
at ClientRequest.emit (events.js:211:7)
at Socket.socketErrorListener (_http_client.js:387:9)
Anyone got an idea what the problem could be?
EDIT:
After I stopped using the HttpClientModule
and switched to the HttpClientTestingModule
the connection Error went away but now I have problem with the async call:
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
95 | function createdPatchedSpec(OriginalSpec, registry) {
96 | function PatchedSpec(attrs) {
> 97 | OriginalSpec.apply(this, arguments);
angular karma-jasmine jestjs
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Actually jest doesn't run in any browser, it currently runs only injsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.
– Ludevik
Nov 9 at 12:58
1
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should useHttpClientTestingModule
and mock all request in your tests.
– Ludevik
Nov 9 at 14:27
|
show 3 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am working on an Ionic/Angular project and tried switching from Karma to Jest for my testing.
The following test was running fine before the switch:
import {TestBed, inject, async} from '@angular/core/testing';
import { TreasureTableService } from './treasure-table.service';
import {PapaParseModule} from 'ngx-papaparse';
import {HttpClientModule} from '@angular/common/http';
describe('TreasureTableService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientModule, PapaParseModule]
});
});
beforeEach(inject([TreasureTableService], s => {
treasureTableService = s;
}));
it('should get treasure type list', async() => {
let result: string = await treasureTableService.getTreasureTypeList();
expect(result).toContain("A");
expect(result.length).toBe(33);
});
});
After I switched to Jest I got the following error:
console.error node_modules/jsdom/lib/jsdom/virtual-console.js:29
Error: Error: connect ECONNREFUSED 127.0.0.1:80
at Object.dispatchError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxhr-utils.js:65:19)
at Request.client.on.err (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxmlhttprequest.js:676:20)
at emitOne (events.js:121:20)
at Request.emit (events.js:211:7)
at Request.onRequestError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesrequestrequest.js:881:8)
at emitOne (events.js:116:13)
at ClientRequest.emit (events.js:211:7)
at Socket.socketErrorListener (_http_client.js:387:9)
Anyone got an idea what the problem could be?
EDIT:
After I stopped using the HttpClientModule
and switched to the HttpClientTestingModule
the connection Error went away but now I have problem with the async call:
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
95 | function createdPatchedSpec(OriginalSpec, registry) {
96 | function PatchedSpec(attrs) {
> 97 | OriginalSpec.apply(this, arguments);
angular karma-jasmine jestjs
I am working on an Ionic/Angular project and tried switching from Karma to Jest for my testing.
The following test was running fine before the switch:
import {TestBed, inject, async} from '@angular/core/testing';
import { TreasureTableService } from './treasure-table.service';
import {PapaParseModule} from 'ngx-papaparse';
import {HttpClientModule} from '@angular/common/http';
describe('TreasureTableService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientModule, PapaParseModule]
});
});
beforeEach(inject([TreasureTableService], s => {
treasureTableService = s;
}));
it('should get treasure type list', async() => {
let result: string = await treasureTableService.getTreasureTypeList();
expect(result).toContain("A");
expect(result.length).toBe(33);
});
});
After I switched to Jest I got the following error:
console.error node_modules/jsdom/lib/jsdom/virtual-console.js:29
Error: Error: connect ECONNREFUSED 127.0.0.1:80
at Object.dispatchError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxhr-utils.js:65:19)
at Request.client.on.err (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesjsdomlibjsdomlivingxmlhttprequest.js:676:20)
at emitOne (events.js:121:20)
at Request.emit (events.js:211:7)
at Request.onRequestError (C:UsersfhuWebstormProjectsAcksAssistantnode_modulesrequestrequest.js:881:8)
at emitOne (events.js:116:13)
at ClientRequest.emit (events.js:211:7)
at Socket.socketErrorListener (_http_client.js:387:9)
Anyone got an idea what the problem could be?
EDIT:
After I stopped using the HttpClientModule
and switched to the HttpClientTestingModule
the connection Error went away but now I have problem with the async call:
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
95 | function createdPatchedSpec(OriginalSpec, registry) {
96 | function PatchedSpec(attrs) {
> 97 | OriginalSpec.apply(this, arguments);
angular karma-jasmine jestjs
angular karma-jasmine jestjs
edited Nov 10 at 22:25


Jonathan
5,07722153
5,07722153
asked Nov 9 at 7:09
Florian
134
134
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Actually jest doesn't run in any browser, it currently runs only injsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.
– Ludevik
Nov 9 at 12:58
1
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should useHttpClientTestingModule
and mock all request in your tests.
– Ludevik
Nov 9 at 14:27
|
show 3 more comments
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Actually jest doesn't run in any browser, it currently runs only injsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.
– Ludevik
Nov 9 at 12:58
1
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should useHttpClientTestingModule
and mock all request in your tests.
– Ludevik
Nov 9 at 14:27
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Actually jest doesn't run in any browser, it currently runs only in
jsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.– Ludevik
Nov 9 at 12:58
Actually jest doesn't run in any browser, it currently runs only in
jsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.– Ludevik
Nov 9 at 12:58
1
1
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should use
HttpClientTestingModule
and mock all request in your tests.– Ludevik
Nov 9 at 14:27
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should use
HttpClientTestingModule
and mock all request in your tests.– Ludevik
Nov 9 at 14:27
|
show 3 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53221240%2funit-test-stopped-working-when-switching-from-karma-to-jest%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZRET9 rsAue5 xr67vE,rk9Eb6y
For the note: There are Jest test that are running just fine so I think its not just a configuration problem.
– Florian
Nov 9 at 7:13
How could it work with karma? It seems that your test is doing http request to localhost. Did your localhost run when you ran your karma test?
– Ludevik
Nov 9 at 7:37
Yes, Karma did open a browser window. I thought that one of big advantages with Jest is that they can do it without an open browser by probably doing it in a headless chrome in the background.
– Florian
Nov 9 at 12:33
Actually jest doesn't run in any browser, it currently runs only in
jsdom
. My question was whether your dev server with your app was running when you ran your karma tests or not.– Ludevik
Nov 9 at 12:58
1
Hm, strange. Could you inspect how your karma test managed to correctly call the http request and trace it down? Nevertheless, your test should not do any xhr requests to any server at all. You should use
HttpClientTestingModule
and mock all request in your tests.– Ludevik
Nov 9 at 14:27