How to Read XML in .NET?
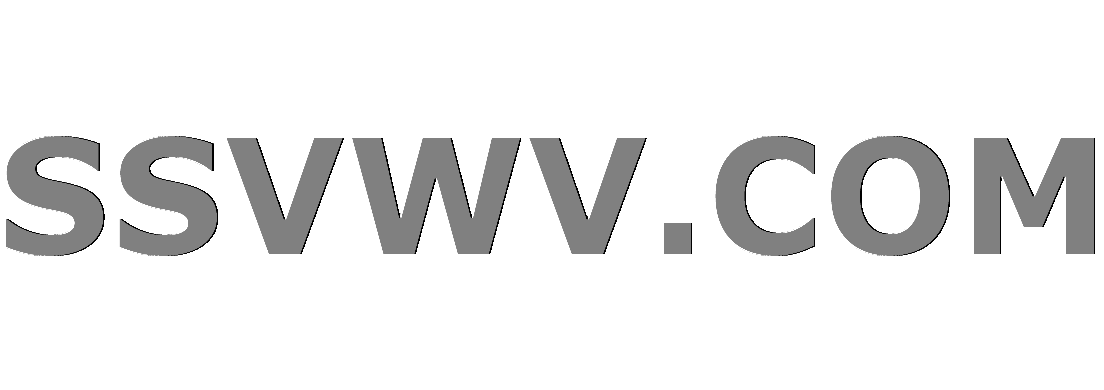
Multi tool use
up vote
26
down vote
favorite
XML noob here!
So I have some xml data:
<DataChunk>
<ResponseChunk>
<errors>
<error code="0">
Something happened here: Line 1, position 1.
</error>
</errors>
</ResponseChunk>
</DataChunk>
How would I get the a list of "errors" where I can get access to the "error code" and the text description following...?
Also, I'm using .net4.0 in c#...thanks!
c# xml
add a comment |
up vote
26
down vote
favorite
XML noob here!
So I have some xml data:
<DataChunk>
<ResponseChunk>
<errors>
<error code="0">
Something happened here: Line 1, position 1.
</error>
</errors>
</ResponseChunk>
</DataChunk>
How would I get the a list of "errors" where I can get access to the "error code" and the text description following...?
Also, I'm using .net4.0 in c#...thanks!
c# xml
1
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01
add a comment |
up vote
26
down vote
favorite
up vote
26
down vote
favorite
XML noob here!
So I have some xml data:
<DataChunk>
<ResponseChunk>
<errors>
<error code="0">
Something happened here: Line 1, position 1.
</error>
</errors>
</ResponseChunk>
</DataChunk>
How would I get the a list of "errors" where I can get access to the "error code" and the text description following...?
Also, I'm using .net4.0 in c#...thanks!
c# xml
XML noob here!
So I have some xml data:
<DataChunk>
<ResponseChunk>
<errors>
<error code="0">
Something happened here: Line 1, position 1.
</error>
</errors>
</ResponseChunk>
</DataChunk>
How would I get the a list of "errors" where I can get access to the "error code" and the text description following...?
Also, I'm using .net4.0 in c#...thanks!
c# xml
c# xml
edited Jan 20 '11 at 21:30
John Saunders
147k22202357
147k22202357
asked Jan 20 '11 at 21:27
sringer
3751414
3751414
1
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01
add a comment |
1
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01
1
1
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01
add a comment |
4 Answers
4
active
oldest
votes
up vote
48
down vote
accepted
Load the XML into an XmlDocument
and then use xpath queries to extract the data you need.
For example
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNode errorNode = doc.DocumentElement.SelectSingleNode("/DataChunk/ResponseChunk/Errors/error");
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
If there is potential for the XML having multiple error elements you can use SelectNodes
to get an XmlNodeList
that contains all elements at that xpath. For example:
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNodeList errorNodes = doc.DocumentElement.SelectNodes("/DataChunk/ResponseChunk/Errors/error");
foreach(XmlNode errorNode in errorNodes)
{
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
}
Option 2
If you have a XML schema for the XML you could bind the schema to a class (using the .NET xsd.exe tool). Once you have that you can deserialise the XML into an object and work with it from that object rather than the raw XML. This is an entire subject in itself so if you do have the schema it is worth looking into.
You haveSelectSingleNode
, while the OP wants a list of theerror
nodes.
– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
add a comment |
up vote
23
down vote
You can use Linq to XML:
var doc = XDocument.Parse(xml);
var errors = from e in doc.Descendants("error")
select new
{
code = e.Attribute("code").Value,
msg = e.Value.Trim()
};
foreach (var e in errors)
{
// use e.code & e.msg
}
If your input XML is very large however, it might be better to go through the document with an XMLReader
.
add a comment |
up vote
3
down vote
XmlReader xmlReader = XmlReader.Create(new StringReader(response));
AmortizationCalculatorBE amortization = new AmortizationCalculatorBE();
List<PaymentCalculator> paymentList = new List<PaymentCalculator>();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(new StringReader(response));
XmlNodeList nodeList = xmlDoc.DocumentElement.SelectNodes("response/amortizationschedule/payment");
XmlNodeList nodeList2 = xmlDoc.DocumentElement.SelectNodes("response");
foreach (XmlNode node in nodeList)
{
PaymentCalculator payment = new PaymentCalculator();
payment.beginningbalance = node.SelectSingleNode("beginningbalance").InnerText;
payment.principal = node.SelectSingleNode("principal").InnerText;
payment.interest = node.SelectSingleNode("interest").InnerText;
paymentList.Add(payment);
}
amortization._PaymentCalculator = paymentList;
foreach (XmlNode node in nodeList2)
{
amortization.totalprincipal = node.SelectSingleNode("totalprincipal").InnerText;
amortization.totalinterest = node.SelectSingleNode("totalinterest").InnerText;
}
add a comment |
up vote
0
down vote
using SelectNodes
with Cast
to IEnumerable<XmlElement>
type collection. then you can try to use linq
get your value.
XmlDocument doc = new XmlDocument();
doc.LoadXml(xml_str);
var errors = doc
.SelectNodes("/DataChunk/ResponseChunk/Errors/error")
.Cast<XmlElement>()
.Select(x => new {
errorCode = x.Attributes["code"].Value,
errorMessage = x.InnerText
});
foreach (var item in errors)
{
}
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
48
down vote
accepted
Load the XML into an XmlDocument
and then use xpath queries to extract the data you need.
For example
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNode errorNode = doc.DocumentElement.SelectSingleNode("/DataChunk/ResponseChunk/Errors/error");
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
If there is potential for the XML having multiple error elements you can use SelectNodes
to get an XmlNodeList
that contains all elements at that xpath. For example:
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNodeList errorNodes = doc.DocumentElement.SelectNodes("/DataChunk/ResponseChunk/Errors/error");
foreach(XmlNode errorNode in errorNodes)
{
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
}
Option 2
If you have a XML schema for the XML you could bind the schema to a class (using the .NET xsd.exe tool). Once you have that you can deserialise the XML into an object and work with it from that object rather than the raw XML. This is an entire subject in itself so if you do have the schema it is worth looking into.
You haveSelectSingleNode
, while the OP wants a list of theerror
nodes.
– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
add a comment |
up vote
48
down vote
accepted
Load the XML into an XmlDocument
and then use xpath queries to extract the data you need.
For example
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNode errorNode = doc.DocumentElement.SelectSingleNode("/DataChunk/ResponseChunk/Errors/error");
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
If there is potential for the XML having multiple error elements you can use SelectNodes
to get an XmlNodeList
that contains all elements at that xpath. For example:
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNodeList errorNodes = doc.DocumentElement.SelectNodes("/DataChunk/ResponseChunk/Errors/error");
foreach(XmlNode errorNode in errorNodes)
{
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
}
Option 2
If you have a XML schema for the XML you could bind the schema to a class (using the .NET xsd.exe tool). Once you have that you can deserialise the XML into an object and work with it from that object rather than the raw XML. This is an entire subject in itself so if you do have the schema it is worth looking into.
You haveSelectSingleNode
, while the OP wants a list of theerror
nodes.
– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
add a comment |
up vote
48
down vote
accepted
up vote
48
down vote
accepted
Load the XML into an XmlDocument
and then use xpath queries to extract the data you need.
For example
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNode errorNode = doc.DocumentElement.SelectSingleNode("/DataChunk/ResponseChunk/Errors/error");
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
If there is potential for the XML having multiple error elements you can use SelectNodes
to get an XmlNodeList
that contains all elements at that xpath. For example:
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNodeList errorNodes = doc.DocumentElement.SelectNodes("/DataChunk/ResponseChunk/Errors/error");
foreach(XmlNode errorNode in errorNodes)
{
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
}
Option 2
If you have a XML schema for the XML you could bind the schema to a class (using the .NET xsd.exe tool). Once you have that you can deserialise the XML into an object and work with it from that object rather than the raw XML. This is an entire subject in itself so if you do have the schema it is worth looking into.
Load the XML into an XmlDocument
and then use xpath queries to extract the data you need.
For example
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNode errorNode = doc.DocumentElement.SelectSingleNode("/DataChunk/ResponseChunk/Errors/error");
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
If there is potential for the XML having multiple error elements you can use SelectNodes
to get an XmlNodeList
that contains all elements at that xpath. For example:
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlstring);
XmlNodeList errorNodes = doc.DocumentElement.SelectNodes("/DataChunk/ResponseChunk/Errors/error");
foreach(XmlNode errorNode in errorNodes)
{
string errorCode = errorNode.Attributes["code"].Value;
string errorMessage = errorNode.InnerText;
}
Option 2
If you have a XML schema for the XML you could bind the schema to a class (using the .NET xsd.exe tool). Once you have that you can deserialise the XML into an object and work with it from that object rather than the raw XML. This is an entire subject in itself so if you do have the schema it is worth looking into.
edited Feb 19 '15 at 15:43
Kevin DiTraglia
18.6k1377114
18.6k1377114
answered Jan 20 '11 at 21:37
MrEyes
8,21774061
8,21774061
You haveSelectSingleNode
, while the OP wants a list of theerror
nodes.
– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
add a comment |
You haveSelectSingleNode
, while the OP wants a list of theerror
nodes.
– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
You have
SelectSingleNode
, while the OP wants a list of the error
nodes.– Gabe
Jan 20 '11 at 21:44
You have
SelectSingleNode
, while the OP wants a list of the error
nodes.– Gabe
Jan 20 '11 at 21:44
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@Gabe : But I also have "If there is potential for the XML having multiple error elements you can use SelectNodes to get an XmlNodeList that contains all elements at that xpath." - however added sample code for multiple error nodes.
– MrEyes
Jan 20 '11 at 21:46
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
@joey : thats what I get for half posting on SO half watching television from the sofa :)
– MrEyes
Jan 20 '11 at 21:48
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
+1 for a good answer after edits
– James Walford
Jan 20 '11 at 22:22
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
And that's why TV is bad ;-)
– Joey
Jan 20 '11 at 22:50
add a comment |
up vote
23
down vote
You can use Linq to XML:
var doc = XDocument.Parse(xml);
var errors = from e in doc.Descendants("error")
select new
{
code = e.Attribute("code").Value,
msg = e.Value.Trim()
};
foreach (var e in errors)
{
// use e.code & e.msg
}
If your input XML is very large however, it might be better to go through the document with an XMLReader
.
add a comment |
up vote
23
down vote
You can use Linq to XML:
var doc = XDocument.Parse(xml);
var errors = from e in doc.Descendants("error")
select new
{
code = e.Attribute("code").Value,
msg = e.Value.Trim()
};
foreach (var e in errors)
{
// use e.code & e.msg
}
If your input XML is very large however, it might be better to go through the document with an XMLReader
.
add a comment |
up vote
23
down vote
up vote
23
down vote
You can use Linq to XML:
var doc = XDocument.Parse(xml);
var errors = from e in doc.Descendants("error")
select new
{
code = e.Attribute("code").Value,
msg = e.Value.Trim()
};
foreach (var e in errors)
{
// use e.code & e.msg
}
If your input XML is very large however, it might be better to go through the document with an XMLReader
.
You can use Linq to XML:
var doc = XDocument.Parse(xml);
var errors = from e in doc.Descendants("error")
select new
{
code = e.Attribute("code").Value,
msg = e.Value.Trim()
};
foreach (var e in errors)
{
// use e.code & e.msg
}
If your input XML is very large however, it might be better to go through the document with an XMLReader
.
edited Jan 20 '11 at 21:49
answered Jan 20 '11 at 21:40
Josef Pfleger
67.1k148595
67.1k148595
add a comment |
add a comment |
up vote
3
down vote
XmlReader xmlReader = XmlReader.Create(new StringReader(response));
AmortizationCalculatorBE amortization = new AmortizationCalculatorBE();
List<PaymentCalculator> paymentList = new List<PaymentCalculator>();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(new StringReader(response));
XmlNodeList nodeList = xmlDoc.DocumentElement.SelectNodes("response/amortizationschedule/payment");
XmlNodeList nodeList2 = xmlDoc.DocumentElement.SelectNodes("response");
foreach (XmlNode node in nodeList)
{
PaymentCalculator payment = new PaymentCalculator();
payment.beginningbalance = node.SelectSingleNode("beginningbalance").InnerText;
payment.principal = node.SelectSingleNode("principal").InnerText;
payment.interest = node.SelectSingleNode("interest").InnerText;
paymentList.Add(payment);
}
amortization._PaymentCalculator = paymentList;
foreach (XmlNode node in nodeList2)
{
amortization.totalprincipal = node.SelectSingleNode("totalprincipal").InnerText;
amortization.totalinterest = node.SelectSingleNode("totalinterest").InnerText;
}
add a comment |
up vote
3
down vote
XmlReader xmlReader = XmlReader.Create(new StringReader(response));
AmortizationCalculatorBE amortization = new AmortizationCalculatorBE();
List<PaymentCalculator> paymentList = new List<PaymentCalculator>();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(new StringReader(response));
XmlNodeList nodeList = xmlDoc.DocumentElement.SelectNodes("response/amortizationschedule/payment");
XmlNodeList nodeList2 = xmlDoc.DocumentElement.SelectNodes("response");
foreach (XmlNode node in nodeList)
{
PaymentCalculator payment = new PaymentCalculator();
payment.beginningbalance = node.SelectSingleNode("beginningbalance").InnerText;
payment.principal = node.SelectSingleNode("principal").InnerText;
payment.interest = node.SelectSingleNode("interest").InnerText;
paymentList.Add(payment);
}
amortization._PaymentCalculator = paymentList;
foreach (XmlNode node in nodeList2)
{
amortization.totalprincipal = node.SelectSingleNode("totalprincipal").InnerText;
amortization.totalinterest = node.SelectSingleNode("totalinterest").InnerText;
}
add a comment |
up vote
3
down vote
up vote
3
down vote
XmlReader xmlReader = XmlReader.Create(new StringReader(response));
AmortizationCalculatorBE amortization = new AmortizationCalculatorBE();
List<PaymentCalculator> paymentList = new List<PaymentCalculator>();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(new StringReader(response));
XmlNodeList nodeList = xmlDoc.DocumentElement.SelectNodes("response/amortizationschedule/payment");
XmlNodeList nodeList2 = xmlDoc.DocumentElement.SelectNodes("response");
foreach (XmlNode node in nodeList)
{
PaymentCalculator payment = new PaymentCalculator();
payment.beginningbalance = node.SelectSingleNode("beginningbalance").InnerText;
payment.principal = node.SelectSingleNode("principal").InnerText;
payment.interest = node.SelectSingleNode("interest").InnerText;
paymentList.Add(payment);
}
amortization._PaymentCalculator = paymentList;
foreach (XmlNode node in nodeList2)
{
amortization.totalprincipal = node.SelectSingleNode("totalprincipal").InnerText;
amortization.totalinterest = node.SelectSingleNode("totalinterest").InnerText;
}
XmlReader xmlReader = XmlReader.Create(new StringReader(response));
AmortizationCalculatorBE amortization = new AmortizationCalculatorBE();
List<PaymentCalculator> paymentList = new List<PaymentCalculator>();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(new StringReader(response));
XmlNodeList nodeList = xmlDoc.DocumentElement.SelectNodes("response/amortizationschedule/payment");
XmlNodeList nodeList2 = xmlDoc.DocumentElement.SelectNodes("response");
foreach (XmlNode node in nodeList)
{
PaymentCalculator payment = new PaymentCalculator();
payment.beginningbalance = node.SelectSingleNode("beginningbalance").InnerText;
payment.principal = node.SelectSingleNode("principal").InnerText;
payment.interest = node.SelectSingleNode("interest").InnerText;
paymentList.Add(payment);
}
amortization._PaymentCalculator = paymentList;
foreach (XmlNode node in nodeList2)
{
amortization.totalprincipal = node.SelectSingleNode("totalprincipal").InnerText;
amortization.totalinterest = node.SelectSingleNode("totalinterest").InnerText;
}
answered Feb 19 '15 at 13:03


Mike Clark
1,433721
1,433721
add a comment |
add a comment |
up vote
0
down vote
using SelectNodes
with Cast
to IEnumerable<XmlElement>
type collection. then you can try to use linq
get your value.
XmlDocument doc = new XmlDocument();
doc.LoadXml(xml_str);
var errors = doc
.SelectNodes("/DataChunk/ResponseChunk/Errors/error")
.Cast<XmlElement>()
.Select(x => new {
errorCode = x.Attributes["code"].Value,
errorMessage = x.InnerText
});
foreach (var item in errors)
{
}
add a comment |
up vote
0
down vote
using SelectNodes
with Cast
to IEnumerable<XmlElement>
type collection. then you can try to use linq
get your value.
XmlDocument doc = new XmlDocument();
doc.LoadXml(xml_str);
var errors = doc
.SelectNodes("/DataChunk/ResponseChunk/Errors/error")
.Cast<XmlElement>()
.Select(x => new {
errorCode = x.Attributes["code"].Value,
errorMessage = x.InnerText
});
foreach (var item in errors)
{
}
add a comment |
up vote
0
down vote
up vote
0
down vote
using SelectNodes
with Cast
to IEnumerable<XmlElement>
type collection. then you can try to use linq
get your value.
XmlDocument doc = new XmlDocument();
doc.LoadXml(xml_str);
var errors = doc
.SelectNodes("/DataChunk/ResponseChunk/Errors/error")
.Cast<XmlElement>()
.Select(x => new {
errorCode = x.Attributes["code"].Value,
errorMessage = x.InnerText
});
foreach (var item in errors)
{
}
using SelectNodes
with Cast
to IEnumerable<XmlElement>
type collection. then you can try to use linq
get your value.
XmlDocument doc = new XmlDocument();
doc.LoadXml(xml_str);
var errors = doc
.SelectNodes("/DataChunk/ResponseChunk/Errors/error")
.Cast<XmlElement>()
.Select(x => new {
errorCode = x.Attributes["code"].Value,
errorMessage = x.InnerText
});
foreach (var item in errors)
{
}
answered Nov 10 at 22:47


D-Shih
24.1k61331
24.1k61331
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f4752796%2fhow-to-read-xml-in-net%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y8z444FZojO,v gpXD4a1 PqqMybbZRl,2HU A3I9,lk8Bc,U2HNqMXnpkboqaMUNCzKngz 6uPzPonCKTqrm1 fiXpvjpGTXXrA,aj9tG sv,q
1
I'm assuming this: ´<error code="0"> ´ isn't what your XML actually looks like? Those slashes will break it, is it just C# escaping you've cut and pasted?
– James Walford
Jan 20 '11 at 21:47
@james Yeah >.< I had the xml loaded into a string and copied heh
– sringer
Jan 20 '11 at 22:01