Placing random values in array
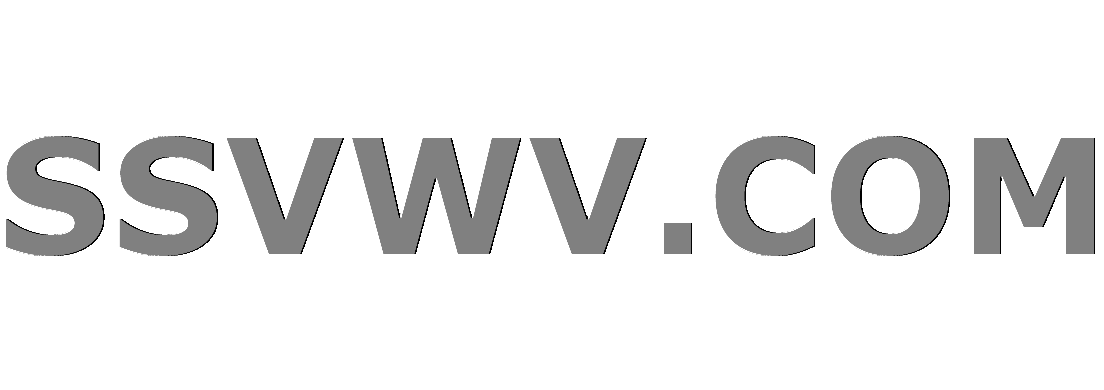
Multi tool use
up vote
2
down vote
favorite
I'm new to coding and debugging. As an assignment I'm trying to make a Nim game for two players. However whenever I reach a point in my code it give me a segmentation fault. I thought the problem would be something as pointing to a wrong place in an array, but changing those values did not help. Eventually I tried to debug using gdb, and it said that I had a segmentation fault at the line where I assign a random number to the array. I wouldn't know what to do with the information I got while debugging though. Here's the part where I assign random numbers from 1 to 6 into an array:
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
Surprisingly enough I only get the segmentation fault if I enter a 1 or a 10 as aantalrijen, but not when I enter anything inbetween. However the code still doesn;t work as it should when I do enter a number from 2 to 9. This is the entire code I have written up to now, even though it's not complete yet:
#include <stdio.h>
#include <stdlib.h>
//Initialiseren van speler namen, wiens beurt het is, de aantal rijen,
de aantal fiches op ieder rij, het totaal aantal fiches en de variant
die gespeeld wordt.
char speler1[10];
char speler2[10];
int geinitialiseerd;
int beurt;
int aantalrijen;
int fiches[10];
int fichesum;
int rijnemen;
int aantalnemen;
int variant;
int output () {
//For loop voor initialisatie van het spel. Hiermee wordt bedoelt dat x aantal rijen een random waarde krijgen tussen 1 en 6. Deze worden ook meteen geprint.
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
int main () {
//Introductie en vraag om namen
printf("==========================================================================================n**Welkom bij Nim! Dit is een spel gemaakt door Michael Francis voor twee spelers!t**n==========================================================================================n**Wat is de naam van speler 1?tttttttt**n");
scanf("%s", &speler1);
printf("**Wat is de naam van speler 2?tttttttt**n");
scanf("%s", &speler2);
printf("n**Kies het aantal rijen waarmee u wilt spelen (tussen de 1 en 10):ttt**n==========================================================================================n");
scanf("%d", &aantalrijen);
printf("==========================================================================================n**Kies de variant die u wilt spelen:ttttttt**nt1. Verliezer neemt laatste fichent2. Winnaar neemt laatste fichen==========================================================================================n");
scanf("%d", &variant);
printf("==========================================================================================n**Nieuw gegenereerd spel:tttttttt**n");
output();
printf("nn==========================================================================================n");
fichesum = 100;
while (fichesum != 0) {
//For-loop om huidige fiches uit te printen
//for (int a; a < aantalrijen; a++) {
// printf("ntRij %d:t", a+1);
// for (int b = 0; b < fiches[a]; b++) {
// printf(" @ ");
// }
//}
output();
fichesum = 0;
if (beurt == 0) {
printf("nn==========================================================================================n%s is aan de beurt!", speler1);
beurt = 1;
} else {
printf("nn==========================================================================================n%s is aan de beurt!", speler2);
beurt = 0;
}
printf("nUit welk rij wilt u fiches nemen?n");
scanf("%d", &rijnemen - 1);
printf("nHoeveel fiches wilt u nemen?n");
scanf("%d", &aantalnemen);
fiches[rijnemen] = fiches[rijnemen] - aantalnemen;
printf("%d", fiches[rijnemen]);
}
return 0;
}
c
add a comment |
up vote
2
down vote
favorite
I'm new to coding and debugging. As an assignment I'm trying to make a Nim game for two players. However whenever I reach a point in my code it give me a segmentation fault. I thought the problem would be something as pointing to a wrong place in an array, but changing those values did not help. Eventually I tried to debug using gdb, and it said that I had a segmentation fault at the line where I assign a random number to the array. I wouldn't know what to do with the information I got while debugging though. Here's the part where I assign random numbers from 1 to 6 into an array:
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
Surprisingly enough I only get the segmentation fault if I enter a 1 or a 10 as aantalrijen, but not when I enter anything inbetween. However the code still doesn;t work as it should when I do enter a number from 2 to 9. This is the entire code I have written up to now, even though it's not complete yet:
#include <stdio.h>
#include <stdlib.h>
//Initialiseren van speler namen, wiens beurt het is, de aantal rijen,
de aantal fiches op ieder rij, het totaal aantal fiches en de variant
die gespeeld wordt.
char speler1[10];
char speler2[10];
int geinitialiseerd;
int beurt;
int aantalrijen;
int fiches[10];
int fichesum;
int rijnemen;
int aantalnemen;
int variant;
int output () {
//For loop voor initialisatie van het spel. Hiermee wordt bedoelt dat x aantal rijen een random waarde krijgen tussen 1 en 6. Deze worden ook meteen geprint.
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
int main () {
//Introductie en vraag om namen
printf("==========================================================================================n**Welkom bij Nim! Dit is een spel gemaakt door Michael Francis voor twee spelers!t**n==========================================================================================n**Wat is de naam van speler 1?tttttttt**n");
scanf("%s", &speler1);
printf("**Wat is de naam van speler 2?tttttttt**n");
scanf("%s", &speler2);
printf("n**Kies het aantal rijen waarmee u wilt spelen (tussen de 1 en 10):ttt**n==========================================================================================n");
scanf("%d", &aantalrijen);
printf("==========================================================================================n**Kies de variant die u wilt spelen:ttttttt**nt1. Verliezer neemt laatste fichent2. Winnaar neemt laatste fichen==========================================================================================n");
scanf("%d", &variant);
printf("==========================================================================================n**Nieuw gegenereerd spel:tttttttt**n");
output();
printf("nn==========================================================================================n");
fichesum = 100;
while (fichesum != 0) {
//For-loop om huidige fiches uit te printen
//for (int a; a < aantalrijen; a++) {
// printf("ntRij %d:t", a+1);
// for (int b = 0; b < fiches[a]; b++) {
// printf(" @ ");
// }
//}
output();
fichesum = 0;
if (beurt == 0) {
printf("nn==========================================================================================n%s is aan de beurt!", speler1);
beurt = 1;
} else {
printf("nn==========================================================================================n%s is aan de beurt!", speler2);
beurt = 0;
}
printf("nUit welk rij wilt u fiches nemen?n");
scanf("%d", &rijnemen - 1);
printf("nHoeveel fiches wilt u nemen?n");
scanf("%d", &aantalnemen);
fiches[rijnemen] = fiches[rijnemen] - aantalnemen;
printf("%d", fiches[rijnemen]);
}
return 0;
}
c
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm new to coding and debugging. As an assignment I'm trying to make a Nim game for two players. However whenever I reach a point in my code it give me a segmentation fault. I thought the problem would be something as pointing to a wrong place in an array, but changing those values did not help. Eventually I tried to debug using gdb, and it said that I had a segmentation fault at the line where I assign a random number to the array. I wouldn't know what to do with the information I got while debugging though. Here's the part where I assign random numbers from 1 to 6 into an array:
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
Surprisingly enough I only get the segmentation fault if I enter a 1 or a 10 as aantalrijen, but not when I enter anything inbetween. However the code still doesn;t work as it should when I do enter a number from 2 to 9. This is the entire code I have written up to now, even though it's not complete yet:
#include <stdio.h>
#include <stdlib.h>
//Initialiseren van speler namen, wiens beurt het is, de aantal rijen,
de aantal fiches op ieder rij, het totaal aantal fiches en de variant
die gespeeld wordt.
char speler1[10];
char speler2[10];
int geinitialiseerd;
int beurt;
int aantalrijen;
int fiches[10];
int fichesum;
int rijnemen;
int aantalnemen;
int variant;
int output () {
//For loop voor initialisatie van het spel. Hiermee wordt bedoelt dat x aantal rijen een random waarde krijgen tussen 1 en 6. Deze worden ook meteen geprint.
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
int main () {
//Introductie en vraag om namen
printf("==========================================================================================n**Welkom bij Nim! Dit is een spel gemaakt door Michael Francis voor twee spelers!t**n==========================================================================================n**Wat is de naam van speler 1?tttttttt**n");
scanf("%s", &speler1);
printf("**Wat is de naam van speler 2?tttttttt**n");
scanf("%s", &speler2);
printf("n**Kies het aantal rijen waarmee u wilt spelen (tussen de 1 en 10):ttt**n==========================================================================================n");
scanf("%d", &aantalrijen);
printf("==========================================================================================n**Kies de variant die u wilt spelen:ttttttt**nt1. Verliezer neemt laatste fichent2. Winnaar neemt laatste fichen==========================================================================================n");
scanf("%d", &variant);
printf("==========================================================================================n**Nieuw gegenereerd spel:tttttttt**n");
output();
printf("nn==========================================================================================n");
fichesum = 100;
while (fichesum != 0) {
//For-loop om huidige fiches uit te printen
//for (int a; a < aantalrijen; a++) {
// printf("ntRij %d:t", a+1);
// for (int b = 0; b < fiches[a]; b++) {
// printf(" @ ");
// }
//}
output();
fichesum = 0;
if (beurt == 0) {
printf("nn==========================================================================================n%s is aan de beurt!", speler1);
beurt = 1;
} else {
printf("nn==========================================================================================n%s is aan de beurt!", speler2);
beurt = 0;
}
printf("nUit welk rij wilt u fiches nemen?n");
scanf("%d", &rijnemen - 1);
printf("nHoeveel fiches wilt u nemen?n");
scanf("%d", &aantalnemen);
fiches[rijnemen] = fiches[rijnemen] - aantalnemen;
printf("%d", fiches[rijnemen]);
}
return 0;
}
c
I'm new to coding and debugging. As an assignment I'm trying to make a Nim game for two players. However whenever I reach a point in my code it give me a segmentation fault. I thought the problem would be something as pointing to a wrong place in an array, but changing those values did not help. Eventually I tried to debug using gdb, and it said that I had a segmentation fault at the line where I assign a random number to the array. I wouldn't know what to do with the information I got while debugging though. Here's the part where I assign random numbers from 1 to 6 into an array:
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
Surprisingly enough I only get the segmentation fault if I enter a 1 or a 10 as aantalrijen, but not when I enter anything inbetween. However the code still doesn;t work as it should when I do enter a number from 2 to 9. This is the entire code I have written up to now, even though it's not complete yet:
#include <stdio.h>
#include <stdlib.h>
//Initialiseren van speler namen, wiens beurt het is, de aantal rijen,
de aantal fiches op ieder rij, het totaal aantal fiches en de variant
die gespeeld wordt.
char speler1[10];
char speler2[10];
int geinitialiseerd;
int beurt;
int aantalrijen;
int fiches[10];
int fichesum;
int rijnemen;
int aantalnemen;
int variant;
int output () {
//For loop voor initialisatie van het spel. Hiermee wordt bedoelt dat x aantal rijen een random waarde krijgen tussen 1 en 6. Deze worden ook meteen geprint.
for (int x; x < aantalrijen; x++) {
if (geinitialiseerd == 0) {
fiches[x] = rand()% 6 + 1 ;
geinitialiseerd = 1;
}
printf("ntRij %d:t", x+1);
for (int y = 0; y < fiches[x]; y++) {
printf(" @ ");
}
fichesum = fichesum + fiches[x];
}
}
int main () {
//Introductie en vraag om namen
printf("==========================================================================================n**Welkom bij Nim! Dit is een spel gemaakt door Michael Francis voor twee spelers!t**n==========================================================================================n**Wat is de naam van speler 1?tttttttt**n");
scanf("%s", &speler1);
printf("**Wat is de naam van speler 2?tttttttt**n");
scanf("%s", &speler2);
printf("n**Kies het aantal rijen waarmee u wilt spelen (tussen de 1 en 10):ttt**n==========================================================================================n");
scanf("%d", &aantalrijen);
printf("==========================================================================================n**Kies de variant die u wilt spelen:ttttttt**nt1. Verliezer neemt laatste fichent2. Winnaar neemt laatste fichen==========================================================================================n");
scanf("%d", &variant);
printf("==========================================================================================n**Nieuw gegenereerd spel:tttttttt**n");
output();
printf("nn==========================================================================================n");
fichesum = 100;
while (fichesum != 0) {
//For-loop om huidige fiches uit te printen
//for (int a; a < aantalrijen; a++) {
// printf("ntRij %d:t", a+1);
// for (int b = 0; b < fiches[a]; b++) {
// printf(" @ ");
// }
//}
output();
fichesum = 0;
if (beurt == 0) {
printf("nn==========================================================================================n%s is aan de beurt!", speler1);
beurt = 1;
} else {
printf("nn==========================================================================================n%s is aan de beurt!", speler2);
beurt = 0;
}
printf("nUit welk rij wilt u fiches nemen?n");
scanf("%d", &rijnemen - 1);
printf("nHoeveel fiches wilt u nemen?n");
scanf("%d", &aantalnemen);
fiches[rijnemen] = fiches[rijnemen] - aantalnemen;
printf("%d", fiches[rijnemen]);
}
return 0;
}
c
c
asked Nov 10 at 22:53
0963038
133
133
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
If you compile with -Wall -O2
, you'd get a warning on the for
loop in output
:
dut.c: In function ‘output’:
dut.c:21:11: warning: ‘x’ may be used uninitialized in this function [-Wmaybe-uninitialized]
for (int x; x < aantalrijen; x++) {
^
Change:
for (int x; x < aantalrijen; x++) {
Into:
for (int x = 0; x < aantalrijen; x++) {
Another warning to fix
Change:
scanf("%s", &speler1);
scanf("%s", &speler2);
Into:
scanf("%s", speler1);
scanf("%s", speler2);
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
If you compile with -Wall -O2
, you'd get a warning on the for
loop in output
:
dut.c: In function ‘output’:
dut.c:21:11: warning: ‘x’ may be used uninitialized in this function [-Wmaybe-uninitialized]
for (int x; x < aantalrijen; x++) {
^
Change:
for (int x; x < aantalrijen; x++) {
Into:
for (int x = 0; x < aantalrijen; x++) {
Another warning to fix
Change:
scanf("%s", &speler1);
scanf("%s", &speler2);
Into:
scanf("%s", speler1);
scanf("%s", speler2);
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
add a comment |
up vote
0
down vote
accepted
If you compile with -Wall -O2
, you'd get a warning on the for
loop in output
:
dut.c: In function ‘output’:
dut.c:21:11: warning: ‘x’ may be used uninitialized in this function [-Wmaybe-uninitialized]
for (int x; x < aantalrijen; x++) {
^
Change:
for (int x; x < aantalrijen; x++) {
Into:
for (int x = 0; x < aantalrijen; x++) {
Another warning to fix
Change:
scanf("%s", &speler1);
scanf("%s", &speler2);
Into:
scanf("%s", speler1);
scanf("%s", speler2);
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
If you compile with -Wall -O2
, you'd get a warning on the for
loop in output
:
dut.c: In function ‘output’:
dut.c:21:11: warning: ‘x’ may be used uninitialized in this function [-Wmaybe-uninitialized]
for (int x; x < aantalrijen; x++) {
^
Change:
for (int x; x < aantalrijen; x++) {
Into:
for (int x = 0; x < aantalrijen; x++) {
Another warning to fix
Change:
scanf("%s", &speler1);
scanf("%s", &speler2);
Into:
scanf("%s", speler1);
scanf("%s", speler2);
If you compile with -Wall -O2
, you'd get a warning on the for
loop in output
:
dut.c: In function ‘output’:
dut.c:21:11: warning: ‘x’ may be used uninitialized in this function [-Wmaybe-uninitialized]
for (int x; x < aantalrijen; x++) {
^
Change:
for (int x; x < aantalrijen; x++) {
Into:
for (int x = 0; x < aantalrijen; x++) {
Another warning to fix
Change:
scanf("%s", &speler1);
scanf("%s", &speler2);
Into:
scanf("%s", speler1);
scanf("%s", speler2);
answered Nov 10 at 23:33
Craig Estey
13.5k21029
13.5k21029
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
add a comment |
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
Awesome, that did the trick! Thank you very much!
– 0963038
Nov 11 at 13:22
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244216%2fplacing-random-values-in-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ea8xeZkh4GNm689e7,ofDFoh ngV0,sKf,X1Z