how to sum values from all objects within one dictionary in python
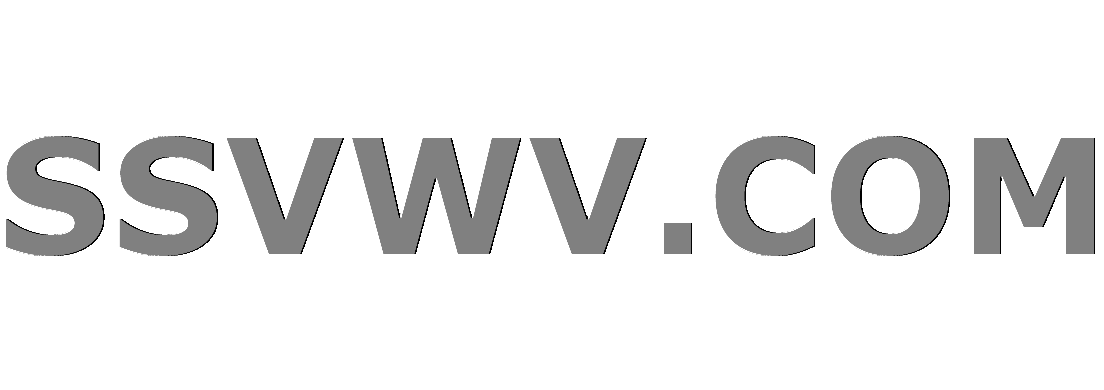
Multi tool use
up vote
-1
down vote
favorite
I have the following dict:
data = {
'test1': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
},
'test2': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
}
}
And what I would like to do is to sum all z2 objects within 'test1' and ,in this case, get 45
I know that I can do this by:
data['test1']['x1']['z2'] + data['test1']['x2']['z2']
but would like to know if there is a chance to take it in one code, for example
data['test1'][ * ]['z2']
where the star would represent all objects ( like in excel - this example doesn't work of course
Wondering if there is a better solution for this problem
Will be thankful for your support
python python-3.x
add a comment |
up vote
-1
down vote
favorite
I have the following dict:
data = {
'test1': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
},
'test2': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
}
}
And what I would like to do is to sum all z2 objects within 'test1' and ,in this case, get 45
I know that I can do this by:
data['test1']['x1']['z2'] + data['test1']['x2']['z2']
but would like to know if there is a chance to take it in one code, for example
data['test1'][ * ]['z2']
where the star would represent all objects ( like in excel - this example doesn't work of course
Wondering if there is a better solution for this problem
Will be thankful for your support
python python-3.x
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I have the following dict:
data = {
'test1': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
},
'test2': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
}
}
And what I would like to do is to sum all z2 objects within 'test1' and ,in this case, get 45
I know that I can do this by:
data['test1']['x1']['z2'] + data['test1']['x2']['z2']
but would like to know if there is a chance to take it in one code, for example
data['test1'][ * ]['z2']
where the star would represent all objects ( like in excel - this example doesn't work of course
Wondering if there is a better solution for this problem
Will be thankful for your support
python python-3.x
I have the following dict:
data = {
'test1': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
},
'test2': {
'x1': {
'z1': 22,
'z2': 11,
'z3': 21,
},
'x2': {
'z1': 15,
'z2': 34,
'z3': 54,
}
}
}
And what I would like to do is to sum all z2 objects within 'test1' and ,in this case, get 45
I know that I can do this by:
data['test1']['x1']['z2'] + data['test1']['x2']['z2']
but would like to know if there is a chance to take it in one code, for example
data['test1'][ * ]['z2']
where the star would represent all objects ( like in excel - this example doesn't work of course
Wondering if there is a better solution for this problem
Will be thankful for your support
python python-3.x
python python-3.x
asked Nov 10 at 16:03
akaribi
192
192
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
You can use sum
:
d = {'test1': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}, 'test2': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}}
result = sum(b['z2'] for a, b in d['test1'].items())
Output:
45
Edit: without an explicit loop, you can use reduce
:
from functools import reduce
new_result = reduce(lambda x, y:x+y['z2'], d['test1'].values(), 0)
Output:
45
1
Or just iterated['test1'].values()
?
– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
|
show 1 more comment
up vote
0
down vote
I think, Python's pandas
library would be a great to easily tackle this kind of problems.
Please have a look at the below code.
>>> import pandas as pd
>>>
>>> data = {
... 'test1': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... },
... 'test2': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... }
... }
>>>
>>> d = data["test1"]
>>> d
{'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}
>>>
>>> df = pd.DataFrame(list(d.values()), index=list(d.keys()))
>>> df
z1 z2 z3
x1 22 11 21
x2 15 34 54
>>>
>>> df.z2.sum() # 1st way
45
>>>
>>> df["z2"].sum() # 2nd way
45
>>>
References »
- https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.sum.html
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You can use sum
:
d = {'test1': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}, 'test2': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}}
result = sum(b['z2'] for a, b in d['test1'].items())
Output:
45
Edit: without an explicit loop, you can use reduce
:
from functools import reduce
new_result = reduce(lambda x, y:x+y['z2'], d['test1'].values(), 0)
Output:
45
1
Or just iterated['test1'].values()
?
– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
|
show 1 more comment
up vote
0
down vote
accepted
You can use sum
:
d = {'test1': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}, 'test2': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}}
result = sum(b['z2'] for a, b in d['test1'].items())
Output:
45
Edit: without an explicit loop, you can use reduce
:
from functools import reduce
new_result = reduce(lambda x, y:x+y['z2'], d['test1'].values(), 0)
Output:
45
1
Or just iterated['test1'].values()
?
– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
|
show 1 more comment
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You can use sum
:
d = {'test1': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}, 'test2': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}}
result = sum(b['z2'] for a, b in d['test1'].items())
Output:
45
Edit: without an explicit loop, you can use reduce
:
from functools import reduce
new_result = reduce(lambda x, y:x+y['z2'], d['test1'].values(), 0)
Output:
45
You can use sum
:
d = {'test1': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}, 'test2': {'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}}
result = sum(b['z2'] for a, b in d['test1'].items())
Output:
45
Edit: without an explicit loop, you can use reduce
:
from functools import reduce
new_result = reduce(lambda x, y:x+y['z2'], d['test1'].values(), 0)
Output:
45
edited Nov 10 at 16:10
answered Nov 10 at 16:05


Ajax1234
38.3k42351
38.3k42351
1
Or just iterated['test1'].values()
?
– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
|
show 1 more comment
1
Or just iterated['test1'].values()
?
– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
1
1
Or just iterate
d['test1'].values()
?– jpp
Nov 10 at 16:07
Or just iterate
d['test1'].values()
?– jpp
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
This is the option, but is there a way to do this without a loop ?
– akaribi
Nov 10 at 16:07
3
3
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi how do you imagine repeating an operation over multiple items without a loop would work? What exactly about this answer isn't suitable for you?
– Jon Clements♦
Nov 10 at 16:08
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
@akaribi Please see my recent edit.
– Ajax1234
Nov 10 at 16:10
thanks man ! perfect
– akaribi
Nov 10 at 16:19
thanks man ! perfect
– akaribi
Nov 10 at 16:19
|
show 1 more comment
up vote
0
down vote
I think, Python's pandas
library would be a great to easily tackle this kind of problems.
Please have a look at the below code.
>>> import pandas as pd
>>>
>>> data = {
... 'test1': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... },
... 'test2': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... }
... }
>>>
>>> d = data["test1"]
>>> d
{'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}
>>>
>>> df = pd.DataFrame(list(d.values()), index=list(d.keys()))
>>> df
z1 z2 z3
x1 22 11 21
x2 15 34 54
>>>
>>> df.z2.sum() # 1st way
45
>>>
>>> df["z2"].sum() # 2nd way
45
>>>
References »
- https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.sum.html
add a comment |
up vote
0
down vote
I think, Python's pandas
library would be a great to easily tackle this kind of problems.
Please have a look at the below code.
>>> import pandas as pd
>>>
>>> data = {
... 'test1': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... },
... 'test2': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... }
... }
>>>
>>> d = data["test1"]
>>> d
{'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}
>>>
>>> df = pd.DataFrame(list(d.values()), index=list(d.keys()))
>>> df
z1 z2 z3
x1 22 11 21
x2 15 34 54
>>>
>>> df.z2.sum() # 1st way
45
>>>
>>> df["z2"].sum() # 2nd way
45
>>>
References »
- https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.sum.html
add a comment |
up vote
0
down vote
up vote
0
down vote
I think, Python's pandas
library would be a great to easily tackle this kind of problems.
Please have a look at the below code.
>>> import pandas as pd
>>>
>>> data = {
... 'test1': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... },
... 'test2': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... }
... }
>>>
>>> d = data["test1"]
>>> d
{'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}
>>>
>>> df = pd.DataFrame(list(d.values()), index=list(d.keys()))
>>> df
z1 z2 z3
x1 22 11 21
x2 15 34 54
>>>
>>> df.z2.sum() # 1st way
45
>>>
>>> df["z2"].sum() # 2nd way
45
>>>
References »
- https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.sum.html
I think, Python's pandas
library would be a great to easily tackle this kind of problems.
Please have a look at the below code.
>>> import pandas as pd
>>>
>>> data = {
... 'test1': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... },
... 'test2': {
... 'x1': {
... 'z1': 22,
... 'z2': 11,
... 'z3': 21,
... },
... 'x2': {
... 'z1': 15,
... 'z2': 34,
... 'z3': 54,
... }
... }
... }
>>>
>>> d = data["test1"]
>>> d
{'x1': {'z1': 22, 'z2': 11, 'z3': 21}, 'x2': {'z1': 15, 'z2': 34, 'z3': 54}}
>>>
>>> df = pd.DataFrame(list(d.values()), index=list(d.keys()))
>>> df
z1 z2 z3
x1 22 11 21
x2 15 34 54
>>>
>>> df.z2.sum() # 1st way
45
>>>
>>> df["z2"].sum() # 2nd way
45
>>>
References »
- https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.sum.html
answered Nov 10 at 16:31


hygull
2,70311126
2,70311126
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240766%2fhow-to-sum-values-from-all-objects-within-one-dictionary-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
pWg7D e6,Boh3K4LoGHt6FU0WCmsLPBM9N9clck,idsTuJcJqegkJn eJAZEK4LRoif rMs pgpF