How to add a null character to the end of a string?
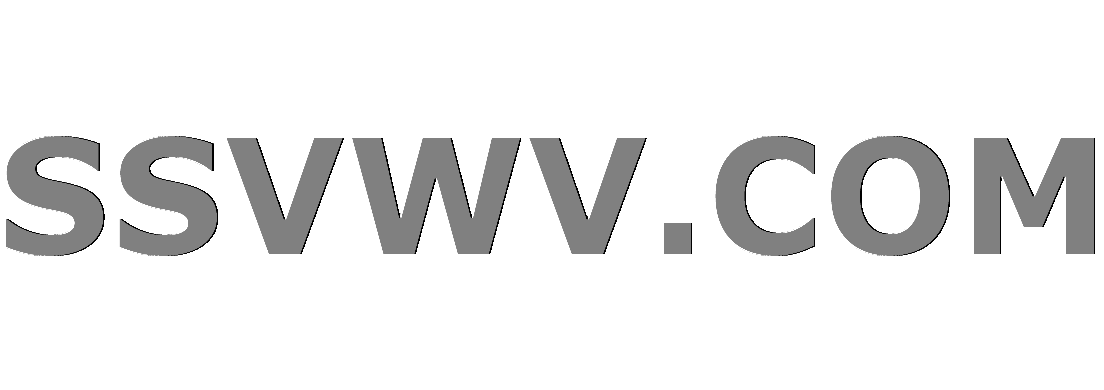
Multi tool use
up vote
-1
down vote
favorite
I start of with a
memcpy(g->db_cmd,l->db.param_value.val,l->db.param_value.len);
which contains the value "function" however I want a null character to be appended like "function''" I've tried a
memcpy(&g->db_cmd[l->db.param_value.len],0,1);
This crashes the program. I've tried memset also
memset(&g->db_cmd[l->db.param_value.len],0,1);
This doesnt work. Any idea?
c++ c
add a comment |
up vote
-1
down vote
favorite
I start of with a
memcpy(g->db_cmd,l->db.param_value.val,l->db.param_value.len);
which contains the value "function" however I want a null character to be appended like "function''" I've tried a
memcpy(&g->db_cmd[l->db.param_value.len],0,1);
This crashes the program. I've tried memset also
memset(&g->db_cmd[l->db.param_value.len],0,1);
This doesnt work. Any idea?
c++ c
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I start of with a
memcpy(g->db_cmd,l->db.param_value.val,l->db.param_value.len);
which contains the value "function" however I want a null character to be appended like "function''" I've tried a
memcpy(&g->db_cmd[l->db.param_value.len],0,1);
This crashes the program. I've tried memset also
memset(&g->db_cmd[l->db.param_value.len],0,1);
This doesnt work. Any idea?
c++ c
I start of with a
memcpy(g->db_cmd,l->db.param_value.val,l->db.param_value.len);
which contains the value "function" however I want a null character to be appended like "function''" I've tried a
memcpy(&g->db_cmd[l->db.param_value.len],0,1);
This crashes the program. I've tried memset also
memset(&g->db_cmd[l->db.param_value.len],0,1);
This doesnt work. Any idea?
c++ c
c++ c
edited Nov 10 at 16:00


PGCodeRider
2,0051624
2,0051624
asked Aug 4 '11 at 20:26
ken
1181410
1181410
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
up vote
4
down vote
g->db_cmd[l->db.param_value.len] = 0;
assuming you have allocated space for that.
add a comment |
up vote
4
down vote
First off, C (and C++) is not dynamic like you know it from Java, C#, PHP and others. When you are presented with a string in C, the string is pretty much static in length.
To make the answer simpler, lets redefine your variables:
g->db_cmd
will be calleddest
,
l->db.param_value.val
will be calledsrc
, and
l->db.param_value.len
will be calledlen
.
You should allocate a new string of size len
plus one (for the extra null).
Allocate a new dest
:
dest = calloc(sizeof(char), len + 1);
calloc allocates an array of chars as long as len
plus one. After calloc() has allocated the array it fills it with nulls (or ) thus you automatically will have a null appended to your dest
string.
Next, copy the src
to dest
with strncpy:
strncpy(dest, src, len);
To convert this back to your variable names:
g->db_cmd = calloc(sizeof(char), l->db.param_value.len + 1);
strncpy(g->db_cmd, l->db.param_value.val, l->db.param_value.len);
In my opinion,memcpy()
would be a more appropriate function to use thanstrncpy()
in this example. Unless it's possible that the length ofl->db.param_value.val
is actually less thanl->db.param_value.len
. In which case, that's a confusing set of data and/or member names.
– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
add a comment |
up vote
3
down vote
If you want string-copying semantics, why not use a string-copying function?
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code usesstrlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.
– Michael Burr
Aug 4 '11 at 21:32
add a comment |
up vote
0
down vote
Strings are by default null-terminated.
If you want a to ad an extra NULL at the end you can write "String"
or db_cmd[len]=''
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
add a comment |
up vote
0
down vote
If the source you're copying from also contains a NULL terminated string use
memcpy( g->db_cmd, l->db.param_value.val, l->db.param_value.len + 1 );
Otherwise you'll have to add the terminator yourself.
g->db_cmd[l->db.param_value.len] = '';
Of course, you need to ensure that the destination has enough room for this character.
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
add a comment |
up vote
0
down vote
memcpy takes two pointers, and an integer. In the lines that you say are crashing, you pass it a pointer, and two integers. The code cannot dereference the second argument (0).
If you really really want to use memcpy, you have to have a pointer to a zero
char zero = 0;
memcpy(&g->db_cmd[l->db.param_value.len], &zero , 1);
But I would really suggest pyroscope's answer. It's faster, and clearer.
add a comment |
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
g->db_cmd[l->db.param_value.len] = 0;
assuming you have allocated space for that.
add a comment |
up vote
4
down vote
g->db_cmd[l->db.param_value.len] = 0;
assuming you have allocated space for that.
add a comment |
up vote
4
down vote
up vote
4
down vote
g->db_cmd[l->db.param_value.len] = 0;
assuming you have allocated space for that.
g->db_cmd[l->db.param_value.len] = 0;
assuming you have allocated space for that.
answered Aug 4 '11 at 20:30
pyroscope
3,73211213
3,73211213
add a comment |
add a comment |
up vote
4
down vote
First off, C (and C++) is not dynamic like you know it from Java, C#, PHP and others. When you are presented with a string in C, the string is pretty much static in length.
To make the answer simpler, lets redefine your variables:
g->db_cmd
will be calleddest
,
l->db.param_value.val
will be calledsrc
, and
l->db.param_value.len
will be calledlen
.
You should allocate a new string of size len
plus one (for the extra null).
Allocate a new dest
:
dest = calloc(sizeof(char), len + 1);
calloc allocates an array of chars as long as len
plus one. After calloc() has allocated the array it fills it with nulls (or ) thus you automatically will have a null appended to your dest
string.
Next, copy the src
to dest
with strncpy:
strncpy(dest, src, len);
To convert this back to your variable names:
g->db_cmd = calloc(sizeof(char), l->db.param_value.len + 1);
strncpy(g->db_cmd, l->db.param_value.val, l->db.param_value.len);
In my opinion,memcpy()
would be a more appropriate function to use thanstrncpy()
in this example. Unless it's possible that the length ofl->db.param_value.val
is actually less thanl->db.param_value.len
. In which case, that's a confusing set of data and/or member names.
– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
add a comment |
up vote
4
down vote
First off, C (and C++) is not dynamic like you know it from Java, C#, PHP and others. When you are presented with a string in C, the string is pretty much static in length.
To make the answer simpler, lets redefine your variables:
g->db_cmd
will be calleddest
,
l->db.param_value.val
will be calledsrc
, and
l->db.param_value.len
will be calledlen
.
You should allocate a new string of size len
plus one (for the extra null).
Allocate a new dest
:
dest = calloc(sizeof(char), len + 1);
calloc allocates an array of chars as long as len
plus one. After calloc() has allocated the array it fills it with nulls (or ) thus you automatically will have a null appended to your dest
string.
Next, copy the src
to dest
with strncpy:
strncpy(dest, src, len);
To convert this back to your variable names:
g->db_cmd = calloc(sizeof(char), l->db.param_value.len + 1);
strncpy(g->db_cmd, l->db.param_value.val, l->db.param_value.len);
In my opinion,memcpy()
would be a more appropriate function to use thanstrncpy()
in this example. Unless it's possible that the length ofl->db.param_value.val
is actually less thanl->db.param_value.len
. In which case, that's a confusing set of data and/or member names.
– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
add a comment |
up vote
4
down vote
up vote
4
down vote
First off, C (and C++) is not dynamic like you know it from Java, C#, PHP and others. When you are presented with a string in C, the string is pretty much static in length.
To make the answer simpler, lets redefine your variables:
g->db_cmd
will be calleddest
,
l->db.param_value.val
will be calledsrc
, and
l->db.param_value.len
will be calledlen
.
You should allocate a new string of size len
plus one (for the extra null).
Allocate a new dest
:
dest = calloc(sizeof(char), len + 1);
calloc allocates an array of chars as long as len
plus one. After calloc() has allocated the array it fills it with nulls (or ) thus you automatically will have a null appended to your dest
string.
Next, copy the src
to dest
with strncpy:
strncpy(dest, src, len);
To convert this back to your variable names:
g->db_cmd = calloc(sizeof(char), l->db.param_value.len + 1);
strncpy(g->db_cmd, l->db.param_value.val, l->db.param_value.len);
First off, C (and C++) is not dynamic like you know it from Java, C#, PHP and others. When you are presented with a string in C, the string is pretty much static in length.
To make the answer simpler, lets redefine your variables:
g->db_cmd
will be calleddest
,
l->db.param_value.val
will be calledsrc
, and
l->db.param_value.len
will be calledlen
.
You should allocate a new string of size len
plus one (for the extra null).
Allocate a new dest
:
dest = calloc(sizeof(char), len + 1);
calloc allocates an array of chars as long as len
plus one. After calloc() has allocated the array it fills it with nulls (or ) thus you automatically will have a null appended to your dest
string.
Next, copy the src
to dest
with strncpy:
strncpy(dest, src, len);
To convert this back to your variable names:
g->db_cmd = calloc(sizeof(char), l->db.param_value.len + 1);
strncpy(g->db_cmd, l->db.param_value.val, l->db.param_value.len);
edited Aug 4 '11 at 21:14
Sean
2,2521726
2,2521726
answered Aug 4 '11 at 20:44
Martin Olsen
1,41611420
1,41611420
In my opinion,memcpy()
would be a more appropriate function to use thanstrncpy()
in this example. Unless it's possible that the length ofl->db.param_value.val
is actually less thanl->db.param_value.len
. In which case, that's a confusing set of data and/or member names.
– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
add a comment |
In my opinion,memcpy()
would be a more appropriate function to use thanstrncpy()
in this example. Unless it's possible that the length ofl->db.param_value.val
is actually less thanl->db.param_value.len
. In which case, that's a confusing set of data and/or member names.
– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
In my opinion,
memcpy()
would be a more appropriate function to use than strncpy()
in this example. Unless it's possible that the length of l->db.param_value.val
is actually less than l->db.param_value.len
. In which case, that's a confusing set of data and/or member names.– Michael Burr
Aug 4 '11 at 21:39
In my opinion,
memcpy()
would be a more appropriate function to use than strncpy()
in this example. Unless it's possible that the length of l->db.param_value.val
is actually less than l->db.param_value.len
. In which case, that's a confusing set of data and/or member names.– Michael Burr
Aug 4 '11 at 21:39
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
Michael: you are absolutely right, given there is an Len variable. That would probably also shave of a few cycles when not checking for null in src. And Ken mentions elsewhere that he has to use memcpy(), so I should probably just change it!
– Martin Olsen
Aug 4 '11 at 23:00
add a comment |
up vote
3
down vote
If you want string-copying semantics, why not use a string-copying function?
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code usesstrlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.
– Michael Burr
Aug 4 '11 at 21:32
add a comment |
up vote
3
down vote
If you want string-copying semantics, why not use a string-copying function?
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code usesstrlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.
– Michael Burr
Aug 4 '11 at 21:32
add a comment |
up vote
3
down vote
up vote
3
down vote
If you want string-copying semantics, why not use a string-copying function?
If you want string-copying semantics, why not use a string-copying function?
answered Aug 4 '11 at 20:31
ildjarn
55.7k7100184
55.7k7100184
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code usesstrlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.
– Michael Burr
Aug 4 '11 at 21:32
add a comment |
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code usesstrlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.
– Michael Burr
Aug 4 '11 at 21:32
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
cant use it i need to use memcpy
– ken
Aug 4 '11 at 20:32
4
4
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
@ken : Why though? If this is homework, then please tag your question as such.
– ildjarn
Aug 4 '11 at 20:33
1
1
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
Who says the source value is NUL terminated, esp. when it's a struct with a len attribute?
– pyroscope
Aug 4 '11 at 20:38
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
@pyroscope : Indeed, nobody said that. Vague questions sometimes yield incorrect answers -- that's just how it goes.
– ildjarn
Aug 4 '11 at 20:39
3
3
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code uses
strlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.– Michael Burr
Aug 4 '11 at 21:32
In this case it might be reasonable to assume the source and destination are null terminated (or should be) since another question by the OP using similar code uses
strlen()
all over the place: stackoverflow.com/questions/6931193/concatenate-with-memcpy However, maybe that's the problem the OP was having in that question... I think the OP needs to figure out what the data they're dealing with is supposed to be.– Michael Burr
Aug 4 '11 at 21:32
add a comment |
up vote
0
down vote
Strings are by default null-terminated.
If you want a to ad an extra NULL at the end you can write "String"
or db_cmd[len]=''
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
add a comment |
up vote
0
down vote
Strings are by default null-terminated.
If you want a to ad an extra NULL at the end you can write "String"
or db_cmd[len]=''
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
add a comment |
up vote
0
down vote
up vote
0
down vote
Strings are by default null-terminated.
If you want a to ad an extra NULL at the end you can write "String"
or db_cmd[len]=''
Strings are by default null-terminated.
If you want a to ad an extra NULL at the end you can write "String"
or db_cmd[len]=''
answered Aug 4 '11 at 20:34
cprogrammer
3,22432345
3,22432345
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
add a comment |
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
2
2
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
Strings may be null terminated or may not be. String literals are always null terminated. </pedantic>
– Billy ONeal
Aug 4 '11 at 20:37
1
1
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
@Billy : I thought C++ answerers were pedantic by default? ;-]
– ildjarn
Aug 4 '11 at 20:38
add a comment |
up vote
0
down vote
If the source you're copying from also contains a NULL terminated string use
memcpy( g->db_cmd, l->db.param_value.val, l->db.param_value.len + 1 );
Otherwise you'll have to add the terminator yourself.
g->db_cmd[l->db.param_value.len] = '';
Of course, you need to ensure that the destination has enough room for this character.
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
add a comment |
up vote
0
down vote
If the source you're copying from also contains a NULL terminated string use
memcpy( g->db_cmd, l->db.param_value.val, l->db.param_value.len + 1 );
Otherwise you'll have to add the terminator yourself.
g->db_cmd[l->db.param_value.len] = '';
Of course, you need to ensure that the destination has enough room for this character.
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
add a comment |
up vote
0
down vote
up vote
0
down vote
If the source you're copying from also contains a NULL terminated string use
memcpy( g->db_cmd, l->db.param_value.val, l->db.param_value.len + 1 );
Otherwise you'll have to add the terminator yourself.
g->db_cmd[l->db.param_value.len] = '';
Of course, you need to ensure that the destination has enough room for this character.
If the source you're copying from also contains a NULL terminated string use
memcpy( g->db_cmd, l->db.param_value.val, l->db.param_value.len + 1 );
Otherwise you'll have to add the terminator yourself.
g->db_cmd[l->db.param_value.len] = '';
Of course, you need to ensure that the destination has enough room for this character.
answered Aug 4 '11 at 20:40
Praetorian
86.5k10181266
86.5k10181266
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
add a comment |
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
Hey, good point the value i am copying does contain it but even with the +1 it does not append ??
– ken
Aug 4 '11 at 21:02
add a comment |
up vote
0
down vote
memcpy takes two pointers, and an integer. In the lines that you say are crashing, you pass it a pointer, and two integers. The code cannot dereference the second argument (0).
If you really really want to use memcpy, you have to have a pointer to a zero
char zero = 0;
memcpy(&g->db_cmd[l->db.param_value.len], &zero , 1);
But I would really suggest pyroscope's answer. It's faster, and clearer.
add a comment |
up vote
0
down vote
memcpy takes two pointers, and an integer. In the lines that you say are crashing, you pass it a pointer, and two integers. The code cannot dereference the second argument (0).
If you really really want to use memcpy, you have to have a pointer to a zero
char zero = 0;
memcpy(&g->db_cmd[l->db.param_value.len], &zero , 1);
But I would really suggest pyroscope's answer. It's faster, and clearer.
add a comment |
up vote
0
down vote
up vote
0
down vote
memcpy takes two pointers, and an integer. In the lines that you say are crashing, you pass it a pointer, and two integers. The code cannot dereference the second argument (0).
If you really really want to use memcpy, you have to have a pointer to a zero
char zero = 0;
memcpy(&g->db_cmd[l->db.param_value.len], &zero , 1);
But I would really suggest pyroscope's answer. It's faster, and clearer.
memcpy takes two pointers, and an integer. In the lines that you say are crashing, you pass it a pointer, and two integers. The code cannot dereference the second argument (0).
If you really really want to use memcpy, you have to have a pointer to a zero
char zero = 0;
memcpy(&g->db_cmd[l->db.param_value.len], &zero , 1);
But I would really suggest pyroscope's answer. It's faster, and clearer.
answered Aug 4 '11 at 23:21
Mooing Duck
46.1k1072126
46.1k1072126
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6948107%2fhow-to-add-a-null-character-to-the-end-of-a-string%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
eK9,tfQiHOpnOPVdHfwn7qiUF,yUTgDZQecADxFe5CcVEl2gjPkEWEHY,H,aAC 4GK,DW1QdeFoVK,oZSYw5Ys