C# >> Something with the numbers get scrambled up
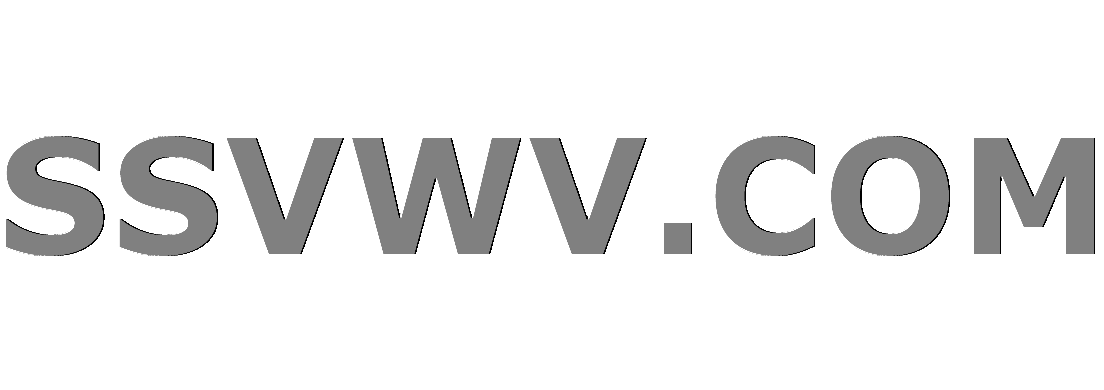
Multi tool use
up vote
-1
down vote
favorite
So this is what happens: I enter a number and what happens is that it writes out only 1 number: 324, like the variable c is getting this value for no reason..
class Program
{
static void Main(string args)
{
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
num = (int)num;
int c = 0;
Console.WriteLine(num);
while (num != 0)
{
num /= 10;
c++;
}
Console.WriteLine(c);
}
}
c#
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
-1
down vote
favorite
So this is what happens: I enter a number and what happens is that it writes out only 1 number: 324, like the variable c is getting this value for no reason..
class Program
{
static void Main(string args)
{
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
num = (int)num;
int c = 0;
Console.WriteLine(num);
while (num != 0)
{
num /= 10;
c++;
}
Console.WriteLine(c);
}
}
c#
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
You mean, as input you enter324
andc
become324
?
– Mehdi Dehghani
Nov 10 at 16:05
Try and print the values ofnum
within your loop. I think the calculation doesn't end when you think it should
– Hans Kesting
Nov 10 at 16:07
1
num
is a variable of typedouble
(a floating point number). How many times do you think thewhile
loop has to dividenum
by 10 to become zero?
– elgonzo
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
So this is what happens: I enter a number and what happens is that it writes out only 1 number: 324, like the variable c is getting this value for no reason..
class Program
{
static void Main(string args)
{
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
num = (int)num;
int c = 0;
Console.WriteLine(num);
while (num != 0)
{
num /= 10;
c++;
}
Console.WriteLine(c);
}
}
c#
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
So this is what happens: I enter a number and what happens is that it writes out only 1 number: 324, like the variable c is getting this value for no reason..
class Program
{
static void Main(string args)
{
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
num = (int)num;
int c = 0;
Console.WriteLine(num);
while (num != 0)
{
num /= 10;
c++;
}
Console.WriteLine(c);
}
}
c#
c#
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 10 at 16:09
D Stanley
122k9110168
122k9110168
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 10 at 16:02
Lidor Cohen
11
11
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Lidor Cohen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
You mean, as input you enter324
andc
become324
?
– Mehdi Dehghani
Nov 10 at 16:05
Try and print the values ofnum
within your loop. I think the calculation doesn't end when you think it should
– Hans Kesting
Nov 10 at 16:07
1
num
is a variable of typedouble
(a floating point number). How many times do you think thewhile
loop has to dividenum
by 10 to become zero?
– elgonzo
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08
add a comment |
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
You mean, as input you enter324
andc
become324
?
– Mehdi Dehghani
Nov 10 at 16:05
Try and print the values ofnum
within your loop. I think the calculation doesn't end when you think it should
– Hans Kesting
Nov 10 at 16:07
1
num
is a variable of typedouble
(a floating point number). How many times do you think thewhile
loop has to dividenum
by 10 to become zero?
– elgonzo
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
You mean, as input you enter
324
and c
become 324
?– Mehdi Dehghani
Nov 10 at 16:05
You mean, as input you enter
324
and c
become 324
?– Mehdi Dehghani
Nov 10 at 16:05
Try and print the values of
num
within your loop. I think the calculation doesn't end when you think it should– Hans Kesting
Nov 10 at 16:07
Try and print the values of
num
within your loop. I think the calculation doesn't end when you think it should– Hans Kesting
Nov 10 at 16:07
1
1
num
is a variable of type double
(a floating point number). How many times do you think the while
loop has to divide num
by 10 to become zero?– elgonzo
Nov 10 at 16:08
num
is a variable of type double
(a floating point number). How many times do you think the while
loop has to divide num
by 10 to become zero?– elgonzo
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08
add a comment |
2 Answers
2
active
oldest
votes
up vote
9
down vote
This program should loop infinitely if double
could represent every real number. Say you enter in 1
. Then the loop will divide it by 10, leaving 0.1
. Since 0.1
is not equal to 0
, the loop will continue, resulting in 0.01
, etc.
double
, however, only can support a minimum value of about 5E-324
, so if you get a number that small and try to divide it by 10, you'll get zero.
So your program loops about 324 times, and quits after it gets a small enough number.
Nice analysis and well explained.
– johey
Nov 10 at 16:10
add a comment |
up vote
0
down vote
Try printing out num
inside the loop. You'll see it tries to divide by 10 (division like this can never end in 0
) infinitely until it can no longer hold that small of a value and == 0
will return true
.
Just because you set num
to it's rounded value by using (int)num
doesn't mean it'll behave like an int
it'll still end up as a fractional when dividing, use an int
if you want it to be:
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
int num2 = (int)num;
int c = 0;
Console.WriteLine(num2);
while (num2 != 0)
{
num2 /= 10;
c++;
}
Console.WriteLine(c);
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
9
down vote
This program should loop infinitely if double
could represent every real number. Say you enter in 1
. Then the loop will divide it by 10, leaving 0.1
. Since 0.1
is not equal to 0
, the loop will continue, resulting in 0.01
, etc.
double
, however, only can support a minimum value of about 5E-324
, so if you get a number that small and try to divide it by 10, you'll get zero.
So your program loops about 324 times, and quits after it gets a small enough number.
Nice analysis and well explained.
– johey
Nov 10 at 16:10
add a comment |
up vote
9
down vote
This program should loop infinitely if double
could represent every real number. Say you enter in 1
. Then the loop will divide it by 10, leaving 0.1
. Since 0.1
is not equal to 0
, the loop will continue, resulting in 0.01
, etc.
double
, however, only can support a minimum value of about 5E-324
, so if you get a number that small and try to divide it by 10, you'll get zero.
So your program loops about 324 times, and quits after it gets a small enough number.
Nice analysis and well explained.
– johey
Nov 10 at 16:10
add a comment |
up vote
9
down vote
up vote
9
down vote
This program should loop infinitely if double
could represent every real number. Say you enter in 1
. Then the loop will divide it by 10, leaving 0.1
. Since 0.1
is not equal to 0
, the loop will continue, resulting in 0.01
, etc.
double
, however, only can support a minimum value of about 5E-324
, so if you get a number that small and try to divide it by 10, you'll get zero.
So your program loops about 324 times, and quits after it gets a small enough number.
This program should loop infinitely if double
could represent every real number. Say you enter in 1
. Then the loop will divide it by 10, leaving 0.1
. Since 0.1
is not equal to 0
, the loop will continue, resulting in 0.01
, etc.
double
, however, only can support a minimum value of about 5E-324
, so if you get a number that small and try to divide it by 10, you'll get zero.
So your program loops about 324 times, and quits after it gets a small enough number.
answered Nov 10 at 16:08
D Stanley
122k9110168
122k9110168
Nice analysis and well explained.
– johey
Nov 10 at 16:10
add a comment |
Nice analysis and well explained.
– johey
Nov 10 at 16:10
Nice analysis and well explained.
– johey
Nov 10 at 16:10
Nice analysis and well explained.
– johey
Nov 10 at 16:10
add a comment |
up vote
0
down vote
Try printing out num
inside the loop. You'll see it tries to divide by 10 (division like this can never end in 0
) infinitely until it can no longer hold that small of a value and == 0
will return true
.
Just because you set num
to it's rounded value by using (int)num
doesn't mean it'll behave like an int
it'll still end up as a fractional when dividing, use an int
if you want it to be:
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
int num2 = (int)num;
int c = 0;
Console.WriteLine(num2);
while (num2 != 0)
{
num2 /= 10;
c++;
}
Console.WriteLine(c);
add a comment |
up vote
0
down vote
Try printing out num
inside the loop. You'll see it tries to divide by 10 (division like this can never end in 0
) infinitely until it can no longer hold that small of a value and == 0
will return true
.
Just because you set num
to it's rounded value by using (int)num
doesn't mean it'll behave like an int
it'll still end up as a fractional when dividing, use an int
if you want it to be:
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
int num2 = (int)num;
int c = 0;
Console.WriteLine(num2);
while (num2 != 0)
{
num2 /= 10;
c++;
}
Console.WriteLine(c);
add a comment |
up vote
0
down vote
up vote
0
down vote
Try printing out num
inside the loop. You'll see it tries to divide by 10 (division like this can never end in 0
) infinitely until it can no longer hold that small of a value and == 0
will return true
.
Just because you set num
to it's rounded value by using (int)num
doesn't mean it'll behave like an int
it'll still end up as a fractional when dividing, use an int
if you want it to be:
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
int num2 = (int)num;
int c = 0;
Console.WriteLine(num2);
while (num2 != 0)
{
num2 /= 10;
c++;
}
Console.WriteLine(c);
Try printing out num
inside the loop. You'll see it tries to divide by 10 (division like this can never end in 0
) infinitely until it can no longer hold that small of a value and == 0
will return true
.
Just because you set num
to it's rounded value by using (int)num
doesn't mean it'll behave like an int
it'll still end up as a fractional when dividing, use an int
if you want it to be:
Console.WriteLine("enter a number with change");
double num = double.Parse(Console.ReadLine());
int num2 = (int)num;
int c = 0;
Console.WriteLine(num2);
while (num2 != 0)
{
num2 /= 10;
c++;
}
Console.WriteLine(c);
answered Nov 10 at 16:10


Sombrero Chicken
22.3k32772
22.3k32772
add a comment |
add a comment |
Lidor Cohen is a new contributor. Be nice, and check out our Code of Conduct.
Lidor Cohen is a new contributor. Be nice, and check out our Code of Conduct.
Lidor Cohen is a new contributor. Be nice, and check out our Code of Conduct.
Lidor Cohen is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240757%2fc-sharp-something-with-the-numbers-get-scrambled-up%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
HJDu4x Pje dj1ZaX,ORJCAoRPbvZZEEwdh0hKQpNej9fvx9jUcXh
What number are you entering? What output are you expecting?
– Carcigenicate
Nov 10 at 16:04
You mean, as input you enter
324
andc
become324
?– Mehdi Dehghani
Nov 10 at 16:05
Try and print the values of
num
within your loop. I think the calculation doesn't end when you think it should– Hans Kesting
Nov 10 at 16:07
1
num
is a variable of typedouble
(a floating point number). How many times do you think thewhile
loop has to dividenum
by 10 to become zero?– elgonzo
Nov 10 at 16:08
What is this program supposed to do?
– Mureinik
Nov 10 at 16:08