Is there a way to print all of a certain element of a class that is in a list?
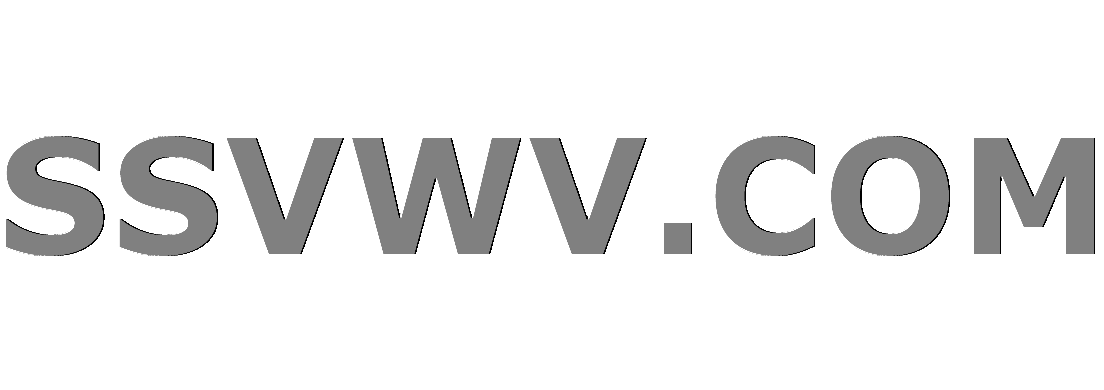
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
The title is terrible, but hopefully I can explain in my post. Creating a little game as my pet project for python, and I'm currently creating the inventory. Everything was... ok when developing the game until it came to making the function that will show all of the player's inventory.
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
Ok, so I created a list that basically has a bunch of classes from Items in it. To call on the name element of these classes I have to do something like this, otherwise I just get its memory location which is largely useless to the player. I've tried
elif (prompt == "examine"):
print(inventory.name)
gameprompt()
Thought that this above example would print only the name of all the Item objects, but there's a compilation error instead because I didn't specify which one. So I then tried~
elif (prompt == "examine"):
print(inventory[1:1000].name)
gameprompt()
Thinking that it would print all of the Item objects names up to 1000, but I obviously don't have that so I thought it would print the names up to the latest object that was there and stop but there was another compilation error from this...
If there is anyway to print out an element of a class for all class objects in a list please let me know. The full code of this game is here, although I don't think you'll need it to help me solve my problem (it is also very large.)
playername = input("What is your name?")
zone = 1
movement = 0
restcounter = 0
searchcounter = 0
class Player:
def __init__(self, name, hp, mp, atk, xp, dodgerate, atkrate):
self.name = playername
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
self.dodgerate = dodgerate
self.atkrate = atkrate
class Enemy(Player):
def __init__(self, name, gold, maxhp, hp, mp, atk, xp):
self.name = name
self.gold = gold
self.maxhp = maxhp
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
class Items:
def __init__(self, name, quantity, description, price, weight):
self.name = name
self.quantity = quantity
self.description = description
self.price = price
self.weight = weight
Player = Player(playername, 1, 1, 1, 1, 25, 3)
print(Player.name + " has been created. ")
def raceselection():
raceinput = input("Do you float towards the TEMPLE, CAVE or FOREST?")
if raceinput == "TEMPLE":
print("You are now a high elf. High elves utlize a lot of magical power at the cost of being very frail.")
Player.hp = Player.hp + 24
Player.mp = Player.mp + 100
Player.atk = Player.atk + 50
print("You awaken from your slumber. Your room's walls are gold plated, and you rested on a flat board.")
print("Out the door, you see many elves with robes praying to some goddess.")
print("You walk out of your door and into the praying area. You are immediately greeted by a tall man.")
elif raceinput == "CAVE":
print("You are now an orc.")
Player.hp = Player.hp + 1000
Player.mp = Player.mp + 15
Player.atk = Player.atk + 50
print("cave")
elif raceinput == "FOREST":
print("You are now a human.")
Player.hp = Player.hp + 50
Player.mp = Player.mp + 25
Player.atk = Player.atk + 25
else:
print("You can't float there!")
raceselection()
raceselection()
inventory =
def gameprompt():
global inventory
global zone
global movement
global restcounter
global searchcounter
if (movement == 5):
movement = movement - movement
zone = zone + 1
print("You have advanced to zone",zone,"!!!")
gameprompt()
if (zone == 1):
print("Welcome to the first zone! Easy enemies are here with not very good loot./fix grammar, add description of zone/")
elif (zone == 2):
print("Hey, it actually travelled to the second zone, awesome!")
elif (zone == 3):
print("No way would this actually work!")
prompt = input("Would you like to walk, search or rest?: ")
if (prompt == "walk"):
encounterchance = random.randint(1, 3)
if (encounterchance == 2):
if (zone == 1):
mobspawnrate = random.randint(1,3)
if (mobspawnrate == 1):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 2):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 3):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
else:
movement = movement + 1
print("You have walked a step. You are now at ",movement," steps")
gameprompt()
elif (prompt == "search"):
if (searchcounter == 3):
print("You cannot search this area anymore! Wait until you reach the next zone!")
gameprompt()
else:
searchchance = random.randint(1, 5)
if (searchchance == 1 or 2 or 3 or 4):
searchcounter = searchcounter + 1
print(searchcounter)
print("You have found something!")
searchchance = random.randint(1,4)
if (searchchance == 1 or 2):
inventory.append(Items("Old Boot", 1, "An old smelly boot. It's a mystery as to who it belongs to...", 5, 50))
print("You have found a Boot!")
print(inventory)
elif(searchchance == 3):
inventory.append(Items("Shiny Boot", 1, "Looks like a boot that was lightly worn. You could still wear this.", 5, 50))
print(inventory)
print("You have found a Shiny Boot!")
elif(searchchance == 4):
inventory.append(Items("Golden Boot", 1, "It's too heavy to wear, but it looks like it could sell for a fortune!", 5, 50))
print("You have found a Golden Boot?")
print(inventory)
else:
searchcounter = searchcounter + 1
print(searchcounter)
print("You did not find anything of value")
gameprompt()
elif (prompt == "rest"):
if (restcounter == 1):
print("Wait until you reach the next zone to rest again!")
gameprompt()
else:
# Add a MaxHP value to the player later, and the command rest will give 25% of that HP back.
Player.hp = Player.hp + (Player.hp / 5)
print("You have restored ",(Player.hp / 5)," hit points!")
restcounter = restcounter + 1
gameprompt()
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
gameprompt()
python python-3.x
add a comment |
The title is terrible, but hopefully I can explain in my post. Creating a little game as my pet project for python, and I'm currently creating the inventory. Everything was... ok when developing the game until it came to making the function that will show all of the player's inventory.
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
Ok, so I created a list that basically has a bunch of classes from Items in it. To call on the name element of these classes I have to do something like this, otherwise I just get its memory location which is largely useless to the player. I've tried
elif (prompt == "examine"):
print(inventory.name)
gameprompt()
Thought that this above example would print only the name of all the Item objects, but there's a compilation error instead because I didn't specify which one. So I then tried~
elif (prompt == "examine"):
print(inventory[1:1000].name)
gameprompt()
Thinking that it would print all of the Item objects names up to 1000, but I obviously don't have that so I thought it would print the names up to the latest object that was there and stop but there was another compilation error from this...
If there is anyway to print out an element of a class for all class objects in a list please let me know. The full code of this game is here, although I don't think you'll need it to help me solve my problem (it is also very large.)
playername = input("What is your name?")
zone = 1
movement = 0
restcounter = 0
searchcounter = 0
class Player:
def __init__(self, name, hp, mp, atk, xp, dodgerate, atkrate):
self.name = playername
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
self.dodgerate = dodgerate
self.atkrate = atkrate
class Enemy(Player):
def __init__(self, name, gold, maxhp, hp, mp, atk, xp):
self.name = name
self.gold = gold
self.maxhp = maxhp
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
class Items:
def __init__(self, name, quantity, description, price, weight):
self.name = name
self.quantity = quantity
self.description = description
self.price = price
self.weight = weight
Player = Player(playername, 1, 1, 1, 1, 25, 3)
print(Player.name + " has been created. ")
def raceselection():
raceinput = input("Do you float towards the TEMPLE, CAVE or FOREST?")
if raceinput == "TEMPLE":
print("You are now a high elf. High elves utlize a lot of magical power at the cost of being very frail.")
Player.hp = Player.hp + 24
Player.mp = Player.mp + 100
Player.atk = Player.atk + 50
print("You awaken from your slumber. Your room's walls are gold plated, and you rested on a flat board.")
print("Out the door, you see many elves with robes praying to some goddess.")
print("You walk out of your door and into the praying area. You are immediately greeted by a tall man.")
elif raceinput == "CAVE":
print("You are now an orc.")
Player.hp = Player.hp + 1000
Player.mp = Player.mp + 15
Player.atk = Player.atk + 50
print("cave")
elif raceinput == "FOREST":
print("You are now a human.")
Player.hp = Player.hp + 50
Player.mp = Player.mp + 25
Player.atk = Player.atk + 25
else:
print("You can't float there!")
raceselection()
raceselection()
inventory =
def gameprompt():
global inventory
global zone
global movement
global restcounter
global searchcounter
if (movement == 5):
movement = movement - movement
zone = zone + 1
print("You have advanced to zone",zone,"!!!")
gameprompt()
if (zone == 1):
print("Welcome to the first zone! Easy enemies are here with not very good loot./fix grammar, add description of zone/")
elif (zone == 2):
print("Hey, it actually travelled to the second zone, awesome!")
elif (zone == 3):
print("No way would this actually work!")
prompt = input("Would you like to walk, search or rest?: ")
if (prompt == "walk"):
encounterchance = random.randint(1, 3)
if (encounterchance == 2):
if (zone == 1):
mobspawnrate = random.randint(1,3)
if (mobspawnrate == 1):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 2):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 3):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
else:
movement = movement + 1
print("You have walked a step. You are now at ",movement," steps")
gameprompt()
elif (prompt == "search"):
if (searchcounter == 3):
print("You cannot search this area anymore! Wait until you reach the next zone!")
gameprompt()
else:
searchchance = random.randint(1, 5)
if (searchchance == 1 or 2 or 3 or 4):
searchcounter = searchcounter + 1
print(searchcounter)
print("You have found something!")
searchchance = random.randint(1,4)
if (searchchance == 1 or 2):
inventory.append(Items("Old Boot", 1, "An old smelly boot. It's a mystery as to who it belongs to...", 5, 50))
print("You have found a Boot!")
print(inventory)
elif(searchchance == 3):
inventory.append(Items("Shiny Boot", 1, "Looks like a boot that was lightly worn. You could still wear this.", 5, 50))
print(inventory)
print("You have found a Shiny Boot!")
elif(searchchance == 4):
inventory.append(Items("Golden Boot", 1, "It's too heavy to wear, but it looks like it could sell for a fortune!", 5, 50))
print("You have found a Golden Boot?")
print(inventory)
else:
searchcounter = searchcounter + 1
print(searchcounter)
print("You did not find anything of value")
gameprompt()
elif (prompt == "rest"):
if (restcounter == 1):
print("Wait until you reach the next zone to rest again!")
gameprompt()
else:
# Add a MaxHP value to the player later, and the command rest will give 25% of that HP back.
Player.hp = Player.hp + (Player.hp / 5)
print("You have restored ",(Player.hp / 5)," hit points!")
restcounter = restcounter + 1
gameprompt()
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
gameprompt()
python python-3.x
Something likeprint([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18
add a comment |
The title is terrible, but hopefully I can explain in my post. Creating a little game as my pet project for python, and I'm currently creating the inventory. Everything was... ok when developing the game until it came to making the function that will show all of the player's inventory.
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
Ok, so I created a list that basically has a bunch of classes from Items in it. To call on the name element of these classes I have to do something like this, otherwise I just get its memory location which is largely useless to the player. I've tried
elif (prompt == "examine"):
print(inventory.name)
gameprompt()
Thought that this above example would print only the name of all the Item objects, but there's a compilation error instead because I didn't specify which one. So I then tried~
elif (prompt == "examine"):
print(inventory[1:1000].name)
gameprompt()
Thinking that it would print all of the Item objects names up to 1000, but I obviously don't have that so I thought it would print the names up to the latest object that was there and stop but there was another compilation error from this...
If there is anyway to print out an element of a class for all class objects in a list please let me know. The full code of this game is here, although I don't think you'll need it to help me solve my problem (it is also very large.)
playername = input("What is your name?")
zone = 1
movement = 0
restcounter = 0
searchcounter = 0
class Player:
def __init__(self, name, hp, mp, atk, xp, dodgerate, atkrate):
self.name = playername
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
self.dodgerate = dodgerate
self.atkrate = atkrate
class Enemy(Player):
def __init__(self, name, gold, maxhp, hp, mp, atk, xp):
self.name = name
self.gold = gold
self.maxhp = maxhp
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
class Items:
def __init__(self, name, quantity, description, price, weight):
self.name = name
self.quantity = quantity
self.description = description
self.price = price
self.weight = weight
Player = Player(playername, 1, 1, 1, 1, 25, 3)
print(Player.name + " has been created. ")
def raceselection():
raceinput = input("Do you float towards the TEMPLE, CAVE or FOREST?")
if raceinput == "TEMPLE":
print("You are now a high elf. High elves utlize a lot of magical power at the cost of being very frail.")
Player.hp = Player.hp + 24
Player.mp = Player.mp + 100
Player.atk = Player.atk + 50
print("You awaken from your slumber. Your room's walls are gold plated, and you rested on a flat board.")
print("Out the door, you see many elves with robes praying to some goddess.")
print("You walk out of your door and into the praying area. You are immediately greeted by a tall man.")
elif raceinput == "CAVE":
print("You are now an orc.")
Player.hp = Player.hp + 1000
Player.mp = Player.mp + 15
Player.atk = Player.atk + 50
print("cave")
elif raceinput == "FOREST":
print("You are now a human.")
Player.hp = Player.hp + 50
Player.mp = Player.mp + 25
Player.atk = Player.atk + 25
else:
print("You can't float there!")
raceselection()
raceselection()
inventory =
def gameprompt():
global inventory
global zone
global movement
global restcounter
global searchcounter
if (movement == 5):
movement = movement - movement
zone = zone + 1
print("You have advanced to zone",zone,"!!!")
gameprompt()
if (zone == 1):
print("Welcome to the first zone! Easy enemies are here with not very good loot./fix grammar, add description of zone/")
elif (zone == 2):
print("Hey, it actually travelled to the second zone, awesome!")
elif (zone == 3):
print("No way would this actually work!")
prompt = input("Would you like to walk, search or rest?: ")
if (prompt == "walk"):
encounterchance = random.randint(1, 3)
if (encounterchance == 2):
if (zone == 1):
mobspawnrate = random.randint(1,3)
if (mobspawnrate == 1):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 2):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 3):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
else:
movement = movement + 1
print("You have walked a step. You are now at ",movement," steps")
gameprompt()
elif (prompt == "search"):
if (searchcounter == 3):
print("You cannot search this area anymore! Wait until you reach the next zone!")
gameprompt()
else:
searchchance = random.randint(1, 5)
if (searchchance == 1 or 2 or 3 or 4):
searchcounter = searchcounter + 1
print(searchcounter)
print("You have found something!")
searchchance = random.randint(1,4)
if (searchchance == 1 or 2):
inventory.append(Items("Old Boot", 1, "An old smelly boot. It's a mystery as to who it belongs to...", 5, 50))
print("You have found a Boot!")
print(inventory)
elif(searchchance == 3):
inventory.append(Items("Shiny Boot", 1, "Looks like a boot that was lightly worn. You could still wear this.", 5, 50))
print(inventory)
print("You have found a Shiny Boot!")
elif(searchchance == 4):
inventory.append(Items("Golden Boot", 1, "It's too heavy to wear, but it looks like it could sell for a fortune!", 5, 50))
print("You have found a Golden Boot?")
print(inventory)
else:
searchcounter = searchcounter + 1
print(searchcounter)
print("You did not find anything of value")
gameprompt()
elif (prompt == "rest"):
if (restcounter == 1):
print("Wait until you reach the next zone to rest again!")
gameprompt()
else:
# Add a MaxHP value to the player later, and the command rest will give 25% of that HP back.
Player.hp = Player.hp + (Player.hp / 5)
print("You have restored ",(Player.hp / 5)," hit points!")
restcounter = restcounter + 1
gameprompt()
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
gameprompt()
python python-3.x
The title is terrible, but hopefully I can explain in my post. Creating a little game as my pet project for python, and I'm currently creating the inventory. Everything was... ok when developing the game until it came to making the function that will show all of the player's inventory.
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
Ok, so I created a list that basically has a bunch of classes from Items in it. To call on the name element of these classes I have to do something like this, otherwise I just get its memory location which is largely useless to the player. I've tried
elif (prompt == "examine"):
print(inventory.name)
gameprompt()
Thought that this above example would print only the name of all the Item objects, but there's a compilation error instead because I didn't specify which one. So I then tried~
elif (prompt == "examine"):
print(inventory[1:1000].name)
gameprompt()
Thinking that it would print all of the Item objects names up to 1000, but I obviously don't have that so I thought it would print the names up to the latest object that was there and stop but there was another compilation error from this...
If there is anyway to print out an element of a class for all class objects in a list please let me know. The full code of this game is here, although I don't think you'll need it to help me solve my problem (it is also very large.)
playername = input("What is your name?")
zone = 1
movement = 0
restcounter = 0
searchcounter = 0
class Player:
def __init__(self, name, hp, mp, atk, xp, dodgerate, atkrate):
self.name = playername
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
self.dodgerate = dodgerate
self.atkrate = atkrate
class Enemy(Player):
def __init__(self, name, gold, maxhp, hp, mp, atk, xp):
self.name = name
self.gold = gold
self.maxhp = maxhp
self.hp = hp
self.mp = mp
self.atk = atk
self.xp = xp
class Items:
def __init__(self, name, quantity, description, price, weight):
self.name = name
self.quantity = quantity
self.description = description
self.price = price
self.weight = weight
Player = Player(playername, 1, 1, 1, 1, 25, 3)
print(Player.name + " has been created. ")
def raceselection():
raceinput = input("Do you float towards the TEMPLE, CAVE or FOREST?")
if raceinput == "TEMPLE":
print("You are now a high elf. High elves utlize a lot of magical power at the cost of being very frail.")
Player.hp = Player.hp + 24
Player.mp = Player.mp + 100
Player.atk = Player.atk + 50
print("You awaken from your slumber. Your room's walls are gold plated, and you rested on a flat board.")
print("Out the door, you see many elves with robes praying to some goddess.")
print("You walk out of your door and into the praying area. You are immediately greeted by a tall man.")
elif raceinput == "CAVE":
print("You are now an orc.")
Player.hp = Player.hp + 1000
Player.mp = Player.mp + 15
Player.atk = Player.atk + 50
print("cave")
elif raceinput == "FOREST":
print("You are now a human.")
Player.hp = Player.hp + 50
Player.mp = Player.mp + 25
Player.atk = Player.atk + 25
else:
print("You can't float there!")
raceselection()
raceselection()
inventory =
def gameprompt():
global inventory
global zone
global movement
global restcounter
global searchcounter
if (movement == 5):
movement = movement - movement
zone = zone + 1
print("You have advanced to zone",zone,"!!!")
gameprompt()
if (zone == 1):
print("Welcome to the first zone! Easy enemies are here with not very good loot./fix grammar, add description of zone/")
elif (zone == 2):
print("Hey, it actually travelled to the second zone, awesome!")
elif (zone == 3):
print("No way would this actually work!")
prompt = input("Would you like to walk, search or rest?: ")
if (prompt == "walk"):
encounterchance = random.randint(1, 3)
if (encounterchance == 2):
if (zone == 1):
mobspawnrate = random.randint(1,3)
if (mobspawnrate == 1):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 2):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
elif (mobspawnrate == 3):
Enemy = Enemy("Blue SlimeBall", 50, 0, 25, 15, 25, 0.500)
print("You have encountered a " + Enemy.name + "!!!")
else:
movement = movement + 1
print("You have walked a step. You are now at ",movement," steps")
gameprompt()
elif (prompt == "search"):
if (searchcounter == 3):
print("You cannot search this area anymore! Wait until you reach the next zone!")
gameprompt()
else:
searchchance = random.randint(1, 5)
if (searchchance == 1 or 2 or 3 or 4):
searchcounter = searchcounter + 1
print(searchcounter)
print("You have found something!")
searchchance = random.randint(1,4)
if (searchchance == 1 or 2):
inventory.append(Items("Old Boot", 1, "An old smelly boot. It's a mystery as to who it belongs to...", 5, 50))
print("You have found a Boot!")
print(inventory)
elif(searchchance == 3):
inventory.append(Items("Shiny Boot", 1, "Looks like a boot that was lightly worn. You could still wear this.", 5, 50))
print(inventory)
print("You have found a Shiny Boot!")
elif(searchchance == 4):
inventory.append(Items("Golden Boot", 1, "It's too heavy to wear, but it looks like it could sell for a fortune!", 5, 50))
print("You have found a Golden Boot?")
print(inventory)
else:
searchcounter = searchcounter + 1
print(searchcounter)
print("You did not find anything of value")
gameprompt()
elif (prompt == "rest"):
if (restcounter == 1):
print("Wait until you reach the next zone to rest again!")
gameprompt()
else:
# Add a MaxHP value to the player later, and the command rest will give 25% of that HP back.
Player.hp = Player.hp + (Player.hp / 5)
print("You have restored ",(Player.hp / 5)," hit points!")
restcounter = restcounter + 1
gameprompt()
elif (prompt == "examine"):
print(inventory[1].name)
gameprompt()
gameprompt()
python python-3.x
python python-3.x
edited Nov 17 '18 at 1:34
Anthony Sottile
21.1k64776
21.1k64776
asked Nov 17 '18 at 1:08


Samuel QuansahSamuel Quansah
325
325
Something likeprint([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18
add a comment |
Something likeprint([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18
Something like
print([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18
Something like
print([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18
add a comment |
1 Answer
1
active
oldest
votes
A list comprehension or map
would work perfectly here:
print([item.name for item in inventory])
The comprehension iterates the list, and "replaces" each element in the list with whatever the part before for
evaluates to. In this case, it's item.name
.
° It actually doesn't replace the element in the original list. It evaluates to a new list full of replaced items.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53347279%2fis-there-a-way-to-print-all-of-a-certain-element-of-a-class-that-is-in-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
A list comprehension or map
would work perfectly here:
print([item.name for item in inventory])
The comprehension iterates the list, and "replaces" each element in the list with whatever the part before for
evaluates to. In this case, it's item.name
.
° It actually doesn't replace the element in the original list. It evaluates to a new list full of replaced items.
add a comment |
A list comprehension or map
would work perfectly here:
print([item.name for item in inventory])
The comprehension iterates the list, and "replaces" each element in the list with whatever the part before for
evaluates to. In this case, it's item.name
.
° It actually doesn't replace the element in the original list. It evaluates to a new list full of replaced items.
add a comment |
A list comprehension or map
would work perfectly here:
print([item.name for item in inventory])
The comprehension iterates the list, and "replaces" each element in the list with whatever the part before for
evaluates to. In this case, it's item.name
.
° It actually doesn't replace the element in the original list. It evaluates to a new list full of replaced items.
A list comprehension or map
would work perfectly here:
print([item.name for item in inventory])
The comprehension iterates the list, and "replaces" each element in the list with whatever the part before for
evaluates to. In this case, it's item.name
.
° It actually doesn't replace the element in the original list. It evaluates to a new list full of replaced items.
edited Nov 30 '18 at 4:48
answered Nov 17 '18 at 1:18


CarcigenicateCarcigenicate
18.6k53262
18.6k53262
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53347279%2fis-there-a-way-to-print-all-of-a-certain-element-of-a-class-that-is-in-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oBQMsD WkpR1B
Something like
print([i.name for i in inventory])
– Michael Butscher
Nov 17 '18 at 1:18