How to handle Pop-up in Selenium WebDriver using Java
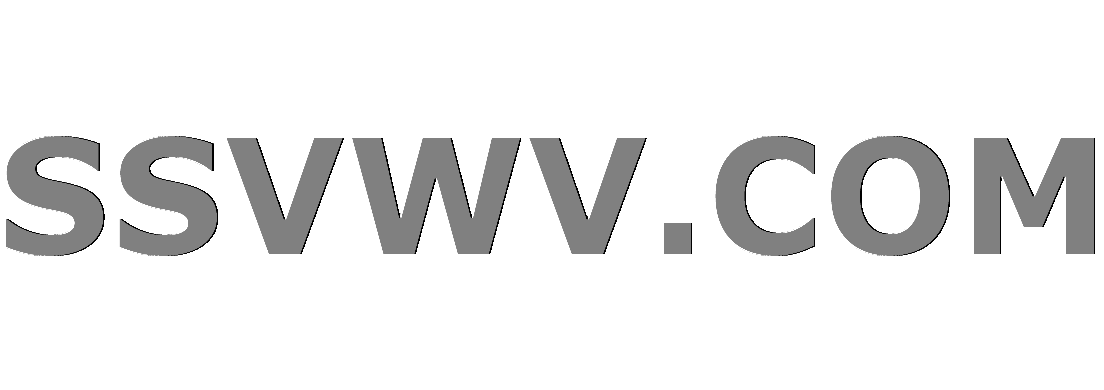
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I want to handle sign-in part in rediff.com, but the below code doesn't work for that:
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
String myWindowHandle = driver.getWindowHandle();
driver.switchTo().window(myWindowHandle);
WebElement email_id= driver.findElement(By.xpath("//*[@id='signin_info']/a[1]"));
email_id.sendKeys("hi");
If myWindowHandle
is not the correct string, then let me know how to get the pop-up Window name, because I can't find the name of the pop-up window.
java selenium-webdriver popup popupwindow
add a comment |
I want to handle sign-in part in rediff.com, but the below code doesn't work for that:
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
String myWindowHandle = driver.getWindowHandle();
driver.switchTo().window(myWindowHandle);
WebElement email_id= driver.findElement(By.xpath("//*[@id='signin_info']/a[1]"));
email_id.sendKeys("hi");
If myWindowHandle
is not the correct string, then let me know how to get the pop-up Window name, because I can't find the name of the pop-up window.
java selenium-webdriver popup popupwindow
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22
add a comment |
I want to handle sign-in part in rediff.com, but the below code doesn't work for that:
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
String myWindowHandle = driver.getWindowHandle();
driver.switchTo().window(myWindowHandle);
WebElement email_id= driver.findElement(By.xpath("//*[@id='signin_info']/a[1]"));
email_id.sendKeys("hi");
If myWindowHandle
is not the correct string, then let me know how to get the pop-up Window name, because I can't find the name of the pop-up window.
java selenium-webdriver popup popupwindow
I want to handle sign-in part in rediff.com, but the below code doesn't work for that:
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
String myWindowHandle = driver.getWindowHandle();
driver.switchTo().window(myWindowHandle);
WebElement email_id= driver.findElement(By.xpath("//*[@id='signin_info']/a[1]"));
email_id.sendKeys("hi");
If myWindowHandle
is not the correct string, then let me know how to get the pop-up Window name, because I can't find the name of the pop-up window.
java selenium-webdriver popup popupwindow
java selenium-webdriver popup popupwindow
edited Aug 22 '18 at 23:50
pnuts
49.3k764101
49.3k764101
asked Oct 16 '13 at 12:52


NiyatiNiyati
2683419
2683419
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22
add a comment |
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22
add a comment |
9 Answers
9
active
oldest
votes
To switch to a popup window, you need to use getWindowHandles()
and iterate through them.
In your code you are using getWindowHandle()
which will give you the parent window itself.
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
while (iterator.hasNext()){
subWindowHandler = iterator.next();
}
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
add a comment |
I found the solution for the above program, which had the goal of signing in to http://rediff.com
public class Handle_popupNAlert
{
public static void main(String args ) throws InterruptedException
{
WebDriver driver= new FirefoxDriver();
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
Set<String> windowId = driver.getWindowHandles(); // get window id of current window
Iterator<String> itererator = windowId.iterator();
String mainWinID = itererator.next();
String newAdwinID = itererator.next();
driver.switchTo().window(newAdwinID);
System.out.println(driver.getTitle());
Thread.sleep(3000);
driver.close();
driver.switchTo().window(mainWinID);
System.out.println(driver.getTitle());
Thread.sleep(2000);
WebElement email_id= driver.findElement(By.xpath("//*[@id='c_uname']"));
email_id.sendKeys("hi");
Thread.sleep(5000);
driver.close();
driver.quit();
}
}
add a comment |
You can handle popup window or alert box:
Alert alert = driver.switchTo().alert();
alert.accept();
You can also decline the alert box:
Alert alert = driver.switchTo().alert();
alert().dismiss();
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
add a comment |
You can use the below code inside your code when you get any web browser pop-up alert message box.
// Accepts (Click on OK) Chrome Alert Browser for RESET button.
Alert alertOK = driver.switchTo().alert();
alertOK.accept();
//Rejects (Click on Cancel) Chrome Browser Alert for RESET button.
Alert alertCancel = driver.switchTo().alert();
alertCancel.dismiss();
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
add a comment |
Do not make the situation complex. Use ID
if they are available.
driver.get("http://www.rediff.com");
WebElement sign = driver.findElement(By.linkText("Sign in"));
sign.click();
WebElement email_id= driver.findElement(By.id("c_uname"));
email_id.sendKeys("hi");
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking onsign-in
link? Any permission error?
– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
|
show 2 more comments
When the toastr message poped up on the screen of firefox. the below tag was displayed in fire bug.
<div class="toast-message">Invalid Credentials, Please check Password</div>.
I took the screenshot at that time.
And did the below changes in selenium java code.
String alertText = "";
WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("toast-message")));
WebElement toast1 = driver.findElement(By.className("toast-message"));
alertText = toast1.getText();
System.out.println( alertText);
And my issue of toastr popup got resolved.
add a comment |
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
subWindowHandler = iterator.next();
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
add a comment |
//get the main handle and remove it
//whatever remains is the child pop up window handle
String mainHandle = driver.getWindowHandle();
Set<String> allHandles = driver.getWindowHandles();
Iterator<String> iter = allHandles.iterator();
allHandles.remove(mainHandle);
String childHandle=iter.next();
add a comment |
public void Test(){
WebElement sign = fc.findElement(By.xpath(".//*[@id='login-scroll']/a"));
sign.click();
WebElement LoginAsGuest=fc.findElement(By.xpath(".//*[@id='guest-login-option']"));
LoginAsGuest.click();
WebElement email_id= fc.findElement(By.xpath(".//*[@id='guestemail']"));
email_id.sendKeys("ankushdeoladore@gmail.com");
WebElement ContinueButton=fc.findElement(By.xpath(".//*[@id='contibutton']"));
ContinueButton.click();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f19403949%2fhow-to-handle-pop-up-in-selenium-webdriver-using-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
9 Answers
9
active
oldest
votes
9 Answers
9
active
oldest
votes
active
oldest
votes
active
oldest
votes
To switch to a popup window, you need to use getWindowHandles()
and iterate through them.
In your code you are using getWindowHandle()
which will give you the parent window itself.
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
while (iterator.hasNext()){
subWindowHandler = iterator.next();
}
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
add a comment |
To switch to a popup window, you need to use getWindowHandles()
and iterate through them.
In your code you are using getWindowHandle()
which will give you the parent window itself.
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
while (iterator.hasNext()){
subWindowHandler = iterator.next();
}
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
add a comment |
To switch to a popup window, you need to use getWindowHandles()
and iterate through them.
In your code you are using getWindowHandle()
which will give you the parent window itself.
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
while (iterator.hasNext()){
subWindowHandler = iterator.next();
}
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
To switch to a popup window, you need to use getWindowHandles()
and iterate through them.
In your code you are using getWindowHandle()
which will give you the parent window itself.
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
while (iterator.hasNext()){
subWindowHandler = iterator.next();
}
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
edited Jun 3 '17 at 17:45
answered Oct 16 '13 at 16:46
LINGSLINGS
2,43442141
2,43442141
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
add a comment |
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
But i just need to handle only one window than i think so no meaning to use iterator ...do u have another way . i just need to write email address and password in sign in form of rediff.com .pls helm me in this .
– Niyati
Oct 17 '13 at 5:09
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
The above code will handle that. Once you switch to sub window which is your popup. Pick the webelements and fill in your email id, password and submit. Then switch back to your parent window.
– LINGS
Oct 17 '13 at 5:14
1
1
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
Tried but code is not working on link : rediff.com -> click on sign in link and try to signed in
– Niyati
Oct 17 '13 at 5:58
works for me thx
– mosaad
Dec 5 '16 at 15:43
works for me thx
– mosaad
Dec 5 '16 at 15:43
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
@Johnp2: please don't hijack the answer
– Hovercraft Full Of Eels
Jun 18 '17 at 2:11
add a comment |
I found the solution for the above program, which had the goal of signing in to http://rediff.com
public class Handle_popupNAlert
{
public static void main(String args ) throws InterruptedException
{
WebDriver driver= new FirefoxDriver();
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
Set<String> windowId = driver.getWindowHandles(); // get window id of current window
Iterator<String> itererator = windowId.iterator();
String mainWinID = itererator.next();
String newAdwinID = itererator.next();
driver.switchTo().window(newAdwinID);
System.out.println(driver.getTitle());
Thread.sleep(3000);
driver.close();
driver.switchTo().window(mainWinID);
System.out.println(driver.getTitle());
Thread.sleep(2000);
WebElement email_id= driver.findElement(By.xpath("//*[@id='c_uname']"));
email_id.sendKeys("hi");
Thread.sleep(5000);
driver.close();
driver.quit();
}
}
add a comment |
I found the solution for the above program, which had the goal of signing in to http://rediff.com
public class Handle_popupNAlert
{
public static void main(String args ) throws InterruptedException
{
WebDriver driver= new FirefoxDriver();
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
Set<String> windowId = driver.getWindowHandles(); // get window id of current window
Iterator<String> itererator = windowId.iterator();
String mainWinID = itererator.next();
String newAdwinID = itererator.next();
driver.switchTo().window(newAdwinID);
System.out.println(driver.getTitle());
Thread.sleep(3000);
driver.close();
driver.switchTo().window(mainWinID);
System.out.println(driver.getTitle());
Thread.sleep(2000);
WebElement email_id= driver.findElement(By.xpath("//*[@id='c_uname']"));
email_id.sendKeys("hi");
Thread.sleep(5000);
driver.close();
driver.quit();
}
}
add a comment |
I found the solution for the above program, which had the goal of signing in to http://rediff.com
public class Handle_popupNAlert
{
public static void main(String args ) throws InterruptedException
{
WebDriver driver= new FirefoxDriver();
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
Set<String> windowId = driver.getWindowHandles(); // get window id of current window
Iterator<String> itererator = windowId.iterator();
String mainWinID = itererator.next();
String newAdwinID = itererator.next();
driver.switchTo().window(newAdwinID);
System.out.println(driver.getTitle());
Thread.sleep(3000);
driver.close();
driver.switchTo().window(mainWinID);
System.out.println(driver.getTitle());
Thread.sleep(2000);
WebElement email_id= driver.findElement(By.xpath("//*[@id='c_uname']"));
email_id.sendKeys("hi");
Thread.sleep(5000);
driver.close();
driver.quit();
}
}
I found the solution for the above program, which had the goal of signing in to http://rediff.com
public class Handle_popupNAlert
{
public static void main(String args ) throws InterruptedException
{
WebDriver driver= new FirefoxDriver();
driver.get("http://www.rediff.com/");
WebElement sign = driver.findElement(By.xpath("//html/body/div[3]/div[3]/span[4]/span/a"));
sign.click();
Set<String> windowId = driver.getWindowHandles(); // get window id of current window
Iterator<String> itererator = windowId.iterator();
String mainWinID = itererator.next();
String newAdwinID = itererator.next();
driver.switchTo().window(newAdwinID);
System.out.println(driver.getTitle());
Thread.sleep(3000);
driver.close();
driver.switchTo().window(mainWinID);
System.out.println(driver.getTitle());
Thread.sleep(2000);
WebElement email_id= driver.findElement(By.xpath("//*[@id='c_uname']"));
email_id.sendKeys("hi");
Thread.sleep(5000);
driver.close();
driver.quit();
}
}
edited Oct 17 '13 at 7:39
Seanny123
2,70943871
2,70943871
answered Oct 17 '13 at 7:08


NiyatiNiyati
2683419
2683419
add a comment |
add a comment |
You can handle popup window or alert box:
Alert alert = driver.switchTo().alert();
alert.accept();
You can also decline the alert box:
Alert alert = driver.switchTo().alert();
alert().dismiss();
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
add a comment |
You can handle popup window or alert box:
Alert alert = driver.switchTo().alert();
alert.accept();
You can also decline the alert box:
Alert alert = driver.switchTo().alert();
alert().dismiss();
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
add a comment |
You can handle popup window or alert box:
Alert alert = driver.switchTo().alert();
alert.accept();
You can also decline the alert box:
Alert alert = driver.switchTo().alert();
alert().dismiss();
You can handle popup window or alert box:
Alert alert = driver.switchTo().alert();
alert.accept();
You can also decline the alert box:
Alert alert = driver.switchTo().alert();
alert().dismiss();
edited Jan 31 '15 at 7:36


smottt
2,73373140
2,73373140
answered Jan 31 '15 at 7:24


ER.swatantraER.swatantra
1155
1155
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
add a comment |
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
This worked for me
– sinisterrook
Mar 11 '15 at 19:48
add a comment |
You can use the below code inside your code when you get any web browser pop-up alert message box.
// Accepts (Click on OK) Chrome Alert Browser for RESET button.
Alert alertOK = driver.switchTo().alert();
alertOK.accept();
//Rejects (Click on Cancel) Chrome Browser Alert for RESET button.
Alert alertCancel = driver.switchTo().alert();
alertCancel.dismiss();
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
add a comment |
You can use the below code inside your code when you get any web browser pop-up alert message box.
// Accepts (Click on OK) Chrome Alert Browser for RESET button.
Alert alertOK = driver.switchTo().alert();
alertOK.accept();
//Rejects (Click on Cancel) Chrome Browser Alert for RESET button.
Alert alertCancel = driver.switchTo().alert();
alertCancel.dismiss();
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
add a comment |
You can use the below code inside your code when you get any web browser pop-up alert message box.
// Accepts (Click on OK) Chrome Alert Browser for RESET button.
Alert alertOK = driver.switchTo().alert();
alertOK.accept();
//Rejects (Click on Cancel) Chrome Browser Alert for RESET button.
Alert alertCancel = driver.switchTo().alert();
alertCancel.dismiss();
You can use the below code inside your code when you get any web browser pop-up alert message box.
// Accepts (Click on OK) Chrome Alert Browser for RESET button.
Alert alertOK = driver.switchTo().alert();
alertOK.accept();
//Rejects (Click on Cancel) Chrome Browser Alert for RESET button.
Alert alertCancel = driver.switchTo().alert();
alertCancel.dismiss();
edited May 16 '16 at 6:30


Sandeep
1,23251631
1,23251631
answered Mar 25 '16 at 9:47
Manasis MishraManasis Mishra
413
413
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
add a comment |
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
You can use the above code inside your code when you get any web browser pop up alert message box.
– Manasis Mishra
Mar 25 '16 at 9:53
add a comment |
Do not make the situation complex. Use ID
if they are available.
driver.get("http://www.rediff.com");
WebElement sign = driver.findElement(By.linkText("Sign in"));
sign.click();
WebElement email_id= driver.findElement(By.id("c_uname"));
email_id.sendKeys("hi");
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking onsign-in
link? Any permission error?
– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
|
show 2 more comments
Do not make the situation complex. Use ID
if they are available.
driver.get("http://www.rediff.com");
WebElement sign = driver.findElement(By.linkText("Sign in"));
sign.click();
WebElement email_id= driver.findElement(By.id("c_uname"));
email_id.sendKeys("hi");
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking onsign-in
link? Any permission error?
– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
|
show 2 more comments
Do not make the situation complex. Use ID
if they are available.
driver.get("http://www.rediff.com");
WebElement sign = driver.findElement(By.linkText("Sign in"));
sign.click();
WebElement email_id= driver.findElement(By.id("c_uname"));
email_id.sendKeys("hi");
Do not make the situation complex. Use ID
if they are available.
driver.get("http://www.rediff.com");
WebElement sign = driver.findElement(By.linkText("Sign in"));
sign.click();
WebElement email_id= driver.findElement(By.id("c_uname"));
email_id.sendKeys("hi");
edited Oct 1 '14 at 15:22


alecxe
333k72665886
333k72665886
answered Oct 16 '13 at 13:11
HemanthHemanth
2,04711429
2,04711429
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking onsign-in
link? Any permission error?
– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
|
show 2 more comments
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking onsign-in
link? Any permission error?
– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
I can''t directly use email_id field as its in pop up window ..pls visit rediff.com as i want to write email address and password in sign in form of rediff.com. link: rediff.com
– Niyati
Oct 17 '13 at 5:11
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
which browser are you using? Because i am not seeing any pop up window after clicking on sign-in. it is just another modal window (inside main window). i am using firefox23.
– Hemanth
Oct 17 '13 at 5:34
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
yeh i need to access that and signed in
– Niyati
Oct 17 '13 at 5:38
what is error you are getting while clicking on
sign-in
link? Any permission error?– Hemanth
Oct 17 '13 at 5:48
what is error you are getting while clicking on
sign-in
link? Any permission error?– Hemanth
Oct 17 '13 at 5:48
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
didn't get any error but not able to handle email address field
– Niyati
Oct 17 '13 at 5:59
|
show 2 more comments
When the toastr message poped up on the screen of firefox. the below tag was displayed in fire bug.
<div class="toast-message">Invalid Credentials, Please check Password</div>.
I took the screenshot at that time.
And did the below changes in selenium java code.
String alertText = "";
WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("toast-message")));
WebElement toast1 = driver.findElement(By.className("toast-message"));
alertText = toast1.getText();
System.out.println( alertText);
And my issue of toastr popup got resolved.
add a comment |
When the toastr message poped up on the screen of firefox. the below tag was displayed in fire bug.
<div class="toast-message">Invalid Credentials, Please check Password</div>.
I took the screenshot at that time.
And did the below changes in selenium java code.
String alertText = "";
WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("toast-message")));
WebElement toast1 = driver.findElement(By.className("toast-message"));
alertText = toast1.getText();
System.out.println( alertText);
And my issue of toastr popup got resolved.
add a comment |
When the toastr message poped up on the screen of firefox. the below tag was displayed in fire bug.
<div class="toast-message">Invalid Credentials, Please check Password</div>.
I took the screenshot at that time.
And did the below changes in selenium java code.
String alertText = "";
WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("toast-message")));
WebElement toast1 = driver.findElement(By.className("toast-message"));
alertText = toast1.getText();
System.out.println( alertText);
And my issue of toastr popup got resolved.
When the toastr message poped up on the screen of firefox. the below tag was displayed in fire bug.
<div class="toast-message">Invalid Credentials, Please check Password</div>.
I took the screenshot at that time.
And did the below changes in selenium java code.
String alertText = "";
WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("toast-message")));
WebElement toast1 = driver.findElement(By.className("toast-message"));
alertText = toast1.getText();
System.out.println( alertText);
And my issue of toastr popup got resolved.
answered Jul 28 '15 at 8:58
SandipSandip
1
1
add a comment |
add a comment |
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
subWindowHandler = iterator.next();
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
add a comment |
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
subWindowHandler = iterator.next();
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
add a comment |
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
subWindowHandler = iterator.next();
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
String parentWindowHandler = driver.getWindowHandle(); // Store your parent window
String subWindowHandler = null;
Set<String> handles = driver.getWindowHandles(); // get all window handles
Iterator<String> iterator = handles.iterator();
subWindowHandler = iterator.next();
driver.switchTo().window(subWindowHandler); // switch to popup window
// Now you are in the popup window, perform necessary actions here
driver.switchTo().window(parentWindowHandler); // switch back to parent window
edited Nov 17 '18 at 3:02
Machavity
24.8k135981
24.8k135981
answered Nov 17 '18 at 3:00
user3150673user3150673
1
1
add a comment |
add a comment |
//get the main handle and remove it
//whatever remains is the child pop up window handle
String mainHandle = driver.getWindowHandle();
Set<String> allHandles = driver.getWindowHandles();
Iterator<String> iter = allHandles.iterator();
allHandles.remove(mainHandle);
String childHandle=iter.next();
add a comment |
//get the main handle and remove it
//whatever remains is the child pop up window handle
String mainHandle = driver.getWindowHandle();
Set<String> allHandles = driver.getWindowHandles();
Iterator<String> iter = allHandles.iterator();
allHandles.remove(mainHandle);
String childHandle=iter.next();
add a comment |
//get the main handle and remove it
//whatever remains is the child pop up window handle
String mainHandle = driver.getWindowHandle();
Set<String> allHandles = driver.getWindowHandles();
Iterator<String> iter = allHandles.iterator();
allHandles.remove(mainHandle);
String childHandle=iter.next();
//get the main handle and remove it
//whatever remains is the child pop up window handle
String mainHandle = driver.getWindowHandle();
Set<String> allHandles = driver.getWindowHandles();
Iterator<String> iter = allHandles.iterator();
allHandles.remove(mainHandle);
String childHandle=iter.next();
answered Nov 20 '14 at 11:46


Satheesh kumarSatheesh kumar
392
392
add a comment |
add a comment |
public void Test(){
WebElement sign = fc.findElement(By.xpath(".//*[@id='login-scroll']/a"));
sign.click();
WebElement LoginAsGuest=fc.findElement(By.xpath(".//*[@id='guest-login-option']"));
LoginAsGuest.click();
WebElement email_id= fc.findElement(By.xpath(".//*[@id='guestemail']"));
email_id.sendKeys("ankushdeoladore@gmail.com");
WebElement ContinueButton=fc.findElement(By.xpath(".//*[@id='contibutton']"));
ContinueButton.click();
}
add a comment |
public void Test(){
WebElement sign = fc.findElement(By.xpath(".//*[@id='login-scroll']/a"));
sign.click();
WebElement LoginAsGuest=fc.findElement(By.xpath(".//*[@id='guest-login-option']"));
LoginAsGuest.click();
WebElement email_id= fc.findElement(By.xpath(".//*[@id='guestemail']"));
email_id.sendKeys("ankushdeoladore@gmail.com");
WebElement ContinueButton=fc.findElement(By.xpath(".//*[@id='contibutton']"));
ContinueButton.click();
}
add a comment |
public void Test(){
WebElement sign = fc.findElement(By.xpath(".//*[@id='login-scroll']/a"));
sign.click();
WebElement LoginAsGuest=fc.findElement(By.xpath(".//*[@id='guest-login-option']"));
LoginAsGuest.click();
WebElement email_id= fc.findElement(By.xpath(".//*[@id='guestemail']"));
email_id.sendKeys("ankushdeoladore@gmail.com");
WebElement ContinueButton=fc.findElement(By.xpath(".//*[@id='contibutton']"));
ContinueButton.click();
}
public void Test(){
WebElement sign = fc.findElement(By.xpath(".//*[@id='login-scroll']/a"));
sign.click();
WebElement LoginAsGuest=fc.findElement(By.xpath(".//*[@id='guest-login-option']"));
LoginAsGuest.click();
WebElement email_id= fc.findElement(By.xpath(".//*[@id='guestemail']"));
email_id.sendKeys("ankushdeoladore@gmail.com");
WebElement ContinueButton=fc.findElement(By.xpath(".//*[@id='contibutton']"));
ContinueButton.click();
}
edited Dec 16 '15 at 7:46


SHAZ
2,35261827
2,35261827
answered Dec 16 '15 at 7:41


ankush deolankush deol
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f19403949%2fhow-to-handle-pop-up-in-selenium-webdriver-using-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hNTh,YO8YJzNroR4ypIJWeRr3fOhcjNIvP5u86,SeI3kLDWIASlZnnS6xIJA1oVoSW0K0qXfMd gUrLa34viM
Take the list of windows by using driver.getWindowHandles().
– Ripon Al Wasim
Aug 29 '17 at 11:22