values not getting stored one by one in an array after each button click
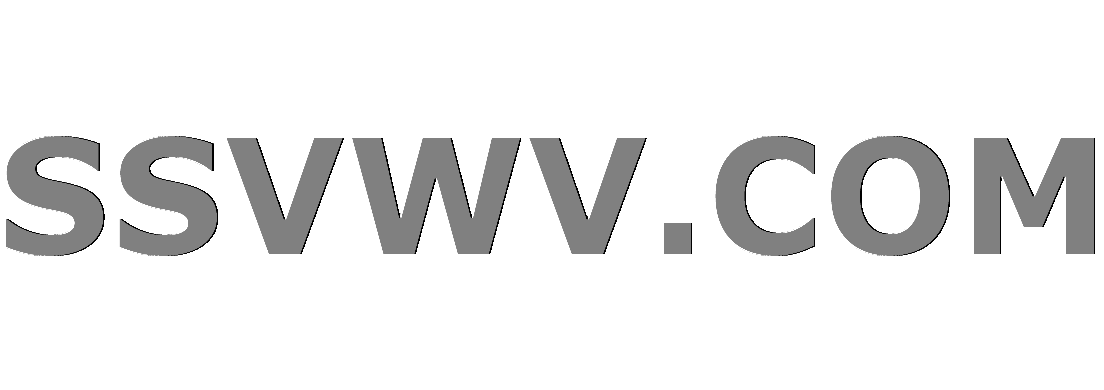
Multi tool use
I was trying to build a program and got a problem of storing data into an array after each click of a button. After each click, I am sending some data through ajax to a php page and i want to store all sent data in an array and display them in an tabular format later.
I have checked in the console by inspect element and the problem arising is its always overwrites the old value in an array to a new value. I dont find where i am wrong. if someone please help me will be of great help.
$(document).ready(function() {
$("#feed_btn").hide();
$("#finish_btn").hide();
$("#start_btn").click(function() {
$("#start_btn").hide();
$("#feed_btn").show();
});
});
var i = 0;
function PerformGame() {
i++;
$.ajax({
url: 'perform_game.php',
type: "POST",
data: {
'button_clicked': i
},
dataType: 'json',
success: function(data) {
alert(data);
}
});
}
.game-body {
background-color: AED264;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<form name="farm_game" method="POST">
<button type="button" name="start_btn" id="start_btn">Start Game !</button>
<button type="button" name="feed_btn" id="feed_btn" onclick="PerformGame();">Let's Feed Now</button>
<button type="button" name="finish_btn" id="finish_btn">Finish Game !</button>
</form>
<?php
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array(Farmer,Cow1,Cow2,Bunny1,Bunny2,Bunny3,Bunny4);
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
?>
javascript php jquery ajax
|
show 9 more comments
I was trying to build a program and got a problem of storing data into an array after each click of a button. After each click, I am sending some data through ajax to a php page and i want to store all sent data in an array and display them in an tabular format later.
I have checked in the console by inspect element and the problem arising is its always overwrites the old value in an array to a new value. I dont find where i am wrong. if someone please help me will be of great help.
$(document).ready(function() {
$("#feed_btn").hide();
$("#finish_btn").hide();
$("#start_btn").click(function() {
$("#start_btn").hide();
$("#feed_btn").show();
});
});
var i = 0;
function PerformGame() {
i++;
$.ajax({
url: 'perform_game.php',
type: "POST",
data: {
'button_clicked': i
},
dataType: 'json',
success: function(data) {
alert(data);
}
});
}
.game-body {
background-color: AED264;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<form name="farm_game" method="POST">
<button type="button" name="start_btn" id="start_btn">Start Game !</button>
<button type="button" name="feed_btn" id="feed_btn" onclick="PerformGame();">Let's Feed Now</button>
<button type="button" name="finish_btn" id="finish_btn">Finish Game !</button>
</form>
<?php
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array(Farmer,Cow1,Cow2,Bunny1,Bunny2,Bunny3,Bunny4);
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
?>
javascript php jquery ajax
1
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
1
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
1
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
2
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55
|
show 9 more comments
I was trying to build a program and got a problem of storing data into an array after each click of a button. After each click, I am sending some data through ajax to a php page and i want to store all sent data in an array and display them in an tabular format later.
I have checked in the console by inspect element and the problem arising is its always overwrites the old value in an array to a new value. I dont find where i am wrong. if someone please help me will be of great help.
$(document).ready(function() {
$("#feed_btn").hide();
$("#finish_btn").hide();
$("#start_btn").click(function() {
$("#start_btn").hide();
$("#feed_btn").show();
});
});
var i = 0;
function PerformGame() {
i++;
$.ajax({
url: 'perform_game.php',
type: "POST",
data: {
'button_clicked': i
},
dataType: 'json',
success: function(data) {
alert(data);
}
});
}
.game-body {
background-color: AED264;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<form name="farm_game" method="POST">
<button type="button" name="start_btn" id="start_btn">Start Game !</button>
<button type="button" name="feed_btn" id="feed_btn" onclick="PerformGame();">Let's Feed Now</button>
<button type="button" name="finish_btn" id="finish_btn">Finish Game !</button>
</form>
<?php
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array(Farmer,Cow1,Cow2,Bunny1,Bunny2,Bunny3,Bunny4);
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
?>
javascript php jquery ajax
I was trying to build a program and got a problem of storing data into an array after each click of a button. After each click, I am sending some data through ajax to a php page and i want to store all sent data in an array and display them in an tabular format later.
I have checked in the console by inspect element and the problem arising is its always overwrites the old value in an array to a new value. I dont find where i am wrong. if someone please help me will be of great help.
$(document).ready(function() {
$("#feed_btn").hide();
$("#finish_btn").hide();
$("#start_btn").click(function() {
$("#start_btn").hide();
$("#feed_btn").show();
});
});
var i = 0;
function PerformGame() {
i++;
$.ajax({
url: 'perform_game.php',
type: "POST",
data: {
'button_clicked': i
},
dataType: 'json',
success: function(data) {
alert(data);
}
});
}
.game-body {
background-color: AED264;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<form name="farm_game" method="POST">
<button type="button" name="start_btn" id="start_btn">Start Game !</button>
<button type="button" name="feed_btn" id="feed_btn" onclick="PerformGame();">Let's Feed Now</button>
<button type="button" name="finish_btn" id="finish_btn">Finish Game !</button>
</form>
<?php
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array(Farmer,Cow1,Cow2,Bunny1,Bunny2,Bunny3,Bunny4);
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
?>
javascript php jquery ajax
javascript php jquery ajax
edited Nov 15 '18 at 16:00


Erik Kalkoken
13.2k32552
13.2k32552
asked Nov 15 '18 at 15:44
SnehasisSnehasis
550410
550410
1
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
1
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
1
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
2
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55
|
show 9 more comments
1
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
1
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
1
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
2
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55
1
1
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
1
1
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
1
1
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
2
2
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55
|
show 9 more comments
1 Answer
1
active
oldest
votes
This does not work, because each time you make the AJAX call you start a new instance of the PHP script, that does not know about the other calls.
You have a couple of options:
- Store the previous array entries in a server session
- Store the previous array entries in a DB
- Collect all data about buttons on client side (in Java Script) and send the whole array to your PHP script
For your case I would recommend a server session.
Here is an example code (untested) with rudimentary session handling:
<?php
session_start();
if ($_POST['start_game'] !== null)
{
$_SESSION['final_result_array'] = ;
die();
}
$final_result_array = isset($_SESSION['final_result_array'])
? $_SESSION['final_result_array']
: ;
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array("Farmer","Cow1","Cow2","Bunny1","Bunny2","Bunny3","Bunny4");
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
$_SESSION['final_result_array'] = $final_result_array;
?>
Important: You need to re-initialize your array at every start of a game or it will keep adding to it. For that just call the script with the parameter start_game = true
when clicking on the start_game
button.
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323029%2fvalues-not-getting-stored-one-by-one-in-an-array-after-each-button-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This does not work, because each time you make the AJAX call you start a new instance of the PHP script, that does not know about the other calls.
You have a couple of options:
- Store the previous array entries in a server session
- Store the previous array entries in a DB
- Collect all data about buttons on client side (in Java Script) and send the whole array to your PHP script
For your case I would recommend a server session.
Here is an example code (untested) with rudimentary session handling:
<?php
session_start();
if ($_POST['start_game'] !== null)
{
$_SESSION['final_result_array'] = ;
die();
}
$final_result_array = isset($_SESSION['final_result_array'])
? $_SESSION['final_result_array']
: ;
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array("Farmer","Cow1","Cow2","Bunny1","Bunny2","Bunny3","Bunny4");
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
$_SESSION['final_result_array'] = $final_result_array;
?>
Important: You need to re-initialize your array at every start of a game or it will keep adding to it. For that just call the script with the parameter start_game = true
when clicking on the start_game
button.
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
|
show 3 more comments
This does not work, because each time you make the AJAX call you start a new instance of the PHP script, that does not know about the other calls.
You have a couple of options:
- Store the previous array entries in a server session
- Store the previous array entries in a DB
- Collect all data about buttons on client side (in Java Script) and send the whole array to your PHP script
For your case I would recommend a server session.
Here is an example code (untested) with rudimentary session handling:
<?php
session_start();
if ($_POST['start_game'] !== null)
{
$_SESSION['final_result_array'] = ;
die();
}
$final_result_array = isset($_SESSION['final_result_array'])
? $_SESSION['final_result_array']
: ;
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array("Farmer","Cow1","Cow2","Bunny1","Bunny2","Bunny3","Bunny4");
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
$_SESSION['final_result_array'] = $final_result_array;
?>
Important: You need to re-initialize your array at every start of a game or it will keep adding to it. For that just call the script with the parameter start_game = true
when clicking on the start_game
button.
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
|
show 3 more comments
This does not work, because each time you make the AJAX call you start a new instance of the PHP script, that does not know about the other calls.
You have a couple of options:
- Store the previous array entries in a server session
- Store the previous array entries in a DB
- Collect all data about buttons on client side (in Java Script) and send the whole array to your PHP script
For your case I would recommend a server session.
Here is an example code (untested) with rudimentary session handling:
<?php
session_start();
if ($_POST['start_game'] !== null)
{
$_SESSION['final_result_array'] = ;
die();
}
$final_result_array = isset($_SESSION['final_result_array'])
? $_SESSION['final_result_array']
: ;
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array("Farmer","Cow1","Cow2","Bunny1","Bunny2","Bunny3","Bunny4");
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
$_SESSION['final_result_array'] = $final_result_array;
?>
Important: You need to re-initialize your array at every start of a game or it will keep adding to it. For that just call the script with the parameter start_game = true
when clicking on the start_game
button.
This does not work, because each time you make the AJAX call you start a new instance of the PHP script, that does not know about the other calls.
You have a couple of options:
- Store the previous array entries in a server session
- Store the previous array entries in a DB
- Collect all data about buttons on client side (in Java Script) and send the whole array to your PHP script
For your case I would recommend a server session.
Here is an example code (untested) with rudimentary session handling:
<?php
session_start();
if ($_POST['start_game'] !== null)
{
$_SESSION['final_result_array'] = ;
die();
}
$final_result_array = isset($_SESSION['final_result_array'])
? $_SESSION['final_result_array']
: ;
$no_of_times_button_clicked = $_POST['button_clicked'];
$animal_person_array = array("Farmer","Cow1","Cow2","Bunny1","Bunny2","Bunny3","Bunny4");
$a = $animal_person_array[array_rand($animal_person_array,1)];
$final_result_array = array($no_of_times_button_clicked => $a);
echo json_encode($final_result_array);
$_SESSION['final_result_array'] = $final_result_array;
?>
Important: You need to re-initialize your array at every start of a game or it will keep adding to it. For that just call the script with the parameter start_game = true
when clicking on the start_game
button.
edited Nov 15 '18 at 16:42
answered Nov 15 '18 at 15:50


Erik KalkokenErik Kalkoken
13.2k32552
13.2k32552
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
|
show 3 more comments
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
Thanks for your kind help. But am a newbee to this type of session management. Could you please elaborate more like how can i store those values in a session and then retrieve them at last ?
– Snehasis
Nov 15 '18 at 16:01
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
All you need to do is start a sever session and retrieve your array from the session at start and store your array into the session at the end. PHP will then make sure that information is kept persistent between calls to the script.
– Erik Kalkoken
Nov 15 '18 at 16:04
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
It's a bit much to expect someone to type out the code for you, he has told you what your options are, time to get googlin'
– Nunchy
Nov 15 '18 at 16:06
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
To be fair, even if you get the session going your code has other issues. Personally, I don't think php is necessarily the best way to do this, do you have to use php for this?
– Nunchy
Nov 15 '18 at 16:08
1
1
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
@ErikKalkoken Hey Buddy, Thanks to your kind help. Yours untested piece of code is working fine for me and its made my day. Thanks again !
– Snehasis
Nov 16 '18 at 3:13
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323029%2fvalues-not-getting-stored-one-by-one-in-an-array-after-each-button-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EAD2y5nKEu0Wu CfU5,my7VkCxzNrY
1
You need some sort of storage like db so when button is clicked you store the num of clicks on second click you increment the previous stored result
– Masivuye Cokile
Nov 15 '18 at 15:48
@MasivuyeCokile But I dont want to maintain a Db for the simple task. I think of something like array where i can store the values and later display it in an tabular format.
– Snehasis
Nov 15 '18 at 15:51
1
You'll neeed to at least initialise a session on the server-side.
– Nunchy
Nov 15 '18 at 15:53
1
If you want to avoid a DB you can either: remove php from the picture and just keep your variables in javascript; or post the entire array back to the server in addition to your current parameters.
– Steve0
Nov 15 '18 at 15:55
2
If you don't want to use server side storage you could use a cookie, sessionStorage or localStorage. This would also remove the need for the AJAX request too
– Rory McCrossan
Nov 15 '18 at 15:55