how to get the index of a reoccurring character in swift
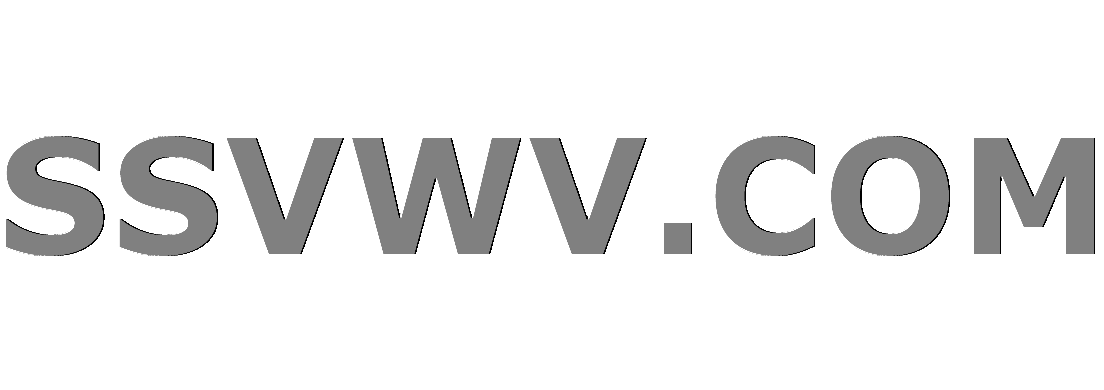
Multi tool use
var string = "HELLO WOLRD"
I want to get all of the indexes of the letter "L" in the string (which in this case would be 2,3 and 8). How do I do that in swift?
swift string
add a comment |
var string = "HELLO WOLRD"
I want to get all of the indexes of the letter "L" in the string (which in this case would be 2,3 and 8). How do I do that in swift?
swift string
3
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59
add a comment |
var string = "HELLO WOLRD"
I want to get all of the indexes of the letter "L" in the string (which in this case would be 2,3 and 8). How do I do that in swift?
swift string
var string = "HELLO WOLRD"
I want to get all of the indexes of the letter "L" in the string (which in this case would be 2,3 and 8). How do I do that in swift?
swift string
swift string
edited Nov 15 '18 at 16:07
Dávid Pásztor
22.4k82850
22.4k82850
asked Nov 15 '18 at 15:47
Orane PereiraOrane Pereira
31
31
3
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59
add a comment |
3
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59
3
3
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59
add a comment |
2 Answers
2
active
oldest
votes
You could use enumerated()
along with compactMap
like this:
var string = "HELLO WOLRD"
let indices = string.enumerated().compactMap { $0.element == "L" ? $0.offset : nil }
print(indices)
[2, 3, 8]
Explanation:
string.enumerated()
generates a sequence of tuple pairs where the first item is the offset of the character, and the second is the character.- The closure used by
compactMap
takes each tuple in turn and returns the offset of the character if it matchesL
or returnsnil
if it does not match.compactMap
leaves out thenil
values and just returns the offsets.
add a comment |
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[Int]](), { result, current in
if result[current.element] != nil {
result[current.element]!.append(current.offset)
} else {
result[current.element] = [current.offset]
}
})
print(charsWithIndices)
["W": [6], "D": [10], "R": [8], "E": [1], "H": [0], " ": [5], "O": [4, 7], "L": [2, 3, 9]]
Or if you want to use the indices straight away for indexing your String, you can change make charsWithIndices
of type [Character:[String.Index]]
:
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[String.Index]](), { result, current in
let index = helloWorld.index(helloWorld.startIndex, offsetBy: current.offset)
if result[current.element] != nil {
result[current.element]!.append(index)
} else {
result[current.element] = [index]
}
})
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323069%2fhow-to-get-the-index-of-a-reoccurring-character-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could use enumerated()
along with compactMap
like this:
var string = "HELLO WOLRD"
let indices = string.enumerated().compactMap { $0.element == "L" ? $0.offset : nil }
print(indices)
[2, 3, 8]
Explanation:
string.enumerated()
generates a sequence of tuple pairs where the first item is the offset of the character, and the second is the character.- The closure used by
compactMap
takes each tuple in turn and returns the offset of the character if it matchesL
or returnsnil
if it does not match.compactMap
leaves out thenil
values and just returns the offsets.
add a comment |
You could use enumerated()
along with compactMap
like this:
var string = "HELLO WOLRD"
let indices = string.enumerated().compactMap { $0.element == "L" ? $0.offset : nil }
print(indices)
[2, 3, 8]
Explanation:
string.enumerated()
generates a sequence of tuple pairs where the first item is the offset of the character, and the second is the character.- The closure used by
compactMap
takes each tuple in turn and returns the offset of the character if it matchesL
or returnsnil
if it does not match.compactMap
leaves out thenil
values and just returns the offsets.
add a comment |
You could use enumerated()
along with compactMap
like this:
var string = "HELLO WOLRD"
let indices = string.enumerated().compactMap { $0.element == "L" ? $0.offset : nil }
print(indices)
[2, 3, 8]
Explanation:
string.enumerated()
generates a sequence of tuple pairs where the first item is the offset of the character, and the second is the character.- The closure used by
compactMap
takes each tuple in turn and returns the offset of the character if it matchesL
or returnsnil
if it does not match.compactMap
leaves out thenil
values and just returns the offsets.
You could use enumerated()
along with compactMap
like this:
var string = "HELLO WOLRD"
let indices = string.enumerated().compactMap { $0.element == "L" ? $0.offset : nil }
print(indices)
[2, 3, 8]
Explanation:
string.enumerated()
generates a sequence of tuple pairs where the first item is the offset of the character, and the second is the character.- The closure used by
compactMap
takes each tuple in turn and returns the offset of the character if it matchesL
or returnsnil
if it does not match.compactMap
leaves out thenil
values and just returns the offsets.
edited Nov 15 '18 at 16:44
answered Nov 15 '18 at 16:08


vacawamavacawama
98.7k15177203
98.7k15177203
add a comment |
add a comment |
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[Int]](), { result, current in
if result[current.element] != nil {
result[current.element]!.append(current.offset)
} else {
result[current.element] = [current.offset]
}
})
print(charsWithIndices)
["W": [6], "D": [10], "R": [8], "E": [1], "H": [0], " ": [5], "O": [4, 7], "L": [2, 3, 9]]
Or if you want to use the indices straight away for indexing your String, you can change make charsWithIndices
of type [Character:[String.Index]]
:
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[String.Index]](), { result, current in
let index = helloWorld.index(helloWorld.startIndex, offsetBy: current.offset)
if result[current.element] != nil {
result[current.element]!.append(index)
} else {
result[current.element] = [index]
}
})
add a comment |
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[Int]](), { result, current in
if result[current.element] != nil {
result[current.element]!.append(current.offset)
} else {
result[current.element] = [current.offset]
}
})
print(charsWithIndices)
["W": [6], "D": [10], "R": [8], "E": [1], "H": [0], " ": [5], "O": [4, 7], "L": [2, 3, 9]]
Or if you want to use the indices straight away for indexing your String, you can change make charsWithIndices
of type [Character:[String.Index]]
:
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[String.Index]](), { result, current in
let index = helloWorld.index(helloWorld.startIndex, offsetBy: current.offset)
if result[current.element] != nil {
result[current.element]!.append(index)
} else {
result[current.element] = [index]
}
})
add a comment |
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[Int]](), { result, current in
if result[current.element] != nil {
result[current.element]!.append(current.offset)
} else {
result[current.element] = [current.offset]
}
})
print(charsWithIndices)
["W": [6], "D": [10], "R": [8], "E": [1], "H": [0], " ": [5], "O": [4, 7], "L": [2, 3, 9]]
Or if you want to use the indices straight away for indexing your String, you can change make charsWithIndices
of type [Character:[String.Index]]
:
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[String.Index]](), { result, current in
let index = helloWorld.index(helloWorld.startIndex, offsetBy: current.offset)
if result[current.element] != nil {
result[current.element]!.append(index)
} else {
result[current.element] = [index]
}
})
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[Int]](), { result, current in
if result[current.element] != nil {
result[current.element]!.append(current.offset)
} else {
result[current.element] = [current.offset]
}
})
print(charsWithIndices)
["W": [6], "D": [10], "R": [8], "E": [1], "H": [0], " ": [5], "O": [4, 7], "L": [2, 3, 9]]
Or if you want to use the indices straight away for indexing your String, you can change make charsWithIndices
of type [Character:[String.Index]]
:
You can use reduce(into:)
on the enumerated
String.
let helloWorld = "HELLO WORLD"
let charsWithIndices = helloWorld.enumerated().reduce(into: [Character:[String.Index]](), { result, current in
let index = helloWorld.index(helloWorld.startIndex, offsetBy: current.offset)
if result[current.element] != nil {
result[current.element]!.append(index)
} else {
result[current.element] = [index]
}
})
answered Nov 15 '18 at 16:03
Dávid PásztorDávid Pásztor
22.4k82850
22.4k82850
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323069%2fhow-to-get-the-index-of-a-reoccurring-character-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KRDc 4SnYgHcW1pB,RtQ,CMMnQFJ 9kwfYUpQSaPAHVKq4eTb,Zeyx1v2rEdNwWRN4T
3
It would be useful to show what you have already tried
– Matt
Nov 15 '18 at 15:59