@property setter not called in python 3
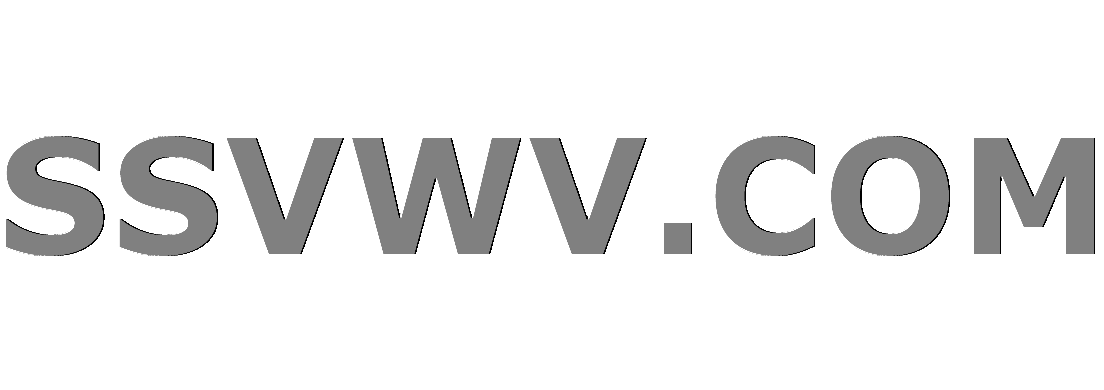
Multi tool use
I have a big issue, I'm new with @property and setter but I need to use them for a University Assignment: my problem is that the setters are always bypassed by the getters and also, when i try to pass an argument to the setter, it doesn't work:
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def getCiao(self):
return self.ciao
@getCiao.setter
def setCiao(self,dummy):
self.ciao = dummy
Then, when i call it I have either:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao('setter')
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-18-78a6b4607757> in < module>()
1 pizzo = DumbClass('getter')
----> 2 pozza = pizzo.getCiao('setter')
3 print(pizzo.ciao, pozza)
TypeError: 'str' object is not callable
Or, if I don't pass any arguments:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
getter getter
I can I make the setter to be called?
python python-3.x setter getter python-decorators
add a comment |
I have a big issue, I'm new with @property and setter but I need to use them for a University Assignment: my problem is that the setters are always bypassed by the getters and also, when i try to pass an argument to the setter, it doesn't work:
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def getCiao(self):
return self.ciao
@getCiao.setter
def setCiao(self,dummy):
self.ciao = dummy
Then, when i call it I have either:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao('setter')
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-18-78a6b4607757> in < module>()
1 pizzo = DumbClass('getter')
----> 2 pozza = pizzo.getCiao('setter')
3 print(pizzo.ciao, pozza)
TypeError: 'str' object is not callable
Or, if I don't pass any arguments:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
getter getter
I can I make the setter to be called?
python python-3.x setter getter python-decorators
3
You've defined a property calledgetCiao
, notciao
.ciao
is still just an attribute.
– khelwood
Nov 15 '18 at 15:45
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58
add a comment |
I have a big issue, I'm new with @property and setter but I need to use them for a University Assignment: my problem is that the setters are always bypassed by the getters and also, when i try to pass an argument to the setter, it doesn't work:
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def getCiao(self):
return self.ciao
@getCiao.setter
def setCiao(self,dummy):
self.ciao = dummy
Then, when i call it I have either:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao('setter')
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-18-78a6b4607757> in < module>()
1 pizzo = DumbClass('getter')
----> 2 pozza = pizzo.getCiao('setter')
3 print(pizzo.ciao, pozza)
TypeError: 'str' object is not callable
Or, if I don't pass any arguments:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
getter getter
I can I make the setter to be called?
python python-3.x setter getter python-decorators
I have a big issue, I'm new with @property and setter but I need to use them for a University Assignment: my problem is that the setters are always bypassed by the getters and also, when i try to pass an argument to the setter, it doesn't work:
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def getCiao(self):
return self.ciao
@getCiao.setter
def setCiao(self,dummy):
self.ciao = dummy
Then, when i call it I have either:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao('setter')
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-18-78a6b4607757> in < module>()
1 pizzo = DumbClass('getter')
----> 2 pozza = pizzo.getCiao('setter')
3 print(pizzo.ciao, pozza)
TypeError: 'str' object is not callable
Or, if I don't pass any arguments:
pizzo = DumbClass('getter')
pozza = pizzo.getCiao
print(pizzo.ciao, pozza)
----------------------------------------------------------------------
getter getter
I can I make the setter to be called?
python python-3.x setter getter python-decorators
python python-3.x setter getter python-decorators
edited Nov 15 '18 at 15:45


khelwood
31.6k74365
31.6k74365
asked Nov 15 '18 at 15:43
Fabio MagarelliFabio Magarelli
298
298
3
You've defined a property calledgetCiao
, notciao
.ciao
is still just an attribute.
– khelwood
Nov 15 '18 at 15:45
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58
add a comment |
3
You've defined a property calledgetCiao
, notciao
.ciao
is still just an attribute.
– khelwood
Nov 15 '18 at 15:45
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58
3
3
You've defined a property called
getCiao
, not ciao
. ciao
is still just an attribute.– khelwood
Nov 15 '18 at 15:45
You've defined a property called
getCiao
, not ciao
. ciao
is still just an attribute.– khelwood
Nov 15 '18 at 15:45
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58
add a comment |
2 Answers
2
active
oldest
votes
The setter is only invoked when you try to assign to the property. In the line
pozza = pizzo.getCiao('setter')
you first access the value of the property with pizzo.getCiao
(which calls the getter and returns the string "getter"
, then attempts to call the string as a function with the argument 'setter'
.
You have basially created a read-only property:
>>> pizzo = DumbClass('getter')
>>> pizzo.getCiao
'getter'
>>> pizzo.getCiao = 'foo'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
This is because you didn't use the same name when defining both the getter and setter, so your property doesn't have a setter. (Arguably, getCiao.setter
should probably raise an error right away, since the name matters, but it doesn't. C'est la vie.)
So the right thing to do is use the same name for both. The usual convention is to use an private variable with the "same" name as the property to store the underlying data for simple properties like this.
class DumbClass:
def __init__(self, p):
self._ciao = p
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, v):
self._ciao = v
Now you should be able to both get and set the value of the propery.
>>> d = DumbClass("hi")
>>> d.ciao
'hi'
>>> d.ciao = 'bye'
>>> d.ciao
'bye'
add a comment |
Your property name should be ciao
and the real variable something like _ciao
So when you do self.ciao = ...
it will call the property
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, dummy):
self._ciao = dummy
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can dopizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.
– Moses Koledoye
Nov 15 '18 at 15:53
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323001%2fproperty-setter-not-called-in-python-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The setter is only invoked when you try to assign to the property. In the line
pozza = pizzo.getCiao('setter')
you first access the value of the property with pizzo.getCiao
(which calls the getter and returns the string "getter"
, then attempts to call the string as a function with the argument 'setter'
.
You have basially created a read-only property:
>>> pizzo = DumbClass('getter')
>>> pizzo.getCiao
'getter'
>>> pizzo.getCiao = 'foo'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
This is because you didn't use the same name when defining both the getter and setter, so your property doesn't have a setter. (Arguably, getCiao.setter
should probably raise an error right away, since the name matters, but it doesn't. C'est la vie.)
So the right thing to do is use the same name for both. The usual convention is to use an private variable with the "same" name as the property to store the underlying data for simple properties like this.
class DumbClass:
def __init__(self, p):
self._ciao = p
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, v):
self._ciao = v
Now you should be able to both get and set the value of the propery.
>>> d = DumbClass("hi")
>>> d.ciao
'hi'
>>> d.ciao = 'bye'
>>> d.ciao
'bye'
add a comment |
The setter is only invoked when you try to assign to the property. In the line
pozza = pizzo.getCiao('setter')
you first access the value of the property with pizzo.getCiao
(which calls the getter and returns the string "getter"
, then attempts to call the string as a function with the argument 'setter'
.
You have basially created a read-only property:
>>> pizzo = DumbClass('getter')
>>> pizzo.getCiao
'getter'
>>> pizzo.getCiao = 'foo'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
This is because you didn't use the same name when defining both the getter and setter, so your property doesn't have a setter. (Arguably, getCiao.setter
should probably raise an error right away, since the name matters, but it doesn't. C'est la vie.)
So the right thing to do is use the same name for both. The usual convention is to use an private variable with the "same" name as the property to store the underlying data for simple properties like this.
class DumbClass:
def __init__(self, p):
self._ciao = p
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, v):
self._ciao = v
Now you should be able to both get and set the value of the propery.
>>> d = DumbClass("hi")
>>> d.ciao
'hi'
>>> d.ciao = 'bye'
>>> d.ciao
'bye'
add a comment |
The setter is only invoked when you try to assign to the property. In the line
pozza = pizzo.getCiao('setter')
you first access the value of the property with pizzo.getCiao
(which calls the getter and returns the string "getter"
, then attempts to call the string as a function with the argument 'setter'
.
You have basially created a read-only property:
>>> pizzo = DumbClass('getter')
>>> pizzo.getCiao
'getter'
>>> pizzo.getCiao = 'foo'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
This is because you didn't use the same name when defining both the getter and setter, so your property doesn't have a setter. (Arguably, getCiao.setter
should probably raise an error right away, since the name matters, but it doesn't. C'est la vie.)
So the right thing to do is use the same name for both. The usual convention is to use an private variable with the "same" name as the property to store the underlying data for simple properties like this.
class DumbClass:
def __init__(self, p):
self._ciao = p
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, v):
self._ciao = v
Now you should be able to both get and set the value of the propery.
>>> d = DumbClass("hi")
>>> d.ciao
'hi'
>>> d.ciao = 'bye'
>>> d.ciao
'bye'
The setter is only invoked when you try to assign to the property. In the line
pozza = pizzo.getCiao('setter')
you first access the value of the property with pizzo.getCiao
(which calls the getter and returns the string "getter"
, then attempts to call the string as a function with the argument 'setter'
.
You have basially created a read-only property:
>>> pizzo = DumbClass('getter')
>>> pizzo.getCiao
'getter'
>>> pizzo.getCiao = 'foo'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
This is because you didn't use the same name when defining both the getter and setter, so your property doesn't have a setter. (Arguably, getCiao.setter
should probably raise an error right away, since the name matters, but it doesn't. C'est la vie.)
So the right thing to do is use the same name for both. The usual convention is to use an private variable with the "same" name as the property to store the underlying data for simple properties like this.
class DumbClass:
def __init__(self, p):
self._ciao = p
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, v):
self._ciao = v
Now you should be able to both get and set the value of the propery.
>>> d = DumbClass("hi")
>>> d.ciao
'hi'
>>> d.ciao = 'bye'
>>> d.ciao
'bye'
answered Nov 15 '18 at 15:57
chepnerchepner
256k34244337
256k34244337
add a comment |
add a comment |
Your property name should be ciao
and the real variable something like _ciao
So when you do self.ciao = ...
it will call the property
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, dummy):
self._ciao = dummy
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can dopizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.
– Moses Koledoye
Nov 15 '18 at 15:53
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
add a comment |
Your property name should be ciao
and the real variable something like _ciao
So when you do self.ciao = ...
it will call the property
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, dummy):
self._ciao = dummy
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can dopizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.
– Moses Koledoye
Nov 15 '18 at 15:53
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
add a comment |
Your property name should be ciao
and the real variable something like _ciao
So when you do self.ciao = ...
it will call the property
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, dummy):
self._ciao = dummy
Your property name should be ciao
and the real variable something like _ciao
So when you do self.ciao = ...
it will call the property
class DumbClass():
def __init__(self, classParam):
self.ciao = classParam
@property
def ciao(self):
return self._ciao
@ciao.setter
def ciao(self, dummy):
self._ciao = dummy
answered Nov 15 '18 at 15:46
JBernardoJBernardo
22.7k56397
22.7k56397
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can dopizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.
– Moses Koledoye
Nov 15 '18 at 15:53
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
add a comment |
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can dopizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.
– Moses Koledoye
Nov 15 '18 at 15:53
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
@MosesKoledoye The explaining is quite simple and in the 1st line of this answer. The property name should be the one he wants to use.
– JBernardo
Nov 15 '18 at 15:51
Although a misnomer, they can do
pizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.– Moses Koledoye
Nov 15 '18 at 15:53
Although a misnomer, they can do
pizzo.getCiao = ...
to set the property. OP seems to be misunderstanding how properties work, not just the naming convention which you've very well explained.– Moses Koledoye
Nov 15 '18 at 15:53
1
1
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
Guys thank you very much you saved me! :)
– Fabio Magarelli
Nov 15 '18 at 15:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323001%2fproperty-setter-not-called-in-python-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RpaMHZT0fGr078Cn9NZ OZ Suksk7J,7,L4ydElm
3
You've defined a property called
getCiao
, notciao
.ciao
is still just an attribute.– khelwood
Nov 15 '18 at 15:45
Thank you Khelwood, I knew that there was something wrong with that...
– Fabio Magarelli
Nov 15 '18 at 15:58