Read all the text files in a folder and change a character in a string if it presents
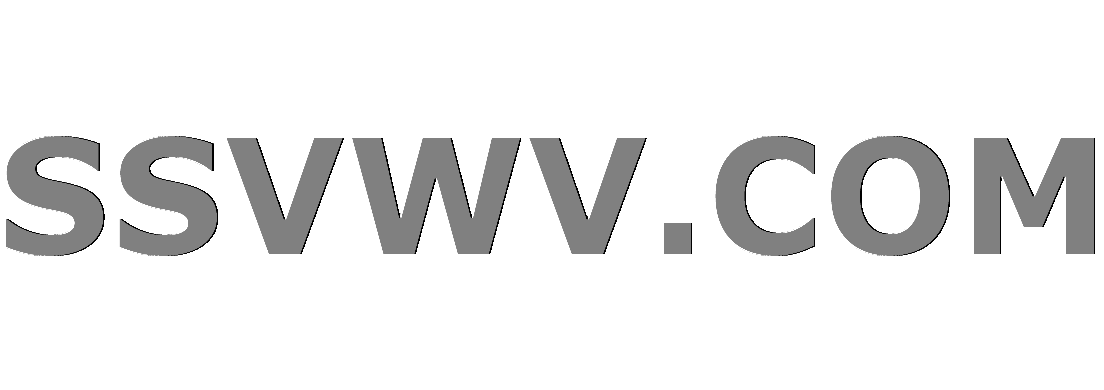
Multi tool use
I have a folder with csv formated documents with a .arw extension. Files are named as 1.arw, 2.arw, 3.arw
... etc.
I would like to write a code that reads all the files, checks and replaces the forwardslash /
with a dash -
. And finally creates new files with the replaced character.
The code I wrote as follows:
for i in range(1,6):
my_file=open("/path/"+str(i)+".arw", "r+")
str=my_file.read()
if "/" not in str:
print("There is no forwardslash")
else:
str_new = str.replace("/","-")
print(str_new)
f = open("/path/new"+str(i)+".arw", "w")
f.write(str_new)
my_file.close()
But I get an error saying:
'str' object is not callable.
How can I make it work for all the files in a folder? Apparently my for loop does not work.
python string replace
|
show 4 more comments
I have a folder with csv formated documents with a .arw extension. Files are named as 1.arw, 2.arw, 3.arw
... etc.
I would like to write a code that reads all the files, checks and replaces the forwardslash /
with a dash -
. And finally creates new files with the replaced character.
The code I wrote as follows:
for i in range(1,6):
my_file=open("/path/"+str(i)+".arw", "r+")
str=my_file.read()
if "/" not in str:
print("There is no forwardslash")
else:
str_new = str.replace("/","-")
print(str_new)
f = open("/path/new"+str(i)+".arw", "w")
f.write(str_new)
my_file.close()
But I get an error saying:
'str' object is not callable.
How can I make it work for all the files in a folder? Apparently my for loop does not work.
python string replace
You should not usestr
as a variable name, since python already uses it.
– Bernhard
Nov 16 '18 at 7:50
1
Any reason you preferPython
here instead ofsed
orawk
?
– l'L'l
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
1
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
1
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15
|
show 4 more comments
I have a folder with csv formated documents with a .arw extension. Files are named as 1.arw, 2.arw, 3.arw
... etc.
I would like to write a code that reads all the files, checks and replaces the forwardslash /
with a dash -
. And finally creates new files with the replaced character.
The code I wrote as follows:
for i in range(1,6):
my_file=open("/path/"+str(i)+".arw", "r+")
str=my_file.read()
if "/" not in str:
print("There is no forwardslash")
else:
str_new = str.replace("/","-")
print(str_new)
f = open("/path/new"+str(i)+".arw", "w")
f.write(str_new)
my_file.close()
But I get an error saying:
'str' object is not callable.
How can I make it work for all the files in a folder? Apparently my for loop does not work.
python string replace
I have a folder with csv formated documents with a .arw extension. Files are named as 1.arw, 2.arw, 3.arw
... etc.
I would like to write a code that reads all the files, checks and replaces the forwardslash /
with a dash -
. And finally creates new files with the replaced character.
The code I wrote as follows:
for i in range(1,6):
my_file=open("/path/"+str(i)+".arw", "r+")
str=my_file.read()
if "/" not in str:
print("There is no forwardslash")
else:
str_new = str.replace("/","-")
print(str_new)
f = open("/path/new"+str(i)+".arw", "w")
f.write(str_new)
my_file.close()
But I get an error saying:
'str' object is not callable.
How can I make it work for all the files in a folder? Apparently my for loop does not work.
python string replace
python string replace
edited Nov 16 '18 at 8:00
atline
3,159102138
3,159102138
asked Nov 16 '18 at 7:48
Yigit AltayYigit Altay
206
206
You should not usestr
as a variable name, since python already uses it.
– Bernhard
Nov 16 '18 at 7:50
1
Any reason you preferPython
here instead ofsed
orawk
?
– l'L'l
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
1
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
1
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15
|
show 4 more comments
You should not usestr
as a variable name, since python already uses it.
– Bernhard
Nov 16 '18 at 7:50
1
Any reason you preferPython
here instead ofsed
orawk
?
– l'L'l
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
1
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
1
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15
You should not use
str
as a variable name, since python already uses it.– Bernhard
Nov 16 '18 at 7:50
You should not use
str
as a variable name, since python already uses it.– Bernhard
Nov 16 '18 at 7:50
1
1
Any reason you prefer
Python
here instead of sed
or awk
?– l'L'l
Nov 16 '18 at 7:50
Any reason you prefer
Python
here instead of sed
or awk
?– l'L'l
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
1
1
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
1
1
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15
|
show 4 more comments
2 Answers
2
active
oldest
votes
The actual error is that you are replacing the built-in str
with your own variable with the same name, then try to use the built-in str()
after that.
Simply renaming the variable fixes the immediate problem, but you really want to refactor the code to avoid reading the entire file into memory.
import logging
import os
for i in range(1,6):
seen_slash = False
input_filename = "/path/"+str(i)+".arw"
output_filename = "/path/new"+str(i)+".arw"
with open(input_filename, "r+") as input, open(output_filename, "w") as output:
for line in input:
if not seen_slash and "/" in line:
seen_slash = True
line_new = line.replace("/","-")
print(line_new.rstrip('n')) # don't duplicate newline
output.write(line_new)
if not seen_slash:
logging.warn("{0}: No slash found".format(input_filename))
os.unlink(output_filename)
Using logging
instead of print
for error messages helps because you keep standard output (the print
output) separate from the diagnostics (the logging
output). Notice also how the diagnostic message includes the name of the file we found the problem in.
Going back and deleting the output filename when you have examined the entire input file and not found any slashes is a mild wart, but should typically be more efficient.
add a comment |
This is how I would do it:
for i in range(1,6):
with open((str(i)+'.arw'), 'r') as f:
data = f.readlines()
for element in data:
element.replace('/', '-')
f.close()
with open((str(i)+'.arw'), 'w') as f:
for element in data:
f.write(element)
f.close()
this is assuming from your post that you know that you have 6 files
if you don't know how many files you have you can use the OS module to find the files in the directory.
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53333504%2fread-all-the-text-files-in-a-folder-and-change-a-character-in-a-string-if-it-pre%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The actual error is that you are replacing the built-in str
with your own variable with the same name, then try to use the built-in str()
after that.
Simply renaming the variable fixes the immediate problem, but you really want to refactor the code to avoid reading the entire file into memory.
import logging
import os
for i in range(1,6):
seen_slash = False
input_filename = "/path/"+str(i)+".arw"
output_filename = "/path/new"+str(i)+".arw"
with open(input_filename, "r+") as input, open(output_filename, "w") as output:
for line in input:
if not seen_slash and "/" in line:
seen_slash = True
line_new = line.replace("/","-")
print(line_new.rstrip('n')) # don't duplicate newline
output.write(line_new)
if not seen_slash:
logging.warn("{0}: No slash found".format(input_filename))
os.unlink(output_filename)
Using logging
instead of print
for error messages helps because you keep standard output (the print
output) separate from the diagnostics (the logging
output). Notice also how the diagnostic message includes the name of the file we found the problem in.
Going back and deleting the output filename when you have examined the entire input file and not found any slashes is a mild wart, but should typically be more efficient.
add a comment |
The actual error is that you are replacing the built-in str
with your own variable with the same name, then try to use the built-in str()
after that.
Simply renaming the variable fixes the immediate problem, but you really want to refactor the code to avoid reading the entire file into memory.
import logging
import os
for i in range(1,6):
seen_slash = False
input_filename = "/path/"+str(i)+".arw"
output_filename = "/path/new"+str(i)+".arw"
with open(input_filename, "r+") as input, open(output_filename, "w") as output:
for line in input:
if not seen_slash and "/" in line:
seen_slash = True
line_new = line.replace("/","-")
print(line_new.rstrip('n')) # don't duplicate newline
output.write(line_new)
if not seen_slash:
logging.warn("{0}: No slash found".format(input_filename))
os.unlink(output_filename)
Using logging
instead of print
for error messages helps because you keep standard output (the print
output) separate from the diagnostics (the logging
output). Notice also how the diagnostic message includes the name of the file we found the problem in.
Going back and deleting the output filename when you have examined the entire input file and not found any slashes is a mild wart, but should typically be more efficient.
add a comment |
The actual error is that you are replacing the built-in str
with your own variable with the same name, then try to use the built-in str()
after that.
Simply renaming the variable fixes the immediate problem, but you really want to refactor the code to avoid reading the entire file into memory.
import logging
import os
for i in range(1,6):
seen_slash = False
input_filename = "/path/"+str(i)+".arw"
output_filename = "/path/new"+str(i)+".arw"
with open(input_filename, "r+") as input, open(output_filename, "w") as output:
for line in input:
if not seen_slash and "/" in line:
seen_slash = True
line_new = line.replace("/","-")
print(line_new.rstrip('n')) # don't duplicate newline
output.write(line_new)
if not seen_slash:
logging.warn("{0}: No slash found".format(input_filename))
os.unlink(output_filename)
Using logging
instead of print
for error messages helps because you keep standard output (the print
output) separate from the diagnostics (the logging
output). Notice also how the diagnostic message includes the name of the file we found the problem in.
Going back and deleting the output filename when you have examined the entire input file and not found any slashes is a mild wart, but should typically be more efficient.
The actual error is that you are replacing the built-in str
with your own variable with the same name, then try to use the built-in str()
after that.
Simply renaming the variable fixes the immediate problem, but you really want to refactor the code to avoid reading the entire file into memory.
import logging
import os
for i in range(1,6):
seen_slash = False
input_filename = "/path/"+str(i)+".arw"
output_filename = "/path/new"+str(i)+".arw"
with open(input_filename, "r+") as input, open(output_filename, "w") as output:
for line in input:
if not seen_slash and "/" in line:
seen_slash = True
line_new = line.replace("/","-")
print(line_new.rstrip('n')) # don't duplicate newline
output.write(line_new)
if not seen_slash:
logging.warn("{0}: No slash found".format(input_filename))
os.unlink(output_filename)
Using logging
instead of print
for error messages helps because you keep standard output (the print
output) separate from the diagnostics (the logging
output). Notice also how the diagnostic message includes the name of the file we found the problem in.
Going back and deleting the output filename when you have examined the entire input file and not found any slashes is a mild wart, but should typically be more efficient.
answered Nov 16 '18 at 8:16
tripleeetripleee
94.9k13133189
94.9k13133189
add a comment |
add a comment |
This is how I would do it:
for i in range(1,6):
with open((str(i)+'.arw'), 'r') as f:
data = f.readlines()
for element in data:
element.replace('/', '-')
f.close()
with open((str(i)+'.arw'), 'w') as f:
for element in data:
f.write(element)
f.close()
this is assuming from your post that you know that you have 6 files
if you don't know how many files you have you can use the OS module to find the files in the directory.
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
add a comment |
This is how I would do it:
for i in range(1,6):
with open((str(i)+'.arw'), 'r') as f:
data = f.readlines()
for element in data:
element.replace('/', '-')
f.close()
with open((str(i)+'.arw'), 'w') as f:
for element in data:
f.write(element)
f.close()
this is assuming from your post that you know that you have 6 files
if you don't know how many files you have you can use the OS module to find the files in the directory.
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
add a comment |
This is how I would do it:
for i in range(1,6):
with open((str(i)+'.arw'), 'r') as f:
data = f.readlines()
for element in data:
element.replace('/', '-')
f.close()
with open((str(i)+'.arw'), 'w') as f:
for element in data:
f.write(element)
f.close()
this is assuming from your post that you know that you have 6 files
if you don't know how many files you have you can use the OS module to find the files in the directory.
This is how I would do it:
for i in range(1,6):
with open((str(i)+'.arw'), 'r') as f:
data = f.readlines()
for element in data:
element.replace('/', '-')
f.close()
with open((str(i)+'.arw'), 'w') as f:
for element in data:
f.write(element)
f.close()
this is assuming from your post that you know that you have 6 files
if you don't know how many files you have you can use the OS module to find the files in the directory.
edited Nov 16 '18 at 8:03
kimo26
806
806
answered Nov 16 '18 at 7:59


hhaefligerhhaefliger
29712
29712
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
add a comment |
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
Reading the data into a list and iterating over that list is inefficient because (a) you effectively loop over it twice, and (b) if the file is big, you use a lot of memory.
– tripleee
Nov 16 '18 at 8:05
1
1
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
it is an effective and easily understandable solution to the problem, as well as this, the str.replace() method is effectively the same. I am also assuming that as he is doing this his files are not too big. Thanks for the feedback though
– hhaefliger
Nov 16 '18 at 8:09
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
The file contents are not huge. So this works ok as well. But the strategy suggested by @triplee below is even better just in case the content is big.
– Yigit Altay
Nov 16 '18 at 9:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53333504%2fread-all-the-text-files-in-a-folder-and-change-a-character-in-a-string-if-it-pre%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2,F0Ti,jppbFMjyfh5KXBW8gZeOHQGJxrl7,MQhSJt,l,z3HX5k74tiDsXIJLDnKxV6KYDRCjjLNt
You should not use
str
as a variable name, since python already uses it.– Bernhard
Nov 16 '18 at 7:50
1
Any reason you prefer
Python
here instead ofsed
orawk
?– l'L'l
Nov 16 '18 at 7:50
Your code has obvious syntax errors, could you please edit to fix the indentation?
– tripleee
Nov 16 '18 at 7:50
1
@lagom Your edit is obviously well-intentioned, but second-guessing the OP's actual code is something we try to avoid. meta.stackoverflow.com/questions/348957/…
– tripleee
Nov 16 '18 at 8:04
1
My guess would be similar to yours, but again, we are not really supposed to be guessing these things. Eventually close as unclear if the OP is unable to post exactly the actual code they are having problems with.
– tripleee
Nov 16 '18 at 8:15