Parse JSON in Python
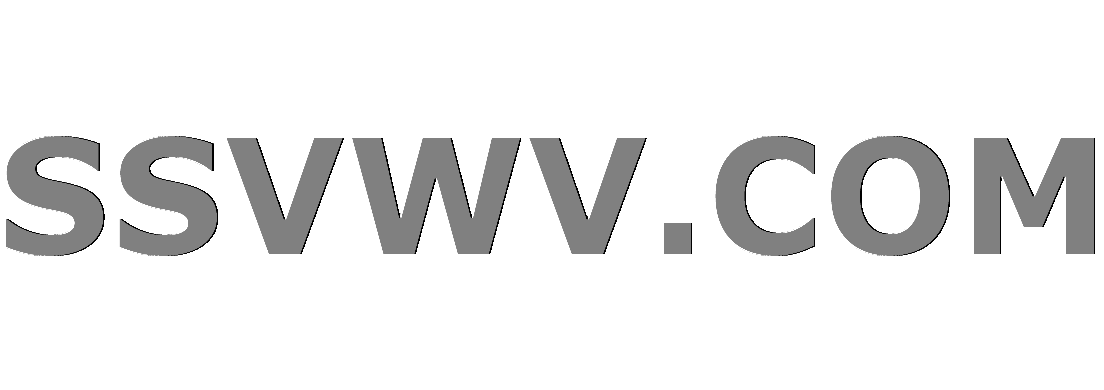
Multi tool use
My project is currently receiving a JSON message in python which I need to get bits of information out of. For the purposes of this, lets set it to some simple JSON in a string:
jsonStr = '{"one" : "1", "two" : "2", "three" : "3"}'
So far I've been generating JSON requests using a list and then json.dumps
but to do the opposite of this I think I need to use json.loads
but I haven't had much luck with it. Could anyone provide me a snippet that would return "2" with the input of "two" in the above example?
python json parsing
add a comment |
My project is currently receiving a JSON message in python which I need to get bits of information out of. For the purposes of this, lets set it to some simple JSON in a string:
jsonStr = '{"one" : "1", "two" : "2", "three" : "3"}'
So far I've been generating JSON requests using a list and then json.dumps
but to do the opposite of this I think I need to use json.loads
but I haven't had much luck with it. Could anyone provide me a snippet that would return "2" with the input of "two" in the above example?
python json parsing
3
Note: For those that come here with data that uses'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use"
delimiters. If so, repair your code that produces that output to usejson.dumps()
instead ofstr()
orrepr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look forNone
,True
orFalse
, JSON would usenull
,true
&false
.
– Martijn Pieters♦
Nov 22 '18 at 13:25
add a comment |
My project is currently receiving a JSON message in python which I need to get bits of information out of. For the purposes of this, lets set it to some simple JSON in a string:
jsonStr = '{"one" : "1", "two" : "2", "three" : "3"}'
So far I've been generating JSON requests using a list and then json.dumps
but to do the opposite of this I think I need to use json.loads
but I haven't had much luck with it. Could anyone provide me a snippet that would return "2" with the input of "two" in the above example?
python json parsing
My project is currently receiving a JSON message in python which I need to get bits of information out of. For the purposes of this, lets set it to some simple JSON in a string:
jsonStr = '{"one" : "1", "two" : "2", "three" : "3"}'
So far I've been generating JSON requests using a list and then json.dumps
but to do the opposite of this I think I need to use json.loads
but I haven't had much luck with it. Could anyone provide me a snippet that would return "2" with the input of "two" in the above example?
python json parsing
python json parsing
edited Nov 8 '15 at 6:00


Kevin Guan
14.1k103968
14.1k103968
asked Oct 14 '11 at 17:00
ingh.amingh.am
14.9k37120174
14.9k37120174
3
Note: For those that come here with data that uses'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use"
delimiters. If so, repair your code that produces that output to usejson.dumps()
instead ofstr()
orrepr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look forNone
,True
orFalse
, JSON would usenull
,true
&false
.
– Martijn Pieters♦
Nov 22 '18 at 13:25
add a comment |
3
Note: For those that come here with data that uses'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use"
delimiters. If so, repair your code that produces that output to usejson.dumps()
instead ofstr()
orrepr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look forNone
,True
orFalse
, JSON would usenull
,true
&false
.
– Martijn Pieters♦
Nov 22 '18 at 13:25
3
3
Note: For those that come here with data that uses
'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use "
delimiters. If so, repair your code that produces that output to use json.dumps()
instead of str()
or repr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look for None
, True
or False
, JSON would use null
, true
& false
.– Martijn Pieters♦
Nov 22 '18 at 13:25
Note: For those that come here with data that uses
'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use "
delimiters. If so, repair your code that produces that output to use json.dumps()
instead of str()
or repr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look for None
, True
or False
, JSON would use null
, true
& false
.– Martijn Pieters♦
Nov 22 '18 at 13:25
add a comment |
4 Answers
4
active
oldest
votes
Very simple:
import json
j = json.loads('{"one" : "1", "two" : "2", "three" : "3"}')
print j['two']
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
I'm actually using simplejson already:import simplejson as json
. Forgot to mention but thanks :)
– ingh.am
Oct 15 '11 at 20:22
Got it. Was using.load
method instead of.loads
– Sunil Kumar
Sep 21 '18 at 6:33
add a comment |
Sometimes your json is not a string. For example if you are getting a json from a url like this:
j = urllib2.urlopen('http://site.com/data.json')
you will need to use json.load, not json.loads:
j_obj = json.load(j)
(it is easy to forget: the 's' is for 'string')
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
add a comment |
For URL or FIle, use json.load(). For string having .json content, use json.loads().
#! /usr/bin/python
import json
from pprint import pprint
#json_file='a.json'
json_file='my_cube.json'
cube='1'
json_data=open(json_file)
data = json.load(json_data)
#pprint(data)
json_data.close()
print "Dimension: ", data['cubes'][cube]['dim']
print "Measures: ", data['cubes'][cube]['meas']
add a comment |
Following is simple example that may help you:
json_string = """
{
"pk": 1,
"fa": "cc.ee",
"fb": {
"fc": "",
"fd_id": "12345"
}
}"""
import json
data = json.loads(json_string)
if data["fa"] == "cc.ee":
data["fb"]["new_key"] = "cc.ee was present!"
print json.dumps(data)
The output for the above code will be:
{"pk": 1, "fb": {"new_key": "cc.ee was present!", "fd_id": "12345",
"fc": ""}, "fa": "cc.ee"}
Note that you can set the ident argument of dump to print it like so (for example,when using print json.dumps(data , indent=4)):
{
"pk": 1,
"fb": {
"new_key": "cc.ee was present!",
"fd_id": "12345",
"fc": ""
},
"fa": "cc.ee"
}
add a comment |
protected by Community♦ Apr 12 '17 at 1:50
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Very simple:
import json
j = json.loads('{"one" : "1", "two" : "2", "three" : "3"}')
print j['two']
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
I'm actually using simplejson already:import simplejson as json
. Forgot to mention but thanks :)
– ingh.am
Oct 15 '11 at 20:22
Got it. Was using.load
method instead of.loads
– Sunil Kumar
Sep 21 '18 at 6:33
add a comment |
Very simple:
import json
j = json.loads('{"one" : "1", "two" : "2", "three" : "3"}')
print j['two']
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
I'm actually using simplejson already:import simplejson as json
. Forgot to mention but thanks :)
– ingh.am
Oct 15 '11 at 20:22
Got it. Was using.load
method instead of.loads
– Sunil Kumar
Sep 21 '18 at 6:33
add a comment |
Very simple:
import json
j = json.loads('{"one" : "1", "two" : "2", "three" : "3"}')
print j['two']
Very simple:
import json
j = json.loads('{"one" : "1", "two" : "2", "three" : "3"}')
print j['two']
answered Oct 14 '11 at 17:05
John GiottaJohn Giotta
12.3k43871
12.3k43871
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
I'm actually using simplejson already:import simplejson as json
. Forgot to mention but thanks :)
– ingh.am
Oct 15 '11 at 20:22
Got it. Was using.load
method instead of.loads
– Sunil Kumar
Sep 21 '18 at 6:33
add a comment |
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
I'm actually using simplejson already:import simplejson as json
. Forgot to mention but thanks :)
– ingh.am
Oct 15 '11 at 20:22
Got it. Was using.load
method instead of.loads
– Sunil Kumar
Sep 21 '18 at 6:33
1
1
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Thanks. I feel silly now, I was trying to do it by index...
– ingh.am
Oct 14 '11 at 17:06
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
Also, have a look at simplejson if you need better performance. More recent versions provide optimizations that greatly improve read and writing.
– unode
Oct 14 '11 at 19:11
2
2
I'm actually using simplejson already:
import simplejson as json
. Forgot to mention but thanks :)– ingh.am
Oct 15 '11 at 20:22
I'm actually using simplejson already:
import simplejson as json
. Forgot to mention but thanks :)– ingh.am
Oct 15 '11 at 20:22
Got it. Was using
.load
method instead of .loads
– Sunil Kumar
Sep 21 '18 at 6:33
Got it. Was using
.load
method instead of .loads
– Sunil Kumar
Sep 21 '18 at 6:33
add a comment |
Sometimes your json is not a string. For example if you are getting a json from a url like this:
j = urllib2.urlopen('http://site.com/data.json')
you will need to use json.load, not json.loads:
j_obj = json.load(j)
(it is easy to forget: the 's' is for 'string')
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
add a comment |
Sometimes your json is not a string. For example if you are getting a json from a url like this:
j = urllib2.urlopen('http://site.com/data.json')
you will need to use json.load, not json.loads:
j_obj = json.load(j)
(it is easy to forget: the 's' is for 'string')
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
add a comment |
Sometimes your json is not a string. For example if you are getting a json from a url like this:
j = urllib2.urlopen('http://site.com/data.json')
you will need to use json.load, not json.loads:
j_obj = json.load(j)
(it is easy to forget: the 's' is for 'string')
Sometimes your json is not a string. For example if you are getting a json from a url like this:
j = urllib2.urlopen('http://site.com/data.json')
you will need to use json.load, not json.loads:
j_obj = json.load(j)
(it is easy to forget: the 's' is for 'string')
answered Oct 14 '11 at 17:09
jisaacstonejisaacstone
3,12121934
3,12121934
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
add a comment |
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
1
1
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Didn't know that, thanks!
– ingh.am
Oct 15 '11 at 20:23
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
Just to add that you can get the string content by calling j.read() and then use the loads method. Any way in this case the load() method takes care of calling the .read()
– rkachach
Jan 15 '16 at 6:27
add a comment |
For URL or FIle, use json.load(). For string having .json content, use json.loads().
#! /usr/bin/python
import json
from pprint import pprint
#json_file='a.json'
json_file='my_cube.json'
cube='1'
json_data=open(json_file)
data = json.load(json_data)
#pprint(data)
json_data.close()
print "Dimension: ", data['cubes'][cube]['dim']
print "Measures: ", data['cubes'][cube]['meas']
add a comment |
For URL or FIle, use json.load(). For string having .json content, use json.loads().
#! /usr/bin/python
import json
from pprint import pprint
#json_file='a.json'
json_file='my_cube.json'
cube='1'
json_data=open(json_file)
data = json.load(json_data)
#pprint(data)
json_data.close()
print "Dimension: ", data['cubes'][cube]['dim']
print "Measures: ", data['cubes'][cube]['meas']
add a comment |
For URL or FIle, use json.load(). For string having .json content, use json.loads().
#! /usr/bin/python
import json
from pprint import pprint
#json_file='a.json'
json_file='my_cube.json'
cube='1'
json_data=open(json_file)
data = json.load(json_data)
#pprint(data)
json_data.close()
print "Dimension: ", data['cubes'][cube]['dim']
print "Measures: ", data['cubes'][cube]['meas']
For URL or FIle, use json.load(). For string having .json content, use json.loads().
#! /usr/bin/python
import json
from pprint import pprint
#json_file='a.json'
json_file='my_cube.json'
cube='1'
json_data=open(json_file)
data = json.load(json_data)
#pprint(data)
json_data.close()
print "Dimension: ", data['cubes'][cube]['dim']
print "Measures: ", data['cubes'][cube]['meas']
edited Jul 20 '13 at 4:53
Genghis Khan
6191821
6191821
answered Jul 5 '13 at 16:33


Mohammad Shahid SiddiquiMohammad Shahid Siddiqui
1,7981410
1,7981410
add a comment |
add a comment |
Following is simple example that may help you:
json_string = """
{
"pk": 1,
"fa": "cc.ee",
"fb": {
"fc": "",
"fd_id": "12345"
}
}"""
import json
data = json.loads(json_string)
if data["fa"] == "cc.ee":
data["fb"]["new_key"] = "cc.ee was present!"
print json.dumps(data)
The output for the above code will be:
{"pk": 1, "fb": {"new_key": "cc.ee was present!", "fd_id": "12345",
"fc": ""}, "fa": "cc.ee"}
Note that you can set the ident argument of dump to print it like so (for example,when using print json.dumps(data , indent=4)):
{
"pk": 1,
"fb": {
"new_key": "cc.ee was present!",
"fd_id": "12345",
"fc": ""
},
"fa": "cc.ee"
}
add a comment |
Following is simple example that may help you:
json_string = """
{
"pk": 1,
"fa": "cc.ee",
"fb": {
"fc": "",
"fd_id": "12345"
}
}"""
import json
data = json.loads(json_string)
if data["fa"] == "cc.ee":
data["fb"]["new_key"] = "cc.ee was present!"
print json.dumps(data)
The output for the above code will be:
{"pk": 1, "fb": {"new_key": "cc.ee was present!", "fd_id": "12345",
"fc": ""}, "fa": "cc.ee"}
Note that you can set the ident argument of dump to print it like so (for example,when using print json.dumps(data , indent=4)):
{
"pk": 1,
"fb": {
"new_key": "cc.ee was present!",
"fd_id": "12345",
"fc": ""
},
"fa": "cc.ee"
}
add a comment |
Following is simple example that may help you:
json_string = """
{
"pk": 1,
"fa": "cc.ee",
"fb": {
"fc": "",
"fd_id": "12345"
}
}"""
import json
data = json.loads(json_string)
if data["fa"] == "cc.ee":
data["fb"]["new_key"] = "cc.ee was present!"
print json.dumps(data)
The output for the above code will be:
{"pk": 1, "fb": {"new_key": "cc.ee was present!", "fd_id": "12345",
"fc": ""}, "fa": "cc.ee"}
Note that you can set the ident argument of dump to print it like so (for example,when using print json.dumps(data , indent=4)):
{
"pk": 1,
"fb": {
"new_key": "cc.ee was present!",
"fd_id": "12345",
"fc": ""
},
"fa": "cc.ee"
}
Following is simple example that may help you:
json_string = """
{
"pk": 1,
"fa": "cc.ee",
"fb": {
"fc": "",
"fd_id": "12345"
}
}"""
import json
data = json.loads(json_string)
if data["fa"] == "cc.ee":
data["fb"]["new_key"] = "cc.ee was present!"
print json.dumps(data)
The output for the above code will be:
{"pk": 1, "fb": {"new_key": "cc.ee was present!", "fd_id": "12345",
"fc": ""}, "fa": "cc.ee"}
Note that you can set the ident argument of dump to print it like so (for example,when using print json.dumps(data , indent=4)):
{
"pk": 1,
"fb": {
"new_key": "cc.ee was present!",
"fd_id": "12345",
"fc": ""
},
"fa": "cc.ee"
}
edited Nov 8 '17 at 14:18


Guy Avraham
1,62622233
1,62622233
answered Sep 30 '15 at 6:39
VenkatVenkat
26738
26738
add a comment |
add a comment |
protected by Community♦ Apr 12 '17 at 1:50
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
UG,jImCjnRPn bLUCL502 Q3HLw4FBgDwQhHjc vd 0mbfvvZgt5FIa hrGDkp V0YaYccd
3
Note: For those that come here with data that uses
'
single-quote string delimiters, you may have accidentally created string representations for Python dictionaries instead. JSON will always use"
delimiters. If so, repair your code that produces that output to usejson.dumps()
instead ofstr()
orrepr()
, and head over to Convert a String representation of a Dictionary to a dictionary? to figure out how to recover your Python data. Other clues you have a Python literal? Look forNone
,True
orFalse
, JSON would usenull
,true
&false
.– Martijn Pieters♦
Nov 22 '18 at 13:25