CIRadialGradient reduces image size
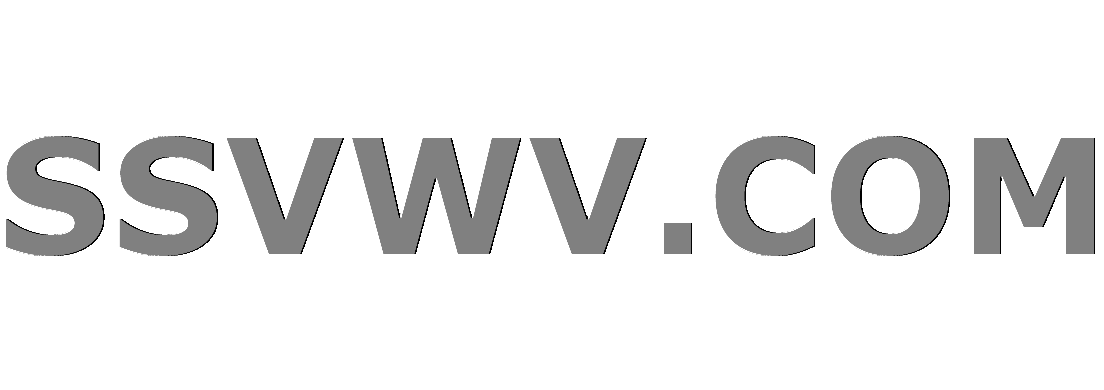
Multi tool use
After applying CIRadialGradient to my image it gets reduced in width by about 20%.
guard let image = bgImage.image, let cgimg = image.cgImage else {
print("imageView doesn't have an image!")
return
}
let coreImage = CIImage(cgImage:cgimg)
guard let radialMask = CIFilter(name:"CIRadialGradient") else {
return
}
guard let maskedVariableBlur = CIFilter(name:"CIMaskedVariableBlur") else {
print("CIMaskedVariableBlur does not exist")
return
}
maskedVariableBlur.setValue(coreImage, forKey: kCIInputImageKey)
maskedVariableBlur.setValue(radialMask.outputImage, forKey: "inputMask")
guard let selectivelyFocusedCIImage = maskedVariableBlur.outputImage else {
print("Setting maskedVariableBlur failed")
return
}
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage)
To clarify, bgImage
is a UIImageView
.
Why does this happen and how do I fix it?
Without RadialMask:
With RadialMask:
With the difference that on my physical iPhone the smaller image is aligned to the left.
ios swift xcode core-image
add a comment |
After applying CIRadialGradient to my image it gets reduced in width by about 20%.
guard let image = bgImage.image, let cgimg = image.cgImage else {
print("imageView doesn't have an image!")
return
}
let coreImage = CIImage(cgImage:cgimg)
guard let radialMask = CIFilter(name:"CIRadialGradient") else {
return
}
guard let maskedVariableBlur = CIFilter(name:"CIMaskedVariableBlur") else {
print("CIMaskedVariableBlur does not exist")
return
}
maskedVariableBlur.setValue(coreImage, forKey: kCIInputImageKey)
maskedVariableBlur.setValue(radialMask.outputImage, forKey: "inputMask")
guard let selectivelyFocusedCIImage = maskedVariableBlur.outputImage else {
print("Setting maskedVariableBlur failed")
return
}
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage)
To clarify, bgImage
is a UIImageView
.
Why does this happen and how do I fix it?
Without RadialMask:
With RadialMask:
With the difference that on my physical iPhone the smaller image is aligned to the left.
ios swift xcode core-image
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34
add a comment |
After applying CIRadialGradient to my image it gets reduced in width by about 20%.
guard let image = bgImage.image, let cgimg = image.cgImage else {
print("imageView doesn't have an image!")
return
}
let coreImage = CIImage(cgImage:cgimg)
guard let radialMask = CIFilter(name:"CIRadialGradient") else {
return
}
guard let maskedVariableBlur = CIFilter(name:"CIMaskedVariableBlur") else {
print("CIMaskedVariableBlur does not exist")
return
}
maskedVariableBlur.setValue(coreImage, forKey: kCIInputImageKey)
maskedVariableBlur.setValue(radialMask.outputImage, forKey: "inputMask")
guard let selectivelyFocusedCIImage = maskedVariableBlur.outputImage else {
print("Setting maskedVariableBlur failed")
return
}
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage)
To clarify, bgImage
is a UIImageView
.
Why does this happen and how do I fix it?
Without RadialMask:
With RadialMask:
With the difference that on my physical iPhone the smaller image is aligned to the left.
ios swift xcode core-image
After applying CIRadialGradient to my image it gets reduced in width by about 20%.
guard let image = bgImage.image, let cgimg = image.cgImage else {
print("imageView doesn't have an image!")
return
}
let coreImage = CIImage(cgImage:cgimg)
guard let radialMask = CIFilter(name:"CIRadialGradient") else {
return
}
guard let maskedVariableBlur = CIFilter(name:"CIMaskedVariableBlur") else {
print("CIMaskedVariableBlur does not exist")
return
}
maskedVariableBlur.setValue(coreImage, forKey: kCIInputImageKey)
maskedVariableBlur.setValue(radialMask.outputImage, forKey: "inputMask")
guard let selectivelyFocusedCIImage = maskedVariableBlur.outputImage else {
print("Setting maskedVariableBlur failed")
return
}
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage)
To clarify, bgImage
is a UIImageView
.
Why does this happen and how do I fix it?
Without RadialMask:
With RadialMask:
With the difference that on my physical iPhone the smaller image is aligned to the left.
ios swift xcode core-image
ios swift xcode core-image
edited Nov 9 '18 at 15:33
Sinan Samet
asked Nov 9 '18 at 15:18
Sinan SametSinan Samet
1,56442759
1,56442759
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34
add a comment |
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34
add a comment |
3 Answers
3
active
oldest
votes
I tend to explicitly state how big the image is by using a CIContext
and creating a specifically sized CGImage instead of simply using UIImage(ciImage:)
. Try this, assuming your inputImage is called coreGraphics
:
let ciCtx = CIContext()
let cgiig = ctx.createCGImage(selectivelyFocusedCIImage, from: coreImage.extent)
let uiImage = UIImage(cgImage: cgIMG!)
A few notes....
(1) I pulled this code out from an app I'm wrapping up. This is untested code (including the forced-unwrap), but the concept of what I'm doing is solid.
(2) You don't explain a lot of what you are trying to do, but when I see a variable named selectivelyFocusedCIImage
I get concerned that you may be trying to use CoreImage in a more interactive way than "just" creating one image. If you want "near real-time" performance, render the CIImage in either a (deprecated as of iOS 12) GLKView
or an MTKView
instead of a UIImageView
. The latter only uses the CPU where the two former use the GPU.
(3) Finally, a word of warning on CIContexts
- they are expensive to create! Usually you can code it such that there's only one context that can be shared by everything n your app.
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
I honestly cannot comment on whyUIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in theextent
you need. Note thatCIRadialGradient
could use an actualinputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.
– dfd
Nov 9 '18 at 21:19
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think usingUIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!
– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating aCIContext
every time.
– Frank Schlegel
Nov 16 '18 at 9:02
add a comment |
Look up the documentation, it's a mask that being applied to the image:
Docs: CIRadialGradient
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is aRadialGradient
, not aRadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:inputRadius1
andinputColor1
do seem to be as candidates to play around with.
– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
add a comment |
The different sizes are caused by the kernel size of the blur filter:
The blur filter needs to sample a region around each pixel. Since there are no pixels beyond the image bounds, Core Image reduces the extend
of the result image by half the kernel size (blur radius) to signal that for those pixels there is not enough information for a proper blur.
However, you can tell Core Image to treat the border pixels as extending infinitely in all directions so that the blur filter gets enough information even on the edges of the image. Afterwards you can crop the result back to the original dimension.
In your code, just change the following two lines:
maskedVariableBlur.setValue(coreImage.clampedToExtent(), forKey: kCIInputImageKey)
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage.cropped(to:coreImage.extend))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228471%2fciradialgradient-reduces-image-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I tend to explicitly state how big the image is by using a CIContext
and creating a specifically sized CGImage instead of simply using UIImage(ciImage:)
. Try this, assuming your inputImage is called coreGraphics
:
let ciCtx = CIContext()
let cgiig = ctx.createCGImage(selectivelyFocusedCIImage, from: coreImage.extent)
let uiImage = UIImage(cgImage: cgIMG!)
A few notes....
(1) I pulled this code out from an app I'm wrapping up. This is untested code (including the forced-unwrap), but the concept of what I'm doing is solid.
(2) You don't explain a lot of what you are trying to do, but when I see a variable named selectivelyFocusedCIImage
I get concerned that you may be trying to use CoreImage in a more interactive way than "just" creating one image. If you want "near real-time" performance, render the CIImage in either a (deprecated as of iOS 12) GLKView
or an MTKView
instead of a UIImageView
. The latter only uses the CPU where the two former use the GPU.
(3) Finally, a word of warning on CIContexts
- they are expensive to create! Usually you can code it such that there's only one context that can be shared by everything n your app.
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
I honestly cannot comment on whyUIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in theextent
you need. Note thatCIRadialGradient
could use an actualinputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.
– dfd
Nov 9 '18 at 21:19
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think usingUIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!
– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating aCIContext
every time.
– Frank Schlegel
Nov 16 '18 at 9:02
add a comment |
I tend to explicitly state how big the image is by using a CIContext
and creating a specifically sized CGImage instead of simply using UIImage(ciImage:)
. Try this, assuming your inputImage is called coreGraphics
:
let ciCtx = CIContext()
let cgiig = ctx.createCGImage(selectivelyFocusedCIImage, from: coreImage.extent)
let uiImage = UIImage(cgImage: cgIMG!)
A few notes....
(1) I pulled this code out from an app I'm wrapping up. This is untested code (including the forced-unwrap), but the concept of what I'm doing is solid.
(2) You don't explain a lot of what you are trying to do, but when I see a variable named selectivelyFocusedCIImage
I get concerned that you may be trying to use CoreImage in a more interactive way than "just" creating one image. If you want "near real-time" performance, render the CIImage in either a (deprecated as of iOS 12) GLKView
or an MTKView
instead of a UIImageView
. The latter only uses the CPU where the two former use the GPU.
(3) Finally, a word of warning on CIContexts
- they are expensive to create! Usually you can code it such that there's only one context that can be shared by everything n your app.
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
I honestly cannot comment on whyUIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in theextent
you need. Note thatCIRadialGradient
could use an actualinputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.
– dfd
Nov 9 '18 at 21:19
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think usingUIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!
– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating aCIContext
every time.
– Frank Schlegel
Nov 16 '18 at 9:02
add a comment |
I tend to explicitly state how big the image is by using a CIContext
and creating a specifically sized CGImage instead of simply using UIImage(ciImage:)
. Try this, assuming your inputImage is called coreGraphics
:
let ciCtx = CIContext()
let cgiig = ctx.createCGImage(selectivelyFocusedCIImage, from: coreImage.extent)
let uiImage = UIImage(cgImage: cgIMG!)
A few notes....
(1) I pulled this code out from an app I'm wrapping up. This is untested code (including the forced-unwrap), but the concept of what I'm doing is solid.
(2) You don't explain a lot of what you are trying to do, but when I see a variable named selectivelyFocusedCIImage
I get concerned that you may be trying to use CoreImage in a more interactive way than "just" creating one image. If you want "near real-time" performance, render the CIImage in either a (deprecated as of iOS 12) GLKView
or an MTKView
instead of a UIImageView
. The latter only uses the CPU where the two former use the GPU.
(3) Finally, a word of warning on CIContexts
- they are expensive to create! Usually you can code it such that there's only one context that can be shared by everything n your app.
I tend to explicitly state how big the image is by using a CIContext
and creating a specifically sized CGImage instead of simply using UIImage(ciImage:)
. Try this, assuming your inputImage is called coreGraphics
:
let ciCtx = CIContext()
let cgiig = ctx.createCGImage(selectivelyFocusedCIImage, from: coreImage.extent)
let uiImage = UIImage(cgImage: cgIMG!)
A few notes....
(1) I pulled this code out from an app I'm wrapping up. This is untested code (including the forced-unwrap), but the concept of what I'm doing is solid.
(2) You don't explain a lot of what you are trying to do, but when I see a variable named selectivelyFocusedCIImage
I get concerned that you may be trying to use CoreImage in a more interactive way than "just" creating one image. If you want "near real-time" performance, render the CIImage in either a (deprecated as of iOS 12) GLKView
or an MTKView
instead of a UIImageView
. The latter only uses the CPU where the two former use the GPU.
(3) Finally, a word of warning on CIContexts
- they are expensive to create! Usually you can code it such that there's only one context that can be shared by everything n your app.
answered Nov 9 '18 at 19:55
dfddfd
5,26831630
5,26831630
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
I honestly cannot comment on whyUIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in theextent
you need. Note thatCIRadialGradient
could use an actualinputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.
– dfd
Nov 9 '18 at 21:19
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think usingUIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!
– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating aCIContext
every time.
– Frank Schlegel
Nov 16 '18 at 9:02
add a comment |
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
I honestly cannot comment on whyUIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in theextent
you need. Note thatCIRadialGradient
could use an actualinputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.
– dfd
Nov 9 '18 at 21:19
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think usingUIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!
– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating aCIContext
every time.
– Frank Schlegel
Nov 16 '18 at 9:02
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
This really solved the problem thank you! My purpose was to add a blur but since the image is already blurred it's hard to see. But now that it is solved I can play around with it and make it better. I'm just not sure about how it solved the problem.
– Sinan Samet
Nov 9 '18 at 20:11
1
1
I honestly cannot comment on why
UIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in the extent
you need. Note that CIRadialGradient
could use an actual inputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.– dfd
Nov 9 '18 at 21:19
I honestly cannot comment on why
UIImage(ciImage:)
sometimes takes liberties with things, but using the method I described works because (1) it works only with a CoreImage - CIImage, CIContext, etc. - and then (2) renders the final CIImage in the extent
you need. Note that CIRadialGradient
could use an actual inputImage
of "something" but the output is actually meant to be use as something else - in your case a mask. In my case? I want an output CIImage of it but needed an "image size", so my input image is a black CIImage with a particular user-defined extent.– dfd
Nov 9 '18 at 21:19
1
1
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think using
UIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!– dfd
Nov 9 '18 at 21:24
In your case - based on personal experience - I knew that you needed to make your CIImage a specific size (the original image). While blur filters will distort the size of the output image, a gradient filter? That was new to me. BUT, using a CIContext to size the output isn't. Remember, a CIImage isn't really an image. It's a "recipe". Some filters give you a single pixel output (yes, pixel, not point). How you choose to make this "recipe" what you want is where I think using
UIImage(ciImage:)
breaks down... BADLY. It's a shortcut that just works until it doesn't!– dfd
Nov 9 '18 at 21:24
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating a
CIContext
every time.– Frank Schlegel
Nov 16 '18 at 9:02
The size difference happens because the blur filter changes the (virtual) extend of the image. Check my answer—you can easily fix that without creating a
CIContext
every time.– Frank Schlegel
Nov 16 '18 at 9:02
add a comment |
Look up the documentation, it's a mask that being applied to the image:
Docs: CIRadialGradient
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is aRadialGradient
, not aRadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:inputRadius1
andinputColor1
do seem to be as candidates to play around with.
– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
add a comment |
Look up the documentation, it's a mask that being applied to the image:
Docs: CIRadialGradient
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is aRadialGradient
, not aRadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:inputRadius1
andinputColor1
do seem to be as candidates to play around with.
– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
add a comment |
Look up the documentation, it's a mask that being applied to the image:
Docs: CIRadialGradient
Look up the documentation, it's a mask that being applied to the image:
Docs: CIRadialGradient
answered Nov 9 '18 at 15:27


inokeyinokey
1,120919
1,120919
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is aRadialGradient
, not aRadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:inputRadius1
andinputColor1
do seem to be as candidates to play around with.
– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
add a comment |
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is aRadialGradient
, not aRadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:inputRadius1
andinputColor1
do seem to be as candidates to play around with.
– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Yes this seems to be the case indeed. But how do I solve this problem and keep the original size instead?
– Sinan Samet
Nov 9 '18 at 15:36
Also this is a
RadialGradient
, not a RadialMask
– Sinan Samet
Nov 9 '18 at 15:38
Also this is a
RadialGradient
, not a RadialMask
– Sinan Samet
Nov 9 '18 at 15:38
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:
inputRadius1
and inputColor1
do seem to be as candidates to play around with.– inokey
Nov 9 '18 at 15:40
It's is a gradient but it treats your image as mask. The same link from documentation leads us to parameters that could be customized:
inputRadius1
and inputColor1
do seem to be as candidates to play around with.– inokey
Nov 9 '18 at 15:40
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
I did play around with those but unfortunately it doesn't change anything beside the blur effect.
– Sinan Samet
Nov 9 '18 at 15:44
add a comment |
The different sizes are caused by the kernel size of the blur filter:
The blur filter needs to sample a region around each pixel. Since there are no pixels beyond the image bounds, Core Image reduces the extend
of the result image by half the kernel size (blur radius) to signal that for those pixels there is not enough information for a proper blur.
However, you can tell Core Image to treat the border pixels as extending infinitely in all directions so that the blur filter gets enough information even on the edges of the image. Afterwards you can crop the result back to the original dimension.
In your code, just change the following two lines:
maskedVariableBlur.setValue(coreImage.clampedToExtent(), forKey: kCIInputImageKey)
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage.cropped(to:coreImage.extend))
add a comment |
The different sizes are caused by the kernel size of the blur filter:
The blur filter needs to sample a region around each pixel. Since there are no pixels beyond the image bounds, Core Image reduces the extend
of the result image by half the kernel size (blur radius) to signal that for those pixels there is not enough information for a proper blur.
However, you can tell Core Image to treat the border pixels as extending infinitely in all directions so that the blur filter gets enough information even on the edges of the image. Afterwards you can crop the result back to the original dimension.
In your code, just change the following two lines:
maskedVariableBlur.setValue(coreImage.clampedToExtent(), forKey: kCIInputImageKey)
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage.cropped(to:coreImage.extend))
add a comment |
The different sizes are caused by the kernel size of the blur filter:
The blur filter needs to sample a region around each pixel. Since there are no pixels beyond the image bounds, Core Image reduces the extend
of the result image by half the kernel size (blur radius) to signal that for those pixels there is not enough information for a proper blur.
However, you can tell Core Image to treat the border pixels as extending infinitely in all directions so that the blur filter gets enough information even on the edges of the image. Afterwards you can crop the result back to the original dimension.
In your code, just change the following two lines:
maskedVariableBlur.setValue(coreImage.clampedToExtent(), forKey: kCIInputImageKey)
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage.cropped(to:coreImage.extend))
The different sizes are caused by the kernel size of the blur filter:
The blur filter needs to sample a region around each pixel. Since there are no pixels beyond the image bounds, Core Image reduces the extend
of the result image by half the kernel size (blur radius) to signal that for those pixels there is not enough information for a proper blur.
However, you can tell Core Image to treat the border pixels as extending infinitely in all directions so that the blur filter gets enough information even on the edges of the image. Afterwards you can crop the result back to the original dimension.
In your code, just change the following two lines:
maskedVariableBlur.setValue(coreImage.clampedToExtent(), forKey: kCIInputImageKey)
bgImage.image = UIImage(ciImage: selectivelyFocusedCIImage.cropped(to:coreImage.extend))
answered Nov 16 '18 at 8:45
Frank SchlegelFrank Schlegel
1,2131227
1,2131227
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228471%2fciradialgradient-reduces-image-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7 0mT4a7XLFCWRji d,Cz km
Can you post the image before and after applying the filters?
– EmilioPelaez
Nov 9 '18 at 15:26
Yes I've just editted my post.
– Sinan Samet
Nov 9 '18 at 15:34