I'm trying to pass the instance of my controller to another controller but it returns null
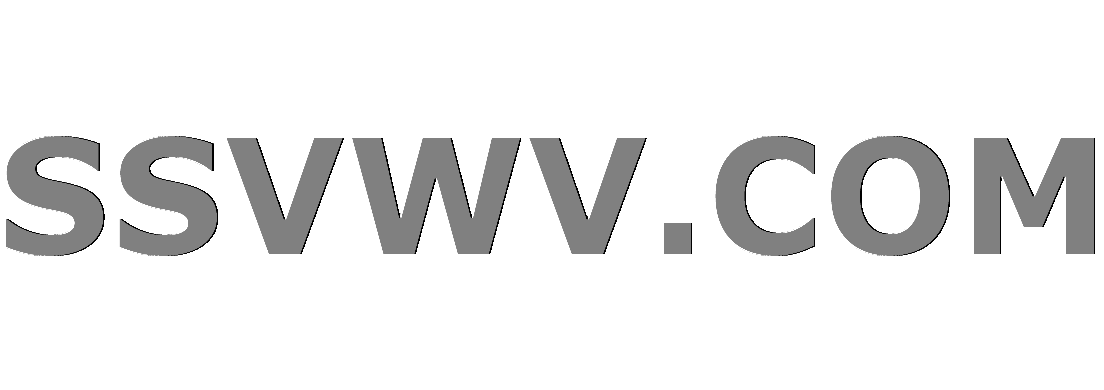
Multi tool use
I have a controller that receives data from another(login page) through loading it's fxml
fxmlLoader = new FXMLLoader(UserMainController.class.getResource("FXMLDocument.fxml"));
//User
Parent userParent = (Parent) fxmlLoader.load();
userController = fxmlLoader.<UserMainController>getController();
userController.setUser(userID, collegeID, username, password, key);
Scene userScene = new Scene(userParent);
//get stage information
Stage window = (Stage) ((Node)event.getSource()).getScene().getWindow();
window.setScene(userScene);
window.show();
this is working fine.
Inside the controller of FXMLDocument.fxml(the one i'm loading above), i'm trying to pass it's instance to other controllers
@Override
public void initialize(URL url, ResourceBundle rb) {
tab1.init(this);
tab2.init(this);
tab3.init(this);
}
and also inside the fxml of FXMLDocument.fxml, they are included like this
<BorderPane fx:id="tab1BP">
<center>
<fx:include fx:id="tab1" source="Tab1/tab1.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab2BP" prefHeight="200.0" prefWidth="200.0">
<center>
<fx:include fx:id="tab2" source="Tab2/tab2.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab3BP">
<center>
<fx:include fx:id="tab3" source="Tab3/tab3.fxml"/>
</center>
</BorderPane>
I'm getting an error at the line tab3.init(this);
Caused by: java.lang.NullPointerException
at ara_thesis.User.UserMainController.initialize(UserMainController.java:149)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2548)
... 61 more
and tab3's initialize function looks like this
@Override
public void initialize(URL url, ResourceBundle rb) {
Platform.runLater(() -> {
this.user = userMainController.getUser();
try {
initializeProgramTable();
} catch (SQLException ex) {
Logger.getLogger(CourseSectionController.class.getName()).log(Level.SEVERE, null, ex);
}
});
it returns null at the line 149 that is
this.user = userMainController.getUser(); (which is a function inside UserMainController that gets data that came from the login controller.)
and also after trying the built in debugger for netbeans, i found out that tab1.init(this) and tab3.init(this) contains they're controller's instance, as there is a value shown when I hovered above it, and null for tab2.init(this). Removing tab2.init(this) and removing it's inclusion inside the fxml file would result to no errors, so that gave me the conclusion that tab3 is the one with error. But I can't seem to find it. Enclosing tab1.init......tab3.init(this); inside a Platform.runLater would also result to null pointer exception as tab3.initialize would look for a value that would be coming from the usermaincontroller.
Am I missing something here? Thank you for the help
java javafx fxml
|
show 4 more comments
I have a controller that receives data from another(login page) through loading it's fxml
fxmlLoader = new FXMLLoader(UserMainController.class.getResource("FXMLDocument.fxml"));
//User
Parent userParent = (Parent) fxmlLoader.load();
userController = fxmlLoader.<UserMainController>getController();
userController.setUser(userID, collegeID, username, password, key);
Scene userScene = new Scene(userParent);
//get stage information
Stage window = (Stage) ((Node)event.getSource()).getScene().getWindow();
window.setScene(userScene);
window.show();
this is working fine.
Inside the controller of FXMLDocument.fxml(the one i'm loading above), i'm trying to pass it's instance to other controllers
@Override
public void initialize(URL url, ResourceBundle rb) {
tab1.init(this);
tab2.init(this);
tab3.init(this);
}
and also inside the fxml of FXMLDocument.fxml, they are included like this
<BorderPane fx:id="tab1BP">
<center>
<fx:include fx:id="tab1" source="Tab1/tab1.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab2BP" prefHeight="200.0" prefWidth="200.0">
<center>
<fx:include fx:id="tab2" source="Tab2/tab2.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab3BP">
<center>
<fx:include fx:id="tab3" source="Tab3/tab3.fxml"/>
</center>
</BorderPane>
I'm getting an error at the line tab3.init(this);
Caused by: java.lang.NullPointerException
at ara_thesis.User.UserMainController.initialize(UserMainController.java:149)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2548)
... 61 more
and tab3's initialize function looks like this
@Override
public void initialize(URL url, ResourceBundle rb) {
Platform.runLater(() -> {
this.user = userMainController.getUser();
try {
initializeProgramTable();
} catch (SQLException ex) {
Logger.getLogger(CourseSectionController.class.getName()).log(Level.SEVERE, null, ex);
}
});
it returns null at the line 149 that is
this.user = userMainController.getUser(); (which is a function inside UserMainController that gets data that came from the login controller.)
and also after trying the built in debugger for netbeans, i found out that tab1.init(this) and tab3.init(this) contains they're controller's instance, as there is a value shown when I hovered above it, and null for tab2.init(this). Removing tab2.init(this) and removing it's inclusion inside the fxml file would result to no errors, so that gave me the conclusion that tab3 is the one with error. But I can't seem to find it. Enclosing tab1.init......tab3.init(this); inside a Platform.runLater would also result to null pointer exception as tab3.initialize would look for a value that would be coming from the usermaincontroller.
Am I missing something here? Thank you for the help
java javafx fxml
I wonder whyFXMLDocument.fxml
loads at all.tab1
,tab2
andtab3
are thefx:id
s used with thefx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading sinceFXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...
– fabian
Nov 15 '18 at 10:40
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
Usually you just need to appendController
to thefx:id
of the<fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used withPlatform.runLater
to theinit
method instead?
– fabian
Nov 15 '18 at 10:53
Can you show the parts where you setuserMainController
in the tabs? Why do you say the error is attab3.init(this)
but the stack trace points toinitialize
method? Can you also show the code ofinit
?
– Gnas
Nov 15 '18 at 11:52
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03
|
show 4 more comments
I have a controller that receives data from another(login page) through loading it's fxml
fxmlLoader = new FXMLLoader(UserMainController.class.getResource("FXMLDocument.fxml"));
//User
Parent userParent = (Parent) fxmlLoader.load();
userController = fxmlLoader.<UserMainController>getController();
userController.setUser(userID, collegeID, username, password, key);
Scene userScene = new Scene(userParent);
//get stage information
Stage window = (Stage) ((Node)event.getSource()).getScene().getWindow();
window.setScene(userScene);
window.show();
this is working fine.
Inside the controller of FXMLDocument.fxml(the one i'm loading above), i'm trying to pass it's instance to other controllers
@Override
public void initialize(URL url, ResourceBundle rb) {
tab1.init(this);
tab2.init(this);
tab3.init(this);
}
and also inside the fxml of FXMLDocument.fxml, they are included like this
<BorderPane fx:id="tab1BP">
<center>
<fx:include fx:id="tab1" source="Tab1/tab1.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab2BP" prefHeight="200.0" prefWidth="200.0">
<center>
<fx:include fx:id="tab2" source="Tab2/tab2.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab3BP">
<center>
<fx:include fx:id="tab3" source="Tab3/tab3.fxml"/>
</center>
</BorderPane>
I'm getting an error at the line tab3.init(this);
Caused by: java.lang.NullPointerException
at ara_thesis.User.UserMainController.initialize(UserMainController.java:149)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2548)
... 61 more
and tab3's initialize function looks like this
@Override
public void initialize(URL url, ResourceBundle rb) {
Platform.runLater(() -> {
this.user = userMainController.getUser();
try {
initializeProgramTable();
} catch (SQLException ex) {
Logger.getLogger(CourseSectionController.class.getName()).log(Level.SEVERE, null, ex);
}
});
it returns null at the line 149 that is
this.user = userMainController.getUser(); (which is a function inside UserMainController that gets data that came from the login controller.)
and also after trying the built in debugger for netbeans, i found out that tab1.init(this) and tab3.init(this) contains they're controller's instance, as there is a value shown when I hovered above it, and null for tab2.init(this). Removing tab2.init(this) and removing it's inclusion inside the fxml file would result to no errors, so that gave me the conclusion that tab3 is the one with error. But I can't seem to find it. Enclosing tab1.init......tab3.init(this); inside a Platform.runLater would also result to null pointer exception as tab3.initialize would look for a value that would be coming from the usermaincontroller.
Am I missing something here? Thank you for the help
java javafx fxml
I have a controller that receives data from another(login page) through loading it's fxml
fxmlLoader = new FXMLLoader(UserMainController.class.getResource("FXMLDocument.fxml"));
//User
Parent userParent = (Parent) fxmlLoader.load();
userController = fxmlLoader.<UserMainController>getController();
userController.setUser(userID, collegeID, username, password, key);
Scene userScene = new Scene(userParent);
//get stage information
Stage window = (Stage) ((Node)event.getSource()).getScene().getWindow();
window.setScene(userScene);
window.show();
this is working fine.
Inside the controller of FXMLDocument.fxml(the one i'm loading above), i'm trying to pass it's instance to other controllers
@Override
public void initialize(URL url, ResourceBundle rb) {
tab1.init(this);
tab2.init(this);
tab3.init(this);
}
and also inside the fxml of FXMLDocument.fxml, they are included like this
<BorderPane fx:id="tab1BP">
<center>
<fx:include fx:id="tab1" source="Tab1/tab1.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab2BP" prefHeight="200.0" prefWidth="200.0">
<center>
<fx:include fx:id="tab2" source="Tab2/tab2.fxml"/>
</center></BorderPane>
<BorderPane fx:id="tab3BP">
<center>
<fx:include fx:id="tab3" source="Tab3/tab3.fxml"/>
</center>
</BorderPane>
I'm getting an error at the line tab3.init(this);
Caused by: java.lang.NullPointerException
at ara_thesis.User.UserMainController.initialize(UserMainController.java:149)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2548)
... 61 more
and tab3's initialize function looks like this
@Override
public void initialize(URL url, ResourceBundle rb) {
Platform.runLater(() -> {
this.user = userMainController.getUser();
try {
initializeProgramTable();
} catch (SQLException ex) {
Logger.getLogger(CourseSectionController.class.getName()).log(Level.SEVERE, null, ex);
}
});
it returns null at the line 149 that is
this.user = userMainController.getUser(); (which is a function inside UserMainController that gets data that came from the login controller.)
and also after trying the built in debugger for netbeans, i found out that tab1.init(this) and tab3.init(this) contains they're controller's instance, as there is a value shown when I hovered above it, and null for tab2.init(this). Removing tab2.init(this) and removing it's inclusion inside the fxml file would result to no errors, so that gave me the conclusion that tab3 is the one with error. But I can't seem to find it. Enclosing tab1.init......tab3.init(this); inside a Platform.runLater would also result to null pointer exception as tab3.initialize would look for a value that would be coming from the usermaincontroller.
Am I missing something here? Thank you for the help
java javafx fxml
java javafx fxml
edited Nov 15 '18 at 10:29
Fatnam
asked Nov 15 '18 at 10:24
FatnamFatnam
165
165
I wonder whyFXMLDocument.fxml
loads at all.tab1
,tab2
andtab3
are thefx:id
s used with thefx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading sinceFXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...
– fabian
Nov 15 '18 at 10:40
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
Usually you just need to appendController
to thefx:id
of the<fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used withPlatform.runLater
to theinit
method instead?
– fabian
Nov 15 '18 at 10:53
Can you show the parts where you setuserMainController
in the tabs? Why do you say the error is attab3.init(this)
but the stack trace points toinitialize
method? Can you also show the code ofinit
?
– Gnas
Nov 15 '18 at 11:52
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03
|
show 4 more comments
I wonder whyFXMLDocument.fxml
loads at all.tab1
,tab2
andtab3
are thefx:id
s used with thefx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading sinceFXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...
– fabian
Nov 15 '18 at 10:40
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
Usually you just need to appendController
to thefx:id
of the<fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used withPlatform.runLater
to theinit
method instead?
– fabian
Nov 15 '18 at 10:53
Can you show the parts where you setuserMainController
in the tabs? Why do you say the error is attab3.init(this)
but the stack trace points toinitialize
method? Can you also show the code ofinit
?
– Gnas
Nov 15 '18 at 11:52
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03
I wonder why
FXMLDocument.fxml
loads at all. tab1
, tab2
and tab3
are the fx:id
s used with the fx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading since FXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...– fabian
Nov 15 '18 at 10:40
I wonder why
FXMLDocument.fxml
loads at all. tab1
, tab2
and tab3
are the fx:id
s used with the fx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading since FXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...– fabian
Nov 15 '18 at 10:40
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
Usually you just need to append
Controller
to the fx:id
of the <fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used with Platform.runLater
to the init
method instead?– fabian
Nov 15 '18 at 10:53
Usually you just need to append
Controller
to the fx:id
of the <fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used with Platform.runLater
to the init
method instead?– fabian
Nov 15 '18 at 10:53
Can you show the parts where you set
userMainController
in the tabs? Why do you say the error is at tab3.init(this)
but the stack trace points to initialize
method? Can you also show the code of init
?– Gnas
Nov 15 '18 at 11:52
Can you show the parts where you set
userMainController
in the tabs? Why do you say the error is at tab3.init(this)
but the stack trace points to initialize
method? Can you also show the code of init
?– Gnas
Nov 15 '18 at 11:52
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03
|
show 4 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317265%2fim-trying-to-pass-the-instance-of-my-controller-to-another-controller-but-it-re%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317265%2fim-trying-to-pass-the-instance-of-my-controller-to-another-controller-but-it-re%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zwa,qMgyB,XkYTamoB RWhUQ96HR,3AqElMFCC,djzyXanwsplQ,MespNZD1ipFMj i 2EqpedRrUavGmF,GDT,4KVilbGR2Z,JofXEjed
I wonder why
FXMLDocument.fxml
loads at all.tab1
,tab2
andtab3
are thefx:id
s used with thefx:include
elements which means the object created for the root element of the included element is injected to this field, not the controller of the fxml. This should result in an error when loading sinceFXMLLoader
should be unable to assign the value for the root element to a field with a type the root's type is not assignable to...– fabian
Nov 15 '18 at 10:40
What should I do? The reason why I'm loading the FXMLDocument.fxml is to pass login information to another controller and change the scene to UserMainController(FXMLDocument.fxml is the view of UserMainController). Then UserMainController contains tabs in it that has different FXMLs and Controllers. I tried to pass the instance of usermaincontroller to all the tabs for them to communicate. Also I want to get a specific id from the login user that will be used for query in tab 3
– Fatnam
Nov 15 '18 at 10:48
Usually you just need to append
Controller
to thefx:id
of the<fx:include>
to get the name of the field you need to use for the controller of the included fxml. However i don't know why your code does not fail in the first place... Furthermore why not move the code you used withPlatform.runLater
to theinit
method instead?– fabian
Nov 15 '18 at 10:53
Can you show the parts where you set
userMainController
in the tabs? Why do you say the error is attab3.init(this)
but the stack trace points toinitialize
method? Can you also show the code ofinit
?– Gnas
Nov 15 '18 at 11:52
@Gnas yes it says that tab3.init(this) was null. And another error below that, points to the initialize method of tab3 which is the one above. Here is the code for init: 'public void init(UserMainController userMainController) { this.userMainController = userMainController; }'
– Fatnam
Nov 15 '18 at 12:03