How to enlarge dynamically an UILabel (label and font size)?
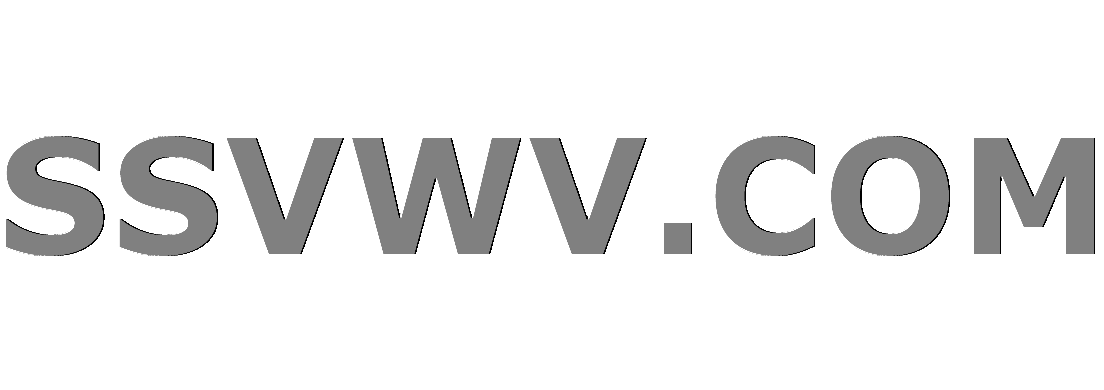
Multi tool use
Im currently working on an iPhone project. I want to enlarge dynamically an UILabel in Objective-C like this:
alt text http://img268.imageshack.us/img268/9683/bildschirmfoto20100323u.png
How is this possible? I thought I have to do it with CoreAnimation, but I didn't worked. Here is the code I tried:
UILabel * fooL = //[…]
fooL.frame = CGRectMake(fooL.frame.origin.x, fooL.frame.origin.y, fooL.frame.size.width, fooL.frame.size.height);
fooL.font = [UIFont fontWithName:@"Helvetica" size:80];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationCurve:UIViewAnimationCurveEaseIn];
[UIView setAnimationDelegate:self];
[UIView setAnimationDuration:0.5];
[UIView setAnimationBeginsFromCurrentState:YES];
fooL.font = [UIFont fontWithName:@"Helvetica" size:144]; //bigger size
fooL.frame = CGRectMake(20 , 44, 728, 167); //bigger frame
[UIView commitAnimations];
The problem with this code is that it doesn't change the fontsize dynamically.
objective-c core-animation uilabel
add a comment |
Im currently working on an iPhone project. I want to enlarge dynamically an UILabel in Objective-C like this:
alt text http://img268.imageshack.us/img268/9683/bildschirmfoto20100323u.png
How is this possible? I thought I have to do it with CoreAnimation, but I didn't worked. Here is the code I tried:
UILabel * fooL = //[…]
fooL.frame = CGRectMake(fooL.frame.origin.x, fooL.frame.origin.y, fooL.frame.size.width, fooL.frame.size.height);
fooL.font = [UIFont fontWithName:@"Helvetica" size:80];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationCurve:UIViewAnimationCurveEaseIn];
[UIView setAnimationDelegate:self];
[UIView setAnimationDuration:0.5];
[UIView setAnimationBeginsFromCurrentState:YES];
fooL.font = [UIFont fontWithName:@"Helvetica" size:144]; //bigger size
fooL.frame = CGRectMake(20 , 44, 728, 167); //bigger frame
[UIView commitAnimations];
The problem with this code is that it doesn't change the fontsize dynamically.
objective-c core-animation uilabel
add a comment |
Im currently working on an iPhone project. I want to enlarge dynamically an UILabel in Objective-C like this:
alt text http://img268.imageshack.us/img268/9683/bildschirmfoto20100323u.png
How is this possible? I thought I have to do it with CoreAnimation, but I didn't worked. Here is the code I tried:
UILabel * fooL = //[…]
fooL.frame = CGRectMake(fooL.frame.origin.x, fooL.frame.origin.y, fooL.frame.size.width, fooL.frame.size.height);
fooL.font = [UIFont fontWithName:@"Helvetica" size:80];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationCurve:UIViewAnimationCurveEaseIn];
[UIView setAnimationDelegate:self];
[UIView setAnimationDuration:0.5];
[UIView setAnimationBeginsFromCurrentState:YES];
fooL.font = [UIFont fontWithName:@"Helvetica" size:144]; //bigger size
fooL.frame = CGRectMake(20 , 44, 728, 167); //bigger frame
[UIView commitAnimations];
The problem with this code is that it doesn't change the fontsize dynamically.
objective-c core-animation uilabel
Im currently working on an iPhone project. I want to enlarge dynamically an UILabel in Objective-C like this:
alt text http://img268.imageshack.us/img268/9683/bildschirmfoto20100323u.png
How is this possible? I thought I have to do it with CoreAnimation, but I didn't worked. Here is the code I tried:
UILabel * fooL = //[…]
fooL.frame = CGRectMake(fooL.frame.origin.x, fooL.frame.origin.y, fooL.frame.size.width, fooL.frame.size.height);
fooL.font = [UIFont fontWithName:@"Helvetica" size:80];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationCurve:UIViewAnimationCurveEaseIn];
[UIView setAnimationDelegate:self];
[UIView setAnimationDuration:0.5];
[UIView setAnimationBeginsFromCurrentState:YES];
fooL.font = [UIFont fontWithName:@"Helvetica" size:144]; //bigger size
fooL.frame = CGRectMake(20 , 44, 728, 167); //bigger frame
[UIView commitAnimations];
The problem with this code is that it doesn't change the fontsize dynamically.
objective-c core-animation uilabel
objective-c core-animation uilabel
edited Dec 16 '11 at 21:00
WrightsCS
45.9k21125171
45.9k21125171
asked Mar 23 '10 at 1:40


FlockedFlocked
1,10823254
1,10823254
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
All you need to do is apply an affine transform to the UILabel
object.
CGFloat scaleFactor = 2.0f;
label.transform = CGAffineTransformMakeScale(scaleFactor, scaleFactor); // Enlarge by a factor of 2.
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
add a comment |
Scaling your label as suggested by others using the transform
property will work great. One thing to keep in mind is that as the label gets larger, the font is not increasing, but just the rendered text, which means it will appear "fuzzier" as it gets larger.
add a comment |
Just scale your Label instead of changing the fontSize.
add a comment |
Try this method:
+ (void)setAnimationTransition:(UIViewAnimationTransition)transition
forView:(UIView *)view
cache:(BOOL)cache
Parameters:
transition
A transition to apply to view. Possible values are described in UIViewAnimationTransition.
view
The view to apply the transition to.
cache
If YES, the before and after images of view are rendered once and used to create the frames in the animation. Caching can improve performance but if you set this parameter to YES, you must not update the view or its subviews during the transition. Updating the view and its subviews may interfere with the caching behaviors and cause the view contents to be rendered incorrectly (or in the wrong location) during the animation. You must wait until the transition ends to update the view.
If NO, the view and its contents must be updated for each frame of the transition animation, which may noticeably affect the frame rate.
Discussion
If you want to change the appearance of a view during a transition—for example, flip from one view to another—then use a container view, an instance of UIView, as follows:
Begin an animation block.
Set the transition on the container view.
Remove the subview from the container view.
Add the new subview to the container view.
Commit the animation block.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2497007%2fhow-to-enlarge-dynamically-an-uilabel-label-and-font-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
All you need to do is apply an affine transform to the UILabel
object.
CGFloat scaleFactor = 2.0f;
label.transform = CGAffineTransformMakeScale(scaleFactor, scaleFactor); // Enlarge by a factor of 2.
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
add a comment |
All you need to do is apply an affine transform to the UILabel
object.
CGFloat scaleFactor = 2.0f;
label.transform = CGAffineTransformMakeScale(scaleFactor, scaleFactor); // Enlarge by a factor of 2.
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
add a comment |
All you need to do is apply an affine transform to the UILabel
object.
CGFloat scaleFactor = 2.0f;
label.transform = CGAffineTransformMakeScale(scaleFactor, scaleFactor); // Enlarge by a factor of 2.
All you need to do is apply an affine transform to the UILabel
object.
CGFloat scaleFactor = 2.0f;
label.transform = CGAffineTransformMakeScale(scaleFactor, scaleFactor); // Enlarge by a factor of 2.
answered Dec 16 '11 at 21:34
Mark AdamsMark Adams
27k107077
27k107077
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
add a comment |
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
I would rather prefer a MakeScale transformation that manually changing the frame and font size.
– Mugunth
Feb 19 '12 at 7:47
add a comment |
Scaling your label as suggested by others using the transform
property will work great. One thing to keep in mind is that as the label gets larger, the font is not increasing, but just the rendered text, which means it will appear "fuzzier" as it gets larger.
add a comment |
Scaling your label as suggested by others using the transform
property will work great. One thing to keep in mind is that as the label gets larger, the font is not increasing, but just the rendered text, which means it will appear "fuzzier" as it gets larger.
add a comment |
Scaling your label as suggested by others using the transform
property will work great. One thing to keep in mind is that as the label gets larger, the font is not increasing, but just the rendered text, which means it will appear "fuzzier" as it gets larger.
Scaling your label as suggested by others using the transform
property will work great. One thing to keep in mind is that as the label gets larger, the font is not increasing, but just the rendered text, which means it will appear "fuzzier" as it gets larger.
answered Dec 16 '11 at 22:00
gschandlergschandler
3,1311116
3,1311116
add a comment |
add a comment |
Just scale your Label instead of changing the fontSize.
add a comment |
Just scale your Label instead of changing the fontSize.
add a comment |
Just scale your Label instead of changing the fontSize.
Just scale your Label instead of changing the fontSize.
answered Dec 15 '11 at 19:21
ChakalakaChakalaka
2,5301324
2,5301324
add a comment |
add a comment |
Try this method:
+ (void)setAnimationTransition:(UIViewAnimationTransition)transition
forView:(UIView *)view
cache:(BOOL)cache
Parameters:
transition
A transition to apply to view. Possible values are described in UIViewAnimationTransition.
view
The view to apply the transition to.
cache
If YES, the before and after images of view are rendered once and used to create the frames in the animation. Caching can improve performance but if you set this parameter to YES, you must not update the view or its subviews during the transition. Updating the view and its subviews may interfere with the caching behaviors and cause the view contents to be rendered incorrectly (or in the wrong location) during the animation. You must wait until the transition ends to update the view.
If NO, the view and its contents must be updated for each frame of the transition animation, which may noticeably affect the frame rate.
Discussion
If you want to change the appearance of a view during a transition—for example, flip from one view to another—then use a container view, an instance of UIView, as follows:
Begin an animation block.
Set the transition on the container view.
Remove the subview from the container view.
Add the new subview to the container view.
Commit the animation block.
add a comment |
Try this method:
+ (void)setAnimationTransition:(UIViewAnimationTransition)transition
forView:(UIView *)view
cache:(BOOL)cache
Parameters:
transition
A transition to apply to view. Possible values are described in UIViewAnimationTransition.
view
The view to apply the transition to.
cache
If YES, the before and after images of view are rendered once and used to create the frames in the animation. Caching can improve performance but if you set this parameter to YES, you must not update the view or its subviews during the transition. Updating the view and its subviews may interfere with the caching behaviors and cause the view contents to be rendered incorrectly (or in the wrong location) during the animation. You must wait until the transition ends to update the view.
If NO, the view and its contents must be updated for each frame of the transition animation, which may noticeably affect the frame rate.
Discussion
If you want to change the appearance of a view during a transition—for example, flip from one view to another—then use a container view, an instance of UIView, as follows:
Begin an animation block.
Set the transition on the container view.
Remove the subview from the container view.
Add the new subview to the container view.
Commit the animation block.
add a comment |
Try this method:
+ (void)setAnimationTransition:(UIViewAnimationTransition)transition
forView:(UIView *)view
cache:(BOOL)cache
Parameters:
transition
A transition to apply to view. Possible values are described in UIViewAnimationTransition.
view
The view to apply the transition to.
cache
If YES, the before and after images of view are rendered once and used to create the frames in the animation. Caching can improve performance but if you set this parameter to YES, you must not update the view or its subviews during the transition. Updating the view and its subviews may interfere with the caching behaviors and cause the view contents to be rendered incorrectly (or in the wrong location) during the animation. You must wait until the transition ends to update the view.
If NO, the view and its contents must be updated for each frame of the transition animation, which may noticeably affect the frame rate.
Discussion
If you want to change the appearance of a view during a transition—for example, flip from one view to another—then use a container view, an instance of UIView, as follows:
Begin an animation block.
Set the transition on the container view.
Remove the subview from the container view.
Add the new subview to the container view.
Commit the animation block.
Try this method:
+ (void)setAnimationTransition:(UIViewAnimationTransition)transition
forView:(UIView *)view
cache:(BOOL)cache
Parameters:
transition
A transition to apply to view. Possible values are described in UIViewAnimationTransition.
view
The view to apply the transition to.
cache
If YES, the before and after images of view are rendered once and used to create the frames in the animation. Caching can improve performance but if you set this parameter to YES, you must not update the view or its subviews during the transition. Updating the view and its subviews may interfere with the caching behaviors and cause the view contents to be rendered incorrectly (or in the wrong location) during the animation. You must wait until the transition ends to update the view.
If NO, the view and its contents must be updated for each frame of the transition animation, which may noticeably affect the frame rate.
Discussion
If you want to change the appearance of a view during a transition—for example, flip from one view to another—then use a container view, an instance of UIView, as follows:
Begin an animation block.
Set the transition on the container view.
Remove the subview from the container view.
Add the new subview to the container view.
Commit the animation block.
edited Nov 15 '18 at 16:14
elp
5,938753106
5,938753106
answered Mar 24 '10 at 18:39
jekmacjekmac
212
212
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2497007%2fhow-to-enlarge-dynamically-an-uilabel-label-and-font-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iD0juVjcx3r3ubxPPl0L,uWZ,b4h7SU276muIwnKGWNMvAQkf2Yl6jbUWdJZMabYGMgT C,Jv FM1