Database still in use after a selenium test in Django
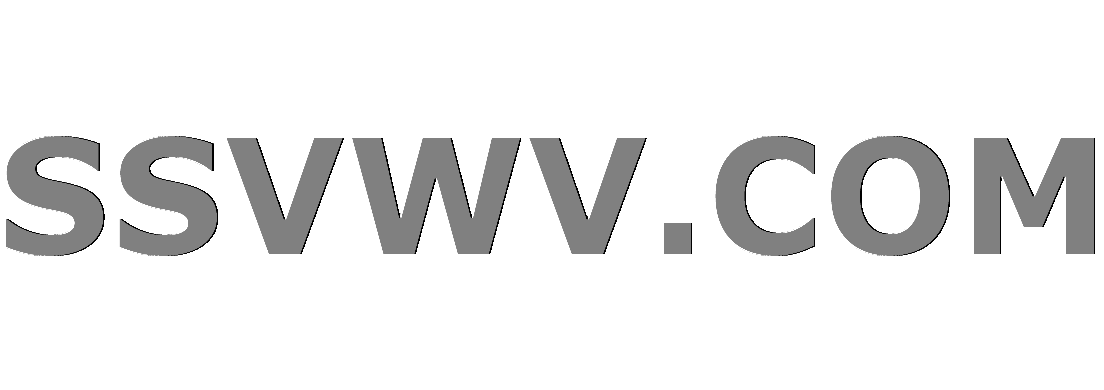
Multi tool use
I have a Django project in which I'm starting to write Selenium tests. The first one looking like this:
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from core.models import User
from example import settings
BACH_EMAIL = "johann.sebastian.bach@classics.com"
PASSWORD = "password"
class TestImportCRMData(StaticLiveServerTestCase):
@classmethod
def setUpClass(cls):
super().setUpClass()
cls.webdriver = webdriver.Chrome()
cls.webdriver.implicitly_wait(10)
@classmethod
def tearDownClass(cls):
cls.webdriver.close()
cls.webdriver.quit()
super().tearDownClass()
def setUp(self):
self.admin = User.objects.create_superuser(email=BACH_EMAIL, password=PASSWORD)
def test_admin_tool(self):
self.webdriver.get(f"http://{settings.ADMIN_HOST}:{self.server_thread.port}/admin")
self.webdriver.find_element_by_id("id_username").send_keys(BACH_EMAIL)
self.webdriver.find_element_by_id("id_password").send_keys(PASSWORD)
self.webdriver.find_element_by_id("id_password").send_keys(Keys.RETURN)
self.webdriver.find_element_by_link_text("Users").click()
When I run it, the test pass but still ends with this error:
Traceback (most recent call last):
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
psycopg2.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 168, in <module>
utility.execute()
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 142, in execute
_create_command().run_from_argv(self.argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementcommandstest.py", line 26, in run_from_argv
super().run_from_argv(argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 316, in run_from_argv
self.execute(*args, **cmd_options)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 353, in execute
output = self.handle(*args, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 104, in handle
failures = TestRunner(test_labels, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 255, in run_tests
extra_tests=extra_tests, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 156, in run_tests
return super(DjangoTeamcityTestRunner, self).run_tests(test_labels, extra_tests, **kwargs)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 607, in run_tests
self.teardown_databases(old_config)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 580, in teardown_databases
keepdb=self.keepdb,
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestutils.py", line 297, in teardown_databases
connection.creation.destroy_test_db(old_name, verbosity, keepdb)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 257, in destroy_test_db
self._destroy_test_db(test_database_name, verbosity)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 274, in _destroy_test_db
% self.connection.ops.quote_name(test_database_name))
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 68, in execute
return self._execute_with_wrappers(sql, params, many=False, executor=self._execute)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 77, in _execute_with_wrappers
return executor(sql, params, many, context)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbutils.py", line 89, in __exit__
raise dj_exc_value.with_traceback(traceback) from exc_value
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
django.db.utils.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The problem of course is that the next run of the tests, the database still exists, so, the tests don't run without confirming deletion of the database.
If I comment out the last line:
self.webdriver.find_element_by_link_text("Users").click()
then I don't get this error. I guess just because the database connection is not established. Sometimes it's 1 other session, sometimes it's up to 4. In one of the cases of 4 sessions, these were the running sessions:
select * from pg_stat_activity where datname = 'test_example';
100123 test_example 29892 16393 pupeno "" ::1 61967 2018-11-15 17:28:19.552431 2018-11-15 17:28:19.562398 2018-11-15 17:28:19.564623 idle SELECT "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined" FROM "core_user" WHERE "core_user"."id" = 1
100123 test_example 33028 16393 pupeno "" ::1 61930 2018-11-15 17:28:18.792466 2018-11-15 17:28:18.843383 2018-11-15 17:28:18.851828 idle SELECT "django_admin_log"."id", "django_admin_log"."action_time", "django_admin_log"."user_id", "django_admin_log"."content_type_id", "django_admin_log"."object_id", "django_admin_log"."object_repr", "django_admin_log"."action_flag", "django_admin_log"."change_message", "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined", "django_content_type"."id", "django_content_type"."app_label", "django_content_type"."model" FROM "django_admin_log" INNER JOIN "core_user" ON ("django_admin_log"."user_id" = "core_user"."id") LEFT OUTER JOIN "django_content_type" ON ("django_admin_log"."content_type_id" = "django_content_type"."id") WHERE "django_admin_log"."user_id" = 1 ORDER BY "django_admin_log"."action_time" DESC LIMIT 10
100123 test_example 14128 16393 pupeno "" ::1 61988 2018-11-15 17:28:19.767225 2018-11-15 17:28:19.776150 2018-11-15 17:28:19.776479 idle SELECT "core_firm"."id", "core_firm"."name", "core_firm"."host_name" FROM "core_firm" WHERE "core_firm"."id" = 1
100123 test_example 9604 16393 pupeno "" ::1 61960 2018-11-15 17:28:19.469197 2018-11-15 17:28:19.478775 2018-11-15 17:28:19.478788 idle COMMIT
I've been trying to find the minimum reproducible example of this problem, but so far I haven't succeeded.
Any ideas what could be causing this or how to find out more about what the issue could be?
python django selenium testing
|
show 1 more comment
I have a Django project in which I'm starting to write Selenium tests. The first one looking like this:
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from core.models import User
from example import settings
BACH_EMAIL = "johann.sebastian.bach@classics.com"
PASSWORD = "password"
class TestImportCRMData(StaticLiveServerTestCase):
@classmethod
def setUpClass(cls):
super().setUpClass()
cls.webdriver = webdriver.Chrome()
cls.webdriver.implicitly_wait(10)
@classmethod
def tearDownClass(cls):
cls.webdriver.close()
cls.webdriver.quit()
super().tearDownClass()
def setUp(self):
self.admin = User.objects.create_superuser(email=BACH_EMAIL, password=PASSWORD)
def test_admin_tool(self):
self.webdriver.get(f"http://{settings.ADMIN_HOST}:{self.server_thread.port}/admin")
self.webdriver.find_element_by_id("id_username").send_keys(BACH_EMAIL)
self.webdriver.find_element_by_id("id_password").send_keys(PASSWORD)
self.webdriver.find_element_by_id("id_password").send_keys(Keys.RETURN)
self.webdriver.find_element_by_link_text("Users").click()
When I run it, the test pass but still ends with this error:
Traceback (most recent call last):
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
psycopg2.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 168, in <module>
utility.execute()
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 142, in execute
_create_command().run_from_argv(self.argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementcommandstest.py", line 26, in run_from_argv
super().run_from_argv(argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 316, in run_from_argv
self.execute(*args, **cmd_options)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 353, in execute
output = self.handle(*args, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 104, in handle
failures = TestRunner(test_labels, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 255, in run_tests
extra_tests=extra_tests, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 156, in run_tests
return super(DjangoTeamcityTestRunner, self).run_tests(test_labels, extra_tests, **kwargs)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 607, in run_tests
self.teardown_databases(old_config)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 580, in teardown_databases
keepdb=self.keepdb,
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestutils.py", line 297, in teardown_databases
connection.creation.destroy_test_db(old_name, verbosity, keepdb)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 257, in destroy_test_db
self._destroy_test_db(test_database_name, verbosity)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 274, in _destroy_test_db
% self.connection.ops.quote_name(test_database_name))
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 68, in execute
return self._execute_with_wrappers(sql, params, many=False, executor=self._execute)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 77, in _execute_with_wrappers
return executor(sql, params, many, context)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbutils.py", line 89, in __exit__
raise dj_exc_value.with_traceback(traceback) from exc_value
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
django.db.utils.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The problem of course is that the next run of the tests, the database still exists, so, the tests don't run without confirming deletion of the database.
If I comment out the last line:
self.webdriver.find_element_by_link_text("Users").click()
then I don't get this error. I guess just because the database connection is not established. Sometimes it's 1 other session, sometimes it's up to 4. In one of the cases of 4 sessions, these were the running sessions:
select * from pg_stat_activity where datname = 'test_example';
100123 test_example 29892 16393 pupeno "" ::1 61967 2018-11-15 17:28:19.552431 2018-11-15 17:28:19.562398 2018-11-15 17:28:19.564623 idle SELECT "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined" FROM "core_user" WHERE "core_user"."id" = 1
100123 test_example 33028 16393 pupeno "" ::1 61930 2018-11-15 17:28:18.792466 2018-11-15 17:28:18.843383 2018-11-15 17:28:18.851828 idle SELECT "django_admin_log"."id", "django_admin_log"."action_time", "django_admin_log"."user_id", "django_admin_log"."content_type_id", "django_admin_log"."object_id", "django_admin_log"."object_repr", "django_admin_log"."action_flag", "django_admin_log"."change_message", "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined", "django_content_type"."id", "django_content_type"."app_label", "django_content_type"."model" FROM "django_admin_log" INNER JOIN "core_user" ON ("django_admin_log"."user_id" = "core_user"."id") LEFT OUTER JOIN "django_content_type" ON ("django_admin_log"."content_type_id" = "django_content_type"."id") WHERE "django_admin_log"."user_id" = 1 ORDER BY "django_admin_log"."action_time" DESC LIMIT 10
100123 test_example 14128 16393 pupeno "" ::1 61988 2018-11-15 17:28:19.767225 2018-11-15 17:28:19.776150 2018-11-15 17:28:19.776479 idle SELECT "core_firm"."id", "core_firm"."name", "core_firm"."host_name" FROM "core_firm" WHERE "core_firm"."id" = 1
100123 test_example 9604 16393 pupeno "" ::1 61960 2018-11-15 17:28:19.469197 2018-11-15 17:28:19.478775 2018-11-15 17:28:19.478788 idle COMMIT
I've been trying to find the minimum reproducible example of this problem, but so far I haven't succeeded.
Any ideas what could be causing this or how to find out more about what the issue could be?
python django selenium testing
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
Please runselect * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.
– Shlomi Bazel
Nov 15 '18 at 17:24
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
Maybe you are limited? checkSELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first
– Shlomi Bazel
Nov 15 '18 at 17:53
|
show 1 more comment
I have a Django project in which I'm starting to write Selenium tests. The first one looking like this:
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from core.models import User
from example import settings
BACH_EMAIL = "johann.sebastian.bach@classics.com"
PASSWORD = "password"
class TestImportCRMData(StaticLiveServerTestCase):
@classmethod
def setUpClass(cls):
super().setUpClass()
cls.webdriver = webdriver.Chrome()
cls.webdriver.implicitly_wait(10)
@classmethod
def tearDownClass(cls):
cls.webdriver.close()
cls.webdriver.quit()
super().tearDownClass()
def setUp(self):
self.admin = User.objects.create_superuser(email=BACH_EMAIL, password=PASSWORD)
def test_admin_tool(self):
self.webdriver.get(f"http://{settings.ADMIN_HOST}:{self.server_thread.port}/admin")
self.webdriver.find_element_by_id("id_username").send_keys(BACH_EMAIL)
self.webdriver.find_element_by_id("id_password").send_keys(PASSWORD)
self.webdriver.find_element_by_id("id_password").send_keys(Keys.RETURN)
self.webdriver.find_element_by_link_text("Users").click()
When I run it, the test pass but still ends with this error:
Traceback (most recent call last):
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
psycopg2.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 168, in <module>
utility.execute()
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 142, in execute
_create_command().run_from_argv(self.argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementcommandstest.py", line 26, in run_from_argv
super().run_from_argv(argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 316, in run_from_argv
self.execute(*args, **cmd_options)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 353, in execute
output = self.handle(*args, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 104, in handle
failures = TestRunner(test_labels, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 255, in run_tests
extra_tests=extra_tests, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 156, in run_tests
return super(DjangoTeamcityTestRunner, self).run_tests(test_labels, extra_tests, **kwargs)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 607, in run_tests
self.teardown_databases(old_config)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 580, in teardown_databases
keepdb=self.keepdb,
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestutils.py", line 297, in teardown_databases
connection.creation.destroy_test_db(old_name, verbosity, keepdb)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 257, in destroy_test_db
self._destroy_test_db(test_database_name, verbosity)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 274, in _destroy_test_db
% self.connection.ops.quote_name(test_database_name))
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 68, in execute
return self._execute_with_wrappers(sql, params, many=False, executor=self._execute)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 77, in _execute_with_wrappers
return executor(sql, params, many, context)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbutils.py", line 89, in __exit__
raise dj_exc_value.with_traceback(traceback) from exc_value
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
django.db.utils.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The problem of course is that the next run of the tests, the database still exists, so, the tests don't run without confirming deletion of the database.
If I comment out the last line:
self.webdriver.find_element_by_link_text("Users").click()
then I don't get this error. I guess just because the database connection is not established. Sometimes it's 1 other session, sometimes it's up to 4. In one of the cases of 4 sessions, these were the running sessions:
select * from pg_stat_activity where datname = 'test_example';
100123 test_example 29892 16393 pupeno "" ::1 61967 2018-11-15 17:28:19.552431 2018-11-15 17:28:19.562398 2018-11-15 17:28:19.564623 idle SELECT "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined" FROM "core_user" WHERE "core_user"."id" = 1
100123 test_example 33028 16393 pupeno "" ::1 61930 2018-11-15 17:28:18.792466 2018-11-15 17:28:18.843383 2018-11-15 17:28:18.851828 idle SELECT "django_admin_log"."id", "django_admin_log"."action_time", "django_admin_log"."user_id", "django_admin_log"."content_type_id", "django_admin_log"."object_id", "django_admin_log"."object_repr", "django_admin_log"."action_flag", "django_admin_log"."change_message", "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined", "django_content_type"."id", "django_content_type"."app_label", "django_content_type"."model" FROM "django_admin_log" INNER JOIN "core_user" ON ("django_admin_log"."user_id" = "core_user"."id") LEFT OUTER JOIN "django_content_type" ON ("django_admin_log"."content_type_id" = "django_content_type"."id") WHERE "django_admin_log"."user_id" = 1 ORDER BY "django_admin_log"."action_time" DESC LIMIT 10
100123 test_example 14128 16393 pupeno "" ::1 61988 2018-11-15 17:28:19.767225 2018-11-15 17:28:19.776150 2018-11-15 17:28:19.776479 idle SELECT "core_firm"."id", "core_firm"."name", "core_firm"."host_name" FROM "core_firm" WHERE "core_firm"."id" = 1
100123 test_example 9604 16393 pupeno "" ::1 61960 2018-11-15 17:28:19.469197 2018-11-15 17:28:19.478775 2018-11-15 17:28:19.478788 idle COMMIT
I've been trying to find the minimum reproducible example of this problem, but so far I haven't succeeded.
Any ideas what could be causing this or how to find out more about what the issue could be?
python django selenium testing
I have a Django project in which I'm starting to write Selenium tests. The first one looking like this:
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from core.models import User
from example import settings
BACH_EMAIL = "johann.sebastian.bach@classics.com"
PASSWORD = "password"
class TestImportCRMData(StaticLiveServerTestCase):
@classmethod
def setUpClass(cls):
super().setUpClass()
cls.webdriver = webdriver.Chrome()
cls.webdriver.implicitly_wait(10)
@classmethod
def tearDownClass(cls):
cls.webdriver.close()
cls.webdriver.quit()
super().tearDownClass()
def setUp(self):
self.admin = User.objects.create_superuser(email=BACH_EMAIL, password=PASSWORD)
def test_admin_tool(self):
self.webdriver.get(f"http://{settings.ADMIN_HOST}:{self.server_thread.port}/admin")
self.webdriver.find_element_by_id("id_username").send_keys(BACH_EMAIL)
self.webdriver.find_element_by_id("id_password").send_keys(PASSWORD)
self.webdriver.find_element_by_id("id_password").send_keys(Keys.RETURN)
self.webdriver.find_element_by_link_text("Users").click()
When I run it, the test pass but still ends with this error:
Traceback (most recent call last):
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
psycopg2.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 168, in <module>
utility.execute()
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 142, in execute
_create_command().run_from_argv(self.argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementcommandstest.py", line 26, in run_from_argv
super().run_from_argv(argv)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 316, in run_from_argv
self.execute(*args, **cmd_options)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangocoremanagementbase.py", line 353, in execute
output = self.handle(*args, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_manage.py", line 104, in handle
failures = TestRunner(test_labels, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 255, in run_tests
extra_tests=extra_tests, **options)
File "C:Program FilesJetBrainsPyCharm 2018.2.4helperspycharmdjango_test_runner.py", line 156, in run_tests
return super(DjangoTeamcityTestRunner, self).run_tests(test_labels, extra_tests, **kwargs)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 607, in run_tests
self.teardown_databases(old_config)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestrunner.py", line 580, in teardown_databases
keepdb=self.keepdb,
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangotestutils.py", line 297, in teardown_databases
connection.creation.destroy_test_db(old_name, verbosity, keepdb)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 257, in destroy_test_db
self._destroy_test_db(test_database_name, verbosity)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsbasecreation.py", line 274, in _destroy_test_db
% self.connection.ops.quote_name(test_database_name))
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 68, in execute
return self._execute_with_wrappers(sql, params, many=False, executor=self._execute)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 77, in _execute_with_wrappers
return executor(sql, params, many, context)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbutils.py", line 89, in __exit__
raise dj_exc_value.with_traceback(traceback) from exc_value
File "C:UserspupenoDocumentsEligiblecodeexamplevenvlibsite-packagesdjangodbbackendsutils.py", line 83, in _execute
return self.cursor.execute(sql)
django.db.utils.OperationalError: database "test_example" is being accessed by other users
DETAIL: There is 1 other session using the database.
The problem of course is that the next run of the tests, the database still exists, so, the tests don't run without confirming deletion of the database.
If I comment out the last line:
self.webdriver.find_element_by_link_text("Users").click()
then I don't get this error. I guess just because the database connection is not established. Sometimes it's 1 other session, sometimes it's up to 4. In one of the cases of 4 sessions, these were the running sessions:
select * from pg_stat_activity where datname = 'test_example';
100123 test_example 29892 16393 pupeno "" ::1 61967 2018-11-15 17:28:19.552431 2018-11-15 17:28:19.562398 2018-11-15 17:28:19.564623 idle SELECT "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined" FROM "core_user" WHERE "core_user"."id" = 1
100123 test_example 33028 16393 pupeno "" ::1 61930 2018-11-15 17:28:18.792466 2018-11-15 17:28:18.843383 2018-11-15 17:28:18.851828 idle SELECT "django_admin_log"."id", "django_admin_log"."action_time", "django_admin_log"."user_id", "django_admin_log"."content_type_id", "django_admin_log"."object_id", "django_admin_log"."object_repr", "django_admin_log"."action_flag", "django_admin_log"."change_message", "core_user"."id", "core_user"."password", "core_user"."last_login", "core_user"."is_superuser", "core_user"."email", "core_user"."is_staff", "core_user"."is_active", "core_user"."date_joined", "django_content_type"."id", "django_content_type"."app_label", "django_content_type"."model" FROM "django_admin_log" INNER JOIN "core_user" ON ("django_admin_log"."user_id" = "core_user"."id") LEFT OUTER JOIN "django_content_type" ON ("django_admin_log"."content_type_id" = "django_content_type"."id") WHERE "django_admin_log"."user_id" = 1 ORDER BY "django_admin_log"."action_time" DESC LIMIT 10
100123 test_example 14128 16393 pupeno "" ::1 61988 2018-11-15 17:28:19.767225 2018-11-15 17:28:19.776150 2018-11-15 17:28:19.776479 idle SELECT "core_firm"."id", "core_firm"."name", "core_firm"."host_name" FROM "core_firm" WHERE "core_firm"."id" = 1
100123 test_example 9604 16393 pupeno "" ::1 61960 2018-11-15 17:28:19.469197 2018-11-15 17:28:19.478775 2018-11-15 17:28:19.478788 idle COMMIT
I've been trying to find the minimum reproducible example of this problem, but so far I haven't succeeded.
Any ideas what could be causing this or how to find out more about what the issue could be?
python django selenium testing
python django selenium testing
edited Nov 15 '18 at 17:31
pupeno
asked Nov 15 '18 at 16:23
pupenopupeno
106k99274441
106k99274441
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
Please runselect * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.
– Shlomi Bazel
Nov 15 '18 at 17:24
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
Maybe you are limited? checkSELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first
– Shlomi Bazel
Nov 15 '18 at 17:53
|
show 1 more comment
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
Please runselect * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.
– Shlomi Bazel
Nov 15 '18 at 17:24
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
Maybe you are limited? checkSELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first
– Shlomi Bazel
Nov 15 '18 at 17:53
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
Please run
select * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.– Shlomi Bazel
Nov 15 '18 at 17:24
Please run
select * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.– Shlomi Bazel
Nov 15 '18 at 17:24
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
Maybe you are limited? check
SELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first– Shlomi Bazel
Nov 15 '18 at 17:53
Maybe you are limited? check
SELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first– Shlomi Bazel
Nov 15 '18 at 17:53
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323775%2fdatabase-still-in-use-after-a-selenium-test-in-django%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323775%2fdatabase-still-in-use-after-a-selenium-test-in-django%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RIQAfKAzgXf3sa 9fYuSzTxqY FA6EZIblu2RtkTN Ny 4j45A4Bbon6SUwpvc
So you have no direct access to the database and no other tests being run that interact with the database, and yet you're getting this error?
– natn2323
Nov 15 '18 at 16:57
@natn2323: correct. I'm running this and only this test class. There's nothing else connecting or it would cause the problem regardless of me commenting out the last line.
– pupeno
Nov 15 '18 at 17:02
Please run
select * from pg_stat_activity where datname = 'TARGET_DB';
when the error happens to see who is connected and post info about it, so we can proceed.– Shlomi Bazel
Nov 15 '18 at 17:24
@ShlomiBazel: done.
– pupeno
Nov 15 '18 at 17:31
Maybe you are limited? check
SELECT rolname, rolconnlimit FROM pg_roles WHERE rolconnlimit <> -1;
if it doesn't show your your user then it's not limited... let me know first– Shlomi Bazel
Nov 15 '18 at 17:53