c# console get array value with index from user input
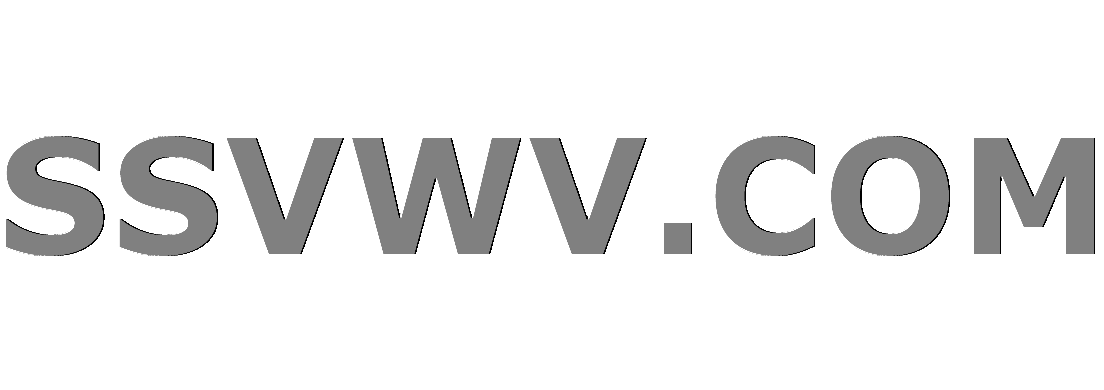
Multi tool use
I'm in an intro to programming class. Is it possible to output an array value when a user selects its index? Its not much but this is my code so far:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
c# console-application
add a comment |
I'm in an intro to programming class. Is it possible to output an array value when a user selects its index? Its not much but this is my code so far:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
c# console-application
Hi Jade - Are you asking to simply print out (ala,WriteLine
or similar) one of the values in your array? Akin toConsole.WriteLine(cisTuition[0])
printing out0.00
?
– gravitymixes
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26
add a comment |
I'm in an intro to programming class. Is it possible to output an array value when a user selects its index? Its not much but this is my code so far:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
c# console-application
I'm in an intro to programming class. Is it possible to output an array value when a user selects its index? Its not much but this is my code so far:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
c# console-application
c# console-application
asked Nov 15 '18 at 16:22


JadeJade
84
84
Hi Jade - Are you asking to simply print out (ala,WriteLine
or similar) one of the values in your array? Akin toConsole.WriteLine(cisTuition[0])
printing out0.00
?
– gravitymixes
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26
add a comment |
Hi Jade - Are you asking to simply print out (ala,WriteLine
or similar) one of the values in your array? Akin toConsole.WriteLine(cisTuition[0])
printing out0.00
?
– gravitymixes
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26
Hi Jade - Are you asking to simply print out (ala,
WriteLine
or similar) one of the values in your array? Akin to Console.WriteLine(cisTuition[0])
printing out 0.00
?– gravitymixes
Nov 15 '18 at 16:26
Hi Jade - Are you asking to simply print out (ala,
WriteLine
or similar) one of the values in your array? Akin to Console.WriteLine(cisTuition[0])
printing out 0.00
?– gravitymixes
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26
add a comment |
6 Answers
6
active
oldest
votes
You need to access using index of the array
Console.WriteLine(cisTuition[index]);
For example, if you need to get the index from the user entered input value,
int input = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[input]);
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
add a comment |
Yes, you can get the value of the cisTuition
array by their index: Check the snippet below
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
int index = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[index]);
Of course, you can improve that snippet validating that the index is inside the bounds of the array, but that's the basic idea.
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
add a comment |
Yes, you can reach an value inside and array at a particular index position as below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine(cisTuition[0]);
Ouput:
0.00
Then, as you requested to have an index provided by an input, I would use Console.ReadLine()
to get the user choice and record it into a variable (index
).
Lastly, I would use the variable as index with cisTuition[index]
.
See the full code below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Enter input:"); // Prompt the question
string index = Console.ReadLine(); // Record the input
Console.WriteLine(cisTuition[index]); // Show the array value of this index
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
add a comment |
bool Keeplooping = true; //Boolean to tell whether the loops continues
while (Keeplooping == true) //while the user hasn't chosen a valid index
{
Console.WriteLine("Select an index");
try //if this fails then the input is not an int or too big/small
{
int index = int.Parse(Console.ReadLine()); //receive input
Console.WriteLine(cisTuition[index].ToString()); //output the value
Keeplooping = false; //loop will end after this iteration
}
catch //alerts user that the input is bad and tries again
{
Console.WriteLine("Please select a valid index");
}
}
This should do the trick (I tested it already to be sure)
add a comment |
Well first you need the users input, when you got this you can do your proper checks, like is it NaN, or if it's outside the arrays index. After this it should be easy for look out the index in the array and then print it. I dont program that much in C#, but i guess it could look something like:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
var UserInput = getUserInput();
if(UserInput == NaN || UserInput > cisTuition.length)
print( cisTuition[UserInput] )
I know this is definitely not the correct syntax, but the logic should work.
Hope it helps.
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
add a comment |
I think what is being missed here is how to read in a value, convert it to an int
if possible, and then use that as the reference to the array index.
So, if that's the case, you'd want something akin to this added to the end of your code:
string strUserInput = Console.ReadLine();
int? iConverted = null;
int.TryParse(strUserInput, out iConverted);
if ((iConverted != null) && (iConverted <= (cisTuition.Length - 1)) )
{
Console.WriteLine(cisTuition[iConverted]);
}
else
{
Console.WriteLine("Invalid value or index of the array");
}
Full explanation:
ReadLine()
pulls in the value the user provides as the index to the array
iConverted
is instantiated (as null) to start, to allow an integer to also be null we have to use the ?
as well
We TryParse
the strUserInput
into an Integer, and dump it into iConverted
if successful (if not, iConverted
remains null)
Finally, we print the requested index array, or notify the user that their index was out of bounds, or not properly parsed.
1
AFAIKiConverted
would have to be anint?
for it to be able to storenull
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323768%2fc-sharp-console-get-array-value-with-index-from-user-input%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to access using index of the array
Console.WriteLine(cisTuition[index]);
For example, if you need to get the index from the user entered input value,
int input = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[input]);
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
add a comment |
You need to access using index of the array
Console.WriteLine(cisTuition[index]);
For example, if you need to get the index from the user entered input value,
int input = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[input]);
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
add a comment |
You need to access using index of the array
Console.WriteLine(cisTuition[index]);
For example, if you need to get the index from the user entered input value,
int input = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[input]);
You need to access using index of the array
Console.WriteLine(cisTuition[index]);
For example, if you need to get the index from the user entered input value,
int input = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[input]);
answered Nov 15 '18 at 16:27


SajeetharanSajeetharan
124k30180243
124k30180243
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
add a comment |
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
1
1
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
Thank you! This totally worked!
– Jade
Nov 15 '18 at 16:48
add a comment |
Yes, you can get the value of the cisTuition
array by their index: Check the snippet below
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
int index = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[index]);
Of course, you can improve that snippet validating that the index is inside the bounds of the array, but that's the basic idea.
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
add a comment |
Yes, you can get the value of the cisTuition
array by their index: Check the snippet below
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
int index = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[index]);
Of course, you can improve that snippet validating that the index is inside the bounds of the array, but that's the basic idea.
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
add a comment |
Yes, you can get the value of the cisTuition
array by their index: Check the snippet below
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
int index = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[index]);
Of course, you can improve that snippet validating that the index is inside the bounds of the array, but that's the basic idea.
Yes, you can get the value of the cisTuition
array by their index: Check the snippet below
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
int index = int.Parse(Console.ReadLine());
Console.WriteLine("value is: " + cisTuition[index]);
Of course, you can improve that snippet validating that the index is inside the bounds of the array, but that's the basic idea.
edited Nov 19 '18 at 21:37
answered Nov 15 '18 at 16:28
Daniel CorzoDaniel Corzo
1,46221527
1,46221527
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
add a comment |
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
Yes! Thank you!
– Jade
Nov 15 '18 at 16:50
add a comment |
Yes, you can reach an value inside and array at a particular index position as below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine(cisTuition[0]);
Ouput:
0.00
Then, as you requested to have an index provided by an input, I would use Console.ReadLine()
to get the user choice and record it into a variable (index
).
Lastly, I would use the variable as index with cisTuition[index]
.
See the full code below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Enter input:"); // Prompt the question
string index = Console.ReadLine(); // Record the input
Console.WriteLine(cisTuition[index]); // Show the array value of this index
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
add a comment |
Yes, you can reach an value inside and array at a particular index position as below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine(cisTuition[0]);
Ouput:
0.00
Then, as you requested to have an index provided by an input, I would use Console.ReadLine()
to get the user choice and record it into a variable (index
).
Lastly, I would use the variable as index with cisTuition[index]
.
See the full code below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Enter input:"); // Prompt the question
string index = Console.ReadLine(); // Record the input
Console.WriteLine(cisTuition[index]); // Show the array value of this index
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
add a comment |
Yes, you can reach an value inside and array at a particular index position as below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine(cisTuition[0]);
Ouput:
0.00
Then, as you requested to have an index provided by an input, I would use Console.ReadLine()
to get the user choice and record it into a variable (index
).
Lastly, I would use the variable as index with cisTuition[index]
.
See the full code below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Enter input:"); // Prompt the question
string index = Console.ReadLine(); // Record the input
Console.WriteLine(cisTuition[index]); // Show the array value of this index
Yes, you can reach an value inside and array at a particular index position as below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine(cisTuition[0]);
Ouput:
0.00
Then, as you requested to have an index provided by an input, I would use Console.ReadLine()
to get the user choice and record it into a variable (index
).
Lastly, I would use the variable as index with cisTuition[index]
.
See the full code below:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Enter input:"); // Prompt the question
string index = Console.ReadLine(); // Record the input
Console.WriteLine(cisTuition[index]); // Show the array value of this index
edited Nov 15 '18 at 17:01
answered Nov 15 '18 at 16:25


Jonathan GagneJonathan Gagne
2,1993722
2,1993722
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
add a comment |
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes, but how would a user be able to select the index with like a Console.ReadLine()?
– Jade
Nov 15 '18 at 16:29
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
Yes totally, please look at the code.
– Jonathan Gagne
Nov 15 '18 at 16:56
add a comment |
bool Keeplooping = true; //Boolean to tell whether the loops continues
while (Keeplooping == true) //while the user hasn't chosen a valid index
{
Console.WriteLine("Select an index");
try //if this fails then the input is not an int or too big/small
{
int index = int.Parse(Console.ReadLine()); //receive input
Console.WriteLine(cisTuition[index].ToString()); //output the value
Keeplooping = false; //loop will end after this iteration
}
catch //alerts user that the input is bad and tries again
{
Console.WriteLine("Please select a valid index");
}
}
This should do the trick (I tested it already to be sure)
add a comment |
bool Keeplooping = true; //Boolean to tell whether the loops continues
while (Keeplooping == true) //while the user hasn't chosen a valid index
{
Console.WriteLine("Select an index");
try //if this fails then the input is not an int or too big/small
{
int index = int.Parse(Console.ReadLine()); //receive input
Console.WriteLine(cisTuition[index].ToString()); //output the value
Keeplooping = false; //loop will end after this iteration
}
catch //alerts user that the input is bad and tries again
{
Console.WriteLine("Please select a valid index");
}
}
This should do the trick (I tested it already to be sure)
add a comment |
bool Keeplooping = true; //Boolean to tell whether the loops continues
while (Keeplooping == true) //while the user hasn't chosen a valid index
{
Console.WriteLine("Select an index");
try //if this fails then the input is not an int or too big/small
{
int index = int.Parse(Console.ReadLine()); //receive input
Console.WriteLine(cisTuition[index].ToString()); //output the value
Keeplooping = false; //loop will end after this iteration
}
catch //alerts user that the input is bad and tries again
{
Console.WriteLine("Please select a valid index");
}
}
This should do the trick (I tested it already to be sure)
bool Keeplooping = true; //Boolean to tell whether the loops continues
while (Keeplooping == true) //while the user hasn't chosen a valid index
{
Console.WriteLine("Select an index");
try //if this fails then the input is not an int or too big/small
{
int index = int.Parse(Console.ReadLine()); //receive input
Console.WriteLine(cisTuition[index].ToString()); //output the value
Keeplooping = false; //loop will end after this iteration
}
catch //alerts user that the input is bad and tries again
{
Console.WriteLine("Please select a valid index");
}
}
This should do the trick (I tested it already to be sure)
edited Nov 15 '18 at 18:10
answered Nov 15 '18 at 16:51


DJ Spicy Deluxe-LeviDJ Spicy Deluxe-Levi
1596
1596
add a comment |
add a comment |
Well first you need the users input, when you got this you can do your proper checks, like is it NaN, or if it's outside the arrays index. After this it should be easy for look out the index in the array and then print it. I dont program that much in C#, but i guess it could look something like:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
var UserInput = getUserInput();
if(UserInput == NaN || UserInput > cisTuition.length)
print( cisTuition[UserInput] )
I know this is definitely not the correct syntax, but the logic should work.
Hope it helps.
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
add a comment |
Well first you need the users input, when you got this you can do your proper checks, like is it NaN, or if it's outside the arrays index. After this it should be easy for look out the index in the array and then print it. I dont program that much in C#, but i guess it could look something like:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
var UserInput = getUserInput();
if(UserInput == NaN || UserInput > cisTuition.length)
print( cisTuition[UserInput] )
I know this is definitely not the correct syntax, but the logic should work.
Hope it helps.
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
add a comment |
Well first you need the users input, when you got this you can do your proper checks, like is it NaN, or if it's outside the arrays index. After this it should be easy for look out the index in the array and then print it. I dont program that much in C#, but i guess it could look something like:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
var UserInput = getUserInput();
if(UserInput == NaN || UserInput > cisTuition.length)
print( cisTuition[UserInput] )
I know this is definitely not the correct syntax, but the logic should work.
Hope it helps.
Well first you need the users input, when you got this you can do your proper checks, like is it NaN, or if it's outside the arrays index. After this it should be easy for look out the index in the array and then print it. I dont program that much in C#, but i guess it could look something like:
double cisTuition = new double { 0.00, 1.00, 1.50, 2.00, 2.50 };
Console.WriteLine("Please choose the semester");
var UserInput = getUserInput();
if(UserInput == NaN || UserInput > cisTuition.length)
print( cisTuition[UserInput] )
I know this is definitely not the correct syntax, but the logic should work.
Hope it helps.
answered Nov 15 '18 at 16:29
Daniel EDaniel E
1
1
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
add a comment |
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
1
1
Please format your code block.
– Tau
Nov 15 '18 at 17:08
Please format your code block.
– Tau
Nov 15 '18 at 17:08
add a comment |
I think what is being missed here is how to read in a value, convert it to an int
if possible, and then use that as the reference to the array index.
So, if that's the case, you'd want something akin to this added to the end of your code:
string strUserInput = Console.ReadLine();
int? iConverted = null;
int.TryParse(strUserInput, out iConverted);
if ((iConverted != null) && (iConverted <= (cisTuition.Length - 1)) )
{
Console.WriteLine(cisTuition[iConverted]);
}
else
{
Console.WriteLine("Invalid value or index of the array");
}
Full explanation:
ReadLine()
pulls in the value the user provides as the index to the array
iConverted
is instantiated (as null) to start, to allow an integer to also be null we have to use the ?
as well
We TryParse
the strUserInput
into an Integer, and dump it into iConverted
if successful (if not, iConverted
remains null)
Finally, we print the requested index array, or notify the user that their index was out of bounds, or not properly parsed.
1
AFAIKiConverted
would have to be anint?
for it to be able to storenull
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
add a comment |
I think what is being missed here is how to read in a value, convert it to an int
if possible, and then use that as the reference to the array index.
So, if that's the case, you'd want something akin to this added to the end of your code:
string strUserInput = Console.ReadLine();
int? iConverted = null;
int.TryParse(strUserInput, out iConverted);
if ((iConverted != null) && (iConverted <= (cisTuition.Length - 1)) )
{
Console.WriteLine(cisTuition[iConverted]);
}
else
{
Console.WriteLine("Invalid value or index of the array");
}
Full explanation:
ReadLine()
pulls in the value the user provides as the index to the array
iConverted
is instantiated (as null) to start, to allow an integer to also be null we have to use the ?
as well
We TryParse
the strUserInput
into an Integer, and dump it into iConverted
if successful (if not, iConverted
remains null)
Finally, we print the requested index array, or notify the user that their index was out of bounds, or not properly parsed.
1
AFAIKiConverted
would have to be anint?
for it to be able to storenull
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
add a comment |
I think what is being missed here is how to read in a value, convert it to an int
if possible, and then use that as the reference to the array index.
So, if that's the case, you'd want something akin to this added to the end of your code:
string strUserInput = Console.ReadLine();
int? iConverted = null;
int.TryParse(strUserInput, out iConverted);
if ((iConverted != null) && (iConverted <= (cisTuition.Length - 1)) )
{
Console.WriteLine(cisTuition[iConverted]);
}
else
{
Console.WriteLine("Invalid value or index of the array");
}
Full explanation:
ReadLine()
pulls in the value the user provides as the index to the array
iConverted
is instantiated (as null) to start, to allow an integer to also be null we have to use the ?
as well
We TryParse
the strUserInput
into an Integer, and dump it into iConverted
if successful (if not, iConverted
remains null)
Finally, we print the requested index array, or notify the user that their index was out of bounds, or not properly parsed.
I think what is being missed here is how to read in a value, convert it to an int
if possible, and then use that as the reference to the array index.
So, if that's the case, you'd want something akin to this added to the end of your code:
string strUserInput = Console.ReadLine();
int? iConverted = null;
int.TryParse(strUserInput, out iConverted);
if ((iConverted != null) && (iConverted <= (cisTuition.Length - 1)) )
{
Console.WriteLine(cisTuition[iConverted]);
}
else
{
Console.WriteLine("Invalid value or index of the array");
}
Full explanation:
ReadLine()
pulls in the value the user provides as the index to the array
iConverted
is instantiated (as null) to start, to allow an integer to also be null we have to use the ?
as well
We TryParse
the strUserInput
into an Integer, and dump it into iConverted
if successful (if not, iConverted
remains null)
Finally, we print the requested index array, or notify the user that their index was out of bounds, or not properly parsed.
edited Nov 15 '18 at 17:34
answered Nov 15 '18 at 16:31


gravitymixesgravitymixes
1,64911425
1,64911425
1
AFAIKiConverted
would have to be anint?
for it to be able to storenull
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
add a comment |
1
AFAIKiConverted
would have to be anint?
for it to be able to storenull
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
1
1
AFAIK
iConverted
would have to be an int?
for it to be able to store null
– Tau
Nov 15 '18 at 17:02
AFAIK
iConverted
would have to be an int?
for it to be able to store null
– Tau
Nov 15 '18 at 17:02
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
Fair point @Tau - What I get for writing off the cuff. :)
– gravitymixes
Nov 15 '18 at 17:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323768%2fc-sharp-console-get-array-value-with-index-from-user-input%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QZz,qdQlRRiKvD ITfznm,Jyf,yX klm,4 cBaGzy
Hi Jade - Are you asking to simply print out (ala,
WriteLine
or similar) one of the values in your array? Akin toConsole.WriteLine(cisTuition[0])
printing out0.00
?– gravitymixes
Nov 15 '18 at 16:26
Hint: you can access the value of an element of an array by its index. Ex. cisTuition[n], when n is the index number. Remember that arrays are indexed starting at 0.
– Michael Roy
Nov 15 '18 at 16:26