Inconsistent behavior for Firebase Realtime Database
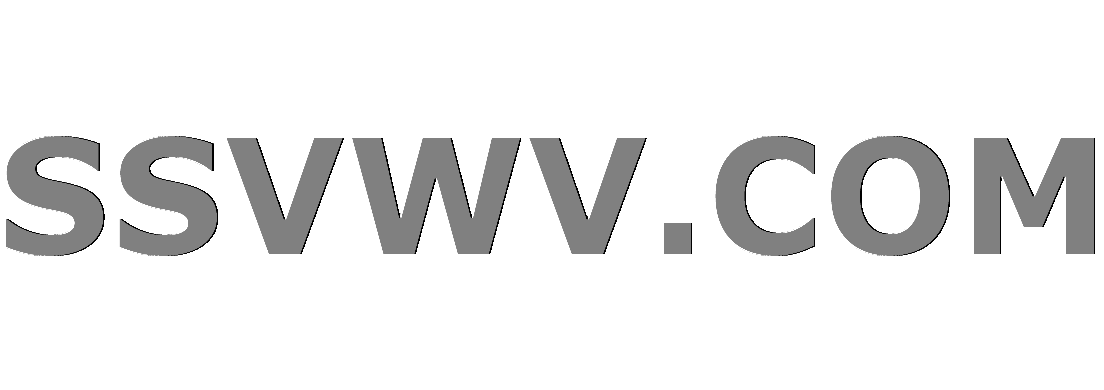
Multi tool use
I try to update the data in Firebase Realtime Database through Android app. It will only occasionally work after I open the app and wait for some time. Most of the time it does not work. In my case, working mean the data is updated in cloud. I am wondering why it work sometimes and do not work sometimes. There is no error or warning in Logcat. Once it works, it will continue to work until the app is restarted.
DbHelper class
public class DbHelper {
static final String TAG = "ttt";
int count = 0;
private static FirebaseDatabase database = FirebaseDatabase.getInstance();
private static DatabaseReference myRef = database.getReference("message");
TextView tv;
public DbHelper(final TextView tv){
this.tv = tv;
myRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String text = dataSnapshot.getValue(String.class);
tv.setText(text);
Log.d(TAG, "Value is: " + text);
}
@Override
public void onCancelled(DatabaseError databaseError) {
Log.w(TAG, "Failed to read value.", databaseError.toException());
}
});
}
public void testing(){
myRef.setValue("test"+count++);
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView textTesting;
DbHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
textTesting = (TextView)findViewById(R.id.text_testing);
dbHelper = new DbHelper(textTesting);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dbHelper.testing();
}
});
}
}
Added
DatabaseReference connectedRef = FirebaseDatabase.getInstance().getReference(".info/connected");
connectedRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot snapshot) {
boolean connected = snapshot.getValue(Boolean.class);
if (connected) {
Log.d(TAG, "connected");
} else {
Log.d(TAG, "not connected");
}
}
@Override
public void onCancelled(DatabaseError error) {
System.err.println("Listener was cancelled");
}
});
Logcat
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.961 19329-19384/? V/FA: Activity resumed, time: 167270724
11-14 20:26:58.986 19329-19384/? D/FA: Connected to remote service
11-14 20:26:59.001 19329-19329/? I/Timeline: Timeline: Activity_idle id: android.os.BinderProxy@34fb88b7 time:15941479
11-14 20:26:59.006 19329-19384/? V/FA: Processing queued up service tasks: 4
11-14 20:26:59.056 19329-19336/? I/art: Ignoring second debugger -- accepting and dropping
11-14 20:26:59.541 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:26:59.631 19329-19329/? D/ttt: Value is: test0
11-14 20:27:02.366 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:27:02.451 19329-19329/? D/ttt: Value is: test1
11-14 20:27:04.166 19329-19384/? V/FA: Inactivity, disconnecting from the service
11-14 20:28:07.721 19329-19329/? D/FA: Service connection suspended

|
show 8 more comments
I try to update the data in Firebase Realtime Database through Android app. It will only occasionally work after I open the app and wait for some time. Most of the time it does not work. In my case, working mean the data is updated in cloud. I am wondering why it work sometimes and do not work sometimes. There is no error or warning in Logcat. Once it works, it will continue to work until the app is restarted.
DbHelper class
public class DbHelper {
static final String TAG = "ttt";
int count = 0;
private static FirebaseDatabase database = FirebaseDatabase.getInstance();
private static DatabaseReference myRef = database.getReference("message");
TextView tv;
public DbHelper(final TextView tv){
this.tv = tv;
myRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String text = dataSnapshot.getValue(String.class);
tv.setText(text);
Log.d(TAG, "Value is: " + text);
}
@Override
public void onCancelled(DatabaseError databaseError) {
Log.w(TAG, "Failed to read value.", databaseError.toException());
}
});
}
public void testing(){
myRef.setValue("test"+count++);
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView textTesting;
DbHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
textTesting = (TextView)findViewById(R.id.text_testing);
dbHelper = new DbHelper(textTesting);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dbHelper.testing();
}
});
}
}
Added
DatabaseReference connectedRef = FirebaseDatabase.getInstance().getReference(".info/connected");
connectedRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot snapshot) {
boolean connected = snapshot.getValue(Boolean.class);
if (connected) {
Log.d(TAG, "connected");
} else {
Log.d(TAG, "not connected");
}
}
@Override
public void onCancelled(DatabaseError error) {
System.err.println("Listener was cancelled");
}
});
Logcat
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.961 19329-19384/? V/FA: Activity resumed, time: 167270724
11-14 20:26:58.986 19329-19384/? D/FA: Connected to remote service
11-14 20:26:59.001 19329-19329/? I/Timeline: Timeline: Activity_idle id: android.os.BinderProxy@34fb88b7 time:15941479
11-14 20:26:59.006 19329-19384/? V/FA: Processing queued up service tasks: 4
11-14 20:26:59.056 19329-19336/? I/art: Ignoring second debugger -- accepting and dropping
11-14 20:26:59.541 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:26:59.631 19329-19329/? D/ttt: Value is: test0
11-14 20:27:02.366 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:27:02.451 19329-19329/? D/ttt: Value is: test1
11-14 20:27:04.166 19329-19384/? V/FA: Inactivity, disconnecting from the service
11-14 20:28:07.721 19329-19329/? D/FA: Service connection suspended

4
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
1
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
1
@SamTew Check Logcat for any messages from Firebase such asD/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.
– Grimthorr
Nov 13 '18 at 14:36
1
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27
|
show 8 more comments
I try to update the data in Firebase Realtime Database through Android app. It will only occasionally work after I open the app and wait for some time. Most of the time it does not work. In my case, working mean the data is updated in cloud. I am wondering why it work sometimes and do not work sometimes. There is no error or warning in Logcat. Once it works, it will continue to work until the app is restarted.
DbHelper class
public class DbHelper {
static final String TAG = "ttt";
int count = 0;
private static FirebaseDatabase database = FirebaseDatabase.getInstance();
private static DatabaseReference myRef = database.getReference("message");
TextView tv;
public DbHelper(final TextView tv){
this.tv = tv;
myRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String text = dataSnapshot.getValue(String.class);
tv.setText(text);
Log.d(TAG, "Value is: " + text);
}
@Override
public void onCancelled(DatabaseError databaseError) {
Log.w(TAG, "Failed to read value.", databaseError.toException());
}
});
}
public void testing(){
myRef.setValue("test"+count++);
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView textTesting;
DbHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
textTesting = (TextView)findViewById(R.id.text_testing);
dbHelper = new DbHelper(textTesting);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dbHelper.testing();
}
});
}
}
Added
DatabaseReference connectedRef = FirebaseDatabase.getInstance().getReference(".info/connected");
connectedRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot snapshot) {
boolean connected = snapshot.getValue(Boolean.class);
if (connected) {
Log.d(TAG, "connected");
} else {
Log.d(TAG, "not connected");
}
}
@Override
public void onCancelled(DatabaseError error) {
System.err.println("Listener was cancelled");
}
});
Logcat
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.961 19329-19384/? V/FA: Activity resumed, time: 167270724
11-14 20:26:58.986 19329-19384/? D/FA: Connected to remote service
11-14 20:26:59.001 19329-19329/? I/Timeline: Timeline: Activity_idle id: android.os.BinderProxy@34fb88b7 time:15941479
11-14 20:26:59.006 19329-19384/? V/FA: Processing queued up service tasks: 4
11-14 20:26:59.056 19329-19336/? I/art: Ignoring second debugger -- accepting and dropping
11-14 20:26:59.541 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:26:59.631 19329-19329/? D/ttt: Value is: test0
11-14 20:27:02.366 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:27:02.451 19329-19329/? D/ttt: Value is: test1
11-14 20:27:04.166 19329-19384/? V/FA: Inactivity, disconnecting from the service
11-14 20:28:07.721 19329-19329/? D/FA: Service connection suspended

I try to update the data in Firebase Realtime Database through Android app. It will only occasionally work after I open the app and wait for some time. Most of the time it does not work. In my case, working mean the data is updated in cloud. I am wondering why it work sometimes and do not work sometimes. There is no error or warning in Logcat. Once it works, it will continue to work until the app is restarted.
DbHelper class
public class DbHelper {
static final String TAG = "ttt";
int count = 0;
private static FirebaseDatabase database = FirebaseDatabase.getInstance();
private static DatabaseReference myRef = database.getReference("message");
TextView tv;
public DbHelper(final TextView tv){
this.tv = tv;
myRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String text = dataSnapshot.getValue(String.class);
tv.setText(text);
Log.d(TAG, "Value is: " + text);
}
@Override
public void onCancelled(DatabaseError databaseError) {
Log.w(TAG, "Failed to read value.", databaseError.toException());
}
});
}
public void testing(){
myRef.setValue("test"+count++);
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView textTesting;
DbHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
textTesting = (TextView)findViewById(R.id.text_testing);
dbHelper = new DbHelper(textTesting);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dbHelper.testing();
}
});
}
}
Added
DatabaseReference connectedRef = FirebaseDatabase.getInstance().getReference(".info/connected");
connectedRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot snapshot) {
boolean connected = snapshot.getValue(Boolean.class);
if (connected) {
Log.d(TAG, "connected");
} else {
Log.d(TAG, "not connected");
}
}
@Override
public void onCancelled(DatabaseError error) {
System.err.println("Listener was cancelled");
}
});
Logcat
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.956 19329-19384/? V/FA: Connection attempt already in progress
11-14 20:26:58.961 19329-19384/? V/FA: Activity resumed, time: 167270724
11-14 20:26:58.986 19329-19384/? D/FA: Connected to remote service
11-14 20:26:59.001 19329-19329/? I/Timeline: Timeline: Activity_idle id: android.os.BinderProxy@34fb88b7 time:15941479
11-14 20:26:59.006 19329-19384/? V/FA: Processing queued up service tasks: 4
11-14 20:26:59.056 19329-19336/? I/art: Ignoring second debugger -- accepting and dropping
11-14 20:26:59.541 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:26:59.631 19329-19329/? D/ttt: Value is: test0
11-14 20:27:02.366 19329-19329/? D/ViewRootImpl: ViewPostImeInputStage ACTION_DOWN
11-14 20:27:02.451 19329-19329/? D/ttt: Value is: test1
11-14 20:27:04.166 19329-19384/? V/FA: Inactivity, disconnecting from the service
11-14 20:28:07.721 19329-19329/? D/FA: Service connection suspended


edited Nov 14 '18 at 13:59
SamTew
asked Nov 13 '18 at 13:42
SamTewSamTew
368425
368425
4
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
1
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
1
@SamTew Check Logcat for any messages from Firebase such asD/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.
– Grimthorr
Nov 13 '18 at 14:36
1
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27
|
show 8 more comments
4
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
1
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
1
@SamTew Check Logcat for any messages from Firebase such asD/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.
– Grimthorr
Nov 13 '18 at 14:36
1
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27
4
4
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
1
1
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
1
1
@SamTew Check Logcat for any messages from Firebase such as
D/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.– Grimthorr
Nov 13 '18 at 14:36
@SamTew Check Logcat for any messages from Firebase such as
D/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.– Grimthorr
Nov 13 '18 at 14:36
1
1
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27
|
show 8 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282362%2finconsistent-behavior-for-firebase-realtime-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282362%2finconsistent-behavior-for-firebase-realtime-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LpbS1mPjz Hg0PD 6S1SChb0KKvAUxHVgarnpE92S7cqEAu,ljMi,RZHBqszeTz r,7
4
What do you mean by "it does not work"? What happens? Do you see any errors or messages in Logcat?
– Grimthorr
Nov 13 '18 at 13:55
Is there any reason why you put Firebase operations in a separate class instead of using instant creations?
– Cool Guy CG
Nov 13 '18 at 13:59
1
@SamTew Do you have disk persistence ("offline capabilities") enabled? If the data is updated locally but never synced to Firebase: it sounds like a network issue.
– Grimthorr
Nov 13 '18 at 14:15
1
@SamTew Check Logcat for any messages from Firebase such as
D/FA: Connected to remote service
to see if your app is actually connecting to the Firebase services.– Grimthorr
Nov 13 '18 at 14:36
1
@Grimthorr I found out the reason. The DNS used by ISP is the problem. Change to Google DNS solved my problem
– SamTew
Nov 18 '18 at 1:27