How to check if a user input is equal to the id of a div element in javascript?
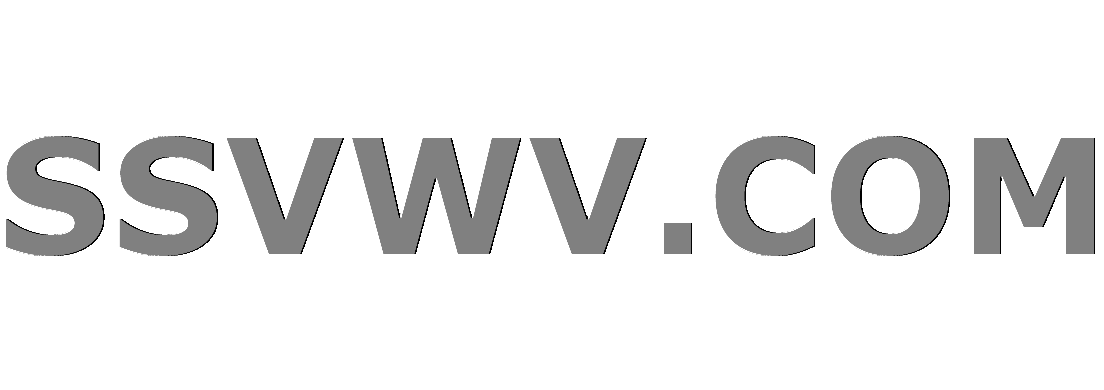
Multi tool use
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
javascript if-statement id
add a comment |
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
javascript if-statement id
Not sure what you're trying to do, but I think what you want isif (correctLetter === $("#" + correctLetter))
.
– Federico klez Culloca
Nov 13 '18 at 13:46
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how isword
defined, orpossibleGuessArray
?
– OliverRadini
Nov 13 '18 at 14:49
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58
add a comment |
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
javascript if-statement id
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
This is for hangman when the user guesses the correct letter I want to be able to append that letter to the div that I have dynamically created with an id of that letter.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
//event listener
document.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
javascript if-statement id
javascript if-statement id
edited Nov 14 '18 at 14:06


OliverRadini
2,792627
2,792627
asked Nov 13 '18 at 13:43


Megan MeluskeyMegan Meluskey
12
12
Not sure what you're trying to do, but I think what you want isif (correctLetter === $("#" + correctLetter))
.
– Federico klez Culloca
Nov 13 '18 at 13:46
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how isword
defined, orpossibleGuessArray
?
– OliverRadini
Nov 13 '18 at 14:49
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58
add a comment |
Not sure what you're trying to do, but I think what you want isif (correctLetter === $("#" + correctLetter))
.
– Federico klez Culloca
Nov 13 '18 at 13:46
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how isword
defined, orpossibleGuessArray
?
– OliverRadini
Nov 13 '18 at 14:49
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58
Not sure what you're trying to do, but I think what you want is
if (correctLetter === $("#" + correctLetter))
.– Federico klez Culloca
Nov 13 '18 at 13:46
Not sure what you're trying to do, but I think what you want is
if (correctLetter === $("#" + correctLetter))
.– Federico klez Culloca
Nov 13 '18 at 13:46
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how is
word
defined, or possibleGuessArray
?– OliverRadini
Nov 13 '18 at 14:49
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how is
word
defined, or possibleGuessArray
?– OliverRadini
Nov 13 '18 at 14:49
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58
add a comment |
2 Answers
2
active
oldest
votes
Checking ID value is not required, can be done like below. also added running code snippet.
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
add a comment |
I believe the code below goes some way to doing what you are looking to do. The only really important change are the lines:
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
and
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
These setup the class rather than the id of the guess results, and then append to them with the letter which was guessed. It's normally best to use a class
rather than an id
when you're looking to select multiple elements (and you may have more than one of the same letter)
I added an input element and set the keyup
event from that, rather than document
. Some of the key presses on document
don't seem to be heard correctly for me, but if you are having no issues there then document
should be fine.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282378%2fhow-to-check-if-a-user-input-is-equal-to-the-id-of-a-div-element-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Checking ID value is not required, can be done like below. also added running code snippet.
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
add a comment |
Checking ID value is not required, can be done like below. also added running code snippet.
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
add a comment |
Checking ID value is not required, can be done like below. also added running code snippet.
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
Checking ID value is not required, can be done like below. also added running code snippet.
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
var str = 'word';
var lettersOfWordArray = str.split("");
var correctGuess=;
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: "blank-box",
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
document.onkeyup = function (event) {
var userGuess = event.key;
if (correctGuess.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
console.log(userGuess + " is correct");
var correctLetter = userGuess;
correctGuess.push(userGuess);
}
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<html>
<body>
<div id="word-guessed"> </div>
</body>
</html>
answered Nov 13 '18 at 14:45


BhalkeBhalke
1,36952136
1,36952136
add a comment |
add a comment |
I believe the code below goes some way to doing what you are looking to do. The only really important change are the lines:
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
and
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
These setup the class rather than the id of the guess results, and then append to them with the letter which was guessed. It's normally best to use a class
rather than an id
when you're looking to select multiple elements (and you may have more than one of the same letter)
I added an input element and set the keyup
event from that, rather than document
. Some of the key presses on document
don't seem to be heard correctly for me, but if you are having no issues there then document
should be fine.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
add a comment |
I believe the code below goes some way to doing what you are looking to do. The only really important change are the lines:
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
and
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
These setup the class rather than the id of the guess results, and then append to them with the letter which was guessed. It's normally best to use a class
rather than an id
when you're looking to select multiple elements (and you may have more than one of the same letter)
I added an input element and set the keyup
event from that, rather than document
. Some of the key presses on document
don't seem to be heard correctly for me, but if you are having no issues there then document
should be fine.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
add a comment |
I believe the code below goes some way to doing what you are looking to do. The only really important change are the lines:
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
and
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
These setup the class rather than the id of the guess results, and then append to them with the letter which was guessed. It's normally best to use a class
rather than an id
when you're looking to select multiple elements (and you may have more than one of the same letter)
I added an input element and set the keyup
event from that, rather than document
. Some of the key presses on document
don't seem to be heard correctly for me, but if you are having no issues there then document
should be fine.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
I believe the code below goes some way to doing what you are looking to do. The only really important change are the lines:
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
and
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
These setup the class rather than the id of the guess results, and then append to them with the letter which was guessed. It's normally best to use a class
rather than an id
when you're looking to select multiple elements (and you may have more than one of the same letter)
I added an input element and set the keyup
event from that, rather than document
. Some of the key presses on document
don't seem to be heard correctly for me, but if you are having no issues there then document
should be fine.
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
$(document).ready(function() {
console.log("ready!");
possibleGuessArray = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"]
userGuessArray = ;
correctGuessArray = ;
var randomWords = [
"dog",
"cat",
"america",
"bootcamp",
"javascript",
"philadelphia"
]
var word = randomWords[Math.floor(Math.random() * randomWords.length)]; {
console.log(word);
}
var amount = word.length;
console.log(amount);
$("#display-word").on("click", function(event) {
$("#word").html("New Word is: " + amount + " letters long.")
})
var str = word;
var lettersOfWordArray = str.split("");
console.log(lettersOfWordArray);
for (var i = 0; i < lettersOfWordArray.length; i++) {
jQuery('<div/>', {
class: `blank-box guess-result-${lettersOfWordArray[i]}`,
value: i,
id: lettersOfWordArray[i]
}).appendTo("#word-guessed");
}
var ids = ;
$(".blank-box").each(function() {
ids.push(this.id);
});
console.log("ids: " + ids);
//if the letter guessed equals the id of the div, append the user guess to that div
var inputElement = document.getElementById('letter-input');
//event listener
inputElement.onkeyup = function(event) {
var userGuess = event.key;
if (userGuessArray.includes(userGuess) || correctGuessArray.includes(userGuess)) {
confirm("You have already guessed letter " + userGuess);
} else {
if (possibleGuessArray.includes(userGuess)) {
if (word.includes(userGuess)) {
console.log(userGuess + " is correct");
correctGuessArray.push(userGuess);
$(`.guess-result-${userGuess}`).each(function(i, e) {
e.append(userGuess)
})
} else {
$("#guesses").append(userGuess + "-");
console.log("You guessed the wrong letter");
userGuessArray.push(userGuess);
console.log(userGuessArray);
}
} else {
confirm(userGuess + "is not a valid guess. Please enter a letter!")
}
} //end of else for userGuessArray.includes(userGuess)
} //document on key up
}); //document.ready
#word-guessed {
font-family: arial;
}
#word-guessed div {
display: inline-block;
width: 15px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="word-guessed"></div>
<input id="letter-input" />
edited Nov 14 '18 at 14:31
answered Nov 14 '18 at 14:16


OliverRadiniOliverRadini
2,792627
2,792627
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282378%2fhow-to-check-if-a-user-input-is-equal-to-the-id-of-a-div-element-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ks,evZhEV8PWK9eJ,MlAr,cdpcwOm0cHsnImwP5W,HNvofz vt,kKASdZD4 Z CQaRn7bAyJGUmMAV
Not sure what you're trying to do, but I think what you want is
if (correctLetter === $("#" + correctLetter))
.– Federico klez Culloca
Nov 13 '18 at 13:46
try correctLetter === $("#id").val()
– Bhalke
Nov 13 '18 at 13:56
Are you able to provide some more of your code? There are few things for which we can't see a definition, for instance, how is
word
defined, orpossibleGuessArray
?– OliverRadini
Nov 13 '18 at 14:49
I just edited the original post with the full code, thanks for your help.
– Megan Meluskey
Nov 13 '18 at 14:58