How do I clip an entity or geometry against underside of terrain
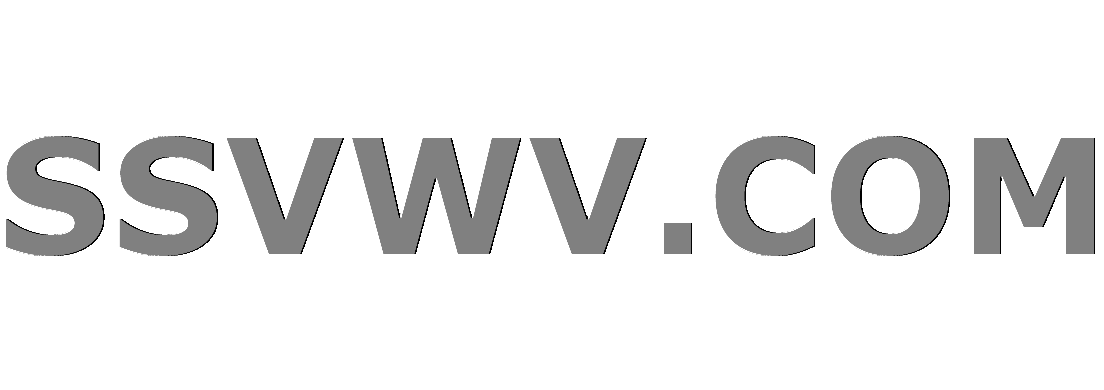
Multi tool use
I am using the Cesium.Globe clipping planes to extract a square geographic section. But one problem is that terrain back-faces are not rendered which makes it look weird when the user selects a shallow viewing angle.
I thought one way to hide this was to render the "soil" as a box or wall under the terrain, but I would need the top of the box or wall to confirm to the terrain geometry (red line).
I figure I can achieve this by using a WallGeometry around the culled terrain instead of a box, and set the height of each section based on the result of a sampleTerrain call.
But I was wondering if Cesium doesn't provide an easier and cleaner way to do this. (Like perhaps some Boolean union function or something)
Cesium Sandcastle link
var viewer = new Cesium.Viewer('cesiumContainer', {
skyAtmosphere: false,
shouldAnimate : true,
terrainProvider: Cesium.createWorldTerrain()
});
var globe = viewer.scene.globe;
var position = Cesium.Cartographic.toCartesian(new Cesium.Cartographic.fromDegrees(-113.2665534, 36.0939345, 100));
var distance = 3000.0;
globe.clippingPlanes = new Cesium.ClippingPlaneCollection({
modelMatrix : Cesium.Transforms.eastNorthUpToFixedFrame(position),
planes : [
new Cesium.ClippingPlane(new Cesium.Cartesian3( 1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3(-1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, 1.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, -1.0, 0.0), distance)
],
unionClippingRegions : true,
edgeWidth:3,
edgeColor: Cesium.Color.RED,
enabled : true
});
var rockBox = viewer.entities.add({
name : 'RockBox',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 900),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2,800.0),
material : Cesium.Color.GRAY.withAlpha(0.4),
outline : true,
outlineColor : Cesium.Color.GRAY
}
});
var waterTable = viewer.entities.add({
name : 'WaterTable',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 440),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2, 160.0),
material : Cesium.Color.BLUE.withAlpha(0.9),
outline : true,
outlineColor : Cesium.Color.BLUE
}
});
viewer.zoomTo(viewer.entities);
javascript cesium
add a comment |
I am using the Cesium.Globe clipping planes to extract a square geographic section. But one problem is that terrain back-faces are not rendered which makes it look weird when the user selects a shallow viewing angle.
I thought one way to hide this was to render the "soil" as a box or wall under the terrain, but I would need the top of the box or wall to confirm to the terrain geometry (red line).
I figure I can achieve this by using a WallGeometry around the culled terrain instead of a box, and set the height of each section based on the result of a sampleTerrain call.
But I was wondering if Cesium doesn't provide an easier and cleaner way to do this. (Like perhaps some Boolean union function or something)
Cesium Sandcastle link
var viewer = new Cesium.Viewer('cesiumContainer', {
skyAtmosphere: false,
shouldAnimate : true,
terrainProvider: Cesium.createWorldTerrain()
});
var globe = viewer.scene.globe;
var position = Cesium.Cartographic.toCartesian(new Cesium.Cartographic.fromDegrees(-113.2665534, 36.0939345, 100));
var distance = 3000.0;
globe.clippingPlanes = new Cesium.ClippingPlaneCollection({
modelMatrix : Cesium.Transforms.eastNorthUpToFixedFrame(position),
planes : [
new Cesium.ClippingPlane(new Cesium.Cartesian3( 1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3(-1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, 1.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, -1.0, 0.0), distance)
],
unionClippingRegions : true,
edgeWidth:3,
edgeColor: Cesium.Color.RED,
enabled : true
});
var rockBox = viewer.entities.add({
name : 'RockBox',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 900),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2,800.0),
material : Cesium.Color.GRAY.withAlpha(0.4),
outline : true,
outlineColor : Cesium.Color.GRAY
}
});
var waterTable = viewer.entities.add({
name : 'WaterTable',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 440),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2, 160.0),
material : Cesium.Color.BLUE.withAlpha(0.9),
outline : true,
outlineColor : Cesium.Color.BLUE
}
});
viewer.zoomTo(viewer.entities);
javascript cesium
1
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52
add a comment |
I am using the Cesium.Globe clipping planes to extract a square geographic section. But one problem is that terrain back-faces are not rendered which makes it look weird when the user selects a shallow viewing angle.
I thought one way to hide this was to render the "soil" as a box or wall under the terrain, but I would need the top of the box or wall to confirm to the terrain geometry (red line).
I figure I can achieve this by using a WallGeometry around the culled terrain instead of a box, and set the height of each section based on the result of a sampleTerrain call.
But I was wondering if Cesium doesn't provide an easier and cleaner way to do this. (Like perhaps some Boolean union function or something)
Cesium Sandcastle link
var viewer = new Cesium.Viewer('cesiumContainer', {
skyAtmosphere: false,
shouldAnimate : true,
terrainProvider: Cesium.createWorldTerrain()
});
var globe = viewer.scene.globe;
var position = Cesium.Cartographic.toCartesian(new Cesium.Cartographic.fromDegrees(-113.2665534, 36.0939345, 100));
var distance = 3000.0;
globe.clippingPlanes = new Cesium.ClippingPlaneCollection({
modelMatrix : Cesium.Transforms.eastNorthUpToFixedFrame(position),
planes : [
new Cesium.ClippingPlane(new Cesium.Cartesian3( 1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3(-1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, 1.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, -1.0, 0.0), distance)
],
unionClippingRegions : true,
edgeWidth:3,
edgeColor: Cesium.Color.RED,
enabled : true
});
var rockBox = viewer.entities.add({
name : 'RockBox',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 900),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2,800.0),
material : Cesium.Color.GRAY.withAlpha(0.4),
outline : true,
outlineColor : Cesium.Color.GRAY
}
});
var waterTable = viewer.entities.add({
name : 'WaterTable',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 440),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2, 160.0),
material : Cesium.Color.BLUE.withAlpha(0.9),
outline : true,
outlineColor : Cesium.Color.BLUE
}
});
viewer.zoomTo(viewer.entities);
javascript cesium
I am using the Cesium.Globe clipping planes to extract a square geographic section. But one problem is that terrain back-faces are not rendered which makes it look weird when the user selects a shallow viewing angle.
I thought one way to hide this was to render the "soil" as a box or wall under the terrain, but I would need the top of the box or wall to confirm to the terrain geometry (red line).
I figure I can achieve this by using a WallGeometry around the culled terrain instead of a box, and set the height of each section based on the result of a sampleTerrain call.
But I was wondering if Cesium doesn't provide an easier and cleaner way to do this. (Like perhaps some Boolean union function or something)
Cesium Sandcastle link
var viewer = new Cesium.Viewer('cesiumContainer', {
skyAtmosphere: false,
shouldAnimate : true,
terrainProvider: Cesium.createWorldTerrain()
});
var globe = viewer.scene.globe;
var position = Cesium.Cartographic.toCartesian(new Cesium.Cartographic.fromDegrees(-113.2665534, 36.0939345, 100));
var distance = 3000.0;
globe.clippingPlanes = new Cesium.ClippingPlaneCollection({
modelMatrix : Cesium.Transforms.eastNorthUpToFixedFrame(position),
planes : [
new Cesium.ClippingPlane(new Cesium.Cartesian3( 1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3(-1.0, 0.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, 1.0, 0.0), distance),
new Cesium.ClippingPlane(new Cesium.Cartesian3( 0.0, -1.0, 0.0), distance)
],
unionClippingRegions : true,
edgeWidth:3,
edgeColor: Cesium.Color.RED,
enabled : true
});
var rockBox = viewer.entities.add({
name : 'RockBox',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 900),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2,800.0),
material : Cesium.Color.GRAY.withAlpha(0.4),
outline : true,
outlineColor : Cesium.Color.GRAY
}
});
var waterTable = viewer.entities.add({
name : 'WaterTable',
position: Cesium.Cartesian3.fromDegrees(-113.2665534, 36.0939345, 440),
box : {
dimensions : new Cesium.Cartesian3(distance*2, distance*2, 160.0),
material : Cesium.Color.BLUE.withAlpha(0.9),
outline : true,
outlineColor : Cesium.Color.BLUE
}
});
viewer.zoomTo(viewer.entities);
javascript cesium
javascript cesium
asked Nov 13 '18 at 8:47


visiblemanvisibleman
1,148719
1,148719
1
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52
add a comment |
1
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52
1
1
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277048%2fhow-do-i-clip-an-entity-or-geometry-against-underside-of-terrain%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277048%2fhow-do-i-clip-an-entity-or-geometry-against-underside-of-terrain%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
b,NGTUm4rH5,NnLZywiI j2sWf1qizfqACtk
1
Unfortunately, I don't think there's an easy way to do this with the public API, but this does look like a good use case. This isn't documented but you can set a custom renderstate for most objects. For terrain you'd have to modify this source to set cull to disabled here: github.com/AnalyticalGraphicsInc/cesium/blob/master/Source/… And this is what it looks like: imgur.com/aWIo86B
– Omar Shehata
Nov 14 '18 at 14:01
This might be a good opportunity to open a pull request to maybe just add a boolean property on terrain to set this, since I think that's vastly easier than constructing all this extra geometry just to hide it.
– Omar Shehata
Nov 14 '18 at 14:03
@OmarShehata, thank you that looks really good and for most use cases it would be enough I think. I'm going to have to give this some thought, as I suspect that at a later point I will have to visualize the "soil walls" anyway for soil layer concentrations of various substances. The Ideal solution for me would be if I could use the terrain as a clipping plane against subsurface geometries.
– visibleman
Nov 15 '18 at 0:41
I opened an issue for this, feel free to chime in there with anything I missed github.com/AnalyticalGraphicsInc/cesium/issues/7266 Terrain as clipping plane sounds like an interesting use case. I'm not sure how hard that'd be or if there's a simpler way.
– Omar Shehata
Nov 15 '18 at 14:52