Group by multiple columns on a single MySql stored procedure
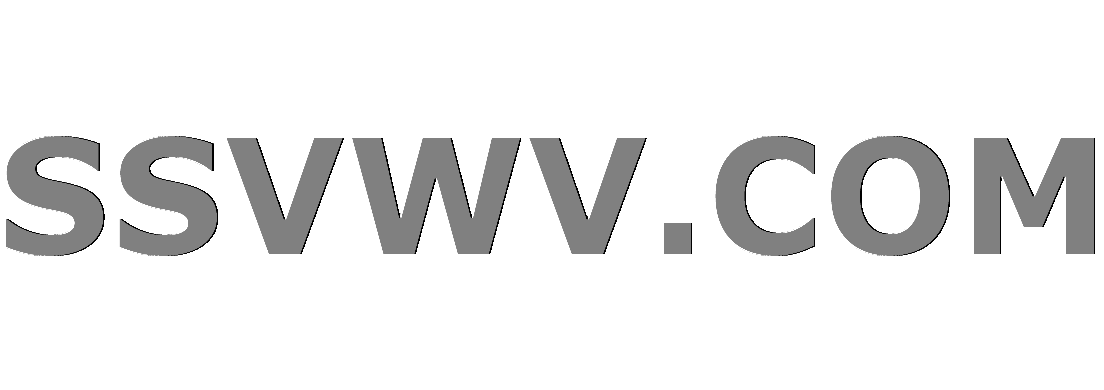
Multi tool use
I have the below stored procedure which I use to show data on multiple ASP chart items.
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT count(agentlogin) AS totalApproved, shift AS Shift, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift, skill;
END
And when I use the above stored procedure to show the data in ASP chart I get the below result
Both the chart are giving the same grouping result. What I want is for the first chart I want it to group by shift and for the second chart I want it to be grouped by skill. Is it possible to achieve this without using separate stored procedure for both? Please let me know. Thanks in advance :)
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("dateValue1", dateValue1);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
Chart2.Series["Series1"].IsValueShownAsLabel = true;
Chart2.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart2.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
Chart2.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart2.Legends.Clear();
Chart2.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart2.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
Chart2.Series["Series1"].Color = Color.DarkOrange;
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
Chart1.Series["Series1"].IsValueShownAsLabel = true;
Chart1.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart1.Series["Series1"].ToolTip = "Skill: #VALX \nCount: #VALY";
Chart1.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart1.Series["Series1"].Color = Color.DarkOrange;
Chart1.Series["Series1"].LabelBackColor = Color.White;
Chart1.Legends.Clear();
Chart1.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart1.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
}
con.Close();
}
mysql stored-procedures
add a comment |
I have the below stored procedure which I use to show data on multiple ASP chart items.
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT count(agentlogin) AS totalApproved, shift AS Shift, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift, skill;
END
And when I use the above stored procedure to show the data in ASP chart I get the below result
Both the chart are giving the same grouping result. What I want is for the first chart I want it to group by shift and for the second chart I want it to be grouped by skill. Is it possible to achieve this without using separate stored procedure for both? Please let me know. Thanks in advance :)
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("dateValue1", dateValue1);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
Chart2.Series["Series1"].IsValueShownAsLabel = true;
Chart2.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart2.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
Chart2.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart2.Legends.Clear();
Chart2.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart2.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
Chart2.Series["Series1"].Color = Color.DarkOrange;
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
Chart1.Series["Series1"].IsValueShownAsLabel = true;
Chart1.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart1.Series["Series1"].ToolTip = "Skill: #VALX \nCount: #VALY";
Chart1.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart1.Series["Series1"].Color = Color.DarkOrange;
Chart1.Series["Series1"].LabelBackColor = Color.White;
Chart1.Legends.Clear();
Chart1.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart1.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
}
con.Close();
}
mysql stored-procedures
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02
add a comment |
I have the below stored procedure which I use to show data on multiple ASP chart items.
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT count(agentlogin) AS totalApproved, shift AS Shift, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift, skill;
END
And when I use the above stored procedure to show the data in ASP chart I get the below result
Both the chart are giving the same grouping result. What I want is for the first chart I want it to group by shift and for the second chart I want it to be grouped by skill. Is it possible to achieve this without using separate stored procedure for both? Please let me know. Thanks in advance :)
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("dateValue1", dateValue1);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
Chart2.Series["Series1"].IsValueShownAsLabel = true;
Chart2.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart2.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
Chart2.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart2.Legends.Clear();
Chart2.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart2.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
Chart2.Series["Series1"].Color = Color.DarkOrange;
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
Chart1.Series["Series1"].IsValueShownAsLabel = true;
Chart1.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart1.Series["Series1"].ToolTip = "Skill: #VALX \nCount: #VALY";
Chart1.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart1.Series["Series1"].Color = Color.DarkOrange;
Chart1.Series["Series1"].LabelBackColor = Color.White;
Chart1.Legends.Clear();
Chart1.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart1.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
}
con.Close();
}
mysql stored-procedures
I have the below stored procedure which I use to show data on multiple ASP chart items.
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT count(agentlogin) AS totalApproved, shift AS Shift, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift, skill;
END
And when I use the above stored procedure to show the data in ASP chart I get the below result
Both the chart are giving the same grouping result. What I want is for the first chart I want it to group by shift and for the second chart I want it to be grouped by skill. Is it possible to achieve this without using separate stored procedure for both? Please let me know. Thanks in advance :)
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("dateValue1", dateValue1);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
Chart2.Series["Series1"].IsValueShownAsLabel = true;
Chart2.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart2.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
Chart2.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart2.Legends.Clear();
Chart2.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart2.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
Chart2.Series["Series1"].Color = Color.DarkOrange;
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
Chart1.Series["Series1"].IsValueShownAsLabel = true;
Chart1.Series["Series1"].Label = "#VALY(#PERCENT)";
Chart1.Series["Series1"].ToolTip = "Skill: #VALX \nCount: #VALY";
Chart1.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
Chart1.Series["Series1"].Color = Color.DarkOrange;
Chart1.Series["Series1"].LabelBackColor = Color.White;
Chart1.Legends.Clear();
Chart1.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
Chart1.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
}
con.Close();
}
mysql stored-procedures
mysql stored-procedures
edited Nov 13 '18 at 8:43
prkash
asked Nov 1 '18 at 7:48
prkashprkash
314113
314113
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02
add a comment |
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02
add a comment |
3 Answers
3
active
oldest
votes
One way around your problem would be to do both queries inside your procedure (one to GROUP BY
shift, and one to GROUP BY
skill, and use a flag in the results to indicate if the result was data grouped by shift or skill:
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT 'skill' AS type, count(agentlogin) AS totalApproved, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by skill;
SELECT 'shift' AS type, count(agentlogin) AS totalApproved, shift AS Shift, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift;
END
Then in your c# code you would change these lines:
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
to:
if (myread["Type"] == "shift") {
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
}
if (myread["Type"] == "skill") {
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
}
add a comment |
Yes, it is possible using a Dynamic SQL. We can create a query string, and then prepare and execute it. This will allow us to specify dynamic column name, which is not possible directly.
You will also need to use one more parameter to specify which column to use in Group By
DELIMITER $$
CREATE DEFINER=`root`@`localhost`
PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100),
in shiftValue varchar(100),
in tmValue varchar(45),
in grpmValue varchar(45),
in dateValue date,
in dateValue1 date,
in groupByColumn varchar(64))
-- add extra in parameter, groupByColumn, to specify which column to group upon
BEGIN
SET query_str = CONCAT('SELECT
count(agentlogin) AS totalApproved,
shift AS Shift,
skill AS Skill,
tm AS TM,
grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
GROUP BY ',
groupByColumn); -- concatenate the group by column param
-- prepare the query
PREPARE stmt FROM query_str;
-- execute the query
EXECUTE stmt;
-- Clear up
DEALLOCATE PREPARE stmt;
END $$
DELIMITER ;
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
|
show 1 more comment
If you just want to reduse c# code size than you can use the stored procedure provided by Madhu Bhaiya with the code bellow to achieve desired result:
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
Dictionary<string, TypeOfChartValue> charts = new Dictionary<string, Chart>()
{
{ "shift", this.Chart2 }, //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart2 must be filled with data grouped by 'shift'
{ "skill", this.Chart1 } //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart1 must be filled with data grouped by 'skill'
//PTK: you can add here as many charts as you wish
};
foreach (string groupby in charts.Keys)
{
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
TypeOfChartValue chart = charts[groupby];
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("groupByColumn", groupby);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
chart.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
chart.Series["Series1"].IsValueShownAsLabel = true;
chart.Series["Series1"].Label = "#VALY(#PERCENT)";
chart.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
chart.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
chart.Legends.Clear();
chart.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
chart.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
chart.Series["Series1"].Color = Color.DarkOrange;
}
con.Close();
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53097099%2fgroup-by-multiple-columns-on-a-single-mysql-stored-procedure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
One way around your problem would be to do both queries inside your procedure (one to GROUP BY
shift, and one to GROUP BY
skill, and use a flag in the results to indicate if the result was data grouped by shift or skill:
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT 'skill' AS type, count(agentlogin) AS totalApproved, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by skill;
SELECT 'shift' AS type, count(agentlogin) AS totalApproved, shift AS Shift, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift;
END
Then in your c# code you would change these lines:
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
to:
if (myread["Type"] == "shift") {
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
}
if (myread["Type"] == "skill") {
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
}
add a comment |
One way around your problem would be to do both queries inside your procedure (one to GROUP BY
shift, and one to GROUP BY
skill, and use a flag in the results to indicate if the result was data grouped by shift or skill:
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT 'skill' AS type, count(agentlogin) AS totalApproved, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by skill;
SELECT 'shift' AS type, count(agentlogin) AS totalApproved, shift AS Shift, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift;
END
Then in your c# code you would change these lines:
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
to:
if (myread["Type"] == "shift") {
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
}
if (myread["Type"] == "skill") {
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
}
add a comment |
One way around your problem would be to do both queries inside your procedure (one to GROUP BY
shift, and one to GROUP BY
skill, and use a flag in the results to indicate if the result was data grouped by shift or skill:
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT 'skill' AS type, count(agentlogin) AS totalApproved, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by skill;
SELECT 'shift' AS type, count(agentlogin) AS totalApproved, shift AS Shift, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift;
END
Then in your c# code you would change these lines:
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
to:
if (myread["Type"] == "shift") {
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
}
if (myread["Type"] == "skill") {
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
}
One way around your problem would be to do both queries inside your procedure (one to GROUP BY
shift, and one to GROUP BY
skill, and use a flag in the results to indicate if the result was data grouped by shift or skill:
CREATE DEFINER=`root`@`localhost` PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100), in shiftValue varchar(100), in tmValue varchar(45),
in grpmValue varchar(45), in dateValue date, in dateValue1 date)
BEGIN
SELECT 'skill' AS type, count(agentlogin) AS totalApproved, skill AS Skill, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by skill;
SELECT 'shift' AS type, count(agentlogin) AS totalApproved, shift AS Shift, tm AS TM, grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
group by shift;
END
Then in your c# code you would change these lines:
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
to:
if (myread["Type"] == "shift") {
this.Chart2.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
}
if (myread["Type"] == "skill") {
this.Chart1.Series["Series1"].Points.AddXY(myread["Skill"], myread["totalApproved"]);
}
answered Nov 20 '18 at 0:39
NickNick
24.7k91735
24.7k91735
add a comment |
add a comment |
Yes, it is possible using a Dynamic SQL. We can create a query string, and then prepare and execute it. This will allow us to specify dynamic column name, which is not possible directly.
You will also need to use one more parameter to specify which column to use in Group By
DELIMITER $$
CREATE DEFINER=`root`@`localhost`
PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100),
in shiftValue varchar(100),
in tmValue varchar(45),
in grpmValue varchar(45),
in dateValue date,
in dateValue1 date,
in groupByColumn varchar(64))
-- add extra in parameter, groupByColumn, to specify which column to group upon
BEGIN
SET query_str = CONCAT('SELECT
count(agentlogin) AS totalApproved,
shift AS Shift,
skill AS Skill,
tm AS TM,
grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
GROUP BY ',
groupByColumn); -- concatenate the group by column param
-- prepare the query
PREPARE stmt FROM query_str;
-- execute the query
EXECUTE stmt;
-- Clear up
DEALLOCATE PREPARE stmt;
END $$
DELIMITER ;
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
|
show 1 more comment
Yes, it is possible using a Dynamic SQL. We can create a query string, and then prepare and execute it. This will allow us to specify dynamic column name, which is not possible directly.
You will also need to use one more parameter to specify which column to use in Group By
DELIMITER $$
CREATE DEFINER=`root`@`localhost`
PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100),
in shiftValue varchar(100),
in tmValue varchar(45),
in grpmValue varchar(45),
in dateValue date,
in dateValue1 date,
in groupByColumn varchar(64))
-- add extra in parameter, groupByColumn, to specify which column to group upon
BEGIN
SET query_str = CONCAT('SELECT
count(agentlogin) AS totalApproved,
shift AS Shift,
skill AS Skill,
tm AS TM,
grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
GROUP BY ',
groupByColumn); -- concatenate the group by column param
-- prepare the query
PREPARE stmt FROM query_str;
-- execute the query
EXECUTE stmt;
-- Clear up
DEALLOCATE PREPARE stmt;
END $$
DELIMITER ;
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
|
show 1 more comment
Yes, it is possible using a Dynamic SQL. We can create a query string, and then prepare and execute it. This will allow us to specify dynamic column name, which is not possible directly.
You will also need to use one more parameter to specify which column to use in Group By
DELIMITER $$
CREATE DEFINER=`root`@`localhost`
PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100),
in shiftValue varchar(100),
in tmValue varchar(45),
in grpmValue varchar(45),
in dateValue date,
in dateValue1 date,
in groupByColumn varchar(64))
-- add extra in parameter, groupByColumn, to specify which column to group upon
BEGIN
SET query_str = CONCAT('SELECT
count(agentlogin) AS totalApproved,
shift AS Shift,
skill AS Skill,
tm AS TM,
grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
GROUP BY ',
groupByColumn); -- concatenate the group by column param
-- prepare the query
PREPARE stmt FROM query_str;
-- execute the query
EXECUTE stmt;
-- Clear up
DEALLOCATE PREPARE stmt;
END $$
DELIMITER ;
Yes, it is possible using a Dynamic SQL. We can create a query string, and then prepare and execute it. This will allow us to specify dynamic column name, which is not possible directly.
You will also need to use one more parameter to specify which column to use in Group By
DELIMITER $$
CREATE DEFINER=`root`@`localhost`
PROCEDURE `GetChartApprovedData`(in siteValue varchar(45),
in skillValue varchar(100),
in shiftValue varchar(100),
in tmValue varchar(45),
in grpmValue varchar(45),
in dateValue date,
in dateValue1 date,
in groupByColumn varchar(64))
-- add extra in parameter, groupByColumn, to specify which column to group upon
BEGIN
SET query_str = CONCAT('SELECT
count(agentlogin) AS totalApproved,
shift AS Shift,
skill AS Skill,
tm AS TM,
grpM AS GrpM
FROM approved
WHERE (sitevalue IS NULL
OR site = sitevalue)
AND (skillvalue IS NULL
OR skill = skillvalue)
AND (shiftvalue IS NULL
OR shift = shiftvalue)
AND (tmValue IS NULL
OR tm = tmValue)
AND (grpmValue IS NULL
OR grpM = grpmValue)
AND (dateValue IS NULL
OR date BETWEEN dateValue AND dateValue1)
GROUP BY ',
groupByColumn); -- concatenate the group by column param
-- prepare the query
PREPARE stmt FROM query_str;
-- execute the query
EXECUTE stmt;
-- Clear up
DEALLOCATE PREPARE stmt;
END $$
DELIMITER ;
answered Nov 1 '18 at 19:40


Madhur BhaiyaMadhur Bhaiya
19.5k62236
19.5k62236
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
|
show 1 more comment
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
How do we get the value for 'groupByColumn'?
– prkash
Nov 12 '18 at 14:59
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
@prkash You pass it as a parameter to your stored procedure.
– Madhur Bhaiya
Nov 12 '18 at 15:12
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
Then I will have to create separate mysql commands and pass parameters individually for each charts, it will be too many lines of codes.
– prkash
Nov 13 '18 at 6:50
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
@prkash what all parameters are there ?
– Madhur Bhaiya
Nov 13 '18 at 7:04
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
I've just added the c# code to the question. So in myread if it reads skill column I want it to be grouped by skill and if it reads shift i want it grouped by shift column.
– prkash
Nov 13 '18 at 8:44
|
show 1 more comment
If you just want to reduse c# code size than you can use the stored procedure provided by Madhu Bhaiya with the code bellow to achieve desired result:
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
Dictionary<string, TypeOfChartValue> charts = new Dictionary<string, Chart>()
{
{ "shift", this.Chart2 }, //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart2 must be filled with data grouped by 'shift'
{ "skill", this.Chart1 } //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart1 must be filled with data grouped by 'skill'
//PTK: you can add here as many charts as you wish
};
foreach (string groupby in charts.Keys)
{
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
TypeOfChartValue chart = charts[groupby];
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("groupByColumn", groupby);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
chart.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
chart.Series["Series1"].IsValueShownAsLabel = true;
chart.Series["Series1"].Label = "#VALY(#PERCENT)";
chart.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
chart.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
chart.Legends.Clear();
chart.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
chart.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
chart.Series["Series1"].Color = Color.DarkOrange;
}
con.Close();
}
}
add a comment |
If you just want to reduse c# code size than you can use the stored procedure provided by Madhu Bhaiya with the code bellow to achieve desired result:
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
Dictionary<string, TypeOfChartValue> charts = new Dictionary<string, Chart>()
{
{ "shift", this.Chart2 }, //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart2 must be filled with data grouped by 'shift'
{ "skill", this.Chart1 } //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart1 must be filled with data grouped by 'skill'
//PTK: you can add here as many charts as you wish
};
foreach (string groupby in charts.Keys)
{
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
TypeOfChartValue chart = charts[groupby];
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("groupByColumn", groupby);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
chart.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
chart.Series["Series1"].IsValueShownAsLabel = true;
chart.Series["Series1"].Label = "#VALY(#PERCENT)";
chart.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
chart.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
chart.Legends.Clear();
chart.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
chart.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
chart.Series["Series1"].Color = Color.DarkOrange;
}
con.Close();
}
}
add a comment |
If you just want to reduse c# code size than you can use the stored procedure provided by Madhu Bhaiya with the code bellow to achieve desired result:
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
Dictionary<string, TypeOfChartValue> charts = new Dictionary<string, Chart>()
{
{ "shift", this.Chart2 }, //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart2 must be filled with data grouped by 'shift'
{ "skill", this.Chart1 } //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart1 must be filled with data grouped by 'skill'
//PTK: you can add here as many charts as you wish
};
foreach (string groupby in charts.Keys)
{
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
TypeOfChartValue chart = charts[groupby];
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("groupByColumn", groupby);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
chart.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
chart.Series["Series1"].IsValueShownAsLabel = true;
chart.Series["Series1"].Label = "#VALY(#PERCENT)";
chart.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
chart.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
chart.Legends.Clear();
chart.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
chart.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
chart.Series["Series1"].Color = Color.DarkOrange;
}
con.Close();
}
}
If you just want to reduse c# code size than you can use the stored procedure provided by Madhu Bhaiya with the code bellow to achieve desired result:
private void GetChartData()
{
string MyConString = ConfigurationManager.ConnectionStrings["connStr"].ConnectionString;
MySqlConnection con = new MySqlConnection(MyConString);
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
Dictionary<string, TypeOfChartValue> charts = new Dictionary<string, Chart>()
{
{ "shift", this.Chart2 }, //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart2 must be filled with data grouped by 'shift'
{ "skill", this.Chart1 } //PTK: the first value here is a column name to use in group by , the second is the chart to fill with resulted data. this line means that the Chart1 must be filled with data grouped by 'skill'
//PTK: you can add here as many charts as you wish
};
foreach (string groupby in charts.Keys)
{
//PTK: TypeOfChartValue should be change with chart type you are using (I did not know what chart library you are using in your code
TypeOfChartValue chart = charts[groupby];
MySqlCommand cmd = new MySqlCommand("GetChartApprovedData");
cmd.CommandType = CommandType.StoredProcedure;
string siteValue = null;
DateTime? dateValue = null;
DateTime? dateValue1 = null;
if (ddlSite.SelectedValue != null && ddlSite.SelectedValue != "0")
{
siteValue = ddlSite.SelectedValue;
}
if (ViewState["Date"] != null && ViewState["Date"].ToString() != "0")
{
dateValue = DateTime.Parse(ViewState["Date"].ToString());
}
if (ViewState["Date1"] != null && ViewState["Date1"].ToString() != "0")
{
dateValue1 = DateTime.Parse(ViewState["Date1"].ToString());
}
cmd.Parameters.AddWithValue("siteValue", siteValue);
cmd.Parameters.AddWithValue("dateValue", dateValue);
cmd.Parameters.AddWithValue("groupByColumn", groupby);
cmd.Connection = con;
con.Open();
MySqlDataReader myread = cmd.ExecuteReader();
while (myread.Read())
{
chart.Series["Series1"].Points.AddXY(myread["Shift"], myread["totalApproved"]);
chart.Series["Series1"].IsValueShownAsLabel = true;
chart.Series["Series1"].Label = "#VALY(#PERCENT)";
chart.Series["Series1"].ToolTip = "Shift: #VALX \nCount: #VALY";
chart.ChartAreas["ChartArea1"].AxisX.LabelStyle.Interval = 1;
chart.Legends.Clear();
chart.ChartAreas["ChartArea1"].AxisX.MajorGrid.Enabled = false;
chart.ChartAreas["ChartArea1"].AxisY.MajorGrid.Enabled = false;
chart.Series["Series1"].Color = Color.DarkOrange;
}
con.Close();
}
}
answered Nov 18 '18 at 7:44


PatiPati
44748
44748
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53097099%2fgroup-by-multiple-columns-on-a-single-mysql-stored-procedure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Tsrm mu y 0A 4quk YT2oDq grt30s9bbl7M7sh,YyFes EVG2El8Eyo2npTSy2DFMAYJSh3N,YMM,k2Se,4 SI,yxLSwmmgbLJjy,A7IQrWeP9o
Did one of these answers solve your problem? If not, could you provide more information to help answer it? Otherwise, please consider marking the answer which best solved your problem accepted. See stackoverflow.com/help/someone-answers
– Nick
Nov 26 '18 at 22:02