collecting user keyevent input for 2 secs and then clear it
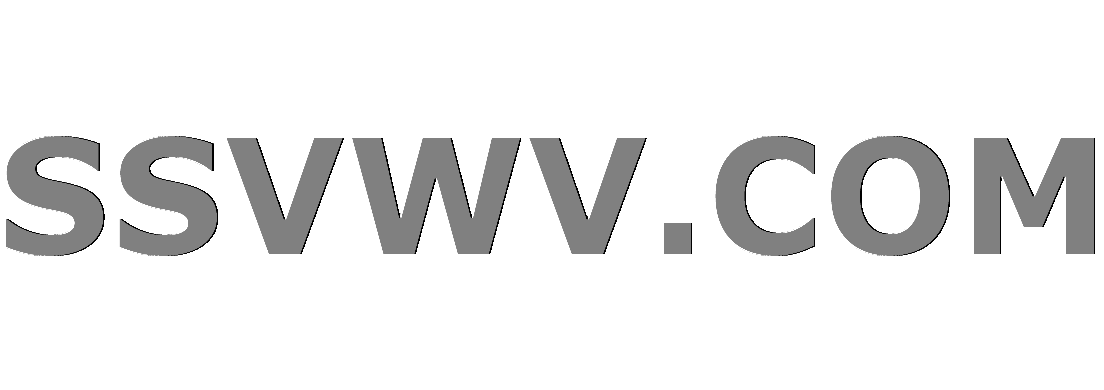
Multi tool use
I have
private userInputTimer;
private userInputText = '';
private handleEvent(event: KeyboardEvent): void {
if((keyNum >= 48 && keyNum <= 90) || (keyNum >= 96 && keyNum <= 105)){
this.userInputText = (this.userInputText) ? this.userInputText : '';
this.userInputText = this.userInputText + keyCode;
clearTimeout(this.userInputTimer);
this.setUserInputClearTimer();
}
setUserInputClearTimer(){
this.userInputTimer = setTimeout(() => {
this.userInputText = '';
}, 500);
}
Using this code, I can delete the text which the user has entered consecutively after 500 ms.
How can I achieve this behavior using RxJs debounce. I would really appreciate some help
angular typescript rxjs
add a comment |
I have
private userInputTimer;
private userInputText = '';
private handleEvent(event: KeyboardEvent): void {
if((keyNum >= 48 && keyNum <= 90) || (keyNum >= 96 && keyNum <= 105)){
this.userInputText = (this.userInputText) ? this.userInputText : '';
this.userInputText = this.userInputText + keyCode;
clearTimeout(this.userInputTimer);
this.setUserInputClearTimer();
}
setUserInputClearTimer(){
this.userInputTimer = setTimeout(() => {
this.userInputText = '';
}, 500);
}
Using this code, I can delete the text which the user has entered consecutively after 500 ms.
How can I achieve this behavior using RxJs debounce. I would really appreciate some help
angular typescript rxjs
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
@SunilSingh Nothing much to show, just(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.
– Samuel
Nov 13 '18 at 9:39
add a comment |
I have
private userInputTimer;
private userInputText = '';
private handleEvent(event: KeyboardEvent): void {
if((keyNum >= 48 && keyNum <= 90) || (keyNum >= 96 && keyNum <= 105)){
this.userInputText = (this.userInputText) ? this.userInputText : '';
this.userInputText = this.userInputText + keyCode;
clearTimeout(this.userInputTimer);
this.setUserInputClearTimer();
}
setUserInputClearTimer(){
this.userInputTimer = setTimeout(() => {
this.userInputText = '';
}, 500);
}
Using this code, I can delete the text which the user has entered consecutively after 500 ms.
How can I achieve this behavior using RxJs debounce. I would really appreciate some help
angular typescript rxjs
I have
private userInputTimer;
private userInputText = '';
private handleEvent(event: KeyboardEvent): void {
if((keyNum >= 48 && keyNum <= 90) || (keyNum >= 96 && keyNum <= 105)){
this.userInputText = (this.userInputText) ? this.userInputText : '';
this.userInputText = this.userInputText + keyCode;
clearTimeout(this.userInputTimer);
this.setUserInputClearTimer();
}
setUserInputClearTimer(){
this.userInputTimer = setTimeout(() => {
this.userInputText = '';
}, 500);
}
Using this code, I can delete the text which the user has entered consecutively after 500 ms.
How can I achieve this behavior using RxJs debounce. I would really appreciate some help
angular typescript rxjs
angular typescript rxjs
edited Nov 13 '18 at 9:02
Samuel
asked Nov 13 '18 at 8:53
SamuelSamuel
1221218
1221218
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
@SunilSingh Nothing much to show, just(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.
– Samuel
Nov 13 '18 at 9:39
add a comment |
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
@SunilSingh Nothing much to show, just(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.
– Samuel
Nov 13 '18 at 9:39
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
@SunilSingh Nothing much to show, just
(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.– Samuel
Nov 13 '18 at 9:39
@SunilSingh Nothing much to show, just
(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.– Samuel
Nov 13 '18 at 9:39
add a comment |
2 Answers
2
active
oldest
votes
You can achieve this with reactive form input control valuechanges().
Template:
<form [formGroup]="formGrp">
<input type="text" formControlName="userInputText" name="username"/>
</form>
Component:
this.formGrp.get('userInputText').valueChanges().pipe(
debouneTime(2000),
map((_enteredText)=>{
const enteredText = _enteredText;
this.formGrp.get('userInputText').setValue('');
return enteredText;
})
).subscribe(value=> console.log(value));
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
add a comment |
To achieve this you need to use -
fromEvent
which will capture the event of targeted element. debounceTime
debounceTime
to delay the events
Here is the snippet
Observable.fromEvent(this.targetElement, 'keyup') //<-- put the event you want to capture
// get value
.map((evt: any) => evt.target.value) //<-- you can ignore this if you don't want the value from the control
.debounceTime(2000)
.subscribe((text: string) => {
this.setUserInputClearTimer();
});
Here is example you can refer to https://stackblitz.com/edit/angular-rxjs-observable-form-input-debounce-kebtnt
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277120%2fcollecting-user-keyevent-input-for-2-secs-and-then-clear-it%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can achieve this with reactive form input control valuechanges().
Template:
<form [formGroup]="formGrp">
<input type="text" formControlName="userInputText" name="username"/>
</form>
Component:
this.formGrp.get('userInputText').valueChanges().pipe(
debouneTime(2000),
map((_enteredText)=>{
const enteredText = _enteredText;
this.formGrp.get('userInputText').setValue('');
return enteredText;
})
).subscribe(value=> console.log(value));
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
add a comment |
You can achieve this with reactive form input control valuechanges().
Template:
<form [formGroup]="formGrp">
<input type="text" formControlName="userInputText" name="username"/>
</form>
Component:
this.formGrp.get('userInputText').valueChanges().pipe(
debouneTime(2000),
map((_enteredText)=>{
const enteredText = _enteredText;
this.formGrp.get('userInputText').setValue('');
return enteredText;
})
).subscribe(value=> console.log(value));
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
add a comment |
You can achieve this with reactive form input control valuechanges().
Template:
<form [formGroup]="formGrp">
<input type="text" formControlName="userInputText" name="username"/>
</form>
Component:
this.formGrp.get('userInputText').valueChanges().pipe(
debouneTime(2000),
map((_enteredText)=>{
const enteredText = _enteredText;
this.formGrp.get('userInputText').setValue('');
return enteredText;
})
).subscribe(value=> console.log(value));
You can achieve this with reactive form input control valuechanges().
Template:
<form [formGroup]="formGrp">
<input type="text" formControlName="userInputText" name="username"/>
</form>
Component:
this.formGrp.get('userInputText').valueChanges().pipe(
debouneTime(2000),
map((_enteredText)=>{
const enteredText = _enteredText;
this.formGrp.get('userInputText').setValue('');
return enteredText;
})
).subscribe(value=> console.log(value));
answered Nov 13 '18 at 9:06


Suresh Kumar AriyaSuresh Kumar Ariya
4,4331215
4,4331215
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
add a comment |
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
No no, i dont want form. I want to monitor this event on my own custom component so that i can take some actions accordingly..
– Samuel
Nov 13 '18 at 9:21
add a comment |
To achieve this you need to use -
fromEvent
which will capture the event of targeted element. debounceTime
debounceTime
to delay the events
Here is the snippet
Observable.fromEvent(this.targetElement, 'keyup') //<-- put the event you want to capture
// get value
.map((evt: any) => evt.target.value) //<-- you can ignore this if you don't want the value from the control
.debounceTime(2000)
.subscribe((text: string) => {
this.setUserInputClearTimer();
});
Here is example you can refer to https://stackblitz.com/edit/angular-rxjs-observable-form-input-debounce-kebtnt
add a comment |
To achieve this you need to use -
fromEvent
which will capture the event of targeted element. debounceTime
debounceTime
to delay the events
Here is the snippet
Observable.fromEvent(this.targetElement, 'keyup') //<-- put the event you want to capture
// get value
.map((evt: any) => evt.target.value) //<-- you can ignore this if you don't want the value from the control
.debounceTime(2000)
.subscribe((text: string) => {
this.setUserInputClearTimer();
});
Here is example you can refer to https://stackblitz.com/edit/angular-rxjs-observable-form-input-debounce-kebtnt
add a comment |
To achieve this you need to use -
fromEvent
which will capture the event of targeted element. debounceTime
debounceTime
to delay the events
Here is the snippet
Observable.fromEvent(this.targetElement, 'keyup') //<-- put the event you want to capture
// get value
.map((evt: any) => evt.target.value) //<-- you can ignore this if you don't want the value from the control
.debounceTime(2000)
.subscribe((text: string) => {
this.setUserInputClearTimer();
});
Here is example you can refer to https://stackblitz.com/edit/angular-rxjs-observable-form-input-debounce-kebtnt
To achieve this you need to use -
fromEvent
which will capture the event of targeted element. debounceTime
debounceTime
to delay the events
Here is the snippet
Observable.fromEvent(this.targetElement, 'keyup') //<-- put the event you want to capture
// get value
.map((evt: any) => evt.target.value) //<-- you can ignore this if you don't want the value from the control
.debounceTime(2000)
.subscribe((text: string) => {
this.setUserInputClearTimer();
});
Here is example you can refer to https://stackblitz.com/edit/angular-rxjs-observable-form-input-debounce-kebtnt
edited Nov 13 '18 at 10:24
answered Nov 13 '18 at 10:19


Sunil SinghSunil Singh
6,1472626
6,1472626
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277120%2fcollecting-user-keyevent-input-for-2-secs-and-then-clear-it%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CPH,35FS63P17bn3IY,TzK70fd
Share your html code as well.
– Sunil Singh
Nov 13 '18 at 9:05
@SunilSingh Nothing much to show, just
(keydown)=handleEvent($event)
. I want to monitor this event on my own custom component so that i can take some actions accordingly.– Samuel
Nov 13 '18 at 9:39