Ignore folders/files when Directory.GetFiles() is denied access
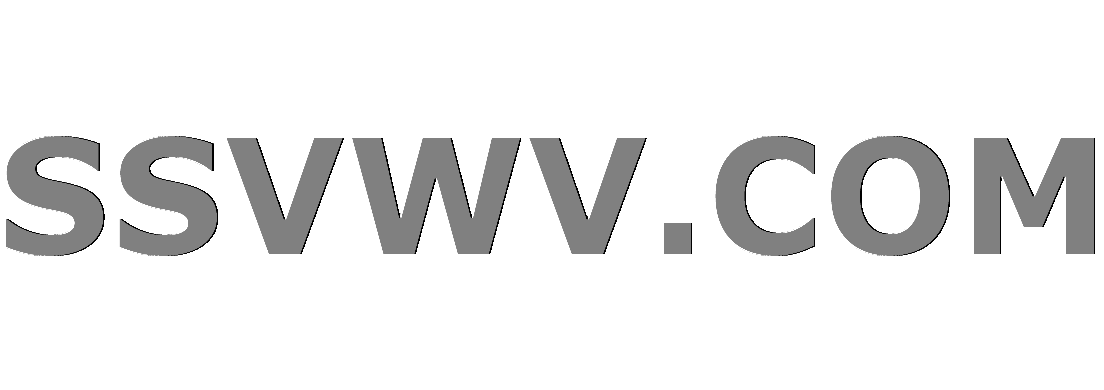
Multi tool use
I am trying to display a list of all files found in the selected directory (and optionally any subdirectories). The problem I am having is that when the GetFiles() method comes across a folder that it cannot access, it throws an exception and the process stops.
How do I ignore this exception (and ignore the protected folder/file) and continue adding accessible files to the list?
try
{
if (cbSubFolders.Checked == false)
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath);
foreach (string fileName in files)
ProcessFile(fileName);
}
else
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath, "*.*", SearchOption.AllDirectories);
foreach (string fileName in files)
ProcessFile(fileName);
}
lblNumberOfFilesDisplay.Enabled = true;
}
catch (UnauthorizedAccessException) { }
finally {}
c# getfiles
add a comment |
I am trying to display a list of all files found in the selected directory (and optionally any subdirectories). The problem I am having is that when the GetFiles() method comes across a folder that it cannot access, it throws an exception and the process stops.
How do I ignore this exception (and ignore the protected folder/file) and continue adding accessible files to the list?
try
{
if (cbSubFolders.Checked == false)
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath);
foreach (string fileName in files)
ProcessFile(fileName);
}
else
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath, "*.*", SearchOption.AllDirectories);
foreach (string fileName in files)
ProcessFile(fileName);
}
lblNumberOfFilesDisplay.Enabled = true;
}
catch (UnauthorizedAccessException) { }
finally {}
c# getfiles
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30
add a comment |
I am trying to display a list of all files found in the selected directory (and optionally any subdirectories). The problem I am having is that when the GetFiles() method comes across a folder that it cannot access, it throws an exception and the process stops.
How do I ignore this exception (and ignore the protected folder/file) and continue adding accessible files to the list?
try
{
if (cbSubFolders.Checked == false)
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath);
foreach (string fileName in files)
ProcessFile(fileName);
}
else
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath, "*.*", SearchOption.AllDirectories);
foreach (string fileName in files)
ProcessFile(fileName);
}
lblNumberOfFilesDisplay.Enabled = true;
}
catch (UnauthorizedAccessException) { }
finally {}
c# getfiles
I am trying to display a list of all files found in the selected directory (and optionally any subdirectories). The problem I am having is that when the GetFiles() method comes across a folder that it cannot access, it throws an exception and the process stops.
How do I ignore this exception (and ignore the protected folder/file) and continue adding accessible files to the list?
try
{
if (cbSubFolders.Checked == false)
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath);
foreach (string fileName in files)
ProcessFile(fileName);
}
else
{
string files = Directory.GetFiles(folderBrowserDialog1.SelectedPath, "*.*", SearchOption.AllDirectories);
foreach (string fileName in files)
ProcessFile(fileName);
}
lblNumberOfFilesDisplay.Enabled = true;
}
catch (UnauthorizedAccessException) { }
finally {}
c# getfiles
c# getfiles
edited Sep 3 '13 at 6:09
abatishchev
69.1k69261392
69.1k69261392
asked Oct 5 '08 at 20:00
RowanRowan
1,63421821
1,63421821
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30
add a comment |
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30
add a comment |
8 Answers
8
active
oldest
votes
You will have to do the recursion manually; don't use AllDirectories - look one folder at a time, then try getting the files from sub-dirs. Untested, but something like below (note uses a delegate rather than building an array):
using System;
using System.IO;
static class Program
{
static void Main()
{
string path = ""; // TODO
ApplyAllFiles(path, ProcessFile);
}
static void ProcessFile(string path) {/* ... */}
static void ApplyAllFiles(string folder, Action<string> fileAction)
{
foreach (string file in Directory.GetFiles(folder))
{
fileAction(file);
}
foreach (string subDir in Directory.GetDirectories(folder))
{
try
{
ApplyAllFiles(subDir, fileAction);
}
catch
{
// swallow, log, whatever
}
}
}
}
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
add a comment |
This simple function works well and meets the questions requirements.
private List<string> GetFiles(string path, string pattern)
{
var files = new List<string>();
try
{
files.AddRange(Directory.GetFiles(path, pattern, SearchOption.TopDirectoryOnly));
foreach (var directory in Directory.GetDirectories(path))
files.AddRange(GetFiles(directory, pattern));
}
catch (UnauthorizedAccessException) { }
return files;
}
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
add a comment |
Also check, How to: Iterate Through a Directory Tree.
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
add a comment |
A simple way to do this is by using a List for files and a Queue for directories.
It conserves memory.
If you use a recursive program to do the same task, that could throw OutOfMemory exception.
The output: files added in the List, are organised according to the top to bottom (breadth first) directory tree.
public static List<string> GetAllFilesFromFolder(string root, bool searchSubfolders) {
Queue<string> folders = new Queue<string>();
List<string> files = new List<string>();
folders.Enqueue(root);
while (folders.Count != 0) {
string currentFolder = folders.Dequeue();
try {
string filesInCurrent = System.IO.Directory.GetFiles(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
files.AddRange(filesInCurrent);
}
catch {
// Do Nothing
}
try {
if (searchSubfolders) {
string foldersInCurrent = System.IO.Directory.GetDirectories(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
foreach (string _current in foldersInCurrent) {
folders.Enqueue(_current);
}
}
}
catch {
// Do Nothing
}
}
return files;
}
Steps:
- Enqueue the root in the queue
- In a loop, Dequeue it, Add the files in that directory to the list, and Add the subfolders to the queue.
- Repeat untill the queue is empty.
add a comment |
I know this question is somewhat old, but I had this same problem today and I found the following article that explains a 'folder recursion' solution in detail.
The article acknowledges the flaws of the GetDirectories()
method... :
Unfortunately, this [using the GetDirectories() method] has problems. Key amongst these is that some of
the folders that you attempt to read could be configured so that the
current user may not access them. Rather than ignoring folders to
which you have restricted access, the method throws an
UnauthorizedAccessException. However, we can circumvent this problem
by creating our own recursive folder search code.
... and then introduces the solution in detail:
http://www.blackwasp.co.uk/FolderRecursion.aspx
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
add a comment |
This should answer the question. I've ignored the issue of going through subdirectories, I'm assuming you have that figured out.
Of course, you don't need to have a seperate method for this, but you might find it a useful place to also verify the path is valid, and deal with the other exceptions that you could encounter when calling GetFiles().
Hope this helps.
private string GetFiles(string path)
{
string files = null;
try
{
files = Directory.GetFiles(path);
}
catch (UnauthorizedAccessException)
{
// might be nice to log this, or something ...
}
return files;
}
private void Processor(string path, bool recursive)
{
// leaving the recursive directory navigation out.
string files = this.GetFiles(path);
if (null != files)
{
foreach (string file in files)
{
this.Process(file);
}
}
else
{
// again, might want to do something when you can't access the path?
}
}
add a comment |
see https://stackoverflow.com/a/10728792/89584 for a solution that handles the UnauthorisedAccessException problem.
All the solutions above will miss files and/or directories if any calls to GetFiles() or GetDirectories() are on folders with a mix of permissions.
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
add a comment |
Here's a full-featured, .NET 2.0-compatible implementation.
You can even alter the yielded List
of files to skip over directories in the FileSystemInfo
version!
(Beware null
values!)
public static IEnumerable<KeyValuePair<string, string>> GetFileSystemInfosRecursive(string dir, bool depth_first)
{
foreach (var item in GetFileSystemObjectsRecursive(new DirectoryInfo(dir), depth_first))
{
string result;
var children = item.Value;
if (children != null)
{
result = new string[children.Count];
for (int i = 0; i < result.Length; i++)
{ result[i] = children[i].Name; }
}
else { result = null; }
string fullname;
try { fullname = item.Key.FullName; }
catch (IOException) { fullname = null; }
catch (UnauthorizedAccessException) { fullname = null; }
yield return new KeyValuePair<string, string>(fullname, result);
}
}
public static IEnumerable<KeyValuePair<DirectoryInfo, List<FileSystemInfo>>> GetFileSystemInfosRecursive(DirectoryInfo dir, bool depth_first)
{
var stack = depth_first ? new Stack<DirectoryInfo>() : null;
var queue = depth_first ? null : new Queue<DirectoryInfo>();
if (depth_first) { stack.Push(dir); }
else { queue.Enqueue(dir); }
for (var list = new List<FileSystemInfo>(); (depth_first ? stack.Count : queue.Count) > 0; list.Clear())
{
dir = depth_first ? stack.Pop() : queue.Dequeue();
FileSystemInfo children;
try { children = dir.GetFileSystemInfos(); }
catch (UnauthorizedAccessException) { children = null; }
catch (IOException) { children = null; }
if (children != null) { list.AddRange(children); }
yield return new KeyValuePair<DirectoryInfo, List<FileSystemInfo>>(dir, children != null ? list : null);
if (depth_first) { list.Reverse(); }
foreach (var child in list)
{
var asdir = child as DirectoryInfo;
if (asdir != null)
{
if (depth_first) { stack.Push(asdir); }
else { queue.Enqueue(asdir); }
}
}
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f172544%2fignore-folders-files-when-directory-getfiles-is-denied-access%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
You will have to do the recursion manually; don't use AllDirectories - look one folder at a time, then try getting the files from sub-dirs. Untested, but something like below (note uses a delegate rather than building an array):
using System;
using System.IO;
static class Program
{
static void Main()
{
string path = ""; // TODO
ApplyAllFiles(path, ProcessFile);
}
static void ProcessFile(string path) {/* ... */}
static void ApplyAllFiles(string folder, Action<string> fileAction)
{
foreach (string file in Directory.GetFiles(folder))
{
fileAction(file);
}
foreach (string subDir in Directory.GetDirectories(folder))
{
try
{
ApplyAllFiles(subDir, fileAction);
}
catch
{
// swallow, log, whatever
}
}
}
}
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
add a comment |
You will have to do the recursion manually; don't use AllDirectories - look one folder at a time, then try getting the files from sub-dirs. Untested, but something like below (note uses a delegate rather than building an array):
using System;
using System.IO;
static class Program
{
static void Main()
{
string path = ""; // TODO
ApplyAllFiles(path, ProcessFile);
}
static void ProcessFile(string path) {/* ... */}
static void ApplyAllFiles(string folder, Action<string> fileAction)
{
foreach (string file in Directory.GetFiles(folder))
{
fileAction(file);
}
foreach (string subDir in Directory.GetDirectories(folder))
{
try
{
ApplyAllFiles(subDir, fileAction);
}
catch
{
// swallow, log, whatever
}
}
}
}
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
add a comment |
You will have to do the recursion manually; don't use AllDirectories - look one folder at a time, then try getting the files from sub-dirs. Untested, but something like below (note uses a delegate rather than building an array):
using System;
using System.IO;
static class Program
{
static void Main()
{
string path = ""; // TODO
ApplyAllFiles(path, ProcessFile);
}
static void ProcessFile(string path) {/* ... */}
static void ApplyAllFiles(string folder, Action<string> fileAction)
{
foreach (string file in Directory.GetFiles(folder))
{
fileAction(file);
}
foreach (string subDir in Directory.GetDirectories(folder))
{
try
{
ApplyAllFiles(subDir, fileAction);
}
catch
{
// swallow, log, whatever
}
}
}
}
You will have to do the recursion manually; don't use AllDirectories - look one folder at a time, then try getting the files from sub-dirs. Untested, but something like below (note uses a delegate rather than building an array):
using System;
using System.IO;
static class Program
{
static void Main()
{
string path = ""; // TODO
ApplyAllFiles(path, ProcessFile);
}
static void ProcessFile(string path) {/* ... */}
static void ApplyAllFiles(string folder, Action<string> fileAction)
{
foreach (string file in Directory.GetFiles(folder))
{
fileAction(file);
}
foreach (string subDir in Directory.GetDirectories(folder))
{
try
{
ApplyAllFiles(subDir, fileAction);
}
catch
{
// swallow, log, whatever
}
}
}
}
edited Oct 5 '08 at 20:48
answered Oct 5 '08 at 20:21


Marc Gravell♦Marc Gravell
778k19221282541
778k19221282541
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
add a comment |
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
Too good, I have not found anything like this in VB.NET. Hope you don't mind if I have translated this in VB.NET here
– Steve
Jan 21 '16 at 19:31
4
4
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
still not enough: GetFiles throws internally when only one file is inaccessible in a folder. So the whole folder will be untreated.
– v.oddou
Nov 24 '16 at 8:15
add a comment |
This simple function works well and meets the questions requirements.
private List<string> GetFiles(string path, string pattern)
{
var files = new List<string>();
try
{
files.AddRange(Directory.GetFiles(path, pattern, SearchOption.TopDirectoryOnly));
foreach (var directory in Directory.GetDirectories(path))
files.AddRange(GetFiles(directory, pattern));
}
catch (UnauthorizedAccessException) { }
return files;
}
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
add a comment |
This simple function works well and meets the questions requirements.
private List<string> GetFiles(string path, string pattern)
{
var files = new List<string>();
try
{
files.AddRange(Directory.GetFiles(path, pattern, SearchOption.TopDirectoryOnly));
foreach (var directory in Directory.GetDirectories(path))
files.AddRange(GetFiles(directory, pattern));
}
catch (UnauthorizedAccessException) { }
return files;
}
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
add a comment |
This simple function works well and meets the questions requirements.
private List<string> GetFiles(string path, string pattern)
{
var files = new List<string>();
try
{
files.AddRange(Directory.GetFiles(path, pattern, SearchOption.TopDirectoryOnly));
foreach (var directory in Directory.GetDirectories(path))
files.AddRange(GetFiles(directory, pattern));
}
catch (UnauthorizedAccessException) { }
return files;
}
This simple function works well and meets the questions requirements.
private List<string> GetFiles(string path, string pattern)
{
var files = new List<string>();
try
{
files.AddRange(Directory.GetFiles(path, pattern, SearchOption.TopDirectoryOnly));
foreach (var directory in Directory.GetDirectories(path))
files.AddRange(GetFiles(directory, pattern));
}
catch (UnauthorizedAccessException) { }
return files;
}
answered Jun 26 '14 at 20:58
Ben GripkaBen Gripka
11.4k43435
11.4k43435
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
add a comment |
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
That's the most convienent and transparent way to do it (y)
– derFunk
Apr 24 '15 at 14:15
8
8
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
Unfortunately it stops on first exception...
– Alexei Levenkov
Apr 19 '16 at 22:28
1
1
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
Yes, because of the missing error handling, it is of not much use. Just try to search the whole c: tree. There are a number of areas in Windows file system where the user maybe even with admin rights has not enough access rights. That's what the main challenge is all about here (besides junction points, and such).
– Philm
Feb 23 '17 at 16:54
add a comment |
Also check, How to: Iterate Through a Directory Tree.
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
add a comment |
Also check, How to: Iterate Through a Directory Tree.
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
add a comment |
Also check, How to: Iterate Through a Directory Tree.
Also check, How to: Iterate Through a Directory Tree.
answered May 14 '09 at 17:07
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
add a comment |
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
Quite old, use instead: How to: Enumerate Directories and Files: msdn.microsoft.com/en-us/library/dd997370.aspx
– Philm
Feb 23 '17 at 16:57
add a comment |
A simple way to do this is by using a List for files and a Queue for directories.
It conserves memory.
If you use a recursive program to do the same task, that could throw OutOfMemory exception.
The output: files added in the List, are organised according to the top to bottom (breadth first) directory tree.
public static List<string> GetAllFilesFromFolder(string root, bool searchSubfolders) {
Queue<string> folders = new Queue<string>();
List<string> files = new List<string>();
folders.Enqueue(root);
while (folders.Count != 0) {
string currentFolder = folders.Dequeue();
try {
string filesInCurrent = System.IO.Directory.GetFiles(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
files.AddRange(filesInCurrent);
}
catch {
// Do Nothing
}
try {
if (searchSubfolders) {
string foldersInCurrent = System.IO.Directory.GetDirectories(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
foreach (string _current in foldersInCurrent) {
folders.Enqueue(_current);
}
}
}
catch {
// Do Nothing
}
}
return files;
}
Steps:
- Enqueue the root in the queue
- In a loop, Dequeue it, Add the files in that directory to the list, and Add the subfolders to the queue.
- Repeat untill the queue is empty.
add a comment |
A simple way to do this is by using a List for files and a Queue for directories.
It conserves memory.
If you use a recursive program to do the same task, that could throw OutOfMemory exception.
The output: files added in the List, are organised according to the top to bottom (breadth first) directory tree.
public static List<string> GetAllFilesFromFolder(string root, bool searchSubfolders) {
Queue<string> folders = new Queue<string>();
List<string> files = new List<string>();
folders.Enqueue(root);
while (folders.Count != 0) {
string currentFolder = folders.Dequeue();
try {
string filesInCurrent = System.IO.Directory.GetFiles(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
files.AddRange(filesInCurrent);
}
catch {
// Do Nothing
}
try {
if (searchSubfolders) {
string foldersInCurrent = System.IO.Directory.GetDirectories(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
foreach (string _current in foldersInCurrent) {
folders.Enqueue(_current);
}
}
}
catch {
// Do Nothing
}
}
return files;
}
Steps:
- Enqueue the root in the queue
- In a loop, Dequeue it, Add the files in that directory to the list, and Add the subfolders to the queue.
- Repeat untill the queue is empty.
add a comment |
A simple way to do this is by using a List for files and a Queue for directories.
It conserves memory.
If you use a recursive program to do the same task, that could throw OutOfMemory exception.
The output: files added in the List, are organised according to the top to bottom (breadth first) directory tree.
public static List<string> GetAllFilesFromFolder(string root, bool searchSubfolders) {
Queue<string> folders = new Queue<string>();
List<string> files = new List<string>();
folders.Enqueue(root);
while (folders.Count != 0) {
string currentFolder = folders.Dequeue();
try {
string filesInCurrent = System.IO.Directory.GetFiles(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
files.AddRange(filesInCurrent);
}
catch {
// Do Nothing
}
try {
if (searchSubfolders) {
string foldersInCurrent = System.IO.Directory.GetDirectories(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
foreach (string _current in foldersInCurrent) {
folders.Enqueue(_current);
}
}
}
catch {
// Do Nothing
}
}
return files;
}
Steps:
- Enqueue the root in the queue
- In a loop, Dequeue it, Add the files in that directory to the list, and Add the subfolders to the queue.
- Repeat untill the queue is empty.
A simple way to do this is by using a List for files and a Queue for directories.
It conserves memory.
If you use a recursive program to do the same task, that could throw OutOfMemory exception.
The output: files added in the List, are organised according to the top to bottom (breadth first) directory tree.
public static List<string> GetAllFilesFromFolder(string root, bool searchSubfolders) {
Queue<string> folders = new Queue<string>();
List<string> files = new List<string>();
folders.Enqueue(root);
while (folders.Count != 0) {
string currentFolder = folders.Dequeue();
try {
string filesInCurrent = System.IO.Directory.GetFiles(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
files.AddRange(filesInCurrent);
}
catch {
// Do Nothing
}
try {
if (searchSubfolders) {
string foldersInCurrent = System.IO.Directory.GetDirectories(currentFolder, "*.*", System.IO.SearchOption.TopDirectoryOnly);
foreach (string _current in foldersInCurrent) {
folders.Enqueue(_current);
}
}
}
catch {
// Do Nothing
}
}
return files;
}
Steps:
- Enqueue the root in the queue
- In a loop, Dequeue it, Add the files in that directory to the list, and Add the subfolders to the queue.
- Repeat untill the queue is empty.
answered Aug 15 '16 at 16:40


Shubham KumarShubham Kumar
885
885
add a comment |
add a comment |
I know this question is somewhat old, but I had this same problem today and I found the following article that explains a 'folder recursion' solution in detail.
The article acknowledges the flaws of the GetDirectories()
method... :
Unfortunately, this [using the GetDirectories() method] has problems. Key amongst these is that some of
the folders that you attempt to read could be configured so that the
current user may not access them. Rather than ignoring folders to
which you have restricted access, the method throws an
UnauthorizedAccessException. However, we can circumvent this problem
by creating our own recursive folder search code.
... and then introduces the solution in detail:
http://www.blackwasp.co.uk/FolderRecursion.aspx
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
add a comment |
I know this question is somewhat old, but I had this same problem today and I found the following article that explains a 'folder recursion' solution in detail.
The article acknowledges the flaws of the GetDirectories()
method... :
Unfortunately, this [using the GetDirectories() method] has problems. Key amongst these is that some of
the folders that you attempt to read could be configured so that the
current user may not access them. Rather than ignoring folders to
which you have restricted access, the method throws an
UnauthorizedAccessException. However, we can circumvent this problem
by creating our own recursive folder search code.
... and then introduces the solution in detail:
http://www.blackwasp.co.uk/FolderRecursion.aspx
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
add a comment |
I know this question is somewhat old, but I had this same problem today and I found the following article that explains a 'folder recursion' solution in detail.
The article acknowledges the flaws of the GetDirectories()
method... :
Unfortunately, this [using the GetDirectories() method] has problems. Key amongst these is that some of
the folders that you attempt to read could be configured so that the
current user may not access them. Rather than ignoring folders to
which you have restricted access, the method throws an
UnauthorizedAccessException. However, we can circumvent this problem
by creating our own recursive folder search code.
... and then introduces the solution in detail:
http://www.blackwasp.co.uk/FolderRecursion.aspx
I know this question is somewhat old, but I had this same problem today and I found the following article that explains a 'folder recursion' solution in detail.
The article acknowledges the flaws of the GetDirectories()
method... :
Unfortunately, this [using the GetDirectories() method] has problems. Key amongst these is that some of
the folders that you attempt to read could be configured so that the
current user may not access them. Rather than ignoring folders to
which you have restricted access, the method throws an
UnauthorizedAccessException. However, we can circumvent this problem
by creating our own recursive folder search code.
... and then introduces the solution in detail:
http://www.blackwasp.co.uk/FolderRecursion.aspx
answered Feb 6 '13 at 18:43


sergeidavesergeidave
3924822
3924822
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
add a comment |
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
Easiest to implement in my requirement.
– Rajesh Thampi
Dec 6 '17 at 8:59
add a comment |
This should answer the question. I've ignored the issue of going through subdirectories, I'm assuming you have that figured out.
Of course, you don't need to have a seperate method for this, but you might find it a useful place to also verify the path is valid, and deal with the other exceptions that you could encounter when calling GetFiles().
Hope this helps.
private string GetFiles(string path)
{
string files = null;
try
{
files = Directory.GetFiles(path);
}
catch (UnauthorizedAccessException)
{
// might be nice to log this, or something ...
}
return files;
}
private void Processor(string path, bool recursive)
{
// leaving the recursive directory navigation out.
string files = this.GetFiles(path);
if (null != files)
{
foreach (string file in files)
{
this.Process(file);
}
}
else
{
// again, might want to do something when you can't access the path?
}
}
add a comment |
This should answer the question. I've ignored the issue of going through subdirectories, I'm assuming you have that figured out.
Of course, you don't need to have a seperate method for this, but you might find it a useful place to also verify the path is valid, and deal with the other exceptions that you could encounter when calling GetFiles().
Hope this helps.
private string GetFiles(string path)
{
string files = null;
try
{
files = Directory.GetFiles(path);
}
catch (UnauthorizedAccessException)
{
// might be nice to log this, or something ...
}
return files;
}
private void Processor(string path, bool recursive)
{
// leaving the recursive directory navigation out.
string files = this.GetFiles(path);
if (null != files)
{
foreach (string file in files)
{
this.Process(file);
}
}
else
{
// again, might want to do something when you can't access the path?
}
}
add a comment |
This should answer the question. I've ignored the issue of going through subdirectories, I'm assuming you have that figured out.
Of course, you don't need to have a seperate method for this, but you might find it a useful place to also verify the path is valid, and deal with the other exceptions that you could encounter when calling GetFiles().
Hope this helps.
private string GetFiles(string path)
{
string files = null;
try
{
files = Directory.GetFiles(path);
}
catch (UnauthorizedAccessException)
{
// might be nice to log this, or something ...
}
return files;
}
private void Processor(string path, bool recursive)
{
// leaving the recursive directory navigation out.
string files = this.GetFiles(path);
if (null != files)
{
foreach (string file in files)
{
this.Process(file);
}
}
else
{
// again, might want to do something when you can't access the path?
}
}
This should answer the question. I've ignored the issue of going through subdirectories, I'm assuming you have that figured out.
Of course, you don't need to have a seperate method for this, but you might find it a useful place to also verify the path is valid, and deal with the other exceptions that you could encounter when calling GetFiles().
Hope this helps.
private string GetFiles(string path)
{
string files = null;
try
{
files = Directory.GetFiles(path);
}
catch (UnauthorizedAccessException)
{
// might be nice to log this, or something ...
}
return files;
}
private void Processor(string path, bool recursive)
{
// leaving the recursive directory navigation out.
string files = this.GetFiles(path);
if (null != files)
{
foreach (string file in files)
{
this.Process(file);
}
}
else
{
// again, might want to do something when you can't access the path?
}
}
answered Oct 5 '08 at 20:35
user25306user25306
323247
323247
add a comment |
add a comment |
see https://stackoverflow.com/a/10728792/89584 for a solution that handles the UnauthorisedAccessException problem.
All the solutions above will miss files and/or directories if any calls to GetFiles() or GetDirectories() are on folders with a mix of permissions.
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
add a comment |
see https://stackoverflow.com/a/10728792/89584 for a solution that handles the UnauthorisedAccessException problem.
All the solutions above will miss files and/or directories if any calls to GetFiles() or GetDirectories() are on folders with a mix of permissions.
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
add a comment |
see https://stackoverflow.com/a/10728792/89584 for a solution that handles the UnauthorisedAccessException problem.
All the solutions above will miss files and/or directories if any calls to GetFiles() or GetDirectories() are on folders with a mix of permissions.
see https://stackoverflow.com/a/10728792/89584 for a solution that handles the UnauthorisedAccessException problem.
All the solutions above will miss files and/or directories if any calls to GetFiles() or GetDirectories() are on folders with a mix of permissions.
edited May 23 '17 at 12:26
Community♦
11
11
answered May 24 '12 at 13:54
MalcolmMalcolm
920917
920917
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
add a comment |
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
1
1
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
all solutions involving GetFiles/GetDirectories are bound to have the same problem, and thus are a bit inelegant
– hello_earth
Dec 30 '14 at 9:54
add a comment |
Here's a full-featured, .NET 2.0-compatible implementation.
You can even alter the yielded List
of files to skip over directories in the FileSystemInfo
version!
(Beware null
values!)
public static IEnumerable<KeyValuePair<string, string>> GetFileSystemInfosRecursive(string dir, bool depth_first)
{
foreach (var item in GetFileSystemObjectsRecursive(new DirectoryInfo(dir), depth_first))
{
string result;
var children = item.Value;
if (children != null)
{
result = new string[children.Count];
for (int i = 0; i < result.Length; i++)
{ result[i] = children[i].Name; }
}
else { result = null; }
string fullname;
try { fullname = item.Key.FullName; }
catch (IOException) { fullname = null; }
catch (UnauthorizedAccessException) { fullname = null; }
yield return new KeyValuePair<string, string>(fullname, result);
}
}
public static IEnumerable<KeyValuePair<DirectoryInfo, List<FileSystemInfo>>> GetFileSystemInfosRecursive(DirectoryInfo dir, bool depth_first)
{
var stack = depth_first ? new Stack<DirectoryInfo>() : null;
var queue = depth_first ? null : new Queue<DirectoryInfo>();
if (depth_first) { stack.Push(dir); }
else { queue.Enqueue(dir); }
for (var list = new List<FileSystemInfo>(); (depth_first ? stack.Count : queue.Count) > 0; list.Clear())
{
dir = depth_first ? stack.Pop() : queue.Dequeue();
FileSystemInfo children;
try { children = dir.GetFileSystemInfos(); }
catch (UnauthorizedAccessException) { children = null; }
catch (IOException) { children = null; }
if (children != null) { list.AddRange(children); }
yield return new KeyValuePair<DirectoryInfo, List<FileSystemInfo>>(dir, children != null ? list : null);
if (depth_first) { list.Reverse(); }
foreach (var child in list)
{
var asdir = child as DirectoryInfo;
if (asdir != null)
{
if (depth_first) { stack.Push(asdir); }
else { queue.Enqueue(asdir); }
}
}
}
}
add a comment |
Here's a full-featured, .NET 2.0-compatible implementation.
You can even alter the yielded List
of files to skip over directories in the FileSystemInfo
version!
(Beware null
values!)
public static IEnumerable<KeyValuePair<string, string>> GetFileSystemInfosRecursive(string dir, bool depth_first)
{
foreach (var item in GetFileSystemObjectsRecursive(new DirectoryInfo(dir), depth_first))
{
string result;
var children = item.Value;
if (children != null)
{
result = new string[children.Count];
for (int i = 0; i < result.Length; i++)
{ result[i] = children[i].Name; }
}
else { result = null; }
string fullname;
try { fullname = item.Key.FullName; }
catch (IOException) { fullname = null; }
catch (UnauthorizedAccessException) { fullname = null; }
yield return new KeyValuePair<string, string>(fullname, result);
}
}
public static IEnumerable<KeyValuePair<DirectoryInfo, List<FileSystemInfo>>> GetFileSystemInfosRecursive(DirectoryInfo dir, bool depth_first)
{
var stack = depth_first ? new Stack<DirectoryInfo>() : null;
var queue = depth_first ? null : new Queue<DirectoryInfo>();
if (depth_first) { stack.Push(dir); }
else { queue.Enqueue(dir); }
for (var list = new List<FileSystemInfo>(); (depth_first ? stack.Count : queue.Count) > 0; list.Clear())
{
dir = depth_first ? stack.Pop() : queue.Dequeue();
FileSystemInfo children;
try { children = dir.GetFileSystemInfos(); }
catch (UnauthorizedAccessException) { children = null; }
catch (IOException) { children = null; }
if (children != null) { list.AddRange(children); }
yield return new KeyValuePair<DirectoryInfo, List<FileSystemInfo>>(dir, children != null ? list : null);
if (depth_first) { list.Reverse(); }
foreach (var child in list)
{
var asdir = child as DirectoryInfo;
if (asdir != null)
{
if (depth_first) { stack.Push(asdir); }
else { queue.Enqueue(asdir); }
}
}
}
}
add a comment |
Here's a full-featured, .NET 2.0-compatible implementation.
You can even alter the yielded List
of files to skip over directories in the FileSystemInfo
version!
(Beware null
values!)
public static IEnumerable<KeyValuePair<string, string>> GetFileSystemInfosRecursive(string dir, bool depth_first)
{
foreach (var item in GetFileSystemObjectsRecursive(new DirectoryInfo(dir), depth_first))
{
string result;
var children = item.Value;
if (children != null)
{
result = new string[children.Count];
for (int i = 0; i < result.Length; i++)
{ result[i] = children[i].Name; }
}
else { result = null; }
string fullname;
try { fullname = item.Key.FullName; }
catch (IOException) { fullname = null; }
catch (UnauthorizedAccessException) { fullname = null; }
yield return new KeyValuePair<string, string>(fullname, result);
}
}
public static IEnumerable<KeyValuePair<DirectoryInfo, List<FileSystemInfo>>> GetFileSystemInfosRecursive(DirectoryInfo dir, bool depth_first)
{
var stack = depth_first ? new Stack<DirectoryInfo>() : null;
var queue = depth_first ? null : new Queue<DirectoryInfo>();
if (depth_first) { stack.Push(dir); }
else { queue.Enqueue(dir); }
for (var list = new List<FileSystemInfo>(); (depth_first ? stack.Count : queue.Count) > 0; list.Clear())
{
dir = depth_first ? stack.Pop() : queue.Dequeue();
FileSystemInfo children;
try { children = dir.GetFileSystemInfos(); }
catch (UnauthorizedAccessException) { children = null; }
catch (IOException) { children = null; }
if (children != null) { list.AddRange(children); }
yield return new KeyValuePair<DirectoryInfo, List<FileSystemInfo>>(dir, children != null ? list : null);
if (depth_first) { list.Reverse(); }
foreach (var child in list)
{
var asdir = child as DirectoryInfo;
if (asdir != null)
{
if (depth_first) { stack.Push(asdir); }
else { queue.Enqueue(asdir); }
}
}
}
}
Here's a full-featured, .NET 2.0-compatible implementation.
You can even alter the yielded List
of files to skip over directories in the FileSystemInfo
version!
(Beware null
values!)
public static IEnumerable<KeyValuePair<string, string>> GetFileSystemInfosRecursive(string dir, bool depth_first)
{
foreach (var item in GetFileSystemObjectsRecursive(new DirectoryInfo(dir), depth_first))
{
string result;
var children = item.Value;
if (children != null)
{
result = new string[children.Count];
for (int i = 0; i < result.Length; i++)
{ result[i] = children[i].Name; }
}
else { result = null; }
string fullname;
try { fullname = item.Key.FullName; }
catch (IOException) { fullname = null; }
catch (UnauthorizedAccessException) { fullname = null; }
yield return new KeyValuePair<string, string>(fullname, result);
}
}
public static IEnumerable<KeyValuePair<DirectoryInfo, List<FileSystemInfo>>> GetFileSystemInfosRecursive(DirectoryInfo dir, bool depth_first)
{
var stack = depth_first ? new Stack<DirectoryInfo>() : null;
var queue = depth_first ? null : new Queue<DirectoryInfo>();
if (depth_first) { stack.Push(dir); }
else { queue.Enqueue(dir); }
for (var list = new List<FileSystemInfo>(); (depth_first ? stack.Count : queue.Count) > 0; list.Clear())
{
dir = depth_first ? stack.Pop() : queue.Dequeue();
FileSystemInfo children;
try { children = dir.GetFileSystemInfos(); }
catch (UnauthorizedAccessException) { children = null; }
catch (IOException) { children = null; }
if (children != null) { list.AddRange(children); }
yield return new KeyValuePair<DirectoryInfo, List<FileSystemInfo>>(dir, children != null ? list : null);
if (depth_first) { list.Reverse(); }
foreach (var child in list)
{
var asdir = child as DirectoryInfo;
if (asdir != null)
{
if (depth_first) { stack.Push(asdir); }
else { queue.Enqueue(asdir); }
}
}
}
}
edited Apr 16 '18 at 6:10
answered Apr 16 '18 at 6:04
MehrdadMehrdad
127k87404749
127k87404749
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f172544%2fignore-folders-files-when-directory-getfiles-is-denied-access%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZJDACO7pA6iM C0zqGxgJBHXdTODoyp6zkxRiN J0jZ hUzec7GCK8Rev3e7kuy9j8phZpQ VllC B5Bq7BaW Wfy SiHC
Another (marked as duplicate) question - stackoverflow.com/questions/1393178/…
– Alexei Levenkov
Apr 19 '16 at 22:30