Define enum as flag with an or
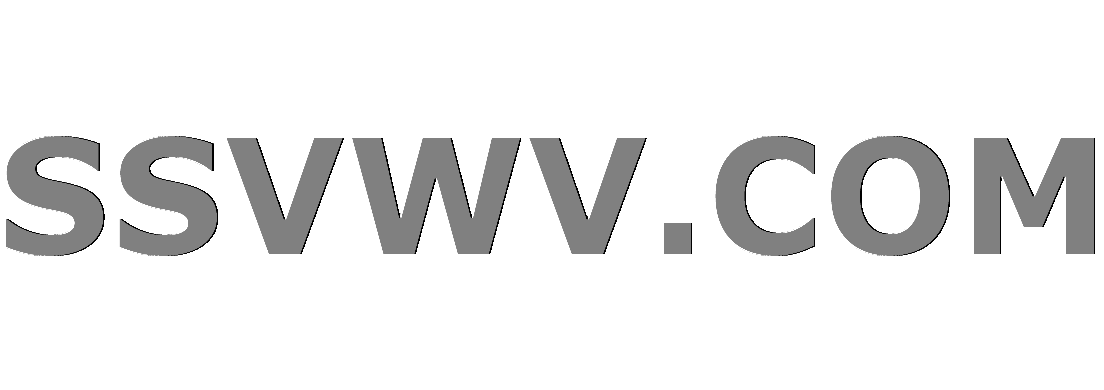
Multi tool use
I'm trying to create an enum as flag. I come from C#, and I want to create an equivalent in C++. I found how to create an enum as a flag in C++, but not how to re-use a member for another member value.
I do not know how to ask my question clearly so here's what I'm trying to do (C# code):
[Flags]
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
}
What I wrote in C++:
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
inline myEnum operator|(myEnum a, myEnum b)
{
return static_cast<myEnum>(static_cast<int>(a) | static_cast<int>(b));
}
But I have an error :
redefinition of 'TypeA '
Edit:
I'm very new to C++ and I forgot the compiler. There is my environment:
- I'm working on windows 10
- with Visual studio 2017
- I compile for android with Clang 3.8 and I target KitKat 4.4
- I'm using C++ 11
Edit2:
I identified the problem. In my namespace I have a class name typeA:
In a .h :
namespace myNameSpace
{
namespace myOtherNameSpace
{
class typeA {...}
}
}
There is a conflict between both. Can I modify my enum to keep myEnum.TypeA
or should I rename this member ?
c++ enums
|
show 13 more comments
I'm trying to create an enum as flag. I come from C#, and I want to create an equivalent in C++. I found how to create an enum as a flag in C++, but not how to re-use a member for another member value.
I do not know how to ask my question clearly so here's what I'm trying to do (C# code):
[Flags]
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
}
What I wrote in C++:
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
inline myEnum operator|(myEnum a, myEnum b)
{
return static_cast<myEnum>(static_cast<int>(a) | static_cast<int>(b));
}
But I have an error :
redefinition of 'TypeA '
Edit:
I'm very new to C++ and I forgot the compiler. There is my environment:
- I'm working on windows 10
- with Visual studio 2017
- I compile for android with Clang 3.8 and I target KitKat 4.4
- I'm using C++ 11
Edit2:
I identified the problem. In my namespace I have a class name typeA:
In a .h :
namespace myNameSpace
{
namespace myOtherNameSpace
{
class typeA {...}
}
}
There is a conflict between both. Can I modify my enum to keep myEnum.TypeA
or should I rename this member ?
c++ enums
1
You don't need to implement a custormoperator|
for yourenum
since it is not a ("strongly typed") scopedenum
.
– Swordfish
Nov 13 '18 at 9:48
2
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
3
@Swordfish - You do if you want to assign the result back tomyEnum
without a cast.
– StoryTeller
Nov 13 '18 at 9:48
1
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So whenTypeAB = TypeA | TypeB
is defined|
is the built-in integer or operator, whilemyEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?
– StoryTeller
Nov 13 '18 at 9:53
1
Use aenum class
if you don't want the enumerators populate the surrounding namespace.
– Swordfish
Nov 13 '18 at 10:15
|
show 13 more comments
I'm trying to create an enum as flag. I come from C#, and I want to create an equivalent in C++. I found how to create an enum as a flag in C++, but not how to re-use a member for another member value.
I do not know how to ask my question clearly so here's what I'm trying to do (C# code):
[Flags]
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
}
What I wrote in C++:
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
inline myEnum operator|(myEnum a, myEnum b)
{
return static_cast<myEnum>(static_cast<int>(a) | static_cast<int>(b));
}
But I have an error :
redefinition of 'TypeA '
Edit:
I'm very new to C++ and I forgot the compiler. There is my environment:
- I'm working on windows 10
- with Visual studio 2017
- I compile for android with Clang 3.8 and I target KitKat 4.4
- I'm using C++ 11
Edit2:
I identified the problem. In my namespace I have a class name typeA:
In a .h :
namespace myNameSpace
{
namespace myOtherNameSpace
{
class typeA {...}
}
}
There is a conflict between both. Can I modify my enum to keep myEnum.TypeA
or should I rename this member ?
c++ enums
I'm trying to create an enum as flag. I come from C#, and I want to create an equivalent in C++. I found how to create an enum as a flag in C++, but not how to re-use a member for another member value.
I do not know how to ask my question clearly so here's what I'm trying to do (C# code):
[Flags]
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
}
What I wrote in C++:
enum myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
inline myEnum operator|(myEnum a, myEnum b)
{
return static_cast<myEnum>(static_cast<int>(a) | static_cast<int>(b));
}
But I have an error :
redefinition of 'TypeA '
Edit:
I'm very new to C++ and I forgot the compiler. There is my environment:
- I'm working on windows 10
- with Visual studio 2017
- I compile for android with Clang 3.8 and I target KitKat 4.4
- I'm using C++ 11
Edit2:
I identified the problem. In my namespace I have a class name typeA:
In a .h :
namespace myNameSpace
{
namespace myOtherNameSpace
{
class typeA {...}
}
}
There is a conflict between both. Can I modify my enum to keep myEnum.TypeA
or should I rename this member ?
c++ enums
c++ enums
edited Nov 13 '18 at 10:36


ctrl-alt-delor
4,15532443
4,15532443
asked Nov 13 '18 at 9:44


A.PissicatA.Pissicat
7131834
7131834
1
You don't need to implement a custormoperator|
for yourenum
since it is not a ("strongly typed") scopedenum
.
– Swordfish
Nov 13 '18 at 9:48
2
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
3
@Swordfish - You do if you want to assign the result back tomyEnum
without a cast.
– StoryTeller
Nov 13 '18 at 9:48
1
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So whenTypeAB = TypeA | TypeB
is defined|
is the built-in integer or operator, whilemyEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?
– StoryTeller
Nov 13 '18 at 9:53
1
Use aenum class
if you don't want the enumerators populate the surrounding namespace.
– Swordfish
Nov 13 '18 at 10:15
|
show 13 more comments
1
You don't need to implement a custormoperator|
for yourenum
since it is not a ("strongly typed") scopedenum
.
– Swordfish
Nov 13 '18 at 9:48
2
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
3
@Swordfish - You do if you want to assign the result back tomyEnum
without a cast.
– StoryTeller
Nov 13 '18 at 9:48
1
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So whenTypeAB = TypeA | TypeB
is defined|
is the built-in integer or operator, whilemyEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?
– StoryTeller
Nov 13 '18 at 9:53
1
Use aenum class
if you don't want the enumerators populate the surrounding namespace.
– Swordfish
Nov 13 '18 at 10:15
1
1
You don't need to implement a custorm
operator|
for your enum
since it is not a ("strongly typed") scoped enum
.– Swordfish
Nov 13 '18 at 9:48
You don't need to implement a custorm
operator|
for your enum
since it is not a ("strongly typed") scoped enum
.– Swordfish
Nov 13 '18 at 9:48
2
2
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
3
3
@Swordfish - You do if you want to assign the result back to
myEnum
without a cast.– StoryTeller
Nov 13 '18 at 9:48
@Swordfish - You do if you want to assign the result back to
myEnum
without a cast.– StoryTeller
Nov 13 '18 at 9:48
1
1
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So when
TypeAB = TypeA | TypeB
is defined |
is the built-in integer or operator, while myEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?– StoryTeller
Nov 13 '18 at 9:53
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So when
TypeAB = TypeA | TypeB
is defined |
is the built-in integer or operator, while myEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?– StoryTeller
Nov 13 '18 at 9:53
1
1
Use a
enum class
if you don't want the enumerators populate the surrounding namespace.– Swordfish
Nov 13 '18 at 10:15
Use a
enum class
if you don't want the enumerators populate the surrounding namespace.– Swordfish
Nov 13 '18 at 10:15
|
show 13 more comments
2 Answers
2
active
oldest
votes
To close this topic, there is the answer found thanks to Swordfish :
Replace enum
by enum class
enum class myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
With simple enum
you can write myEnum e = TypeA
, so if a class is named TypeA
, there is a conflit and you have a compilation error.
With enum class
you must specify the enum myEnum e = myEnum::TypeA
. This can avoid some errors and allow to have a class named TypeA
add a comment |
There is nothing wrong with your code - so maybe a different issue.
However, instead of the casts, etc. you may just specify the underlying enum, so your enum becomes
enum myEnum : int
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
UPDATE: that code may not work on an older compiler such as clang 3.8 - since it's a 'modern' c++ feature.
and is utterly useless withoutoperator|()
if it should be used as flags.
– Swordfish
Nov 13 '18 at 10:04
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53278076%2fdefine-enum-as-flag-with-an-or%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
To close this topic, there is the answer found thanks to Swordfish :
Replace enum
by enum class
enum class myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
With simple enum
you can write myEnum e = TypeA
, so if a class is named TypeA
, there is a conflit and you have a compilation error.
With enum class
you must specify the enum myEnum e = myEnum::TypeA
. This can avoid some errors and allow to have a class named TypeA
add a comment |
To close this topic, there is the answer found thanks to Swordfish :
Replace enum
by enum class
enum class myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
With simple enum
you can write myEnum e = TypeA
, so if a class is named TypeA
, there is a conflit and you have a compilation error.
With enum class
you must specify the enum myEnum e = myEnum::TypeA
. This can avoid some errors and allow to have a class named TypeA
add a comment |
To close this topic, there is the answer found thanks to Swordfish :
Replace enum
by enum class
enum class myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
With simple enum
you can write myEnum e = TypeA
, so if a class is named TypeA
, there is a conflit and you have a compilation error.
With enum class
you must specify the enum myEnum e = myEnum::TypeA
. This can avoid some errors and allow to have a class named TypeA
To close this topic, there is the answer found thanks to Swordfish :
Replace enum
by enum class
enum class myEnum
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
With simple enum
you can write myEnum e = TypeA
, so if a class is named TypeA
, there is a conflit and you have a compilation error.
With enum class
you must specify the enum myEnum e = myEnum::TypeA
. This can avoid some errors and allow to have a class named TypeA
edited Nov 14 '18 at 9:05
answered Nov 14 '18 at 8:55


A.PissicatA.Pissicat
7131834
7131834
add a comment |
add a comment |
There is nothing wrong with your code - so maybe a different issue.
However, instead of the casts, etc. you may just specify the underlying enum, so your enum becomes
enum myEnum : int
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
UPDATE: that code may not work on an older compiler such as clang 3.8 - since it's a 'modern' c++ feature.
and is utterly useless withoutoperator|()
if it should be used as flags.
– Swordfish
Nov 13 '18 at 10:04
add a comment |
There is nothing wrong with your code - so maybe a different issue.
However, instead of the casts, etc. you may just specify the underlying enum, so your enum becomes
enum myEnum : int
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
UPDATE: that code may not work on an older compiler such as clang 3.8 - since it's a 'modern' c++ feature.
and is utterly useless withoutoperator|()
if it should be used as flags.
– Swordfish
Nov 13 '18 at 10:04
add a comment |
There is nothing wrong with your code - so maybe a different issue.
However, instead of the casts, etc. you may just specify the underlying enum, so your enum becomes
enum myEnum : int
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
UPDATE: that code may not work on an older compiler such as clang 3.8 - since it's a 'modern' c++ feature.
There is nothing wrong with your code - so maybe a different issue.
However, instead of the casts, etc. you may just specify the underlying enum, so your enum becomes
enum myEnum : int
{
TypeA = 0x01,
TypeB = 0x02,
TypeC = 0x04,
TypeAB = TypeA | TypeB
};
UPDATE: that code may not work on an older compiler such as clang 3.8 - since it's a 'modern' c++ feature.
edited Nov 13 '18 at 10:38


ctrl-alt-delor
4,15532443
4,15532443
answered Nov 13 '18 at 10:03
darunedarune
1,084516
1,084516
and is utterly useless withoutoperator|()
if it should be used as flags.
– Swordfish
Nov 13 '18 at 10:04
add a comment |
and is utterly useless withoutoperator|()
if it should be used as flags.
– Swordfish
Nov 13 '18 at 10:04
and is utterly useless without
operator|()
if it should be used as flags.– Swordfish
Nov 13 '18 at 10:04
and is utterly useless without
operator|()
if it should be used as flags.– Swordfish
Nov 13 '18 at 10:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53278076%2fdefine-enum-as-flag-with-an-or%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ixc0m49B,ZYm,gX6TX1KiU3w6S8lDWMuiTgEUg,aFckvl5ugfjd4I6hyjUVdU5,wtE,MoTrsK7HcmsOibNGf8ZXtHuksjTuJDVRP
1
You don't need to implement a custorm
operator|
for yourenum
since it is not a ("strongly typed") scopedenum
.– Swordfish
Nov 13 '18 at 9:48
2
Yes, please provide a Minimal, Complete, and Verifiable example. Your code builds fine for me coliru.stacked-crooked.com/a/61d1123fa137fbd2
– StoryTeller
Nov 13 '18 at 9:48
3
@Swordfish - You do if you want to assign the result back to
myEnum
without a cast.– StoryTeller
Nov 13 '18 at 9:48
1
@Swordfish - It's not used in definition of the enumeration. The enumerators are just names of specific values from the underlying type. So when
TypeAB = TypeA | TypeB
is defined|
is the built-in integer or operator, whilemyEnum e = TypeA | TypeB;
would in fact use the overloaded operator (or cause an error if the operator isn't present). Same tokens mean different things in different contexts. Gotta love C++, huh?– StoryTeller
Nov 13 '18 at 9:53
1
Use a
enum class
if you don't want the enumerators populate the surrounding namespace.– Swordfish
Nov 13 '18 at 10:15