Using a service worker to get list of files to cache from server
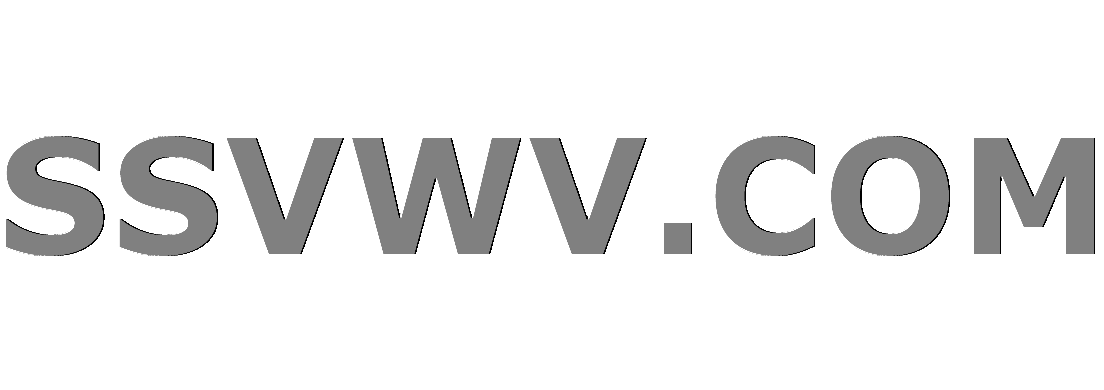
Multi tool use
up vote
0
down vote
favorite
I´m trying to use a service worker in an existing asp mvc app. Generally, it´s working fine: I can cache files and so on. Problem is, that there are many files to be cached and I´m trying to return an array of paths to the service worker, so that the files can be added to cache without adding them manually.
Here´s what I have so far:
Controller:
public ActionResult GetFilesToCache()
{
string filePaths = Directory.GetFiles(Server.MapPath(@"~Content"), "*", SearchOption.AllDirectories);
string cuttedFiles = new string[filePaths.Length];
int i = 0;
foreach (var path in filePaths)
{
cuttedFiles[i] = path.Substring(path.IndexOf("Content"));
i++;
}
return Json(new { filesToCache = cuttedFiles }, JsonRequestBehavior.AllowGet);
}
This gives me a string array with entries like "Contentimage1.png" etc.
Service worker:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
})
);
});
The error I get is:
Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
Calling the action works just fine, data is received by the service worker, but not added to cache.
asp.net-mvc service-worker
add a comment |
up vote
0
down vote
favorite
I´m trying to use a service worker in an existing asp mvc app. Generally, it´s working fine: I can cache files and so on. Problem is, that there are many files to be cached and I´m trying to return an array of paths to the service worker, so that the files can be added to cache without adding them manually.
Here´s what I have so far:
Controller:
public ActionResult GetFilesToCache()
{
string filePaths = Directory.GetFiles(Server.MapPath(@"~Content"), "*", SearchOption.AllDirectories);
string cuttedFiles = new string[filePaths.Length];
int i = 0;
foreach (var path in filePaths)
{
cuttedFiles[i] = path.Substring(path.IndexOf("Content"));
i++;
}
return Json(new { filesToCache = cuttedFiles }, JsonRequestBehavior.AllowGet);
}
This gives me a string array with entries like "Contentimage1.png" etc.
Service worker:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
})
);
});
The error I get is:
Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
Calling the action works just fine, data is received by the service worker, but not added to cache.
asp.net-mvc service-worker
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I´m trying to use a service worker in an existing asp mvc app. Generally, it´s working fine: I can cache files and so on. Problem is, that there are many files to be cached and I´m trying to return an array of paths to the service worker, so that the files can be added to cache without adding them manually.
Here´s what I have so far:
Controller:
public ActionResult GetFilesToCache()
{
string filePaths = Directory.GetFiles(Server.MapPath(@"~Content"), "*", SearchOption.AllDirectories);
string cuttedFiles = new string[filePaths.Length];
int i = 0;
foreach (var path in filePaths)
{
cuttedFiles[i] = path.Substring(path.IndexOf("Content"));
i++;
}
return Json(new { filesToCache = cuttedFiles }, JsonRequestBehavior.AllowGet);
}
This gives me a string array with entries like "Contentimage1.png" etc.
Service worker:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
})
);
});
The error I get is:
Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
Calling the action works just fine, data is received by the service worker, but not added to cache.
asp.net-mvc service-worker
I´m trying to use a service worker in an existing asp mvc app. Generally, it´s working fine: I can cache files and so on. Problem is, that there are many files to be cached and I´m trying to return an array of paths to the service worker, so that the files can be added to cache without adding them manually.
Here´s what I have so far:
Controller:
public ActionResult GetFilesToCache()
{
string filePaths = Directory.GetFiles(Server.MapPath(@"~Content"), "*", SearchOption.AllDirectories);
string cuttedFiles = new string[filePaths.Length];
int i = 0;
foreach (var path in filePaths)
{
cuttedFiles[i] = path.Substring(path.IndexOf("Content"));
i++;
}
return Json(new { filesToCache = cuttedFiles }, JsonRequestBehavior.AllowGet);
}
This gives me a string array with entries like "Contentimage1.png" etc.
Service worker:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
})
);
});
The error I get is:
Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
Calling the action works just fine, data is received by the service worker, but not added to cache.
asp.net-mvc service-worker
asp.net-mvc service-worker
edited Nov 12 at 8:44
asked Nov 8 at 14:23
WhoMightThisOneBe
1479
1479
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
In the following code:
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
the value of the files
parameter is going to be a Response
object. You want it to be the JSON deserialization of the Response
object's body. You can get this by changing return response
with return response.json()
, leading to:
return fetch('Home/GetFilesToCache').then(function(response) {
return response.json();
}).then(function(files) {
return cache.addAll(files);
});
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
add a comment |
up vote
1
down vote
Got it working with this code:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response.json();
}).then(function (files) {
var array = files.filesToCache;
return cache.addAll(array);
});
})
);
});
Notice:
Chrome only lists a part of files stored in cache, so you just have to click that little arrow to show the next page:
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
In the following code:
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
the value of the files
parameter is going to be a Response
object. You want it to be the JSON deserialization of the Response
object's body. You can get this by changing return response
with return response.json()
, leading to:
return fetch('Home/GetFilesToCache').then(function(response) {
return response.json();
}).then(function(files) {
return cache.addAll(files);
});
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
add a comment |
up vote
1
down vote
In the following code:
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
the value of the files
parameter is going to be a Response
object. You want it to be the JSON deserialization of the Response
object's body. You can get this by changing return response
with return response.json()
, leading to:
return fetch('Home/GetFilesToCache').then(function(response) {
return response.json();
}).then(function(files) {
return cache.addAll(files);
});
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
add a comment |
up vote
1
down vote
up vote
1
down vote
In the following code:
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
the value of the files
parameter is going to be a Response
object. You want it to be the JSON deserialization of the Response
object's body. You can get this by changing return response
with return response.json()
, leading to:
return fetch('Home/GetFilesToCache').then(function(response) {
return response.json();
}).then(function(files) {
return cache.addAll(files);
});
In the following code:
return fetch('Home/GetFilesToCache').then(function (response) {
return response;
}).then(function (files) {
return cache.addAll(files);
});
the value of the files
parameter is going to be a Response
object. You want it to be the JSON deserialization of the Response
object's body. You can get this by changing return response
with return response.json()
, leading to:
return fetch('Home/GetFilesToCache').then(function(response) {
return response.json();
}).then(function(files) {
return cache.addAll(files);
});
answered Nov 8 at 17:19
Jeff Posnick
28.4k46091
28.4k46091
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
add a comment |
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Thank you for your answer, but it didn´t work: Uncaught (in promise) TypeError: Failed to execute 'addAll' on 'Cache': Iterator getter is not callable.
– WhoMightThisOneBe
Nov 9 at 5:10
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
Setting a breakpoint at .then(function(files)) revealed that files is an array with 84 elements, which I expected it to be. But I don´t know why that error message comes up.
– WhoMightThisOneBe
Nov 9 at 5:19
add a comment |
up vote
1
down vote
Got it working with this code:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response.json();
}).then(function (files) {
var array = files.filesToCache;
return cache.addAll(array);
});
})
);
});
Notice:
Chrome only lists a part of files stored in cache, so you just have to click that little arrow to show the next page:
add a comment |
up vote
1
down vote
Got it working with this code:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response.json();
}).then(function (files) {
var array = files.filesToCache;
return cache.addAll(array);
});
})
);
});
Notice:
Chrome only lists a part of files stored in cache, so you just have to click that little arrow to show the next page:
add a comment |
up vote
1
down vote
up vote
1
down vote
Got it working with this code:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response.json();
}).then(function (files) {
var array = files.filesToCache;
return cache.addAll(array);
});
})
);
});
Notice:
Chrome only lists a part of files stored in cache, so you just have to click that little arrow to show the next page:
Got it working with this code:
self.addEventListener('install', function(e) {
console.log('[ServiceWorker] Install');
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log('[ServiceWorker] Caching app shell');
return fetch('Home/GetFilesToCache').then(function (response) {
return response.json();
}).then(function (files) {
var array = files.filesToCache;
return cache.addAll(array);
});
})
);
});
Notice:
Chrome only lists a part of files stored in cache, so you just have to click that little arrow to show the next page:
answered Nov 12 at 8:43
WhoMightThisOneBe
1479
1479
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53209698%2fusing-a-service-worker-to-get-list-of-files-to-cache-from-server%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Oi1JeUSygERpIsjfD HBDV,mirxg2ZbheosJmxf4K8IhiFa8,1 gYfJtD QKFAXw5 htWoJHUUEyrUhXTrx1GA GmaH