How to properly implement the call to clone() in a generic class [Java]
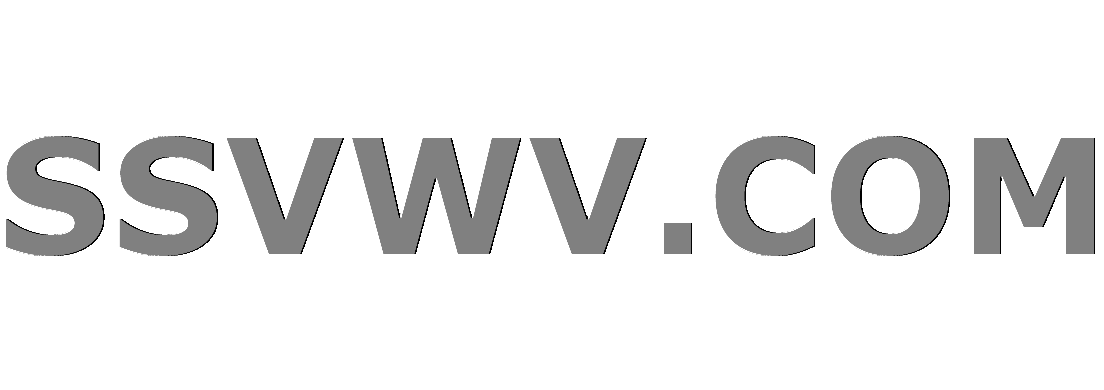
Multi tool use
up vote
2
down vote
favorite
I have a generic interface and I have to create a class to implement it. I thought at first this would work fine, putting it in the documentation that the interface requires a class that implements the clone method
genericClass<E extends Cloneable> implements genericInterface<E extends Cloneable>{
E data;
//code
public E get(){
return this.data.clone()
}
}
However this doesn't work in practice
package securedatacontainer;
public class Demo {
public static void main(String args) throws CloneNotSupportedException {
String s = "user";
A a = new A(5, s);
A b = a.clone();
System.out.println("a equals b: " +(a.equals(b)));
System.out.println("a == b: " + (a == b));
B<A> c = new B<>(a);
System.out.println("a equals c.data: " +(a.equals(c.data)));
System.out.println("a == c.data: " + (a == c.data));
A k = c.get();
System.out.println(k.value);
}
}
class A implements Cloneable{
int value;
String name;
public A(int x, String str ){
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
public boolean equals(A elem){
return (this.name).equals(elem.name) && this.value==elem.value;
}
}
class B <E extends Cloneable>{
E data;
public B(E elem){
this.data=elem;
}
public E get() throws CloneNotSupportedException{
return (E) this.data.clone();
}
}
I get
Exception in thread "main" java.lang.RuntimeException: Uncompilable source code - Erroneous sym type: java.lang.Cloneable.clone
at securedatacontainer.B.get(Demo.java:60)
at securedatacontainer.Demo.main(Demo.java:19)
Because the project is supposed to be a data storage, I really doubt my teacher wants some shallow copy of the generic E element (note that is just a simple test program for clone, not the actual project). Can anyone tell me why this does not work or how I can make it work? I can't make any assumption about the input E element, only that it has its own clone() method
java generics clone
add a comment |
up vote
2
down vote
favorite
I have a generic interface and I have to create a class to implement it. I thought at first this would work fine, putting it in the documentation that the interface requires a class that implements the clone method
genericClass<E extends Cloneable> implements genericInterface<E extends Cloneable>{
E data;
//code
public E get(){
return this.data.clone()
}
}
However this doesn't work in practice
package securedatacontainer;
public class Demo {
public static void main(String args) throws CloneNotSupportedException {
String s = "user";
A a = new A(5, s);
A b = a.clone();
System.out.println("a equals b: " +(a.equals(b)));
System.out.println("a == b: " + (a == b));
B<A> c = new B<>(a);
System.out.println("a equals c.data: " +(a.equals(c.data)));
System.out.println("a == c.data: " + (a == c.data));
A k = c.get();
System.out.println(k.value);
}
}
class A implements Cloneable{
int value;
String name;
public A(int x, String str ){
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
public boolean equals(A elem){
return (this.name).equals(elem.name) && this.value==elem.value;
}
}
class B <E extends Cloneable>{
E data;
public B(E elem){
this.data=elem;
}
public E get() throws CloneNotSupportedException{
return (E) this.data.clone();
}
}
I get
Exception in thread "main" java.lang.RuntimeException: Uncompilable source code - Erroneous sym type: java.lang.Cloneable.clone
at securedatacontainer.B.get(Demo.java:60)
at securedatacontainer.Demo.main(Demo.java:19)
Because the project is supposed to be a data storage, I really doubt my teacher wants some shallow copy of the generic E element (note that is just a simple test program for clone, not the actual project). Can anyone tell me why this does not work or how I can make it work? I can't make any assumption about the input E element, only that it has its own clone() method
java generics clone
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have a generic interface and I have to create a class to implement it. I thought at first this would work fine, putting it in the documentation that the interface requires a class that implements the clone method
genericClass<E extends Cloneable> implements genericInterface<E extends Cloneable>{
E data;
//code
public E get(){
return this.data.clone()
}
}
However this doesn't work in practice
package securedatacontainer;
public class Demo {
public static void main(String args) throws CloneNotSupportedException {
String s = "user";
A a = new A(5, s);
A b = a.clone();
System.out.println("a equals b: " +(a.equals(b)));
System.out.println("a == b: " + (a == b));
B<A> c = new B<>(a);
System.out.println("a equals c.data: " +(a.equals(c.data)));
System.out.println("a == c.data: " + (a == c.data));
A k = c.get();
System.out.println(k.value);
}
}
class A implements Cloneable{
int value;
String name;
public A(int x, String str ){
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
public boolean equals(A elem){
return (this.name).equals(elem.name) && this.value==elem.value;
}
}
class B <E extends Cloneable>{
E data;
public B(E elem){
this.data=elem;
}
public E get() throws CloneNotSupportedException{
return (E) this.data.clone();
}
}
I get
Exception in thread "main" java.lang.RuntimeException: Uncompilable source code - Erroneous sym type: java.lang.Cloneable.clone
at securedatacontainer.B.get(Demo.java:60)
at securedatacontainer.Demo.main(Demo.java:19)
Because the project is supposed to be a data storage, I really doubt my teacher wants some shallow copy of the generic E element (note that is just a simple test program for clone, not the actual project). Can anyone tell me why this does not work or how I can make it work? I can't make any assumption about the input E element, only that it has its own clone() method
java generics clone
I have a generic interface and I have to create a class to implement it. I thought at first this would work fine, putting it in the documentation that the interface requires a class that implements the clone method
genericClass<E extends Cloneable> implements genericInterface<E extends Cloneable>{
E data;
//code
public E get(){
return this.data.clone()
}
}
However this doesn't work in practice
package securedatacontainer;
public class Demo {
public static void main(String args) throws CloneNotSupportedException {
String s = "user";
A a = new A(5, s);
A b = a.clone();
System.out.println("a equals b: " +(a.equals(b)));
System.out.println("a == b: " + (a == b));
B<A> c = new B<>(a);
System.out.println("a equals c.data: " +(a.equals(c.data)));
System.out.println("a == c.data: " + (a == c.data));
A k = c.get();
System.out.println(k.value);
}
}
class A implements Cloneable{
int value;
String name;
public A(int x, String str ){
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
public boolean equals(A elem){
return (this.name).equals(elem.name) && this.value==elem.value;
}
}
class B <E extends Cloneable>{
E data;
public B(E elem){
this.data=elem;
}
public E get() throws CloneNotSupportedException{
return (E) this.data.clone();
}
}
I get
Exception in thread "main" java.lang.RuntimeException: Uncompilable source code - Erroneous sym type: java.lang.Cloneable.clone
at securedatacontainer.B.get(Demo.java:60)
at securedatacontainer.Demo.main(Demo.java:19)
Because the project is supposed to be a data storage, I really doubt my teacher wants some shallow copy of the generic E element (note that is just a simple test program for clone, not the actual project). Can anyone tell me why this does not work or how I can make it work? I can't make any assumption about the input E element, only that it has its own clone() method
java generics clone
java generics clone
edited Nov 11 at 15:27
asked Nov 11 at 15:22
Dario
133
133
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Because the clone
method is marked as protected
on the Object
class, you cannot in general call this method on arbitrary objects. The idea behind the clone()
method is that classes which supported it would override the method, declaring it as public.
The only real solution here that preserves full functionality is to use reflection to access the method and get around the access modifiers.
So here's my solution,
public class B<E extends Cloneable> {
E data;
public B(E elem) {
this.data = elem;
}
@SuppressWarnings("unchecked")
public E get() {
Method clone = null;
try {
clone = data.getClass().getMethod("clone");
Object args = new Object[0];
return (E) clone.invoke(data, args);
} catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
Clonable
determines the behavior of Object’s protected clone implementation: if a class implements Cloneable
, Object’s clone method returns a field-by-field copy of the object; otherwise it throws CloneNotSupportedException
. But the way you have implemented the clone method in class A
does not call Object's
clone
method so this has no effect.
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
If you want to make use of that facility you have to implement it like so,
public class A implements Cloneable {
int value;
String name;
public A(int x, String str) {
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
return (A) super.clone();
}
public boolean equals(A elem) {
return (this.name).equals(elem.name) && this.value == elem.value;
}
}
In this case if your class A
does not implement Cloneable
then the java.lang.CloneNotSupportedException
will be thrown.
Finally the declaration public class B<E extends Cloneable>
gives you a compiler error if you try to pass in something that does not implement Cloneable
to the B
constructor in your Demo
class.
B<A> c = new B<>(doesNotImplCloneable); // Gives a compilation error.
So if you are using the Object's clone method as I have shown here the extends/implements Cloneable
is the way to go.
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Because the clone
method is marked as protected
on the Object
class, you cannot in general call this method on arbitrary objects. The idea behind the clone()
method is that classes which supported it would override the method, declaring it as public.
The only real solution here that preserves full functionality is to use reflection to access the method and get around the access modifiers.
So here's my solution,
public class B<E extends Cloneable> {
E data;
public B(E elem) {
this.data = elem;
}
@SuppressWarnings("unchecked")
public E get() {
Method clone = null;
try {
clone = data.getClass().getMethod("clone");
Object args = new Object[0];
return (E) clone.invoke(data, args);
} catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
Clonable
determines the behavior of Object’s protected clone implementation: if a class implements Cloneable
, Object’s clone method returns a field-by-field copy of the object; otherwise it throws CloneNotSupportedException
. But the way you have implemented the clone method in class A
does not call Object's
clone
method so this has no effect.
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
If you want to make use of that facility you have to implement it like so,
public class A implements Cloneable {
int value;
String name;
public A(int x, String str) {
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
return (A) super.clone();
}
public boolean equals(A elem) {
return (this.name).equals(elem.name) && this.value == elem.value;
}
}
In this case if your class A
does not implement Cloneable
then the java.lang.CloneNotSupportedException
will be thrown.
Finally the declaration public class B<E extends Cloneable>
gives you a compiler error if you try to pass in something that does not implement Cloneable
to the B
constructor in your Demo
class.
B<A> c = new B<>(doesNotImplCloneable); // Gives a compilation error.
So if you are using the Object's clone method as I have shown here the extends/implements Cloneable
is the way to go.
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
add a comment |
up vote
0
down vote
accepted
Because the clone
method is marked as protected
on the Object
class, you cannot in general call this method on arbitrary objects. The idea behind the clone()
method is that classes which supported it would override the method, declaring it as public.
The only real solution here that preserves full functionality is to use reflection to access the method and get around the access modifiers.
So here's my solution,
public class B<E extends Cloneable> {
E data;
public B(E elem) {
this.data = elem;
}
@SuppressWarnings("unchecked")
public E get() {
Method clone = null;
try {
clone = data.getClass().getMethod("clone");
Object args = new Object[0];
return (E) clone.invoke(data, args);
} catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
Clonable
determines the behavior of Object’s protected clone implementation: if a class implements Cloneable
, Object’s clone method returns a field-by-field copy of the object; otherwise it throws CloneNotSupportedException
. But the way you have implemented the clone method in class A
does not call Object's
clone
method so this has no effect.
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
If you want to make use of that facility you have to implement it like so,
public class A implements Cloneable {
int value;
String name;
public A(int x, String str) {
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
return (A) super.clone();
}
public boolean equals(A elem) {
return (this.name).equals(elem.name) && this.value == elem.value;
}
}
In this case if your class A
does not implement Cloneable
then the java.lang.CloneNotSupportedException
will be thrown.
Finally the declaration public class B<E extends Cloneable>
gives you a compiler error if you try to pass in something that does not implement Cloneable
to the B
constructor in your Demo
class.
B<A> c = new B<>(doesNotImplCloneable); // Gives a compilation error.
So if you are using the Object's clone method as I have shown here the extends/implements Cloneable
is the way to go.
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Because the clone
method is marked as protected
on the Object
class, you cannot in general call this method on arbitrary objects. The idea behind the clone()
method is that classes which supported it would override the method, declaring it as public.
The only real solution here that preserves full functionality is to use reflection to access the method and get around the access modifiers.
So here's my solution,
public class B<E extends Cloneable> {
E data;
public B(E elem) {
this.data = elem;
}
@SuppressWarnings("unchecked")
public E get() {
Method clone = null;
try {
clone = data.getClass().getMethod("clone");
Object args = new Object[0];
return (E) clone.invoke(data, args);
} catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
Clonable
determines the behavior of Object’s protected clone implementation: if a class implements Cloneable
, Object’s clone method returns a field-by-field copy of the object; otherwise it throws CloneNotSupportedException
. But the way you have implemented the clone method in class A
does not call Object's
clone
method so this has no effect.
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
If you want to make use of that facility you have to implement it like so,
public class A implements Cloneable {
int value;
String name;
public A(int x, String str) {
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
return (A) super.clone();
}
public boolean equals(A elem) {
return (this.name).equals(elem.name) && this.value == elem.value;
}
}
In this case if your class A
does not implement Cloneable
then the java.lang.CloneNotSupportedException
will be thrown.
Finally the declaration public class B<E extends Cloneable>
gives you a compiler error if you try to pass in something that does not implement Cloneable
to the B
constructor in your Demo
class.
B<A> c = new B<>(doesNotImplCloneable); // Gives a compilation error.
So if you are using the Object's clone method as I have shown here the extends/implements Cloneable
is the way to go.
Because the clone
method is marked as protected
on the Object
class, you cannot in general call this method on arbitrary objects. The idea behind the clone()
method is that classes which supported it would override the method, declaring it as public.
The only real solution here that preserves full functionality is to use reflection to access the method and get around the access modifiers.
So here's my solution,
public class B<E extends Cloneable> {
E data;
public B(E elem) {
this.data = elem;
}
@SuppressWarnings("unchecked")
public E get() {
Method clone = null;
try {
clone = data.getClass().getMethod("clone");
Object args = new Object[0];
return (E) clone.invoke(data, args);
} catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
Clonable
determines the behavior of Object’s protected clone implementation: if a class implements Cloneable
, Object’s clone method returns a field-by-field copy of the object; otherwise it throws CloneNotSupportedException
. But the way you have implemented the clone method in class A
does not call Object's
clone
method so this has no effect.
@Override
public A clone() throws CloneNotSupportedException {
A temp = new A(this.value, this.name);
return temp;
}
If you want to make use of that facility you have to implement it like so,
public class A implements Cloneable {
int value;
String name;
public A(int x, String str) {
this.value = x;
this.name = str;
}
@Override
public A clone() throws CloneNotSupportedException {
return (A) super.clone();
}
public boolean equals(A elem) {
return (this.name).equals(elem.name) && this.value == elem.value;
}
}
In this case if your class A
does not implement Cloneable
then the java.lang.CloneNotSupportedException
will be thrown.
Finally the declaration public class B<E extends Cloneable>
gives you a compiler error if you try to pass in something that does not implement Cloneable
to the B
constructor in your Demo
class.
B<A> c = new B<>(doesNotImplCloneable); // Gives a compilation error.
So if you are using the Object's clone method as I have shown here the extends/implements Cloneable
is the way to go.
edited Nov 12 at 2:30
answered Nov 11 at 16:29


Ravindra Ranwala
8,01931533
8,01931533
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
add a comment |
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
1
1
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Thanks, your answer gave me an insight on the matter. If I'm fetching the clone() method directly this way, does "extends/implements Cloneable" serve any purpose? Can I just use invoke(data) instead of invoke(data, args)?
– Dario
Nov 11 at 18:16
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
Very good question, and I have updated my answer above.
– Ravindra Ranwala
Nov 12 at 2:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250170%2fhow-to-properly-implement-the-call-to-clone-in-a-generic-class-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Y 75MkrY0m,ePispSdv2E