execute Thread.interrupt() Object.notify() at the same time, why does has two results?
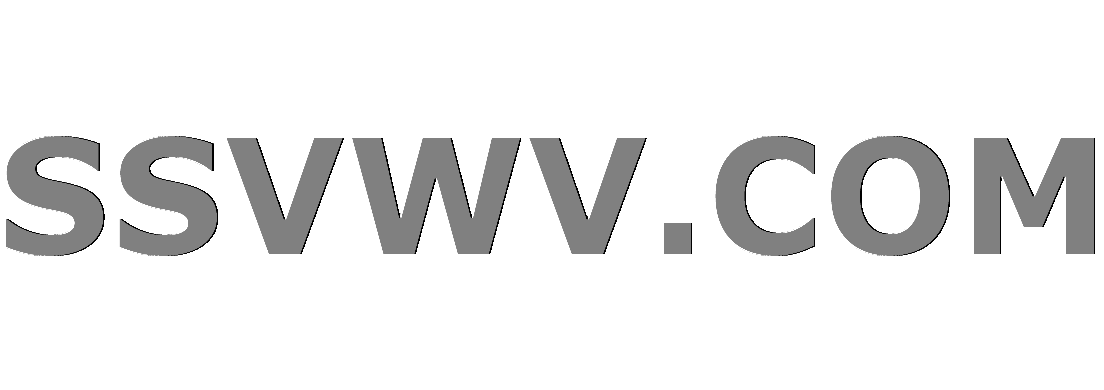
Multi tool use
up vote
2
down vote
favorite
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
There are two possible result here:
throws InterruptedException
normal termination
why?
I don't understand. when threadA is interruptted ,result should throws InterruptedException. but sometimes execute this program, it can normal finish.
environment: java8, mac
java concurrency interrupt notify
add a comment |
up vote
2
down vote
favorite
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
There are two possible result here:
throws InterruptedException
normal termination
why?
I don't understand. when threadA is interruptted ,result should throws InterruptedException. but sometimes execute this program, it can normal finish.
environment: java8, mac
java concurrency interrupt notify
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
There are two possible result here:
throws InterruptedException
normal termination
why?
I don't understand. when threadA is interruptted ,result should throws InterruptedException. but sometimes execute this program, it can normal finish.
environment: java8, mac
java concurrency interrupt notify
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
There are two possible result here:
throws InterruptedException
normal termination
why?
I don't understand. when threadA is interruptted ,result should throws InterruptedException. but sometimes execute this program, it can normal finish.
environment: java8, mac
java concurrency interrupt notify
java concurrency interrupt notify
edited Nov 11 at 15:23
JB Nizet
531k51855989
531k51855989
asked Nov 11 at 15:22
zhenyu wu
212
212
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Because of reordering. In normal termination compiler reordered instruction interrupt and notify, interruption invokes on working thread and no interrupted exception throws.
Try to forbid reordering with reading volatile variable and you always get interrupted exception.
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
System.out.print(resourceA);
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
When a thread receives both an interrupt and a notify, the behaviour may vary.
Please refer to https://docs.oracle.com/javase/specs/jls/se7/html/jls-17.html#jls-17.2.3
Credit - Alex Otenko on the Concurrency Interest mailing list
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Because of reordering. In normal termination compiler reordered instruction interrupt and notify, interruption invokes on working thread and no interrupted exception throws.
Try to forbid reordering with reading volatile variable and you always get interrupted exception.
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
System.out.print(resourceA);
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Because of reordering. In normal termination compiler reordered instruction interrupt and notify, interruption invokes on working thread and no interrupted exception throws.
Try to forbid reordering with reading volatile variable and you always get interrupted exception.
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
System.out.print(resourceA);
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
Because of reordering. In normal termination compiler reordered instruction interrupt and notify, interruption invokes on working thread and no interrupted exception throws.
Try to forbid reordering with reading volatile variable and you always get interrupted exception.
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
System.out.print(resourceA);
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Because of reordering. In normal termination compiler reordered instruction interrupt and notify, interruption invokes on working thread and no interrupted exception throws.
Try to forbid reordering with reading volatile variable and you always get interrupted exception.
public class WaitNotifyAll {
private static volatile Object resourceA = new Object();
public static void main(String args) throws Exception {
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
try {
System.out.println("threadA begin wait");
resourceA.wait();
System.out.println("threadA end wait");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
});
Thread threaB = new Thread(new Runnable() {
@Override
public void run() {
synchronized (resourceA) {
System.out.println("threadC begin notify");
threadA.interrupt();
System.out.print(resourceA);
resourceA.notify();
}
}
});
threadA.start();
Thread.sleep(1000);
threaB.start();
System.out.println("main over");
}
}
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 2 days ago
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 days ago
eSerpentine
11
11
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
eSerpentine is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
up vote
0
down vote
When a thread receives both an interrupt and a notify, the behaviour may vary.
Please refer to https://docs.oracle.com/javase/specs/jls/se7/html/jls-17.html#jls-17.2.3
Credit - Alex Otenko on the Concurrency Interest mailing list
add a comment |
up vote
0
down vote
When a thread receives both an interrupt and a notify, the behaviour may vary.
Please refer to https://docs.oracle.com/javase/specs/jls/se7/html/jls-17.html#jls-17.2.3
Credit - Alex Otenko on the Concurrency Interest mailing list
add a comment |
up vote
0
down vote
up vote
0
down vote
When a thread receives both an interrupt and a notify, the behaviour may vary.
Please refer to https://docs.oracle.com/javase/specs/jls/se7/html/jls-17.html#jls-17.2.3
Credit - Alex Otenko on the Concurrency Interest mailing list
When a thread receives both an interrupt and a notify, the behaviour may vary.
Please refer to https://docs.oracle.com/javase/specs/jls/se7/html/jls-17.html#jls-17.2.3
Credit - Alex Otenko on the Concurrency Interest mailing list
answered 2 days ago
Ashutosh A
63137
63137
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250167%2fexecute-thread-interrupt-object-notify-at-the-same-time-why-does-has-two-re%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eCS9rB6T kV XhAgioV