How would I approach making the Fibonacci Sequence in ARM?
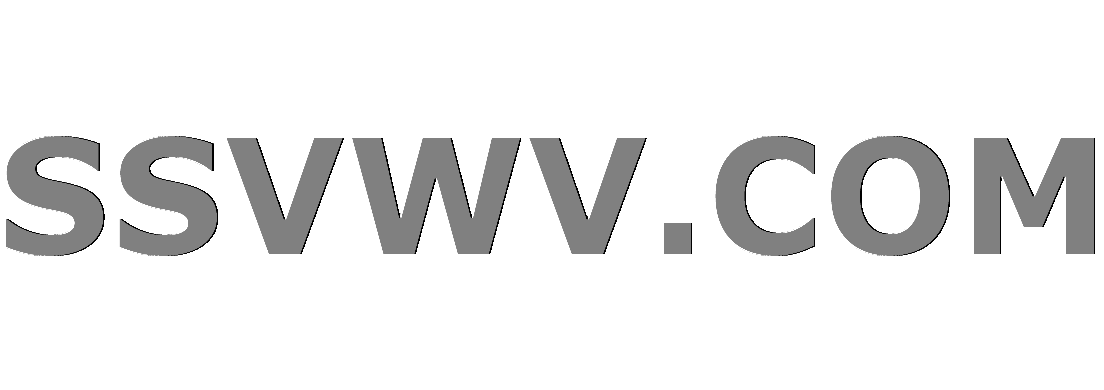
Multi tool use
up vote
0
down vote
favorite
I need to make an ARM assembly program which will print out the Fibonacci Sequence and i'm unsure of how to approach it. The program will ask the user for a number, and when they input that number, the program should print out the Fibonacci sequence for that amount of numbers, so for example, if the user inputs 10, the program will run through printing
"Fibonacci number 1 is 1."
"Fibonacci number 2 is 1."
And so on.
Currently my code for this looks like this:
B main
maxF DEFW 0
enterI DEFB "Please enter the number of fibonacci numbers to print: ",0
newline DEFB "n",0
fibbo DEFB "Fibonacci number ",0
is DEFB " is ",0
end DEFB ".n",0
errorM DEFB "Error, try again!n",0
ALIGN
main ADR R0, enterI
SWI 3
MOV R1, #0
MOV R2, #10
MOV R3, #0 ;lastNumber variable
MOV R4, #1 ;numberbeforeLast variable
MOV R5, #0 ;currentNumber variable
start SWI 1 ;take user input
CMP R0, #10 ;compare R0 with #10 (enter)
BEQ _end ;if equal, go to _end
CMP R0, #48 ;compare R0 with #48 (0)
BLT _error ;if less than, go to _error
CMP R0, #57 ;compare R0 with #57 (9)
BGT _error ;if greater than, go to _error
SUB R0, R0, #48 ;R0 = R0 - #48
SWI 4 ;print the above
MUL R1, R1, R2 ;Multiply the R1 register by R2 and store in R1
ADD R1, R1, R0 ;Add the R1 register to R0 and store in R1
B while_cond
while_loop
ADD R5, R3, R4 ;currentnumber = lastnumber + numberbeforelast
ADR R0, fibbo
SWI 3
STR R5, maxF
LDR R0, value
SWI 4
ADR R0, is
SWI 3
while_cond
CMP R0, #0
BGT while_loop
_end SWI 2
_error ADR R0, errorM
SWI 3
B main
I've been thinking of an approach for this and I have something but i'm unsure of how to do it. I was thinking that the program expects an input from the user for the number, and then it does the calculation for that number and then prints out the line for number currently in the register, branches back up to the top where the register is overwritten with the next value and then does the same thing until the value of that register is equal to the value that the user specified, which is when it stops.
assembly arm fibonacci
add a comment |
up vote
0
down vote
favorite
I need to make an ARM assembly program which will print out the Fibonacci Sequence and i'm unsure of how to approach it. The program will ask the user for a number, and when they input that number, the program should print out the Fibonacci sequence for that amount of numbers, so for example, if the user inputs 10, the program will run through printing
"Fibonacci number 1 is 1."
"Fibonacci number 2 is 1."
And so on.
Currently my code for this looks like this:
B main
maxF DEFW 0
enterI DEFB "Please enter the number of fibonacci numbers to print: ",0
newline DEFB "n",0
fibbo DEFB "Fibonacci number ",0
is DEFB " is ",0
end DEFB ".n",0
errorM DEFB "Error, try again!n",0
ALIGN
main ADR R0, enterI
SWI 3
MOV R1, #0
MOV R2, #10
MOV R3, #0 ;lastNumber variable
MOV R4, #1 ;numberbeforeLast variable
MOV R5, #0 ;currentNumber variable
start SWI 1 ;take user input
CMP R0, #10 ;compare R0 with #10 (enter)
BEQ _end ;if equal, go to _end
CMP R0, #48 ;compare R0 with #48 (0)
BLT _error ;if less than, go to _error
CMP R0, #57 ;compare R0 with #57 (9)
BGT _error ;if greater than, go to _error
SUB R0, R0, #48 ;R0 = R0 - #48
SWI 4 ;print the above
MUL R1, R1, R2 ;Multiply the R1 register by R2 and store in R1
ADD R1, R1, R0 ;Add the R1 register to R0 and store in R1
B while_cond
while_loop
ADD R5, R3, R4 ;currentnumber = lastnumber + numberbeforelast
ADR R0, fibbo
SWI 3
STR R5, maxF
LDR R0, value
SWI 4
ADR R0, is
SWI 3
while_cond
CMP R0, #0
BGT while_loop
_end SWI 2
_error ADR R0, errorM
SWI 3
B main
I've been thinking of an approach for this and I have something but i'm unsure of how to do it. I was thinking that the program expects an input from the user for the number, and then it does the calculation for that number and then prints out the line for number currently in the register, branches back up to the top where the register is overwritten with the next value and then does the same thing until the value of that register is equal to the value that the user specified, which is when it stops.
assembly arm fibonacci
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I need to make an ARM assembly program which will print out the Fibonacci Sequence and i'm unsure of how to approach it. The program will ask the user for a number, and when they input that number, the program should print out the Fibonacci sequence for that amount of numbers, so for example, if the user inputs 10, the program will run through printing
"Fibonacci number 1 is 1."
"Fibonacci number 2 is 1."
And so on.
Currently my code for this looks like this:
B main
maxF DEFW 0
enterI DEFB "Please enter the number of fibonacci numbers to print: ",0
newline DEFB "n",0
fibbo DEFB "Fibonacci number ",0
is DEFB " is ",0
end DEFB ".n",0
errorM DEFB "Error, try again!n",0
ALIGN
main ADR R0, enterI
SWI 3
MOV R1, #0
MOV R2, #10
MOV R3, #0 ;lastNumber variable
MOV R4, #1 ;numberbeforeLast variable
MOV R5, #0 ;currentNumber variable
start SWI 1 ;take user input
CMP R0, #10 ;compare R0 with #10 (enter)
BEQ _end ;if equal, go to _end
CMP R0, #48 ;compare R0 with #48 (0)
BLT _error ;if less than, go to _error
CMP R0, #57 ;compare R0 with #57 (9)
BGT _error ;if greater than, go to _error
SUB R0, R0, #48 ;R0 = R0 - #48
SWI 4 ;print the above
MUL R1, R1, R2 ;Multiply the R1 register by R2 and store in R1
ADD R1, R1, R0 ;Add the R1 register to R0 and store in R1
B while_cond
while_loop
ADD R5, R3, R4 ;currentnumber = lastnumber + numberbeforelast
ADR R0, fibbo
SWI 3
STR R5, maxF
LDR R0, value
SWI 4
ADR R0, is
SWI 3
while_cond
CMP R0, #0
BGT while_loop
_end SWI 2
_error ADR R0, errorM
SWI 3
B main
I've been thinking of an approach for this and I have something but i'm unsure of how to do it. I was thinking that the program expects an input from the user for the number, and then it does the calculation for that number and then prints out the line for number currently in the register, branches back up to the top where the register is overwritten with the next value and then does the same thing until the value of that register is equal to the value that the user specified, which is when it stops.
assembly arm fibonacci
I need to make an ARM assembly program which will print out the Fibonacci Sequence and i'm unsure of how to approach it. The program will ask the user for a number, and when they input that number, the program should print out the Fibonacci sequence for that amount of numbers, so for example, if the user inputs 10, the program will run through printing
"Fibonacci number 1 is 1."
"Fibonacci number 2 is 1."
And so on.
Currently my code for this looks like this:
B main
maxF DEFW 0
enterI DEFB "Please enter the number of fibonacci numbers to print: ",0
newline DEFB "n",0
fibbo DEFB "Fibonacci number ",0
is DEFB " is ",0
end DEFB ".n",0
errorM DEFB "Error, try again!n",0
ALIGN
main ADR R0, enterI
SWI 3
MOV R1, #0
MOV R2, #10
MOV R3, #0 ;lastNumber variable
MOV R4, #1 ;numberbeforeLast variable
MOV R5, #0 ;currentNumber variable
start SWI 1 ;take user input
CMP R0, #10 ;compare R0 with #10 (enter)
BEQ _end ;if equal, go to _end
CMP R0, #48 ;compare R0 with #48 (0)
BLT _error ;if less than, go to _error
CMP R0, #57 ;compare R0 with #57 (9)
BGT _error ;if greater than, go to _error
SUB R0, R0, #48 ;R0 = R0 - #48
SWI 4 ;print the above
MUL R1, R1, R2 ;Multiply the R1 register by R2 and store in R1
ADD R1, R1, R0 ;Add the R1 register to R0 and store in R1
B while_cond
while_loop
ADD R5, R3, R4 ;currentnumber = lastnumber + numberbeforelast
ADR R0, fibbo
SWI 3
STR R5, maxF
LDR R0, value
SWI 4
ADR R0, is
SWI 3
while_cond
CMP R0, #0
BGT while_loop
_end SWI 2
_error ADR R0, errorM
SWI 3
B main
I've been thinking of an approach for this and I have something but i'm unsure of how to do it. I was thinking that the program expects an input from the user for the number, and then it does the calculation for that number and then prints out the line for number currently in the register, branches back up to the top where the register is overwritten with the next value and then does the same thing until the value of that register is equal to the value that the user specified, which is when it stops.
assembly arm fibonacci
assembly arm fibonacci
edited Nov 14 at 18:39


Peter Cordes
117k16177304
117k16177304
asked Nov 11 at 15:21
Entilo
11
11
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40
add a comment |
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
Right now the code stands no chance of working because you don't ever update the values of r3
and r4
(your previous two Fibonacci numbers), and you overwrite r0
(intended to be your loop counter) in a number of places. There may be other problems too.
It's not really clear where you're stuck - it seems perhaps to be a combination of inexperience with assembly language and inexperience with the process of developing an algorithm. My advice would be to separate the two processes. Write some code in C (or another language, but C is the closest you'll get to assembly language without actually using it!) that computes the first n
Fibonacci numbers. Once that works, start thinking about how you would implement the same thing in assembly language.
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updatingr3
andr4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.
– cooperised
Nov 12 at 15:47
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Right now the code stands no chance of working because you don't ever update the values of r3
and r4
(your previous two Fibonacci numbers), and you overwrite r0
(intended to be your loop counter) in a number of places. There may be other problems too.
It's not really clear where you're stuck - it seems perhaps to be a combination of inexperience with assembly language and inexperience with the process of developing an algorithm. My advice would be to separate the two processes. Write some code in C (or another language, but C is the closest you'll get to assembly language without actually using it!) that computes the first n
Fibonacci numbers. Once that works, start thinking about how you would implement the same thing in assembly language.
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updatingr3
andr4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.
– cooperised
Nov 12 at 15:47
add a comment |
up vote
1
down vote
Right now the code stands no chance of working because you don't ever update the values of r3
and r4
(your previous two Fibonacci numbers), and you overwrite r0
(intended to be your loop counter) in a number of places. There may be other problems too.
It's not really clear where you're stuck - it seems perhaps to be a combination of inexperience with assembly language and inexperience with the process of developing an algorithm. My advice would be to separate the two processes. Write some code in C (or another language, but C is the closest you'll get to assembly language without actually using it!) that computes the first n
Fibonacci numbers. Once that works, start thinking about how you would implement the same thing in assembly language.
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updatingr3
andr4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.
– cooperised
Nov 12 at 15:47
add a comment |
up vote
1
down vote
up vote
1
down vote
Right now the code stands no chance of working because you don't ever update the values of r3
and r4
(your previous two Fibonacci numbers), and you overwrite r0
(intended to be your loop counter) in a number of places. There may be other problems too.
It's not really clear where you're stuck - it seems perhaps to be a combination of inexperience with assembly language and inexperience with the process of developing an algorithm. My advice would be to separate the two processes. Write some code in C (or another language, but C is the closest you'll get to assembly language without actually using it!) that computes the first n
Fibonacci numbers. Once that works, start thinking about how you would implement the same thing in assembly language.
Right now the code stands no chance of working because you don't ever update the values of r3
and r4
(your previous two Fibonacci numbers), and you overwrite r0
(intended to be your loop counter) in a number of places. There may be other problems too.
It's not really clear where you're stuck - it seems perhaps to be a combination of inexperience with assembly language and inexperience with the process of developing an algorithm. My advice would be to separate the two processes. Write some code in C (or another language, but C is the closest you'll get to assembly language without actually using it!) that computes the first n
Fibonacci numbers. Once that works, start thinking about how you would implement the same thing in assembly language.
answered Nov 12 at 9:51
cooperised
1,202812
1,202812
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updatingr3
andr4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.
– cooperised
Nov 12 at 15:47
add a comment |
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updatingr3
andr4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.
– cooperised
Nov 12 at 15:47
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updating
r3
and r4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.– cooperised
Nov 12 at 15:47
Sorry, that's still not clear enough. If you've got an algorithm in C that you understand, then there's no reason for the first of the errors that I mentioned in my answer: you're not updating
r3
and r4
so there's no way your code can logically work. That's not an assembly language problem, that's an algorithmic problem.– cooperised
Nov 12 at 15:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250164%2fhow-would-i-approach-making-the-fibonacci-sequence-in-arm%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WbAMwn u,NV6J9cSf07Igg8 TYBU Vnfys,BcbBvdhxLOseT0UyGLBpXscpnDYZKczRDbEaJHW ImaGkIeY1Li3uZ683e,hWyMMb3V,nEiF
Where do you expect the branch to main instruction to be loaded into memory? Please show us your best attempt at writing code for this problem. This is a standard homework question, so we'd like to see you make some effort.
– Elliot Alderson
Nov 11 at 21:36
If you want to delete your question, delete it. But don't edit it into nonsense by removing all the code.
– Peter Cordes
Nov 14 at 18:40