sequence of wait and notify of threads in java
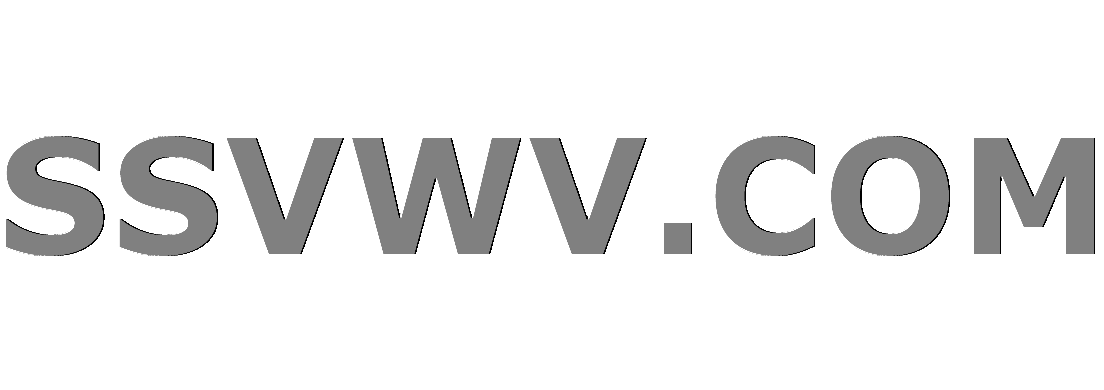
Multi tool use
up vote
1
down vote
favorite
I wrote a producer/consumer program as below.
package com.myjava.concurrency.basics.waitnotify;
import java.util.PriorityQueue;
import java.util.Queue;
public class SharedObject {
private Queue<String> dataObject;
private final Object objLock = new Object();
public SharedObject() {
dataObject = new PriorityQueue<String>(1);
}
public void writeData(String data) {
synchronized (objLock) {
while (!dataObject.isEmpty()) {
System.out.println("Producer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
dataObject.offer(data);
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), data));
objLock.notify();
}
}
public String readData() {
String result = null;
synchronized (objLock) {
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
result = dataObject.poll();
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), result));
objLock.notify();
}
return result;
}
}
package com.myjava.concurrency.basics.waitnotify;
import java.util.Arrays;
import java.util.List;
public class TestWaitNotify {
public static void main(String args) {
SharedObject sharedObject = new SharedObject();
List<String> fruitsList = Arrays.asList("Apple", "Banana", "Orange");
int listSize = fruitsList.size();
Thread producer = new Thread(() -> {
System.out.println("producer thread started");
fruitsList.forEach(p -> {
sharedObject.writeData(p);
});
}, "producer");
Thread consumer = new Thread(() -> {
System.out.println("consumer thread started");
for (int i = 0; i < listSize; i++) {
sharedObject.readData();
}
}, "consumer");
consumer.start();
producer.start();
}
}
I got the output, as below:
producer thread started
consumer thread started
Consumer:Waiting
producer : Apple
Producer:Waiting
consumer : Apple
Consumer:Waiting
producer : Banana
Producer:Waiting
consumer : Banana
Consumer:Waiting
producer : Orange
consumer : Orange
Here is my question:
I expected the below sequence, with this program:
producer thread started
consumer thread started
Consumer:Waiting // assuming consumer thread begins first
producer : Apple
consumer : Apple
producer : Banana
consumer : Banana
producer : Orange
consumer : Orange
Only consumer thread should enter in wait mode only once. After the first notify, the threads should not enter while loop because when producer thread has the object lock, consumer should wait for the lock and when consumer releases the lock the producer should acquire the lock.
Any help is appreciated.
java multithreading concurrency blocking
add a comment |
up vote
1
down vote
favorite
I wrote a producer/consumer program as below.
package com.myjava.concurrency.basics.waitnotify;
import java.util.PriorityQueue;
import java.util.Queue;
public class SharedObject {
private Queue<String> dataObject;
private final Object objLock = new Object();
public SharedObject() {
dataObject = new PriorityQueue<String>(1);
}
public void writeData(String data) {
synchronized (objLock) {
while (!dataObject.isEmpty()) {
System.out.println("Producer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
dataObject.offer(data);
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), data));
objLock.notify();
}
}
public String readData() {
String result = null;
synchronized (objLock) {
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
result = dataObject.poll();
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), result));
objLock.notify();
}
return result;
}
}
package com.myjava.concurrency.basics.waitnotify;
import java.util.Arrays;
import java.util.List;
public class TestWaitNotify {
public static void main(String args) {
SharedObject sharedObject = new SharedObject();
List<String> fruitsList = Arrays.asList("Apple", "Banana", "Orange");
int listSize = fruitsList.size();
Thread producer = new Thread(() -> {
System.out.println("producer thread started");
fruitsList.forEach(p -> {
sharedObject.writeData(p);
});
}, "producer");
Thread consumer = new Thread(() -> {
System.out.println("consumer thread started");
for (int i = 0; i < listSize; i++) {
sharedObject.readData();
}
}, "consumer");
consumer.start();
producer.start();
}
}
I got the output, as below:
producer thread started
consumer thread started
Consumer:Waiting
producer : Apple
Producer:Waiting
consumer : Apple
Consumer:Waiting
producer : Banana
Producer:Waiting
consumer : Banana
Consumer:Waiting
producer : Orange
consumer : Orange
Here is my question:
I expected the below sequence, with this program:
producer thread started
consumer thread started
Consumer:Waiting // assuming consumer thread begins first
producer : Apple
consumer : Apple
producer : Banana
consumer : Banana
producer : Orange
consumer : Orange
Only consumer thread should enter in wait mode only once. After the first notify, the threads should not enter while loop because when producer thread has the object lock, consumer should wait for the lock and when consumer releases the lock the producer should acquire the lock.
Any help is appreciated.
java multithreading concurrency blocking
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I wrote a producer/consumer program as below.
package com.myjava.concurrency.basics.waitnotify;
import java.util.PriorityQueue;
import java.util.Queue;
public class SharedObject {
private Queue<String> dataObject;
private final Object objLock = new Object();
public SharedObject() {
dataObject = new PriorityQueue<String>(1);
}
public void writeData(String data) {
synchronized (objLock) {
while (!dataObject.isEmpty()) {
System.out.println("Producer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
dataObject.offer(data);
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), data));
objLock.notify();
}
}
public String readData() {
String result = null;
synchronized (objLock) {
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
result = dataObject.poll();
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), result));
objLock.notify();
}
return result;
}
}
package com.myjava.concurrency.basics.waitnotify;
import java.util.Arrays;
import java.util.List;
public class TestWaitNotify {
public static void main(String args) {
SharedObject sharedObject = new SharedObject();
List<String> fruitsList = Arrays.asList("Apple", "Banana", "Orange");
int listSize = fruitsList.size();
Thread producer = new Thread(() -> {
System.out.println("producer thread started");
fruitsList.forEach(p -> {
sharedObject.writeData(p);
});
}, "producer");
Thread consumer = new Thread(() -> {
System.out.println("consumer thread started");
for (int i = 0; i < listSize; i++) {
sharedObject.readData();
}
}, "consumer");
consumer.start();
producer.start();
}
}
I got the output, as below:
producer thread started
consumer thread started
Consumer:Waiting
producer : Apple
Producer:Waiting
consumer : Apple
Consumer:Waiting
producer : Banana
Producer:Waiting
consumer : Banana
Consumer:Waiting
producer : Orange
consumer : Orange
Here is my question:
I expected the below sequence, with this program:
producer thread started
consumer thread started
Consumer:Waiting // assuming consumer thread begins first
producer : Apple
consumer : Apple
producer : Banana
consumer : Banana
producer : Orange
consumer : Orange
Only consumer thread should enter in wait mode only once. After the first notify, the threads should not enter while loop because when producer thread has the object lock, consumer should wait for the lock and when consumer releases the lock the producer should acquire the lock.
Any help is appreciated.
java multithreading concurrency blocking
I wrote a producer/consumer program as below.
package com.myjava.concurrency.basics.waitnotify;
import java.util.PriorityQueue;
import java.util.Queue;
public class SharedObject {
private Queue<String> dataObject;
private final Object objLock = new Object();
public SharedObject() {
dataObject = new PriorityQueue<String>(1);
}
public void writeData(String data) {
synchronized (objLock) {
while (!dataObject.isEmpty()) {
System.out.println("Producer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
dataObject.offer(data);
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), data));
objLock.notify();
}
}
public String readData() {
String result = null;
synchronized (objLock) {
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
try {
objLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
result = dataObject.poll();
System.out.println(String.format("%s : %s",
Thread.currentThread().getName(), result));
objLock.notify();
}
return result;
}
}
package com.myjava.concurrency.basics.waitnotify;
import java.util.Arrays;
import java.util.List;
public class TestWaitNotify {
public static void main(String args) {
SharedObject sharedObject = new SharedObject();
List<String> fruitsList = Arrays.asList("Apple", "Banana", "Orange");
int listSize = fruitsList.size();
Thread producer = new Thread(() -> {
System.out.println("producer thread started");
fruitsList.forEach(p -> {
sharedObject.writeData(p);
});
}, "producer");
Thread consumer = new Thread(() -> {
System.out.println("consumer thread started");
for (int i = 0; i < listSize; i++) {
sharedObject.readData();
}
}, "consumer");
consumer.start();
producer.start();
}
}
I got the output, as below:
producer thread started
consumer thread started
Consumer:Waiting
producer : Apple
Producer:Waiting
consumer : Apple
Consumer:Waiting
producer : Banana
Producer:Waiting
consumer : Banana
Consumer:Waiting
producer : Orange
consumer : Orange
Here is my question:
I expected the below sequence, with this program:
producer thread started
consumer thread started
Consumer:Waiting // assuming consumer thread begins first
producer : Apple
consumer : Apple
producer : Banana
consumer : Banana
producer : Orange
consumer : Orange
Only consumer thread should enter in wait mode only once. After the first notify, the threads should not enter while loop because when producer thread has the object lock, consumer should wait for the lock and when consumer releases the lock the producer should acquire the lock.
Any help is appreciated.
java multithreading concurrency blocking
java multithreading concurrency blocking
edited Nov 29 at 15:27
asked Nov 9 at 4:25
JohnySam
851211
851211
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
Object.notify() will wake up a thread waiting on the lock but it doesn't necessarily prioritize it to acquire next and the javadoc identifies this behavior:
The awakened thread will not be able to proceed until the current thread relinquishes the lock on this object. The awakened thread will compete in the usual manner with any other threads that might be actively competing to synchronize on this object; for example, the awakened thread enjoys no reliable privilege or disadvantage in being the next thread to lock this object.
Likely what is happening is the thread that just relinquished the lock is immediately acquiring it again in front of the thread you expect. If you put a sleep after the notify (but not in the synchronized block) you're likely to see the output you were expecting. In this cause you're forcing that thread to effectively yield to the other thread that has been notified.
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
add a comment |
up vote
0
down vote
Examine this tutorial may could help you, it seem's very similiar to your problem even it only differences from your example is method signs they have synchronized
keyword by the way.
https://www.tutorialspoint.com/javaexamples/thread_procon.htm
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
add a comment |
up vote
0
down vote
Here:
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime.
So the producer has to wait for the consumer to consume, then the consumer has to wait for the producer to put something new in.
Therefore the following assumption
Only consumer thread should enter in wait mode only once.
is wrong.
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219870%2fsequence-of-wait-and-notify-of-threads-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Object.notify() will wake up a thread waiting on the lock but it doesn't necessarily prioritize it to acquire next and the javadoc identifies this behavior:
The awakened thread will not be able to proceed until the current thread relinquishes the lock on this object. The awakened thread will compete in the usual manner with any other threads that might be actively competing to synchronize on this object; for example, the awakened thread enjoys no reliable privilege or disadvantage in being the next thread to lock this object.
Likely what is happening is the thread that just relinquished the lock is immediately acquiring it again in front of the thread you expect. If you put a sleep after the notify (but not in the synchronized block) you're likely to see the output you were expecting. In this cause you're forcing that thread to effectively yield to the other thread that has been notified.
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
add a comment |
up vote
1
down vote
accepted
Object.notify() will wake up a thread waiting on the lock but it doesn't necessarily prioritize it to acquire next and the javadoc identifies this behavior:
The awakened thread will not be able to proceed until the current thread relinquishes the lock on this object. The awakened thread will compete in the usual manner with any other threads that might be actively competing to synchronize on this object; for example, the awakened thread enjoys no reliable privilege or disadvantage in being the next thread to lock this object.
Likely what is happening is the thread that just relinquished the lock is immediately acquiring it again in front of the thread you expect. If you put a sleep after the notify (but not in the synchronized block) you're likely to see the output you were expecting. In this cause you're forcing that thread to effectively yield to the other thread that has been notified.
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Object.notify() will wake up a thread waiting on the lock but it doesn't necessarily prioritize it to acquire next and the javadoc identifies this behavior:
The awakened thread will not be able to proceed until the current thread relinquishes the lock on this object. The awakened thread will compete in the usual manner with any other threads that might be actively competing to synchronize on this object; for example, the awakened thread enjoys no reliable privilege or disadvantage in being the next thread to lock this object.
Likely what is happening is the thread that just relinquished the lock is immediately acquiring it again in front of the thread you expect. If you put a sleep after the notify (but not in the synchronized block) you're likely to see the output you were expecting. In this cause you're forcing that thread to effectively yield to the other thread that has been notified.
Object.notify() will wake up a thread waiting on the lock but it doesn't necessarily prioritize it to acquire next and the javadoc identifies this behavior:
The awakened thread will not be able to proceed until the current thread relinquishes the lock on this object. The awakened thread will compete in the usual manner with any other threads that might be actively competing to synchronize on this object; for example, the awakened thread enjoys no reliable privilege or disadvantage in being the next thread to lock this object.
Likely what is happening is the thread that just relinquished the lock is immediately acquiring it again in front of the thread you expect. If you put a sleep after the notify (but not in the synchronized block) you're likely to see the output you were expecting. In this cause you're forcing that thread to effectively yield to the other thread that has been notified.
answered Nov 9 at 4:54
John H
43727
43727
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
add a comment |
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
"the thread that just relinquished the lock is immediately acquiring it again" , this is the only possibility I can see. But, my understanding is by the time the consumer releases the lock the producer should be ahead of the consumer thread for acquiring lock, because producer is waiting since long time compare to consumer thread. Thanks @John
– JohnySam
Nov 9 at 15:44
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
I added print statement at the beginning and ending of each synchronized block of the code and noticed that the thread(eg.producer) that is releasing the lock is the one that acquiring immediately. The thread(consumer in this case) that is in wait is acquiring the lock only when the current thread(i.e producer) moved to wait state. So, @John's explanation is correct and in detail.
– JohnySam
Nov 29 at 2:54
add a comment |
up vote
0
down vote
Examine this tutorial may could help you, it seem's very similiar to your problem even it only differences from your example is method signs they have synchronized
keyword by the way.
https://www.tutorialspoint.com/javaexamples/thread_procon.htm
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
add a comment |
up vote
0
down vote
Examine this tutorial may could help you, it seem's very similiar to your problem even it only differences from your example is method signs they have synchronized
keyword by the way.
https://www.tutorialspoint.com/javaexamples/thread_procon.htm
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
add a comment |
up vote
0
down vote
up vote
0
down vote
Examine this tutorial may could help you, it seem's very similiar to your problem even it only differences from your example is method signs they have synchronized
keyword by the way.
https://www.tutorialspoint.com/javaexamples/thread_procon.htm
Examine this tutorial may could help you, it seem's very similiar to your problem even it only differences from your example is method signs they have synchronized
keyword by the way.
https://www.tutorialspoint.com/javaexamples/thread_procon.htm
answered Nov 9 at 12:52


Alican Beydemir
226312
226312
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
add a comment |
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
After adding print statement inside the while loop of that program, I got the output that matches with mine. Consumer is going to wait mode just after finishing its iteration, thats the point Im confused about. Producer #1 put: 0 Consumer #1 got: 0 Consumer:Waiting Producer #1 put: 1 Consumer #1 got: 1 Consumer:Waiting So, this is not helpful. However, I am curious to know the reason but not the similar/same output.
– JohnySam
Nov 9 at 16:25
add a comment |
up vote
0
down vote
Here:
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime.
So the producer has to wait for the consumer to consume, then the consumer has to wait for the producer to put something new in.
Therefore the following assumption
Only consumer thread should enter in wait mode only once.
is wrong.
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
add a comment |
up vote
0
down vote
Here:
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime.
So the producer has to wait for the consumer to consume, then the consumer has to wait for the producer to put something new in.
Therefore the following assumption
Only consumer thread should enter in wait mode only once.
is wrong.
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
add a comment |
up vote
0
down vote
up vote
0
down vote
Here:
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime.
So the producer has to wait for the consumer to consume, then the consumer has to wait for the producer to put something new in.
Therefore the following assumption
Only consumer thread should enter in wait mode only once.
is wrong.
Here:
while (dataObject.isEmpty()) {
System.out.println("Consumer:Waiting");
The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime.
So the producer has to wait for the consumer to consume, then the consumer has to wait for the producer to put something new in.
Therefore the following assumption
Only consumer thread should enter in wait mode only once.
is wrong.
answered Nov 9 at 12:57


GhostCat
87.6k1684144
87.6k1684144
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
add a comment |
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
"The consumer consumes one entry. But at the same time, the queue is locked, so nothing can be added in the meantime." I agree till this point. But look at the program, after consumer consumes the data, it calls notify(). At this moment the second iteration of the producer thread is waiting to acquire the lock at the synchronized statement . right? and it is not inside the while loop because the lock is still with consumer at this moment. When the consumer thread releases the lock after finishing notify(), it goes for next iteration.
– JohnySam
Nov 9 at 15:39
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
Continuation to the above comment. When the producer thread obtained the lock in the second iteration, the dataObject.isEmpty() should be definitely true and hence it should't enter into the while loop. Based on this assumption, I expected the only 'consumer' should enter while loop only once and after that it is just a game of lock release/acquire between producer/consumer.
– JohnySam
Nov 9 at 15:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219870%2fsequence-of-wait-and-notify-of-threads-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tF UlLG 4K6FbIgM,bpPFOZj3Gqqs7