Python exception handling - line number
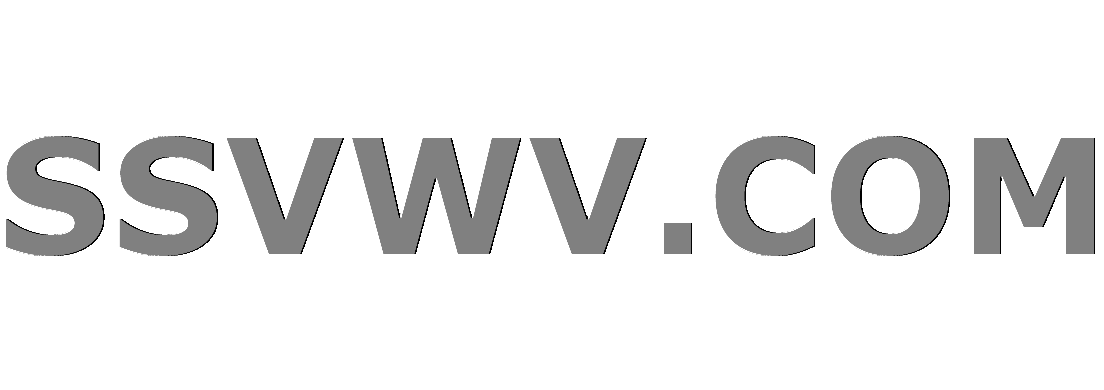
Multi tool use
up vote
39
down vote
favorite
I'm using python to evaluate some measured data. Because of many possible results it is difficult to handle or possible combinations. Sometimes an error happens during the evaluation. It is usually an index error because I get out of range from measured data.
It is very difficult to find out on which place in code the problem happened. It would help a lot if I knew on which line the error was raised. If I use following code:
try:
result = evaluateData(data)
except Exception, err:
print ("Error: %s.n" % str(err))
Unfortunately this only tells me that there is and index error. I would like to know more details about the exception (line in code, variable etc.) to find out what happened. Is it possible?
Thank you.
python exception indexing
add a comment |
up vote
39
down vote
favorite
I'm using python to evaluate some measured data. Because of many possible results it is difficult to handle or possible combinations. Sometimes an error happens during the evaluation. It is usually an index error because I get out of range from measured data.
It is very difficult to find out on which place in code the problem happened. It would help a lot if I knew on which line the error was raised. If I use following code:
try:
result = evaluateData(data)
except Exception, err:
print ("Error: %s.n" % str(err))
Unfortunately this only tells me that there is and index error. I would like to know more details about the exception (line in code, variable etc.) to find out what happened. Is it possible?
Thank you.
python exception indexing
1
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
3
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33
add a comment |
up vote
39
down vote
favorite
up vote
39
down vote
favorite
I'm using python to evaluate some measured data. Because of many possible results it is difficult to handle or possible combinations. Sometimes an error happens during the evaluation. It is usually an index error because I get out of range from measured data.
It is very difficult to find out on which place in code the problem happened. It would help a lot if I knew on which line the error was raised. If I use following code:
try:
result = evaluateData(data)
except Exception, err:
print ("Error: %s.n" % str(err))
Unfortunately this only tells me that there is and index error. I would like to know more details about the exception (line in code, variable etc.) to find out what happened. Is it possible?
Thank you.
python exception indexing
I'm using python to evaluate some measured data. Because of many possible results it is difficult to handle or possible combinations. Sometimes an error happens during the evaluation. It is usually an index error because I get out of range from measured data.
It is very difficult to find out on which place in code the problem happened. It would help a lot if I knew on which line the error was raised. If I use following code:
try:
result = evaluateData(data)
except Exception, err:
print ("Error: %s.n" % str(err))
Unfortunately this only tells me that there is and index error. I would like to know more details about the exception (line in code, variable etc.) to find out what happened. Is it possible?
Thank you.
python exception indexing
python exception indexing
asked Jan 25 '13 at 9:47
JeCh
315138
315138
1
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
3
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33
add a comment |
1
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
3
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33
1
1
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
3
3
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33
add a comment |
4 Answers
4
active
oldest
votes
up vote
57
down vote
Solution, printing filename, linenumber, line itself and exception descrition:
import linecache
import sys
def PrintException():
exc_type, exc_obj, tb = sys.exc_info()
f = tb.tb_frame
lineno = tb.tb_lineno
filename = f.f_code.co_filename
linecache.checkcache(filename)
line = linecache.getline(filename, lineno, f.f_globals)
print 'EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj)
try:
print 1/0
except:
PrintException()
Output:
EXCEPTION IN (D:/Projects/delme3.py, LINE 15 "print 1/0"): integer division or modulo by zero
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
add a comment |
up vote
18
down vote
To simply get the line number you can use sys
, if you would like to have more, try the traceback module.
import sys
try:
[2]
except IndexError:
print 'Error on line {}'.format(sys.exc_info()[-1].tb_lineno)
prints:
Error on line 3
Example from the traceback
module documentation:
import sys, traceback
def lumberjack():
bright_side_of_death()
def bright_side_of_death():
return tuple()[0]
try:
lumberjack()
except IndexError:
exc_type, exc_value, exc_traceback = sys.exc_info()
print "*** print_tb:"
traceback.print_tb(exc_traceback, limit=1, file=sys.stdout)
print "*** print_exception:"
traceback.print_exception(exc_type, exc_value, exc_traceback,
limit=2, file=sys.stdout)
print "*** print_exc:"
traceback.print_exc()
print "*** format_exc, first and last line:"
formatted_lines = traceback.format_exc().splitlines()
print formatted_lines[0]
print formatted_lines[-1]
print "*** format_exception:"
print repr(traceback.format_exception(exc_type, exc_value,
exc_traceback))
print "*** extract_tb:"
print repr(traceback.extract_tb(exc_traceback))
print "*** format_tb:"
print repr(traceback.format_tb(exc_traceback))
print "*** tb_lineno:", exc_traceback.tb_lineno
add a comment |
up vote
4
down vote
The simplest way is just to use:
import traceback
try:
<blah>
except IndexError:
traceback.print_exc()
or if using logging:
import logging
try:
<blah>
except IndexError as e:
logging.exception(e)
add a comment |
up vote
0
down vote
I use the traceback
which is simple and robust:
import traceback
try:
raise ValueError()
except:
print(traceback.format_exc())
Out:
Traceback (most recent call last):
File "catch.py", line 4, in <module>
raise ValueError()
ValueError
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
57
down vote
Solution, printing filename, linenumber, line itself and exception descrition:
import linecache
import sys
def PrintException():
exc_type, exc_obj, tb = sys.exc_info()
f = tb.tb_frame
lineno = tb.tb_lineno
filename = f.f_code.co_filename
linecache.checkcache(filename)
line = linecache.getline(filename, lineno, f.f_globals)
print 'EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj)
try:
print 1/0
except:
PrintException()
Output:
EXCEPTION IN (D:/Projects/delme3.py, LINE 15 "print 1/0"): integer division or modulo by zero
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
add a comment |
up vote
57
down vote
Solution, printing filename, linenumber, line itself and exception descrition:
import linecache
import sys
def PrintException():
exc_type, exc_obj, tb = sys.exc_info()
f = tb.tb_frame
lineno = tb.tb_lineno
filename = f.f_code.co_filename
linecache.checkcache(filename)
line = linecache.getline(filename, lineno, f.f_globals)
print 'EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj)
try:
print 1/0
except:
PrintException()
Output:
EXCEPTION IN (D:/Projects/delme3.py, LINE 15 "print 1/0"): integer division or modulo by zero
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
add a comment |
up vote
57
down vote
up vote
57
down vote
Solution, printing filename, linenumber, line itself and exception descrition:
import linecache
import sys
def PrintException():
exc_type, exc_obj, tb = sys.exc_info()
f = tb.tb_frame
lineno = tb.tb_lineno
filename = f.f_code.co_filename
linecache.checkcache(filename)
line = linecache.getline(filename, lineno, f.f_globals)
print 'EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj)
try:
print 1/0
except:
PrintException()
Output:
EXCEPTION IN (D:/Projects/delme3.py, LINE 15 "print 1/0"): integer division or modulo by zero
Solution, printing filename, linenumber, line itself and exception descrition:
import linecache
import sys
def PrintException():
exc_type, exc_obj, tb = sys.exc_info()
f = tb.tb_frame
lineno = tb.tb_lineno
filename = f.f_code.co_filename
linecache.checkcache(filename)
line = linecache.getline(filename, lineno, f.f_globals)
print 'EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj)
try:
print 1/0
except:
PrintException()
Output:
EXCEPTION IN (D:/Projects/delme3.py, LINE 15 "print 1/0"): integer division or modulo by zero
answered Nov 28 '13 at 10:50
Apogentus
3,3632425
3,3632425
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
add a comment |
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
For my python 3.6.5 I needed to add parens to last line with print: print('EXCEPTION IN ({}, LINE {} "{}"): {}'.format(filename, lineno, line.strip(), exc_obj))
– Chris
Aug 16 at 13:34
add a comment |
up vote
18
down vote
To simply get the line number you can use sys
, if you would like to have more, try the traceback module.
import sys
try:
[2]
except IndexError:
print 'Error on line {}'.format(sys.exc_info()[-1].tb_lineno)
prints:
Error on line 3
Example from the traceback
module documentation:
import sys, traceback
def lumberjack():
bright_side_of_death()
def bright_side_of_death():
return tuple()[0]
try:
lumberjack()
except IndexError:
exc_type, exc_value, exc_traceback = sys.exc_info()
print "*** print_tb:"
traceback.print_tb(exc_traceback, limit=1, file=sys.stdout)
print "*** print_exception:"
traceback.print_exception(exc_type, exc_value, exc_traceback,
limit=2, file=sys.stdout)
print "*** print_exc:"
traceback.print_exc()
print "*** format_exc, first and last line:"
formatted_lines = traceback.format_exc().splitlines()
print formatted_lines[0]
print formatted_lines[-1]
print "*** format_exception:"
print repr(traceback.format_exception(exc_type, exc_value,
exc_traceback))
print "*** extract_tb:"
print repr(traceback.extract_tb(exc_traceback))
print "*** format_tb:"
print repr(traceback.format_tb(exc_traceback))
print "*** tb_lineno:", exc_traceback.tb_lineno
add a comment |
up vote
18
down vote
To simply get the line number you can use sys
, if you would like to have more, try the traceback module.
import sys
try:
[2]
except IndexError:
print 'Error on line {}'.format(sys.exc_info()[-1].tb_lineno)
prints:
Error on line 3
Example from the traceback
module documentation:
import sys, traceback
def lumberjack():
bright_side_of_death()
def bright_side_of_death():
return tuple()[0]
try:
lumberjack()
except IndexError:
exc_type, exc_value, exc_traceback = sys.exc_info()
print "*** print_tb:"
traceback.print_tb(exc_traceback, limit=1, file=sys.stdout)
print "*** print_exception:"
traceback.print_exception(exc_type, exc_value, exc_traceback,
limit=2, file=sys.stdout)
print "*** print_exc:"
traceback.print_exc()
print "*** format_exc, first and last line:"
formatted_lines = traceback.format_exc().splitlines()
print formatted_lines[0]
print formatted_lines[-1]
print "*** format_exception:"
print repr(traceback.format_exception(exc_type, exc_value,
exc_traceback))
print "*** extract_tb:"
print repr(traceback.extract_tb(exc_traceback))
print "*** format_tb:"
print repr(traceback.format_tb(exc_traceback))
print "*** tb_lineno:", exc_traceback.tb_lineno
add a comment |
up vote
18
down vote
up vote
18
down vote
To simply get the line number you can use sys
, if you would like to have more, try the traceback module.
import sys
try:
[2]
except IndexError:
print 'Error on line {}'.format(sys.exc_info()[-1].tb_lineno)
prints:
Error on line 3
Example from the traceback
module documentation:
import sys, traceback
def lumberjack():
bright_side_of_death()
def bright_side_of_death():
return tuple()[0]
try:
lumberjack()
except IndexError:
exc_type, exc_value, exc_traceback = sys.exc_info()
print "*** print_tb:"
traceback.print_tb(exc_traceback, limit=1, file=sys.stdout)
print "*** print_exception:"
traceback.print_exception(exc_type, exc_value, exc_traceback,
limit=2, file=sys.stdout)
print "*** print_exc:"
traceback.print_exc()
print "*** format_exc, first and last line:"
formatted_lines = traceback.format_exc().splitlines()
print formatted_lines[0]
print formatted_lines[-1]
print "*** format_exception:"
print repr(traceback.format_exception(exc_type, exc_value,
exc_traceback))
print "*** extract_tb:"
print repr(traceback.extract_tb(exc_traceback))
print "*** format_tb:"
print repr(traceback.format_tb(exc_traceback))
print "*** tb_lineno:", exc_traceback.tb_lineno
To simply get the line number you can use sys
, if you would like to have more, try the traceback module.
import sys
try:
[2]
except IndexError:
print 'Error on line {}'.format(sys.exc_info()[-1].tb_lineno)
prints:
Error on line 3
Example from the traceback
module documentation:
import sys, traceback
def lumberjack():
bright_side_of_death()
def bright_side_of_death():
return tuple()[0]
try:
lumberjack()
except IndexError:
exc_type, exc_value, exc_traceback = sys.exc_info()
print "*** print_tb:"
traceback.print_tb(exc_traceback, limit=1, file=sys.stdout)
print "*** print_exception:"
traceback.print_exception(exc_type, exc_value, exc_traceback,
limit=2, file=sys.stdout)
print "*** print_exc:"
traceback.print_exc()
print "*** format_exc, first and last line:"
formatted_lines = traceback.format_exc().splitlines()
print formatted_lines[0]
print formatted_lines[-1]
print "*** format_exception:"
print repr(traceback.format_exception(exc_type, exc_value,
exc_traceback))
print "*** extract_tb:"
print repr(traceback.extract_tb(exc_traceback))
print "*** format_tb:"
print repr(traceback.format_tb(exc_traceback))
print "*** tb_lineno:", exc_traceback.tb_lineno
edited Jan 25 '13 at 10:36
answered Jan 25 '13 at 9:53
root
37.2k127599
37.2k127599
add a comment |
add a comment |
up vote
4
down vote
The simplest way is just to use:
import traceback
try:
<blah>
except IndexError:
traceback.print_exc()
or if using logging:
import logging
try:
<blah>
except IndexError as e:
logging.exception(e)
add a comment |
up vote
4
down vote
The simplest way is just to use:
import traceback
try:
<blah>
except IndexError:
traceback.print_exc()
or if using logging:
import logging
try:
<blah>
except IndexError as e:
logging.exception(e)
add a comment |
up vote
4
down vote
up vote
4
down vote
The simplest way is just to use:
import traceback
try:
<blah>
except IndexError:
traceback.print_exc()
or if using logging:
import logging
try:
<blah>
except IndexError as e:
logging.exception(e)
The simplest way is just to use:
import traceback
try:
<blah>
except IndexError:
traceback.print_exc()
or if using logging:
import logging
try:
<blah>
except IndexError as e:
logging.exception(e)
answered Dec 19 '17 at 0:28
acowpy
1115
1115
add a comment |
add a comment |
up vote
0
down vote
I use the traceback
which is simple and robust:
import traceback
try:
raise ValueError()
except:
print(traceback.format_exc())
Out:
Traceback (most recent call last):
File "catch.py", line 4, in <module>
raise ValueError()
ValueError
add a comment |
up vote
0
down vote
I use the traceback
which is simple and robust:
import traceback
try:
raise ValueError()
except:
print(traceback.format_exc())
Out:
Traceback (most recent call last):
File "catch.py", line 4, in <module>
raise ValueError()
ValueError
add a comment |
up vote
0
down vote
up vote
0
down vote
I use the traceback
which is simple and robust:
import traceback
try:
raise ValueError()
except:
print(traceback.format_exc())
Out:
Traceback (most recent call last):
File "catch.py", line 4, in <module>
raise ValueError()
ValueError
I use the traceback
which is simple and robust:
import traceback
try:
raise ValueError()
except:
print(traceback.format_exc())
Out:
Traceback (most recent call last):
File "catch.py", line 4, in <module>
raise ValueError()
ValueError
answered Nov 11 at 13:43


Benyamin Jafari
2,46031833
2,46031833
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f14519177%2fpython-exception-handling-line-number%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Jq8Hjg6q4WuU0n U,fr1yhgyxp 9 0 dA,6j029duLbpx2jvU,zbhn9H,Y0,j 7Vs,m7Skg,YS ENdsdLlU,KY7qbv8Y5t9sEvZn 9jedU9
1
See stackoverflow.com/questions/3702675/…!
– Charles Beattie
Jan 25 '13 at 9:57
docs.python.org/2/library/traceback.html#traceback-examples
– osa
Sep 9 '14 at 23:44
3
@JeCh The answers look good. Please accept one. To accept it click the empty checkmark next to the answer.
– Michael Potter
Aug 9 '15 at 23:53
The answer that is voted highest here is also presented in the stackoverflow question that @CharlesBeattie references above. That answer has important comments associated with it whereas this answer does not. It's definitely worth your time to check that one out.
– harperville
Jan 9 '17 at 17:33