Java Time: Create your own Timezone
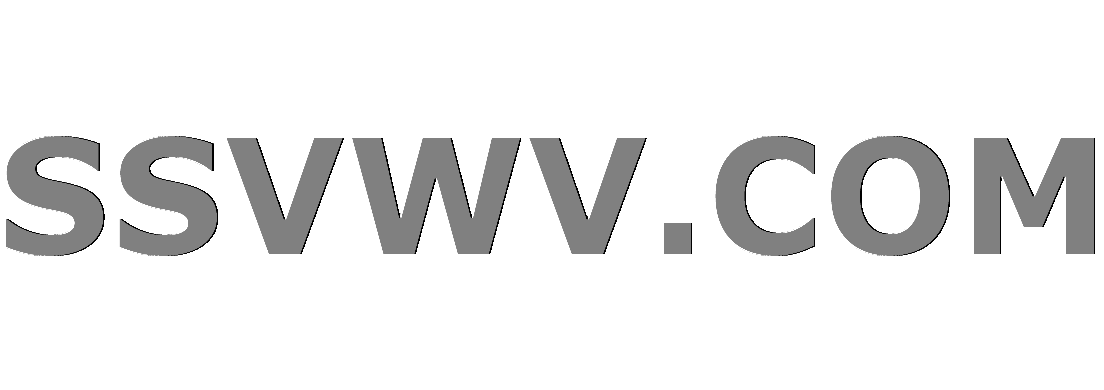
Multi tool use
up vote
6
down vote
favorite
I'm trying to migrate an old library to use the "new" Java time API and struggle on one point.
Context
I developed quite some time now an application to handle charts in the banking industry. So you have a continuous flow of prices coming and you aggregate them by 1 day candles (for instance) with an open, high, low and close.
The main issue came with the fact that the charts need to display candles only when the market is open. For forex, this means from Sunday 17:00 NY till Friday 17:00 NY. So the day starts at 17:00 NY time and your candles need to reflect that even if you live in Geneva.
The way I did this with the old "Java Time" API was to create my own timezone (see code below)
public class RelativeTimezone extends TimeZone {
private final TimeZone zone;
private final int addedTime;
public RelativeTimezone(String id, TimeZone zone, int addedTime) {
this.zone = zone;
this.addedTime = addedTime;
setID(id);
}
public final int getAddedTime() {
return this.addedTime;
}
@Override
public int getRawOffset() {
return this.zone.getRawOffset() + this.addedTime;
}
@Override
public void setRawOffset(int offsetMillis) {
this.zone.setRawOffset(offsetMillis - this.addedTime);
}
// Other overriding methods that simply forward to "this.zone"
// ...
}
By calling:
TimeZone forexTimezone = new RelativeTimezone("NY+7",
TimeZone.getTimeZone("America/New_York"), 7 * 3_600_000L)
I get a Timezone where monday midnight is actually sunday 17:00 NY. This greatly helps with all the computations to know which day it is. And I automatically get the Daylight saving times correctly.
Problem:
I did not find a way to do the same with the "new" time API. The classes are either final or package protected so I'm unable to create my own TimeZone. I tried to find everywhere if someone had done something like that in the past but didn't find anything.
I found that I could create a new ZoneRules to make my own ZoneId, but impossible to use the initial ZoneRules (for NY) to get the initial parameters to feed it (why isn't it an interface that I could "decorate"). The only solution that I see is to use "reflexion" to read the NY ZoneRules fields.
Would there be a way to do this cleanly ?
Of course as long as the "old" API is there I can still use it, but for how long.
java time timezone java-time
add a comment |
up vote
6
down vote
favorite
I'm trying to migrate an old library to use the "new" Java time API and struggle on one point.
Context
I developed quite some time now an application to handle charts in the banking industry. So you have a continuous flow of prices coming and you aggregate them by 1 day candles (for instance) with an open, high, low and close.
The main issue came with the fact that the charts need to display candles only when the market is open. For forex, this means from Sunday 17:00 NY till Friday 17:00 NY. So the day starts at 17:00 NY time and your candles need to reflect that even if you live in Geneva.
The way I did this with the old "Java Time" API was to create my own timezone (see code below)
public class RelativeTimezone extends TimeZone {
private final TimeZone zone;
private final int addedTime;
public RelativeTimezone(String id, TimeZone zone, int addedTime) {
this.zone = zone;
this.addedTime = addedTime;
setID(id);
}
public final int getAddedTime() {
return this.addedTime;
}
@Override
public int getRawOffset() {
return this.zone.getRawOffset() + this.addedTime;
}
@Override
public void setRawOffset(int offsetMillis) {
this.zone.setRawOffset(offsetMillis - this.addedTime);
}
// Other overriding methods that simply forward to "this.zone"
// ...
}
By calling:
TimeZone forexTimezone = new RelativeTimezone("NY+7",
TimeZone.getTimeZone("America/New_York"), 7 * 3_600_000L)
I get a Timezone where monday midnight is actually sunday 17:00 NY. This greatly helps with all the computations to know which day it is. And I automatically get the Daylight saving times correctly.
Problem:
I did not find a way to do the same with the "new" time API. The classes are either final or package protected so I'm unable to create my own TimeZone. I tried to find everywhere if someone had done something like that in the past but didn't find anything.
I found that I could create a new ZoneRules to make my own ZoneId, but impossible to use the initial ZoneRules (for NY) to get the initial parameters to feed it (why isn't it an interface that I could "decorate"). The only solution that I see is to use "reflexion" to read the NY ZoneRules fields.
Would there be a way to do this cleanly ?
Of course as long as the "old" API is there I can still use it, but for how long.
java time timezone java-time
1
I take it that you cannot just useZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?
– Ole V.V.
Nov 9 at 11:27
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
I'm trying to migrate an old library to use the "new" Java time API and struggle on one point.
Context
I developed quite some time now an application to handle charts in the banking industry. So you have a continuous flow of prices coming and you aggregate them by 1 day candles (for instance) with an open, high, low and close.
The main issue came with the fact that the charts need to display candles only when the market is open. For forex, this means from Sunday 17:00 NY till Friday 17:00 NY. So the day starts at 17:00 NY time and your candles need to reflect that even if you live in Geneva.
The way I did this with the old "Java Time" API was to create my own timezone (see code below)
public class RelativeTimezone extends TimeZone {
private final TimeZone zone;
private final int addedTime;
public RelativeTimezone(String id, TimeZone zone, int addedTime) {
this.zone = zone;
this.addedTime = addedTime;
setID(id);
}
public final int getAddedTime() {
return this.addedTime;
}
@Override
public int getRawOffset() {
return this.zone.getRawOffset() + this.addedTime;
}
@Override
public void setRawOffset(int offsetMillis) {
this.zone.setRawOffset(offsetMillis - this.addedTime);
}
// Other overriding methods that simply forward to "this.zone"
// ...
}
By calling:
TimeZone forexTimezone = new RelativeTimezone("NY+7",
TimeZone.getTimeZone("America/New_York"), 7 * 3_600_000L)
I get a Timezone where monday midnight is actually sunday 17:00 NY. This greatly helps with all the computations to know which day it is. And I automatically get the Daylight saving times correctly.
Problem:
I did not find a way to do the same with the "new" time API. The classes are either final or package protected so I'm unable to create my own TimeZone. I tried to find everywhere if someone had done something like that in the past but didn't find anything.
I found that I could create a new ZoneRules to make my own ZoneId, but impossible to use the initial ZoneRules (for NY) to get the initial parameters to feed it (why isn't it an interface that I could "decorate"). The only solution that I see is to use "reflexion" to read the NY ZoneRules fields.
Would there be a way to do this cleanly ?
Of course as long as the "old" API is there I can still use it, but for how long.
java time timezone java-time
I'm trying to migrate an old library to use the "new" Java time API and struggle on one point.
Context
I developed quite some time now an application to handle charts in the banking industry. So you have a continuous flow of prices coming and you aggregate them by 1 day candles (for instance) with an open, high, low and close.
The main issue came with the fact that the charts need to display candles only when the market is open. For forex, this means from Sunday 17:00 NY till Friday 17:00 NY. So the day starts at 17:00 NY time and your candles need to reflect that even if you live in Geneva.
The way I did this with the old "Java Time" API was to create my own timezone (see code below)
public class RelativeTimezone extends TimeZone {
private final TimeZone zone;
private final int addedTime;
public RelativeTimezone(String id, TimeZone zone, int addedTime) {
this.zone = zone;
this.addedTime = addedTime;
setID(id);
}
public final int getAddedTime() {
return this.addedTime;
}
@Override
public int getRawOffset() {
return this.zone.getRawOffset() + this.addedTime;
}
@Override
public void setRawOffset(int offsetMillis) {
this.zone.setRawOffset(offsetMillis - this.addedTime);
}
// Other overriding methods that simply forward to "this.zone"
// ...
}
By calling:
TimeZone forexTimezone = new RelativeTimezone("NY+7",
TimeZone.getTimeZone("America/New_York"), 7 * 3_600_000L)
I get a Timezone where monday midnight is actually sunday 17:00 NY. This greatly helps with all the computations to know which day it is. And I automatically get the Daylight saving times correctly.
Problem:
I did not find a way to do the same with the "new" time API. The classes are either final or package protected so I'm unable to create my own TimeZone. I tried to find everywhere if someone had done something like that in the past but didn't find anything.
I found that I could create a new ZoneRules to make my own ZoneId, but impossible to use the initial ZoneRules (for NY) to get the initial parameters to feed it (why isn't it an interface that I could "decorate"). The only solution that I see is to use "reflexion" to read the NY ZoneRules fields.
Would there be a way to do this cleanly ?
Of course as long as the "old" API is there I can still use it, but for how long.
java time timezone java-time
java time timezone java-time
edited Nov 10 at 13:22
asked Nov 9 at 11:02
John Gonon
313
313
1
I take it that you cannot just useZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?
– Ole V.V.
Nov 9 at 11:27
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44
add a comment |
1
I take it that you cannot just useZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?
– Ole V.V.
Nov 9 at 11:27
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44
1
1
I take it that you cannot just use
ZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?– Ole V.V.
Nov 9 at 11:27
I take it that you cannot just use
ZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?– Ole V.V.
Nov 9 at 11:27
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53224487%2fjava-time-create-your-own-timezone%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZBMm8N,MyGnSKo7cGFIXWBE2cu,HS h8eRq3U,d2gIDN Wobi
1
I take it that you cannot just use
ZoneOffset.ofHours(2)
since that would not take summer time (DST) in New York into account?– Ole V.V.
Nov 9 at 11:27
No, the new ZoneId really needs to duplicate all the DST behavior but just shift time so that, when the clock shows 17:00 in NY, this zone indicates midnight the following day. I also added a bit of info in the "Problem" paragraph
– John Gonon
Nov 10 at 13:15
It’s almost a duplicate of Custom ZoneIds / Time Zones in Java. The added requirement in this question is that the custom time zone should be defined as relative to some existing time zone.
– Ole V.V.
Nov 11 at 14:44