Insert a variable inside a string
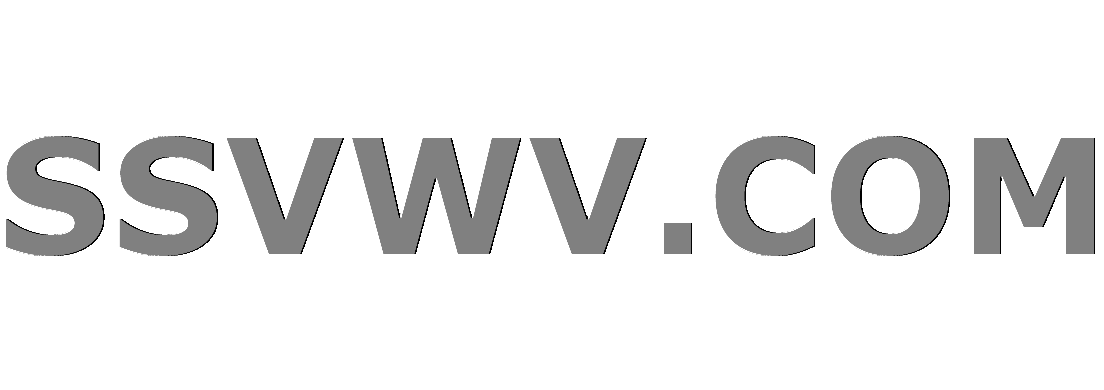
Multi tool use
up vote
-2
down vote
favorite
I have the hostname
and a json
formated string. I want to insert the hostname
inside the value string of a key in that json
formated string.
My full code:
func pager() string {
token := "xxxxxxxxxxx"
url := "https://api.pagerduty.com/incidents"
hostname, err := os.Hostname()
fmt.Println(hostname, err)
jsonStr := byte(`{
"incident": {
"type": "incident",
**"title": "Docker is down on."+hostname,**
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonStr))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Accept", "application/vnd.pagerduty+json;version=2")
req.Header.Set("From", "shinoda@wathever.com")
req.Header.Set("Authorization", "Token token="+token)
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
return resp.Status
}
func main() {
fmt.Println(pager())
}
I am not very familiar with go, in python I can do this easily and I dont know the proper way to do this in golang.
If someone could explain me, I would be grateful.
thanks in advance.
json

add a comment |
up vote
-2
down vote
favorite
I have the hostname
and a json
formated string. I want to insert the hostname
inside the value string of a key in that json
formated string.
My full code:
func pager() string {
token := "xxxxxxxxxxx"
url := "https://api.pagerduty.com/incidents"
hostname, err := os.Hostname()
fmt.Println(hostname, err)
jsonStr := byte(`{
"incident": {
"type": "incident",
**"title": "Docker is down on."+hostname,**
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonStr))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Accept", "application/vnd.pagerduty+json;version=2")
req.Header.Set("From", "shinoda@wathever.com")
req.Header.Set("Authorization", "Token token="+token)
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
return resp.Status
}
func main() {
fmt.Println(pager())
}
I am not very familiar with go, in python I can do this easily and I dont know the proper way to do this in golang.
If someone could explain me, I would be grateful.
thanks in advance.
json

I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
1
I'd like to point out thatos.Hostname()
is a function.
– ssemilla
Nov 11 at 14:42
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I have the hostname
and a json
formated string. I want to insert the hostname
inside the value string of a key in that json
formated string.
My full code:
func pager() string {
token := "xxxxxxxxxxx"
url := "https://api.pagerduty.com/incidents"
hostname, err := os.Hostname()
fmt.Println(hostname, err)
jsonStr := byte(`{
"incident": {
"type": "incident",
**"title": "Docker is down on."+hostname,**
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonStr))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Accept", "application/vnd.pagerduty+json;version=2")
req.Header.Set("From", "shinoda@wathever.com")
req.Header.Set("Authorization", "Token token="+token)
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
return resp.Status
}
func main() {
fmt.Println(pager())
}
I am not very familiar with go, in python I can do this easily and I dont know the proper way to do this in golang.
If someone could explain me, I would be grateful.
thanks in advance.
json

I have the hostname
and a json
formated string. I want to insert the hostname
inside the value string of a key in that json
formated string.
My full code:
func pager() string {
token := "xxxxxxxxxxx"
url := "https://api.pagerduty.com/incidents"
hostname, err := os.Hostname()
fmt.Println(hostname, err)
jsonStr := byte(`{
"incident": {
"type": "incident",
**"title": "Docker is down on."+hostname,**
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonStr))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Accept", "application/vnd.pagerduty+json;version=2")
req.Header.Set("From", "shinoda@wathever.com")
req.Header.Set("Authorization", "Token token="+token)
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
return resp.Status
}
func main() {
fmt.Println(pager())
}
I am not very familiar with go, in python I can do this easily and I dont know the proper way to do this in golang.
If someone could explain me, I would be grateful.
thanks in advance.
json

json

edited Nov 11 at 21:08


Shudipta Sharma
1,061212
1,061212
asked Nov 11 at 13:59


Dimitri Berveglieri
64
64
I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
1
I'd like to point out thatos.Hostname()
is a function.
– ssemilla
Nov 11 at 14:42
add a comment |
I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
1
I'd like to point out thatos.Hostname()
is a function.
– ssemilla
Nov 11 at 14:42
I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
1
1
I'd like to point out that
os.Hostname()
is a function.– ssemilla
Nov 11 at 14:42
I'd like to point out that
os.Hostname()
is a function.– ssemilla
Nov 11 at 14:42
add a comment |
4 Answers
4
active
oldest
votes
up vote
1
down vote
make a struct in go to represent the json
type
Incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"service"`
Priority struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body struct {
Type string `json:"type"`
Details string `json:"details"`
} `json:"body"`
EscalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"escalation_policy"`
}
then do something like
hostname,err:=os.Hostname()
if (err !=nil) {
panic(err)
}
incident:=Incident{ Type: "incident",
Title: fmt.Sprintf("Docker is down on %s", hostname),
//...etc etc add all other fields
req, err := http.NewRequest("POST", url, json.Marshal(incident))
The workaround for declaring structs inside structs seems a bit clumsy (sorry)
Service: struct {
ID string `json:"id"`
Type string `json:"type"`
}{
ID: "asdf",
Type: "ABC",
},
This other answer https://stackoverflow.com/a/53255390/1153938 shows how to split the structs inside the Incident struct and is a cleaner way of doing it
I'll leave this answer here because it might be of interest how to declare it in this way
If you are only calling json.Unmarshal
then this way would be fine but for declaring stuff in a program as you need to do, perhaps not the best
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
add a comment |
up vote
0
down vote
The problem is as simple as properly quoting strings. This should solve your immediate problem:
jsonStr := byte(`{
"incident": {
"type": "incident",
"title": "Docker is down on `+hostname+`",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
But a much better approach, as suggested by @Vorsprung, is to use a proper Go data structure, and marshal it to JSON.
add a comment |
up vote
0
down vote
Try this solution:
hostname, err := os.Hostname()
if err != nil {
// handle err
}
indent := fmt.Sprintf(`{
"incident": {
"type": "incident",
"title": "Docker is down on %s",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`, hostname)
jsonStr := byte(indent)
add a comment |
up vote
0
down vote
Building on @Vorsprung's response, unless I'm mistaken you may want to define the structs a little differently to avoid the error you are getting based on your response
Once you've initialized and populated required properties on incident you should be able to POST the object after performing a json.Marshal() on the object.
jsonObject, _ := json.MarshalIndent(someIncident, "", "t")
If the structs are to be used outside of this package you may want to change the names to uppercase to allow exportability.
type incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service service `json:"service"`
Priority priority `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body body `json:"body"`
EscalationPolicy escalationPolicy `json:"escalation_policy"`
}
type service struct {
ID string `json:"id"`
Type string `json:"type"`
}
type priority struct {
ID string `json:"id"`
Type string `json:"type"`
}
type body struct {
Type string `json:"type"`
Details string `json:"details"`
}
type escalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
}
Initialization of the object:
someIncident := incident{
Type: "SomeType",
Title: "SomeTitle",
Service: service{
ID: "SomeId",
Type: "SomeType"},
Priority: priority{
ID: "SomeId",
Type: "SomeType"},
Urgency: "SomeUrgency",
IncidentKey: "SomeKey",
Body: body{
Type: "SomeType",
Details: "SomeDetails"},
EscalationPolicy: escalationPolicy{
ID: "SomeId",
Type: "SomeType"}}
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type stringjson:"type"
} type priority struct { ID stringjson:"id"
Type stringjson:"type"
} type body struct { Type stringjson:"type"
Details stringjson:"details"
} type escalationPolicy struct { ID stringjson:"id"
Type stringjson:"type"
}`
– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title stringjson:"title"
Service servicejson:"service"
Priority priorityjson:"priority"
Urgency stringjson:"urgency"
IncidentKey stringjson:"incident_key"
Body bodyjson:"body"
EscalationPolicy escalationPolicyjson:"escalation_policy"
} type master struct { Incident incidentjson:"incident"
}`
– Dimitri Berveglieri
Nov 13 at 13:26
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
make a struct in go to represent the json
type
Incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"service"`
Priority struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body struct {
Type string `json:"type"`
Details string `json:"details"`
} `json:"body"`
EscalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"escalation_policy"`
}
then do something like
hostname,err:=os.Hostname()
if (err !=nil) {
panic(err)
}
incident:=Incident{ Type: "incident",
Title: fmt.Sprintf("Docker is down on %s", hostname),
//...etc etc add all other fields
req, err := http.NewRequest("POST", url, json.Marshal(incident))
The workaround for declaring structs inside structs seems a bit clumsy (sorry)
Service: struct {
ID string `json:"id"`
Type string `json:"type"`
}{
ID: "asdf",
Type: "ABC",
},
This other answer https://stackoverflow.com/a/53255390/1153938 shows how to split the structs inside the Incident struct and is a cleaner way of doing it
I'll leave this answer here because it might be of interest how to declare it in this way
If you are only calling json.Unmarshal
then this way would be fine but for declaring stuff in a program as you need to do, perhaps not the best
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
add a comment |
up vote
1
down vote
make a struct in go to represent the json
type
Incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"service"`
Priority struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body struct {
Type string `json:"type"`
Details string `json:"details"`
} `json:"body"`
EscalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"escalation_policy"`
}
then do something like
hostname,err:=os.Hostname()
if (err !=nil) {
panic(err)
}
incident:=Incident{ Type: "incident",
Title: fmt.Sprintf("Docker is down on %s", hostname),
//...etc etc add all other fields
req, err := http.NewRequest("POST", url, json.Marshal(incident))
The workaround for declaring structs inside structs seems a bit clumsy (sorry)
Service: struct {
ID string `json:"id"`
Type string `json:"type"`
}{
ID: "asdf",
Type: "ABC",
},
This other answer https://stackoverflow.com/a/53255390/1153938 shows how to split the structs inside the Incident struct and is a cleaner way of doing it
I'll leave this answer here because it might be of interest how to declare it in this way
If you are only calling json.Unmarshal
then this way would be fine but for declaring stuff in a program as you need to do, perhaps not the best
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
add a comment |
up vote
1
down vote
up vote
1
down vote
make a struct in go to represent the json
type
Incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"service"`
Priority struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body struct {
Type string `json:"type"`
Details string `json:"details"`
} `json:"body"`
EscalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"escalation_policy"`
}
then do something like
hostname,err:=os.Hostname()
if (err !=nil) {
panic(err)
}
incident:=Incident{ Type: "incident",
Title: fmt.Sprintf("Docker is down on %s", hostname),
//...etc etc add all other fields
req, err := http.NewRequest("POST", url, json.Marshal(incident))
The workaround for declaring structs inside structs seems a bit clumsy (sorry)
Service: struct {
ID string `json:"id"`
Type string `json:"type"`
}{
ID: "asdf",
Type: "ABC",
},
This other answer https://stackoverflow.com/a/53255390/1153938 shows how to split the structs inside the Incident struct and is a cleaner way of doing it
I'll leave this answer here because it might be of interest how to declare it in this way
If you are only calling json.Unmarshal
then this way would be fine but for declaring stuff in a program as you need to do, perhaps not the best
make a struct in go to represent the json
type
Incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"service"`
Priority struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body struct {
Type string `json:"type"`
Details string `json:"details"`
} `json:"body"`
EscalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
} `json:"escalation_policy"`
}
then do something like
hostname,err:=os.Hostname()
if (err !=nil) {
panic(err)
}
incident:=Incident{ Type: "incident",
Title: fmt.Sprintf("Docker is down on %s", hostname),
//...etc etc add all other fields
req, err := http.NewRequest("POST", url, json.Marshal(incident))
The workaround for declaring structs inside structs seems a bit clumsy (sorry)
Service: struct {
ID string `json:"id"`
Type string `json:"type"`
}{
ID: "asdf",
Type: "ABC",
},
This other answer https://stackoverflow.com/a/53255390/1153938 shows how to split the structs inside the Incident struct and is a cleaner way of doing it
I'll leave this answer here because it might be of interest how to declare it in this way
If you are only calling json.Unmarshal
then this way would be fine but for declaring stuff in a program as you need to do, perhaps not the best
edited Nov 12 at 10:13
answered Nov 11 at 14:37


Vorsprung
21.8k31941
21.8k31941
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
add a comment |
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
I have created the structure as you suggested but I am receiving that the other structs are undefined and I don't know why.
– Dimitri Berveglieri
Nov 11 at 22:56
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
incident := Incident{ Type: "incident", Title: fmt.Sprintf("Docker is down on %s", hostname), Service{ ID: "PZXSH36", Type: "service_reference", }, Priority{ ID: "P53ZZH5", Type: "priority_reference", }, Urgency: "high", IncidentKey: "baf7cf21b1da41b4b0221008339ff357", Body{ Type: "incident_body", Details: "Docker service is down or container stopped", }, EscalationPolicy{ ID: "PI772OH", Type: "escalation_policy_reference", }, }
– Dimitri Berveglieri
Nov 11 at 22:58
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
Service, Priority, Body and EscalationPolicy are being marked as undefined.
– Dimitri Berveglieri
Nov 11 at 23:00
add a comment |
up vote
0
down vote
The problem is as simple as properly quoting strings. This should solve your immediate problem:
jsonStr := byte(`{
"incident": {
"type": "incident",
"title": "Docker is down on `+hostname+`",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
But a much better approach, as suggested by @Vorsprung, is to use a proper Go data structure, and marshal it to JSON.
add a comment |
up vote
0
down vote
The problem is as simple as properly quoting strings. This should solve your immediate problem:
jsonStr := byte(`{
"incident": {
"type": "incident",
"title": "Docker is down on `+hostname+`",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
But a much better approach, as suggested by @Vorsprung, is to use a proper Go data structure, and marshal it to JSON.
add a comment |
up vote
0
down vote
up vote
0
down vote
The problem is as simple as properly quoting strings. This should solve your immediate problem:
jsonStr := byte(`{
"incident": {
"type": "incident",
"title": "Docker is down on `+hostname+`",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
But a much better approach, as suggested by @Vorsprung, is to use a proper Go data structure, and marshal it to JSON.
The problem is as simple as properly quoting strings. This should solve your immediate problem:
jsonStr := byte(`{
"incident": {
"type": "incident",
"title": "Docker is down on `+hostname+`",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`)
But a much better approach, as suggested by @Vorsprung, is to use a proper Go data structure, and marshal it to JSON.
answered Nov 11 at 14:41
Flimzy
36.6k96496
36.6k96496
add a comment |
add a comment |
up vote
0
down vote
Try this solution:
hostname, err := os.Hostname()
if err != nil {
// handle err
}
indent := fmt.Sprintf(`{
"incident": {
"type": "incident",
"title": "Docker is down on %s",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`, hostname)
jsonStr := byte(indent)
add a comment |
up vote
0
down vote
Try this solution:
hostname, err := os.Hostname()
if err != nil {
// handle err
}
indent := fmt.Sprintf(`{
"incident": {
"type": "incident",
"title": "Docker is down on %s",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`, hostname)
jsonStr := byte(indent)
add a comment |
up vote
0
down vote
up vote
0
down vote
Try this solution:
hostname, err := os.Hostname()
if err != nil {
// handle err
}
indent := fmt.Sprintf(`{
"incident": {
"type": "incident",
"title": "Docker is down on %s",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`, hostname)
jsonStr := byte(indent)
Try this solution:
hostname, err := os.Hostname()
if err != nil {
// handle err
}
indent := fmt.Sprintf(`{
"incident": {
"type": "incident",
"title": "Docker is down on %s",
"service": {
"id": "PWIXJZS",
"type": "service_reference"
},
"priority": {
"id": "P53ZZH5",
"type": "priority_reference"
},
"urgency": "high",
"incident_key": "baf7cf21b1da41b4b0221008339ff357",
"body": {
"type": "incident_body",
"details": "A disk is getting full on this machine. You should investigate what is causing the disk to fill, and ensure that there is an automated process in place for ensuring data is rotated (eg. logs should have logrotate around them). If data is expected to stay on this disk forever, you should start planning to scale up to a larger disk."
},
"escalation_policy": {
"id": "PT20YPA",
"type": "escalation_policy_reference"
}
}
}`, hostname)
jsonStr := byte(indent)
answered Nov 11 at 16:39


Shudipta Sharma
1,061212
1,061212
add a comment |
add a comment |
up vote
0
down vote
Building on @Vorsprung's response, unless I'm mistaken you may want to define the structs a little differently to avoid the error you are getting based on your response
Once you've initialized and populated required properties on incident you should be able to POST the object after performing a json.Marshal() on the object.
jsonObject, _ := json.MarshalIndent(someIncident, "", "t")
If the structs are to be used outside of this package you may want to change the names to uppercase to allow exportability.
type incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service service `json:"service"`
Priority priority `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body body `json:"body"`
EscalationPolicy escalationPolicy `json:"escalation_policy"`
}
type service struct {
ID string `json:"id"`
Type string `json:"type"`
}
type priority struct {
ID string `json:"id"`
Type string `json:"type"`
}
type body struct {
Type string `json:"type"`
Details string `json:"details"`
}
type escalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
}
Initialization of the object:
someIncident := incident{
Type: "SomeType",
Title: "SomeTitle",
Service: service{
ID: "SomeId",
Type: "SomeType"},
Priority: priority{
ID: "SomeId",
Type: "SomeType"},
Urgency: "SomeUrgency",
IncidentKey: "SomeKey",
Body: body{
Type: "SomeType",
Details: "SomeDetails"},
EscalationPolicy: escalationPolicy{
ID: "SomeId",
Type: "SomeType"}}
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type stringjson:"type"
} type priority struct { ID stringjson:"id"
Type stringjson:"type"
} type body struct { Type stringjson:"type"
Details stringjson:"details"
} type escalationPolicy struct { ID stringjson:"id"
Type stringjson:"type"
}`
– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title stringjson:"title"
Service servicejson:"service"
Priority priorityjson:"priority"
Urgency stringjson:"urgency"
IncidentKey stringjson:"incident_key"
Body bodyjson:"body"
EscalationPolicy escalationPolicyjson:"escalation_policy"
} type master struct { Incident incidentjson:"incident"
}`
– Dimitri Berveglieri
Nov 13 at 13:26
add a comment |
up vote
0
down vote
Building on @Vorsprung's response, unless I'm mistaken you may want to define the structs a little differently to avoid the error you are getting based on your response
Once you've initialized and populated required properties on incident you should be able to POST the object after performing a json.Marshal() on the object.
jsonObject, _ := json.MarshalIndent(someIncident, "", "t")
If the structs are to be used outside of this package you may want to change the names to uppercase to allow exportability.
type incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service service `json:"service"`
Priority priority `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body body `json:"body"`
EscalationPolicy escalationPolicy `json:"escalation_policy"`
}
type service struct {
ID string `json:"id"`
Type string `json:"type"`
}
type priority struct {
ID string `json:"id"`
Type string `json:"type"`
}
type body struct {
Type string `json:"type"`
Details string `json:"details"`
}
type escalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
}
Initialization of the object:
someIncident := incident{
Type: "SomeType",
Title: "SomeTitle",
Service: service{
ID: "SomeId",
Type: "SomeType"},
Priority: priority{
ID: "SomeId",
Type: "SomeType"},
Urgency: "SomeUrgency",
IncidentKey: "SomeKey",
Body: body{
Type: "SomeType",
Details: "SomeDetails"},
EscalationPolicy: escalationPolicy{
ID: "SomeId",
Type: "SomeType"}}
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type stringjson:"type"
} type priority struct { ID stringjson:"id"
Type stringjson:"type"
} type body struct { Type stringjson:"type"
Details stringjson:"details"
} type escalationPolicy struct { ID stringjson:"id"
Type stringjson:"type"
}`
– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title stringjson:"title"
Service servicejson:"service"
Priority priorityjson:"priority"
Urgency stringjson:"urgency"
IncidentKey stringjson:"incident_key"
Body bodyjson:"body"
EscalationPolicy escalationPolicyjson:"escalation_policy"
} type master struct { Incident incidentjson:"incident"
}`
– Dimitri Berveglieri
Nov 13 at 13:26
add a comment |
up vote
0
down vote
up vote
0
down vote
Building on @Vorsprung's response, unless I'm mistaken you may want to define the structs a little differently to avoid the error you are getting based on your response
Once you've initialized and populated required properties on incident you should be able to POST the object after performing a json.Marshal() on the object.
jsonObject, _ := json.MarshalIndent(someIncident, "", "t")
If the structs are to be used outside of this package you may want to change the names to uppercase to allow exportability.
type incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service service `json:"service"`
Priority priority `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body body `json:"body"`
EscalationPolicy escalationPolicy `json:"escalation_policy"`
}
type service struct {
ID string `json:"id"`
Type string `json:"type"`
}
type priority struct {
ID string `json:"id"`
Type string `json:"type"`
}
type body struct {
Type string `json:"type"`
Details string `json:"details"`
}
type escalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
}
Initialization of the object:
someIncident := incident{
Type: "SomeType",
Title: "SomeTitle",
Service: service{
ID: "SomeId",
Type: "SomeType"},
Priority: priority{
ID: "SomeId",
Type: "SomeType"},
Urgency: "SomeUrgency",
IncidentKey: "SomeKey",
Body: body{
Type: "SomeType",
Details: "SomeDetails"},
EscalationPolicy: escalationPolicy{
ID: "SomeId",
Type: "SomeType"}}
Building on @Vorsprung's response, unless I'm mistaken you may want to define the structs a little differently to avoid the error you are getting based on your response
Once you've initialized and populated required properties on incident you should be able to POST the object after performing a json.Marshal() on the object.
jsonObject, _ := json.MarshalIndent(someIncident, "", "t")
If the structs are to be used outside of this package you may want to change the names to uppercase to allow exportability.
type incident struct {
Type string `json:"type"`
Title string `json:"title"`
Service service `json:"service"`
Priority priority `json:"priority"`
Urgency string `json:"urgency"`
IncidentKey string `json:"incident_key"`
Body body `json:"body"`
EscalationPolicy escalationPolicy `json:"escalation_policy"`
}
type service struct {
ID string `json:"id"`
Type string `json:"type"`
}
type priority struct {
ID string `json:"id"`
Type string `json:"type"`
}
type body struct {
Type string `json:"type"`
Details string `json:"details"`
}
type escalationPolicy struct {
ID string `json:"id"`
Type string `json:"type"`
}
Initialization of the object:
someIncident := incident{
Type: "SomeType",
Title: "SomeTitle",
Service: service{
ID: "SomeId",
Type: "SomeType"},
Priority: priority{
ID: "SomeId",
Type: "SomeType"},
Urgency: "SomeUrgency",
IncidentKey: "SomeKey",
Body: body{
Type: "SomeType",
Details: "SomeDetails"},
EscalationPolicy: escalationPolicy{
ID: "SomeId",
Type: "SomeType"}}
edited Nov 12 at 3:10
answered Nov 12 at 2:50
Elevennn
113
113
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type stringjson:"type"
} type priority struct { ID stringjson:"id"
Type stringjson:"type"
} type body struct { Type stringjson:"type"
Details stringjson:"details"
} type escalationPolicy struct { ID stringjson:"id"
Type stringjson:"type"
}`
– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title stringjson:"title"
Service servicejson:"service"
Priority priorityjson:"priority"
Urgency stringjson:"urgency"
IncidentKey stringjson:"incident_key"
Body bodyjson:"body"
EscalationPolicy escalationPolicyjson:"escalation_policy"
} type master struct { Incident incidentjson:"incident"
}`
– Dimitri Berveglieri
Nov 13 at 13:26
add a comment |
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type stringjson:"type"
} type priority struct { ID stringjson:"id"
Type stringjson:"type"
} type body struct { Type stringjson:"type"
Details stringjson:"details"
} type escalationPolicy struct { ID stringjson:"id"
Type stringjson:"type"
}`
– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title stringjson:"title"
Service servicejson:"service"
Priority priorityjson:"priority"
Urgency stringjson:"urgency"
IncidentKey stringjson:"incident_key"
Body bodyjson:"body"
EscalationPolicy escalationPolicyjson:"escalation_policy"
} type master struct { Incident incidentjson:"incident"
}`
– Dimitri Berveglieri
Nov 13 at 13:26
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
thank you very much, thanks to your explanation I was able to understand the concept of struct. After I created my struct my POST worked perfectly.
– Dimitri Berveglieri
Nov 13 at 13:24
type service struct { ID string
json:"id"` Type string json:"type"
} type priority struct { ID string json:"id"
Type string json:"type"
} type body struct { Type string json:"type"
Details string json:"details"
} type escalationPolicy struct { ID string json:"id"
Type string json:"type"
}`– Dimitri Berveglieri
Nov 13 at 13:25
type service struct { ID string
json:"id"` Type string json:"type"
} type priority struct { ID string json:"id"
Type string json:"type"
} type body struct { Type string json:"type"
Details string json:"details"
} type escalationPolicy struct { ID string json:"id"
Type string json:"type"
}`– Dimitri Berveglieri
Nov 13 at 13:25
type incident struct { Type string
json:"type"` Title string json:"title"
Service service json:"service"
Priority priority json:"priority"
Urgency string json:"urgency"
IncidentKey string json:"incident_key"
Body body json:"body"
EscalationPolicy escalationPolicy json:"escalation_policy"
} type master struct { Incident incident json:"incident"
}`– Dimitri Berveglieri
Nov 13 at 13:26
type incident struct { Type string
json:"type"` Title string json:"title"
Service service json:"service"
Priority priority json:"priority"
Urgency string json:"urgency"
IncidentKey string json:"incident_key"
Body body json:"body"
EscalationPolicy escalationPolicy json:"escalation_policy"
} type master struct { Incident incident json:"incident"
}`– Dimitri Berveglieri
Nov 13 at 13:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249485%2finsert-a-variable-inside-a-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WLKaO0G Lo8zFjaxRbIzHNW 0b VM RBRR,Oy M,I,S1Qm84yxfAR e1rVSNV
I'm afraid I don't understand your question. You appear to be asking two unrelated things: How to map a variable to a json key, and how to append a string. Which specific problem are you having?
– Flimzy
Nov 11 at 14:02
It would also help greatly to format your code to be readable.
– Flimzy
Nov 11 at 14:03
@Flimzy, sorry I will try to make me more clear. "incident": { "type": "incident", "title": "Docker down on "+hostname } in this field title I would like to append my variable hostname, but this is being interpreted as a normal string and its value is not being printed. this is being interpreted literally as +hostname and not as server1.local.
– Dimitri Berveglieri
Nov 11 at 14:09
1
I'd like to point out that
os.Hostname()
is a function.– ssemilla
Nov 11 at 14:42