How to get warnings for wrong return types in PyCharm?
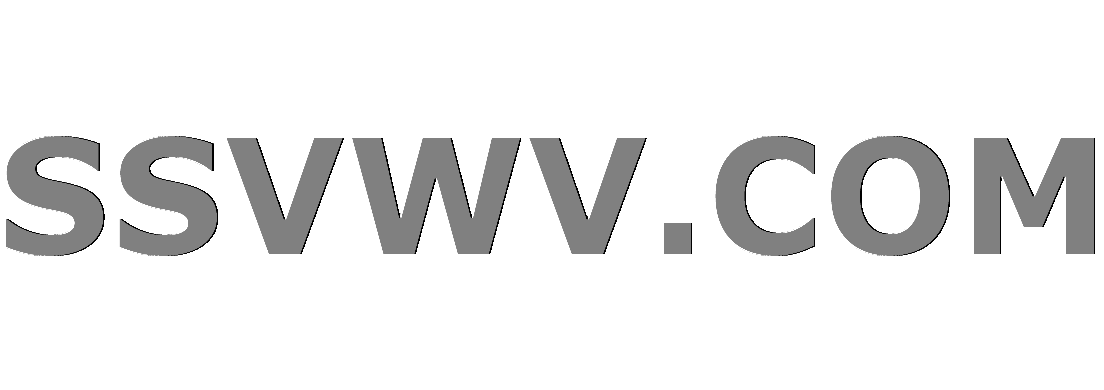
Multi tool use
In a Python 3.7.1 project a method is defined with return type incompatible with the result type. However, the IDE, PyCharm 2018.2, gives no warnings. Here's a code example:
import numpy as np
from dataclasses import dataclass
import typing
# Definitions:
@dataclass(order=True)
class Theta:
vector: np.ndarray
def a(self) -> typing.AnyStr:
return self.vector.size
def b(self) -> str:
return self.vector.size
# Client code:
x = np.array([1, 2])
s = Theta(x).a().capitalize() # runtime errors
u = Theta(x).b().capitalize()
Is there a way to enforce type warnings, both in the definitions and the client code?
Note: There're several questions about Python type hints on SO, e.g., Pycharm strange warning when type hinting. However, they seem to focus on a different aspect.
python pycharm
add a comment |
In a Python 3.7.1 project a method is defined with return type incompatible with the result type. However, the IDE, PyCharm 2018.2, gives no warnings. Here's a code example:
import numpy as np
from dataclasses import dataclass
import typing
# Definitions:
@dataclass(order=True)
class Theta:
vector: np.ndarray
def a(self) -> typing.AnyStr:
return self.vector.size
def b(self) -> str:
return self.vector.size
# Client code:
x = np.array([1, 2])
s = Theta(x).a().capitalize() # runtime errors
u = Theta(x).b().capitalize()
Is there a way to enforce type warnings, both in the definitions and the client code?
Note: There're several questions about Python type hints on SO, e.g., Pycharm strange warning when type hinting. However, they seem to focus on a different aspect.
python pycharm
add a comment |
In a Python 3.7.1 project a method is defined with return type incompatible with the result type. However, the IDE, PyCharm 2018.2, gives no warnings. Here's a code example:
import numpy as np
from dataclasses import dataclass
import typing
# Definitions:
@dataclass(order=True)
class Theta:
vector: np.ndarray
def a(self) -> typing.AnyStr:
return self.vector.size
def b(self) -> str:
return self.vector.size
# Client code:
x = np.array([1, 2])
s = Theta(x).a().capitalize() # runtime errors
u = Theta(x).b().capitalize()
Is there a way to enforce type warnings, both in the definitions and the client code?
Note: There're several questions about Python type hints on SO, e.g., Pycharm strange warning when type hinting. However, they seem to focus on a different aspect.
python pycharm
In a Python 3.7.1 project a method is defined with return type incompatible with the result type. However, the IDE, PyCharm 2018.2, gives no warnings. Here's a code example:
import numpy as np
from dataclasses import dataclass
import typing
# Definitions:
@dataclass(order=True)
class Theta:
vector: np.ndarray
def a(self) -> typing.AnyStr:
return self.vector.size
def b(self) -> str:
return self.vector.size
# Client code:
x = np.array([1, 2])
s = Theta(x).a().capitalize() # runtime errors
u = Theta(x).b().capitalize()
Is there a way to enforce type warnings, both in the definitions and the client code?
Note: There're several questions about Python type hints on SO, e.g., Pycharm strange warning when type hinting. However, they seem to focus on a different aspect.
python pycharm
python pycharm
asked Nov 12 at 17:41


Tupolev._
30829
30829
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
typing.AnyStr
is a type variable. It means that it will be inferred from passed parameter or containing class. In your case neither class, nor method does not use this type variable and as a result inferred a
return type is Any
.
What about the other method, with signature-> str
? It's the same situation with type warnings.
– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click onsize
will opensize
definition where you could see that there is no mention about type ofsize
. Type hints github.com/numpy/numpy-stubs are under development at the moment.
– user2235698
Nov 19 at 16:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267401%2fhow-to-get-warnings-for-wrong-return-types-in-pycharm%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
typing.AnyStr
is a type variable. It means that it will be inferred from passed parameter or containing class. In your case neither class, nor method does not use this type variable and as a result inferred a
return type is Any
.
What about the other method, with signature-> str
? It's the same situation with type warnings.
– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click onsize
will opensize
definition where you could see that there is no mention about type ofsize
. Type hints github.com/numpy/numpy-stubs are under development at the moment.
– user2235698
Nov 19 at 16:53
add a comment |
typing.AnyStr
is a type variable. It means that it will be inferred from passed parameter or containing class. In your case neither class, nor method does not use this type variable and as a result inferred a
return type is Any
.
What about the other method, with signature-> str
? It's the same situation with type warnings.
– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click onsize
will opensize
definition where you could see that there is no mention about type ofsize
. Type hints github.com/numpy/numpy-stubs are under development at the moment.
– user2235698
Nov 19 at 16:53
add a comment |
typing.AnyStr
is a type variable. It means that it will be inferred from passed parameter or containing class. In your case neither class, nor method does not use this type variable and as a result inferred a
return type is Any
.
typing.AnyStr
is a type variable. It means that it will be inferred from passed parameter or containing class. In your case neither class, nor method does not use this type variable and as a result inferred a
return type is Any
.
answered Nov 19 at 15:59
user2235698
3,19011015
3,19011015
What about the other method, with signature-> str
? It's the same situation with type warnings.
– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click onsize
will opensize
definition where you could see that there is no mention about type ofsize
. Type hints github.com/numpy/numpy-stubs are under development at the moment.
– user2235698
Nov 19 at 16:53
add a comment |
What about the other method, with signature-> str
? It's the same situation with type warnings.
– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click onsize
will opensize
definition where you could see that there is no mention about type ofsize
. Type hints github.com/numpy/numpy-stubs are under development at the moment.
– user2235698
Nov 19 at 16:53
What about the other method, with signature
-> str
? It's the same situation with type warnings.– Tupolev._
Nov 19 at 16:42
What about the other method, with signature
-> str
? It's the same situation with type warnings.– Tupolev._
Nov 19 at 16:42
@Tupolev._ Ctrl+click on
size
will open size
definition where you could see that there is no mention about type of size
. Type hints github.com/numpy/numpy-stubs are under development at the moment.– user2235698
Nov 19 at 16:53
@Tupolev._ Ctrl+click on
size
will open size
definition where you could see that there is no mention about type of size
. Type hints github.com/numpy/numpy-stubs are under development at the moment.– user2235698
Nov 19 at 16:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267401%2fhow-to-get-warnings-for-wrong-return-types-in-pycharm%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Bi,RXlmf,gDtzrK0BR,AgcfI,u,22arQtT2A6,sEdvwy 2e2ItViMKOwuzgucSK9TufCpuR,en Xq1kD PPFxte2sM6U33lm,qrq,oMt1,BaEP8n