How to create a admin state in react using firebase?
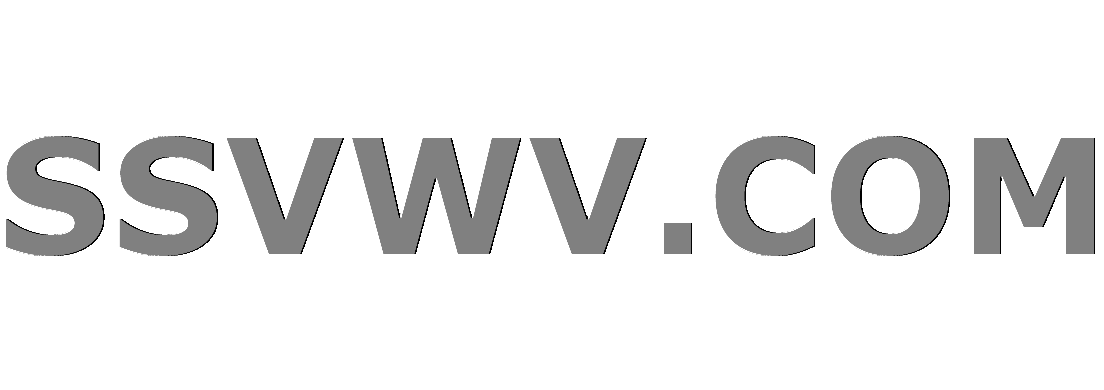
Multi tool use
My web application uses authentication through firebase. I have an appbar which shows buttons based if an user is logged in or not. I want to show another button on the appbar if that user is an admin. I am wondering if it is possible to use a user's uid to make them an admin. Below is my code so far.
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import { MuiThemeProvider, createMuiTheme } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import logIn from '../../auth/login.js'
import logOut from '../../auth/logout.js'
import { Link } from 'react-router-dom'
import profilePage from '../pages/profilePage'
import homePage from '../pages/homePage'
import requestService from '../pages/requestService'
import requestedServices from '../pages/requestedServices'
import { Route } from 'react-router-dom';
import firebase, { auth, provider } from '../../config/firebaseConfig.js'
// All the following keys are optional.
// We try our best to provide a great default value.
const theme = createMuiTheme({
typography: {
useNextVariants: true,
},
palette: {
primary: {
main : '#196f3d'
},
secondary: {
main : '#f7f9f9'
},
// error: will use the default color
},
});
const styles = {
root: {
flexGrow: 1,
},
grow: {
flexGrow: 1,
},
};
class ButtonAppBar extends Component {
static defaultProps = {
classes: PropTypes.object.isRequired,
};
state = { isLoggedIn : false }
state = { isAdmin : false }
componentDidMount = () => {
firebase.auth().onAuthStateChanged(user => {
this.setState({ isLoggedIn: !!user })
this.setState({ isAdmin : firebase.auth().user.uid='8uhMGWgWZENwT7S2YFECOlSFrHD2'})
console.log("user", user)
})
}
render() {
const { classes } = this.props;
return (
<MuiThemeProvider theme={theme}>
<div className={classes.root}>
<AppBar position="static" color='primary' >
<Toolbar>
<Typography variant="h5" color="secondary" className={classes.grow}>
Greene Dog Walking
</Typography>
{this.state.isAdmin ? (
<div>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
</div>
) : (
) }
{this.state.isLoggedIn ? (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Link to={'./service'}>
<Button color="secondary">Request Service</Button>
</Link>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
<Link to={'./profile'}>
<Button color="secondary">Profile</Button>
</Link>
<Button onClick={logOut} color="secondary">Logout</Button>
</div>
) : (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Button onClick={logIn} color="secondary">Login</Button>
</div>
) }
</Toolbar>
</AppBar>
</div>
</MuiThemeProvider>
);
}
}
export default withStyles(styles)(ButtonAppBar);
javascript reactjs

add a comment |
My web application uses authentication through firebase. I have an appbar which shows buttons based if an user is logged in or not. I want to show another button on the appbar if that user is an admin. I am wondering if it is possible to use a user's uid to make them an admin. Below is my code so far.
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import { MuiThemeProvider, createMuiTheme } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import logIn from '../../auth/login.js'
import logOut from '../../auth/logout.js'
import { Link } from 'react-router-dom'
import profilePage from '../pages/profilePage'
import homePage from '../pages/homePage'
import requestService from '../pages/requestService'
import requestedServices from '../pages/requestedServices'
import { Route } from 'react-router-dom';
import firebase, { auth, provider } from '../../config/firebaseConfig.js'
// All the following keys are optional.
// We try our best to provide a great default value.
const theme = createMuiTheme({
typography: {
useNextVariants: true,
},
palette: {
primary: {
main : '#196f3d'
},
secondary: {
main : '#f7f9f9'
},
// error: will use the default color
},
});
const styles = {
root: {
flexGrow: 1,
},
grow: {
flexGrow: 1,
},
};
class ButtonAppBar extends Component {
static defaultProps = {
classes: PropTypes.object.isRequired,
};
state = { isLoggedIn : false }
state = { isAdmin : false }
componentDidMount = () => {
firebase.auth().onAuthStateChanged(user => {
this.setState({ isLoggedIn: !!user })
this.setState({ isAdmin : firebase.auth().user.uid='8uhMGWgWZENwT7S2YFECOlSFrHD2'})
console.log("user", user)
})
}
render() {
const { classes } = this.props;
return (
<MuiThemeProvider theme={theme}>
<div className={classes.root}>
<AppBar position="static" color='primary' >
<Toolbar>
<Typography variant="h5" color="secondary" className={classes.grow}>
Greene Dog Walking
</Typography>
{this.state.isAdmin ? (
<div>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
</div>
) : (
) }
{this.state.isLoggedIn ? (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Link to={'./service'}>
<Button color="secondary">Request Service</Button>
</Link>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
<Link to={'./profile'}>
<Button color="secondary">Profile</Button>
</Link>
<Button onClick={logOut} color="secondary">Logout</Button>
</div>
) : (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Button onClick={logIn} color="secondary">Login</Button>
</div>
) }
</Toolbar>
</AppBar>
</div>
</MuiThemeProvider>
);
}
}
export default withStyles(styles)(ButtonAppBar);
javascript reactjs

add a comment |
My web application uses authentication through firebase. I have an appbar which shows buttons based if an user is logged in or not. I want to show another button on the appbar if that user is an admin. I am wondering if it is possible to use a user's uid to make them an admin. Below is my code so far.
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import { MuiThemeProvider, createMuiTheme } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import logIn from '../../auth/login.js'
import logOut from '../../auth/logout.js'
import { Link } from 'react-router-dom'
import profilePage from '../pages/profilePage'
import homePage from '../pages/homePage'
import requestService from '../pages/requestService'
import requestedServices from '../pages/requestedServices'
import { Route } from 'react-router-dom';
import firebase, { auth, provider } from '../../config/firebaseConfig.js'
// All the following keys are optional.
// We try our best to provide a great default value.
const theme = createMuiTheme({
typography: {
useNextVariants: true,
},
palette: {
primary: {
main : '#196f3d'
},
secondary: {
main : '#f7f9f9'
},
// error: will use the default color
},
});
const styles = {
root: {
flexGrow: 1,
},
grow: {
flexGrow: 1,
},
};
class ButtonAppBar extends Component {
static defaultProps = {
classes: PropTypes.object.isRequired,
};
state = { isLoggedIn : false }
state = { isAdmin : false }
componentDidMount = () => {
firebase.auth().onAuthStateChanged(user => {
this.setState({ isLoggedIn: !!user })
this.setState({ isAdmin : firebase.auth().user.uid='8uhMGWgWZENwT7S2YFECOlSFrHD2'})
console.log("user", user)
})
}
render() {
const { classes } = this.props;
return (
<MuiThemeProvider theme={theme}>
<div className={classes.root}>
<AppBar position="static" color='primary' >
<Toolbar>
<Typography variant="h5" color="secondary" className={classes.grow}>
Greene Dog Walking
</Typography>
{this.state.isAdmin ? (
<div>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
</div>
) : (
) }
{this.state.isLoggedIn ? (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Link to={'./service'}>
<Button color="secondary">Request Service</Button>
</Link>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
<Link to={'./profile'}>
<Button color="secondary">Profile</Button>
</Link>
<Button onClick={logOut} color="secondary">Logout</Button>
</div>
) : (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Button onClick={logIn} color="secondary">Login</Button>
</div>
) }
</Toolbar>
</AppBar>
</div>
</MuiThemeProvider>
);
}
}
export default withStyles(styles)(ButtonAppBar);
javascript reactjs

My web application uses authentication through firebase. I have an appbar which shows buttons based if an user is logged in or not. I want to show another button on the appbar if that user is an admin. I am wondering if it is possible to use a user's uid to make them an admin. Below is my code so far.
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import { MuiThemeProvider, createMuiTheme } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import logIn from '../../auth/login.js'
import logOut from '../../auth/logout.js'
import { Link } from 'react-router-dom'
import profilePage from '../pages/profilePage'
import homePage from '../pages/homePage'
import requestService from '../pages/requestService'
import requestedServices from '../pages/requestedServices'
import { Route } from 'react-router-dom';
import firebase, { auth, provider } from '../../config/firebaseConfig.js'
// All the following keys are optional.
// We try our best to provide a great default value.
const theme = createMuiTheme({
typography: {
useNextVariants: true,
},
palette: {
primary: {
main : '#196f3d'
},
secondary: {
main : '#f7f9f9'
},
// error: will use the default color
},
});
const styles = {
root: {
flexGrow: 1,
},
grow: {
flexGrow: 1,
},
};
class ButtonAppBar extends Component {
static defaultProps = {
classes: PropTypes.object.isRequired,
};
state = { isLoggedIn : false }
state = { isAdmin : false }
componentDidMount = () => {
firebase.auth().onAuthStateChanged(user => {
this.setState({ isLoggedIn: !!user })
this.setState({ isAdmin : firebase.auth().user.uid='8uhMGWgWZENwT7S2YFECOlSFrHD2'})
console.log("user", user)
})
}
render() {
const { classes } = this.props;
return (
<MuiThemeProvider theme={theme}>
<div className={classes.root}>
<AppBar position="static" color='primary' >
<Toolbar>
<Typography variant="h5" color="secondary" className={classes.grow}>
Greene Dog Walking
</Typography>
{this.state.isAdmin ? (
<div>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
</div>
) : (
) }
{this.state.isLoggedIn ? (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Link to={'./service'}>
<Button color="secondary">Request Service</Button>
</Link>
<Link to={'./requestedservices'}>
<Button color="secondary">Requested Services</Button>
</Link>
<Link to={'./profile'}>
<Button color="secondary">Profile</Button>
</Link>
<Button onClick={logOut} color="secondary">Logout</Button>
</div>
) : (
<div>
<Link to={'./'}>
<Button color="secondary">Home</Button>
</Link>
<Button onClick={logIn} color="secondary">Login</Button>
</div>
) }
</Toolbar>
</AppBar>
</div>
</MuiThemeProvider>
);
}
}
export default withStyles(styles)(ButtonAppBar);
javascript reactjs

javascript reactjs

asked Nov 12 at 17:25


SEL
12
12
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I think your code is correct if the application only has 1 administrator but isn't it better to store user role in the firebase instead of deciding the admin state in the application?
User entity has role attribute so you can decide he/she is admin by checking the role attribute
this.setState({ isAdmin : firebase.auth().role='admin'})
There is norole
property offirebase.auth()
. The implementation is a bit more involved than that.
– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
add a comment |
As Eric points out, this method works if you have one user who is an administrator, but keep in mind it's not secure. If someone could take apart the app, they could find that code and easily get around it. If your purpose is simply to hide a tab for a better user experience, then that works fine.
Instead, I'd recommend controlling access via custom claims. I made a video about it that you can check out here. There's also a blog post, a codelab, and a getting started guide in the documentation.
An example from the guide can give you an idea of how this looks in JS:
firebase.auth().currentUser.getIdTokenResult()
.then((idTokenResult) => {
// Confirm the user is an Admin.
if (!!idTokenResult.claims.admin) {
// Show admin UI.
showAdminUI();
} else {
// Show regular user UI.
showRegularUI();
}
})
.catch((error) => {
console.log(error);
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267162%2fhow-to-create-a-admin-state-in-react-using-firebase%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think your code is correct if the application only has 1 administrator but isn't it better to store user role in the firebase instead of deciding the admin state in the application?
User entity has role attribute so you can decide he/she is admin by checking the role attribute
this.setState({ isAdmin : firebase.auth().role='admin'})
There is norole
property offirebase.auth()
. The implementation is a bit more involved than that.
– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
add a comment |
I think your code is correct if the application only has 1 administrator but isn't it better to store user role in the firebase instead of deciding the admin state in the application?
User entity has role attribute so you can decide he/she is admin by checking the role attribute
this.setState({ isAdmin : firebase.auth().role='admin'})
There is norole
property offirebase.auth()
. The implementation is a bit more involved than that.
– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
add a comment |
I think your code is correct if the application only has 1 administrator but isn't it better to store user role in the firebase instead of deciding the admin state in the application?
User entity has role attribute so you can decide he/she is admin by checking the role attribute
this.setState({ isAdmin : firebase.auth().role='admin'})
I think your code is correct if the application only has 1 administrator but isn't it better to store user role in the firebase instead of deciding the admin state in the application?
User entity has role attribute so you can decide he/she is admin by checking the role attribute
this.setState({ isAdmin : firebase.auth().role='admin'})
answered Nov 12 at 17:36


Eric Marcelino
45925
45925
There is norole
property offirebase.auth()
. The implementation is a bit more involved than that.
– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
add a comment |
There is norole
property offirebase.auth()
. The implementation is a bit more involved than that.
– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
There is no
role
property of firebase.auth()
. The implementation is a bit more involved than that.– Jen Person
Nov 12 at 18:56
There is no
role
property of firebase.auth()
. The implementation is a bit more involved than that.– Jen Person
Nov 12 at 18:56
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
Sorry, I answer your question based on my project long ago After I read through the documentation again, I realize the problem isn't that simple But you can add new properties to the User entity firebase.google.com/docs/auth/users
– Eric Marcelino
Nov 13 at 14:03
add a comment |
As Eric points out, this method works if you have one user who is an administrator, but keep in mind it's not secure. If someone could take apart the app, they could find that code and easily get around it. If your purpose is simply to hide a tab for a better user experience, then that works fine.
Instead, I'd recommend controlling access via custom claims. I made a video about it that you can check out here. There's also a blog post, a codelab, and a getting started guide in the documentation.
An example from the guide can give you an idea of how this looks in JS:
firebase.auth().currentUser.getIdTokenResult()
.then((idTokenResult) => {
// Confirm the user is an Admin.
if (!!idTokenResult.claims.admin) {
// Show admin UI.
showAdminUI();
} else {
// Show regular user UI.
showRegularUI();
}
})
.catch((error) => {
console.log(error);
});
add a comment |
As Eric points out, this method works if you have one user who is an administrator, but keep in mind it's not secure. If someone could take apart the app, they could find that code and easily get around it. If your purpose is simply to hide a tab for a better user experience, then that works fine.
Instead, I'd recommend controlling access via custom claims. I made a video about it that you can check out here. There's also a blog post, a codelab, and a getting started guide in the documentation.
An example from the guide can give you an idea of how this looks in JS:
firebase.auth().currentUser.getIdTokenResult()
.then((idTokenResult) => {
// Confirm the user is an Admin.
if (!!idTokenResult.claims.admin) {
// Show admin UI.
showAdminUI();
} else {
// Show regular user UI.
showRegularUI();
}
})
.catch((error) => {
console.log(error);
});
add a comment |
As Eric points out, this method works if you have one user who is an administrator, but keep in mind it's not secure. If someone could take apart the app, they could find that code and easily get around it. If your purpose is simply to hide a tab for a better user experience, then that works fine.
Instead, I'd recommend controlling access via custom claims. I made a video about it that you can check out here. There's also a blog post, a codelab, and a getting started guide in the documentation.
An example from the guide can give you an idea of how this looks in JS:
firebase.auth().currentUser.getIdTokenResult()
.then((idTokenResult) => {
// Confirm the user is an Admin.
if (!!idTokenResult.claims.admin) {
// Show admin UI.
showAdminUI();
} else {
// Show regular user UI.
showRegularUI();
}
})
.catch((error) => {
console.log(error);
});
As Eric points out, this method works if you have one user who is an administrator, but keep in mind it's not secure. If someone could take apart the app, they could find that code and easily get around it. If your purpose is simply to hide a tab for a better user experience, then that works fine.
Instead, I'd recommend controlling access via custom claims. I made a video about it that you can check out here. There's also a blog post, a codelab, and a getting started guide in the documentation.
An example from the guide can give you an idea of how this looks in JS:
firebase.auth().currentUser.getIdTokenResult()
.then((idTokenResult) => {
// Confirm the user is an Admin.
if (!!idTokenResult.claims.admin) {
// Show admin UI.
showAdminUI();
} else {
// Show regular user UI.
showRegularUI();
}
})
.catch((error) => {
console.log(error);
});
answered Nov 12 at 18:55


Jen Person
4,801521
4,801521
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267162%2fhow-to-create-a-admin-state-in-react-using-firebase%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
44QaJU