Nodejs: getting a property of undefined does not throw an error
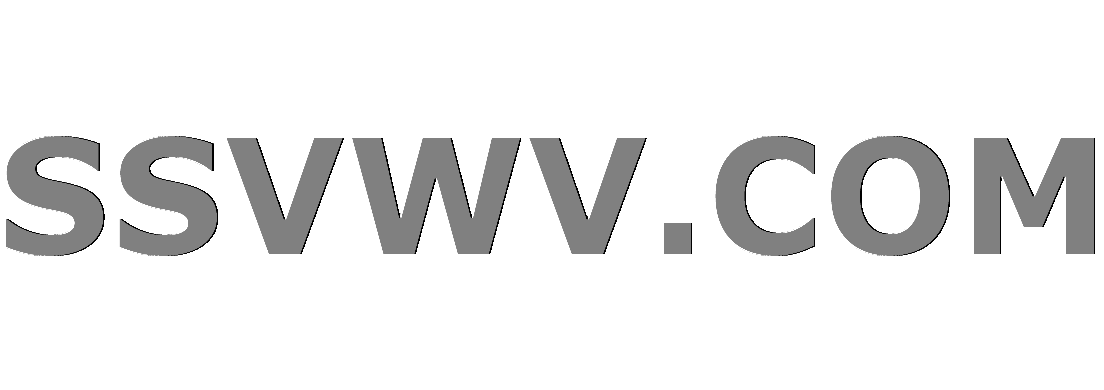
Multi tool use
I'm facing a weird issue in an Express app: No error is thrown, when trying to get a property of a non existing object.
I have a file, with this code:
const bcrypt = require('bcrypt-nodejs');
const hashPassword = async (password) => {
const bcryptGenSalt = util.promisify(bcrypt.genSalt);
const bcryptHash = util.promisify(bcrypt.hash);
try {
const salt = await bcryptGenSalt(10);
const hash = await bcryptHash(password, salt, null)
return hash;
} catch (error) {
throw error;
}
}
module.exports = {
hashPassword
}
As you can see, i forgot to require the "util" module of nodejs. using util.promisify should throw an error "cannot call promisify of undefined", or something like it. But nothing happens. This of course changes if i put the util.promisify call inside the try-catch block, but from what i understand, it should throw an error as it is now.
This is the code that consumes this function, in a different file(a controller):
const registerNewUser = async (req, res, next) => {
const email = req.body.email;
const password = req.body.password;
try {
console.log('before trying to hash')
//THIS IS WHERE I AWAIT THE PROMISE RETURNED FROM THE "PROBLEMATIC" FUNCTION
var hashedPassword = await hashPassword(password)
} catch (error) {
return res.send({ error });
}
const user = new User({
email,
password:hashedPassword
})
try {
const newUser = await user.save();
res.json({
error: null,
data: {
id: newUser._id
}
})
} catch (error) {
res.status(422).send({ error: 'Email already taken' });
}
}
The error doesn't reach the catch block, and the program keeps running, resulting undesired behavior. Can somebody explain what's going on here?
SOLVED: The problem was that for some reason, i was receiving an empty error in my postman response, making me think that no error was caught. It seems that everything works fine, besides the fact that the error object isn't being treated correctly when i do res.send({ error })...
node.js express error-handling async-await try-catch
add a comment |
I'm facing a weird issue in an Express app: No error is thrown, when trying to get a property of a non existing object.
I have a file, with this code:
const bcrypt = require('bcrypt-nodejs');
const hashPassword = async (password) => {
const bcryptGenSalt = util.promisify(bcrypt.genSalt);
const bcryptHash = util.promisify(bcrypt.hash);
try {
const salt = await bcryptGenSalt(10);
const hash = await bcryptHash(password, salt, null)
return hash;
} catch (error) {
throw error;
}
}
module.exports = {
hashPassword
}
As you can see, i forgot to require the "util" module of nodejs. using util.promisify should throw an error "cannot call promisify of undefined", or something like it. But nothing happens. This of course changes if i put the util.promisify call inside the try-catch block, but from what i understand, it should throw an error as it is now.
This is the code that consumes this function, in a different file(a controller):
const registerNewUser = async (req, res, next) => {
const email = req.body.email;
const password = req.body.password;
try {
console.log('before trying to hash')
//THIS IS WHERE I AWAIT THE PROMISE RETURNED FROM THE "PROBLEMATIC" FUNCTION
var hashedPassword = await hashPassword(password)
} catch (error) {
return res.send({ error });
}
const user = new User({
email,
password:hashedPassword
})
try {
const newUser = await user.save();
res.json({
error: null,
data: {
id: newUser._id
}
})
} catch (error) {
res.status(422).send({ error: 'Email already taken' });
}
}
The error doesn't reach the catch block, and the program keeps running, resulting undesired behavior. Can somebody explain what's going on here?
SOLVED: The problem was that for some reason, i was receiving an empty error in my postman response, making me think that no error was caught. It seems that everything works fine, besides the fact that the error object isn't being treated correctly when i do res.send({ error })...
node.js express error-handling async-await try-catch
Error handling should work as you expect. It's not evident thathashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?
– estus
Nov 12 at 18:02
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
Possible duplicate of this Try adding'use strict'
on the top of the files to see if a global util variable leaked.
– lependu
Nov 12 at 18:30
add a comment |
I'm facing a weird issue in an Express app: No error is thrown, when trying to get a property of a non existing object.
I have a file, with this code:
const bcrypt = require('bcrypt-nodejs');
const hashPassword = async (password) => {
const bcryptGenSalt = util.promisify(bcrypt.genSalt);
const bcryptHash = util.promisify(bcrypt.hash);
try {
const salt = await bcryptGenSalt(10);
const hash = await bcryptHash(password, salt, null)
return hash;
} catch (error) {
throw error;
}
}
module.exports = {
hashPassword
}
As you can see, i forgot to require the "util" module of nodejs. using util.promisify should throw an error "cannot call promisify of undefined", or something like it. But nothing happens. This of course changes if i put the util.promisify call inside the try-catch block, but from what i understand, it should throw an error as it is now.
This is the code that consumes this function, in a different file(a controller):
const registerNewUser = async (req, res, next) => {
const email = req.body.email;
const password = req.body.password;
try {
console.log('before trying to hash')
//THIS IS WHERE I AWAIT THE PROMISE RETURNED FROM THE "PROBLEMATIC" FUNCTION
var hashedPassword = await hashPassword(password)
} catch (error) {
return res.send({ error });
}
const user = new User({
email,
password:hashedPassword
})
try {
const newUser = await user.save();
res.json({
error: null,
data: {
id: newUser._id
}
})
} catch (error) {
res.status(422).send({ error: 'Email already taken' });
}
}
The error doesn't reach the catch block, and the program keeps running, resulting undesired behavior. Can somebody explain what's going on here?
SOLVED: The problem was that for some reason, i was receiving an empty error in my postman response, making me think that no error was caught. It seems that everything works fine, besides the fact that the error object isn't being treated correctly when i do res.send({ error })...
node.js express error-handling async-await try-catch
I'm facing a weird issue in an Express app: No error is thrown, when trying to get a property of a non existing object.
I have a file, with this code:
const bcrypt = require('bcrypt-nodejs');
const hashPassword = async (password) => {
const bcryptGenSalt = util.promisify(bcrypt.genSalt);
const bcryptHash = util.promisify(bcrypt.hash);
try {
const salt = await bcryptGenSalt(10);
const hash = await bcryptHash(password, salt, null)
return hash;
} catch (error) {
throw error;
}
}
module.exports = {
hashPassword
}
As you can see, i forgot to require the "util" module of nodejs. using util.promisify should throw an error "cannot call promisify of undefined", or something like it. But nothing happens. This of course changes if i put the util.promisify call inside the try-catch block, but from what i understand, it should throw an error as it is now.
This is the code that consumes this function, in a different file(a controller):
const registerNewUser = async (req, res, next) => {
const email = req.body.email;
const password = req.body.password;
try {
console.log('before trying to hash')
//THIS IS WHERE I AWAIT THE PROMISE RETURNED FROM THE "PROBLEMATIC" FUNCTION
var hashedPassword = await hashPassword(password)
} catch (error) {
return res.send({ error });
}
const user = new User({
email,
password:hashedPassword
})
try {
const newUser = await user.save();
res.json({
error: null,
data: {
id: newUser._id
}
})
} catch (error) {
res.status(422).send({ error: 'Email already taken' });
}
}
The error doesn't reach the catch block, and the program keeps running, resulting undesired behavior. Can somebody explain what's going on here?
SOLVED: The problem was that for some reason, i was receiving an empty error in my postman response, making me think that no error was caught. It seems that everything works fine, besides the fact that the error object isn't being treated correctly when i do res.send({ error })...
node.js express error-handling async-await try-catch
node.js express error-handling async-await try-catch
edited Nov 12 at 18:47
asked Nov 12 at 17:42
sheff2k1
373211
373211
Error handling should work as you expect. It's not evident thathashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?
– estus
Nov 12 at 18:02
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
Possible duplicate of this Try adding'use strict'
on the top of the files to see if a global util variable leaked.
– lependu
Nov 12 at 18:30
add a comment |
Error handling should work as you expect. It's not evident thathashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?
– estus
Nov 12 at 18:02
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
Possible duplicate of this Try adding'use strict'
on the top of the files to see if a global util variable leaked.
– lependu
Nov 12 at 18:30
Error handling should work as you expect. It's not evident that
hashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?– estus
Nov 12 at 18:02
Error handling should work as you expect. It's not evident that
hashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?– estus
Nov 12 at 18:02
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
Possible duplicate of this Try adding
'use strict'
on the top of the files to see if a global util variable leaked.– lependu
Nov 12 at 18:30
Possible duplicate of this Try adding
'use strict'
on the top of the files to see if a global util variable leaked.– lependu
Nov 12 at 18:30
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267421%2fnodejs-getting-a-property-of-undefined-does-not-throw-an-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267421%2fnodejs-getting-a-property-of-undefined-does-not-throw-an-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
a,VaotfPqwdJGHc2r,MZD2YUG1vqGEj1 9eP2bsA
Error handling should work as you expect. It's not evident that
hashPassword
is called at all. Did you debug the code to be sure which line was executed and which one wasn't? How did you do this?– estus
Nov 12 at 18:02
I've updated the code, putting the entire upper function. As u can see, i have a console.log statement "before trying to hash". It's always being invoked, so there is no problem of this code not being executed.
– sheff2k1
Nov 12 at 18:13
Possible duplicate of this Try adding
'use strict'
on the top of the files to see if a global util variable leaked.– lependu
Nov 12 at 18:30