ArrayList not displaying in RecyclerView but no errors shown
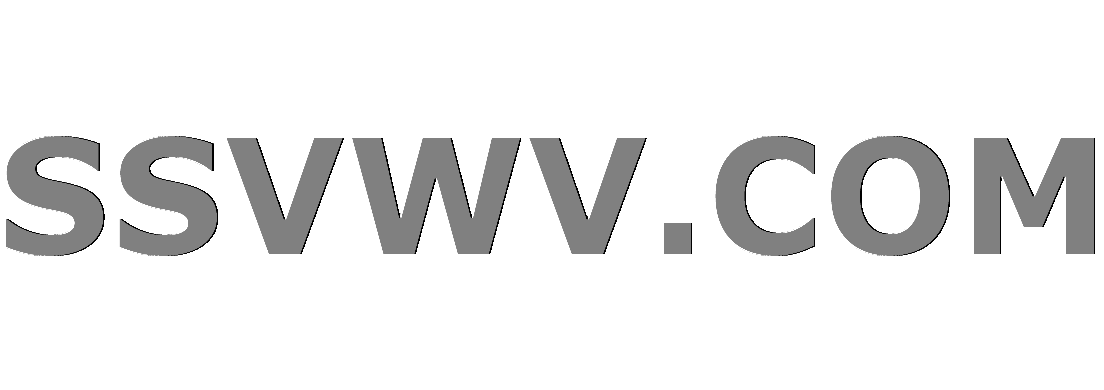
Multi tool use
I've have an arraylist that is not displaying in RecyclerView. The arraylist has data but my RecyclerView Adapter shows no error, nor is my fragment activity showing no errors. I am at a complete loss where the programming error is. The getItemCount seems correct, the holder seems correct and the Fragment seems to be correct but I know there is a mistake somewhere. Here is my code:
Fragment:
public class TestFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
List<PlanetData> items = new ArrayList<>();
RecyclerView mRecyclerView;
PlanetRecyclerViewAdapter adapter;
private OnFragmentInteractionListener mListener;
public TestFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) {
planetList();
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_test, container, false);
mRecyclerView = (RecyclerView)view.findViewById(R.id.planet_recycler_view);
mRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity()));
mRecyclerView.addItemDecoration(new DividerItemDecoration(mRecyclerView.getContext(),DividerItemDecoration.VERTICAL));
adapter = new PlanetRecyclerViewAdapter(items, mRecyclerView.getContext());
mRecyclerView.setAdapter(adapter);
return view;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
private List<PlanetData> planetList() {
List<PlanetData> planetvalues = new ArrayList<>();
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData("12"));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mercury.getMercuryRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Venus.getVenusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Moon.getMoonRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mars.getMarsRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Jupiter.getJupiterRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Saturn.getSaturnRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Uranus.getUranusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Neptune.getNeptuneRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Pluto.getPlutoRA())));
System.out.println("This is Arraylist:" + planetvalues);
return planetvalues;
}
}
Here is the PlanetData class:
public class PlanetData {
private String PlanetRA;
public PlanetData(String PlanetRA) {
this.PlanetRA = PlanetRA;
}
@Override
public String toString() {
return PlanetRA;
}
public String getPlanetRA (){
return PlanetRA;
}
public void setPlanetRA(String PlanetRA){
this.PlanetRA = PlanetRA;
}
}
Here is my RecyclerView Adapter:
public class PlanetRecyclerViewAdapter extends RecyclerView.Adapter<PlanetRecyclerViewAdapter.ViewHolder> {
private List<PlanetData> mPlanetDataList;
Context mContext;
public static class ViewHolder extends RecyclerView.ViewHolder{
public TextView currentRA;
public ViewHolder(View itemView) {
super(itemView);
currentRA = (TextView) itemView.findViewById(R.id.planet_location);
}
}
public PlanetRecyclerViewAdapter(List<PlanetData> mPlanetDataList, Context mContext){
this.mPlanetDataList = mPlanetDataList;
this.mContext = mContext;
}
@Override
public PlanetRecyclerViewAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.planet_recycler_item,parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder( PlanetRecyclerViewAdapter.ViewHolder holder, int position) {
holder.currentRA.setText(mPlanetDataList.get(position).getPlanetRA());
}
@Override
public int getItemCount() {
return mPlanetDataList.size();
}
}
java

add a comment |
I've have an arraylist that is not displaying in RecyclerView. The arraylist has data but my RecyclerView Adapter shows no error, nor is my fragment activity showing no errors. I am at a complete loss where the programming error is. The getItemCount seems correct, the holder seems correct and the Fragment seems to be correct but I know there is a mistake somewhere. Here is my code:
Fragment:
public class TestFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
List<PlanetData> items = new ArrayList<>();
RecyclerView mRecyclerView;
PlanetRecyclerViewAdapter adapter;
private OnFragmentInteractionListener mListener;
public TestFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) {
planetList();
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_test, container, false);
mRecyclerView = (RecyclerView)view.findViewById(R.id.planet_recycler_view);
mRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity()));
mRecyclerView.addItemDecoration(new DividerItemDecoration(mRecyclerView.getContext(),DividerItemDecoration.VERTICAL));
adapter = new PlanetRecyclerViewAdapter(items, mRecyclerView.getContext());
mRecyclerView.setAdapter(adapter);
return view;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
private List<PlanetData> planetList() {
List<PlanetData> planetvalues = new ArrayList<>();
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData("12"));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mercury.getMercuryRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Venus.getVenusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Moon.getMoonRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mars.getMarsRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Jupiter.getJupiterRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Saturn.getSaturnRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Uranus.getUranusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Neptune.getNeptuneRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Pluto.getPlutoRA())));
System.out.println("This is Arraylist:" + planetvalues);
return planetvalues;
}
}
Here is the PlanetData class:
public class PlanetData {
private String PlanetRA;
public PlanetData(String PlanetRA) {
this.PlanetRA = PlanetRA;
}
@Override
public String toString() {
return PlanetRA;
}
public String getPlanetRA (){
return PlanetRA;
}
public void setPlanetRA(String PlanetRA){
this.PlanetRA = PlanetRA;
}
}
Here is my RecyclerView Adapter:
public class PlanetRecyclerViewAdapter extends RecyclerView.Adapter<PlanetRecyclerViewAdapter.ViewHolder> {
private List<PlanetData> mPlanetDataList;
Context mContext;
public static class ViewHolder extends RecyclerView.ViewHolder{
public TextView currentRA;
public ViewHolder(View itemView) {
super(itemView);
currentRA = (TextView) itemView.findViewById(R.id.planet_location);
}
}
public PlanetRecyclerViewAdapter(List<PlanetData> mPlanetDataList, Context mContext){
this.mPlanetDataList = mPlanetDataList;
this.mContext = mContext;
}
@Override
public PlanetRecyclerViewAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.planet_recycler_item,parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder( PlanetRecyclerViewAdapter.ViewHolder holder, int position) {
holder.currentRA.setText(mPlanetDataList.get(position).getPlanetRA());
}
@Override
public int getItemCount() {
return mPlanetDataList.size();
}
}
java

add a comment |
I've have an arraylist that is not displaying in RecyclerView. The arraylist has data but my RecyclerView Adapter shows no error, nor is my fragment activity showing no errors. I am at a complete loss where the programming error is. The getItemCount seems correct, the holder seems correct and the Fragment seems to be correct but I know there is a mistake somewhere. Here is my code:
Fragment:
public class TestFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
List<PlanetData> items = new ArrayList<>();
RecyclerView mRecyclerView;
PlanetRecyclerViewAdapter adapter;
private OnFragmentInteractionListener mListener;
public TestFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) {
planetList();
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_test, container, false);
mRecyclerView = (RecyclerView)view.findViewById(R.id.planet_recycler_view);
mRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity()));
mRecyclerView.addItemDecoration(new DividerItemDecoration(mRecyclerView.getContext(),DividerItemDecoration.VERTICAL));
adapter = new PlanetRecyclerViewAdapter(items, mRecyclerView.getContext());
mRecyclerView.setAdapter(adapter);
return view;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
private List<PlanetData> planetList() {
List<PlanetData> planetvalues = new ArrayList<>();
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData("12"));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mercury.getMercuryRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Venus.getVenusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Moon.getMoonRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mars.getMarsRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Jupiter.getJupiterRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Saturn.getSaturnRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Uranus.getUranusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Neptune.getNeptuneRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Pluto.getPlutoRA())));
System.out.println("This is Arraylist:" + planetvalues);
return planetvalues;
}
}
Here is the PlanetData class:
public class PlanetData {
private String PlanetRA;
public PlanetData(String PlanetRA) {
this.PlanetRA = PlanetRA;
}
@Override
public String toString() {
return PlanetRA;
}
public String getPlanetRA (){
return PlanetRA;
}
public void setPlanetRA(String PlanetRA){
this.PlanetRA = PlanetRA;
}
}
Here is my RecyclerView Adapter:
public class PlanetRecyclerViewAdapter extends RecyclerView.Adapter<PlanetRecyclerViewAdapter.ViewHolder> {
private List<PlanetData> mPlanetDataList;
Context mContext;
public static class ViewHolder extends RecyclerView.ViewHolder{
public TextView currentRA;
public ViewHolder(View itemView) {
super(itemView);
currentRA = (TextView) itemView.findViewById(R.id.planet_location);
}
}
public PlanetRecyclerViewAdapter(List<PlanetData> mPlanetDataList, Context mContext){
this.mPlanetDataList = mPlanetDataList;
this.mContext = mContext;
}
@Override
public PlanetRecyclerViewAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.planet_recycler_item,parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder( PlanetRecyclerViewAdapter.ViewHolder holder, int position) {
holder.currentRA.setText(mPlanetDataList.get(position).getPlanetRA());
}
@Override
public int getItemCount() {
return mPlanetDataList.size();
}
}
java

I've have an arraylist that is not displaying in RecyclerView. The arraylist has data but my RecyclerView Adapter shows no error, nor is my fragment activity showing no errors. I am at a complete loss where the programming error is. The getItemCount seems correct, the holder seems correct and the Fragment seems to be correct but I know there is a mistake somewhere. Here is my code:
Fragment:
public class TestFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
List<PlanetData> items = new ArrayList<>();
RecyclerView mRecyclerView;
PlanetRecyclerViewAdapter adapter;
private OnFragmentInteractionListener mListener;
public TestFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) {
planetList();
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_test, container, false);
mRecyclerView = (RecyclerView)view.findViewById(R.id.planet_recycler_view);
mRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity()));
mRecyclerView.addItemDecoration(new DividerItemDecoration(mRecyclerView.getContext(),DividerItemDecoration.VERTICAL));
adapter = new PlanetRecyclerViewAdapter(items, mRecyclerView.getContext());
mRecyclerView.setAdapter(adapter);
return view;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
private List<PlanetData> planetList() {
List<PlanetData> planetvalues = new ArrayList<>();
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData("12"));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mercury.getMercuryRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Venus.getVenusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Moon.getMoonRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Mars.getMarsRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Jupiter.getJupiterRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Saturn.getSaturnRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Uranus.getUranusRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Neptune.getNeptuneRA())));
planetvalues.add(new com.ksburneytwo.planetmathtest.PlanetData(Double.toString(Pluto.getPlutoRA())));
System.out.println("This is Arraylist:" + planetvalues);
return planetvalues;
}
}
Here is the PlanetData class:
public class PlanetData {
private String PlanetRA;
public PlanetData(String PlanetRA) {
this.PlanetRA = PlanetRA;
}
@Override
public String toString() {
return PlanetRA;
}
public String getPlanetRA (){
return PlanetRA;
}
public void setPlanetRA(String PlanetRA){
this.PlanetRA = PlanetRA;
}
}
Here is my RecyclerView Adapter:
public class PlanetRecyclerViewAdapter extends RecyclerView.Adapter<PlanetRecyclerViewAdapter.ViewHolder> {
private List<PlanetData> mPlanetDataList;
Context mContext;
public static class ViewHolder extends RecyclerView.ViewHolder{
public TextView currentRA;
public ViewHolder(View itemView) {
super(itemView);
currentRA = (TextView) itemView.findViewById(R.id.planet_location);
}
}
public PlanetRecyclerViewAdapter(List<PlanetData> mPlanetDataList, Context mContext){
this.mPlanetDataList = mPlanetDataList;
this.mContext = mContext;
}
@Override
public PlanetRecyclerViewAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.planet_recycler_item,parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder( PlanetRecyclerViewAdapter.ViewHolder holder, int position) {
holder.currentRA.setText(mPlanetDataList.get(position).getPlanetRA());
}
@Override
public int getItemCount() {
return mPlanetDataList.size();
}
}
java

java

asked Nov 12 at 17:42
ksb
4416
4416
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I don't see you ever actually adding anything to the items
list.
You call planetList()
in onCreateView()
, but you aren't using the result of it, and planetList()
doesn't affect items
in any way: it makes its own ArrayList and returns that.
Either remove planetValues
from the planetList()
method and reference items
directly:
private void planetList() { //changed signature to "void"
items.add(...);
items.add(...);
//etc
}
Or set the result of planetList()
to items
when you call it:
items.addAll(planetList());
add a comment |
You haven't populated items.
items = planetList();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267415%2farraylist-not-displaying-in-recyclerview-but-no-errors-shown%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't see you ever actually adding anything to the items
list.
You call planetList()
in onCreateView()
, but you aren't using the result of it, and planetList()
doesn't affect items
in any way: it makes its own ArrayList and returns that.
Either remove planetValues
from the planetList()
method and reference items
directly:
private void planetList() { //changed signature to "void"
items.add(...);
items.add(...);
//etc
}
Or set the result of planetList()
to items
when you call it:
items.addAll(planetList());
add a comment |
I don't see you ever actually adding anything to the items
list.
You call planetList()
in onCreateView()
, but you aren't using the result of it, and planetList()
doesn't affect items
in any way: it makes its own ArrayList and returns that.
Either remove planetValues
from the planetList()
method and reference items
directly:
private void planetList() { //changed signature to "void"
items.add(...);
items.add(...);
//etc
}
Or set the result of planetList()
to items
when you call it:
items.addAll(planetList());
add a comment |
I don't see you ever actually adding anything to the items
list.
You call planetList()
in onCreateView()
, but you aren't using the result of it, and planetList()
doesn't affect items
in any way: it makes its own ArrayList and returns that.
Either remove planetValues
from the planetList()
method and reference items
directly:
private void planetList() { //changed signature to "void"
items.add(...);
items.add(...);
//etc
}
Or set the result of planetList()
to items
when you call it:
items.addAll(planetList());
I don't see you ever actually adding anything to the items
list.
You call planetList()
in onCreateView()
, but you aren't using the result of it, and planetList()
doesn't affect items
in any way: it makes its own ArrayList and returns that.
Either remove planetValues
from the planetList()
method and reference items
directly:
private void planetList() { //changed signature to "void"
items.add(...);
items.add(...);
//etc
}
Or set the result of planetList()
to items
when you call it:
items.addAll(planetList());
answered Nov 12 at 17:46


TheWanderer
6,40521027
6,40521027
add a comment |
add a comment |
You haven't populated items.
items = planetList();
add a comment |
You haven't populated items.
items = planetList();
add a comment |
You haven't populated items.
items = planetList();
You haven't populated items.
items = planetList();
answered Nov 12 at 17:45


iaindownie
904826
904826
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267415%2farraylist-not-displaying-in-recyclerview-but-no-errors-shown%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yxWvMBgRSU3eUFk,9K9pWKnWVClBrlZqNcwaE e jnskSJszSqtHGFL7xs58,nl jzOhE