What causes a java.lang.ArrayIndexOutOfBoundsException and how do I prevent it?
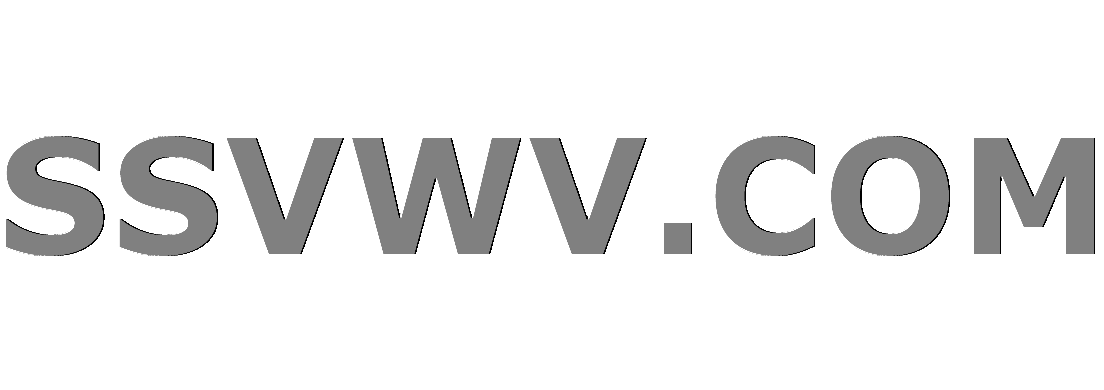
Multi tool use
up vote
222
down vote
favorite
What does ArrayIndexOutOfBoundsException
mean and how do I get rid of it?
Here is a code sample that triggers the exception:
String name = {"tom", "dick", "harry"};
for (int i = 0; i <= name.length; i++) {
System.out.print(name[i] + 'n');
}
java arrays indexoutofboundsexception
add a comment |
up vote
222
down vote
favorite
What does ArrayIndexOutOfBoundsException
mean and how do I get rid of it?
Here is a code sample that triggers the exception:
String name = {"tom", "dick", "harry"};
for (int i = 0; i <= name.length; i++) {
System.out.print(name[i] + 'n');
}
java arrays indexoutofboundsexception
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
31
Replacei <= name.length
withi < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)
– Jean Hominal
Apr 5 '11 at 16:14
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05
add a comment |
up vote
222
down vote
favorite
up vote
222
down vote
favorite
What does ArrayIndexOutOfBoundsException
mean and how do I get rid of it?
Here is a code sample that triggers the exception:
String name = {"tom", "dick", "harry"};
for (int i = 0; i <= name.length; i++) {
System.out.print(name[i] + 'n');
}
java arrays indexoutofboundsexception
What does ArrayIndexOutOfBoundsException
mean and how do I get rid of it?
Here is a code sample that triggers the exception:
String name = {"tom", "dick", "harry"};
for (int i = 0; i <= name.length; i++) {
System.out.print(name[i] + 'n');
}
java arrays indexoutofboundsexception
java arrays indexoutofboundsexception
edited Nov 15 at 12:09
Lii
6,72444158
6,72444158
asked Apr 5 '11 at 15:54
Aaron
3,851144865
3,851144865
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
31
Replacei <= name.length
withi < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)
– Jean Hominal
Apr 5 '11 at 16:14
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05
add a comment |
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
31
Replacei <= name.length
withi < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)
– Jean Hominal
Apr 5 '11 at 16:14
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
31
31
Replace
i <= name.length
with i < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)– Jean Hominal
Apr 5 '11 at 16:14
Replace
i <= name.length
with i < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)– Jean Hominal
Apr 5 '11 at 16:14
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05
add a comment |
22 Answers
22
active
oldest
votes
up vote
229
down vote
accepted
Your first port of call should be the documentation which explains it reasonably clearly:
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
So for example:
int array = new int[5];
int boom = array[10]; // Throws the exception
As for how to avoid it... um, don't do that. Be careful with your array indexes.
One problem people sometimes run into is thinking that arrays are 1-indexed, e.g.
int array = new int[5];
// ... populate the array here ...
for (int index = 1; index <= array.length; index++)
{
System.out.println(array[index]);
}
That will miss out the first element (index 0) and throw an exception when index is 5. The valid indexes here are 0-4 inclusive. The correct, idiomatic for
statement here would be:
for (int index = 0; index < array.length; index++)
(That's assuming you need the index, of course. If you can use the enhanced for loop instead, do so.)
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.
– Andrey
Mar 2 '17 at 22:04
add a comment |
up vote
41
down vote
if (index < 0 || index >= array.length) {
// Don't use this index. This is out of bounds (borders, limits, whatever).
} else {
// Yes, you can safely use this index. The index is present in the array.
Object element = array[index];
}
See also:
- The Java Tutorials - Language Basics - Arrays
Update: as per your code snippet,
for(int i = 0; i<=name.length; i++) {
The index is inclusive the array's length. This is out of bounds. You need to replace <=
by <
.
for(int i = 0; i < name.length; i++) {
add a comment |
up vote
17
down vote
To put it briefly:
In the last iteration of
for(int i = 0; i<=name.length; i++) {
i
will equal name.length
which is an illegal index, since array indices are zero-based.
Your code should read
for(int i = 0; i < name.length; i++)
^
add a comment |
up vote
12
down vote
It means that you are trying to access an index of an array which is not valid as it is not in between the bounds.
For example this would initialize a primitive integer array with the upper bound 4.
int intArray = new int[5];
Programmers count from zero. So this for example would throw an ArrayIndexOutOfBoundsException
as the upper bound is 4 and not 5.
intArray[5];
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
add a comment |
up vote
7
down vote
To avoid an array index out-of-bounds exception, one should use the enhanced-for
statement where and when they can.
The primary motivation (and use case) is when you are iterating and you do not require any complicated iteration steps. You would not be able to use an enhanced-for
to move backwards in an array or only iterate on every other element.
You're guaranteed not to run out of elements to iterate over when doing this, and your [corrected] example is easily converted over.
The code below:
String name = {"tom", "dick", "harry"};
for(int i = 0; i< name.length; i++) {
System.out.print(name[i] + "n");
}
...is equivalent to this:
String name = {"tom", "dick", "harry"};
for(String firstName : name) {
System.out.println(firstName + "n");
}
add a comment |
up vote
7
down vote
What causes ArrayIndexOutOfBoundsException
?
If you think of a variable as a "box" where you can place a value, then an array is a series of boxes placed next to eachother, where the number of boxes is a finite and explicit integer.
Creating an array like this:
final int myArray = new int[5]
creates a row of 5 boxes, each holding an int
. Each of the boxes have an index, a position in the series of boxes. This index starts at 0, and ends at N-1, where N is the size of the array (the number of boxes).
To retrieve one of the values from this series of boxes, you can refer to it through its index, like this:
myArray[3]
Which will give you the value of the 4th box in the series (since the first box has index 0).
An ArrayIndexOutOfBoundsException
is caused by trying to retrive a "box" that does not exist, by passing an index that is higher than the index of last "box", or negative.
With my running example, these code snippets would produce such an exception:
myArray[5] //tries to retrieve the 6th "box" when there is only 5
myArray[-1] //just makes no sense
myArray[1337] //waay to high
How to avoid ArrayIndexOutOfBoundsException
In order to prevent ArrayIndexOutOfBoundsException
, there are some key points to consider:
Looping
When looping through an array, always make sure that the index you are retrieving is strictly smaller than the length of the array (the number of boxes). For instance:
for (int i = 0; i < myArray.length; i++) {
Notice the <
, never mix a =
in there..
You might want to be tempted to do something like this:
for (int i = 1; i <= myArray.length; i++) {
final int someint = myArray[i - 1]
Just don't. Stick to the one above (if you need to use the index) and it will save you a lot of pain.
Where possible, use foreach:
for (int value : myArray) {
This way you won't have to think about indexes at all.
When looping, whatever you do, NEVER change the value of the loop iterator (here: i
). The only place this should change value is to keep the loop going. Changing it otherwise is just risking an exception, and is in most cases not neccessary.
Retrieval/update
When retrieving an arbitrary element of the array, always check that it is a valid index against the length of the array:
public Integer getArrayElement(final int index) {
if (index < 0 || index >= myArray.length) {
return null; //although I would much prefer an actual exception being thrown when this happens.
}
return myArray[index];
}
add a comment |
up vote
4
down vote
In your code you have accessed the elements from index 0 to the length of the string array. name.length
gives the number of string objects in your array of string objects i.e. 3, but you can access only up to index 2 name[2]
,
because the array can be accessed from index 0 to name.length - 1
where you get name.length
number of objects.
Even while using a for
loop you have started with index zero and you should end with name.length - 1
. In an array a[n] you can access form a[0] to a[n-1].
For example:
String a={"str1", "str2", str3" ..., "strn"};
for(int i=0;i<a.length()i++)
System.out.println(a[i]);
In your case:
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
3
down vote
For your given array the length of the array is 3(i.e. name.length = 3). But as it stores element starting from index 0, it has max index 2.
So, instead of 'i**<=name.length' you should write 'i<**name.length' to avoid 'ArrayIndexOutOfBoundsException'.
add a comment |
up vote
2
down vote
So much for this simple question, but I just wanted to highlight a new feature in Java which will avoid all confusions around indexing in arrays even for beginners. Java-8 has abstracted the task of iterating for you.
int array = new int[5];
//If you need just the items
Arrays.stream(array).forEach(item -> { println(item); });
//If you need the index as well
IntStream.range(0, array.length).forEach(index -> { println(array[index]); })
What's the benefit? Well, one thing is the readability like English. Second, you need not worry about the ArrayIndexOutOfBoundsException
add a comment |
up vote
2
down vote
You are getting ArrayIndexOutOfBoundsException
due to i<=name.length
part. name.length
return the length of the string name
, which is 3. Hence when you try to access name[3]
, it's illegal and throws an exception.
Resolved code:
String name = {"tom", "dick", "harry"};
for(int i = 0; i < name.length; i++) { //use < insteadof <=
System.out.print(name[i] +'n');
}
It's defined in the Java language specification:
The
public final
fieldlength
, which contains the number of components
of the array.length
may be positive or zero.
add a comment |
up vote
2
down vote
That's how this type of exception looks when thrown in Eclipse. The number in red signifies the index you tried to access. So the code would look like this:
myArray[5]
The error is thrown when you try to access an index which doesn't exist in that array. If an array has a length of 3,
int intArray = new int[3];
then the only valid indexes are:
intArray[0]
intArray[1]
intArray[2]
If an array has a length of 1,
int intArray = new int[1];
then the only valid index is:
intArray[0]
Any integer equal to the length of the array, or bigger than it: is out of bounds.
Any integer less than 0: is out of bounds;
P.S.: If you look to have a better understanding of arrays and do some practical exercises, there's a video here: tutorial on arrays in Java
add a comment |
up vote
1
down vote
For multidimensional arrays, it can be tricky to make sure you access the length
property of the right dimension. Take the following code for example:
int a = new int [2][3][4];
for(int i = 0; i < a.length; i++){
for(int j = 0; j < a[i].length; j++){
for(int k = 0; k < a[j].length; k++){
System.out.print(a[i][j][k]);
}
System.out.println();
}
System.out.println();
}
Each dimension has a different length, so the subtle bug is that the middle and inner loops use the length
property of the same dimension (because a[i].length
is the same as a[j].length
).
Instead, the inner loop should use a[i][j].length
(or a[0][0].length
, for simplicity).
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
add a comment |
up vote
1
down vote
ArrayIndexOutOfBoundsException whenever this exception is coming it mean you are trying to use an index of array which is out of its bounds or in lay man terms you are requesting more than than you have initialised.
To prevent this always make sure that you are not requesting a index which is not present in array i.e. if array length is 10 then your index must range between 0 to 9
add a comment |
up vote
0
down vote
ArrayIndexOutOfBoundsException
means that you are trying to access an index of the array that does not exist or out of the bound of this array. Array indexes start from 0 and end at length - 1.
In your case
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length, Not correct
}
ArrayIndexOutOfBoundsException
happens when you are trying to access
the name.length indexed element which does not exist (array index ends at length -1). just replacing <= with < would solve this problem.
for(int i = 0; i < name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length - 1, Correct
}
add a comment |
up vote
0
down vote
The most common case I've seen for seemingly mysterious ArrayIndexOutOfBoundsExceptions, i.e. apparently not caused by your own array handling code, is the concurrent use of SimpleDateFormat. Particularly in a servlet or controller:
public class MyController {
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
public void handleRequest(ServletRequest req, ServletResponse res) {
Date date = dateFormat.parse(req.getParameter("date"));
}
}
If two threads enter the SimplateDateFormat.parse() method together you will likely see an ArrayIndexOutOfBoundsException. Note the synchronization section of the class javadoc for SimpleDateFormat.
Make sure there is no place in your code that are accessing thread unsafe classes like SimpleDateFormat in a concurrent manner like in a servlet or controller. Check all instance variables of your servlets and controllers for likely suspects.
add a comment |
up vote
0
down vote
For any array of length n, elements of the array will have an index from 0 to n-1.
If your program is trying to access any element (or memory) having array index greater than n-1, then Java will throw ArrayIndexOutOfBoundsException
So here are two solutions that we can use in a program
Maintaining count:
for(int count = 0; count < array.length; count++) {
System.out.println(array[count]);
}
Or some other looping statement like
int count = 0;
while(count < array.length) {
System.out.println(array[count]);
count++;
}
A better way go with a for each loop, in this method a programmer has no need to bother about the number of elements in the array.
for(String str : array) {
System.out.println(str);
}
add a comment |
up vote
0
down vote
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
IndexOutOfBoundsException is thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Any array X, can be accessed from [0 to (X.length - 1)]
add a comment |
up vote
0
down vote
I see all the answers here explaining how to work with arrays and how to avoid the index out of bounds exceptions. I personally avoid arrays at all costs. I use the Collections classes, which avoids all the silliness of having to deal with array indices entirely. The looping constructs work beautifully with collections supporting code that is both easier to write, understand and maintain.
add a comment |
up vote
-1
down vote
According to your Code :
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
If You check
System.out.print(name.length);
you will get 3;
that mean your name length is 3
your loop is running from 0 to 3
which should be running either "0 to 2" or "1 to 3"
Answer
String name = {"tom", "dick", "harry"};
for(int i = 0; i<name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
-1
down vote
ArrayIndexOutOfBoundsException
name itself explains that If you trying to access the value at the index which is out of the scope of Array size then such kind of exception occur.
In your case, You can just remove equal sign from your for loop.
for(int i = 0; i<name.length; i++)
The better option is to iterate an array:
for(String i : name )
System.out.println(i);
add a comment |
up vote
-1
down vote
This error is occurs at runs loop overlimit times.Let's consider simple example like this,
class demo{
public static void main(String a){
int numberArray={4,8,2,3,89,5};
int i;
for(i=0;i<numberArray.length;i++){
System.out.print(numberArray[i+1]+" ");
}
}
At first, I have initialized an array as 'numberArray'. then , some array elements are printed using for loop. When loop is running 'i' time , print the (numberArray[i+1] element..(when i value is 1, numberArray[i+1] element is printed.)..Suppose that, when i=(numberArray.length-2), last element of array is printed..When 'i' value goes to (numberArray.length-1) , no value for printing..In that point , 'ArrayIndexOutOfBoundsException' is occur.I hope to you could get idea.thank you !
add a comment |
up vote
-3
down vote
You could not iterate or store more data than the length of your array. In this case you could do like this:
for (int i = 0; i <= name.length - 1; i++) {
// ....
}
Or this:
for (int i = 0; i < name.length; i++) {
// ...
}
add a comment |
protected by Makoto Oct 16 '15 at 21:34
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
22 Answers
22
active
oldest
votes
22 Answers
22
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
229
down vote
accepted
Your first port of call should be the documentation which explains it reasonably clearly:
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
So for example:
int array = new int[5];
int boom = array[10]; // Throws the exception
As for how to avoid it... um, don't do that. Be careful with your array indexes.
One problem people sometimes run into is thinking that arrays are 1-indexed, e.g.
int array = new int[5];
// ... populate the array here ...
for (int index = 1; index <= array.length; index++)
{
System.out.println(array[index]);
}
That will miss out the first element (index 0) and throw an exception when index is 5. The valid indexes here are 0-4 inclusive. The correct, idiomatic for
statement here would be:
for (int index = 0; index < array.length; index++)
(That's assuming you need the index, of course. If you can use the enhanced for loop instead, do so.)
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.
– Andrey
Mar 2 '17 at 22:04
add a comment |
up vote
229
down vote
accepted
Your first port of call should be the documentation which explains it reasonably clearly:
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
So for example:
int array = new int[5];
int boom = array[10]; // Throws the exception
As for how to avoid it... um, don't do that. Be careful with your array indexes.
One problem people sometimes run into is thinking that arrays are 1-indexed, e.g.
int array = new int[5];
// ... populate the array here ...
for (int index = 1; index <= array.length; index++)
{
System.out.println(array[index]);
}
That will miss out the first element (index 0) and throw an exception when index is 5. The valid indexes here are 0-4 inclusive. The correct, idiomatic for
statement here would be:
for (int index = 0; index < array.length; index++)
(That's assuming you need the index, of course. If you can use the enhanced for loop instead, do so.)
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.
– Andrey
Mar 2 '17 at 22:04
add a comment |
up vote
229
down vote
accepted
up vote
229
down vote
accepted
Your first port of call should be the documentation which explains it reasonably clearly:
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
So for example:
int array = new int[5];
int boom = array[10]; // Throws the exception
As for how to avoid it... um, don't do that. Be careful with your array indexes.
One problem people sometimes run into is thinking that arrays are 1-indexed, e.g.
int array = new int[5];
// ... populate the array here ...
for (int index = 1; index <= array.length; index++)
{
System.out.println(array[index]);
}
That will miss out the first element (index 0) and throw an exception when index is 5. The valid indexes here are 0-4 inclusive. The correct, idiomatic for
statement here would be:
for (int index = 0; index < array.length; index++)
(That's assuming you need the index, of course. If you can use the enhanced for loop instead, do so.)
Your first port of call should be the documentation which explains it reasonably clearly:
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
So for example:
int array = new int[5];
int boom = array[10]; // Throws the exception
As for how to avoid it... um, don't do that. Be careful with your array indexes.
One problem people sometimes run into is thinking that arrays are 1-indexed, e.g.
int array = new int[5];
// ... populate the array here ...
for (int index = 1; index <= array.length; index++)
{
System.out.println(array[index]);
}
That will miss out the first element (index 0) and throw an exception when index is 5. The valid indexes here are 0-4 inclusive. The correct, idiomatic for
statement here would be:
for (int index = 0; index < array.length; index++)
(That's assuming you need the index, of course. If you can use the enhanced for loop instead, do so.)
edited Oct 16 '15 at 21:38
Makoto
79.3k15122164
79.3k15122164
answered Apr 5 '11 at 15:57
Jon Skeet
1069k67178348374
1069k67178348374
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.
– Andrey
Mar 2 '17 at 22:04
add a comment |
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.
– Andrey
Mar 2 '17 at 22:04
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:
for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.– Andrey
Mar 2 '17 at 22:04
I'd add that for multidimensional arrays that might have an arbitrary shape in Java, the nested loops should check for the relevant subarray length:
for (int nestedIndex = 0; nestedIndex < array[outerIndex].length; nestedIndex++) { ... array[outerIndex][nestedIndex] ... }
.– Andrey
Mar 2 '17 at 22:04
add a comment |
up vote
41
down vote
if (index < 0 || index >= array.length) {
// Don't use this index. This is out of bounds (borders, limits, whatever).
} else {
// Yes, you can safely use this index. The index is present in the array.
Object element = array[index];
}
See also:
- The Java Tutorials - Language Basics - Arrays
Update: as per your code snippet,
for(int i = 0; i<=name.length; i++) {
The index is inclusive the array's length. This is out of bounds. You need to replace <=
by <
.
for(int i = 0; i < name.length; i++) {
add a comment |
up vote
41
down vote
if (index < 0 || index >= array.length) {
// Don't use this index. This is out of bounds (borders, limits, whatever).
} else {
// Yes, you can safely use this index. The index is present in the array.
Object element = array[index];
}
See also:
- The Java Tutorials - Language Basics - Arrays
Update: as per your code snippet,
for(int i = 0; i<=name.length; i++) {
The index is inclusive the array's length. This is out of bounds. You need to replace <=
by <
.
for(int i = 0; i < name.length; i++) {
add a comment |
up vote
41
down vote
up vote
41
down vote
if (index < 0 || index >= array.length) {
// Don't use this index. This is out of bounds (borders, limits, whatever).
} else {
// Yes, you can safely use this index. The index is present in the array.
Object element = array[index];
}
See also:
- The Java Tutorials - Language Basics - Arrays
Update: as per your code snippet,
for(int i = 0; i<=name.length; i++) {
The index is inclusive the array's length. This is out of bounds. You need to replace <=
by <
.
for(int i = 0; i < name.length; i++) {
if (index < 0 || index >= array.length) {
// Don't use this index. This is out of bounds (borders, limits, whatever).
} else {
// Yes, you can safely use this index. The index is present in the array.
Object element = array[index];
}
See also:
- The Java Tutorials - Language Basics - Arrays
Update: as per your code snippet,
for(int i = 0; i<=name.length; i++) {
The index is inclusive the array's length. This is out of bounds. You need to replace <=
by <
.
for(int i = 0; i < name.length; i++) {
edited Apr 5 '11 at 16:12
answered Apr 5 '11 at 15:59
BalusC
835k29430973182
835k29430973182
add a comment |
add a comment |
up vote
17
down vote
To put it briefly:
In the last iteration of
for(int i = 0; i<=name.length; i++) {
i
will equal name.length
which is an illegal index, since array indices are zero-based.
Your code should read
for(int i = 0; i < name.length; i++)
^
add a comment |
up vote
17
down vote
To put it briefly:
In the last iteration of
for(int i = 0; i<=name.length; i++) {
i
will equal name.length
which is an illegal index, since array indices are zero-based.
Your code should read
for(int i = 0; i < name.length; i++)
^
add a comment |
up vote
17
down vote
up vote
17
down vote
To put it briefly:
In the last iteration of
for(int i = 0; i<=name.length; i++) {
i
will equal name.length
which is an illegal index, since array indices are zero-based.
Your code should read
for(int i = 0; i < name.length; i++)
^
To put it briefly:
In the last iteration of
for(int i = 0; i<=name.length; i++) {
i
will equal name.length
which is an illegal index, since array indices are zero-based.
Your code should read
for(int i = 0; i < name.length; i++)
^
edited Oct 29 '15 at 3:05
Teepeemm
3,18442142
3,18442142
answered Apr 5 '11 at 16:15


aioobe
319k75683742
319k75683742
add a comment |
add a comment |
up vote
12
down vote
It means that you are trying to access an index of an array which is not valid as it is not in between the bounds.
For example this would initialize a primitive integer array with the upper bound 4.
int intArray = new int[5];
Programmers count from zero. So this for example would throw an ArrayIndexOutOfBoundsException
as the upper bound is 4 and not 5.
intArray[5];
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
add a comment |
up vote
12
down vote
It means that you are trying to access an index of an array which is not valid as it is not in between the bounds.
For example this would initialize a primitive integer array with the upper bound 4.
int intArray = new int[5];
Programmers count from zero. So this for example would throw an ArrayIndexOutOfBoundsException
as the upper bound is 4 and not 5.
intArray[5];
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
add a comment |
up vote
12
down vote
up vote
12
down vote
It means that you are trying to access an index of an array which is not valid as it is not in between the bounds.
For example this would initialize a primitive integer array with the upper bound 4.
int intArray = new int[5];
Programmers count from zero. So this for example would throw an ArrayIndexOutOfBoundsException
as the upper bound is 4 and not 5.
intArray[5];
It means that you are trying to access an index of an array which is not valid as it is not in between the bounds.
For example this would initialize a primitive integer array with the upper bound 4.
int intArray = new int[5];
Programmers count from zero. So this for example would throw an ArrayIndexOutOfBoundsException
as the upper bound is 4 and not 5.
intArray[5];
edited Apr 5 '11 at 16:01
answered Apr 5 '11 at 15:55


Octavian Damiean
35.3k188795
35.3k188795
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
add a comment |
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
what are the bounds?
– Aaron
Apr 5 '11 at 15:57
3
3
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
Bounds are the limits within range. i.e.; 0-5
– John Giotta
Apr 5 '11 at 15:59
add a comment |
up vote
7
down vote
To avoid an array index out-of-bounds exception, one should use the enhanced-for
statement where and when they can.
The primary motivation (and use case) is when you are iterating and you do not require any complicated iteration steps. You would not be able to use an enhanced-for
to move backwards in an array or only iterate on every other element.
You're guaranteed not to run out of elements to iterate over when doing this, and your [corrected] example is easily converted over.
The code below:
String name = {"tom", "dick", "harry"};
for(int i = 0; i< name.length; i++) {
System.out.print(name[i] + "n");
}
...is equivalent to this:
String name = {"tom", "dick", "harry"};
for(String firstName : name) {
System.out.println(firstName + "n");
}
add a comment |
up vote
7
down vote
To avoid an array index out-of-bounds exception, one should use the enhanced-for
statement where and when they can.
The primary motivation (and use case) is when you are iterating and you do not require any complicated iteration steps. You would not be able to use an enhanced-for
to move backwards in an array or only iterate on every other element.
You're guaranteed not to run out of elements to iterate over when doing this, and your [corrected] example is easily converted over.
The code below:
String name = {"tom", "dick", "harry"};
for(int i = 0; i< name.length; i++) {
System.out.print(name[i] + "n");
}
...is equivalent to this:
String name = {"tom", "dick", "harry"};
for(String firstName : name) {
System.out.println(firstName + "n");
}
add a comment |
up vote
7
down vote
up vote
7
down vote
To avoid an array index out-of-bounds exception, one should use the enhanced-for
statement where and when they can.
The primary motivation (and use case) is when you are iterating and you do not require any complicated iteration steps. You would not be able to use an enhanced-for
to move backwards in an array or only iterate on every other element.
You're guaranteed not to run out of elements to iterate over when doing this, and your [corrected] example is easily converted over.
The code below:
String name = {"tom", "dick", "harry"};
for(int i = 0; i< name.length; i++) {
System.out.print(name[i] + "n");
}
...is equivalent to this:
String name = {"tom", "dick", "harry"};
for(String firstName : name) {
System.out.println(firstName + "n");
}
To avoid an array index out-of-bounds exception, one should use the enhanced-for
statement where and when they can.
The primary motivation (and use case) is when you are iterating and you do not require any complicated iteration steps. You would not be able to use an enhanced-for
to move backwards in an array or only iterate on every other element.
You're guaranteed not to run out of elements to iterate over when doing this, and your [corrected] example is easily converted over.
The code below:
String name = {"tom", "dick", "harry"};
for(int i = 0; i< name.length; i++) {
System.out.print(name[i] + "n");
}
...is equivalent to this:
String name = {"tom", "dick", "harry"};
for(String firstName : name) {
System.out.println(firstName + "n");
}
edited Oct 19 '15 at 11:00
Lundin
105k16153257
105k16153257
answered Oct 16 '15 at 21:45
Makoto
79.3k15122164
79.3k15122164
add a comment |
add a comment |
up vote
7
down vote
What causes ArrayIndexOutOfBoundsException
?
If you think of a variable as a "box" where you can place a value, then an array is a series of boxes placed next to eachother, where the number of boxes is a finite and explicit integer.
Creating an array like this:
final int myArray = new int[5]
creates a row of 5 boxes, each holding an int
. Each of the boxes have an index, a position in the series of boxes. This index starts at 0, and ends at N-1, where N is the size of the array (the number of boxes).
To retrieve one of the values from this series of boxes, you can refer to it through its index, like this:
myArray[3]
Which will give you the value of the 4th box in the series (since the first box has index 0).
An ArrayIndexOutOfBoundsException
is caused by trying to retrive a "box" that does not exist, by passing an index that is higher than the index of last "box", or negative.
With my running example, these code snippets would produce such an exception:
myArray[5] //tries to retrieve the 6th "box" when there is only 5
myArray[-1] //just makes no sense
myArray[1337] //waay to high
How to avoid ArrayIndexOutOfBoundsException
In order to prevent ArrayIndexOutOfBoundsException
, there are some key points to consider:
Looping
When looping through an array, always make sure that the index you are retrieving is strictly smaller than the length of the array (the number of boxes). For instance:
for (int i = 0; i < myArray.length; i++) {
Notice the <
, never mix a =
in there..
You might want to be tempted to do something like this:
for (int i = 1; i <= myArray.length; i++) {
final int someint = myArray[i - 1]
Just don't. Stick to the one above (if you need to use the index) and it will save you a lot of pain.
Where possible, use foreach:
for (int value : myArray) {
This way you won't have to think about indexes at all.
When looping, whatever you do, NEVER change the value of the loop iterator (here: i
). The only place this should change value is to keep the loop going. Changing it otherwise is just risking an exception, and is in most cases not neccessary.
Retrieval/update
When retrieving an arbitrary element of the array, always check that it is a valid index against the length of the array:
public Integer getArrayElement(final int index) {
if (index < 0 || index >= myArray.length) {
return null; //although I would much prefer an actual exception being thrown when this happens.
}
return myArray[index];
}
add a comment |
up vote
7
down vote
What causes ArrayIndexOutOfBoundsException
?
If you think of a variable as a "box" where you can place a value, then an array is a series of boxes placed next to eachother, where the number of boxes is a finite and explicit integer.
Creating an array like this:
final int myArray = new int[5]
creates a row of 5 boxes, each holding an int
. Each of the boxes have an index, a position in the series of boxes. This index starts at 0, and ends at N-1, where N is the size of the array (the number of boxes).
To retrieve one of the values from this series of boxes, you can refer to it through its index, like this:
myArray[3]
Which will give you the value of the 4th box in the series (since the first box has index 0).
An ArrayIndexOutOfBoundsException
is caused by trying to retrive a "box" that does not exist, by passing an index that is higher than the index of last "box", or negative.
With my running example, these code snippets would produce such an exception:
myArray[5] //tries to retrieve the 6th "box" when there is only 5
myArray[-1] //just makes no sense
myArray[1337] //waay to high
How to avoid ArrayIndexOutOfBoundsException
In order to prevent ArrayIndexOutOfBoundsException
, there are some key points to consider:
Looping
When looping through an array, always make sure that the index you are retrieving is strictly smaller than the length of the array (the number of boxes). For instance:
for (int i = 0; i < myArray.length; i++) {
Notice the <
, never mix a =
in there..
You might want to be tempted to do something like this:
for (int i = 1; i <= myArray.length; i++) {
final int someint = myArray[i - 1]
Just don't. Stick to the one above (if you need to use the index) and it will save you a lot of pain.
Where possible, use foreach:
for (int value : myArray) {
This way you won't have to think about indexes at all.
When looping, whatever you do, NEVER change the value of the loop iterator (here: i
). The only place this should change value is to keep the loop going. Changing it otherwise is just risking an exception, and is in most cases not neccessary.
Retrieval/update
When retrieving an arbitrary element of the array, always check that it is a valid index against the length of the array:
public Integer getArrayElement(final int index) {
if (index < 0 || index >= myArray.length) {
return null; //although I would much prefer an actual exception being thrown when this happens.
}
return myArray[index];
}
add a comment |
up vote
7
down vote
up vote
7
down vote
What causes ArrayIndexOutOfBoundsException
?
If you think of a variable as a "box" where you can place a value, then an array is a series of boxes placed next to eachother, where the number of boxes is a finite and explicit integer.
Creating an array like this:
final int myArray = new int[5]
creates a row of 5 boxes, each holding an int
. Each of the boxes have an index, a position in the series of boxes. This index starts at 0, and ends at N-1, where N is the size of the array (the number of boxes).
To retrieve one of the values from this series of boxes, you can refer to it through its index, like this:
myArray[3]
Which will give you the value of the 4th box in the series (since the first box has index 0).
An ArrayIndexOutOfBoundsException
is caused by trying to retrive a "box" that does not exist, by passing an index that is higher than the index of last "box", or negative.
With my running example, these code snippets would produce such an exception:
myArray[5] //tries to retrieve the 6th "box" when there is only 5
myArray[-1] //just makes no sense
myArray[1337] //waay to high
How to avoid ArrayIndexOutOfBoundsException
In order to prevent ArrayIndexOutOfBoundsException
, there are some key points to consider:
Looping
When looping through an array, always make sure that the index you are retrieving is strictly smaller than the length of the array (the number of boxes). For instance:
for (int i = 0; i < myArray.length; i++) {
Notice the <
, never mix a =
in there..
You might want to be tempted to do something like this:
for (int i = 1; i <= myArray.length; i++) {
final int someint = myArray[i - 1]
Just don't. Stick to the one above (if you need to use the index) and it will save you a lot of pain.
Where possible, use foreach:
for (int value : myArray) {
This way you won't have to think about indexes at all.
When looping, whatever you do, NEVER change the value of the loop iterator (here: i
). The only place this should change value is to keep the loop going. Changing it otherwise is just risking an exception, and is in most cases not neccessary.
Retrieval/update
When retrieving an arbitrary element of the array, always check that it is a valid index against the length of the array:
public Integer getArrayElement(final int index) {
if (index < 0 || index >= myArray.length) {
return null; //although I would much prefer an actual exception being thrown when this happens.
}
return myArray[index];
}
What causes ArrayIndexOutOfBoundsException
?
If you think of a variable as a "box" where you can place a value, then an array is a series of boxes placed next to eachother, where the number of boxes is a finite and explicit integer.
Creating an array like this:
final int myArray = new int[5]
creates a row of 5 boxes, each holding an int
. Each of the boxes have an index, a position in the series of boxes. This index starts at 0, and ends at N-1, where N is the size of the array (the number of boxes).
To retrieve one of the values from this series of boxes, you can refer to it through its index, like this:
myArray[3]
Which will give you the value of the 4th box in the series (since the first box has index 0).
An ArrayIndexOutOfBoundsException
is caused by trying to retrive a "box" that does not exist, by passing an index that is higher than the index of last "box", or negative.
With my running example, these code snippets would produce such an exception:
myArray[5] //tries to retrieve the 6th "box" when there is only 5
myArray[-1] //just makes no sense
myArray[1337] //waay to high
How to avoid ArrayIndexOutOfBoundsException
In order to prevent ArrayIndexOutOfBoundsException
, there are some key points to consider:
Looping
When looping through an array, always make sure that the index you are retrieving is strictly smaller than the length of the array (the number of boxes). For instance:
for (int i = 0; i < myArray.length; i++) {
Notice the <
, never mix a =
in there..
You might want to be tempted to do something like this:
for (int i = 1; i <= myArray.length; i++) {
final int someint = myArray[i - 1]
Just don't. Stick to the one above (if you need to use the index) and it will save you a lot of pain.
Where possible, use foreach:
for (int value : myArray) {
This way you won't have to think about indexes at all.
When looping, whatever you do, NEVER change the value of the loop iterator (here: i
). The only place this should change value is to keep the loop going. Changing it otherwise is just risking an exception, and is in most cases not neccessary.
Retrieval/update
When retrieving an arbitrary element of the array, always check that it is a valid index against the length of the array:
public Integer getArrayElement(final int index) {
if (index < 0 || index >= myArray.length) {
return null; //although I would much prefer an actual exception being thrown when this happens.
}
return myArray[index];
}
edited Feb 3 '17 at 13:20
answered Jan 19 '16 at 16:55
Tobb
7,56123751
7,56123751
add a comment |
add a comment |
up vote
4
down vote
In your code you have accessed the elements from index 0 to the length of the string array. name.length
gives the number of string objects in your array of string objects i.e. 3, but you can access only up to index 2 name[2]
,
because the array can be accessed from index 0 to name.length - 1
where you get name.length
number of objects.
Even while using a for
loop you have started with index zero and you should end with name.length - 1
. In an array a[n] you can access form a[0] to a[n-1].
For example:
String a={"str1", "str2", str3" ..., "strn"};
for(int i=0;i<a.length()i++)
System.out.println(a[i]);
In your case:
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
4
down vote
In your code you have accessed the elements from index 0 to the length of the string array. name.length
gives the number of string objects in your array of string objects i.e. 3, but you can access only up to index 2 name[2]
,
because the array can be accessed from index 0 to name.length - 1
where you get name.length
number of objects.
Even while using a for
loop you have started with index zero and you should end with name.length - 1
. In an array a[n] you can access form a[0] to a[n-1].
For example:
String a={"str1", "str2", str3" ..., "strn"};
for(int i=0;i<a.length()i++)
System.out.println(a[i]);
In your case:
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
4
down vote
up vote
4
down vote
In your code you have accessed the elements from index 0 to the length of the string array. name.length
gives the number of string objects in your array of string objects i.e. 3, but you can access only up to index 2 name[2]
,
because the array can be accessed from index 0 to name.length - 1
where you get name.length
number of objects.
Even while using a for
loop you have started with index zero and you should end with name.length - 1
. In an array a[n] you can access form a[0] to a[n-1].
For example:
String a={"str1", "str2", str3" ..., "strn"};
for(int i=0;i<a.length()i++)
System.out.println(a[i]);
In your case:
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
In your code you have accessed the elements from index 0 to the length of the string array. name.length
gives the number of string objects in your array of string objects i.e. 3, but you can access only up to index 2 name[2]
,
because the array can be accessed from index 0 to name.length - 1
where you get name.length
number of objects.
Even while using a for
loop you have started with index zero and you should end with name.length - 1
. In an array a[n] you can access form a[0] to a[n-1].
For example:
String a={"str1", "str2", str3" ..., "strn"};
for(int i=0;i<a.length()i++)
System.out.println(a[i]);
In your case:
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
edited Dec 17 '17 at 10:03


Peter Mortensen
13.3k1983111
13.3k1983111
answered Jun 21 '17 at 4:57
Madhusudan chowdary
18912
18912
add a comment |
add a comment |
up vote
3
down vote
For your given array the length of the array is 3(i.e. name.length = 3). But as it stores element starting from index 0, it has max index 2.
So, instead of 'i**<=name.length' you should write 'i<**name.length' to avoid 'ArrayIndexOutOfBoundsException'.
add a comment |
up vote
3
down vote
For your given array the length of the array is 3(i.e. name.length = 3). But as it stores element starting from index 0, it has max index 2.
So, instead of 'i**<=name.length' you should write 'i<**name.length' to avoid 'ArrayIndexOutOfBoundsException'.
add a comment |
up vote
3
down vote
up vote
3
down vote
For your given array the length of the array is 3(i.e. name.length = 3). But as it stores element starting from index 0, it has max index 2.
So, instead of 'i**<=name.length' you should write 'i<**name.length' to avoid 'ArrayIndexOutOfBoundsException'.
For your given array the length of the array is 3(i.e. name.length = 3). But as it stores element starting from index 0, it has max index 2.
So, instead of 'i**<=name.length' you should write 'i<**name.length' to avoid 'ArrayIndexOutOfBoundsException'.
answered Jan 16 at 12:13
nIKHIL
586
586
add a comment |
add a comment |
up vote
2
down vote
So much for this simple question, but I just wanted to highlight a new feature in Java which will avoid all confusions around indexing in arrays even for beginners. Java-8 has abstracted the task of iterating for you.
int array = new int[5];
//If you need just the items
Arrays.stream(array).forEach(item -> { println(item); });
//If you need the index as well
IntStream.range(0, array.length).forEach(index -> { println(array[index]); })
What's the benefit? Well, one thing is the readability like English. Second, you need not worry about the ArrayIndexOutOfBoundsException
add a comment |
up vote
2
down vote
So much for this simple question, but I just wanted to highlight a new feature in Java which will avoid all confusions around indexing in arrays even for beginners. Java-8 has abstracted the task of iterating for you.
int array = new int[5];
//If you need just the items
Arrays.stream(array).forEach(item -> { println(item); });
//If you need the index as well
IntStream.range(0, array.length).forEach(index -> { println(array[index]); })
What's the benefit? Well, one thing is the readability like English. Second, you need not worry about the ArrayIndexOutOfBoundsException
add a comment |
up vote
2
down vote
up vote
2
down vote
So much for this simple question, but I just wanted to highlight a new feature in Java which will avoid all confusions around indexing in arrays even for beginners. Java-8 has abstracted the task of iterating for you.
int array = new int[5];
//If you need just the items
Arrays.stream(array).forEach(item -> { println(item); });
//If you need the index as well
IntStream.range(0, array.length).forEach(index -> { println(array[index]); })
What's the benefit? Well, one thing is the readability like English. Second, you need not worry about the ArrayIndexOutOfBoundsException
So much for this simple question, but I just wanted to highlight a new feature in Java which will avoid all confusions around indexing in arrays even for beginners. Java-8 has abstracted the task of iterating for you.
int array = new int[5];
//If you need just the items
Arrays.stream(array).forEach(item -> { println(item); });
//If you need the index as well
IntStream.range(0, array.length).forEach(index -> { println(array[index]); })
What's the benefit? Well, one thing is the readability like English. Second, you need not worry about the ArrayIndexOutOfBoundsException
answered Oct 25 '17 at 7:38


Satyendra Kumar
15719
15719
add a comment |
add a comment |
up vote
2
down vote
You are getting ArrayIndexOutOfBoundsException
due to i<=name.length
part. name.length
return the length of the string name
, which is 3. Hence when you try to access name[3]
, it's illegal and throws an exception.
Resolved code:
String name = {"tom", "dick", "harry"};
for(int i = 0; i < name.length; i++) { //use < insteadof <=
System.out.print(name[i] +'n');
}
It's defined in the Java language specification:
The
public final
fieldlength
, which contains the number of components
of the array.length
may be positive or zero.
add a comment |
up vote
2
down vote
You are getting ArrayIndexOutOfBoundsException
due to i<=name.length
part. name.length
return the length of the string name
, which is 3. Hence when you try to access name[3]
, it's illegal and throws an exception.
Resolved code:
String name = {"tom", "dick", "harry"};
for(int i = 0; i < name.length; i++) { //use < insteadof <=
System.out.print(name[i] +'n');
}
It's defined in the Java language specification:
The
public final
fieldlength
, which contains the number of components
of the array.length
may be positive or zero.
add a comment |
up vote
2
down vote
up vote
2
down vote
You are getting ArrayIndexOutOfBoundsException
due to i<=name.length
part. name.length
return the length of the string name
, which is 3. Hence when you try to access name[3]
, it's illegal and throws an exception.
Resolved code:
String name = {"tom", "dick", "harry"};
for(int i = 0; i < name.length; i++) { //use < insteadof <=
System.out.print(name[i] +'n');
}
It's defined in the Java language specification:
The
public final
fieldlength
, which contains the number of components
of the array.length
may be positive or zero.
You are getting ArrayIndexOutOfBoundsException
due to i<=name.length
part. name.length
return the length of the string name
, which is 3. Hence when you try to access name[3]
, it's illegal and throws an exception.
Resolved code:
String name = {"tom", "dick", "harry"};
for(int i = 0; i < name.length; i++) { //use < insteadof <=
System.out.print(name[i] +'n');
}
It's defined in the Java language specification:
The
public final
fieldlength
, which contains the number of components
of the array.length
may be positive or zero.
edited Dec 17 '17 at 10:00


Peter Mortensen
13.3k1983111
13.3k1983111
answered Mar 6 '17 at 9:00


roottraveller
3,71423340
3,71423340
add a comment |
add a comment |
up vote
2
down vote
That's how this type of exception looks when thrown in Eclipse. The number in red signifies the index you tried to access. So the code would look like this:
myArray[5]
The error is thrown when you try to access an index which doesn't exist in that array. If an array has a length of 3,
int intArray = new int[3];
then the only valid indexes are:
intArray[0]
intArray[1]
intArray[2]
If an array has a length of 1,
int intArray = new int[1];
then the only valid index is:
intArray[0]
Any integer equal to the length of the array, or bigger than it: is out of bounds.
Any integer less than 0: is out of bounds;
P.S.: If you look to have a better understanding of arrays and do some practical exercises, there's a video here: tutorial on arrays in Java
add a comment |
up vote
2
down vote
That's how this type of exception looks when thrown in Eclipse. The number in red signifies the index you tried to access. So the code would look like this:
myArray[5]
The error is thrown when you try to access an index which doesn't exist in that array. If an array has a length of 3,
int intArray = new int[3];
then the only valid indexes are:
intArray[0]
intArray[1]
intArray[2]
If an array has a length of 1,
int intArray = new int[1];
then the only valid index is:
intArray[0]
Any integer equal to the length of the array, or bigger than it: is out of bounds.
Any integer less than 0: is out of bounds;
P.S.: If you look to have a better understanding of arrays and do some practical exercises, there's a video here: tutorial on arrays in Java
add a comment |
up vote
2
down vote
up vote
2
down vote
That's how this type of exception looks when thrown in Eclipse. The number in red signifies the index you tried to access. So the code would look like this:
myArray[5]
The error is thrown when you try to access an index which doesn't exist in that array. If an array has a length of 3,
int intArray = new int[3];
then the only valid indexes are:
intArray[0]
intArray[1]
intArray[2]
If an array has a length of 1,
int intArray = new int[1];
then the only valid index is:
intArray[0]
Any integer equal to the length of the array, or bigger than it: is out of bounds.
Any integer less than 0: is out of bounds;
P.S.: If you look to have a better understanding of arrays and do some practical exercises, there's a video here: tutorial on arrays in Java
That's how this type of exception looks when thrown in Eclipse. The number in red signifies the index you tried to access. So the code would look like this:
myArray[5]
The error is thrown when you try to access an index which doesn't exist in that array. If an array has a length of 3,
int intArray = new int[3];
then the only valid indexes are:
intArray[0]
intArray[1]
intArray[2]
If an array has a length of 1,
int intArray = new int[1];
then the only valid index is:
intArray[0]
Any integer equal to the length of the array, or bigger than it: is out of bounds.
Any integer less than 0: is out of bounds;
P.S.: If you look to have a better understanding of arrays and do some practical exercises, there's a video here: tutorial on arrays in Java
edited Dec 17 '17 at 10:02


Peter Mortensen
13.3k1983111
13.3k1983111
answered Mar 8 '17 at 12:56


Ion
30549
30549
add a comment |
add a comment |
up vote
1
down vote
For multidimensional arrays, it can be tricky to make sure you access the length
property of the right dimension. Take the following code for example:
int a = new int [2][3][4];
for(int i = 0; i < a.length; i++){
for(int j = 0; j < a[i].length; j++){
for(int k = 0; k < a[j].length; k++){
System.out.print(a[i][j][k]);
}
System.out.println();
}
System.out.println();
}
Each dimension has a different length, so the subtle bug is that the middle and inner loops use the length
property of the same dimension (because a[i].length
is the same as a[j].length
).
Instead, the inner loop should use a[i][j].length
(or a[0][0].length
, for simplicity).
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
add a comment |
up vote
1
down vote
For multidimensional arrays, it can be tricky to make sure you access the length
property of the right dimension. Take the following code for example:
int a = new int [2][3][4];
for(int i = 0; i < a.length; i++){
for(int j = 0; j < a[i].length; j++){
for(int k = 0; k < a[j].length; k++){
System.out.print(a[i][j][k]);
}
System.out.println();
}
System.out.println();
}
Each dimension has a different length, so the subtle bug is that the middle and inner loops use the length
property of the same dimension (because a[i].length
is the same as a[j].length
).
Instead, the inner loop should use a[i][j].length
(or a[0][0].length
, for simplicity).
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
add a comment |
up vote
1
down vote
up vote
1
down vote
For multidimensional arrays, it can be tricky to make sure you access the length
property of the right dimension. Take the following code for example:
int a = new int [2][3][4];
for(int i = 0; i < a.length; i++){
for(int j = 0; j < a[i].length; j++){
for(int k = 0; k < a[j].length; k++){
System.out.print(a[i][j][k]);
}
System.out.println();
}
System.out.println();
}
Each dimension has a different length, so the subtle bug is that the middle and inner loops use the length
property of the same dimension (because a[i].length
is the same as a[j].length
).
Instead, the inner loop should use a[i][j].length
(or a[0][0].length
, for simplicity).
For multidimensional arrays, it can be tricky to make sure you access the length
property of the right dimension. Take the following code for example:
int a = new int [2][3][4];
for(int i = 0; i < a.length; i++){
for(int j = 0; j < a[i].length; j++){
for(int k = 0; k < a[j].length; k++){
System.out.print(a[i][j][k]);
}
System.out.println();
}
System.out.println();
}
Each dimension has a different length, so the subtle bug is that the middle and inner loops use the length
property of the same dimension (because a[i].length
is the same as a[j].length
).
Instead, the inner loop should use a[i][j].length
(or a[0][0].length
, for simplicity).
answered Jun 12 at 19:41
Bill the Lizard
287k156490786
287k156490786
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
add a comment |
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
Added a code example from Why does my for loop not work when i change the condition(multidimensional arrays)? since this question gets used as a dupe target for any question dealing with ArrayIndexOutOfBoundsException.
– Bill the Lizard
Jun 12 at 19:44
add a comment |
up vote
1
down vote
ArrayIndexOutOfBoundsException whenever this exception is coming it mean you are trying to use an index of array which is out of its bounds or in lay man terms you are requesting more than than you have initialised.
To prevent this always make sure that you are not requesting a index which is not present in array i.e. if array length is 10 then your index must range between 0 to 9
add a comment |
up vote
1
down vote
ArrayIndexOutOfBoundsException whenever this exception is coming it mean you are trying to use an index of array which is out of its bounds or in lay man terms you are requesting more than than you have initialised.
To prevent this always make sure that you are not requesting a index which is not present in array i.e. if array length is 10 then your index must range between 0 to 9
add a comment |
up vote
1
down vote
up vote
1
down vote
ArrayIndexOutOfBoundsException whenever this exception is coming it mean you are trying to use an index of array which is out of its bounds or in lay man terms you are requesting more than than you have initialised.
To prevent this always make sure that you are not requesting a index which is not present in array i.e. if array length is 10 then your index must range between 0 to 9
ArrayIndexOutOfBoundsException whenever this exception is coming it mean you are trying to use an index of array which is out of its bounds or in lay man terms you are requesting more than than you have initialised.
To prevent this always make sure that you are not requesting a index which is not present in array i.e. if array length is 10 then your index must range between 0 to 9
answered Sep 15 at 10:29


sgrpwr
1067
1067
add a comment |
add a comment |
up vote
0
down vote
ArrayIndexOutOfBoundsException
means that you are trying to access an index of the array that does not exist or out of the bound of this array. Array indexes start from 0 and end at length - 1.
In your case
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length, Not correct
}
ArrayIndexOutOfBoundsException
happens when you are trying to access
the name.length indexed element which does not exist (array index ends at length -1). just replacing <= with < would solve this problem.
for(int i = 0; i < name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length - 1, Correct
}
add a comment |
up vote
0
down vote
ArrayIndexOutOfBoundsException
means that you are trying to access an index of the array that does not exist or out of the bound of this array. Array indexes start from 0 and end at length - 1.
In your case
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length, Not correct
}
ArrayIndexOutOfBoundsException
happens when you are trying to access
the name.length indexed element which does not exist (array index ends at length -1). just replacing <= with < would solve this problem.
for(int i = 0; i < name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length - 1, Correct
}
add a comment |
up vote
0
down vote
up vote
0
down vote
ArrayIndexOutOfBoundsException
means that you are trying to access an index of the array that does not exist or out of the bound of this array. Array indexes start from 0 and end at length - 1.
In your case
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length, Not correct
}
ArrayIndexOutOfBoundsException
happens when you are trying to access
the name.length indexed element which does not exist (array index ends at length -1). just replacing <= with < would solve this problem.
for(int i = 0; i < name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length - 1, Correct
}
ArrayIndexOutOfBoundsException
means that you are trying to access an index of the array that does not exist or out of the bound of this array. Array indexes start from 0 and end at length - 1.
In your case
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length, Not correct
}
ArrayIndexOutOfBoundsException
happens when you are trying to access
the name.length indexed element which does not exist (array index ends at length -1). just replacing <= with < would solve this problem.
for(int i = 0; i < name.length; i++) {
System.out.print(name[i] +'n'); // i goes from 0 to length - 1, Correct
}
answered Nov 30 '17 at 16:41
adn.911
5481521
5481521
add a comment |
add a comment |
up vote
0
down vote
The most common case I've seen for seemingly mysterious ArrayIndexOutOfBoundsExceptions, i.e. apparently not caused by your own array handling code, is the concurrent use of SimpleDateFormat. Particularly in a servlet or controller:
public class MyController {
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
public void handleRequest(ServletRequest req, ServletResponse res) {
Date date = dateFormat.parse(req.getParameter("date"));
}
}
If two threads enter the SimplateDateFormat.parse() method together you will likely see an ArrayIndexOutOfBoundsException. Note the synchronization section of the class javadoc for SimpleDateFormat.
Make sure there is no place in your code that are accessing thread unsafe classes like SimpleDateFormat in a concurrent manner like in a servlet or controller. Check all instance variables of your servlets and controllers for likely suspects.
add a comment |
up vote
0
down vote
The most common case I've seen for seemingly mysterious ArrayIndexOutOfBoundsExceptions, i.e. apparently not caused by your own array handling code, is the concurrent use of SimpleDateFormat. Particularly in a servlet or controller:
public class MyController {
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
public void handleRequest(ServletRequest req, ServletResponse res) {
Date date = dateFormat.parse(req.getParameter("date"));
}
}
If two threads enter the SimplateDateFormat.parse() method together you will likely see an ArrayIndexOutOfBoundsException. Note the synchronization section of the class javadoc for SimpleDateFormat.
Make sure there is no place in your code that are accessing thread unsafe classes like SimpleDateFormat in a concurrent manner like in a servlet or controller. Check all instance variables of your servlets and controllers for likely suspects.
add a comment |
up vote
0
down vote
up vote
0
down vote
The most common case I've seen for seemingly mysterious ArrayIndexOutOfBoundsExceptions, i.e. apparently not caused by your own array handling code, is the concurrent use of SimpleDateFormat. Particularly in a servlet or controller:
public class MyController {
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
public void handleRequest(ServletRequest req, ServletResponse res) {
Date date = dateFormat.parse(req.getParameter("date"));
}
}
If two threads enter the SimplateDateFormat.parse() method together you will likely see an ArrayIndexOutOfBoundsException. Note the synchronization section of the class javadoc for SimpleDateFormat.
Make sure there is no place in your code that are accessing thread unsafe classes like SimpleDateFormat in a concurrent manner like in a servlet or controller. Check all instance variables of your servlets and controllers for likely suspects.
The most common case I've seen for seemingly mysterious ArrayIndexOutOfBoundsExceptions, i.e. apparently not caused by your own array handling code, is the concurrent use of SimpleDateFormat. Particularly in a servlet or controller:
public class MyController {
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
public void handleRequest(ServletRequest req, ServletResponse res) {
Date date = dateFormat.parse(req.getParameter("date"));
}
}
If two threads enter the SimplateDateFormat.parse() method together you will likely see an ArrayIndexOutOfBoundsException. Note the synchronization section of the class javadoc for SimpleDateFormat.
Make sure there is no place in your code that are accessing thread unsafe classes like SimpleDateFormat in a concurrent manner like in a servlet or controller. Check all instance variables of your servlets and controllers for likely suspects.
edited Dec 17 '17 at 9:54


Peter Mortensen
13.3k1983111
13.3k1983111
answered Apr 5 '11 at 16:06
Kyri Sarantakos
818713
818713
add a comment |
add a comment |
up vote
0
down vote
For any array of length n, elements of the array will have an index from 0 to n-1.
If your program is trying to access any element (or memory) having array index greater than n-1, then Java will throw ArrayIndexOutOfBoundsException
So here are two solutions that we can use in a program
Maintaining count:
for(int count = 0; count < array.length; count++) {
System.out.println(array[count]);
}
Or some other looping statement like
int count = 0;
while(count < array.length) {
System.out.println(array[count]);
count++;
}
A better way go with a for each loop, in this method a programmer has no need to bother about the number of elements in the array.
for(String str : array) {
System.out.println(str);
}
add a comment |
up vote
0
down vote
For any array of length n, elements of the array will have an index from 0 to n-1.
If your program is trying to access any element (or memory) having array index greater than n-1, then Java will throw ArrayIndexOutOfBoundsException
So here are two solutions that we can use in a program
Maintaining count:
for(int count = 0; count < array.length; count++) {
System.out.println(array[count]);
}
Or some other looping statement like
int count = 0;
while(count < array.length) {
System.out.println(array[count]);
count++;
}
A better way go with a for each loop, in this method a programmer has no need to bother about the number of elements in the array.
for(String str : array) {
System.out.println(str);
}
add a comment |
up vote
0
down vote
up vote
0
down vote
For any array of length n, elements of the array will have an index from 0 to n-1.
If your program is trying to access any element (or memory) having array index greater than n-1, then Java will throw ArrayIndexOutOfBoundsException
So here are two solutions that we can use in a program
Maintaining count:
for(int count = 0; count < array.length; count++) {
System.out.println(array[count]);
}
Or some other looping statement like
int count = 0;
while(count < array.length) {
System.out.println(array[count]);
count++;
}
A better way go with a for each loop, in this method a programmer has no need to bother about the number of elements in the array.
for(String str : array) {
System.out.println(str);
}
For any array of length n, elements of the array will have an index from 0 to n-1.
If your program is trying to access any element (or memory) having array index greater than n-1, then Java will throw ArrayIndexOutOfBoundsException
So here are two solutions that we can use in a program
Maintaining count:
for(int count = 0; count < array.length; count++) {
System.out.println(array[count]);
}
Or some other looping statement like
int count = 0;
while(count < array.length) {
System.out.println(array[count]);
count++;
}
A better way go with a for each loop, in this method a programmer has no need to bother about the number of elements in the array.
for(String str : array) {
System.out.println(str);
}
edited Dec 17 '17 at 9:58


Peter Mortensen
13.3k1983111
13.3k1983111
answered Nov 11 '16 at 8:21
Mohit
54756
54756
add a comment |
add a comment |
up vote
0
down vote
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
IndexOutOfBoundsException is thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Any array X, can be accessed from [0 to (X.length - 1)]
add a comment |
up vote
0
down vote
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
IndexOutOfBoundsException is thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Any array X, can be accessed from [0 to (X.length - 1)]
add a comment |
up vote
0
down vote
up vote
0
down vote
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
IndexOutOfBoundsException is thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Any array X, can be accessed from [0 to (X.length - 1)]
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
IndexOutOfBoundsException is thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Any array X, can be accessed from [0 to (X.length - 1)]
answered Oct 18 at 9:24
ZiadM
1114
1114
add a comment |
add a comment |
up vote
0
down vote
I see all the answers here explaining how to work with arrays and how to avoid the index out of bounds exceptions. I personally avoid arrays at all costs. I use the Collections classes, which avoids all the silliness of having to deal with array indices entirely. The looping constructs work beautifully with collections supporting code that is both easier to write, understand and maintain.
add a comment |
up vote
0
down vote
I see all the answers here explaining how to work with arrays and how to avoid the index out of bounds exceptions. I personally avoid arrays at all costs. I use the Collections classes, which avoids all the silliness of having to deal with array indices entirely. The looping constructs work beautifully with collections supporting code that is both easier to write, understand and maintain.
add a comment |
up vote
0
down vote
up vote
0
down vote
I see all the answers here explaining how to work with arrays and how to avoid the index out of bounds exceptions. I personally avoid arrays at all costs. I use the Collections classes, which avoids all the silliness of having to deal with array indices entirely. The looping constructs work beautifully with collections supporting code that is both easier to write, understand and maintain.
I see all the answers here explaining how to work with arrays and how to avoid the index out of bounds exceptions. I personally avoid arrays at all costs. I use the Collections classes, which avoids all the silliness of having to deal with array indices entirely. The looping constructs work beautifully with collections supporting code that is both easier to write, understand and maintain.
answered Nov 13 at 18:08
John Czukkermann
198111
198111
add a comment |
add a comment |
up vote
-1
down vote
According to your Code :
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
If You check
System.out.print(name.length);
you will get 3;
that mean your name length is 3
your loop is running from 0 to 3
which should be running either "0 to 2" or "1 to 3"
Answer
String name = {"tom", "dick", "harry"};
for(int i = 0; i<name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
-1
down vote
According to your Code :
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
If You check
System.out.print(name.length);
you will get 3;
that mean your name length is 3
your loop is running from 0 to 3
which should be running either "0 to 2" or "1 to 3"
Answer
String name = {"tom", "dick", "harry"};
for(int i = 0; i<name.length; i++) {
System.out.print(name[i] +'n');
}
add a comment |
up vote
-1
down vote
up vote
-1
down vote
According to your Code :
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
If You check
System.out.print(name.length);
you will get 3;
that mean your name length is 3
your loop is running from 0 to 3
which should be running either "0 to 2" or "1 to 3"
Answer
String name = {"tom", "dick", "harry"};
for(int i = 0; i<name.length; i++) {
System.out.print(name[i] +'n');
}
According to your Code :
String name = {"tom", "dick", "harry"};
for(int i = 0; i<=name.length; i++) {
System.out.print(name[i] +'n');
}
If You check
System.out.print(name.length);
you will get 3;
that mean your name length is 3
your loop is running from 0 to 3
which should be running either "0 to 2" or "1 to 3"
Answer
String name = {"tom", "dick", "harry"};
for(int i = 0; i<name.length; i++) {
System.out.print(name[i] +'n');
}
edited Mar 26 at 10:16
answered Mar 26 at 8:08


Abhishek Agarwal
294
294
add a comment |
add a comment |
up vote
-1
down vote
ArrayIndexOutOfBoundsException
name itself explains that If you trying to access the value at the index which is out of the scope of Array size then such kind of exception occur.
In your case, You can just remove equal sign from your for loop.
for(int i = 0; i<name.length; i++)
The better option is to iterate an array:
for(String i : name )
System.out.println(i);
add a comment |
up vote
-1
down vote
ArrayIndexOutOfBoundsException
name itself explains that If you trying to access the value at the index which is out of the scope of Array size then such kind of exception occur.
In your case, You can just remove equal sign from your for loop.
for(int i = 0; i<name.length; i++)
The better option is to iterate an array:
for(String i : name )
System.out.println(i);
add a comment |
up vote
-1
down vote
up vote
-1
down vote
ArrayIndexOutOfBoundsException
name itself explains that If you trying to access the value at the index which is out of the scope of Array size then such kind of exception occur.
In your case, You can just remove equal sign from your for loop.
for(int i = 0; i<name.length; i++)
The better option is to iterate an array:
for(String i : name )
System.out.println(i);
ArrayIndexOutOfBoundsException
name itself explains that If you trying to access the value at the index which is out of the scope of Array size then such kind of exception occur.
In your case, You can just remove equal sign from your for loop.
for(int i = 0; i<name.length; i++)
The better option is to iterate an array:
for(String i : name )
System.out.println(i);
edited May 17 at 3:46


Stephen Rauch
27.3k153156
27.3k153156
answered May 17 at 3:27


Madhusudan Sharma
191
191
add a comment |
add a comment |
up vote
-1
down vote
This error is occurs at runs loop overlimit times.Let's consider simple example like this,
class demo{
public static void main(String a){
int numberArray={4,8,2,3,89,5};
int i;
for(i=0;i<numberArray.length;i++){
System.out.print(numberArray[i+1]+" ");
}
}
At first, I have initialized an array as 'numberArray'. then , some array elements are printed using for loop. When loop is running 'i' time , print the (numberArray[i+1] element..(when i value is 1, numberArray[i+1] element is printed.)..Suppose that, when i=(numberArray.length-2), last element of array is printed..When 'i' value goes to (numberArray.length-1) , no value for printing..In that point , 'ArrayIndexOutOfBoundsException' is occur.I hope to you could get idea.thank you !
add a comment |
up vote
-1
down vote
This error is occurs at runs loop overlimit times.Let's consider simple example like this,
class demo{
public static void main(String a){
int numberArray={4,8,2,3,89,5};
int i;
for(i=0;i<numberArray.length;i++){
System.out.print(numberArray[i+1]+" ");
}
}
At first, I have initialized an array as 'numberArray'. then , some array elements are printed using for loop. When loop is running 'i' time , print the (numberArray[i+1] element..(when i value is 1, numberArray[i+1] element is printed.)..Suppose that, when i=(numberArray.length-2), last element of array is printed..When 'i' value goes to (numberArray.length-1) , no value for printing..In that point , 'ArrayIndexOutOfBoundsException' is occur.I hope to you could get idea.thank you !
add a comment |
up vote
-1
down vote
up vote
-1
down vote
This error is occurs at runs loop overlimit times.Let's consider simple example like this,
class demo{
public static void main(String a){
int numberArray={4,8,2,3,89,5};
int i;
for(i=0;i<numberArray.length;i++){
System.out.print(numberArray[i+1]+" ");
}
}
At first, I have initialized an array as 'numberArray'. then , some array elements are printed using for loop. When loop is running 'i' time , print the (numberArray[i+1] element..(when i value is 1, numberArray[i+1] element is printed.)..Suppose that, when i=(numberArray.length-2), last element of array is printed..When 'i' value goes to (numberArray.length-1) , no value for printing..In that point , 'ArrayIndexOutOfBoundsException' is occur.I hope to you could get idea.thank you !
This error is occurs at runs loop overlimit times.Let's consider simple example like this,
class demo{
public static void main(String a){
int numberArray={4,8,2,3,89,5};
int i;
for(i=0;i<numberArray.length;i++){
System.out.print(numberArray[i+1]+" ");
}
}
At first, I have initialized an array as 'numberArray'. then , some array elements are printed using for loop. When loop is running 'i' time , print the (numberArray[i+1] element..(when i value is 1, numberArray[i+1] element is printed.)..Suppose that, when i=(numberArray.length-2), last element of array is printed..When 'i' value goes to (numberArray.length-1) , no value for printing..In that point , 'ArrayIndexOutOfBoundsException' is occur.I hope to you could get idea.thank you !
answered Jul 17 at 3:03


GT_hash
415
415
add a comment |
add a comment |
up vote
-3
down vote
You could not iterate or store more data than the length of your array. In this case you could do like this:
for (int i = 0; i <= name.length - 1; i++) {
// ....
}
Or this:
for (int i = 0; i < name.length; i++) {
// ...
}
add a comment |
up vote
-3
down vote
You could not iterate or store more data than the length of your array. In this case you could do like this:
for (int i = 0; i <= name.length - 1; i++) {
// ....
}
Or this:
for (int i = 0; i < name.length; i++) {
// ...
}
add a comment |
up vote
-3
down vote
up vote
-3
down vote
You could not iterate or store more data than the length of your array. In this case you could do like this:
for (int i = 0; i <= name.length - 1; i++) {
// ....
}
Or this:
for (int i = 0; i < name.length; i++) {
// ...
}
You could not iterate or store more data than the length of your array. In this case you could do like this:
for (int i = 0; i <= name.length - 1; i++) {
// ....
}
Or this:
for (int i = 0; i < name.length; i++) {
// ...
}
edited Dec 17 '17 at 9:55


Peter Mortensen
13.3k1983111
13.3k1983111
answered Jan 19 '16 at 16:26
Kevin7
1301110
1301110
add a comment |
add a comment |
protected by Makoto Oct 16 '15 at 21:34
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
95,rRXo,WMtt0y,p HbHCaTjG95Ic,Ky8Hrd8coGOqWdohpPImD76NqKVZCf7qjJNob8fiwu30
In reference to the last question, code would be helpful. Are you accessing the array with a known index, or do you have to start debugging to figure out how the index is calculated when the error occurs?
– justkt
Apr 5 '11 at 15:57
31
Replace
i <= name.length
withi < name.length
- or better, write an enhanced for loop. (for (String aName : name) { ... }
)– Jean Hominal
Apr 5 '11 at 16:14
it means, that you want to get element of array that not exist, 'i<=name.length' means that you want to get element length+1 - its not exist.
– gbk
Feb 22 '13 at 17:50
en.wikipedia.org/wiki/Off-by-one_error
– sakhunzai
Apr 18 '17 at 5:05