String concatenation ambiguity
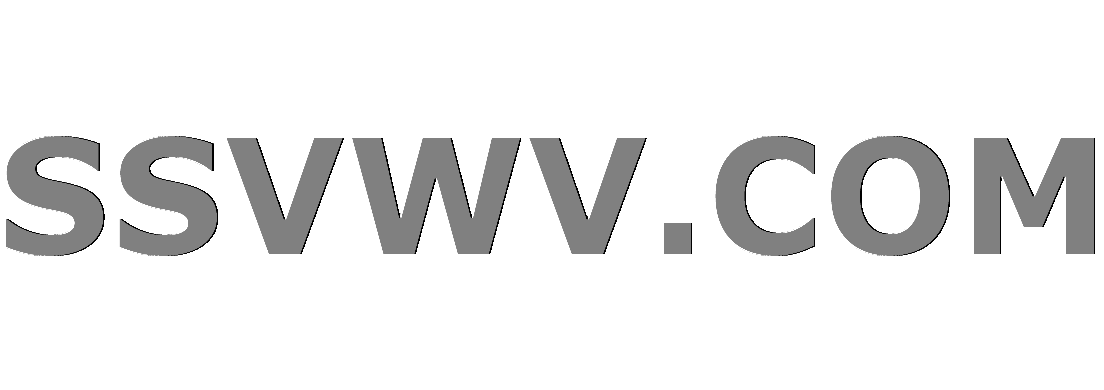
Multi tool use
up vote
0
down vote
favorite
I have the following simple piece of java code where I am trying to understand how string concatenation in java works using '+' operator.
public class Problem {
public static void main(String... args){
String str1 = "abc";
String str2 = "ab";
String str3 = "c";
String str4 = "ab" + "c";//This will use of StringBuilder class for concatenation and return new String object
String str5 = str2 + str3;//This will use of StringBuilder class for concatenation and return new String object
System.out.println(str1 == str4); // This returns true
System.out.println(str1 == str5); // This returns false
}
}
str4
is the resultant of 2 string literals (ab
and c
) and str5
is of references to the 2 string literals (str2
and str3
). In both the cases, java will be calling StringBuilder class to perform the concatenation.
And I believe it should result in creating 2 different StringBuilder objects in java heap space.
If my understanding is correct, why str1 == str4
returns true ? Can some one please help in getting this clear to me ?
Regards,
Maneesh Sharma
string concatenation string-literals
add a comment |
up vote
0
down vote
favorite
I have the following simple piece of java code where I am trying to understand how string concatenation in java works using '+' operator.
public class Problem {
public static void main(String... args){
String str1 = "abc";
String str2 = "ab";
String str3 = "c";
String str4 = "ab" + "c";//This will use of StringBuilder class for concatenation and return new String object
String str5 = str2 + str3;//This will use of StringBuilder class for concatenation and return new String object
System.out.println(str1 == str4); // This returns true
System.out.println(str1 == str5); // This returns false
}
}
str4
is the resultant of 2 string literals (ab
and c
) and str5
is of references to the 2 string literals (str2
and str3
). In both the cases, java will be calling StringBuilder class to perform the concatenation.
And I believe it should result in creating 2 different StringBuilder objects in java heap space.
If my understanding is correct, why str1 == str4
returns true ? Can some one please help in getting this clear to me ?
Regards,
Maneesh Sharma
string concatenation string-literals
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following simple piece of java code where I am trying to understand how string concatenation in java works using '+' operator.
public class Problem {
public static void main(String... args){
String str1 = "abc";
String str2 = "ab";
String str3 = "c";
String str4 = "ab" + "c";//This will use of StringBuilder class for concatenation and return new String object
String str5 = str2 + str3;//This will use of StringBuilder class for concatenation and return new String object
System.out.println(str1 == str4); // This returns true
System.out.println(str1 == str5); // This returns false
}
}
str4
is the resultant of 2 string literals (ab
and c
) and str5
is of references to the 2 string literals (str2
and str3
). In both the cases, java will be calling StringBuilder class to perform the concatenation.
And I believe it should result in creating 2 different StringBuilder objects in java heap space.
If my understanding is correct, why str1 == str4
returns true ? Can some one please help in getting this clear to me ?
Regards,
Maneesh Sharma
string concatenation string-literals
I have the following simple piece of java code where I am trying to understand how string concatenation in java works using '+' operator.
public class Problem {
public static void main(String... args){
String str1 = "abc";
String str2 = "ab";
String str3 = "c";
String str4 = "ab" + "c";//This will use of StringBuilder class for concatenation and return new String object
String str5 = str2 + str3;//This will use of StringBuilder class for concatenation and return new String object
System.out.println(str1 == str4); // This returns true
System.out.println(str1 == str5); // This returns false
}
}
str4
is the resultant of 2 string literals (ab
and c
) and str5
is of references to the 2 string literals (str2
and str3
). In both the cases, java will be calling StringBuilder class to perform the concatenation.
And I believe it should result in creating 2 different StringBuilder objects in java heap space.
If my understanding is correct, why str1 == str4
returns true ? Can some one please help in getting this clear to me ?
Regards,
Maneesh Sharma
string concatenation string-literals
string concatenation string-literals
edited Nov 11 at 8:34
marc_s
566k12610921245
566k12610921245
asked Nov 11 at 3:12
Maneesh
448
448
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37
add a comment |
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
It depends on how the compiler and jvm will optimize this. Only if both variables are optimized to point to the same adress, the evaluation will be true. In general, using == to compare strings is not reliable. Use the 'equals' method instead.
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would befalse
forstr1 == str4
but is not the case :) !
– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It depends on how the compiler and jvm will optimize this. Only if both variables are optimized to point to the same adress, the evaluation will be true. In general, using == to compare strings is not reliable. Use the 'equals' method instead.
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would befalse
forstr1 == str4
but is not the case :) !
– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
add a comment |
up vote
0
down vote
It depends on how the compiler and jvm will optimize this. Only if both variables are optimized to point to the same adress, the evaluation will be true. In general, using == to compare strings is not reliable. Use the 'equals' method instead.
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would befalse
forstr1 == str4
but is not the case :) !
– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
add a comment |
up vote
0
down vote
up vote
0
down vote
It depends on how the compiler and jvm will optimize this. Only if both variables are optimized to point to the same adress, the evaluation will be true. In general, using == to compare strings is not reliable. Use the 'equals' method instead.
It depends on how the compiler and jvm will optimize this. Only if both variables are optimized to point to the same adress, the evaluation will be true. In general, using == to compare strings is not reliable. Use the 'equals' method instead.
answered Nov 11 at 3:20


Pascal Ludwig
6311614
6311614
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would befalse
forstr1 == str4
but is not the case :) !
– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
add a comment |
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would befalse
forstr1 == str4
but is not the case :) !
– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
So the output of the above program may differ for different JVMs, is that correct ?
– Maneesh
Nov 11 at 11:32
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
I'm not 100% sure about the specification for this. But in practice, yes. The address of new variables should be treated as nondeterministic.
– Pascal Ludwig
Nov 11 at 12:30
1
1
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
One more note: The jvm may optimize immutable objects (especially strings) by pointing their variables to the same address. But you don't have control over that. Also, I imagine searching the occupied address space for an equal object is expensive. I don't know in which cases it could do these optimizations.
– Pascal Ludwig
Nov 11 at 12:32
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would be
false
for str1 == str4
but is not the case :) !– Maneesh
Nov 11 at 13:25
Thank you Pascal for the additional info ! I am still wondering if I am given the above problem as an objective question, what my guess should be ? If I go by the explanations so far I came across how string concatenation works, my answer would be
false
for str1 == str4
but is not the case :) !– Maneesh
Nov 11 at 13:25
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
While browsing I found an article in stackoverflow where one of the comments mentioned the following.. if you simply write "ab" + "c", Java compiler will perform concatenation at compile time and the generated code will be exactly the same. This only works if all strings are known at compile time. If any of the strings is a variable and cann't be determined by compiler, it will have a different resultant hash code
– Maneesh
Nov 12 at 8:36
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245535%2fstring-concatenation-ambiguity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yqyL,lblq IlFj,4YiL4fhKzlrxhTAE3ULCyYz0i ZCzznYL,BeCX2G7 dAJGkx5DkSL4k 5k,5eUrUuNne
Possible duplicate of What is the difference between “text” and new String(“text”)?.
– Tim Biegeleisen
Nov 11 at 3:37