How iterate condition checking in python?
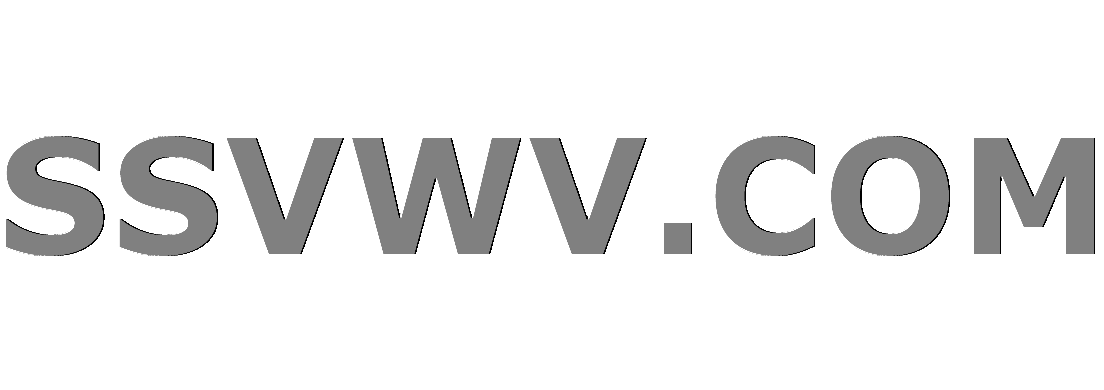
Multi tool use
up vote
1
down vote
favorite
The following function gives the correct result for me. But for a large number of q's, it very difficult to handle this program. So, I would like to iterate q's via a loop or some other manner. How can I do this?
def sgf(a): # here a is a list of two numbers
import random
a2=random.randint(1,1068)
p=1069
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
while ((q1>1060) or (q2>1060) or (q3>1060) or (q4>1060) or (q5>1060) or (q6>1060) or (q7>1060) or (q8>1060) or (q9>1060)):
a2=random.randint(1,1068)
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
if ((q1<=1060) and (q2<=1060) and (q3<=1060) and (q4<=1060) and (q5<=1060) and (q6<=1060) and (q7<=1060) and (q8<=1060) and (q9<=1060)):
break
return q1,q2,q3,q4,q5,q6,q7,q8,q9
For simplicity,
f(x)=(a0+a1*x+a2*x**2)%p
where a0,a1 are in [0,1060] and a2 chosen randomly from [0,1068]
if all f(x)<=1060
, accept f(x) otherwise regenerate f(x)
python python-2.7 loops iteration
add a comment |
up vote
1
down vote
favorite
The following function gives the correct result for me. But for a large number of q's, it very difficult to handle this program. So, I would like to iterate q's via a loop or some other manner. How can I do this?
def sgf(a): # here a is a list of two numbers
import random
a2=random.randint(1,1068)
p=1069
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
while ((q1>1060) or (q2>1060) or (q3>1060) or (q4>1060) or (q5>1060) or (q6>1060) or (q7>1060) or (q8>1060) or (q9>1060)):
a2=random.randint(1,1068)
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
if ((q1<=1060) and (q2<=1060) and (q3<=1060) and (q4<=1060) and (q5<=1060) and (q6<=1060) and (q7<=1060) and (q8<=1060) and (q9<=1060)):
break
return q1,q2,q3,q4,q5,q6,q7,q8,q9
For simplicity,
f(x)=(a0+a1*x+a2*x**2)%p
where a0,a1 are in [0,1060] and a2 chosen randomly from [0,1068]
if all f(x)<=1060
, accept f(x) otherwise regenerate f(x)
python python-2.7 loops iteration
1
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
Try something likewhile any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
The following function gives the correct result for me. But for a large number of q's, it very difficult to handle this program. So, I would like to iterate q's via a loop or some other manner. How can I do this?
def sgf(a): # here a is a list of two numbers
import random
a2=random.randint(1,1068)
p=1069
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
while ((q1>1060) or (q2>1060) or (q3>1060) or (q4>1060) or (q5>1060) or (q6>1060) or (q7>1060) or (q8>1060) or (q9>1060)):
a2=random.randint(1,1068)
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
if ((q1<=1060) and (q2<=1060) and (q3<=1060) and (q4<=1060) and (q5<=1060) and (q6<=1060) and (q7<=1060) and (q8<=1060) and (q9<=1060)):
break
return q1,q2,q3,q4,q5,q6,q7,q8,q9
For simplicity,
f(x)=(a0+a1*x+a2*x**2)%p
where a0,a1 are in [0,1060] and a2 chosen randomly from [0,1068]
if all f(x)<=1060
, accept f(x) otherwise regenerate f(x)
python python-2.7 loops iteration
The following function gives the correct result for me. But for a large number of q's, it very difficult to handle this program. So, I would like to iterate q's via a loop or some other manner. How can I do this?
def sgf(a): # here a is a list of two numbers
import random
a2=random.randint(1,1068)
p=1069
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
while ((q1>1060) or (q2>1060) or (q3>1060) or (q4>1060) or (q5>1060) or (q6>1060) or (q7>1060) or (q8>1060) or (q9>1060)):
a2=random.randint(1,1068)
q1=(a[0]+a[1]*1+a2*1**2)%p
q2=(a[0]+a[1]*2+a2*2**2)%p
q3=(a[0]+a[1]*3+a2*3**2)%p
q4=(a[0]+a[1]*4+a2*4**2)%p
q5=(a[0]+a[1]*5+a2*5**2)%p
q6=(a[0]+a[1]*6+a2*6**2)%p
q7=(a[0]+a[1]*7+a2*7**2)%p
q8=(a[0]+a[1]*8+a2*8**2)%p
q9=(a[0]+a[1]*9+a2*9**2)%p
if ((q1<=1060) and (q2<=1060) and (q3<=1060) and (q4<=1060) and (q5<=1060) and (q6<=1060) and (q7<=1060) and (q8<=1060) and (q9<=1060)):
break
return q1,q2,q3,q4,q5,q6,q7,q8,q9
For simplicity,
f(x)=(a0+a1*x+a2*x**2)%p
where a0,a1 are in [0,1060] and a2 chosen randomly from [0,1068]
if all f(x)<=1060
, accept f(x) otherwise regenerate f(x)
python python-2.7 loops iteration
python python-2.7 loops iteration
edited Nov 11 at 3:28
asked Nov 11 at 3:18
H.S.
326
326
1
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
Try something likewhile any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27
add a comment |
1
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
Try something likewhile any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27
1
1
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
Try something like
while any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27
Try something like
while any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
It looks like the only variables in your generation of q
s are the integers that you multiple a[1]
and a2
by.
You could alter your function to accept the total number of q
s as a second parameter (e.g. total_q
), then iterate over range(1, total_q+1)
and append each new q
to a Python list, returning the list at the end.
Try something like:
def sgf(a, total_q):
import random
a2=random.randint(1,1068)
p=1069
q_list =
for i in range(0,total_q+1):
q = (a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
while any(q > 1060 for q in q_list):
a2=random.randint(1,1068)
q_list =
for i in range(0,total_q+1):
q =(a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
if all(q <= 1060 for q in q_list):
break
return q_list
add a comment |
up vote
3
down vote
Your q
should probably be a list. That way you can use a for
loop with range
to perform your calculation:
for ind in range(len(q)):
q[ind]=(a[0]+a[1]*(ind + 1)+a2*(ind + 1)**2)%p
You can also represent your condition as an iteration, but the simplest way would probably be to use the any
function and write your condition as a generator:
while any(qx > 1060 for qx in q):
add a comment |
up vote
1
down vote
def sgf(a):
import random
a2=random.randint(1,1068)
p=1069
items =
# if items is empty, or any element is greater than 1060, keep looping
while not items or any(item > 1060 for item in items):
items = [(a[0]+a[1]*i+a2*i**2)%p for i in range(1,10)]
return items
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
It looks like the only variables in your generation of q
s are the integers that you multiple a[1]
and a2
by.
You could alter your function to accept the total number of q
s as a second parameter (e.g. total_q
), then iterate over range(1, total_q+1)
and append each new q
to a Python list, returning the list at the end.
Try something like:
def sgf(a, total_q):
import random
a2=random.randint(1,1068)
p=1069
q_list =
for i in range(0,total_q+1):
q = (a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
while any(q > 1060 for q in q_list):
a2=random.randint(1,1068)
q_list =
for i in range(0,total_q+1):
q =(a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
if all(q <= 1060 for q in q_list):
break
return q_list
add a comment |
up vote
1
down vote
accepted
It looks like the only variables in your generation of q
s are the integers that you multiple a[1]
and a2
by.
You could alter your function to accept the total number of q
s as a second parameter (e.g. total_q
), then iterate over range(1, total_q+1)
and append each new q
to a Python list, returning the list at the end.
Try something like:
def sgf(a, total_q):
import random
a2=random.randint(1,1068)
p=1069
q_list =
for i in range(0,total_q+1):
q = (a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
while any(q > 1060 for q in q_list):
a2=random.randint(1,1068)
q_list =
for i in range(0,total_q+1):
q =(a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
if all(q <= 1060 for q in q_list):
break
return q_list
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
It looks like the only variables in your generation of q
s are the integers that you multiple a[1]
and a2
by.
You could alter your function to accept the total number of q
s as a second parameter (e.g. total_q
), then iterate over range(1, total_q+1)
and append each new q
to a Python list, returning the list at the end.
Try something like:
def sgf(a, total_q):
import random
a2=random.randint(1,1068)
p=1069
q_list =
for i in range(0,total_q+1):
q = (a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
while any(q > 1060 for q in q_list):
a2=random.randint(1,1068)
q_list =
for i in range(0,total_q+1):
q =(a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
if all(q <= 1060 for q in q_list):
break
return q_list
It looks like the only variables in your generation of q
s are the integers that you multiple a[1]
and a2
by.
You could alter your function to accept the total number of q
s as a second parameter (e.g. total_q
), then iterate over range(1, total_q+1)
and append each new q
to a Python list, returning the list at the end.
Try something like:
def sgf(a, total_q):
import random
a2=random.randint(1,1068)
p=1069
q_list =
for i in range(0,total_q+1):
q = (a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
while any(q > 1060 for q in q_list):
a2=random.randint(1,1068)
q_list =
for i in range(0,total_q+1):
q =(a[0]+a[1]*i+a2*i**2)%p
q_list.append(q)
if all(q <= 1060 for q in q_list):
break
return q_list
answered Nov 11 at 3:41
dmitriys
1469
1469
add a comment |
add a comment |
up vote
3
down vote
Your q
should probably be a list. That way you can use a for
loop with range
to perform your calculation:
for ind in range(len(q)):
q[ind]=(a[0]+a[1]*(ind + 1)+a2*(ind + 1)**2)%p
You can also represent your condition as an iteration, but the simplest way would probably be to use the any
function and write your condition as a generator:
while any(qx > 1060 for qx in q):
add a comment |
up vote
3
down vote
Your q
should probably be a list. That way you can use a for
loop with range
to perform your calculation:
for ind in range(len(q)):
q[ind]=(a[0]+a[1]*(ind + 1)+a2*(ind + 1)**2)%p
You can also represent your condition as an iteration, but the simplest way would probably be to use the any
function and write your condition as a generator:
while any(qx > 1060 for qx in q):
add a comment |
up vote
3
down vote
up vote
3
down vote
Your q
should probably be a list. That way you can use a for
loop with range
to perform your calculation:
for ind in range(len(q)):
q[ind]=(a[0]+a[1]*(ind + 1)+a2*(ind + 1)**2)%p
You can also represent your condition as an iteration, but the simplest way would probably be to use the any
function and write your condition as a generator:
while any(qx > 1060 for qx in q):
Your q
should probably be a list. That way you can use a for
loop with range
to perform your calculation:
for ind in range(len(q)):
q[ind]=(a[0]+a[1]*(ind + 1)+a2*(ind + 1)**2)%p
You can also represent your condition as an iteration, but the simplest way would probably be to use the any
function and write your condition as a generator:
while any(qx > 1060 for qx in q):
answered Nov 11 at 3:27
Score_Under
822614
822614
add a comment |
add a comment |
up vote
1
down vote
def sgf(a):
import random
a2=random.randint(1,1068)
p=1069
items =
# if items is empty, or any element is greater than 1060, keep looping
while not items or any(item > 1060 for item in items):
items = [(a[0]+a[1]*i+a2*i**2)%p for i in range(1,10)]
return items
add a comment |
up vote
1
down vote
def sgf(a):
import random
a2=random.randint(1,1068)
p=1069
items =
# if items is empty, or any element is greater than 1060, keep looping
while not items or any(item > 1060 for item in items):
items = [(a[0]+a[1]*i+a2*i**2)%p for i in range(1,10)]
return items
add a comment |
up vote
1
down vote
up vote
1
down vote
def sgf(a):
import random
a2=random.randint(1,1068)
p=1069
items =
# if items is empty, or any element is greater than 1060, keep looping
while not items or any(item > 1060 for item in items):
items = [(a[0]+a[1]*i+a2*i**2)%p for i in range(1,10)]
return items
def sgf(a):
import random
a2=random.randint(1,1068)
p=1069
items =
# if items is empty, or any element is greater than 1060, keep looping
while not items or any(item > 1060 for item in items):
items = [(a[0]+a[1]*i+a2*i**2)%p for i in range(1,10)]
return items
answered Nov 11 at 3:30
John Gordon
8,97051727
8,97051727
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245559%2fhow-iterate-condition-checking-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4sQzIHXihEcT,5,1 fKY1nk5,cud
1
You should learn how to use lists and loops... In fact, for this particular problem, you should use NumPy.
– DYZ
Nov 11 at 3:23
Try something like
while any(a[0] + a[1] * 1 + a2 * i ** 2) % p > 1060 for i in range(1, 10))
– Klaus D.
Nov 11 at 3:27