Defining a function that gets the highest product pairs in a list
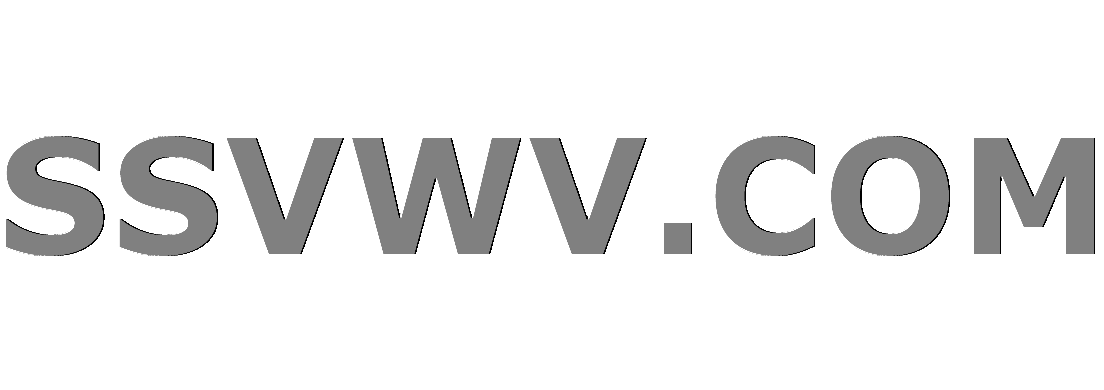
Multi tool use
up vote
1
down vote
favorite
I'm trying to find write a function that gives the product of the highest pair of adjacent elements in a list. For my code,
gala = [1, 2, 3, 4, 5]
def adjacentElementsProduct(inputArray):
for i in range(len(inputArray)):
if inputArray[i] * inputArray[i+1] > inputArray[i+1] * inputArray[i+2]:
return inputArray[i] * inputArray[i+1]
elif inputArray[i+1] * inputArray[i+2] > inputArray[i] * inputArray[i+1] and inputArray[i+1] * inputArray[i+2] > inputArray[i+2] * inputArray[i+3]:
return inputArray[i+1] * inputArray[i+2]
elif inputArray[i+2] * inputArray[i+3] > inputArray[i+1] * inputArray[i+2] and inputArray[i+2] * inputArray[i+3] > inputArray[i+3] * inputArray[i+4]:
return inputArray[i+2] * inputArray[i+3]
else:
return inputArray[i+3] * inputArray[i+4]
return adjacentElementsProduct
adjacentElementsProduct(gala)
Here the output would be 20 (since 4 x 5 is the highest adjacent pairs). This function works for the given list even if I change the order of the numbers and their sign. However, if the length of the list is changed then the code breaks. If the list was
gala = [1, -6]
or
gala = [2, 5, 7, -9, 10, 0, 11]
I wanted the function first list's output to would be -6 and second would be 35. But my function breaks for such lists.
python list func
add a comment |
up vote
1
down vote
favorite
I'm trying to find write a function that gives the product of the highest pair of adjacent elements in a list. For my code,
gala = [1, 2, 3, 4, 5]
def adjacentElementsProduct(inputArray):
for i in range(len(inputArray)):
if inputArray[i] * inputArray[i+1] > inputArray[i+1] * inputArray[i+2]:
return inputArray[i] * inputArray[i+1]
elif inputArray[i+1] * inputArray[i+2] > inputArray[i] * inputArray[i+1] and inputArray[i+1] * inputArray[i+2] > inputArray[i+2] * inputArray[i+3]:
return inputArray[i+1] * inputArray[i+2]
elif inputArray[i+2] * inputArray[i+3] > inputArray[i+1] * inputArray[i+2] and inputArray[i+2] * inputArray[i+3] > inputArray[i+3] * inputArray[i+4]:
return inputArray[i+2] * inputArray[i+3]
else:
return inputArray[i+3] * inputArray[i+4]
return adjacentElementsProduct
adjacentElementsProduct(gala)
Here the output would be 20 (since 4 x 5 is the highest adjacent pairs). This function works for the given list even if I change the order of the numbers and their sign. However, if the length of the list is changed then the code breaks. If the list was
gala = [1, -6]
or
gala = [2, 5, 7, -9, 10, 0, 11]
I wanted the function first list's output to would be -6 and second would be 35. But my function breaks for such lists.
python list func
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to find write a function that gives the product of the highest pair of adjacent elements in a list. For my code,
gala = [1, 2, 3, 4, 5]
def adjacentElementsProduct(inputArray):
for i in range(len(inputArray)):
if inputArray[i] * inputArray[i+1] > inputArray[i+1] * inputArray[i+2]:
return inputArray[i] * inputArray[i+1]
elif inputArray[i+1] * inputArray[i+2] > inputArray[i] * inputArray[i+1] and inputArray[i+1] * inputArray[i+2] > inputArray[i+2] * inputArray[i+3]:
return inputArray[i+1] * inputArray[i+2]
elif inputArray[i+2] * inputArray[i+3] > inputArray[i+1] * inputArray[i+2] and inputArray[i+2] * inputArray[i+3] > inputArray[i+3] * inputArray[i+4]:
return inputArray[i+2] * inputArray[i+3]
else:
return inputArray[i+3] * inputArray[i+4]
return adjacentElementsProduct
adjacentElementsProduct(gala)
Here the output would be 20 (since 4 x 5 is the highest adjacent pairs). This function works for the given list even if I change the order of the numbers and their sign. However, if the length of the list is changed then the code breaks. If the list was
gala = [1, -6]
or
gala = [2, 5, 7, -9, 10, 0, 11]
I wanted the function first list's output to would be -6 and second would be 35. But my function breaks for such lists.
python list func
I'm trying to find write a function that gives the product of the highest pair of adjacent elements in a list. For my code,
gala = [1, 2, 3, 4, 5]
def adjacentElementsProduct(inputArray):
for i in range(len(inputArray)):
if inputArray[i] * inputArray[i+1] > inputArray[i+1] * inputArray[i+2]:
return inputArray[i] * inputArray[i+1]
elif inputArray[i+1] * inputArray[i+2] > inputArray[i] * inputArray[i+1] and inputArray[i+1] * inputArray[i+2] > inputArray[i+2] * inputArray[i+3]:
return inputArray[i+1] * inputArray[i+2]
elif inputArray[i+2] * inputArray[i+3] > inputArray[i+1] * inputArray[i+2] and inputArray[i+2] * inputArray[i+3] > inputArray[i+3] * inputArray[i+4]:
return inputArray[i+2] * inputArray[i+3]
else:
return inputArray[i+3] * inputArray[i+4]
return adjacentElementsProduct
adjacentElementsProduct(gala)
Here the output would be 20 (since 4 x 5 is the highest adjacent pairs). This function works for the given list even if I change the order of the numbers and their sign. However, if the length of the list is changed then the code breaks. If the list was
gala = [1, -6]
or
gala = [2, 5, 7, -9, 10, 0, 11]
I wanted the function first list's output to would be -6 and second would be 35. But my function breaks for such lists.
python list func
python list func
asked Nov 11 at 0:38
user1821176
3141419
3141419
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
If i did correctly understood your issue, i think your function can be reduced to:
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(k*v for k, v in zip(elm, elm[1:]))
So:
>>> adjacentElementsProduct([1, 2, 3, 4, 5])
20
>>> adjacentElementsProduct([1, -6])
-6
>>> adjacentElementsProduct([2, 5, 7, -9, 10, 0, 11])
35
add a comment |
up vote
1
down vote
A modification of @ChihebNexus's approach:
from operator import mul
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(map(mul, elm, elm[1:]))
An even shorter version:
def adjacentElementsProduct(elm):
return max(map(mul, elm, elm[1:])) if len(elm) < 2 else None
And another one:
from operator import mul
from itertools import starmap
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(starmap(mul, zip(elm, elm[1:])))
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
If i did correctly understood your issue, i think your function can be reduced to:
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(k*v for k, v in zip(elm, elm[1:]))
So:
>>> adjacentElementsProduct([1, 2, 3, 4, 5])
20
>>> adjacentElementsProduct([1, -6])
-6
>>> adjacentElementsProduct([2, 5, 7, -9, 10, 0, 11])
35
add a comment |
up vote
3
down vote
accepted
If i did correctly understood your issue, i think your function can be reduced to:
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(k*v for k, v in zip(elm, elm[1:]))
So:
>>> adjacentElementsProduct([1, 2, 3, 4, 5])
20
>>> adjacentElementsProduct([1, -6])
-6
>>> adjacentElementsProduct([2, 5, 7, -9, 10, 0, 11])
35
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
If i did correctly understood your issue, i think your function can be reduced to:
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(k*v for k, v in zip(elm, elm[1:]))
So:
>>> adjacentElementsProduct([1, 2, 3, 4, 5])
20
>>> adjacentElementsProduct([1, -6])
-6
>>> adjacentElementsProduct([2, 5, 7, -9, 10, 0, 11])
35
If i did correctly understood your issue, i think your function can be reduced to:
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(k*v for k, v in zip(elm, elm[1:]))
So:
>>> adjacentElementsProduct([1, 2, 3, 4, 5])
20
>>> adjacentElementsProduct([1, -6])
-6
>>> adjacentElementsProduct([2, 5, 7, -9, 10, 0, 11])
35
answered Nov 11 at 1:00


Chiheb Nexus
4,55531527
4,55531527
add a comment |
add a comment |
up vote
1
down vote
A modification of @ChihebNexus's approach:
from operator import mul
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(map(mul, elm, elm[1:]))
An even shorter version:
def adjacentElementsProduct(elm):
return max(map(mul, elm, elm[1:])) if len(elm) < 2 else None
And another one:
from operator import mul
from itertools import starmap
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(starmap(mul, zip(elm, elm[1:])))
add a comment |
up vote
1
down vote
A modification of @ChihebNexus's approach:
from operator import mul
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(map(mul, elm, elm[1:]))
An even shorter version:
def adjacentElementsProduct(elm):
return max(map(mul, elm, elm[1:])) if len(elm) < 2 else None
And another one:
from operator import mul
from itertools import starmap
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(starmap(mul, zip(elm, elm[1:])))
add a comment |
up vote
1
down vote
up vote
1
down vote
A modification of @ChihebNexus's approach:
from operator import mul
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(map(mul, elm, elm[1:]))
An even shorter version:
def adjacentElementsProduct(elm):
return max(map(mul, elm, elm[1:])) if len(elm) < 2 else None
And another one:
from operator import mul
from itertools import starmap
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(starmap(mul, zip(elm, elm[1:])))
A modification of @ChihebNexus's approach:
from operator import mul
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(map(mul, elm, elm[1:]))
An even shorter version:
def adjacentElementsProduct(elm):
return max(map(mul, elm, elm[1:])) if len(elm) < 2 else None
And another one:
from operator import mul
from itertools import starmap
def adjacentElementsProduct(elm):
if len(elm) < 2:
return None
return max(starmap(mul, zip(elm, elm[1:])))
edited Nov 11 at 3:01
answered Nov 11 at 2:32
DYZ
24k61948
24k61948
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244798%2fdefining-a-function-that-gets-the-highest-product-pairs-in-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fEmBTrWQ,oA4WgEJdc3l9729k99U1NLNuIfW411BZ5F2,GFBUp6bjesyAJxS0y bIu,YUXcJeo